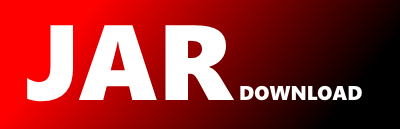
target.apidocs.com.google.api.services.servicemanagement.model.QuotaLimit.html Maven / Gradle / Ivy
QuotaLimit (Service Management API v1-rev20240823-2.0.0)
com.google.api.services.servicemanagement.model
Class QuotaLimit
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.servicemanagement.model.QuotaLimit
-
public final class QuotaLimit
extends com.google.api.client.json.GenericJson
`QuotaLimit` defines a specific limit that applies over a specified duration for a limit type.
There can be at most one limit for a duration and limit type combination defined within a
`QuotaGroup`.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Service Management API. For a detailed explanation
see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
QuotaLimit()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
QuotaLimit
clone()
Long
getDefaultLimit()
Default number of tokens that can be consumed during the specified duration.
String
getDescription()
Optional.
String
getDisplayName()
User-visible display name for this limit.
String
getDuration()
Duration of this limit in textual notation.
Long
getFreeTier()
Free tier value displayed in the Developers Console for this limit.
Long
getMaxLimit()
Maximum number of tokens that can be consumed during the specified duration.
String
getMetric()
The name of the metric this quota limit applies to.
String
getName()
Name of the quota limit.
String
getUnit()
Specify the unit of the quota limit.
Map<String,Long>
getValues()
Tiered limit values.
QuotaLimit
set(String fieldName,
Object value)
QuotaLimit
setDefaultLimit(Long defaultLimit)
Default number of tokens that can be consumed during the specified duration.
QuotaLimit
setDescription(String description)
Optional.
QuotaLimit
setDisplayName(String displayName)
User-visible display name for this limit.
QuotaLimit
setDuration(String duration)
Duration of this limit in textual notation.
QuotaLimit
setFreeTier(Long freeTier)
Free tier value displayed in the Developers Console for this limit.
QuotaLimit
setMaxLimit(Long maxLimit)
Maximum number of tokens that can be consumed during the specified duration.
QuotaLimit
setMetric(String metric)
The name of the metric this quota limit applies to.
QuotaLimit
setName(String name)
Name of the quota limit.
QuotaLimit
setUnit(String unit)
Specify the unit of the quota limit.
QuotaLimit
setValues(Map<String,Long> values)
Tiered limit values.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getDefaultLimit
public Long getDefaultLimit()
Default number of tokens that can be consumed during the specified duration. This is the number
of tokens assigned when a client application developer activates the service for his/her
project. Specifying a value of 0 will block all requests. This can be used if you are
provisioning quota to selected consumers and blocking others. Similarly, a value of -1 will
indicate an unlimited quota. No other negative values are allowed. Used by group-based quotas
only.
- Returns:
- value or
null
for none
-
setDefaultLimit
public QuotaLimit setDefaultLimit(Long defaultLimit)
Default number of tokens that can be consumed during the specified duration. This is the number
of tokens assigned when a client application developer activates the service for his/her
project. Specifying a value of 0 will block all requests. This can be used if you are
provisioning quota to selected consumers and blocking others. Similarly, a value of -1 will
indicate an unlimited quota. No other negative values are allowed. Used by group-based quotas
only.
- Parameters:
defaultLimit
- defaultLimit or null
for none
-
getDescription
public String getDescription()
Optional. User-visible, extended description for this quota limit. Should be used only when
more context is needed to understand this limit than provided by the limit's display name (see:
`display_name`).
- Returns:
- value or
null
for none
-
setDescription
public QuotaLimit setDescription(String description)
Optional. User-visible, extended description for this quota limit. Should be used only when
more context is needed to understand this limit than provided by the limit's display name (see:
`display_name`).
- Parameters:
description
- description or null
for none
-
getDisplayName
public String getDisplayName()
User-visible display name for this limit. Optional. If not set, the UI will provide a default
display name based on the quota configuration. This field can be used to override the default
display name generated from the configuration.
- Returns:
- value or
null
for none
-
setDisplayName
public QuotaLimit setDisplayName(String displayName)
User-visible display name for this limit. Optional. If not set, the UI will provide a default
display name based on the quota configuration. This field can be used to override the default
display name generated from the configuration.
- Parameters:
displayName
- displayName or null
for none
-
getDuration
public String getDuration()
Duration of this limit in textual notation. Must be "100s" or "1d". Used by group-based quotas
only.
- Returns:
- value or
null
for none
-
setDuration
public QuotaLimit setDuration(String duration)
Duration of this limit in textual notation. Must be "100s" or "1d". Used by group-based quotas
only.
- Parameters:
duration
- duration or null
for none
-
getFreeTier
public Long getFreeTier()
Free tier value displayed in the Developers Console for this limit. The free tier is the number
of tokens that will be subtracted from the billed amount when billing is enabled. This field
can only be set on a limit with duration "1d", in a billable group; it is invalid on any other
limit. If this field is not set, it defaults to 0, indicating that there is no free tier for
this service. Used by group-based quotas only.
- Returns:
- value or
null
for none
-
setFreeTier
public QuotaLimit setFreeTier(Long freeTier)
Free tier value displayed in the Developers Console for this limit. The free tier is the number
of tokens that will be subtracted from the billed amount when billing is enabled. This field
can only be set on a limit with duration "1d", in a billable group; it is invalid on any other
limit. If this field is not set, it defaults to 0, indicating that there is no free tier for
this service. Used by group-based quotas only.
- Parameters:
freeTier
- freeTier or null
for none
-
getMaxLimit
public Long getMaxLimit()
Maximum number of tokens that can be consumed during the specified duration. Client application
developers can override the default limit up to this maximum. If specified, this value cannot
be set to a value less than the default limit. If not specified, it is set to the default
limit. To allow clients to apply overrides with no upper bound, set this to -1, indicating
unlimited maximum quota. Used by group-based quotas only.
- Returns:
- value or
null
for none
-
setMaxLimit
public QuotaLimit setMaxLimit(Long maxLimit)
Maximum number of tokens that can be consumed during the specified duration. Client application
developers can override the default limit up to this maximum. If specified, this value cannot
be set to a value less than the default limit. If not specified, it is set to the default
limit. To allow clients to apply overrides with no upper bound, set this to -1, indicating
unlimited maximum quota. Used by group-based quotas only.
- Parameters:
maxLimit
- maxLimit or null
for none
-
getMetric
public String getMetric()
The name of the metric this quota limit applies to. The quota limits with the same metric will
be checked together during runtime. The metric must be defined within the service config.
- Returns:
- value or
null
for none
-
setMetric
public QuotaLimit setMetric(String metric)
The name of the metric this quota limit applies to. The quota limits with the same metric will
be checked together during runtime. The metric must be defined within the service config.
- Parameters:
metric
- metric or null
for none
-
getName
public String getName()
Name of the quota limit. The name must be provided, and it must be unique within the service.
The name can only include alphanumeric characters as well as '-'. The maximum length of the
limit name is 64 characters.
- Returns:
- value or
null
for none
-
setName
public QuotaLimit setName(String name)
Name of the quota limit. The name must be provided, and it must be unique within the service.
The name can only include alphanumeric characters as well as '-'. The maximum length of the
limit name is 64 characters.
- Parameters:
name
- name or null
for none
-
getUnit
public String getUnit()
Specify the unit of the quota limit. It uses the same syntax as Metric.unit. The supported unit
kinds are determined by the quota backend system. Here are some examples: * "1/min/{project}"
for quota per minute per project. Note: the order of unit components is insignificant. The "1"
at the beginning is required to follow the metric unit syntax.
- Returns:
- value or
null
for none
-
setUnit
public QuotaLimit setUnit(String unit)
Specify the unit of the quota limit. It uses the same syntax as Metric.unit. The supported unit
kinds are determined by the quota backend system. Here are some examples: * "1/min/{project}"
for quota per minute per project. Note: the order of unit components is insignificant. The "1"
at the beginning is required to follow the metric unit syntax.
- Parameters:
unit
- unit or null
for none
-
getValues
public Map<String,Long> getValues()
Tiered limit values. You must specify this as a key:value pair, with an integer value that is
the maximum number of requests allowed for the specified unit. Currently only STANDARD is
supported.
- Returns:
- value or
null
for none
-
setValues
public QuotaLimit setValues(Map<String,Long> values)
Tiered limit values. You must specify this as a key:value pair, with an integer value that is
the maximum number of requests allowed for the specified unit. Currently only STANDARD is
supported.
- Parameters:
values
- values or null
for none
-
set
public QuotaLimit set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public QuotaLimit clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2024 Weber Informatics LLC | Privacy Policy