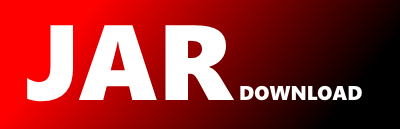
com.google.api.services.servicecontrol.v2.model.AttributeContext Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.servicecontrol.v2.model;
/**
* This message defines the standard attribute vocabulary for Google APIs. An attribute is a piece
* of metadata that describes an activity on a network service. For example, the size of an HTTP
* request, or the status code of an HTTP response. Each attribute has a type and a name, which is
* logically defined as a proto message field in `AttributeContext`. The field type becomes the
* attribute type, and the field path becomes the attribute name. For example, the attribute
* `source.ip` maps to field `AttributeContext.source.ip`. This message definition is guaranteed not
* to have any wire breaking change. So you can use it directly for passing attributes across
* different systems. NOTE: Different system may generate different subset of attributes. Please
* verify the system specification before relying on an attribute generated a system.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Service Control API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class AttributeContext extends com.google.api.client.json.GenericJson {
/**
* Represents an API operation that is involved to a network activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Api api;
/**
* The destination of a network activity, such as accepting a TCP connection. In a multi hop
* network activity, the destination represents the receiver of the last hop.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Peer destination;
/**
* Supports extensions for advanced use cases, such as logs and metrics.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List> extensions;
/**
* The origin of a network activity. In a multi hop network activity, the origin represents the
* sender of the first hop. For the first hop, the `source` and the `origin` must have the same
* content.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Peer origin;
/**
* Represents a network request, such as an HTTP request.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Request request;
/**
* Represents a target resource that is involved with a network activity. If multiple resources
* are involved with an activity, this must be the primary one.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Resource resource;
/**
* Represents a network response, such as an HTTP response.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Response response;
/**
* The source of a network activity, such as starting a TCP connection. In a multi hop network
* activity, the source represents the sender of the last hop.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Peer source;
/**
* Represents an API operation that is involved to a network activity.
* @return value or {@code null} for none
*/
public Api getApi() {
return api;
}
/**
* Represents an API operation that is involved to a network activity.
* @param api api or {@code null} for none
*/
public AttributeContext setApi(Api api) {
this.api = api;
return this;
}
/**
* The destination of a network activity, such as accepting a TCP connection. In a multi hop
* network activity, the destination represents the receiver of the last hop.
* @return value or {@code null} for none
*/
public Peer getDestination() {
return destination;
}
/**
* The destination of a network activity, such as accepting a TCP connection. In a multi hop
* network activity, the destination represents the receiver of the last hop.
* @param destination destination or {@code null} for none
*/
public AttributeContext setDestination(Peer destination) {
this.destination = destination;
return this;
}
/**
* Supports extensions for advanced use cases, such as logs and metrics.
* @return value or {@code null} for none
*/
public java.util.List> getExtensions() {
return extensions;
}
/**
* Supports extensions for advanced use cases, such as logs and metrics.
* @param extensions extensions or {@code null} for none
*/
public AttributeContext setExtensions(java.util.List> extensions) {
this.extensions = extensions;
return this;
}
/**
* The origin of a network activity. In a multi hop network activity, the origin represents the
* sender of the first hop. For the first hop, the `source` and the `origin` must have the same
* content.
* @return value or {@code null} for none
*/
public Peer getOrigin() {
return origin;
}
/**
* The origin of a network activity. In a multi hop network activity, the origin represents the
* sender of the first hop. For the first hop, the `source` and the `origin` must have the same
* content.
* @param origin origin or {@code null} for none
*/
public AttributeContext setOrigin(Peer origin) {
this.origin = origin;
return this;
}
/**
* Represents a network request, such as an HTTP request.
* @return value or {@code null} for none
*/
public Request getRequest() {
return request;
}
/**
* Represents a network request, such as an HTTP request.
* @param request request or {@code null} for none
*/
public AttributeContext setRequest(Request request) {
this.request = request;
return this;
}
/**
* Represents a target resource that is involved with a network activity. If multiple resources
* are involved with an activity, this must be the primary one.
* @return value or {@code null} for none
*/
public Resource getResource() {
return resource;
}
/**
* Represents a target resource that is involved with a network activity. If multiple resources
* are involved with an activity, this must be the primary one.
* @param resource resource or {@code null} for none
*/
public AttributeContext setResource(Resource resource) {
this.resource = resource;
return this;
}
/**
* Represents a network response, such as an HTTP response.
* @return value or {@code null} for none
*/
public Response getResponse() {
return response;
}
/**
* Represents a network response, such as an HTTP response.
* @param response response or {@code null} for none
*/
public AttributeContext setResponse(Response response) {
this.response = response;
return this;
}
/**
* The source of a network activity, such as starting a TCP connection. In a multi hop network
* activity, the source represents the sender of the last hop.
* @return value or {@code null} for none
*/
public Peer getSource() {
return source;
}
/**
* The source of a network activity, such as starting a TCP connection. In a multi hop network
* activity, the source represents the sender of the last hop.
* @param source source or {@code null} for none
*/
public AttributeContext setSource(Peer source) {
this.source = source;
return this;
}
@Override
public AttributeContext set(String fieldName, Object value) {
return (AttributeContext) super.set(fieldName, value);
}
@Override
public AttributeContext clone() {
return (AttributeContext) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy