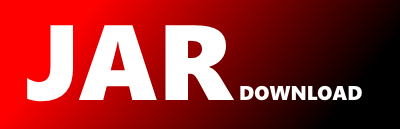
com.google.api.services.securitycenter.v1.model.GoogleCloudSecuritycenterV2Kubernetes Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.securitycenter.v1.model;
/**
* Kubernetes-related attributes.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Security Command Center API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudSecuritycenterV2Kubernetes extends com.google.api.client.json.GenericJson {
/**
* Provides information on any Kubernetes access reviews (privilege checks) relevant to the
* finding.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List accessReviews;
static {
// hack to force ProGuard to consider GoogleCloudSecuritycenterV2AccessReview used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleCloudSecuritycenterV2AccessReview.class);
}
/**
* Provides Kubernetes role binding information for findings that involve [RoleBindings or
* ClusterRoleBindings](https://cloud.google.com/kubernetes-engine/docs/how-to/role-based-access-
* control).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List bindings;
static {
// hack to force ProGuard to consider GoogleCloudSecuritycenterV2Binding used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleCloudSecuritycenterV2Binding.class);
}
/**
* GKE [node pools](https://cloud.google.com/kubernetes-engine/docs/concepts/node-pools)
* associated with the finding. This field contains node pool information for each node, when it
* is available.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List nodePools;
/**
* Provides Kubernetes [node](https://cloud.google.com/kubernetes-engine/docs/concepts/cluster-
* architecture#nodes) information.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List nodes;
/**
* Kubernetes objects related to the finding.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List objects;
/**
* Kubernetes [Pods](https://cloud.google.com/kubernetes-engine/docs/concepts/pod) associated with
* the finding. This field contains Pod records for each container that is owned by a Pod.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List pods;
/**
* Provides Kubernetes role information for findings that involve [Roles or
* ClusterRoles](https://cloud.google.com/kubernetes-engine/docs/how-to/role-based-access-
* control).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List roles;
/**
* Provides information on any Kubernetes access reviews (privilege checks) relevant to the
* finding.
* @return value or {@code null} for none
*/
public java.util.List getAccessReviews() {
return accessReviews;
}
/**
* Provides information on any Kubernetes access reviews (privilege checks) relevant to the
* finding.
* @param accessReviews accessReviews or {@code null} for none
*/
public GoogleCloudSecuritycenterV2Kubernetes setAccessReviews(java.util.List accessReviews) {
this.accessReviews = accessReviews;
return this;
}
/**
* Provides Kubernetes role binding information for findings that involve [RoleBindings or
* ClusterRoleBindings](https://cloud.google.com/kubernetes-engine/docs/how-to/role-based-access-
* control).
* @return value or {@code null} for none
*/
public java.util.List getBindings() {
return bindings;
}
/**
* Provides Kubernetes role binding information for findings that involve [RoleBindings or
* ClusterRoleBindings](https://cloud.google.com/kubernetes-engine/docs/how-to/role-based-access-
* control).
* @param bindings bindings or {@code null} for none
*/
public GoogleCloudSecuritycenterV2Kubernetes setBindings(java.util.List bindings) {
this.bindings = bindings;
return this;
}
/**
* GKE [node pools](https://cloud.google.com/kubernetes-engine/docs/concepts/node-pools)
* associated with the finding. This field contains node pool information for each node, when it
* is available.
* @return value or {@code null} for none
*/
public java.util.List getNodePools() {
return nodePools;
}
/**
* GKE [node pools](https://cloud.google.com/kubernetes-engine/docs/concepts/node-pools)
* associated with the finding. This field contains node pool information for each node, when it
* is available.
* @param nodePools nodePools or {@code null} for none
*/
public GoogleCloudSecuritycenterV2Kubernetes setNodePools(java.util.List nodePools) {
this.nodePools = nodePools;
return this;
}
/**
* Provides Kubernetes [node](https://cloud.google.com/kubernetes-engine/docs/concepts/cluster-
* architecture#nodes) information.
* @return value or {@code null} for none
*/
public java.util.List getNodes() {
return nodes;
}
/**
* Provides Kubernetes [node](https://cloud.google.com/kubernetes-engine/docs/concepts/cluster-
* architecture#nodes) information.
* @param nodes nodes or {@code null} for none
*/
public GoogleCloudSecuritycenterV2Kubernetes setNodes(java.util.List nodes) {
this.nodes = nodes;
return this;
}
/**
* Kubernetes objects related to the finding.
* @return value or {@code null} for none
*/
public java.util.List getObjects() {
return objects;
}
/**
* Kubernetes objects related to the finding.
* @param objects objects or {@code null} for none
*/
public GoogleCloudSecuritycenterV2Kubernetes setObjects(java.util.List objects) {
this.objects = objects;
return this;
}
/**
* Kubernetes [Pods](https://cloud.google.com/kubernetes-engine/docs/concepts/pod) associated with
* the finding. This field contains Pod records for each container that is owned by a Pod.
* @return value or {@code null} for none
*/
public java.util.List getPods() {
return pods;
}
/**
* Kubernetes [Pods](https://cloud.google.com/kubernetes-engine/docs/concepts/pod) associated with
* the finding. This field contains Pod records for each container that is owned by a Pod.
* @param pods pods or {@code null} for none
*/
public GoogleCloudSecuritycenterV2Kubernetes setPods(java.util.List pods) {
this.pods = pods;
return this;
}
/**
* Provides Kubernetes role information for findings that involve [Roles or
* ClusterRoles](https://cloud.google.com/kubernetes-engine/docs/how-to/role-based-access-
* control).
* @return value or {@code null} for none
*/
public java.util.List getRoles() {
return roles;
}
/**
* Provides Kubernetes role information for findings that involve [Roles or
* ClusterRoles](https://cloud.google.com/kubernetes-engine/docs/how-to/role-based-access-
* control).
* @param roles roles or {@code null} for none
*/
public GoogleCloudSecuritycenterV2Kubernetes setRoles(java.util.List roles) {
this.roles = roles;
return this;
}
@Override
public GoogleCloudSecuritycenterV2Kubernetes set(String fieldName, Object value) {
return (GoogleCloudSecuritycenterV2Kubernetes) super.set(fieldName, value);
}
@Override
public GoogleCloudSecuritycenterV2Kubernetes clone() {
return (GoogleCloudSecuritycenterV2Kubernetes) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy