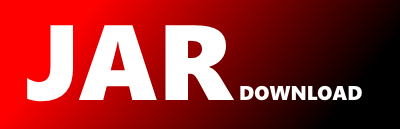
com.google.api.services.securitycenter.v1.model.GoogleCloudSecuritycenterV1Resource Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.securitycenter.v1.model;
/**
* Information related to the Google Cloud resource.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Security Command Center API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudSecuritycenterV1Resource extends com.google.api.client.json.GenericJson {
/**
* The AWS metadata associated with the finding.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AwsMetadata awsMetadata;
/**
* The Azure metadata associated with the finding.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AzureMetadata azureMetadata;
/**
* Indicates which cloud provider the resource resides in.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String cloudProvider;
/**
* The human readable name of the resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String displayName;
/**
* Output only. Contains a Folder message for each folder in the assets ancestry. The first folder
* is the deepest nested folder, and the last folder is the folder directly under the
* Organization.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List folders;
static {
// hack to force ProGuard to consider Folder used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(Folder.class);
}
/**
* The region or location of the service (if applicable).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String location;
/**
* The full resource name of the resource. See:
* https://cloud.google.com/apis/design/resource_names#full_resource_name
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Indicates which organization or tenant in the cloud provider the finding applies to.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String organization;
/**
* The full resource name of resource's parent.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/**
* The human readable name of resource's parent.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String parentDisplayName;
/**
* The full resource name of project that the resource belongs to.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String project;
/**
* The project ID that the resource belongs to.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String projectDisplayName;
/**
* Provides the path to the resource within the resource hierarchy.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ResourcePath resourcePath;
/**
* A string representation of the resource path. For Google Cloud, it has the format of
* `organizations/{organization_id}/folders/{folder_id}/folders/{folder_id}/projects/{project_id}`
* where there can be any number of folders. For AWS, it has the format of `org/{organization_id}/
* ou/{organizational_unit_id}/ou/{organizational_unit_id}/account/{account_id}` where there can
* be any number of organizational units. For Azure, it has the format of `mg/{management_group_id
* }/mg/{management_group_id}/subscription/{subscription_id}/rg/{resource_group_name}` where there
* can be any number of management groups.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String resourcePathString;
/**
* The parent service or product from which the resource is provided, for example, GKE or SNS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String service;
/**
* The full resource type of the resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* The AWS metadata associated with the finding.
* @return value or {@code null} for none
*/
public AwsMetadata getAwsMetadata() {
return awsMetadata;
}
/**
* The AWS metadata associated with the finding.
* @param awsMetadata awsMetadata or {@code null} for none
*/
public GoogleCloudSecuritycenterV1Resource setAwsMetadata(AwsMetadata awsMetadata) {
this.awsMetadata = awsMetadata;
return this;
}
/**
* The Azure metadata associated with the finding.
* @return value or {@code null} for none
*/
public AzureMetadata getAzureMetadata() {
return azureMetadata;
}
/**
* The Azure metadata associated with the finding.
* @param azureMetadata azureMetadata or {@code null} for none
*/
public GoogleCloudSecuritycenterV1Resource setAzureMetadata(AzureMetadata azureMetadata) {
this.azureMetadata = azureMetadata;
return this;
}
/**
* Indicates which cloud provider the resource resides in.
* @return value or {@code null} for none
*/
public java.lang.String getCloudProvider() {
return cloudProvider;
}
/**
* Indicates which cloud provider the resource resides in.
* @param cloudProvider cloudProvider or {@code null} for none
*/
public GoogleCloudSecuritycenterV1Resource setCloudProvider(java.lang.String cloudProvider) {
this.cloudProvider = cloudProvider;
return this;
}
/**
* The human readable name of the resource.
* @return value or {@code null} for none
*/
public java.lang.String getDisplayName() {
return displayName;
}
/**
* The human readable name of the resource.
* @param displayName displayName or {@code null} for none
*/
public GoogleCloudSecuritycenterV1Resource setDisplayName(java.lang.String displayName) {
this.displayName = displayName;
return this;
}
/**
* Output only. Contains a Folder message for each folder in the assets ancestry. The first folder
* is the deepest nested folder, and the last folder is the folder directly under the
* Organization.
* @return value or {@code null} for none
*/
public java.util.List getFolders() {
return folders;
}
/**
* Output only. Contains a Folder message for each folder in the assets ancestry. The first folder
* is the deepest nested folder, and the last folder is the folder directly under the
* Organization.
* @param folders folders or {@code null} for none
*/
public GoogleCloudSecuritycenterV1Resource setFolders(java.util.List folders) {
this.folders = folders;
return this;
}
/**
* The region or location of the service (if applicable).
* @return value or {@code null} for none
*/
public java.lang.String getLocation() {
return location;
}
/**
* The region or location of the service (if applicable).
* @param location location or {@code null} for none
*/
public GoogleCloudSecuritycenterV1Resource setLocation(java.lang.String location) {
this.location = location;
return this;
}
/**
* The full resource name of the resource. See:
* https://cloud.google.com/apis/design/resource_names#full_resource_name
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* The full resource name of the resource. See:
* https://cloud.google.com/apis/design/resource_names#full_resource_name
* @param name name or {@code null} for none
*/
public GoogleCloudSecuritycenterV1Resource setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Indicates which organization or tenant in the cloud provider the finding applies to.
* @return value or {@code null} for none
*/
public java.lang.String getOrganization() {
return organization;
}
/**
* Indicates which organization or tenant in the cloud provider the finding applies to.
* @param organization organization or {@code null} for none
*/
public GoogleCloudSecuritycenterV1Resource setOrganization(java.lang.String organization) {
this.organization = organization;
return this;
}
/**
* The full resource name of resource's parent.
* @return value or {@code null} for none
*/
public java.lang.String getParent() {
return parent;
}
/**
* The full resource name of resource's parent.
* @param parent parent or {@code null} for none
*/
public GoogleCloudSecuritycenterV1Resource setParent(java.lang.String parent) {
this.parent = parent;
return this;
}
/**
* The human readable name of resource's parent.
* @return value or {@code null} for none
*/
public java.lang.String getParentDisplayName() {
return parentDisplayName;
}
/**
* The human readable name of resource's parent.
* @param parentDisplayName parentDisplayName or {@code null} for none
*/
public GoogleCloudSecuritycenterV1Resource setParentDisplayName(java.lang.String parentDisplayName) {
this.parentDisplayName = parentDisplayName;
return this;
}
/**
* The full resource name of project that the resource belongs to.
* @return value or {@code null} for none
*/
public java.lang.String getProject() {
return project;
}
/**
* The full resource name of project that the resource belongs to.
* @param project project or {@code null} for none
*/
public GoogleCloudSecuritycenterV1Resource setProject(java.lang.String project) {
this.project = project;
return this;
}
/**
* The project ID that the resource belongs to.
* @return value or {@code null} for none
*/
public java.lang.String getProjectDisplayName() {
return projectDisplayName;
}
/**
* The project ID that the resource belongs to.
* @param projectDisplayName projectDisplayName or {@code null} for none
*/
public GoogleCloudSecuritycenterV1Resource setProjectDisplayName(java.lang.String projectDisplayName) {
this.projectDisplayName = projectDisplayName;
return this;
}
/**
* Provides the path to the resource within the resource hierarchy.
* @return value or {@code null} for none
*/
public ResourcePath getResourcePath() {
return resourcePath;
}
/**
* Provides the path to the resource within the resource hierarchy.
* @param resourcePath resourcePath or {@code null} for none
*/
public GoogleCloudSecuritycenterV1Resource setResourcePath(ResourcePath resourcePath) {
this.resourcePath = resourcePath;
return this;
}
/**
* A string representation of the resource path. For Google Cloud, it has the format of
* `organizations/{organization_id}/folders/{folder_id}/folders/{folder_id}/projects/{project_id}`
* where there can be any number of folders. For AWS, it has the format of `org/{organization_id}/
* ou/{organizational_unit_id}/ou/{organizational_unit_id}/account/{account_id}` where there can
* be any number of organizational units. For Azure, it has the format of `mg/{management_group_id
* }/mg/{management_group_id}/subscription/{subscription_id}/rg/{resource_group_name}` where there
* can be any number of management groups.
* @return value or {@code null} for none
*/
public java.lang.String getResourcePathString() {
return resourcePathString;
}
/**
* A string representation of the resource path. For Google Cloud, it has the format of
* `organizations/{organization_id}/folders/{folder_id}/folders/{folder_id}/projects/{project_id}`
* where there can be any number of folders. For AWS, it has the format of `org/{organization_id}/
* ou/{organizational_unit_id}/ou/{organizational_unit_id}/account/{account_id}` where there can
* be any number of organizational units. For Azure, it has the format of `mg/{management_group_id
* }/mg/{management_group_id}/subscription/{subscription_id}/rg/{resource_group_name}` where there
* can be any number of management groups.
* @param resourcePathString resourcePathString or {@code null} for none
*/
public GoogleCloudSecuritycenterV1Resource setResourcePathString(java.lang.String resourcePathString) {
this.resourcePathString = resourcePathString;
return this;
}
/**
* The parent service or product from which the resource is provided, for example, GKE or SNS.
* @return value or {@code null} for none
*/
public java.lang.String getService() {
return service;
}
/**
* The parent service or product from which the resource is provided, for example, GKE or SNS.
* @param service service or {@code null} for none
*/
public GoogleCloudSecuritycenterV1Resource setService(java.lang.String service) {
this.service = service;
return this;
}
/**
* The full resource type of the resource.
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* The full resource type of the resource.
* @param type type or {@code null} for none
*/
public GoogleCloudSecuritycenterV1Resource setType(java.lang.String type) {
this.type = type;
return this;
}
@Override
public GoogleCloudSecuritycenterV1Resource set(String fieldName, Object value) {
return (GoogleCloudSecuritycenterV1Resource) super.set(fieldName, value);
}
@Override
public GoogleCloudSecuritycenterV1Resource clone() {
return (GoogleCloudSecuritycenterV1Resource) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy