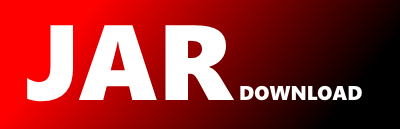
com.google.api.services.securitycenter.v1.model.GoogleCloudSecuritycenterV1CustomConfig Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.securitycenter.v1.model;
/**
* Defines the properties in a custom module configuration for Security Health Analytics. Use the
* custom module configuration to create custom detectors that generate custom findings for
* resources that you specify.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Security Command Center API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudSecuritycenterV1CustomConfig extends com.google.api.client.json.GenericJson {
/**
* The CEL policy spec attached to the custom module.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private CelPolicySpec celPolicy;
/**
* Custom output properties.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudSecuritycenterV1CustomOutputSpec customOutput;
/**
* Text that describes the vulnerability or misconfiguration that the custom module detects. This
* explanation is returned with each finding instance to help investigators understand the
* detected issue. The text must be enclosed in quotation marks.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* The CEL expression to evaluate to produce findings. When the expression evaluates to true
* against a resource, a finding is generated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Expr predicate;
/**
* An explanation of the recommended steps that security teams can take to resolve the detected
* issue. This explanation is returned with each finding generated by this module in the
* `nextSteps` property of the finding JSON.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String recommendation;
/**
* The resource types that the custom module operates on. Each custom module can specify up to 5
* resource types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudSecuritycenterV1ResourceSelector resourceSelector;
/**
* The severity to assign to findings generated by the module.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String severity;
/**
* The CEL policy spec attached to the custom module.
* @return value or {@code null} for none
*/
public CelPolicySpec getCelPolicy() {
return celPolicy;
}
/**
* The CEL policy spec attached to the custom module.
* @param celPolicy celPolicy or {@code null} for none
*/
public GoogleCloudSecuritycenterV1CustomConfig setCelPolicy(CelPolicySpec celPolicy) {
this.celPolicy = celPolicy;
return this;
}
/**
* Custom output properties.
* @return value or {@code null} for none
*/
public GoogleCloudSecuritycenterV1CustomOutputSpec getCustomOutput() {
return customOutput;
}
/**
* Custom output properties.
* @param customOutput customOutput or {@code null} for none
*/
public GoogleCloudSecuritycenterV1CustomConfig setCustomOutput(GoogleCloudSecuritycenterV1CustomOutputSpec customOutput) {
this.customOutput = customOutput;
return this;
}
/**
* Text that describes the vulnerability or misconfiguration that the custom module detects. This
* explanation is returned with each finding instance to help investigators understand the
* detected issue. The text must be enclosed in quotation marks.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* Text that describes the vulnerability or misconfiguration that the custom module detects. This
* explanation is returned with each finding instance to help investigators understand the
* detected issue. The text must be enclosed in quotation marks.
* @param description description or {@code null} for none
*/
public GoogleCloudSecuritycenterV1CustomConfig setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* The CEL expression to evaluate to produce findings. When the expression evaluates to true
* against a resource, a finding is generated.
* @return value or {@code null} for none
*/
public Expr getPredicate() {
return predicate;
}
/**
* The CEL expression to evaluate to produce findings. When the expression evaluates to true
* against a resource, a finding is generated.
* @param predicate predicate or {@code null} for none
*/
public GoogleCloudSecuritycenterV1CustomConfig setPredicate(Expr predicate) {
this.predicate = predicate;
return this;
}
/**
* An explanation of the recommended steps that security teams can take to resolve the detected
* issue. This explanation is returned with each finding generated by this module in the
* `nextSteps` property of the finding JSON.
* @return value or {@code null} for none
*/
public java.lang.String getRecommendation() {
return recommendation;
}
/**
* An explanation of the recommended steps that security teams can take to resolve the detected
* issue. This explanation is returned with each finding generated by this module in the
* `nextSteps` property of the finding JSON.
* @param recommendation recommendation or {@code null} for none
*/
public GoogleCloudSecuritycenterV1CustomConfig setRecommendation(java.lang.String recommendation) {
this.recommendation = recommendation;
return this;
}
/**
* The resource types that the custom module operates on. Each custom module can specify up to 5
* resource types.
* @return value or {@code null} for none
*/
public GoogleCloudSecuritycenterV1ResourceSelector getResourceSelector() {
return resourceSelector;
}
/**
* The resource types that the custom module operates on. Each custom module can specify up to 5
* resource types.
* @param resourceSelector resourceSelector or {@code null} for none
*/
public GoogleCloudSecuritycenterV1CustomConfig setResourceSelector(GoogleCloudSecuritycenterV1ResourceSelector resourceSelector) {
this.resourceSelector = resourceSelector;
return this;
}
/**
* The severity to assign to findings generated by the module.
* @return value or {@code null} for none
*/
public java.lang.String getSeverity() {
return severity;
}
/**
* The severity to assign to findings generated by the module.
* @param severity severity or {@code null} for none
*/
public GoogleCloudSecuritycenterV1CustomConfig setSeverity(java.lang.String severity) {
this.severity = severity;
return this;
}
@Override
public GoogleCloudSecuritycenterV1CustomConfig set(String fieldName, Object value) {
return (GoogleCloudSecuritycenterV1CustomConfig) super.set(fieldName, value);
}
@Override
public GoogleCloudSecuritycenterV1CustomConfig clone() {
return (GoogleCloudSecuritycenterV1CustomConfig) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy