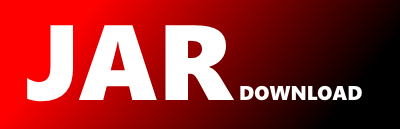
com.google.api.services.run.v2.model.GoogleDevtoolsCloudbuildV1Results Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.run.v2.model;
/**
* Artifacts created by the build pipeline.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Run Admin API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleDevtoolsCloudbuildV1Results extends com.google.api.client.json.GenericJson {
/**
* Path to the artifact manifest for non-container artifacts uploaded to Cloud Storage. Only
* populated when artifacts are uploaded to Cloud Storage.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String artifactManifest;
/**
* Time to push all non-container artifacts to Cloud Storage.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleDevtoolsCloudbuildV1TimeSpan artifactTiming;
/**
* List of build step digests, in the order corresponding to build step indices.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List buildStepImages;
/**
* List of build step outputs, produced by builder images, in the order corresponding to build
* step indices. [Cloud Builders](https://cloud.google.com/cloud-build/docs/cloud-builders) can
* produce this output by writing to `$BUILDER_OUTPUT/output`. Only the first 50KB of data is
* stored. Note that the `$BUILDER_OUTPUT` variable is read-only and can't be substituted.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List buildStepOutputs;
/**
* Container images that were built as a part of the build.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List images;
static {
// hack to force ProGuard to consider GoogleDevtoolsCloudbuildV1BuiltImage used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleDevtoolsCloudbuildV1BuiltImage.class);
}
/**
* Maven artifacts uploaded to Artifact Registry at the end of the build.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List mavenArtifacts;
/**
* Npm packages uploaded to Artifact Registry at the end of the build.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List npmPackages;
/**
* Number of non-container artifacts uploaded to Cloud Storage. Only populated when artifacts are
* uploaded to Cloud Storage.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long numArtifacts;
/**
* Python artifacts uploaded to Artifact Registry at the end of the build.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List pythonPackages;
/**
* Path to the artifact manifest for non-container artifacts uploaded to Cloud Storage. Only
* populated when artifacts are uploaded to Cloud Storage.
* @return value or {@code null} for none
*/
public java.lang.String getArtifactManifest() {
return artifactManifest;
}
/**
* Path to the artifact manifest for non-container artifacts uploaded to Cloud Storage. Only
* populated when artifacts are uploaded to Cloud Storage.
* @param artifactManifest artifactManifest or {@code null} for none
*/
public GoogleDevtoolsCloudbuildV1Results setArtifactManifest(java.lang.String artifactManifest) {
this.artifactManifest = artifactManifest;
return this;
}
/**
* Time to push all non-container artifacts to Cloud Storage.
* @return value or {@code null} for none
*/
public GoogleDevtoolsCloudbuildV1TimeSpan getArtifactTiming() {
return artifactTiming;
}
/**
* Time to push all non-container artifacts to Cloud Storage.
* @param artifactTiming artifactTiming or {@code null} for none
*/
public GoogleDevtoolsCloudbuildV1Results setArtifactTiming(GoogleDevtoolsCloudbuildV1TimeSpan artifactTiming) {
this.artifactTiming = artifactTiming;
return this;
}
/**
* List of build step digests, in the order corresponding to build step indices.
* @return value or {@code null} for none
*/
public java.util.List getBuildStepImages() {
return buildStepImages;
}
/**
* List of build step digests, in the order corresponding to build step indices.
* @param buildStepImages buildStepImages or {@code null} for none
*/
public GoogleDevtoolsCloudbuildV1Results setBuildStepImages(java.util.List buildStepImages) {
this.buildStepImages = buildStepImages;
return this;
}
/**
* List of build step outputs, produced by builder images, in the order corresponding to build
* step indices. [Cloud Builders](https://cloud.google.com/cloud-build/docs/cloud-builders) can
* produce this output by writing to `$BUILDER_OUTPUT/output`. Only the first 50KB of data is
* stored. Note that the `$BUILDER_OUTPUT` variable is read-only and can't be substituted.
* @return value or {@code null} for none
*/
public java.util.List getBuildStepOutputs() {
return buildStepOutputs;
}
/**
* List of build step outputs, produced by builder images, in the order corresponding to build
* step indices. [Cloud Builders](https://cloud.google.com/cloud-build/docs/cloud-builders) can
* produce this output by writing to `$BUILDER_OUTPUT/output`. Only the first 50KB of data is
* stored. Note that the `$BUILDER_OUTPUT` variable is read-only and can't be substituted.
* @param buildStepOutputs buildStepOutputs or {@code null} for none
*/
public GoogleDevtoolsCloudbuildV1Results setBuildStepOutputs(java.util.List buildStepOutputs) {
this.buildStepOutputs = buildStepOutputs;
return this;
}
/**
* Container images that were built as a part of the build.
* @return value or {@code null} for none
*/
public java.util.List getImages() {
return images;
}
/**
* Container images that were built as a part of the build.
* @param images images or {@code null} for none
*/
public GoogleDevtoolsCloudbuildV1Results setImages(java.util.List images) {
this.images = images;
return this;
}
/**
* Maven artifacts uploaded to Artifact Registry at the end of the build.
* @return value or {@code null} for none
*/
public java.util.List getMavenArtifacts() {
return mavenArtifacts;
}
/**
* Maven artifacts uploaded to Artifact Registry at the end of the build.
* @param mavenArtifacts mavenArtifacts or {@code null} for none
*/
public GoogleDevtoolsCloudbuildV1Results setMavenArtifacts(java.util.List mavenArtifacts) {
this.mavenArtifacts = mavenArtifacts;
return this;
}
/**
* Npm packages uploaded to Artifact Registry at the end of the build.
* @return value or {@code null} for none
*/
public java.util.List getNpmPackages() {
return npmPackages;
}
/**
* Npm packages uploaded to Artifact Registry at the end of the build.
* @param npmPackages npmPackages or {@code null} for none
*/
public GoogleDevtoolsCloudbuildV1Results setNpmPackages(java.util.List npmPackages) {
this.npmPackages = npmPackages;
return this;
}
/**
* Number of non-container artifacts uploaded to Cloud Storage. Only populated when artifacts are
* uploaded to Cloud Storage.
* @return value or {@code null} for none
*/
public java.lang.Long getNumArtifacts() {
return numArtifacts;
}
/**
* Number of non-container artifacts uploaded to Cloud Storage. Only populated when artifacts are
* uploaded to Cloud Storage.
* @param numArtifacts numArtifacts or {@code null} for none
*/
public GoogleDevtoolsCloudbuildV1Results setNumArtifacts(java.lang.Long numArtifacts) {
this.numArtifacts = numArtifacts;
return this;
}
/**
* Python artifacts uploaded to Artifact Registry at the end of the build.
* @return value or {@code null} for none
*/
public java.util.List getPythonPackages() {
return pythonPackages;
}
/**
* Python artifacts uploaded to Artifact Registry at the end of the build.
* @param pythonPackages pythonPackages or {@code null} for none
*/
public GoogleDevtoolsCloudbuildV1Results setPythonPackages(java.util.List pythonPackages) {
this.pythonPackages = pythonPackages;
return this;
}
@Override
public GoogleDevtoolsCloudbuildV1Results set(String fieldName, Object value) {
return (GoogleDevtoolsCloudbuildV1Results) super.set(fieldName, value);
}
@Override
public GoogleDevtoolsCloudbuildV1Results clone() {
return (GoogleDevtoolsCloudbuildV1Results) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy