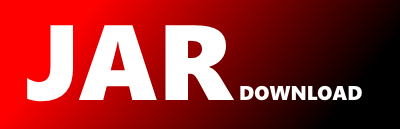
com.google.api.services.safebrowsing.v5.model.GoogleSecuritySafebrowsingV5SearchHashesResponse Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.safebrowsing.v5.model;
/**
* The response returned after searching threat hashes. If nothing is found, the server will return
* an OK status (HTTP status code 200) with the `full_hashes` field empty, rather than returning a
* NOT_FOUND status (HTTP status code 404). **What's new in V5**: There is a separation between
* `FullHash` and `FullHashDetail`. In the case when a hash represents a site having multiple
* threats (e.g. both MALWARE and SOCIAL_ENGINEERING), the full hash does not need to be sent twice
* as in V4. Furthermore, the cache duration has been simplified into a single `cache_duration`
* field.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Safe Browsing API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleSecuritySafebrowsingV5SearchHashesResponse extends com.google.api.client.json.GenericJson {
/**
* The client-side cache duration. The client MUST add this duration to the current time to
* determine the expiration time. The expiration time then applies to every hash prefix queried by
* the client in the request, regardless of how many full hashes are returned in the response.
* Even if the server returns no full hashes for a particular hash prefix, this fact MUST also be
* cached by the client. Important: the client MUST NOT assume that the server will return the
* same cache duration for all responses. The server MAY choose different cache durations for
* different responses depending on the situation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String cacheDuration;
/**
* Unordered list. The unordered list of full hashes found.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List fullHashes;
static {
// hack to force ProGuard to consider GoogleSecuritySafebrowsingV5FullHash used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleSecuritySafebrowsingV5FullHash.class);
}
/**
* The client-side cache duration. The client MUST add this duration to the current time to
* determine the expiration time. The expiration time then applies to every hash prefix queried by
* the client in the request, regardless of how many full hashes are returned in the response.
* Even if the server returns no full hashes for a particular hash prefix, this fact MUST also be
* cached by the client. Important: the client MUST NOT assume that the server will return the
* same cache duration for all responses. The server MAY choose different cache durations for
* different responses depending on the situation.
* @return value or {@code null} for none
*/
public String getCacheDuration() {
return cacheDuration;
}
/**
* The client-side cache duration. The client MUST add this duration to the current time to
* determine the expiration time. The expiration time then applies to every hash prefix queried by
* the client in the request, regardless of how many full hashes are returned in the response.
* Even if the server returns no full hashes for a particular hash prefix, this fact MUST also be
* cached by the client. Important: the client MUST NOT assume that the server will return the
* same cache duration for all responses. The server MAY choose different cache durations for
* different responses depending on the situation.
* @param cacheDuration cacheDuration or {@code null} for none
*/
public GoogleSecuritySafebrowsingV5SearchHashesResponse setCacheDuration(String cacheDuration) {
this.cacheDuration = cacheDuration;
return this;
}
/**
* Unordered list. The unordered list of full hashes found.
* @return value or {@code null} for none
*/
public java.util.List getFullHashes() {
return fullHashes;
}
/**
* Unordered list. The unordered list of full hashes found.
* @param fullHashes fullHashes or {@code null} for none
*/
public GoogleSecuritySafebrowsingV5SearchHashesResponse setFullHashes(java.util.List fullHashes) {
this.fullHashes = fullHashes;
return this;
}
@Override
public GoogleSecuritySafebrowsingV5SearchHashesResponse set(String fieldName, Object value) {
return (GoogleSecuritySafebrowsingV5SearchHashesResponse) super.set(fieldName, value);
}
@Override
public GoogleSecuritySafebrowsingV5SearchHashesResponse clone() {
return (GoogleSecuritySafebrowsingV5SearchHashesResponse) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy