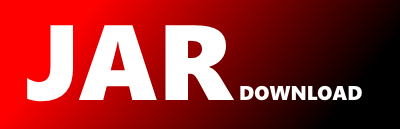
com.google.api.services.securitycenter.v1.model.Asset Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.securitycenter.v1.model;
/**
* Security Command Center representation of a Google Cloud resource. The Asset is a Security
* Command Center resource that captures information about a single Google Cloud resource. All
* modifications to an Asset are only within the context of Security Command Center and don't affect
* the referenced Google Cloud resource.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Security Command Center API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Asset extends com.google.api.client.json.GenericJson {
/**
* The canonical name of the resource. It's either
* "organizations/{organization_id}/assets/{asset_id}", "folders/{folder_id}/assets/{asset_id}" or
* "projects/{project_number}/assets/{asset_id}", depending on the closest CRM ancestor of the
* resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String canonicalName;
/**
* The time at which the asset was created in Security Command Center.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String createTime;
/**
* Cloud IAM Policy information associated with the Google Cloud resource described by the
* Security Command Center asset. This information is managed and defined by the Google Cloud
* resource and cannot be modified by the user.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private IamPolicy iamPolicy;
/**
* The relative resource name of this asset. See:
* https://cloud.google.com/apis/design/resource_names#relative_resource_name Example:
* "organizations/{organization_id}/assets/{asset_id}".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Resource managed properties. These properties are managed and defined by the Google Cloud
* resource and cannot be modified by the user.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map resourceProperties;
/**
* Security Command Center managed properties. These properties are managed by Security Command
* Center and cannot be modified by the user.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SecurityCenterProperties securityCenterProperties;
/**
* User specified security marks. These marks are entirely managed by the user and come from the
* SecurityMarks resource that belongs to the asset.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SecurityMarks securityMarks;
/**
* The time at which the asset was last updated or added in Cloud SCC.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String updateTime;
/**
* The canonical name of the resource. It's either
* "organizations/{organization_id}/assets/{asset_id}", "folders/{folder_id}/assets/{asset_id}" or
* "projects/{project_number}/assets/{asset_id}", depending on the closest CRM ancestor of the
* resource.
* @return value or {@code null} for none
*/
public java.lang.String getCanonicalName() {
return canonicalName;
}
/**
* The canonical name of the resource. It's either
* "organizations/{organization_id}/assets/{asset_id}", "folders/{folder_id}/assets/{asset_id}" or
* "projects/{project_number}/assets/{asset_id}", depending on the closest CRM ancestor of the
* resource.
* @param canonicalName canonicalName or {@code null} for none
*/
public Asset setCanonicalName(java.lang.String canonicalName) {
this.canonicalName = canonicalName;
return this;
}
/**
* The time at which the asset was created in Security Command Center.
* @return value or {@code null} for none
*/
public String getCreateTime() {
return createTime;
}
/**
* The time at which the asset was created in Security Command Center.
* @param createTime createTime or {@code null} for none
*/
public Asset setCreateTime(String createTime) {
this.createTime = createTime;
return this;
}
/**
* Cloud IAM Policy information associated with the Google Cloud resource described by the
* Security Command Center asset. This information is managed and defined by the Google Cloud
* resource and cannot be modified by the user.
* @return value or {@code null} for none
*/
public IamPolicy getIamPolicy() {
return iamPolicy;
}
/**
* Cloud IAM Policy information associated with the Google Cloud resource described by the
* Security Command Center asset. This information is managed and defined by the Google Cloud
* resource and cannot be modified by the user.
* @param iamPolicy iamPolicy or {@code null} for none
*/
public Asset setIamPolicy(IamPolicy iamPolicy) {
this.iamPolicy = iamPolicy;
return this;
}
/**
* The relative resource name of this asset. See:
* https://cloud.google.com/apis/design/resource_names#relative_resource_name Example:
* "organizations/{organization_id}/assets/{asset_id}".
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* The relative resource name of this asset. See:
* https://cloud.google.com/apis/design/resource_names#relative_resource_name Example:
* "organizations/{organization_id}/assets/{asset_id}".
* @param name name or {@code null} for none
*/
public Asset setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Resource managed properties. These properties are managed and defined by the Google Cloud
* resource and cannot be modified by the user.
* @return value or {@code null} for none
*/
public java.util.Map getResourceProperties() {
return resourceProperties;
}
/**
* Resource managed properties. These properties are managed and defined by the Google Cloud
* resource and cannot be modified by the user.
* @param resourceProperties resourceProperties or {@code null} for none
*/
public Asset setResourceProperties(java.util.Map resourceProperties) {
this.resourceProperties = resourceProperties;
return this;
}
/**
* Security Command Center managed properties. These properties are managed by Security Command
* Center and cannot be modified by the user.
* @return value or {@code null} for none
*/
public SecurityCenterProperties getSecurityCenterProperties() {
return securityCenterProperties;
}
/**
* Security Command Center managed properties. These properties are managed by Security Command
* Center and cannot be modified by the user.
* @param securityCenterProperties securityCenterProperties or {@code null} for none
*/
public Asset setSecurityCenterProperties(SecurityCenterProperties securityCenterProperties) {
this.securityCenterProperties = securityCenterProperties;
return this;
}
/**
* User specified security marks. These marks are entirely managed by the user and come from the
* SecurityMarks resource that belongs to the asset.
* @return value or {@code null} for none
*/
public SecurityMarks getSecurityMarks() {
return securityMarks;
}
/**
* User specified security marks. These marks are entirely managed by the user and come from the
* SecurityMarks resource that belongs to the asset.
* @param securityMarks securityMarks or {@code null} for none
*/
public Asset setSecurityMarks(SecurityMarks securityMarks) {
this.securityMarks = securityMarks;
return this;
}
/**
* The time at which the asset was last updated or added in Cloud SCC.
* @return value or {@code null} for none
*/
public String getUpdateTime() {
return updateTime;
}
/**
* The time at which the asset was last updated or added in Cloud SCC.
* @param updateTime updateTime or {@code null} for none
*/
public Asset setUpdateTime(String updateTime) {
this.updateTime = updateTime;
return this;
}
@Override
public Asset set(String fieldName, Object value) {
return (Asset) super.set(fieldName, value);
}
@Override
public Asset clone() {
return (Asset) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy