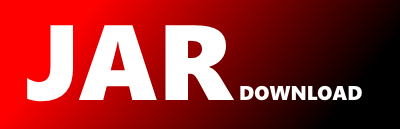
com.google.api.services.run.v2.model.GoogleCloudRunV2Execution Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.run.v2.model;
/**
* Execution represents the configuration of a single execution. A execution an immutable resource
* that references a container image which is run to completion.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Run Admin API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudRunV2Execution extends com.google.api.client.json.GenericJson {
/**
* Output only. Unstructured key value map that may be set by external tools to store and
* arbitrary metadata. They are not queryable and should be preserved when modifying objects.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map annotations;
/**
* Output only. The number of tasks which reached phase Cancelled.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer cancelledCount;
/**
* Output only. Represents time when the execution was completed. It is not guaranteed to be set
* in happens-before order across separate operations.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String completionTime;
/**
* Output only. The Condition of this Execution, containing its readiness status, and detailed
* error information in case it did not reach the desired state.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List conditions;
static {
// hack to force ProGuard to consider GoogleCloudRunV2Condition used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleCloudRunV2Condition.class);
}
/**
* Output only. Represents time when the execution was acknowledged by the execution controller.
* It is not guaranteed to be set in happens-before order across separate operations.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String createTime;
/**
* Output only. For a deleted resource, the deletion time. It is only populated as a response to a
* Delete request.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String deleteTime;
/**
* Output only. A system-generated fingerprint for this version of the resource. May be used to
* detect modification conflict during updates.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String etag;
/**
* Output only. For a deleted resource, the time after which it will be permamently deleted. It is
* only populated as a response to a Delete request.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String expireTime;
/**
* Output only. The number of tasks which reached phase Failed.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer failedCount;
/**
* Output only. A number that monotonically increases every time the user modifies the desired
* state.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long generation;
/**
* Output only. The name of the parent Job.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String job;
/**
* Output only. Unstructured key value map that can be used to organize and categorize objects.
* User-provided labels are shared with Google's billing system, so they can be used to filter, or
* break down billing charges by team, component, environment, state, etc. For more information,
* visit https://cloud.google.com/resource-manager/docs/creating-managing-labels or
* https://cloud.google.com/run/docs/configuring/labels
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map labels;
/**
* The least stable launch stage needed to create this resource, as defined by [Google Cloud
* Platform Launch Stages](https://cloud.google.com/terms/launch-stages). Cloud Run supports
* `ALPHA`, `BETA`, and `GA`. Note that this value might not be what was used as input. For
* example, if ALPHA was provided as input in the parent resource, but only BETA and GA-level
* features are were, this field will be BETA.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String launchStage;
/**
* Output only. URI where logs for this execution can be found in Cloud Console.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String logUri;
/**
* Output only. The unique name of this Execution.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Output only. The generation of this Execution. See comments in `reconciling` for additional
* information on reconciliation process in Cloud Run.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long observedGeneration;
/**
* Output only. Specifies the maximum desired number of tasks the execution should run at any
* given time. Must be <= task_count. The actual number of tasks running in steady state will be
* less than this number when ((.spec.task_count - .status.successful) < .spec.parallelism), i.e.
* when the work left to do is less than max parallelism.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer parallelism;
/**
* Output only. Indicates whether the resource's reconciliation is still in progress. See comments
* in `Job.reconciling` for additional information on reconciliation process in Cloud Run.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean reconciling;
/**
* Output only. The number of tasks which have retried at least once.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer retriedCount;
/**
* Output only. The number of actively running tasks.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer runningCount;
/**
* Output only. Reserved for future use.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean satisfiesPzs;
/**
* Output only. Represents time when the execution started to run. It is not guaranteed to be set
* in happens-before order across separate operations.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String startTime;
/**
* Output only. The number of tasks which reached phase Succeeded.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer succeededCount;
/**
* Output only. Specifies the desired number of tasks the execution should run. Setting to 1 means
* that parallelism is limited to 1 and the success of that task signals the success of the
* execution.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer taskCount;
/**
* Output only. The template used to create tasks for this execution.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRunV2TaskTemplate template;
/**
* Output only. Server assigned unique identifier for the Execution. The value is a UUID4 string
* and guaranteed to remain unchanged until the resource is deleted.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String uid;
/**
* Output only. The last-modified time.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String updateTime;
/**
* Output only. Unstructured key value map that may be set by external tools to store and
* arbitrary metadata. They are not queryable and should be preserved when modifying objects.
* @return value or {@code null} for none
*/
public java.util.Map getAnnotations() {
return annotations;
}
/**
* Output only. Unstructured key value map that may be set by external tools to store and
* arbitrary metadata. They are not queryable and should be preserved when modifying objects.
* @param annotations annotations or {@code null} for none
*/
public GoogleCloudRunV2Execution setAnnotations(java.util.Map annotations) {
this.annotations = annotations;
return this;
}
/**
* Output only. The number of tasks which reached phase Cancelled.
* @return value or {@code null} for none
*/
public java.lang.Integer getCancelledCount() {
return cancelledCount;
}
/**
* Output only. The number of tasks which reached phase Cancelled.
* @param cancelledCount cancelledCount or {@code null} for none
*/
public GoogleCloudRunV2Execution setCancelledCount(java.lang.Integer cancelledCount) {
this.cancelledCount = cancelledCount;
return this;
}
/**
* Output only. Represents time when the execution was completed. It is not guaranteed to be set
* in happens-before order across separate operations.
* @return value or {@code null} for none
*/
public String getCompletionTime() {
return completionTime;
}
/**
* Output only. Represents time when the execution was completed. It is not guaranteed to be set
* in happens-before order across separate operations.
* @param completionTime completionTime or {@code null} for none
*/
public GoogleCloudRunV2Execution setCompletionTime(String completionTime) {
this.completionTime = completionTime;
return this;
}
/**
* Output only. The Condition of this Execution, containing its readiness status, and detailed
* error information in case it did not reach the desired state.
* @return value or {@code null} for none
*/
public java.util.List getConditions() {
return conditions;
}
/**
* Output only. The Condition of this Execution, containing its readiness status, and detailed
* error information in case it did not reach the desired state.
* @param conditions conditions or {@code null} for none
*/
public GoogleCloudRunV2Execution setConditions(java.util.List conditions) {
this.conditions = conditions;
return this;
}
/**
* Output only. Represents time when the execution was acknowledged by the execution controller.
* It is not guaranteed to be set in happens-before order across separate operations.
* @return value or {@code null} for none
*/
public String getCreateTime() {
return createTime;
}
/**
* Output only. Represents time when the execution was acknowledged by the execution controller.
* It is not guaranteed to be set in happens-before order across separate operations.
* @param createTime createTime or {@code null} for none
*/
public GoogleCloudRunV2Execution setCreateTime(String createTime) {
this.createTime = createTime;
return this;
}
/**
* Output only. For a deleted resource, the deletion time. It is only populated as a response to a
* Delete request.
* @return value or {@code null} for none
*/
public String getDeleteTime() {
return deleteTime;
}
/**
* Output only. For a deleted resource, the deletion time. It is only populated as a response to a
* Delete request.
* @param deleteTime deleteTime or {@code null} for none
*/
public GoogleCloudRunV2Execution setDeleteTime(String deleteTime) {
this.deleteTime = deleteTime;
return this;
}
/**
* Output only. A system-generated fingerprint for this version of the resource. May be used to
* detect modification conflict during updates.
* @return value or {@code null} for none
*/
public java.lang.String getEtag() {
return etag;
}
/**
* Output only. A system-generated fingerprint for this version of the resource. May be used to
* detect modification conflict during updates.
* @param etag etag or {@code null} for none
*/
public GoogleCloudRunV2Execution setEtag(java.lang.String etag) {
this.etag = etag;
return this;
}
/**
* Output only. For a deleted resource, the time after which it will be permamently deleted. It is
* only populated as a response to a Delete request.
* @return value or {@code null} for none
*/
public String getExpireTime() {
return expireTime;
}
/**
* Output only. For a deleted resource, the time after which it will be permamently deleted. It is
* only populated as a response to a Delete request.
* @param expireTime expireTime or {@code null} for none
*/
public GoogleCloudRunV2Execution setExpireTime(String expireTime) {
this.expireTime = expireTime;
return this;
}
/**
* Output only. The number of tasks which reached phase Failed.
* @return value or {@code null} for none
*/
public java.lang.Integer getFailedCount() {
return failedCount;
}
/**
* Output only. The number of tasks which reached phase Failed.
* @param failedCount failedCount or {@code null} for none
*/
public GoogleCloudRunV2Execution setFailedCount(java.lang.Integer failedCount) {
this.failedCount = failedCount;
return this;
}
/**
* Output only. A number that monotonically increases every time the user modifies the desired
* state.
* @return value or {@code null} for none
*/
public java.lang.Long getGeneration() {
return generation;
}
/**
* Output only. A number that monotonically increases every time the user modifies the desired
* state.
* @param generation generation or {@code null} for none
*/
public GoogleCloudRunV2Execution setGeneration(java.lang.Long generation) {
this.generation = generation;
return this;
}
/**
* Output only. The name of the parent Job.
* @return value or {@code null} for none
*/
public java.lang.String getJob() {
return job;
}
/**
* Output only. The name of the parent Job.
* @param job job or {@code null} for none
*/
public GoogleCloudRunV2Execution setJob(java.lang.String job) {
this.job = job;
return this;
}
/**
* Output only. Unstructured key value map that can be used to organize and categorize objects.
* User-provided labels are shared with Google's billing system, so they can be used to filter, or
* break down billing charges by team, component, environment, state, etc. For more information,
* visit https://cloud.google.com/resource-manager/docs/creating-managing-labels or
* https://cloud.google.com/run/docs/configuring/labels
* @return value or {@code null} for none
*/
public java.util.Map getLabels() {
return labels;
}
/**
* Output only. Unstructured key value map that can be used to organize and categorize objects.
* User-provided labels are shared with Google's billing system, so they can be used to filter, or
* break down billing charges by team, component, environment, state, etc. For more information,
* visit https://cloud.google.com/resource-manager/docs/creating-managing-labels or
* https://cloud.google.com/run/docs/configuring/labels
* @param labels labels or {@code null} for none
*/
public GoogleCloudRunV2Execution setLabels(java.util.Map labels) {
this.labels = labels;
return this;
}
/**
* The least stable launch stage needed to create this resource, as defined by [Google Cloud
* Platform Launch Stages](https://cloud.google.com/terms/launch-stages). Cloud Run supports
* `ALPHA`, `BETA`, and `GA`. Note that this value might not be what was used as input. For
* example, if ALPHA was provided as input in the parent resource, but only BETA and GA-level
* features are were, this field will be BETA.
* @return value or {@code null} for none
*/
public java.lang.String getLaunchStage() {
return launchStage;
}
/**
* The least stable launch stage needed to create this resource, as defined by [Google Cloud
* Platform Launch Stages](https://cloud.google.com/terms/launch-stages). Cloud Run supports
* `ALPHA`, `BETA`, and `GA`. Note that this value might not be what was used as input. For
* example, if ALPHA was provided as input in the parent resource, but only BETA and GA-level
* features are were, this field will be BETA.
* @param launchStage launchStage or {@code null} for none
*/
public GoogleCloudRunV2Execution setLaunchStage(java.lang.String launchStage) {
this.launchStage = launchStage;
return this;
}
/**
* Output only. URI where logs for this execution can be found in Cloud Console.
* @return value or {@code null} for none
*/
public java.lang.String getLogUri() {
return logUri;
}
/**
* Output only. URI where logs for this execution can be found in Cloud Console.
* @param logUri logUri or {@code null} for none
*/
public GoogleCloudRunV2Execution setLogUri(java.lang.String logUri) {
this.logUri = logUri;
return this;
}
/**
* Output only. The unique name of this Execution.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The unique name of this Execution.
* @param name name or {@code null} for none
*/
public GoogleCloudRunV2Execution setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Output only. The generation of this Execution. See comments in `reconciling` for additional
* information on reconciliation process in Cloud Run.
* @return value or {@code null} for none
*/
public java.lang.Long getObservedGeneration() {
return observedGeneration;
}
/**
* Output only. The generation of this Execution. See comments in `reconciling` for additional
* information on reconciliation process in Cloud Run.
* @param observedGeneration observedGeneration or {@code null} for none
*/
public GoogleCloudRunV2Execution setObservedGeneration(java.lang.Long observedGeneration) {
this.observedGeneration = observedGeneration;
return this;
}
/**
* Output only. Specifies the maximum desired number of tasks the execution should run at any
* given time. Must be <= task_count. The actual number of tasks running in steady state will be
* less than this number when ((.spec.task_count - .status.successful) < .spec.parallelism), i.e.
* when the work left to do is less than max parallelism.
* @return value or {@code null} for none
*/
public java.lang.Integer getParallelism() {
return parallelism;
}
/**
* Output only. Specifies the maximum desired number of tasks the execution should run at any
* given time. Must be <= task_count. The actual number of tasks running in steady state will be
* less than this number when ((.spec.task_count - .status.successful) < .spec.parallelism), i.e.
* when the work left to do is less than max parallelism.
* @param parallelism parallelism or {@code null} for none
*/
public GoogleCloudRunV2Execution setParallelism(java.lang.Integer parallelism) {
this.parallelism = parallelism;
return this;
}
/**
* Output only. Indicates whether the resource's reconciliation is still in progress. See comments
* in `Job.reconciling` for additional information on reconciliation process in Cloud Run.
* @return value or {@code null} for none
*/
public java.lang.Boolean getReconciling() {
return reconciling;
}
/**
* Output only. Indicates whether the resource's reconciliation is still in progress. See comments
* in `Job.reconciling` for additional information on reconciliation process in Cloud Run.
* @param reconciling reconciling or {@code null} for none
*/
public GoogleCloudRunV2Execution setReconciling(java.lang.Boolean reconciling) {
this.reconciling = reconciling;
return this;
}
/**
* Output only. The number of tasks which have retried at least once.
* @return value or {@code null} for none
*/
public java.lang.Integer getRetriedCount() {
return retriedCount;
}
/**
* Output only. The number of tasks which have retried at least once.
* @param retriedCount retriedCount or {@code null} for none
*/
public GoogleCloudRunV2Execution setRetriedCount(java.lang.Integer retriedCount) {
this.retriedCount = retriedCount;
return this;
}
/**
* Output only. The number of actively running tasks.
* @return value or {@code null} for none
*/
public java.lang.Integer getRunningCount() {
return runningCount;
}
/**
* Output only. The number of actively running tasks.
* @param runningCount runningCount or {@code null} for none
*/
public GoogleCloudRunV2Execution setRunningCount(java.lang.Integer runningCount) {
this.runningCount = runningCount;
return this;
}
/**
* Output only. Reserved for future use.
* @return value or {@code null} for none
*/
public java.lang.Boolean getSatisfiesPzs() {
return satisfiesPzs;
}
/**
* Output only. Reserved for future use.
* @param satisfiesPzs satisfiesPzs or {@code null} for none
*/
public GoogleCloudRunV2Execution setSatisfiesPzs(java.lang.Boolean satisfiesPzs) {
this.satisfiesPzs = satisfiesPzs;
return this;
}
/**
* Output only. Represents time when the execution started to run. It is not guaranteed to be set
* in happens-before order across separate operations.
* @return value or {@code null} for none
*/
public String getStartTime() {
return startTime;
}
/**
* Output only. Represents time when the execution started to run. It is not guaranteed to be set
* in happens-before order across separate operations.
* @param startTime startTime or {@code null} for none
*/
public GoogleCloudRunV2Execution setStartTime(String startTime) {
this.startTime = startTime;
return this;
}
/**
* Output only. The number of tasks which reached phase Succeeded.
* @return value or {@code null} for none
*/
public java.lang.Integer getSucceededCount() {
return succeededCount;
}
/**
* Output only. The number of tasks which reached phase Succeeded.
* @param succeededCount succeededCount or {@code null} for none
*/
public GoogleCloudRunV2Execution setSucceededCount(java.lang.Integer succeededCount) {
this.succeededCount = succeededCount;
return this;
}
/**
* Output only. Specifies the desired number of tasks the execution should run. Setting to 1 means
* that parallelism is limited to 1 and the success of that task signals the success of the
* execution.
* @return value or {@code null} for none
*/
public java.lang.Integer getTaskCount() {
return taskCount;
}
/**
* Output only. Specifies the desired number of tasks the execution should run. Setting to 1 means
* that parallelism is limited to 1 and the success of that task signals the success of the
* execution.
* @param taskCount taskCount or {@code null} for none
*/
public GoogleCloudRunV2Execution setTaskCount(java.lang.Integer taskCount) {
this.taskCount = taskCount;
return this;
}
/**
* Output only. The template used to create tasks for this execution.
* @return value or {@code null} for none
*/
public GoogleCloudRunV2TaskTemplate getTemplate() {
return template;
}
/**
* Output only. The template used to create tasks for this execution.
* @param template template or {@code null} for none
*/
public GoogleCloudRunV2Execution setTemplate(GoogleCloudRunV2TaskTemplate template) {
this.template = template;
return this;
}
/**
* Output only. Server assigned unique identifier for the Execution. The value is a UUID4 string
* and guaranteed to remain unchanged until the resource is deleted.
* @return value or {@code null} for none
*/
public java.lang.String getUid() {
return uid;
}
/**
* Output only. Server assigned unique identifier for the Execution. The value is a UUID4 string
* and guaranteed to remain unchanged until the resource is deleted.
* @param uid uid or {@code null} for none
*/
public GoogleCloudRunV2Execution setUid(java.lang.String uid) {
this.uid = uid;
return this;
}
/**
* Output only. The last-modified time.
* @return value or {@code null} for none
*/
public String getUpdateTime() {
return updateTime;
}
/**
* Output only. The last-modified time.
* @param updateTime updateTime or {@code null} for none
*/
public GoogleCloudRunV2Execution setUpdateTime(String updateTime) {
this.updateTime = updateTime;
return this;
}
@Override
public GoogleCloudRunV2Execution set(String fieldName, Object value) {
return (GoogleCloudRunV2Execution) super.set(fieldName, value);
}
@Override
public GoogleCloudRunV2Execution clone() {
return (GoogleCloudRunV2Execution) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy