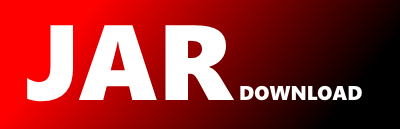
com.google.api.services.run.v2.model.GoogleCloudRunV2Service Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.run.v2.model;
/**
* Service acts as a top-level container that manages a set of configurations and revision templates
* which implement a network service. Service exists to provide a singular abstraction which can be
* access controlled, reasoned about, and which encapsulates software lifecycle decisions such as
* rollout policy and team resource ownership.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Run Admin API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudRunV2Service extends com.google.api.client.json.GenericJson {
/**
* Optional. Unstructured key value map that may be set by external tools to store and arbitrary
* metadata. They are not queryable and should be preserved when modifying objects. Cloud Run API
* v2 does not support annotations with `run.googleapis.com`, `cloud.googleapis.com`,
* `serving.knative.dev`, or `autoscaling.knative.dev` namespaces, and they will be rejected in
* new resources. All system annotations in v1 now have a corresponding field in v2 Service. This
* field follows Kubernetes annotations' namespacing, limits, and rules.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map annotations;
/**
* Optional. Settings for the Binary Authorization feature.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRunV2BinaryAuthorization binaryAuthorization;
/**
* Arbitrary identifier for the API client.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String client;
/**
* Arbitrary version identifier for the API client.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String clientVersion;
/**
* Output only. The Conditions of all other associated sub-resources. They contain additional
* diagnostics information in case the Service does not reach its Serving state. See comments in
* `reconciling` for additional information on reconciliation process in Cloud Run.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List conditions;
static {
// hack to force ProGuard to consider GoogleCloudRunV2Condition used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleCloudRunV2Condition.class);
}
/**
* Output only. The creation time.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String createTime;
/**
* Output only. Email address of the authenticated creator.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String creator;
/**
* One or more custom audiences that you want this service to support. Specify each custom
* audience as the full URL in a string. The custom audiences are encoded in the token and used to
* authenticate requests. For more information, see
* https://cloud.google.com/run/docs/configuring/custom-audiences.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List customAudiences;
/**
* Optional. Disables public resolution of the default URI of this service.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean defaultUriDisabled;
/**
* Output only. The deletion time. It is only populated as a response to a Delete request.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String deleteTime;
/**
* User-provided description of the Service. This field currently has a 512-character limit.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* Output only. A system-generated fingerprint for this version of the resource. May be used to
* detect modification conflict during updates.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String etag;
/**
* Output only. For a deleted resource, the time after which it will be permamently deleted.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String expireTime;
/**
* Output only. A number that monotonically increases every time the user modifies the desired
* state. Please note that unlike v1, this is an int64 value. As with most Google APIs, its JSON
* representation will be a `string` instead of an `integer`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long generation;
/**
* Optional. Provides the ingress settings for this Service. On output, returns the currently
* observed ingress settings, or INGRESS_TRAFFIC_UNSPECIFIED if no revision is active.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String ingress;
/**
* Optional. Disables IAM permission check for run.routes.invoke for callers of this service. This
* feature is available by invitation only. For more information, visit
* https://cloud.google.com/run/docs/securing/managing-access#invoker_check.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean invokerIamDisabled;
/**
* Optional. Unstructured key value map that can be used to organize and categorize objects. User-
* provided labels are shared with Google's billing system, so they can be used to filter, or
* break down billing charges by team, component, environment, state, etc. For more information,
* visit https://cloud.google.com/resource-manager/docs/creating-managing-labels or
* https://cloud.google.com/run/docs/configuring/labels. Cloud Run API v2 does not support labels
* with `run.googleapis.com`, `cloud.googleapis.com`, `serving.knative.dev`, or
* `autoscaling.knative.dev` namespaces, and they will be rejected. All system labels in v1 now
* have a corresponding field in v2 Service.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map labels;
/**
* Output only. Email address of the last authenticated modifier.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String lastModifier;
/**
* Output only. Name of the last created revision. See comments in `reconciling` for additional
* information on reconciliation process in Cloud Run.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String latestCreatedRevision;
/**
* Output only. Name of the latest revision that is serving traffic. See comments in `reconciling`
* for additional information on reconciliation process in Cloud Run.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String latestReadyRevision;
/**
* Optional. The launch stage as defined by [Google Cloud Platform Launch
* Stages](https://cloud.google.com/terms/launch-stages). Cloud Run supports `ALPHA`, `BETA`, and
* `GA`. If no value is specified, GA is assumed. Set the launch stage to a preview stage on input
* to allow use of preview features in that stage. On read (or output), describes whether the
* resource uses preview features. For example, if ALPHA is provided as input, but only BETA and
* GA-level features are used, this field will be BETA on output.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String launchStage;
/**
* The fully qualified name of this Service. In CreateServiceRequest, this field is ignored, and
* instead composed from CreateServiceRequest.parent and CreateServiceRequest.service_id. Format:
* projects/{project}/locations/{location}/services/{service_id}
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Output only. The generation of this Service currently serving traffic. See comments in
* `reconciling` for additional information on reconciliation process in Cloud Run. Please note
* that unlike v1, this is an int64 value. As with most Google APIs, its JSON representation will
* be a `string` instead of an `integer`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long observedGeneration;
/**
* Output only. Returns true if the Service is currently being acted upon by the system to bring
* it into the desired state. When a new Service is created, or an existing one is updated, Cloud
* Run will asynchronously perform all necessary steps to bring the Service to the desired serving
* state. This process is called reconciliation. While reconciliation is in process,
* `observed_generation`, `latest_ready_revison`, `traffic_statuses`, and `uri` will have
* transient values that might mismatch the intended state: Once reconciliation is over (and this
* field is false), there are two possible outcomes: reconciliation succeeded and the serving
* state matches the Service, or there was an error, and reconciliation failed. This state can be
* found in `terminal_condition.state`. If reconciliation succeeded, the following fields will
* match: `traffic` and `traffic_statuses`, `observed_generation` and `generation`,
* `latest_ready_revision` and `latest_created_revision`. If reconciliation failed,
* `traffic_statuses`, `observed_generation`, and `latest_ready_revision` will have the state of
* the last serving revision, or empty for newly created Services. Additional information on the
* failure can be found in `terminal_condition` and `conditions`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean reconciling;
/**
* Output only. Reserved for future use.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean satisfiesPzs;
/**
* Optional. Specifies service-level scaling settings
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRunV2ServiceScaling scaling;
/**
* Required. The template used to create revisions for this Service.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRunV2RevisionTemplate template;
/**
* Output only. The Condition of this Service, containing its readiness status, and detailed error
* information in case it did not reach a serving state. See comments in `reconciling` for
* additional information on reconciliation process in Cloud Run.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRunV2Condition terminalCondition;
/**
* Optional. Specifies how to distribute traffic over a collection of Revisions belonging to the
* Service. If traffic is empty or not provided, defaults to 100% traffic to the latest `Ready`
* Revision.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List traffic;
/**
* Output only. Detailed status information for corresponding traffic targets. See comments in
* `reconciling` for additional information on reconciliation process in Cloud Run.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List trafficStatuses;
/**
* Output only. Server assigned unique identifier for the trigger. The value is a UUID4 string and
* guaranteed to remain unchanged until the resource is deleted.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String uid;
/**
* Output only. The last-modified time.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String updateTime;
/**
* Output only. The main URI in which this Service is serving traffic.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String uri;
/**
* Output only. All URLs serving traffic for this Service.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List urls;
/**
* Optional. Unstructured key value map that may be set by external tools to store and arbitrary
* metadata. They are not queryable and should be preserved when modifying objects. Cloud Run API
* v2 does not support annotations with `run.googleapis.com`, `cloud.googleapis.com`,
* `serving.knative.dev`, or `autoscaling.knative.dev` namespaces, and they will be rejected in
* new resources. All system annotations in v1 now have a corresponding field in v2 Service. This
* field follows Kubernetes annotations' namespacing, limits, and rules.
* @return value or {@code null} for none
*/
public java.util.Map getAnnotations() {
return annotations;
}
/**
* Optional. Unstructured key value map that may be set by external tools to store and arbitrary
* metadata. They are not queryable and should be preserved when modifying objects. Cloud Run API
* v2 does not support annotations with `run.googleapis.com`, `cloud.googleapis.com`,
* `serving.knative.dev`, or `autoscaling.knative.dev` namespaces, and they will be rejected in
* new resources. All system annotations in v1 now have a corresponding field in v2 Service. This
* field follows Kubernetes annotations' namespacing, limits, and rules.
* @param annotations annotations or {@code null} for none
*/
public GoogleCloudRunV2Service setAnnotations(java.util.Map annotations) {
this.annotations = annotations;
return this;
}
/**
* Optional. Settings for the Binary Authorization feature.
* @return value or {@code null} for none
*/
public GoogleCloudRunV2BinaryAuthorization getBinaryAuthorization() {
return binaryAuthorization;
}
/**
* Optional. Settings for the Binary Authorization feature.
* @param binaryAuthorization binaryAuthorization or {@code null} for none
*/
public GoogleCloudRunV2Service setBinaryAuthorization(GoogleCloudRunV2BinaryAuthorization binaryAuthorization) {
this.binaryAuthorization = binaryAuthorization;
return this;
}
/**
* Arbitrary identifier for the API client.
* @return value or {@code null} for none
*/
public java.lang.String getClient() {
return client;
}
/**
* Arbitrary identifier for the API client.
* @param client client or {@code null} for none
*/
public GoogleCloudRunV2Service setClient(java.lang.String client) {
this.client = client;
return this;
}
/**
* Arbitrary version identifier for the API client.
* @return value or {@code null} for none
*/
public java.lang.String getClientVersion() {
return clientVersion;
}
/**
* Arbitrary version identifier for the API client.
* @param clientVersion clientVersion or {@code null} for none
*/
public GoogleCloudRunV2Service setClientVersion(java.lang.String clientVersion) {
this.clientVersion = clientVersion;
return this;
}
/**
* Output only. The Conditions of all other associated sub-resources. They contain additional
* diagnostics information in case the Service does not reach its Serving state. See comments in
* `reconciling` for additional information on reconciliation process in Cloud Run.
* @return value or {@code null} for none
*/
public java.util.List getConditions() {
return conditions;
}
/**
* Output only. The Conditions of all other associated sub-resources. They contain additional
* diagnostics information in case the Service does not reach its Serving state. See comments in
* `reconciling` for additional information on reconciliation process in Cloud Run.
* @param conditions conditions or {@code null} for none
*/
public GoogleCloudRunV2Service setConditions(java.util.List conditions) {
this.conditions = conditions;
return this;
}
/**
* Output only. The creation time.
* @return value or {@code null} for none
*/
public String getCreateTime() {
return createTime;
}
/**
* Output only. The creation time.
* @param createTime createTime or {@code null} for none
*/
public GoogleCloudRunV2Service setCreateTime(String createTime) {
this.createTime = createTime;
return this;
}
/**
* Output only. Email address of the authenticated creator.
* @return value or {@code null} for none
*/
public java.lang.String getCreator() {
return creator;
}
/**
* Output only. Email address of the authenticated creator.
* @param creator creator or {@code null} for none
*/
public GoogleCloudRunV2Service setCreator(java.lang.String creator) {
this.creator = creator;
return this;
}
/**
* One or more custom audiences that you want this service to support. Specify each custom
* audience as the full URL in a string. The custom audiences are encoded in the token and used to
* authenticate requests. For more information, see
* https://cloud.google.com/run/docs/configuring/custom-audiences.
* @return value or {@code null} for none
*/
public java.util.List getCustomAudiences() {
return customAudiences;
}
/**
* One or more custom audiences that you want this service to support. Specify each custom
* audience as the full URL in a string. The custom audiences are encoded in the token and used to
* authenticate requests. For more information, see
* https://cloud.google.com/run/docs/configuring/custom-audiences.
* @param customAudiences customAudiences or {@code null} for none
*/
public GoogleCloudRunV2Service setCustomAudiences(java.util.List customAudiences) {
this.customAudiences = customAudiences;
return this;
}
/**
* Optional. Disables public resolution of the default URI of this service.
* @return value or {@code null} for none
*/
public java.lang.Boolean getDefaultUriDisabled() {
return defaultUriDisabled;
}
/**
* Optional. Disables public resolution of the default URI of this service.
* @param defaultUriDisabled defaultUriDisabled or {@code null} for none
*/
public GoogleCloudRunV2Service setDefaultUriDisabled(java.lang.Boolean defaultUriDisabled) {
this.defaultUriDisabled = defaultUriDisabled;
return this;
}
/**
* Output only. The deletion time. It is only populated as a response to a Delete request.
* @return value or {@code null} for none
*/
public String getDeleteTime() {
return deleteTime;
}
/**
* Output only. The deletion time. It is only populated as a response to a Delete request.
* @param deleteTime deleteTime or {@code null} for none
*/
public GoogleCloudRunV2Service setDeleteTime(String deleteTime) {
this.deleteTime = deleteTime;
return this;
}
/**
* User-provided description of the Service. This field currently has a 512-character limit.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* User-provided description of the Service. This field currently has a 512-character limit.
* @param description description or {@code null} for none
*/
public GoogleCloudRunV2Service setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* Output only. A system-generated fingerprint for this version of the resource. May be used to
* detect modification conflict during updates.
* @return value or {@code null} for none
*/
public java.lang.String getEtag() {
return etag;
}
/**
* Output only. A system-generated fingerprint for this version of the resource. May be used to
* detect modification conflict during updates.
* @param etag etag or {@code null} for none
*/
public GoogleCloudRunV2Service setEtag(java.lang.String etag) {
this.etag = etag;
return this;
}
/**
* Output only. For a deleted resource, the time after which it will be permamently deleted.
* @return value or {@code null} for none
*/
public String getExpireTime() {
return expireTime;
}
/**
* Output only. For a deleted resource, the time after which it will be permamently deleted.
* @param expireTime expireTime or {@code null} for none
*/
public GoogleCloudRunV2Service setExpireTime(String expireTime) {
this.expireTime = expireTime;
return this;
}
/**
* Output only. A number that monotonically increases every time the user modifies the desired
* state. Please note that unlike v1, this is an int64 value. As with most Google APIs, its JSON
* representation will be a `string` instead of an `integer`.
* @return value or {@code null} for none
*/
public java.lang.Long getGeneration() {
return generation;
}
/**
* Output only. A number that monotonically increases every time the user modifies the desired
* state. Please note that unlike v1, this is an int64 value. As with most Google APIs, its JSON
* representation will be a `string` instead of an `integer`.
* @param generation generation or {@code null} for none
*/
public GoogleCloudRunV2Service setGeneration(java.lang.Long generation) {
this.generation = generation;
return this;
}
/**
* Optional. Provides the ingress settings for this Service. On output, returns the currently
* observed ingress settings, or INGRESS_TRAFFIC_UNSPECIFIED if no revision is active.
* @return value or {@code null} for none
*/
public java.lang.String getIngress() {
return ingress;
}
/**
* Optional. Provides the ingress settings for this Service. On output, returns the currently
* observed ingress settings, or INGRESS_TRAFFIC_UNSPECIFIED if no revision is active.
* @param ingress ingress or {@code null} for none
*/
public GoogleCloudRunV2Service setIngress(java.lang.String ingress) {
this.ingress = ingress;
return this;
}
/**
* Optional. Disables IAM permission check for run.routes.invoke for callers of this service. This
* feature is available by invitation only. For more information, visit
* https://cloud.google.com/run/docs/securing/managing-access#invoker_check.
* @return value or {@code null} for none
*/
public java.lang.Boolean getInvokerIamDisabled() {
return invokerIamDisabled;
}
/**
* Optional. Disables IAM permission check for run.routes.invoke for callers of this service. This
* feature is available by invitation only. For more information, visit
* https://cloud.google.com/run/docs/securing/managing-access#invoker_check.
* @param invokerIamDisabled invokerIamDisabled or {@code null} for none
*/
public GoogleCloudRunV2Service setInvokerIamDisabled(java.lang.Boolean invokerIamDisabled) {
this.invokerIamDisabled = invokerIamDisabled;
return this;
}
/**
* Optional. Unstructured key value map that can be used to organize and categorize objects. User-
* provided labels are shared with Google's billing system, so they can be used to filter, or
* break down billing charges by team, component, environment, state, etc. For more information,
* visit https://cloud.google.com/resource-manager/docs/creating-managing-labels or
* https://cloud.google.com/run/docs/configuring/labels. Cloud Run API v2 does not support labels
* with `run.googleapis.com`, `cloud.googleapis.com`, `serving.knative.dev`, or
* `autoscaling.knative.dev` namespaces, and they will be rejected. All system labels in v1 now
* have a corresponding field in v2 Service.
* @return value or {@code null} for none
*/
public java.util.Map getLabels() {
return labels;
}
/**
* Optional. Unstructured key value map that can be used to organize and categorize objects. User-
* provided labels are shared with Google's billing system, so they can be used to filter, or
* break down billing charges by team, component, environment, state, etc. For more information,
* visit https://cloud.google.com/resource-manager/docs/creating-managing-labels or
* https://cloud.google.com/run/docs/configuring/labels. Cloud Run API v2 does not support labels
* with `run.googleapis.com`, `cloud.googleapis.com`, `serving.knative.dev`, or
* `autoscaling.knative.dev` namespaces, and they will be rejected. All system labels in v1 now
* have a corresponding field in v2 Service.
* @param labels labels or {@code null} for none
*/
public GoogleCloudRunV2Service setLabels(java.util.Map labels) {
this.labels = labels;
return this;
}
/**
* Output only. Email address of the last authenticated modifier.
* @return value or {@code null} for none
*/
public java.lang.String getLastModifier() {
return lastModifier;
}
/**
* Output only. Email address of the last authenticated modifier.
* @param lastModifier lastModifier or {@code null} for none
*/
public GoogleCloudRunV2Service setLastModifier(java.lang.String lastModifier) {
this.lastModifier = lastModifier;
return this;
}
/**
* Output only. Name of the last created revision. See comments in `reconciling` for additional
* information on reconciliation process in Cloud Run.
* @return value or {@code null} for none
*/
public java.lang.String getLatestCreatedRevision() {
return latestCreatedRevision;
}
/**
* Output only. Name of the last created revision. See comments in `reconciling` for additional
* information on reconciliation process in Cloud Run.
* @param latestCreatedRevision latestCreatedRevision or {@code null} for none
*/
public GoogleCloudRunV2Service setLatestCreatedRevision(java.lang.String latestCreatedRevision) {
this.latestCreatedRevision = latestCreatedRevision;
return this;
}
/**
* Output only. Name of the latest revision that is serving traffic. See comments in `reconciling`
* for additional information on reconciliation process in Cloud Run.
* @return value or {@code null} for none
*/
public java.lang.String getLatestReadyRevision() {
return latestReadyRevision;
}
/**
* Output only. Name of the latest revision that is serving traffic. See comments in `reconciling`
* for additional information on reconciliation process in Cloud Run.
* @param latestReadyRevision latestReadyRevision or {@code null} for none
*/
public GoogleCloudRunV2Service setLatestReadyRevision(java.lang.String latestReadyRevision) {
this.latestReadyRevision = latestReadyRevision;
return this;
}
/**
* Optional. The launch stage as defined by [Google Cloud Platform Launch
* Stages](https://cloud.google.com/terms/launch-stages). Cloud Run supports `ALPHA`, `BETA`, and
* `GA`. If no value is specified, GA is assumed. Set the launch stage to a preview stage on input
* to allow use of preview features in that stage. On read (or output), describes whether the
* resource uses preview features. For example, if ALPHA is provided as input, but only BETA and
* GA-level features are used, this field will be BETA on output.
* @return value or {@code null} for none
*/
public java.lang.String getLaunchStage() {
return launchStage;
}
/**
* Optional. The launch stage as defined by [Google Cloud Platform Launch
* Stages](https://cloud.google.com/terms/launch-stages). Cloud Run supports `ALPHA`, `BETA`, and
* `GA`. If no value is specified, GA is assumed. Set the launch stage to a preview stage on input
* to allow use of preview features in that stage. On read (or output), describes whether the
* resource uses preview features. For example, if ALPHA is provided as input, but only BETA and
* GA-level features are used, this field will be BETA on output.
* @param launchStage launchStage or {@code null} for none
*/
public GoogleCloudRunV2Service setLaunchStage(java.lang.String launchStage) {
this.launchStage = launchStage;
return this;
}
/**
* The fully qualified name of this Service. In CreateServiceRequest, this field is ignored, and
* instead composed from CreateServiceRequest.parent and CreateServiceRequest.service_id. Format:
* projects/{project}/locations/{location}/services/{service_id}
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* The fully qualified name of this Service. In CreateServiceRequest, this field is ignored, and
* instead composed from CreateServiceRequest.parent and CreateServiceRequest.service_id. Format:
* projects/{project}/locations/{location}/services/{service_id}
* @param name name or {@code null} for none
*/
public GoogleCloudRunV2Service setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Output only. The generation of this Service currently serving traffic. See comments in
* `reconciling` for additional information on reconciliation process in Cloud Run. Please note
* that unlike v1, this is an int64 value. As with most Google APIs, its JSON representation will
* be a `string` instead of an `integer`.
* @return value or {@code null} for none
*/
public java.lang.Long getObservedGeneration() {
return observedGeneration;
}
/**
* Output only. The generation of this Service currently serving traffic. See comments in
* `reconciling` for additional information on reconciliation process in Cloud Run. Please note
* that unlike v1, this is an int64 value. As with most Google APIs, its JSON representation will
* be a `string` instead of an `integer`.
* @param observedGeneration observedGeneration or {@code null} for none
*/
public GoogleCloudRunV2Service setObservedGeneration(java.lang.Long observedGeneration) {
this.observedGeneration = observedGeneration;
return this;
}
/**
* Output only. Returns true if the Service is currently being acted upon by the system to bring
* it into the desired state. When a new Service is created, or an existing one is updated, Cloud
* Run will asynchronously perform all necessary steps to bring the Service to the desired serving
* state. This process is called reconciliation. While reconciliation is in process,
* `observed_generation`, `latest_ready_revison`, `traffic_statuses`, and `uri` will have
* transient values that might mismatch the intended state: Once reconciliation is over (and this
* field is false), there are two possible outcomes: reconciliation succeeded and the serving
* state matches the Service, or there was an error, and reconciliation failed. This state can be
* found in `terminal_condition.state`. If reconciliation succeeded, the following fields will
* match: `traffic` and `traffic_statuses`, `observed_generation` and `generation`,
* `latest_ready_revision` and `latest_created_revision`. If reconciliation failed,
* `traffic_statuses`, `observed_generation`, and `latest_ready_revision` will have the state of
* the last serving revision, or empty for newly created Services. Additional information on the
* failure can be found in `terminal_condition` and `conditions`.
* @return value or {@code null} for none
*/
public java.lang.Boolean getReconciling() {
return reconciling;
}
/**
* Output only. Returns true if the Service is currently being acted upon by the system to bring
* it into the desired state. When a new Service is created, or an existing one is updated, Cloud
* Run will asynchronously perform all necessary steps to bring the Service to the desired serving
* state. This process is called reconciliation. While reconciliation is in process,
* `observed_generation`, `latest_ready_revison`, `traffic_statuses`, and `uri` will have
* transient values that might mismatch the intended state: Once reconciliation is over (and this
* field is false), there are two possible outcomes: reconciliation succeeded and the serving
* state matches the Service, or there was an error, and reconciliation failed. This state can be
* found in `terminal_condition.state`. If reconciliation succeeded, the following fields will
* match: `traffic` and `traffic_statuses`, `observed_generation` and `generation`,
* `latest_ready_revision` and `latest_created_revision`. If reconciliation failed,
* `traffic_statuses`, `observed_generation`, and `latest_ready_revision` will have the state of
* the last serving revision, or empty for newly created Services. Additional information on the
* failure can be found in `terminal_condition` and `conditions`.
* @param reconciling reconciling or {@code null} for none
*/
public GoogleCloudRunV2Service setReconciling(java.lang.Boolean reconciling) {
this.reconciling = reconciling;
return this;
}
/**
* Output only. Reserved for future use.
* @return value or {@code null} for none
*/
public java.lang.Boolean getSatisfiesPzs() {
return satisfiesPzs;
}
/**
* Output only. Reserved for future use.
* @param satisfiesPzs satisfiesPzs or {@code null} for none
*/
public GoogleCloudRunV2Service setSatisfiesPzs(java.lang.Boolean satisfiesPzs) {
this.satisfiesPzs = satisfiesPzs;
return this;
}
/**
* Optional. Specifies service-level scaling settings
* @return value or {@code null} for none
*/
public GoogleCloudRunV2ServiceScaling getScaling() {
return scaling;
}
/**
* Optional. Specifies service-level scaling settings
* @param scaling scaling or {@code null} for none
*/
public GoogleCloudRunV2Service setScaling(GoogleCloudRunV2ServiceScaling scaling) {
this.scaling = scaling;
return this;
}
/**
* Required. The template used to create revisions for this Service.
* @return value or {@code null} for none
*/
public GoogleCloudRunV2RevisionTemplate getTemplate() {
return template;
}
/**
* Required. The template used to create revisions for this Service.
* @param template template or {@code null} for none
*/
public GoogleCloudRunV2Service setTemplate(GoogleCloudRunV2RevisionTemplate template) {
this.template = template;
return this;
}
/**
* Output only. The Condition of this Service, containing its readiness status, and detailed error
* information in case it did not reach a serving state. See comments in `reconciling` for
* additional information on reconciliation process in Cloud Run.
* @return value or {@code null} for none
*/
public GoogleCloudRunV2Condition getTerminalCondition() {
return terminalCondition;
}
/**
* Output only. The Condition of this Service, containing its readiness status, and detailed error
* information in case it did not reach a serving state. See comments in `reconciling` for
* additional information on reconciliation process in Cloud Run.
* @param terminalCondition terminalCondition or {@code null} for none
*/
public GoogleCloudRunV2Service setTerminalCondition(GoogleCloudRunV2Condition terminalCondition) {
this.terminalCondition = terminalCondition;
return this;
}
/**
* Optional. Specifies how to distribute traffic over a collection of Revisions belonging to the
* Service. If traffic is empty or not provided, defaults to 100% traffic to the latest `Ready`
* Revision.
* @return value or {@code null} for none
*/
public java.util.List getTraffic() {
return traffic;
}
/**
* Optional. Specifies how to distribute traffic over a collection of Revisions belonging to the
* Service. If traffic is empty or not provided, defaults to 100% traffic to the latest `Ready`
* Revision.
* @param traffic traffic or {@code null} for none
*/
public GoogleCloudRunV2Service setTraffic(java.util.List traffic) {
this.traffic = traffic;
return this;
}
/**
* Output only. Detailed status information for corresponding traffic targets. See comments in
* `reconciling` for additional information on reconciliation process in Cloud Run.
* @return value or {@code null} for none
*/
public java.util.List getTrafficStatuses() {
return trafficStatuses;
}
/**
* Output only. Detailed status information for corresponding traffic targets. See comments in
* `reconciling` for additional information on reconciliation process in Cloud Run.
* @param trafficStatuses trafficStatuses or {@code null} for none
*/
public GoogleCloudRunV2Service setTrafficStatuses(java.util.List trafficStatuses) {
this.trafficStatuses = trafficStatuses;
return this;
}
/**
* Output only. Server assigned unique identifier for the trigger. The value is a UUID4 string and
* guaranteed to remain unchanged until the resource is deleted.
* @return value or {@code null} for none
*/
public java.lang.String getUid() {
return uid;
}
/**
* Output only. Server assigned unique identifier for the trigger. The value is a UUID4 string and
* guaranteed to remain unchanged until the resource is deleted.
* @param uid uid or {@code null} for none
*/
public GoogleCloudRunV2Service setUid(java.lang.String uid) {
this.uid = uid;
return this;
}
/**
* Output only. The last-modified time.
* @return value or {@code null} for none
*/
public String getUpdateTime() {
return updateTime;
}
/**
* Output only. The last-modified time.
* @param updateTime updateTime or {@code null} for none
*/
public GoogleCloudRunV2Service setUpdateTime(String updateTime) {
this.updateTime = updateTime;
return this;
}
/**
* Output only. The main URI in which this Service is serving traffic.
* @return value or {@code null} for none
*/
public java.lang.String getUri() {
return uri;
}
/**
* Output only. The main URI in which this Service is serving traffic.
* @param uri uri or {@code null} for none
*/
public GoogleCloudRunV2Service setUri(java.lang.String uri) {
this.uri = uri;
return this;
}
/**
* Output only. All URLs serving traffic for this Service.
* @return value or {@code null} for none
*/
public java.util.List getUrls() {
return urls;
}
/**
* Output only. All URLs serving traffic for this Service.
* @param urls urls or {@code null} for none
*/
public GoogleCloudRunV2Service setUrls(java.util.List urls) {
this.urls = urls;
return this;
}
@Override
public GoogleCloudRunV2Service set(String fieldName, Object value) {
return (GoogleCloudRunV2Service) super.set(fieldName, value);
}
@Override
public GoogleCloudRunV2Service clone() {
return (GoogleCloudRunV2Service) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy