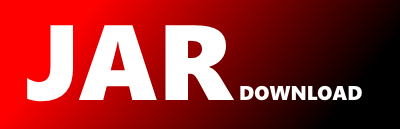
target.apidocs.com.google.api.services.servicemanagement.model.Api.html Maven / Gradle / Ivy
Api (Service Management API v1-rev20241202-2.0.0)
com.google.api.services.servicemanagement.model
Class Api
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.servicemanagement.model.Api
-
public final class Api
extends com.google.api.client.json.GenericJson
Api is a light-weight descriptor for an API Interface. Interfaces are also described as "protocol
buffer services" in some contexts, such as by the "service" keyword in a .proto file, but they
are different from API Services, which represent a concrete implementation of an interface as
opposed to simply a description of methods and bindings. They are also sometimes simply referred
to as "APIs" in other contexts, such as the name of this message itself. See
https://cloud.google.com/apis/design/glossary for detailed terminology.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Service Management API. For a detailed explanation
see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
Api()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Api
clone()
List<Method>
getMethods()
The methods of this interface, in unspecified order.
List<Mixin>
getMixins()
Included interfaces.
String
getName()
The fully qualified name of this interface, including package name followed by the interface's
simple name.
List<Option>
getOptions()
Any metadata attached to the interface.
SourceContext
getSourceContext()
Source context for the protocol buffer service represented by this message.
String
getSyntax()
The source syntax of the service.
String
getVersion()
A version string for this interface.
Api
set(String fieldName,
Object value)
Api
setMethods(List<Method> methods)
The methods of this interface, in unspecified order.
Api
setMixins(List<Mixin> mixins)
Included interfaces.
Api
setName(String name)
The fully qualified name of this interface, including package name followed by the interface's
simple name.
Api
setOptions(List<Option> options)
Any metadata attached to the interface.
Api
setSourceContext(SourceContext sourceContext)
Source context for the protocol buffer service represented by this message.
Api
setSyntax(String syntax)
The source syntax of the service.
Api
setVersion(String version)
A version string for this interface.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getMethods
public List<Method> getMethods()
The methods of this interface, in unspecified order.
- Returns:
- value or
null
for none
-
setMethods
public Api setMethods(List<Method> methods)
The methods of this interface, in unspecified order.
- Parameters:
methods
- methods or null
for none
-
getMixins
public List<Mixin> getMixins()
Included interfaces. See Mixin.
- Returns:
- value or
null
for none
-
setMixins
public Api setMixins(List<Mixin> mixins)
Included interfaces. See Mixin.
- Parameters:
mixins
- mixins or null
for none
-
getName
public String getName()
The fully qualified name of this interface, including package name followed by the interface's
simple name.
- Returns:
- value or
null
for none
-
setName
public Api setName(String name)
The fully qualified name of this interface, including package name followed by the interface's
simple name.
- Parameters:
name
- name or null
for none
-
getOptions
public List<Option> getOptions()
Any metadata attached to the interface.
- Returns:
- value or
null
for none
-
setOptions
public Api setOptions(List<Option> options)
Any metadata attached to the interface.
- Parameters:
options
- options or null
for none
-
getSourceContext
public SourceContext getSourceContext()
Source context for the protocol buffer service represented by this message.
- Returns:
- value or
null
for none
-
setSourceContext
public Api setSourceContext(SourceContext sourceContext)
Source context for the protocol buffer service represented by this message.
- Parameters:
sourceContext
- sourceContext or null
for none
-
getSyntax
public String getSyntax()
The source syntax of the service.
- Returns:
- value or
null
for none
-
setSyntax
public Api setSyntax(String syntax)
The source syntax of the service.
- Parameters:
syntax
- syntax or null
for none
-
getVersion
public String getVersion()
A version string for this interface. If specified, must have the form `major-version.minor-
version`, as in `1.10`. If the minor version is omitted, it defaults to zero. If the entire
version field is empty, the major version is derived from the package name, as outlined below.
If the field is not empty, the version in the package name will be verified to be consistent
with what is provided here. The versioning schema uses [semantic versioning](http://semver.org)
where the major version number indicates a breaking change and the minor version an additive,
non-breaking change. Both version numbers are signals to users what to expect from different
versions, and should be carefully chosen based on the product plan. The major version is also
reflected in the package name of the interface, which must end in `v`, as in
`google.feature.v1`. For major versions 0 and 1, the suffix can be omitted. Zero major versions
must only be used for experimental, non-GA interfaces.
- Returns:
- value or
null
for none
-
setVersion
public Api setVersion(String version)
A version string for this interface. If specified, must have the form `major-version.minor-
version`, as in `1.10`. If the minor version is omitted, it defaults to zero. If the entire
version field is empty, the major version is derived from the package name, as outlined below.
If the field is not empty, the version in the package name will be verified to be consistent
with what is provided here. The versioning schema uses [semantic versioning](http://semver.org)
where the major version number indicates a breaking change and the minor version an additive,
non-breaking change. Both version numbers are signals to users what to expect from different
versions, and should be carefully chosen based on the product plan. The major version is also
reflected in the package name of the interface, which must end in `v`, as in
`google.feature.v1`. For major versions 0 and 1, the suffix can be omitted. Zero major versions
must only be used for experimental, non-GA interfaces.
- Parameters:
version
- version or null
for none
-
set
public Api set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public Api clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2024 Weber Informatics LLC | Privacy Policy