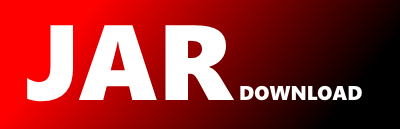
com.google.api.services.securitycenter.v1.model.GoogleCloudSecuritycenterV2CloudArmor Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.securitycenter.v1.model;
/**
* Fields related to Google Cloud Armor findings.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Security Command Center API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudSecuritycenterV2CloudArmor extends com.google.api.client.json.GenericJson {
/**
* Information about potential Layer 7 DDoS attacks identified by [Google Cloud Armor Adaptive
* Protection](https://cloud.google.com/armor/docs/adaptive-protection-overview).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudSecuritycenterV2AdaptiveProtection adaptiveProtection;
/**
* Information about DDoS attack volume and classification.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudSecuritycenterV2Attack attack;
/**
* Duration of attack from the start until the current moment (updated every 5 minutes).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String duration;
/**
* Information about incoming requests evaluated by [Google Cloud Armor security
* policies](https://cloud.google.com/armor/docs/security-policy-overview).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudSecuritycenterV2Requests requests;
/**
* Information about the [Google Cloud Armor security
* policy](https://cloud.google.com/armor/docs/security-policy-overview) relevant to the finding.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudSecuritycenterV2SecurityPolicy securityPolicy;
/**
* Distinguish between volumetric & protocol DDoS attack and application layer attacks. For
* example, "L3_4" for Layer 3 and Layer 4 DDoS attacks, or "L_7" for Layer 7 DDoS attacks.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String threatVector;
/**
* Information about potential Layer 7 DDoS attacks identified by [Google Cloud Armor Adaptive
* Protection](https://cloud.google.com/armor/docs/adaptive-protection-overview).
* @return value or {@code null} for none
*/
public GoogleCloudSecuritycenterV2AdaptiveProtection getAdaptiveProtection() {
return adaptiveProtection;
}
/**
* Information about potential Layer 7 DDoS attacks identified by [Google Cloud Armor Adaptive
* Protection](https://cloud.google.com/armor/docs/adaptive-protection-overview).
* @param adaptiveProtection adaptiveProtection or {@code null} for none
*/
public GoogleCloudSecuritycenterV2CloudArmor setAdaptiveProtection(GoogleCloudSecuritycenterV2AdaptiveProtection adaptiveProtection) {
this.adaptiveProtection = adaptiveProtection;
return this;
}
/**
* Information about DDoS attack volume and classification.
* @return value or {@code null} for none
*/
public GoogleCloudSecuritycenterV2Attack getAttack() {
return attack;
}
/**
* Information about DDoS attack volume and classification.
* @param attack attack or {@code null} for none
*/
public GoogleCloudSecuritycenterV2CloudArmor setAttack(GoogleCloudSecuritycenterV2Attack attack) {
this.attack = attack;
return this;
}
/**
* Duration of attack from the start until the current moment (updated every 5 minutes).
* @return value or {@code null} for none
*/
public String getDuration() {
return duration;
}
/**
* Duration of attack from the start until the current moment (updated every 5 minutes).
* @param duration duration or {@code null} for none
*/
public GoogleCloudSecuritycenterV2CloudArmor setDuration(String duration) {
this.duration = duration;
return this;
}
/**
* Information about incoming requests evaluated by [Google Cloud Armor security
* policies](https://cloud.google.com/armor/docs/security-policy-overview).
* @return value or {@code null} for none
*/
public GoogleCloudSecuritycenterV2Requests getRequests() {
return requests;
}
/**
* Information about incoming requests evaluated by [Google Cloud Armor security
* policies](https://cloud.google.com/armor/docs/security-policy-overview).
* @param requests requests or {@code null} for none
*/
public GoogleCloudSecuritycenterV2CloudArmor setRequests(GoogleCloudSecuritycenterV2Requests requests) {
this.requests = requests;
return this;
}
/**
* Information about the [Google Cloud Armor security
* policy](https://cloud.google.com/armor/docs/security-policy-overview) relevant to the finding.
* @return value or {@code null} for none
*/
public GoogleCloudSecuritycenterV2SecurityPolicy getSecurityPolicy() {
return securityPolicy;
}
/**
* Information about the [Google Cloud Armor security
* policy](https://cloud.google.com/armor/docs/security-policy-overview) relevant to the finding.
* @param securityPolicy securityPolicy or {@code null} for none
*/
public GoogleCloudSecuritycenterV2CloudArmor setSecurityPolicy(GoogleCloudSecuritycenterV2SecurityPolicy securityPolicy) {
this.securityPolicy = securityPolicy;
return this;
}
/**
* Distinguish between volumetric & protocol DDoS attack and application layer attacks. For
* example, "L3_4" for Layer 3 and Layer 4 DDoS attacks, or "L_7" for Layer 7 DDoS attacks.
* @return value or {@code null} for none
*/
public java.lang.String getThreatVector() {
return threatVector;
}
/**
* Distinguish between volumetric & protocol DDoS attack and application layer attacks. For
* example, "L3_4" for Layer 3 and Layer 4 DDoS attacks, or "L_7" for Layer 7 DDoS attacks.
* @param threatVector threatVector or {@code null} for none
*/
public GoogleCloudSecuritycenterV2CloudArmor setThreatVector(java.lang.String threatVector) {
this.threatVector = threatVector;
return this;
}
@Override
public GoogleCloudSecuritycenterV2CloudArmor set(String fieldName, Object value) {
return (GoogleCloudSecuritycenterV2CloudArmor) super.set(fieldName, value);
}
@Override
public GoogleCloudSecuritycenterV2CloudArmor clone() {
return (GoogleCloudSecuritycenterV2CloudArmor) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy