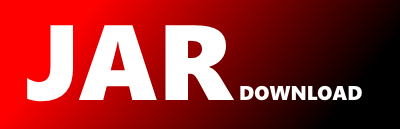
com.google.api.services.solar.v1.model.CashPurchaseSavings Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.solar.v1.model;
/**
* Cost and benefit of an outright purchase of a particular configuration of solar panels with a
* particular electricity usage.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Solar API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class CashPurchaseSavings extends com.google.api.client.json.GenericJson {
/**
* Initial cost before tax incentives: the amount that must be paid out-of-pocket. Contrast with
* `upfront_cost`, which is after tax incentives.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money outOfPocketCost;
/**
* Number of years until payback occurs. A negative value means payback never occurs within the
* lifetime period.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Float paybackYears;
/**
* The value of all tax rebates.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money rebateValue;
/**
* How much is saved (or not) over the lifetime period.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SavingsOverTime savings;
/**
* Initial cost after tax incentives: it's the amount that must be paid during first year.
* Contrast with `out_of_pocket_cost`, which is before tax incentives.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money upfrontCost;
/**
* Initial cost before tax incentives: the amount that must be paid out-of-pocket. Contrast with
* `upfront_cost`, which is after tax incentives.
* @return value or {@code null} for none
*/
public Money getOutOfPocketCost() {
return outOfPocketCost;
}
/**
* Initial cost before tax incentives: the amount that must be paid out-of-pocket. Contrast with
* `upfront_cost`, which is after tax incentives.
* @param outOfPocketCost outOfPocketCost or {@code null} for none
*/
public CashPurchaseSavings setOutOfPocketCost(Money outOfPocketCost) {
this.outOfPocketCost = outOfPocketCost;
return this;
}
/**
* Number of years until payback occurs. A negative value means payback never occurs within the
* lifetime period.
* @return value or {@code null} for none
*/
public java.lang.Float getPaybackYears() {
return paybackYears;
}
/**
* Number of years until payback occurs. A negative value means payback never occurs within the
* lifetime period.
* @param paybackYears paybackYears or {@code null} for none
*/
public CashPurchaseSavings setPaybackYears(java.lang.Float paybackYears) {
this.paybackYears = paybackYears;
return this;
}
/**
* The value of all tax rebates.
* @return value or {@code null} for none
*/
public Money getRebateValue() {
return rebateValue;
}
/**
* The value of all tax rebates.
* @param rebateValue rebateValue or {@code null} for none
*/
public CashPurchaseSavings setRebateValue(Money rebateValue) {
this.rebateValue = rebateValue;
return this;
}
/**
* How much is saved (or not) over the lifetime period.
* @return value or {@code null} for none
*/
public SavingsOverTime getSavings() {
return savings;
}
/**
* How much is saved (or not) over the lifetime period.
* @param savings savings or {@code null} for none
*/
public CashPurchaseSavings setSavings(SavingsOverTime savings) {
this.savings = savings;
return this;
}
/**
* Initial cost after tax incentives: it's the amount that must be paid during first year.
* Contrast with `out_of_pocket_cost`, which is before tax incentives.
* @return value or {@code null} for none
*/
public Money getUpfrontCost() {
return upfrontCost;
}
/**
* Initial cost after tax incentives: it's the amount that must be paid during first year.
* Contrast with `out_of_pocket_cost`, which is before tax incentives.
* @param upfrontCost upfrontCost or {@code null} for none
*/
public CashPurchaseSavings setUpfrontCost(Money upfrontCost) {
this.upfrontCost = upfrontCost;
return this;
}
@Override
public CashPurchaseSavings set(String fieldName, Object value) {
return (CashPurchaseSavings) super.set(fieldName, value);
}
@Override
public CashPurchaseSavings clone() {
return (CashPurchaseSavings) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy