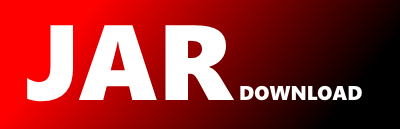
target.apidocs.com.google.api.services.servicemanagement.model.Service.html Maven / Gradle / Ivy
Service (Service Management API v1-rev20240823-2.0.0)
com.google.api.services.servicemanagement.model
Class Service
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.servicemanagement.model.Service
-
public final class Service
extends com.google.api.client.json.GenericJson
`Service` is the root object of Google API service configuration (service config). It describes
the basic information about a logical service, such as the service name and the user-facing
title, and delegates other aspects to sub-sections. Each sub-section is either a proto message or
a repeated proto message that configures a specific aspect, such as auth. For more information,
see each proto message definition. Example: type: google.api.Service name:
calendar.googleapis.com title: Google Calendar API apis: - name: google.calendar.v3.Calendar
visibility: rules: - selector: "google.calendar.v3.*" restriction: PREVIEW backend: rules: -
selector: "google.calendar.v3.*" address: calendar.example.com authentication: providers: - id:
google_calendar_auth jwks_uri: https://www.googleapis.com/oauth2/v1/certs issuer:
https://securetoken.google.com rules: - selector: "*" requirements: provider_id:
google_calendar_auth
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Service Management API. For a detailed explanation
see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
Service()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Service
clone()
List<Api>
getApis()
A list of API interfaces exported by this service.
Authentication
getAuthentication()
Auth configuration.
Backend
getBackend()
API backend configuration.
Billing
getBilling()
Billing configuration.
Long
getConfigVersion()
Obsolete.
Context
getContext()
Context configuration.
Control
getControl()
Configuration for the service control plane.
CustomError
getCustomError()
Custom error configuration.
Documentation
getDocumentation()
Additional API documentation.
List<Endpoint>
getEndpoints()
Configuration for network endpoints.
List<ServiceManagementEnum>
getEnums()
A list of all enum types included in this API service.
Http
getHttp()
HTTP configuration.
String
getId()
A unique ID for a specific instance of this message, typically assigned by the client for
tracking purpose.
Logging
getLogging()
Logging configuration.
List<LogDescriptor>
getLogs()
Defines the logs used by this service.
List<MetricDescriptor>
getMetrics()
Defines the metrics used by this service.
List<MonitoredResourceDescriptor>
getMonitoredResources()
Defines the monitored resources used by this service.
Monitoring
getMonitoring()
Monitoring configuration.
String
getName()
The service name, which is a DNS-like logical identifier for the service, such as
`calendar.googleapis.com`.
String
getProducerProjectId()
The Google project that owns this service.
Publishing
getPublishing()
Settings for [Google Cloud Client libraries](https://cloud.google.com/apis/docs/cloud-client-
libraries) generated from APIs defined as protocol buffers.
Quota
getQuota()
Quota configuration.
SourceInfo
getSourceInfo()
Output only.
SystemParameters
getSystemParameters()
System parameter configuration.
List<Type>
getSystemTypes()
A list of all proto message types included in this API service.
String
getTitle()
The product title for this service, it is the name displayed in Google Cloud Console.
List<Type>
getTypes()
A list of all proto message types included in this API service.
Usage
getUsage()
Configuration controlling usage of this service.
Service
set(String fieldName,
Object value)
Service
setApis(List<Api> apis)
A list of API interfaces exported by this service.
Service
setAuthentication(Authentication authentication)
Auth configuration.
Service
setBackend(Backend backend)
API backend configuration.
Service
setBilling(Billing billing)
Billing configuration.
Service
setConfigVersion(Long configVersion)
Obsolete.
Service
setContext(Context context)
Context configuration.
Service
setControl(Control control)
Configuration for the service control plane.
Service
setCustomError(CustomError customError)
Custom error configuration.
Service
setDocumentation(Documentation documentation)
Additional API documentation.
Service
setEndpoints(List<Endpoint> endpoints)
Configuration for network endpoints.
Service
setEnums(List<ServiceManagementEnum> enums)
A list of all enum types included in this API service.
Service
setHttp(Http http)
HTTP configuration.
Service
setId(String id)
A unique ID for a specific instance of this message, typically assigned by the client for
tracking purpose.
Service
setLogging(Logging logging)
Logging configuration.
Service
setLogs(List<LogDescriptor> logs)
Defines the logs used by this service.
Service
setMetrics(List<MetricDescriptor> metrics)
Defines the metrics used by this service.
Service
setMonitoredResources(List<MonitoredResourceDescriptor> monitoredResources)
Defines the monitored resources used by this service.
Service
setMonitoring(Monitoring monitoring)
Monitoring configuration.
Service
setName(String name)
The service name, which is a DNS-like logical identifier for the service, such as
`calendar.googleapis.com`.
Service
setProducerProjectId(String producerProjectId)
The Google project that owns this service.
Service
setPublishing(Publishing publishing)
Settings for [Google Cloud Client libraries](https://cloud.google.com/apis/docs/cloud-client-
libraries) generated from APIs defined as protocol buffers.
Service
setQuota(Quota quota)
Quota configuration.
Service
setSourceInfo(SourceInfo sourceInfo)
Output only.
Service
setSystemParameters(SystemParameters systemParameters)
System parameter configuration.
Service
setSystemTypes(List<Type> systemTypes)
A list of all proto message types included in this API service.
Service
setTitle(String title)
The product title for this service, it is the name displayed in Google Cloud Console.
Service
setTypes(List<Type> types)
A list of all proto message types included in this API service.
Service
setUsage(Usage usage)
Configuration controlling usage of this service.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getApis
public List<Api> getApis()
A list of API interfaces exported by this service. Only the `name` field of the
google.protobuf.Api needs to be provided by the configuration author, as the remaining fields
will be derived from the IDL during the normalization process. It is an error to specify an API
interface here which cannot be resolved against the associated IDL files.
- Returns:
- value or
null
for none
-
setApis
public Service setApis(List<Api> apis)
A list of API interfaces exported by this service. Only the `name` field of the
google.protobuf.Api needs to be provided by the configuration author, as the remaining fields
will be derived from the IDL during the normalization process. It is an error to specify an API
interface here which cannot be resolved against the associated IDL files.
- Parameters:
apis
- apis or null
for none
-
getAuthentication
public Authentication getAuthentication()
Auth configuration.
- Returns:
- value or
null
for none
-
setAuthentication
public Service setAuthentication(Authentication authentication)
Auth configuration.
- Parameters:
authentication
- authentication or null
for none
-
getBackend
public Backend getBackend()
API backend configuration.
- Returns:
- value or
null
for none
-
setBackend
public Service setBackend(Backend backend)
API backend configuration.
- Parameters:
backend
- backend or null
for none
-
getBilling
public Billing getBilling()
Billing configuration.
- Returns:
- value or
null
for none
-
setBilling
public Service setBilling(Billing billing)
Billing configuration.
- Parameters:
billing
- billing or null
for none
-
getConfigVersion
public Long getConfigVersion()
Obsolete. Do not use. This field has no semantic meaning. The service config compiler always
sets this field to `3`.
- Returns:
- value or
null
for none
-
setConfigVersion
public Service setConfigVersion(Long configVersion)
Obsolete. Do not use. This field has no semantic meaning. The service config compiler always
sets this field to `3`.
- Parameters:
configVersion
- configVersion or null
for none
-
getContext
public Context getContext()
Context configuration.
- Returns:
- value or
null
for none
-
setContext
public Service setContext(Context context)
Context configuration.
- Parameters:
context
- context or null
for none
-
getControl
public Control getControl()
Configuration for the service control plane.
- Returns:
- value or
null
for none
-
setControl
public Service setControl(Control control)
Configuration for the service control plane.
- Parameters:
control
- control or null
for none
-
getCustomError
public CustomError getCustomError()
Custom error configuration.
- Returns:
- value or
null
for none
-
setCustomError
public Service setCustomError(CustomError customError)
Custom error configuration.
- Parameters:
customError
- customError or null
for none
-
getDocumentation
public Documentation getDocumentation()
Additional API documentation.
- Returns:
- value or
null
for none
-
setDocumentation
public Service setDocumentation(Documentation documentation)
Additional API documentation.
- Parameters:
documentation
- documentation or null
for none
-
getEndpoints
public List<Endpoint> getEndpoints()
Configuration for network endpoints. If this is empty, then an endpoint with the same name as
the service is automatically generated to service all defined APIs.
- Returns:
- value or
null
for none
-
setEndpoints
public Service setEndpoints(List<Endpoint> endpoints)
Configuration for network endpoints. If this is empty, then an endpoint with the same name as
the service is automatically generated to service all defined APIs.
- Parameters:
endpoints
- endpoints or null
for none
-
getEnums
public List<ServiceManagementEnum> getEnums()
A list of all enum types included in this API service. Enums referenced directly or indirectly
by the `apis` are automatically included. Enums which are not referenced but shall be included
should be listed here by name by the configuration author. Example: enums: - name:
google.someapi.v1.SomeEnum
- Returns:
- value or
null
for none
-
setEnums
public Service setEnums(List<ServiceManagementEnum> enums)
A list of all enum types included in this API service. Enums referenced directly or indirectly
by the `apis` are automatically included. Enums which are not referenced but shall be included
should be listed here by name by the configuration author. Example: enums: - name:
google.someapi.v1.SomeEnum
- Parameters:
enums
- enums or null
for none
-
getHttp
public Http getHttp()
HTTP configuration.
- Returns:
- value or
null
for none
-
setHttp
public Service setHttp(Http http)
HTTP configuration.
- Parameters:
http
- http or null
for none
-
getId
public String getId()
A unique ID for a specific instance of this message, typically assigned by the client for
tracking purpose. Must be no longer than 63 characters and only lower case letters, digits,
'.', '_' and '-' are allowed. If empty, the server may choose to generate one instead.
- Returns:
- value or
null
for none
-
setId
public Service setId(String id)
A unique ID for a specific instance of this message, typically assigned by the client for
tracking purpose. Must be no longer than 63 characters and only lower case letters, digits,
'.', '_' and '-' are allowed. If empty, the server may choose to generate one instead.
- Parameters:
id
- id or null
for none
-
getLogging
public Logging getLogging()
Logging configuration.
- Returns:
- value or
null
for none
-
setLogging
public Service setLogging(Logging logging)
Logging configuration.
- Parameters:
logging
- logging or null
for none
-
getLogs
public List<LogDescriptor> getLogs()
Defines the logs used by this service.
- Returns:
- value or
null
for none
-
setLogs
public Service setLogs(List<LogDescriptor> logs)
Defines the logs used by this service.
- Parameters:
logs
- logs or null
for none
-
getMetrics
public List<MetricDescriptor> getMetrics()
Defines the metrics used by this service.
- Returns:
- value or
null
for none
-
setMetrics
public Service setMetrics(List<MetricDescriptor> metrics)
Defines the metrics used by this service.
- Parameters:
metrics
- metrics or null
for none
-
getMonitoredResources
public List<MonitoredResourceDescriptor> getMonitoredResources()
Defines the monitored resources used by this service. This is required by the
Service.monitoring and Service.logging configurations.
- Returns:
- value or
null
for none
-
setMonitoredResources
public Service setMonitoredResources(List<MonitoredResourceDescriptor> monitoredResources)
Defines the monitored resources used by this service. This is required by the
Service.monitoring and Service.logging configurations.
- Parameters:
monitoredResources
- monitoredResources or null
for none
-
getMonitoring
public Monitoring getMonitoring()
Monitoring configuration.
- Returns:
- value or
null
for none
-
setMonitoring
public Service setMonitoring(Monitoring monitoring)
Monitoring configuration.
- Parameters:
monitoring
- monitoring or null
for none
-
getName
public String getName()
The service name, which is a DNS-like logical identifier for the service, such as
`calendar.googleapis.com`. The service name typically goes through DNS verification to make
sure the owner of the service also owns the DNS name.
- Returns:
- value or
null
for none
-
setName
public Service setName(String name)
The service name, which is a DNS-like logical identifier for the service, such as
`calendar.googleapis.com`. The service name typically goes through DNS verification to make
sure the owner of the service also owns the DNS name.
- Parameters:
name
- name or null
for none
-
getProducerProjectId
public String getProducerProjectId()
The Google project that owns this service.
- Returns:
- value or
null
for none
-
setProducerProjectId
public Service setProducerProjectId(String producerProjectId)
The Google project that owns this service.
- Parameters:
producerProjectId
- producerProjectId or null
for none
-
getPublishing
public Publishing getPublishing()
Settings for [Google Cloud Client libraries](https://cloud.google.com/apis/docs/cloud-client-
libraries) generated from APIs defined as protocol buffers.
- Returns:
- value or
null
for none
-
setPublishing
public Service setPublishing(Publishing publishing)
Settings for [Google Cloud Client libraries](https://cloud.google.com/apis/docs/cloud-client-
libraries) generated from APIs defined as protocol buffers.
- Parameters:
publishing
- publishing or null
for none
-
getQuota
public Quota getQuota()
Quota configuration.
- Returns:
- value or
null
for none
-
setQuota
public Service setQuota(Quota quota)
Quota configuration.
- Parameters:
quota
- quota or null
for none
-
getSourceInfo
public SourceInfo getSourceInfo()
Output only. The source information for this configuration if available.
- Returns:
- value or
null
for none
-
setSourceInfo
public Service setSourceInfo(SourceInfo sourceInfo)
Output only. The source information for this configuration if available.
- Parameters:
sourceInfo
- sourceInfo or null
for none
-
getSystemParameters
public SystemParameters getSystemParameters()
System parameter configuration.
- Returns:
- value or
null
for none
-
setSystemParameters
public Service setSystemParameters(SystemParameters systemParameters)
System parameter configuration.
- Parameters:
systemParameters
- systemParameters or null
for none
-
getSystemTypes
public List<Type> getSystemTypes()
A list of all proto message types included in this API service. It serves similar purpose as
[google.api.Service.types], except that these types are not needed by user-defined APIs.
Therefore, they will not show up in the generated discovery doc. This field should only be used
to define system APIs in ESF.
- Returns:
- value or
null
for none
-
setSystemTypes
public Service setSystemTypes(List<Type> systemTypes)
A list of all proto message types included in this API service. It serves similar purpose as
[google.api.Service.types], except that these types are not needed by user-defined APIs.
Therefore, they will not show up in the generated discovery doc. This field should only be used
to define system APIs in ESF.
- Parameters:
systemTypes
- systemTypes or null
for none
-
getTitle
public String getTitle()
The product title for this service, it is the name displayed in Google Cloud Console.
- Returns:
- value or
null
for none
-
setTitle
public Service setTitle(String title)
The product title for this service, it is the name displayed in Google Cloud Console.
- Parameters:
title
- title or null
for none
-
getTypes
public List<Type> getTypes()
A list of all proto message types included in this API service. Types referenced directly or
indirectly by the `apis` are automatically included. Messages which are not referenced but
shall be included, such as types used by the `google.protobuf.Any` type, should be listed here
by name by the configuration author. Example: types: - name: google.protobuf.Int32
- Returns:
- value or
null
for none
-
setTypes
public Service setTypes(List<Type> types)
A list of all proto message types included in this API service. Types referenced directly or
indirectly by the `apis` are automatically included. Messages which are not referenced but
shall be included, such as types used by the `google.protobuf.Any` type, should be listed here
by name by the configuration author. Example: types: - name: google.protobuf.Int32
- Parameters:
types
- types or null
for none
-
getUsage
public Usage getUsage()
Configuration controlling usage of this service.
- Returns:
- value or
null
for none
-
setUsage
public Service setUsage(Usage usage)
Configuration controlling usage of this service.
- Parameters:
usage
- usage or null
for none
-
set
public Service set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public Service clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2024 Weber Informatics LLC | Privacy Policy