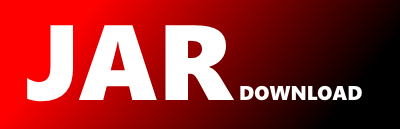
com.google.api.services.securitycenter.v1.model.Database Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.securitycenter.v1.model;
/**
* Represents database access information, such as queries. A database may be a sub-resource of an
* instance (as in the case of Cloud SQL instances or Cloud Spanner instances), or the database
* instance itself. Some database resources might not have the [full resource
* name](https://google.aip.dev/122#full-resource-names) populated because these resource types,
* such as Cloud SQL databases, are not yet supported by Cloud Asset Inventory. In these cases only
* the display name is provided.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Security Command Center API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Database extends com.google.api.client.json.GenericJson {
/**
* The human-readable name of the database that the user connected to.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String displayName;
/**
* The target usernames, roles, or groups of an SQL privilege grant, which is not an IAM policy
* change.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List grantees;
/**
* Some database resources may not have the [full resource name](https://google.aip.dev/122#full-
* resource-names) populated because these resource types are not yet supported by Cloud Asset
* Inventory (e.g. Cloud SQL databases). In these cases only the display name will be provided.
* The [full resource name](https://google.aip.dev/122#full-resource-names) of the database that
* the user connected to, if it is supported by Cloud Asset Inventory.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* The SQL statement that is associated with the database access.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String query;
/**
* The username used to connect to the database. The username might not be an IAM principal and
* does not have a set format.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String userName;
/**
* The version of the database, for example, POSTGRES_14. See [the complete
* list](https://cloud.google.com/sql/docs/mysql/admin-api/rest/v1/SqlDatabaseVersion).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String version;
/**
* The human-readable name of the database that the user connected to.
* @return value or {@code null} for none
*/
public java.lang.String getDisplayName() {
return displayName;
}
/**
* The human-readable name of the database that the user connected to.
* @param displayName displayName or {@code null} for none
*/
public Database setDisplayName(java.lang.String displayName) {
this.displayName = displayName;
return this;
}
/**
* The target usernames, roles, or groups of an SQL privilege grant, which is not an IAM policy
* change.
* @return value or {@code null} for none
*/
public java.util.List getGrantees() {
return grantees;
}
/**
* The target usernames, roles, or groups of an SQL privilege grant, which is not an IAM policy
* change.
* @param grantees grantees or {@code null} for none
*/
public Database setGrantees(java.util.List grantees) {
this.grantees = grantees;
return this;
}
/**
* Some database resources may not have the [full resource name](https://google.aip.dev/122#full-
* resource-names) populated because these resource types are not yet supported by Cloud Asset
* Inventory (e.g. Cloud SQL databases). In these cases only the display name will be provided.
* The [full resource name](https://google.aip.dev/122#full-resource-names) of the database that
* the user connected to, if it is supported by Cloud Asset Inventory.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Some database resources may not have the [full resource name](https://google.aip.dev/122#full-
* resource-names) populated because these resource types are not yet supported by Cloud Asset
* Inventory (e.g. Cloud SQL databases). In these cases only the display name will be provided.
* The [full resource name](https://google.aip.dev/122#full-resource-names) of the database that
* the user connected to, if it is supported by Cloud Asset Inventory.
* @param name name or {@code null} for none
*/
public Database setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* The SQL statement that is associated with the database access.
* @return value or {@code null} for none
*/
public java.lang.String getQuery() {
return query;
}
/**
* The SQL statement that is associated with the database access.
* @param query query or {@code null} for none
*/
public Database setQuery(java.lang.String query) {
this.query = query;
return this;
}
/**
* The username used to connect to the database. The username might not be an IAM principal and
* does not have a set format.
* @return value or {@code null} for none
*/
public java.lang.String getUserName() {
return userName;
}
/**
* The username used to connect to the database. The username might not be an IAM principal and
* does not have a set format.
* @param userName userName or {@code null} for none
*/
public Database setUserName(java.lang.String userName) {
this.userName = userName;
return this;
}
/**
* The version of the database, for example, POSTGRES_14. See [the complete
* list](https://cloud.google.com/sql/docs/mysql/admin-api/rest/v1/SqlDatabaseVersion).
* @return value or {@code null} for none
*/
public java.lang.String getVersion() {
return version;
}
/**
* The version of the database, for example, POSTGRES_14. See [the complete
* list](https://cloud.google.com/sql/docs/mysql/admin-api/rest/v1/SqlDatabaseVersion).
* @param version version or {@code null} for none
*/
public Database setVersion(java.lang.String version) {
this.version = version;
return this;
}
@Override
public Database set(String fieldName, Object value) {
return (Database) super.set(fieldName, value);
}
@Override
public Database clone() {
return (Database) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy