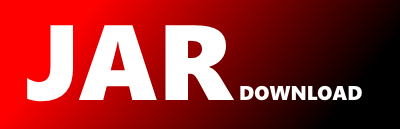
com.google.api.services.solar.v1.Solar Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.solar.v1;
/**
* Service definition for Solar (v1).
*
*
* Solar API.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link SolarRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class Solar extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Solar API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://solar.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://solar.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Solar(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
Solar(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the BuildingInsights collection.
*
* The typical use is:
*
* {@code Solar solar = new Solar(...);}
* {@code Solar.BuildingInsights.List request = solar.buildingInsights().list(parameters ...)}
*
*
* @return the resource collection
*/
public BuildingInsights buildingInsights() {
return new BuildingInsights();
}
/**
* The "buildingInsights" collection of methods.
*/
public class BuildingInsights {
/**
* Locates the closest building to a query point. Returns an error with code `NOT_FOUND` if there
* are no buildings within approximately 50m of the query point.
*
* Create a request for the method "buildingInsights.findClosest".
*
* This request holds the parameters needed by the solar server. After setting any optional
* parameters, call the {@link FindClosest#execute()} method to invoke the remote operation.
*
* @return the request
*/
public FindClosest findClosest() throws java.io.IOException {
FindClosest result = new FindClosest();
initialize(result);
return result;
}
public class FindClosest extends SolarRequest {
private static final String REST_PATH = "v1/buildingInsights:findClosest";
/**
* Locates the closest building to a query point. Returns an error with code `NOT_FOUND` if there
* are no buildings within approximately 50m of the query point.
*
* Create a request for the method "buildingInsights.findClosest".
*
* This request holds the parameters needed by the the solar server. After setting any optional
* parameters, call the {@link FindClosest#execute()} method to invoke the remote operation.
* {@link
* FindClosest#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected FindClosest() {
super(Solar.this, "GET", REST_PATH, null, com.google.api.services.solar.v1.model.BuildingInsights.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public FindClosest set$Xgafv(java.lang.String $Xgafv) {
return (FindClosest) super.set$Xgafv($Xgafv);
}
@Override
public FindClosest setAccessToken(java.lang.String accessToken) {
return (FindClosest) super.setAccessToken(accessToken);
}
@Override
public FindClosest setAlt(java.lang.String alt) {
return (FindClosest) super.setAlt(alt);
}
@Override
public FindClosest setCallback(java.lang.String callback) {
return (FindClosest) super.setCallback(callback);
}
@Override
public FindClosest setFields(java.lang.String fields) {
return (FindClosest) super.setFields(fields);
}
@Override
public FindClosest setKey(java.lang.String key) {
return (FindClosest) super.setKey(key);
}
@Override
public FindClosest setOauthToken(java.lang.String oauthToken) {
return (FindClosest) super.setOauthToken(oauthToken);
}
@Override
public FindClosest setPrettyPrint(java.lang.Boolean prettyPrint) {
return (FindClosest) super.setPrettyPrint(prettyPrint);
}
@Override
public FindClosest setQuotaUser(java.lang.String quotaUser) {
return (FindClosest) super.setQuotaUser(quotaUser);
}
@Override
public FindClosest setUploadType(java.lang.String uploadType) {
return (FindClosest) super.setUploadType(uploadType);
}
@Override
public FindClosest setUploadProtocol(java.lang.String uploadProtocol) {
return (FindClosest) super.setUploadProtocol(uploadProtocol);
}
/** Optional. Specifies the pre-GA features to enable. */
@com.google.api.client.util.Key
private java.util.List experiments;
/** Optional. Specifies the pre-GA features to enable.
*/
public java.util.List getExperiments() {
return experiments;
}
/** Optional. Specifies the pre-GA features to enable. */
public FindClosest setExperiments(java.util.List experiments) {
this.experiments = experiments;
return this;
}
/** The latitude in degrees. It must be in the range [-90.0, +90.0]. */
@com.google.api.client.util.Key("location.latitude")
private java.lang.Double locationLatitude;
/** The latitude in degrees. It must be in the range [-90.0, +90.0].
*/
public java.lang.Double getLocationLatitude() {
return locationLatitude;
}
/** The latitude in degrees. It must be in the range [-90.0, +90.0]. */
public FindClosest setLocationLatitude(java.lang.Double locationLatitude) {
this.locationLatitude = locationLatitude;
return this;
}
/** The longitude in degrees. It must be in the range [-180.0, +180.0]. */
@com.google.api.client.util.Key("location.longitude")
private java.lang.Double locationLongitude;
/** The longitude in degrees. It must be in the range [-180.0, +180.0].
*/
public java.lang.Double getLocationLongitude() {
return locationLongitude;
}
/** The longitude in degrees. It must be in the range [-180.0, +180.0]. */
public FindClosest setLocationLongitude(java.lang.Double locationLongitude) {
this.locationLongitude = locationLongitude;
return this;
}
/**
* Optional. The minimum quality level allowed in the results. No result with lower quality
* than this will be returned. Not specifying this is equivalent to restricting to HIGH
* quality only.
*/
@com.google.api.client.util.Key
private java.lang.String requiredQuality;
/** Optional. The minimum quality level allowed in the results. No result with lower quality than this
will be returned. Not specifying this is equivalent to restricting to HIGH quality only.
*/
public java.lang.String getRequiredQuality() {
return requiredQuality;
}
/**
* Optional. The minimum quality level allowed in the results. No result with lower quality
* than this will be returned. Not specifying this is equivalent to restricting to HIGH
* quality only.
*/
public FindClosest setRequiredQuality(java.lang.String requiredQuality) {
this.requiredQuality = requiredQuality;
return this;
}
@Override
public FindClosest set(String parameterName, Object value) {
return (FindClosest) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the DataLayers collection.
*
* The typical use is:
*
* {@code Solar solar = new Solar(...);}
* {@code Solar.DataLayers.List request = solar.dataLayers().list(parameters ...)}
*
*
* @return the resource collection
*/
public DataLayers dataLayers() {
return new DataLayers();
}
/**
* The "dataLayers" collection of methods.
*/
public class DataLayers {
/**
* Gets solar information for a region surrounding a location. Returns an error with code
* `NOT_FOUND` if the location is outside the coverage area.
*
* Create a request for the method "dataLayers.get".
*
* This request holds the parameters needed by the solar server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @return the request
*/
public Get get() throws java.io.IOException {
Get result = new Get();
initialize(result);
return result;
}
public class Get extends SolarRequest {
private static final String REST_PATH = "v1/dataLayers:get";
/**
* Gets solar information for a region surrounding a location. Returns an error with code
* `NOT_FOUND` if the location is outside the coverage area.
*
* Create a request for the method "dataLayers.get".
*
* This request holds the parameters needed by the the solar server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected Get() {
super(Solar.this, "GET", REST_PATH, null, com.google.api.services.solar.v1.model.DataLayers.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Optional. Whether to require exact quality of the imagery. If set to false, the
* `required_quality` field is interpreted as the minimum required quality, such that HIGH
* quality imagery may be returned when `required_quality` is set to MEDIUM. If set to true,
* `required_quality` is interpreted as the exact required quality and only `MEDIUM` quality
* imagery is returned if `required_quality` is set to `MEDIUM`.
*/
@com.google.api.client.util.Key
private java.lang.Boolean exactQualityRequired;
/** Optional. Whether to require exact quality of the imagery. If set to false, the `required_quality`
field is interpreted as the minimum required quality, such that HIGH quality imagery may be
returned when `required_quality` is set to MEDIUM. If set to true, `required_quality` is
interpreted as the exact required quality and only `MEDIUM` quality imagery is returned if
`required_quality` is set to `MEDIUM`.
*/
public java.lang.Boolean getExactQualityRequired() {
return exactQualityRequired;
}
/**
* Optional. Whether to require exact quality of the imagery. If set to false, the
* `required_quality` field is interpreted as the minimum required quality, such that HIGH
* quality imagery may be returned when `required_quality` is set to MEDIUM. If set to true,
* `required_quality` is interpreted as the exact required quality and only `MEDIUM` quality
* imagery is returned if `required_quality` is set to `MEDIUM`.
*/
public Get setExactQualityRequired(java.lang.Boolean exactQualityRequired) {
this.exactQualityRequired = exactQualityRequired;
return this;
}
/** Optional. Specifies the pre-GA experiments to enable. */
@com.google.api.client.util.Key
private java.util.List experiments;
/** Optional. Specifies the pre-GA experiments to enable.
*/
public java.util.List getExperiments() {
return experiments;
}
/** Optional. Specifies the pre-GA experiments to enable. */
public Get setExperiments(java.util.List experiments) {
this.experiments = experiments;
return this;
}
/** The latitude in degrees. It must be in the range [-90.0, +90.0]. */
@com.google.api.client.util.Key("location.latitude")
private java.lang.Double locationLatitude;
/** The latitude in degrees. It must be in the range [-90.0, +90.0].
*/
public java.lang.Double getLocationLatitude() {
return locationLatitude;
}
/** The latitude in degrees. It must be in the range [-90.0, +90.0]. */
public Get setLocationLatitude(java.lang.Double locationLatitude) {
this.locationLatitude = locationLatitude;
return this;
}
/** The longitude in degrees. It must be in the range [-180.0, +180.0]. */
@com.google.api.client.util.Key("location.longitude")
private java.lang.Double locationLongitude;
/** The longitude in degrees. It must be in the range [-180.0, +180.0].
*/
public java.lang.Double getLocationLongitude() {
return locationLongitude;
}
/** The longitude in degrees. It must be in the range [-180.0, +180.0]. */
public Get setLocationLongitude(java.lang.Double locationLongitude) {
this.locationLongitude = locationLongitude;
return this;
}
/**
* Optional. The minimum scale, in meters per pixel, of the data to return. Values of 0.1 (the
* default, if this field is not set explicitly), 0.25, 0.5, and 1.0 are supported. Imagery
* components whose normal resolution is less than `pixel_size_meters` will be returned at the
* resolution specified by `pixel_size_meters`; imagery components whose normal resolution is
* equal to or greater than `pixel_size_meters` will be returned at that normal resolution.
*/
@com.google.api.client.util.Key
private java.lang.Float pixelSizeMeters;
/** Optional. The minimum scale, in meters per pixel, of the data to return. Values of 0.1 (the
default, if this field is not set explicitly), 0.25, 0.5, and 1.0 are supported. Imagery components
whose normal resolution is less than `pixel_size_meters` will be returned at the resolution
specified by `pixel_size_meters`; imagery components whose normal resolution is equal to or greater
than `pixel_size_meters` will be returned at that normal resolution.
*/
public java.lang.Float getPixelSizeMeters() {
return pixelSizeMeters;
}
/**
* Optional. The minimum scale, in meters per pixel, of the data to return. Values of 0.1 (the
* default, if this field is not set explicitly), 0.25, 0.5, and 1.0 are supported. Imagery
* components whose normal resolution is less than `pixel_size_meters` will be returned at the
* resolution specified by `pixel_size_meters`; imagery components whose normal resolution is
* equal to or greater than `pixel_size_meters` will be returned at that normal resolution.
*/
public Get setPixelSizeMeters(java.lang.Float pixelSizeMeters) {
this.pixelSizeMeters = pixelSizeMeters;
return this;
}
/**
* Required. The radius, in meters, defining the region surrounding that centre point for
* which data should be returned. The limitations on this value are: * Any value up to 100m
* can always be specified. * Values over 100m can be specified, as long as `radius_meters` <=
* `pixel_size_meters * 1000`. * However, for values over 175m, the `DataLayerView` in the
* request must not include monthly flux or hourly shade.
*/
@com.google.api.client.util.Key
private java.lang.Float radiusMeters;
/** Required. The radius, in meters, defining the region surrounding that centre point for which data
should be returned. The limitations on this value are: * Any value up to 100m can always be
specified. * Values over 100m can be specified, as long as `radius_meters` <= `pixel_size_meters *
1000`. * However, for values over 175m, the `DataLayerView` in the request must not include monthly
flux or hourly shade.
*/
public java.lang.Float getRadiusMeters() {
return radiusMeters;
}
/**
* Required. The radius, in meters, defining the region surrounding that centre point for
* which data should be returned. The limitations on this value are: * Any value up to 100m
* can always be specified. * Values over 100m can be specified, as long as `radius_meters` <=
* `pixel_size_meters * 1000`. * However, for values over 175m, the `DataLayerView` in the
* request must not include monthly flux or hourly shade.
*/
public Get setRadiusMeters(java.lang.Float radiusMeters) {
this.radiusMeters = radiusMeters;
return this;
}
/**
* Optional. The minimum quality level allowed in the results. No result with lower quality
* than this will be returned. Not specifying this is equivalent to restricting to HIGH
* quality only.
*/
@com.google.api.client.util.Key
private java.lang.String requiredQuality;
/** Optional. The minimum quality level allowed in the results. No result with lower quality than this
will be returned. Not specifying this is equivalent to restricting to HIGH quality only.
*/
public java.lang.String getRequiredQuality() {
return requiredQuality;
}
/**
* Optional. The minimum quality level allowed in the results. No result with lower quality
* than this will be returned. Not specifying this is equivalent to restricting to HIGH
* quality only.
*/
public Get setRequiredQuality(java.lang.String requiredQuality) {
this.requiredQuality = requiredQuality;
return this;
}
/** Optional. The desired subset of the data to return. */
@com.google.api.client.util.Key
private java.lang.String view;
/** Optional. The desired subset of the data to return.
*/
public java.lang.String getView() {
return view;
}
/** Optional. The desired subset of the data to return. */
public Get setView(java.lang.String view) {
this.view = view;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the GeoTiff collection.
*
* The typical use is:
*
* {@code Solar solar = new Solar(...);}
* {@code Solar.GeoTiff.List request = solar.geoTiff().list(parameters ...)}
*
*
* @return the resource collection
*/
public GeoTiff geoTiff() {
return new GeoTiff();
}
/**
* The "geoTiff" collection of methods.
*/
public class GeoTiff {
/**
* Returns an image by its ID.
*
* Create a request for the method "geoTiff.get".
*
* This request holds the parameters needed by the solar server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @return the request
*/
public Get get() throws java.io.IOException {
Get result = new Get();
initialize(result);
return result;
}
public class Get extends SolarRequest {
private static final String REST_PATH = "v1/geoTiff:get";
/**
* Returns an image by its ID.
*
* Create a request for the method "geoTiff.get".
*
* This request holds the parameters needed by the the solar server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected Get() {
super(Solar.this, "GET", REST_PATH, null, com.google.api.services.solar.v1.model.HttpBody.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Required. The ID of the asset being requested. */
@com.google.api.client.util.Key
private java.lang.String id;
/** Required. The ID of the asset being requested.
*/
public java.lang.String getId() {
return id;
}
/** Required. The ID of the asset being requested. */
public Get setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
}
/**
* Builder for {@link Solar}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link Solar}. */
@Override
public Solar build() {
return new Solar(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link SolarRequestInitializer}.
*
* @since 1.12
*/
public Builder setSolarRequestInitializer(
SolarRequestInitializer solarRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(solarRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}