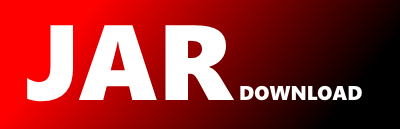
com.google.api.services.servicecontrol.v1.model.Operation Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-10-17 16:43:55 UTC)
* on 2016-11-10 at 17:05:21 UTC
* Modify at your own risk.
*/
package com.google.api.services.servicecontrol.v1.model;
/**
* Represents information regarding an operation.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Service Control API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Operation extends com.google.api.client.json.GenericJson {
/**
* Identity of the consumer who is using the service. This field should be filled in for the
* operations initiated by a consumer, but not for service-initiated operations that are not
* related to a specific consumer.
*
* This can be in one of the following formats: project:, project_number:, api_key:.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String consumerId;
/**
* End time of the operation. Required when the operation is used in ServiceController.Report, but
* optional when the operation is used in ServiceController.Check.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String endTime;
/**
* DO NOT USE. This is an experimental field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String importance;
/**
* Labels describing the operation. Only the following labels are allowed:
*
* - Labels describing monitored resources as defined in the service configuration. - Default
* labels of metric values. When specified, labels defined in the metric value override these
* default. - The following labels defined by Google Cloud Platform: -
* `cloud.googleapis.com/location` describing the location where the operation happened,
* - `servicecontrol.googleapis.com/user_agent` describing the user agent of the API
* request, - `servicecontrol.googleapis.com/service_agent` describing the service used
* to handle the API request (e.g. ESP), - `servicecontrol.googleapis.com/platform` describing
* the platform where the API is served (e.g. GAE, GCE, GKE).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map labels;
/**
* Represents information to be logged.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List logEntries;
/**
* Represents information about this operation. Each MetricValueSet corresponds to a metric
* defined in the service configuration. The data type used in the MetricValueSet must agree with
* the data type specified in the metric definition.
*
* Within a single operation, it is not allowed to have more than one MetricValue instances that
* have the same metric names and identical label value combinations. If a request has such
* duplicated MetricValue instances, the entire request is rejected with an invalid argument
* error.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List metricValueSets;
/**
* Identity of the operation. This must be unique within the scope of the service that generated
* the operation. If the service calls Check() and Report() on the same operation, the two calls
* should carry the same id.
*
* UUID version 4 is recommended, though not required. In scenarios where an operation is computed
* from existing information and an idempotent id is desirable for deduplication purpose, UUID
* version 5 is recommended. See RFC 4122 for details.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String operationId;
/**
* Fully qualified name of the operation. Reserved for future use.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String operationName;
/**
* Required. Start time of the operation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String startTime;
/**
* Identity of the consumer who is using the service. This field should be filled in for the
* operations initiated by a consumer, but not for service-initiated operations that are not
* related to a specific consumer.
*
* This can be in one of the following formats: project:, project_number:, api_key:.
* @return value or {@code null} for none
*/
public java.lang.String getConsumerId() {
return consumerId;
}
/**
* Identity of the consumer who is using the service. This field should be filled in for the
* operations initiated by a consumer, but not for service-initiated operations that are not
* related to a specific consumer.
*
* This can be in one of the following formats: project:, project_number:, api_key:.
* @param consumerId consumerId or {@code null} for none
*/
public Operation setConsumerId(java.lang.String consumerId) {
this.consumerId = consumerId;
return this;
}
/**
* End time of the operation. Required when the operation is used in ServiceController.Report, but
* optional when the operation is used in ServiceController.Check.
* @return value or {@code null} for none
*/
public String getEndTime() {
return endTime;
}
/**
* End time of the operation. Required when the operation is used in ServiceController.Report, but
* optional when the operation is used in ServiceController.Check.
* @param endTime endTime or {@code null} for none
*/
public Operation setEndTime(String endTime) {
this.endTime = endTime;
return this;
}
/**
* DO NOT USE. This is an experimental field.
* @return value or {@code null} for none
*/
public java.lang.String getImportance() {
return importance;
}
/**
* DO NOT USE. This is an experimental field.
* @param importance importance or {@code null} for none
*/
public Operation setImportance(java.lang.String importance) {
this.importance = importance;
return this;
}
/**
* Labels describing the operation. Only the following labels are allowed:
*
* - Labels describing monitored resources as defined in the service configuration. - Default
* labels of metric values. When specified, labels defined in the metric value override these
* default. - The following labels defined by Google Cloud Platform: -
* `cloud.googleapis.com/location` describing the location where the operation happened,
* - `servicecontrol.googleapis.com/user_agent` describing the user agent of the API
* request, - `servicecontrol.googleapis.com/service_agent` describing the service used
* to handle the API request (e.g. ESP), - `servicecontrol.googleapis.com/platform` describing
* the platform where the API is served (e.g. GAE, GCE, GKE).
* @return value or {@code null} for none
*/
public java.util.Map getLabels() {
return labels;
}
/**
* Labels describing the operation. Only the following labels are allowed:
*
* - Labels describing monitored resources as defined in the service configuration. - Default
* labels of metric values. When specified, labels defined in the metric value override these
* default. - The following labels defined by Google Cloud Platform: -
* `cloud.googleapis.com/location` describing the location where the operation happened,
* - `servicecontrol.googleapis.com/user_agent` describing the user agent of the API
* request, - `servicecontrol.googleapis.com/service_agent` describing the service used
* to handle the API request (e.g. ESP), - `servicecontrol.googleapis.com/platform` describing
* the platform where the API is served (e.g. GAE, GCE, GKE).
* @param labels labels or {@code null} for none
*/
public Operation setLabels(java.util.Map labels) {
this.labels = labels;
return this;
}
/**
* Represents information to be logged.
* @return value or {@code null} for none
*/
public java.util.List getLogEntries() {
return logEntries;
}
/**
* Represents information to be logged.
* @param logEntries logEntries or {@code null} for none
*/
public Operation setLogEntries(java.util.List logEntries) {
this.logEntries = logEntries;
return this;
}
/**
* Represents information about this operation. Each MetricValueSet corresponds to a metric
* defined in the service configuration. The data type used in the MetricValueSet must agree with
* the data type specified in the metric definition.
*
* Within a single operation, it is not allowed to have more than one MetricValue instances that
* have the same metric names and identical label value combinations. If a request has such
* duplicated MetricValue instances, the entire request is rejected with an invalid argument
* error.
* @return value or {@code null} for none
*/
public java.util.List getMetricValueSets() {
return metricValueSets;
}
/**
* Represents information about this operation. Each MetricValueSet corresponds to a metric
* defined in the service configuration. The data type used in the MetricValueSet must agree with
* the data type specified in the metric definition.
*
* Within a single operation, it is not allowed to have more than one MetricValue instances that
* have the same metric names and identical label value combinations. If a request has such
* duplicated MetricValue instances, the entire request is rejected with an invalid argument
* error.
* @param metricValueSets metricValueSets or {@code null} for none
*/
public Operation setMetricValueSets(java.util.List metricValueSets) {
this.metricValueSets = metricValueSets;
return this;
}
/**
* Identity of the operation. This must be unique within the scope of the service that generated
* the operation. If the service calls Check() and Report() on the same operation, the two calls
* should carry the same id.
*
* UUID version 4 is recommended, though not required. In scenarios where an operation is computed
* from existing information and an idempotent id is desirable for deduplication purpose, UUID
* version 5 is recommended. See RFC 4122 for details.
* @return value or {@code null} for none
*/
public java.lang.String getOperationId() {
return operationId;
}
/**
* Identity of the operation. This must be unique within the scope of the service that generated
* the operation. If the service calls Check() and Report() on the same operation, the two calls
* should carry the same id.
*
* UUID version 4 is recommended, though not required. In scenarios where an operation is computed
* from existing information and an idempotent id is desirable for deduplication purpose, UUID
* version 5 is recommended. See RFC 4122 for details.
* @param operationId operationId or {@code null} for none
*/
public Operation setOperationId(java.lang.String operationId) {
this.operationId = operationId;
return this;
}
/**
* Fully qualified name of the operation. Reserved for future use.
* @return value or {@code null} for none
*/
public java.lang.String getOperationName() {
return operationName;
}
/**
* Fully qualified name of the operation. Reserved for future use.
* @param operationName operationName or {@code null} for none
*/
public Operation setOperationName(java.lang.String operationName) {
this.operationName = operationName;
return this;
}
/**
* Required. Start time of the operation.
* @return value or {@code null} for none
*/
public String getStartTime() {
return startTime;
}
/**
* Required. Start time of the operation.
* @param startTime startTime or {@code null} for none
*/
public Operation setStartTime(String startTime) {
this.startTime = startTime;
return this;
}
@Override
public Operation set(String fieldName, Object value) {
return (Operation) super.set(fieldName, value);
}
@Override
public Operation clone() {
return (Operation) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy