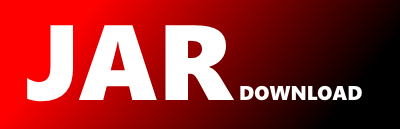
com.google.api.services.run.v2.model.UtilStatusProto Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.run.v2.model;
/**
* Wire-format for a Status object
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Run Admin API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class UtilStatusProto extends com.google.api.client.json.GenericJson {
/**
* The canonical error code (see codes.proto) that most closely corresponds to this status. This
* may be missing, and in the common case of the generic space, it definitely will be.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer canonicalCode;
/**
* Numeric code drawn from the space specified below. Often, this is the canonical error space,
* and code is drawn from google3/util/task/codes.proto
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer code;
/**
* Detail message
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String message;
/**
* message_set associates an arbitrary proto message with the status.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Proto2BridgeMessageSet messageSet;
/**
* The following are usually only present when code != 0 Space to which this status belongs
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String space;
/**
* The canonical error code (see codes.proto) that most closely corresponds to this status. This
* may be missing, and in the common case of the generic space, it definitely will be.
* @return value or {@code null} for none
*/
public java.lang.Integer getCanonicalCode() {
return canonicalCode;
}
/**
* The canonical error code (see codes.proto) that most closely corresponds to this status. This
* may be missing, and in the common case of the generic space, it definitely will be.
* @param canonicalCode canonicalCode or {@code null} for none
*/
public UtilStatusProto setCanonicalCode(java.lang.Integer canonicalCode) {
this.canonicalCode = canonicalCode;
return this;
}
/**
* Numeric code drawn from the space specified below. Often, this is the canonical error space,
* and code is drawn from google3/util/task/codes.proto
* @return value or {@code null} for none
*/
public java.lang.Integer getCode() {
return code;
}
/**
* Numeric code drawn from the space specified below. Often, this is the canonical error space,
* and code is drawn from google3/util/task/codes.proto
* @param code code or {@code null} for none
*/
public UtilStatusProto setCode(java.lang.Integer code) {
this.code = code;
return this;
}
/**
* Detail message
* @return value or {@code null} for none
*/
public java.lang.String getMessage() {
return message;
}
/**
* Detail message
* @param message message or {@code null} for none
*/
public UtilStatusProto setMessage(java.lang.String message) {
this.message = message;
return this;
}
/**
* message_set associates an arbitrary proto message with the status.
* @return value or {@code null} for none
*/
public Proto2BridgeMessageSet getMessageSet() {
return messageSet;
}
/**
* message_set associates an arbitrary proto message with the status.
* @param messageSet messageSet or {@code null} for none
*/
public UtilStatusProto setMessageSet(Proto2BridgeMessageSet messageSet) {
this.messageSet = messageSet;
return this;
}
/**
* The following are usually only present when code != 0 Space to which this status belongs
* @return value or {@code null} for none
*/
public java.lang.String getSpace() {
return space;
}
/**
* The following are usually only present when code != 0 Space to which this status belongs
* @param space space or {@code null} for none
*/
public UtilStatusProto setSpace(java.lang.String space) {
this.space = space;
return this;
}
@Override
public UtilStatusProto set(String fieldName, Object value) {
return (UtilStatusProto) super.set(fieldName, value);
}
@Override
public UtilStatusProto clone() {
return (UtilStatusProto) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy