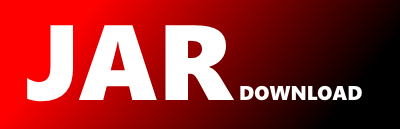
com.google.api.services.securitycenter.v1.model.SecurityCenterProperties Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.securitycenter.v1.model;
/**
* Security Command Center managed properties. These properties are managed by Security Command
* Center and cannot be modified by the user.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Security Command Center API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class SecurityCenterProperties extends com.google.api.client.json.GenericJson {
/**
* Contains a Folder message for each folder in the assets ancestry. The first folder is the
* deepest nested folder, and the last folder is the folder directly under the Organization.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List folders;
static {
// hack to force ProGuard to consider Folder used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(Folder.class);
}
/**
* The user defined display name for this resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String resourceDisplayName;
/**
* The full resource name of the Google Cloud resource this asset represents. This field is
* immutable after create time. See:
* https://cloud.google.com/apis/design/resource_names#full_resource_name
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String resourceName;
/**
* Owners of the Google Cloud resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List resourceOwners;
/**
* The full resource name of the immediate parent of the resource. See:
* https://cloud.google.com/apis/design/resource_names#full_resource_name
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String resourceParent;
/**
* The user defined display name for the parent of this resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String resourceParentDisplayName;
/**
* The full resource name of the project the resource belongs to. See:
* https://cloud.google.com/apis/design/resource_names#full_resource_name
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String resourceProject;
/**
* The user defined display name for the project of this resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String resourceProjectDisplayName;
/**
* The type of the Google Cloud resource. Examples include: APPLICATION, PROJECT, and
* ORGANIZATION. This is a case insensitive field defined by Security Command Center and/or the
* producer of the resource and is immutable after create time.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String resourceType;
/**
* Contains a Folder message for each folder in the assets ancestry. The first folder is the
* deepest nested folder, and the last folder is the folder directly under the Organization.
* @return value or {@code null} for none
*/
public java.util.List getFolders() {
return folders;
}
/**
* Contains a Folder message for each folder in the assets ancestry. The first folder is the
* deepest nested folder, and the last folder is the folder directly under the Organization.
* @param folders folders or {@code null} for none
*/
public SecurityCenterProperties setFolders(java.util.List folders) {
this.folders = folders;
return this;
}
/**
* The user defined display name for this resource.
* @return value or {@code null} for none
*/
public java.lang.String getResourceDisplayName() {
return resourceDisplayName;
}
/**
* The user defined display name for this resource.
* @param resourceDisplayName resourceDisplayName or {@code null} for none
*/
public SecurityCenterProperties setResourceDisplayName(java.lang.String resourceDisplayName) {
this.resourceDisplayName = resourceDisplayName;
return this;
}
/**
* The full resource name of the Google Cloud resource this asset represents. This field is
* immutable after create time. See:
* https://cloud.google.com/apis/design/resource_names#full_resource_name
* @return value or {@code null} for none
*/
public java.lang.String getResourceName() {
return resourceName;
}
/**
* The full resource name of the Google Cloud resource this asset represents. This field is
* immutable after create time. See:
* https://cloud.google.com/apis/design/resource_names#full_resource_name
* @param resourceName resourceName or {@code null} for none
*/
public SecurityCenterProperties setResourceName(java.lang.String resourceName) {
this.resourceName = resourceName;
return this;
}
/**
* Owners of the Google Cloud resource.
* @return value or {@code null} for none
*/
public java.util.List getResourceOwners() {
return resourceOwners;
}
/**
* Owners of the Google Cloud resource.
* @param resourceOwners resourceOwners or {@code null} for none
*/
public SecurityCenterProperties setResourceOwners(java.util.List resourceOwners) {
this.resourceOwners = resourceOwners;
return this;
}
/**
* The full resource name of the immediate parent of the resource. See:
* https://cloud.google.com/apis/design/resource_names#full_resource_name
* @return value or {@code null} for none
*/
public java.lang.String getResourceParent() {
return resourceParent;
}
/**
* The full resource name of the immediate parent of the resource. See:
* https://cloud.google.com/apis/design/resource_names#full_resource_name
* @param resourceParent resourceParent or {@code null} for none
*/
public SecurityCenterProperties setResourceParent(java.lang.String resourceParent) {
this.resourceParent = resourceParent;
return this;
}
/**
* The user defined display name for the parent of this resource.
* @return value or {@code null} for none
*/
public java.lang.String getResourceParentDisplayName() {
return resourceParentDisplayName;
}
/**
* The user defined display name for the parent of this resource.
* @param resourceParentDisplayName resourceParentDisplayName or {@code null} for none
*/
public SecurityCenterProperties setResourceParentDisplayName(java.lang.String resourceParentDisplayName) {
this.resourceParentDisplayName = resourceParentDisplayName;
return this;
}
/**
* The full resource name of the project the resource belongs to. See:
* https://cloud.google.com/apis/design/resource_names#full_resource_name
* @return value or {@code null} for none
*/
public java.lang.String getResourceProject() {
return resourceProject;
}
/**
* The full resource name of the project the resource belongs to. See:
* https://cloud.google.com/apis/design/resource_names#full_resource_name
* @param resourceProject resourceProject or {@code null} for none
*/
public SecurityCenterProperties setResourceProject(java.lang.String resourceProject) {
this.resourceProject = resourceProject;
return this;
}
/**
* The user defined display name for the project of this resource.
* @return value or {@code null} for none
*/
public java.lang.String getResourceProjectDisplayName() {
return resourceProjectDisplayName;
}
/**
* The user defined display name for the project of this resource.
* @param resourceProjectDisplayName resourceProjectDisplayName or {@code null} for none
*/
public SecurityCenterProperties setResourceProjectDisplayName(java.lang.String resourceProjectDisplayName) {
this.resourceProjectDisplayName = resourceProjectDisplayName;
return this;
}
/**
* The type of the Google Cloud resource. Examples include: APPLICATION, PROJECT, and
* ORGANIZATION. This is a case insensitive field defined by Security Command Center and/or the
* producer of the resource and is immutable after create time.
* @return value or {@code null} for none
*/
public java.lang.String getResourceType() {
return resourceType;
}
/**
* The type of the Google Cloud resource. Examples include: APPLICATION, PROJECT, and
* ORGANIZATION. This is a case insensitive field defined by Security Command Center and/or the
* producer of the resource and is immutable after create time.
* @param resourceType resourceType or {@code null} for none
*/
public SecurityCenterProperties setResourceType(java.lang.String resourceType) {
this.resourceType = resourceType;
return this;
}
@Override
public SecurityCenterProperties set(String fieldName, Object value) {
return (SecurityCenterProperties) super.set(fieldName, value);
}
@Override
public SecurityCenterProperties clone() {
return (SecurityCenterProperties) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy