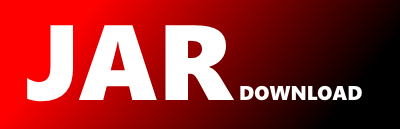
com.google.api.services.securitycenter.v1.model.GoogleCloudSecuritycenterV2ResourceValueConfig Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.securitycenter.v1.model;
/**
* A resource value configuration (RVC) is a mapping configuration of user's resources to resource
* values. Used in Attack path simulations.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Security Command Center API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudSecuritycenterV2ResourceValueConfig extends com.google.api.client.json.GenericJson {
/**
* Cloud provider this configuration applies to
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String cloudProvider;
/**
* Output only. Timestamp this resource value configuration was created.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String createTime;
/**
* Description of the resource value configuration.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* Identifier. Name for the resource value configuration
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* List of resource labels to search for, evaluated with `AND`. For example,
* "resource_labels_selector": {"key": "value", "env": "prod"} will match resources with labels
* "key": "value" `AND` "env": "prod" https://cloud.google.com/resource-manager/docs/creating-
* managing-labels
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map resourceLabelsSelector;
/**
* Apply resource_value only to resources that match resource_type. resource_type will be checked
* with `AND` of other resources. For example, "storage.googleapis.com/Bucket" with resource_value
* "HIGH" will apply "HIGH" value only to "storage.googleapis.com/Bucket" resources.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String resourceType;
/**
* Resource value level this expression represents Only required when there is no Sensitive Data
* Protection mapping in the request
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String resourceValue;
/**
* Project or folder to scope this configuration to. For example, "project/456" would apply this
* configuration only to resources in "project/456" scope and will be checked with `AND` of other
* resources.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String scope;
/**
* A mapping of the sensitivity on Sensitive Data Protection finding to resource values. This
* mapping can only be used in combination with a resource_type that is related to BigQuery, e.g.
* "bigquery.googleapis.com/Dataset".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudSecuritycenterV2SensitiveDataProtectionMapping sensitiveDataProtectionMapping;
/**
* Tag values combined with `AND` to check against. Values in the form "tagValues/123" Example: `[
* "tagValues/123", "tagValues/456", "tagValues/789" ]` https://cloud.google.com/resource-
* manager/docs/tags/tags-creating-and-managing
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List tagValues;
/**
* Output only. Timestamp this resource value configuration was last updated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String updateTime;
/**
* Cloud provider this configuration applies to
* @return value or {@code null} for none
*/
public java.lang.String getCloudProvider() {
return cloudProvider;
}
/**
* Cloud provider this configuration applies to
* @param cloudProvider cloudProvider or {@code null} for none
*/
public GoogleCloudSecuritycenterV2ResourceValueConfig setCloudProvider(java.lang.String cloudProvider) {
this.cloudProvider = cloudProvider;
return this;
}
/**
* Output only. Timestamp this resource value configuration was created.
* @return value or {@code null} for none
*/
public String getCreateTime() {
return createTime;
}
/**
* Output only. Timestamp this resource value configuration was created.
* @param createTime createTime or {@code null} for none
*/
public GoogleCloudSecuritycenterV2ResourceValueConfig setCreateTime(String createTime) {
this.createTime = createTime;
return this;
}
/**
* Description of the resource value configuration.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* Description of the resource value configuration.
* @param description description or {@code null} for none
*/
public GoogleCloudSecuritycenterV2ResourceValueConfig setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* Identifier. Name for the resource value configuration
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Identifier. Name for the resource value configuration
* @param name name or {@code null} for none
*/
public GoogleCloudSecuritycenterV2ResourceValueConfig setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* List of resource labels to search for, evaluated with `AND`. For example,
* "resource_labels_selector": {"key": "value", "env": "prod"} will match resources with labels
* "key": "value" `AND` "env": "prod" https://cloud.google.com/resource-manager/docs/creating-
* managing-labels
* @return value or {@code null} for none
*/
public java.util.Map getResourceLabelsSelector() {
return resourceLabelsSelector;
}
/**
* List of resource labels to search for, evaluated with `AND`. For example,
* "resource_labels_selector": {"key": "value", "env": "prod"} will match resources with labels
* "key": "value" `AND` "env": "prod" https://cloud.google.com/resource-manager/docs/creating-
* managing-labels
* @param resourceLabelsSelector resourceLabelsSelector or {@code null} for none
*/
public GoogleCloudSecuritycenterV2ResourceValueConfig setResourceLabelsSelector(java.util.Map resourceLabelsSelector) {
this.resourceLabelsSelector = resourceLabelsSelector;
return this;
}
/**
* Apply resource_value only to resources that match resource_type. resource_type will be checked
* with `AND` of other resources. For example, "storage.googleapis.com/Bucket" with resource_value
* "HIGH" will apply "HIGH" value only to "storage.googleapis.com/Bucket" resources.
* @return value or {@code null} for none
*/
public java.lang.String getResourceType() {
return resourceType;
}
/**
* Apply resource_value only to resources that match resource_type. resource_type will be checked
* with `AND` of other resources. For example, "storage.googleapis.com/Bucket" with resource_value
* "HIGH" will apply "HIGH" value only to "storage.googleapis.com/Bucket" resources.
* @param resourceType resourceType or {@code null} for none
*/
public GoogleCloudSecuritycenterV2ResourceValueConfig setResourceType(java.lang.String resourceType) {
this.resourceType = resourceType;
return this;
}
/**
* Resource value level this expression represents Only required when there is no Sensitive Data
* Protection mapping in the request
* @return value or {@code null} for none
*/
public java.lang.String getResourceValue() {
return resourceValue;
}
/**
* Resource value level this expression represents Only required when there is no Sensitive Data
* Protection mapping in the request
* @param resourceValue resourceValue or {@code null} for none
*/
public GoogleCloudSecuritycenterV2ResourceValueConfig setResourceValue(java.lang.String resourceValue) {
this.resourceValue = resourceValue;
return this;
}
/**
* Project or folder to scope this configuration to. For example, "project/456" would apply this
* configuration only to resources in "project/456" scope and will be checked with `AND` of other
* resources.
* @return value or {@code null} for none
*/
public java.lang.String getScope() {
return scope;
}
/**
* Project or folder to scope this configuration to. For example, "project/456" would apply this
* configuration only to resources in "project/456" scope and will be checked with `AND` of other
* resources.
* @param scope scope or {@code null} for none
*/
public GoogleCloudSecuritycenterV2ResourceValueConfig setScope(java.lang.String scope) {
this.scope = scope;
return this;
}
/**
* A mapping of the sensitivity on Sensitive Data Protection finding to resource values. This
* mapping can only be used in combination with a resource_type that is related to BigQuery, e.g.
* "bigquery.googleapis.com/Dataset".
* @return value or {@code null} for none
*/
public GoogleCloudSecuritycenterV2SensitiveDataProtectionMapping getSensitiveDataProtectionMapping() {
return sensitiveDataProtectionMapping;
}
/**
* A mapping of the sensitivity on Sensitive Data Protection finding to resource values. This
* mapping can only be used in combination with a resource_type that is related to BigQuery, e.g.
* "bigquery.googleapis.com/Dataset".
* @param sensitiveDataProtectionMapping sensitiveDataProtectionMapping or {@code null} for none
*/
public GoogleCloudSecuritycenterV2ResourceValueConfig setSensitiveDataProtectionMapping(GoogleCloudSecuritycenterV2SensitiveDataProtectionMapping sensitiveDataProtectionMapping) {
this.sensitiveDataProtectionMapping = sensitiveDataProtectionMapping;
return this;
}
/**
* Tag values combined with `AND` to check against. Values in the form "tagValues/123" Example: `[
* "tagValues/123", "tagValues/456", "tagValues/789" ]` https://cloud.google.com/resource-
* manager/docs/tags/tags-creating-and-managing
* @return value or {@code null} for none
*/
public java.util.List getTagValues() {
return tagValues;
}
/**
* Tag values combined with `AND` to check against. Values in the form "tagValues/123" Example: `[
* "tagValues/123", "tagValues/456", "tagValues/789" ]` https://cloud.google.com/resource-
* manager/docs/tags/tags-creating-and-managing
* @param tagValues tagValues or {@code null} for none
*/
public GoogleCloudSecuritycenterV2ResourceValueConfig setTagValues(java.util.List tagValues) {
this.tagValues = tagValues;
return this;
}
/**
* Output only. Timestamp this resource value configuration was last updated.
* @return value or {@code null} for none
*/
public String getUpdateTime() {
return updateTime;
}
/**
* Output only. Timestamp this resource value configuration was last updated.
* @param updateTime updateTime or {@code null} for none
*/
public GoogleCloudSecuritycenterV2ResourceValueConfig setUpdateTime(String updateTime) {
this.updateTime = updateTime;
return this;
}
@Override
public GoogleCloudSecuritycenterV2ResourceValueConfig set(String fieldName, Object value) {
return (GoogleCloudSecuritycenterV2ResourceValueConfig) super.set(fieldName, value);
}
@Override
public GoogleCloudSecuritycenterV2ResourceValueConfig clone() {
return (GoogleCloudSecuritycenterV2ResourceValueConfig) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy