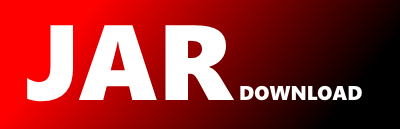
com.google.api.services.run.v2.model.GoogleCloudRunV2Job Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.run.v2.model;
/**
* Job represents the configuration of a single job, which references a container image that is run
* to completion.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Run Admin API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudRunV2Job extends com.google.api.client.json.GenericJson {
/**
* Unstructured key value map that may be set by external tools to store and arbitrary metadata.
* They are not queryable and should be preserved when modifying objects. Cloud Run API v2 does
* not support annotations with `run.googleapis.com`, `cloud.googleapis.com`,
* `serving.knative.dev`, or `autoscaling.knative.dev` namespaces, and they will be rejected on
* new resources. All system annotations in v1 now have a corresponding field in v2 Job. This
* field follows Kubernetes annotations' namespacing, limits, and rules.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map annotations;
/**
* Settings for the Binary Authorization feature.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRunV2BinaryAuthorization binaryAuthorization;
/**
* Arbitrary identifier for the API client.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String client;
/**
* Arbitrary version identifier for the API client.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String clientVersion;
/**
* Output only. The Conditions of all other associated sub-resources. They contain additional
* diagnostics information in case the Job does not reach its desired state. See comments in
* `reconciling` for additional information on reconciliation process in Cloud Run.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List conditions;
static {
// hack to force ProGuard to consider GoogleCloudRunV2Condition used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleCloudRunV2Condition.class);
}
/**
* Output only. The creation time.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String createTime;
/**
* Output only. Email address of the authenticated creator.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String creator;
/**
* Output only. The deletion time. It is only populated as a response to a Delete request.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String deleteTime;
/**
* Output only. A system-generated fingerprint for this version of the resource. May be used to
* detect modification conflict during updates.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String etag;
/**
* Output only. Number of executions created for this job.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer executionCount;
/**
* Output only. For a deleted resource, the time after which it will be permamently deleted.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String expireTime;
/**
* Output only. A number that monotonically increases every time the user modifies the desired
* state.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long generation;
/**
* Unstructured key value map that can be used to organize and categorize objects. User-provided
* labels are shared with Google's billing system, so they can be used to filter, or break down
* billing charges by team, component, environment, state, etc. For more information, visit
* https://cloud.google.com/resource-manager/docs/creating-managing-labels or
* https://cloud.google.com/run/docs/configuring/labels. Cloud Run API v2 does not support labels
* with `run.googleapis.com`, `cloud.googleapis.com`, `serving.knative.dev`, or
* `autoscaling.knative.dev` namespaces, and they will be rejected. All system labels in v1 now
* have a corresponding field in v2 Job.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map labels;
/**
* Output only. Email address of the last authenticated modifier.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String lastModifier;
/**
* Output only. Name of the last created execution.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRunV2ExecutionReference latestCreatedExecution;
/**
* The launch stage as defined by [Google Cloud Platform Launch
* Stages](https://cloud.google.com/terms/launch-stages). Cloud Run supports `ALPHA`, `BETA`, and
* `GA`. If no value is specified, GA is assumed. Set the launch stage to a preview stage on input
* to allow use of preview features in that stage. On read (or output), describes whether the
* resource uses preview features. For example, if ALPHA is provided as input, but only BETA and
* GA-level features are used, this field will be BETA on output.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String launchStage;
/**
* The fully qualified name of this Job. Format:
* projects/{project}/locations/{location}/jobs/{job}
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Output only. The generation of this Job. See comments in `reconciling` for additional
* information on reconciliation process in Cloud Run.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long observedGeneration;
/**
* Output only. Returns true if the Job is currently being acted upon by the system to bring it
* into the desired state. When a new Job is created, or an existing one is updated, Cloud Run
* will asynchronously perform all necessary steps to bring the Job to the desired state. This
* process is called reconciliation. While reconciliation is in process, `observed_generation` and
* `latest_succeeded_execution`, will have transient values that might mismatch the intended
* state: Once reconciliation is over (and this field is false), there are two possible outcomes:
* reconciliation succeeded and the state matches the Job, or there was an error, and
* reconciliation failed. This state can be found in `terminal_condition.state`. If reconciliation
* succeeded, the following fields will match: `observed_generation` and `generation`,
* `latest_succeeded_execution` and `latest_created_execution`. If reconciliation failed,
* `observed_generation` and `latest_succeeded_execution` will have the state of the last
* succeeded execution or empty for newly created Job. Additional information on the failure can
* be found in `terminal_condition` and `conditions`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean reconciling;
/**
* A unique string used as a suffix for creating a new execution. The Job will become ready when
* the execution is successfully completed. The sum of job name and token length must be fewer
* than 63 characters.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String runExecutionToken;
/**
* Output only. Reserved for future use.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean satisfiesPzs;
/**
* A unique string used as a suffix creating a new execution. The Job will become ready when the
* execution is successfully started. The sum of job name and token length must be fewer than 63
* characters.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String startExecutionToken;
/**
* Required. The template used to create executions for this Job.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRunV2ExecutionTemplate template;
/**
* Output only. The Condition of this Job, containing its readiness status, and detailed error
* information in case it did not reach the desired state.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRunV2Condition terminalCondition;
/**
* Output only. Server assigned unique identifier for the Execution. The value is a UUID4 string
* and guaranteed to remain unchanged until the resource is deleted.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String uid;
/**
* Output only. The last-modified time.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String updateTime;
/**
* Unstructured key value map that may be set by external tools to store and arbitrary metadata.
* They are not queryable and should be preserved when modifying objects. Cloud Run API v2 does
* not support annotations with `run.googleapis.com`, `cloud.googleapis.com`,
* `serving.knative.dev`, or `autoscaling.knative.dev` namespaces, and they will be rejected on
* new resources. All system annotations in v1 now have a corresponding field in v2 Job. This
* field follows Kubernetes annotations' namespacing, limits, and rules.
* @return value or {@code null} for none
*/
public java.util.Map getAnnotations() {
return annotations;
}
/**
* Unstructured key value map that may be set by external tools to store and arbitrary metadata.
* They are not queryable and should be preserved when modifying objects. Cloud Run API v2 does
* not support annotations with `run.googleapis.com`, `cloud.googleapis.com`,
* `serving.knative.dev`, or `autoscaling.knative.dev` namespaces, and they will be rejected on
* new resources. All system annotations in v1 now have a corresponding field in v2 Job. This
* field follows Kubernetes annotations' namespacing, limits, and rules.
* @param annotations annotations or {@code null} for none
*/
public GoogleCloudRunV2Job setAnnotations(java.util.Map annotations) {
this.annotations = annotations;
return this;
}
/**
* Settings for the Binary Authorization feature.
* @return value or {@code null} for none
*/
public GoogleCloudRunV2BinaryAuthorization getBinaryAuthorization() {
return binaryAuthorization;
}
/**
* Settings for the Binary Authorization feature.
* @param binaryAuthorization binaryAuthorization or {@code null} for none
*/
public GoogleCloudRunV2Job setBinaryAuthorization(GoogleCloudRunV2BinaryAuthorization binaryAuthorization) {
this.binaryAuthorization = binaryAuthorization;
return this;
}
/**
* Arbitrary identifier for the API client.
* @return value or {@code null} for none
*/
public java.lang.String getClient() {
return client;
}
/**
* Arbitrary identifier for the API client.
* @param client client or {@code null} for none
*/
public GoogleCloudRunV2Job setClient(java.lang.String client) {
this.client = client;
return this;
}
/**
* Arbitrary version identifier for the API client.
* @return value or {@code null} for none
*/
public java.lang.String getClientVersion() {
return clientVersion;
}
/**
* Arbitrary version identifier for the API client.
* @param clientVersion clientVersion or {@code null} for none
*/
public GoogleCloudRunV2Job setClientVersion(java.lang.String clientVersion) {
this.clientVersion = clientVersion;
return this;
}
/**
* Output only. The Conditions of all other associated sub-resources. They contain additional
* diagnostics information in case the Job does not reach its desired state. See comments in
* `reconciling` for additional information on reconciliation process in Cloud Run.
* @return value or {@code null} for none
*/
public java.util.List getConditions() {
return conditions;
}
/**
* Output only. The Conditions of all other associated sub-resources. They contain additional
* diagnostics information in case the Job does not reach its desired state. See comments in
* `reconciling` for additional information on reconciliation process in Cloud Run.
* @param conditions conditions or {@code null} for none
*/
public GoogleCloudRunV2Job setConditions(java.util.List conditions) {
this.conditions = conditions;
return this;
}
/**
* Output only. The creation time.
* @return value or {@code null} for none
*/
public String getCreateTime() {
return createTime;
}
/**
* Output only. The creation time.
* @param createTime createTime or {@code null} for none
*/
public GoogleCloudRunV2Job setCreateTime(String createTime) {
this.createTime = createTime;
return this;
}
/**
* Output only. Email address of the authenticated creator.
* @return value or {@code null} for none
*/
public java.lang.String getCreator() {
return creator;
}
/**
* Output only. Email address of the authenticated creator.
* @param creator creator or {@code null} for none
*/
public GoogleCloudRunV2Job setCreator(java.lang.String creator) {
this.creator = creator;
return this;
}
/**
* Output only. The deletion time. It is only populated as a response to a Delete request.
* @return value or {@code null} for none
*/
public String getDeleteTime() {
return deleteTime;
}
/**
* Output only. The deletion time. It is only populated as a response to a Delete request.
* @param deleteTime deleteTime or {@code null} for none
*/
public GoogleCloudRunV2Job setDeleteTime(String deleteTime) {
this.deleteTime = deleteTime;
return this;
}
/**
* Output only. A system-generated fingerprint for this version of the resource. May be used to
* detect modification conflict during updates.
* @return value or {@code null} for none
*/
public java.lang.String getEtag() {
return etag;
}
/**
* Output only. A system-generated fingerprint for this version of the resource. May be used to
* detect modification conflict during updates.
* @param etag etag or {@code null} for none
*/
public GoogleCloudRunV2Job setEtag(java.lang.String etag) {
this.etag = etag;
return this;
}
/**
* Output only. Number of executions created for this job.
* @return value or {@code null} for none
*/
public java.lang.Integer getExecutionCount() {
return executionCount;
}
/**
* Output only. Number of executions created for this job.
* @param executionCount executionCount or {@code null} for none
*/
public GoogleCloudRunV2Job setExecutionCount(java.lang.Integer executionCount) {
this.executionCount = executionCount;
return this;
}
/**
* Output only. For a deleted resource, the time after which it will be permamently deleted.
* @return value or {@code null} for none
*/
public String getExpireTime() {
return expireTime;
}
/**
* Output only. For a deleted resource, the time after which it will be permamently deleted.
* @param expireTime expireTime or {@code null} for none
*/
public GoogleCloudRunV2Job setExpireTime(String expireTime) {
this.expireTime = expireTime;
return this;
}
/**
* Output only. A number that monotonically increases every time the user modifies the desired
* state.
* @return value or {@code null} for none
*/
public java.lang.Long getGeneration() {
return generation;
}
/**
* Output only. A number that monotonically increases every time the user modifies the desired
* state.
* @param generation generation or {@code null} for none
*/
public GoogleCloudRunV2Job setGeneration(java.lang.Long generation) {
this.generation = generation;
return this;
}
/**
* Unstructured key value map that can be used to organize and categorize objects. User-provided
* labels are shared with Google's billing system, so they can be used to filter, or break down
* billing charges by team, component, environment, state, etc. For more information, visit
* https://cloud.google.com/resource-manager/docs/creating-managing-labels or
* https://cloud.google.com/run/docs/configuring/labels. Cloud Run API v2 does not support labels
* with `run.googleapis.com`, `cloud.googleapis.com`, `serving.knative.dev`, or
* `autoscaling.knative.dev` namespaces, and they will be rejected. All system labels in v1 now
* have a corresponding field in v2 Job.
* @return value or {@code null} for none
*/
public java.util.Map getLabels() {
return labels;
}
/**
* Unstructured key value map that can be used to organize and categorize objects. User-provided
* labels are shared with Google's billing system, so they can be used to filter, or break down
* billing charges by team, component, environment, state, etc. For more information, visit
* https://cloud.google.com/resource-manager/docs/creating-managing-labels or
* https://cloud.google.com/run/docs/configuring/labels. Cloud Run API v2 does not support labels
* with `run.googleapis.com`, `cloud.googleapis.com`, `serving.knative.dev`, or
* `autoscaling.knative.dev` namespaces, and they will be rejected. All system labels in v1 now
* have a corresponding field in v2 Job.
* @param labels labels or {@code null} for none
*/
public GoogleCloudRunV2Job setLabels(java.util.Map labels) {
this.labels = labels;
return this;
}
/**
* Output only. Email address of the last authenticated modifier.
* @return value or {@code null} for none
*/
public java.lang.String getLastModifier() {
return lastModifier;
}
/**
* Output only. Email address of the last authenticated modifier.
* @param lastModifier lastModifier or {@code null} for none
*/
public GoogleCloudRunV2Job setLastModifier(java.lang.String lastModifier) {
this.lastModifier = lastModifier;
return this;
}
/**
* Output only. Name of the last created execution.
* @return value or {@code null} for none
*/
public GoogleCloudRunV2ExecutionReference getLatestCreatedExecution() {
return latestCreatedExecution;
}
/**
* Output only. Name of the last created execution.
* @param latestCreatedExecution latestCreatedExecution or {@code null} for none
*/
public GoogleCloudRunV2Job setLatestCreatedExecution(GoogleCloudRunV2ExecutionReference latestCreatedExecution) {
this.latestCreatedExecution = latestCreatedExecution;
return this;
}
/**
* The launch stage as defined by [Google Cloud Platform Launch
* Stages](https://cloud.google.com/terms/launch-stages). Cloud Run supports `ALPHA`, `BETA`, and
* `GA`. If no value is specified, GA is assumed. Set the launch stage to a preview stage on input
* to allow use of preview features in that stage. On read (or output), describes whether the
* resource uses preview features. For example, if ALPHA is provided as input, but only BETA and
* GA-level features are used, this field will be BETA on output.
* @return value or {@code null} for none
*/
public java.lang.String getLaunchStage() {
return launchStage;
}
/**
* The launch stage as defined by [Google Cloud Platform Launch
* Stages](https://cloud.google.com/terms/launch-stages). Cloud Run supports `ALPHA`, `BETA`, and
* `GA`. If no value is specified, GA is assumed. Set the launch stage to a preview stage on input
* to allow use of preview features in that stage. On read (or output), describes whether the
* resource uses preview features. For example, if ALPHA is provided as input, but only BETA and
* GA-level features are used, this field will be BETA on output.
* @param launchStage launchStage or {@code null} for none
*/
public GoogleCloudRunV2Job setLaunchStage(java.lang.String launchStage) {
this.launchStage = launchStage;
return this;
}
/**
* The fully qualified name of this Job. Format:
* projects/{project}/locations/{location}/jobs/{job}
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* The fully qualified name of this Job. Format:
* projects/{project}/locations/{location}/jobs/{job}
* @param name name or {@code null} for none
*/
public GoogleCloudRunV2Job setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Output only. The generation of this Job. See comments in `reconciling` for additional
* information on reconciliation process in Cloud Run.
* @return value or {@code null} for none
*/
public java.lang.Long getObservedGeneration() {
return observedGeneration;
}
/**
* Output only. The generation of this Job. See comments in `reconciling` for additional
* information on reconciliation process in Cloud Run.
* @param observedGeneration observedGeneration or {@code null} for none
*/
public GoogleCloudRunV2Job setObservedGeneration(java.lang.Long observedGeneration) {
this.observedGeneration = observedGeneration;
return this;
}
/**
* Output only. Returns true if the Job is currently being acted upon by the system to bring it
* into the desired state. When a new Job is created, or an existing one is updated, Cloud Run
* will asynchronously perform all necessary steps to bring the Job to the desired state. This
* process is called reconciliation. While reconciliation is in process, `observed_generation` and
* `latest_succeeded_execution`, will have transient values that might mismatch the intended
* state: Once reconciliation is over (and this field is false), there are two possible outcomes:
* reconciliation succeeded and the state matches the Job, or there was an error, and
* reconciliation failed. This state can be found in `terminal_condition.state`. If reconciliation
* succeeded, the following fields will match: `observed_generation` and `generation`,
* `latest_succeeded_execution` and `latest_created_execution`. If reconciliation failed,
* `observed_generation` and `latest_succeeded_execution` will have the state of the last
* succeeded execution or empty for newly created Job. Additional information on the failure can
* be found in `terminal_condition` and `conditions`.
* @return value or {@code null} for none
*/
public java.lang.Boolean getReconciling() {
return reconciling;
}
/**
* Output only. Returns true if the Job is currently being acted upon by the system to bring it
* into the desired state. When a new Job is created, or an existing one is updated, Cloud Run
* will asynchronously perform all necessary steps to bring the Job to the desired state. This
* process is called reconciliation. While reconciliation is in process, `observed_generation` and
* `latest_succeeded_execution`, will have transient values that might mismatch the intended
* state: Once reconciliation is over (and this field is false), there are two possible outcomes:
* reconciliation succeeded and the state matches the Job, or there was an error, and
* reconciliation failed. This state can be found in `terminal_condition.state`. If reconciliation
* succeeded, the following fields will match: `observed_generation` and `generation`,
* `latest_succeeded_execution` and `latest_created_execution`. If reconciliation failed,
* `observed_generation` and `latest_succeeded_execution` will have the state of the last
* succeeded execution or empty for newly created Job. Additional information on the failure can
* be found in `terminal_condition` and `conditions`.
* @param reconciling reconciling or {@code null} for none
*/
public GoogleCloudRunV2Job setReconciling(java.lang.Boolean reconciling) {
this.reconciling = reconciling;
return this;
}
/**
* A unique string used as a suffix for creating a new execution. The Job will become ready when
* the execution is successfully completed. The sum of job name and token length must be fewer
* than 63 characters.
* @return value or {@code null} for none
*/
public java.lang.String getRunExecutionToken() {
return runExecutionToken;
}
/**
* A unique string used as a suffix for creating a new execution. The Job will become ready when
* the execution is successfully completed. The sum of job name and token length must be fewer
* than 63 characters.
* @param runExecutionToken runExecutionToken or {@code null} for none
*/
public GoogleCloudRunV2Job setRunExecutionToken(java.lang.String runExecutionToken) {
this.runExecutionToken = runExecutionToken;
return this;
}
/**
* Output only. Reserved for future use.
* @return value or {@code null} for none
*/
public java.lang.Boolean getSatisfiesPzs() {
return satisfiesPzs;
}
/**
* Output only. Reserved for future use.
* @param satisfiesPzs satisfiesPzs or {@code null} for none
*/
public GoogleCloudRunV2Job setSatisfiesPzs(java.lang.Boolean satisfiesPzs) {
this.satisfiesPzs = satisfiesPzs;
return this;
}
/**
* A unique string used as a suffix creating a new execution. The Job will become ready when the
* execution is successfully started. The sum of job name and token length must be fewer than 63
* characters.
* @return value or {@code null} for none
*/
public java.lang.String getStartExecutionToken() {
return startExecutionToken;
}
/**
* A unique string used as a suffix creating a new execution. The Job will become ready when the
* execution is successfully started. The sum of job name and token length must be fewer than 63
* characters.
* @param startExecutionToken startExecutionToken or {@code null} for none
*/
public GoogleCloudRunV2Job setStartExecutionToken(java.lang.String startExecutionToken) {
this.startExecutionToken = startExecutionToken;
return this;
}
/**
* Required. The template used to create executions for this Job.
* @return value or {@code null} for none
*/
public GoogleCloudRunV2ExecutionTemplate getTemplate() {
return template;
}
/**
* Required. The template used to create executions for this Job.
* @param template template or {@code null} for none
*/
public GoogleCloudRunV2Job setTemplate(GoogleCloudRunV2ExecutionTemplate template) {
this.template = template;
return this;
}
/**
* Output only. The Condition of this Job, containing its readiness status, and detailed error
* information in case it did not reach the desired state.
* @return value or {@code null} for none
*/
public GoogleCloudRunV2Condition getTerminalCondition() {
return terminalCondition;
}
/**
* Output only. The Condition of this Job, containing its readiness status, and detailed error
* information in case it did not reach the desired state.
* @param terminalCondition terminalCondition or {@code null} for none
*/
public GoogleCloudRunV2Job setTerminalCondition(GoogleCloudRunV2Condition terminalCondition) {
this.terminalCondition = terminalCondition;
return this;
}
/**
* Output only. Server assigned unique identifier for the Execution. The value is a UUID4 string
* and guaranteed to remain unchanged until the resource is deleted.
* @return value or {@code null} for none
*/
public java.lang.String getUid() {
return uid;
}
/**
* Output only. Server assigned unique identifier for the Execution. The value is a UUID4 string
* and guaranteed to remain unchanged until the resource is deleted.
* @param uid uid or {@code null} for none
*/
public GoogleCloudRunV2Job setUid(java.lang.String uid) {
this.uid = uid;
return this;
}
/**
* Output only. The last-modified time.
* @return value or {@code null} for none
*/
public String getUpdateTime() {
return updateTime;
}
/**
* Output only. The last-modified time.
* @param updateTime updateTime or {@code null} for none
*/
public GoogleCloudRunV2Job setUpdateTime(String updateTime) {
this.updateTime = updateTime;
return this;
}
@Override
public GoogleCloudRunV2Job set(String fieldName, Object value) {
return (GoogleCloudRunV2Job) super.set(fieldName, value);
}
@Override
public GoogleCloudRunV2Job clone() {
return (GoogleCloudRunV2Job) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy