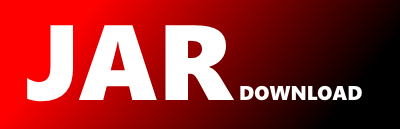
com.google.api.services.securitycenter.v1.model.File Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.securitycenter.v1.model;
/**
* File information about the related binary/library used by an executable, or the script used by a
* script interpreter
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Security Command Center API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class File extends com.google.api.client.json.GenericJson {
/**
* Prefix of the file contents as a JSON-encoded string.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String contents;
/**
* Path of the file in terms of underlying disk/partition identifiers.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DiskPath diskPath;
/**
* The length in bytes of the file prefix that was hashed. If hashed_size == size, any hashes
* reported represent the entire file.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long hashedSize;
/**
* True when the hash covers only a prefix of the file.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean partiallyHashed;
/**
* Absolute path of the file as a JSON encoded string.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String path;
/**
* SHA256 hash of the first hashed_size bytes of the file encoded as a hex string. If hashed_size
* == size, sha256 represents the SHA256 hash of the entire file.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String sha256;
/**
* Size of the file in bytes.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long size;
/**
* Prefix of the file contents as a JSON-encoded string.
* @return value or {@code null} for none
*/
public java.lang.String getContents() {
return contents;
}
/**
* Prefix of the file contents as a JSON-encoded string.
* @param contents contents or {@code null} for none
*/
public File setContents(java.lang.String contents) {
this.contents = contents;
return this;
}
/**
* Path of the file in terms of underlying disk/partition identifiers.
* @return value or {@code null} for none
*/
public DiskPath getDiskPath() {
return diskPath;
}
/**
* Path of the file in terms of underlying disk/partition identifiers.
* @param diskPath diskPath or {@code null} for none
*/
public File setDiskPath(DiskPath diskPath) {
this.diskPath = diskPath;
return this;
}
/**
* The length in bytes of the file prefix that was hashed. If hashed_size == size, any hashes
* reported represent the entire file.
* @return value or {@code null} for none
*/
public java.lang.Long getHashedSize() {
return hashedSize;
}
/**
* The length in bytes of the file prefix that was hashed. If hashed_size == size, any hashes
* reported represent the entire file.
* @param hashedSize hashedSize or {@code null} for none
*/
public File setHashedSize(java.lang.Long hashedSize) {
this.hashedSize = hashedSize;
return this;
}
/**
* True when the hash covers only a prefix of the file.
* @return value or {@code null} for none
*/
public java.lang.Boolean getPartiallyHashed() {
return partiallyHashed;
}
/**
* True when the hash covers only a prefix of the file.
* @param partiallyHashed partiallyHashed or {@code null} for none
*/
public File setPartiallyHashed(java.lang.Boolean partiallyHashed) {
this.partiallyHashed = partiallyHashed;
return this;
}
/**
* Absolute path of the file as a JSON encoded string.
* @return value or {@code null} for none
*/
public java.lang.String getPath() {
return path;
}
/**
* Absolute path of the file as a JSON encoded string.
* @param path path or {@code null} for none
*/
public File setPath(java.lang.String path) {
this.path = path;
return this;
}
/**
* SHA256 hash of the first hashed_size bytes of the file encoded as a hex string. If hashed_size
* == size, sha256 represents the SHA256 hash of the entire file.
* @return value or {@code null} for none
*/
public java.lang.String getSha256() {
return sha256;
}
/**
* SHA256 hash of the first hashed_size bytes of the file encoded as a hex string. If hashed_size
* == size, sha256 represents the SHA256 hash of the entire file.
* @param sha256 sha256 or {@code null} for none
*/
public File setSha256(java.lang.String sha256) {
this.sha256 = sha256;
return this;
}
/**
* Size of the file in bytes.
* @return value or {@code null} for none
*/
public java.lang.Long getSize() {
return size;
}
/**
* Size of the file in bytes.
* @param size size or {@code null} for none
*/
public File setSize(java.lang.Long size) {
this.size = size;
return this;
}
@Override
public File set(String fieldName, Object value) {
return (File) super.set(fieldName, value);
}
@Override
public File clone() {
return (File) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy