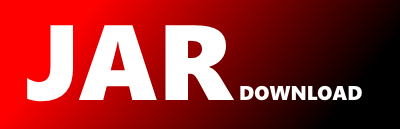
com.google.api.services.run.v2.model.GoogleCloudRunV2SubmitBuildRequest Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.run.v2.model;
/**
* Request message for submitting a Build.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Run Admin API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudRunV2SubmitBuildRequest extends com.google.api.client.json.GenericJson {
/**
* Build the source using Buildpacks.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRunV2BuildpacksBuild buildpackBuild;
/**
* Build the source using Docker. This means the source has a Dockerfile.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRunV2DockerBuild dockerBuild;
/**
* Required. Artifact Registry URI to store the built image.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String imageUri;
/**
* Optional. The service account to use for the build. If not set, the default Cloud Build service
* account for the project will be used.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String serviceAccount;
/**
* Required. Source for the build.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRunV2StorageSource storageSource;
/**
* Optional. Additional tags to annotate the build.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List tags;
/**
* Optional. Name of the Cloud Build Custom Worker Pool that should be used to build the function.
* The format of this field is `projects/{project}/locations/{region}/workerPools/{workerPool}`
* where `{project}` and `{region}` are the project id and region respectively where the worker
* pool is defined and `{workerPool}` is the short name of the worker pool.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String workerPool;
/**
* Build the source using Buildpacks.
* @return value or {@code null} for none
*/
public GoogleCloudRunV2BuildpacksBuild getBuildpackBuild() {
return buildpackBuild;
}
/**
* Build the source using Buildpacks.
* @param buildpackBuild buildpackBuild or {@code null} for none
*/
public GoogleCloudRunV2SubmitBuildRequest setBuildpackBuild(GoogleCloudRunV2BuildpacksBuild buildpackBuild) {
this.buildpackBuild = buildpackBuild;
return this;
}
/**
* Build the source using Docker. This means the source has a Dockerfile.
* @return value or {@code null} for none
*/
public GoogleCloudRunV2DockerBuild getDockerBuild() {
return dockerBuild;
}
/**
* Build the source using Docker. This means the source has a Dockerfile.
* @param dockerBuild dockerBuild or {@code null} for none
*/
public GoogleCloudRunV2SubmitBuildRequest setDockerBuild(GoogleCloudRunV2DockerBuild dockerBuild) {
this.dockerBuild = dockerBuild;
return this;
}
/**
* Required. Artifact Registry URI to store the built image.
* @return value or {@code null} for none
*/
public java.lang.String getImageUri() {
return imageUri;
}
/**
* Required. Artifact Registry URI to store the built image.
* @param imageUri imageUri or {@code null} for none
*/
public GoogleCloudRunV2SubmitBuildRequest setImageUri(java.lang.String imageUri) {
this.imageUri = imageUri;
return this;
}
/**
* Optional. The service account to use for the build. If not set, the default Cloud Build service
* account for the project will be used.
* @return value or {@code null} for none
*/
public java.lang.String getServiceAccount() {
return serviceAccount;
}
/**
* Optional. The service account to use for the build. If not set, the default Cloud Build service
* account for the project will be used.
* @param serviceAccount serviceAccount or {@code null} for none
*/
public GoogleCloudRunV2SubmitBuildRequest setServiceAccount(java.lang.String serviceAccount) {
this.serviceAccount = serviceAccount;
return this;
}
/**
* Required. Source for the build.
* @return value or {@code null} for none
*/
public GoogleCloudRunV2StorageSource getStorageSource() {
return storageSource;
}
/**
* Required. Source for the build.
* @param storageSource storageSource or {@code null} for none
*/
public GoogleCloudRunV2SubmitBuildRequest setStorageSource(GoogleCloudRunV2StorageSource storageSource) {
this.storageSource = storageSource;
return this;
}
/**
* Optional. Additional tags to annotate the build.
* @return value or {@code null} for none
*/
public java.util.List getTags() {
return tags;
}
/**
* Optional. Additional tags to annotate the build.
* @param tags tags or {@code null} for none
*/
public GoogleCloudRunV2SubmitBuildRequest setTags(java.util.List tags) {
this.tags = tags;
return this;
}
/**
* Optional. Name of the Cloud Build Custom Worker Pool that should be used to build the function.
* The format of this field is `projects/{project}/locations/{region}/workerPools/{workerPool}`
* where `{project}` and `{region}` are the project id and region respectively where the worker
* pool is defined and `{workerPool}` is the short name of the worker pool.
* @return value or {@code null} for none
*/
public java.lang.String getWorkerPool() {
return workerPool;
}
/**
* Optional. Name of the Cloud Build Custom Worker Pool that should be used to build the function.
* The format of this field is `projects/{project}/locations/{region}/workerPools/{workerPool}`
* where `{project}` and `{region}` are the project id and region respectively where the worker
* pool is defined and `{workerPool}` is the short name of the worker pool.
* @param workerPool workerPool or {@code null} for none
*/
public GoogleCloudRunV2SubmitBuildRequest setWorkerPool(java.lang.String workerPool) {
this.workerPool = workerPool;
return this;
}
@Override
public GoogleCloudRunV2SubmitBuildRequest set(String fieldName, Object value) {
return (GoogleCloudRunV2SubmitBuildRequest) super.set(fieldName, value);
}
@Override
public GoogleCloudRunV2SubmitBuildRequest clone() {
return (GoogleCloudRunV2SubmitBuildRequest) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy