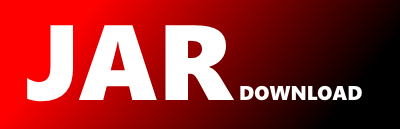
target.apidocs.com.google.api.services.servicemanagement.model.AuthProvider.html Maven / Gradle / Ivy
AuthProvider (Service Management API v1-rev20241202-2.0.0)
com.google.api.services.servicemanagement.model
Class AuthProvider
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.servicemanagement.model.AuthProvider
-
public final class AuthProvider
extends com.google.api.client.json.GenericJson
Configuration for an authentication provider, including support for [JSON Web Token
(JWT)](https://tools.ietf.org/html/draft-ietf-oauth-json-web-token-32).
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Service Management API. For a detailed explanation
see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
AuthProvider()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
AuthProvider
clone()
String
getAudiences()
The list of JWT [audiences](https://tools.ietf.org/html/draft-ietf-oauth-json-web-
token-32#section-4.1.3).
String
getAuthorizationUrl()
Redirect URL if JWT token is required but not present or is expired.
String
getId()
The unique identifier of the auth provider.
String
getIssuer()
Identifies the principal that issued the JWT.
String
getJwksUri()
URL of the provider's public key set to validate signature of the JWT.
List<JwtLocation>
getJwtLocations()
Defines the locations to extract the JWT.
AuthProvider
set(String fieldName,
Object value)
AuthProvider
setAudiences(String audiences)
The list of JWT [audiences](https://tools.ietf.org/html/draft-ietf-oauth-json-web-
token-32#section-4.1.3).
AuthProvider
setAuthorizationUrl(String authorizationUrl)
Redirect URL if JWT token is required but not present or is expired.
AuthProvider
setId(String id)
The unique identifier of the auth provider.
AuthProvider
setIssuer(String issuer)
Identifies the principal that issued the JWT.
AuthProvider
setJwksUri(String jwksUri)
URL of the provider's public key set to validate signature of the JWT.
AuthProvider
setJwtLocations(List<JwtLocation> jwtLocations)
Defines the locations to extract the JWT.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getAudiences
public String getAudiences()
The list of JWT [audiences](https://tools.ietf.org/html/draft-ietf-oauth-json-web-
token-32#section-4.1.3). that are allowed to access. A JWT containing any of these audiences
will be accepted. When this setting is absent, JWTs with audiences: -
"https://[service.name]/[google.protobuf.Api.name]" - "https://[service.name]/" will be
accepted. For example, if no audiences are in the setting, LibraryService API will accept JWTs
with the following audiences: - https://library-
example.googleapis.com/google.example.library.v1.LibraryService - https://library-
example.googleapis.com/ Example: audiences: bookstore_android.apps.googleusercontent.com,
bookstore_web.apps.googleusercontent.com
- Returns:
- value or
null
for none
-
setAudiences
public AuthProvider setAudiences(String audiences)
The list of JWT [audiences](https://tools.ietf.org/html/draft-ietf-oauth-json-web-
token-32#section-4.1.3). that are allowed to access. A JWT containing any of these audiences
will be accepted. When this setting is absent, JWTs with audiences: -
"https://[service.name]/[google.protobuf.Api.name]" - "https://[service.name]/" will be
accepted. For example, if no audiences are in the setting, LibraryService API will accept JWTs
with the following audiences: - https://library-
example.googleapis.com/google.example.library.v1.LibraryService - https://library-
example.googleapis.com/ Example: audiences: bookstore_android.apps.googleusercontent.com,
bookstore_web.apps.googleusercontent.com
- Parameters:
audiences
- audiences or null
for none
-
getAuthorizationUrl
public String getAuthorizationUrl()
Redirect URL if JWT token is required but not present or is expired. Implement authorizationUrl
of securityDefinitions in OpenAPI spec.
- Returns:
- value or
null
for none
-
setAuthorizationUrl
public AuthProvider setAuthorizationUrl(String authorizationUrl)
Redirect URL if JWT token is required but not present or is expired. Implement authorizationUrl
of securityDefinitions in OpenAPI spec.
- Parameters:
authorizationUrl
- authorizationUrl or null
for none
-
getId
public String getId()
The unique identifier of the auth provider. It will be referred to by
`AuthRequirement.provider_id`. Example: "bookstore_auth".
- Returns:
- value or
null
for none
-
setId
public AuthProvider setId(String id)
The unique identifier of the auth provider. It will be referred to by
`AuthRequirement.provider_id`. Example: "bookstore_auth".
- Parameters:
id
- id or null
for none
-
getIssuer
public String getIssuer()
Identifies the principal that issued the JWT. See https://tools.ietf.org/html/draft-ietf-oauth-
json-web-token-32#section-4.1.1 Usually a URL or an email address. Example:
https://securetoken.google.com Example: [email protected]
- Returns:
- value or
null
for none
-
setIssuer
public AuthProvider setIssuer(String issuer)
Identifies the principal that issued the JWT. See https://tools.ietf.org/html/draft-ietf-oauth-
json-web-token-32#section-4.1.1 Usually a URL or an email address. Example:
https://securetoken.google.com Example: [email protected]
- Parameters:
issuer
- issuer or null
for none
-
getJwksUri
public String getJwksUri()
URL of the provider's public key set to validate signature of the JWT. See [OpenID
Discovery](https://openid.net/specs/openid-connect-discovery-1_0.html#ProviderMetadata).
Optional if the key set document: - can be retrieved from [OpenID
Discovery](https://openid.net/specs/openid-connect-discovery-1_0.html) of the issuer. - can be
inferred from the email domain of the issuer (e.g. a Google service account). Example:
https://www.googleapis.com/oauth2/v1/certs
- Returns:
- value or
null
for none
-
setJwksUri
public AuthProvider setJwksUri(String jwksUri)
URL of the provider's public key set to validate signature of the JWT. See [OpenID
Discovery](https://openid.net/specs/openid-connect-discovery-1_0.html#ProviderMetadata).
Optional if the key set document: - can be retrieved from [OpenID
Discovery](https://openid.net/specs/openid-connect-discovery-1_0.html) of the issuer. - can be
inferred from the email domain of the issuer (e.g. a Google service account). Example:
https://www.googleapis.com/oauth2/v1/certs
- Parameters:
jwksUri
- jwksUri or null
for none
-
getJwtLocations
public List<JwtLocation> getJwtLocations()
Defines the locations to extract the JWT. For now it is only used by the Cloud Endpoints to
store the OpenAPI extension [x-google-jwt-locations]
(https://cloud.google.com/endpoints/docs/openapi/openapi-extensions#x-google-jwt-locations) JWT
locations can be one of HTTP headers, URL query parameters or cookies. The rule is that the
first match wins. If not specified, default to use following 3 locations: 1) Authorization:
Bearer 2) x-goog-iap-jwt-assertion 3) access_token query parameter Default locations can be
specified as followings: jwt_locations: - header: Authorization value_prefix: "Bearer " -
header: x-goog-iap-jwt-assertion - query: access_token
- Returns:
- value or
null
for none
-
setJwtLocations
public AuthProvider setJwtLocations(List<JwtLocation> jwtLocations)
Defines the locations to extract the JWT. For now it is only used by the Cloud Endpoints to
store the OpenAPI extension [x-google-jwt-locations]
(https://cloud.google.com/endpoints/docs/openapi/openapi-extensions#x-google-jwt-locations) JWT
locations can be one of HTTP headers, URL query parameters or cookies. The rule is that the
first match wins. If not specified, default to use following 3 locations: 1) Authorization:
Bearer 2) x-goog-iap-jwt-assertion 3) access_token query parameter Default locations can be
specified as followings: jwt_locations: - header: Authorization value_prefix: "Bearer " -
header: x-goog-iap-jwt-assertion - query: access_token
- Parameters:
jwtLocations
- jwtLocations or null
for none
-
set
public AuthProvider set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public AuthProvider clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2024 Weber Informatics LLC | Privacy Policy