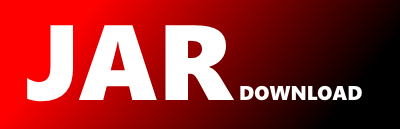
com.google.api.services.solar.v1.model.DataLayers Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.solar.v1.model;
/**
* Information about the solar potential of a region. The actual data are contained in a number of
* GeoTIFF files covering the requested region, for which this message contains URLs: Each string in
* the `DataLayers` message contains a URL from which the corresponding GeoTIFF can be fetched.
* These URLs are valid for a few hours after they've been generated. Most of the GeoTIFF files are
* at a resolution of 0.1m/pixel, but the monthly flux file is at 0.5m/pixel, and the hourly shade
* files are at 1m/pixel. If a `pixel_size_meters` value was specified in the
* `GetDataLayersRequest`, then the minimum resolution in the GeoTIFF files will be that value.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Solar API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class DataLayers extends com.google.api.client.json.GenericJson {
/**
* The URL for the annual flux map (annual sunlight on roofs) of the region. Values are
* kWh/kW/year. This is *unmasked flux*: flux is computed for every location, not just building
* rooftops. Invalid locations are stored as -9999: locations outside our coverage area will be
* invalid, and a few locations inside the coverage area, where we were unable to calculate flux,
* will also be invalid.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String annualFluxUrl;
/**
* The URL for an image of the DSM (Digital Surface Model) of the region. Values are in meters
* above EGM96 geoid (i.e., sea level). Invalid locations (where we don't have data) are stored as
* -9999.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String dsmUrl;
/**
* Twelve URLs for hourly shade, corresponding to January...December, in order. Each GeoTIFF will
* contain 24 bands, corresponding to the 24 hours of the day. Each pixel is a 32 bit integer,
* corresponding to the (up to) 31 days of that month; a 1 bit means that the corresponding
* location is able to see the sun at that day, of that hour, of that month. Invalid locations are
* stored as -9999 (since this is negative, it has bit 31 set, and no valid value could have bit
* 31 set as that would correspond to the 32nd day of the month). An example may be useful. If you
* want to know whether a point (at pixel location (x, y)) saw sun at 4pm on the 22nd of June you
* would: 1. fetch the sixth URL in this list (corresponding to June). 1. look up the 17th channel
* (corresponding to 4pm). 1. read the 32-bit value at (x, y). 1. read bit 21 of the value
* (corresponding to the 22nd of the month). 1. if that bit is a 1, then that spot saw the sun at
* 4pm 22 June. More formally: Given `month` (1-12), `day` (1...month max; February has 28 days)
* and `hour` (0-23), the shade/sun for that month/day/hour at a position `(x, y)` is the bit ```
* (hourly_shade[month - 1])(x, y)[hour] & (1 << (day - 1)) ``` where `(x, y)` is spatial
* indexing, `[month - 1]` refers to fetching the `month - 1`st URL (indexing from zero), `[hour]`
* is indexing into the channels, and a final non-zero result means "sunny". There are no leap
* days, and DST doesn't exist (all days are 24 hours long; noon is always "standard time" noon).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List hourlyShadeUrls;
/**
* When the source imagery (from which all the other data are derived) in this region was taken.
* It is necessarily somewhat approximate, as the images may have been taken over more than one
* day.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Date imageryDate;
/**
* When processing was completed on this imagery.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Date imageryProcessedDate;
/**
* The quality of the result's imagery.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String imageryQuality;
/**
* The URL for the building mask image: one bit per pixel saying whether that pixel is considered
* to be part of a rooftop or not.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String maskUrl;
/**
* The URL for the monthly flux map (sunlight on roofs, broken down by month) of the region.
* Values are kWh/kW/year. The GeoTIFF pointed to by this URL will contain twelve bands,
* corresponding to January...December, in order.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String monthlyFluxUrl;
/**
* The URL for an image of RGB data (aerial photo) of the region.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String rgbUrl;
/**
* The URL for the annual flux map (annual sunlight on roofs) of the region. Values are
* kWh/kW/year. This is *unmasked flux*: flux is computed for every location, not just building
* rooftops. Invalid locations are stored as -9999: locations outside our coverage area will be
* invalid, and a few locations inside the coverage area, where we were unable to calculate flux,
* will also be invalid.
* @return value or {@code null} for none
*/
public java.lang.String getAnnualFluxUrl() {
return annualFluxUrl;
}
/**
* The URL for the annual flux map (annual sunlight on roofs) of the region. Values are
* kWh/kW/year. This is *unmasked flux*: flux is computed for every location, not just building
* rooftops. Invalid locations are stored as -9999: locations outside our coverage area will be
* invalid, and a few locations inside the coverage area, where we were unable to calculate flux,
* will also be invalid.
* @param annualFluxUrl annualFluxUrl or {@code null} for none
*/
public DataLayers setAnnualFluxUrl(java.lang.String annualFluxUrl) {
this.annualFluxUrl = annualFluxUrl;
return this;
}
/**
* The URL for an image of the DSM (Digital Surface Model) of the region. Values are in meters
* above EGM96 geoid (i.e., sea level). Invalid locations (where we don't have data) are stored as
* -9999.
* @return value or {@code null} for none
*/
public java.lang.String getDsmUrl() {
return dsmUrl;
}
/**
* The URL for an image of the DSM (Digital Surface Model) of the region. Values are in meters
* above EGM96 geoid (i.e., sea level). Invalid locations (where we don't have data) are stored as
* -9999.
* @param dsmUrl dsmUrl or {@code null} for none
*/
public DataLayers setDsmUrl(java.lang.String dsmUrl) {
this.dsmUrl = dsmUrl;
return this;
}
/**
* Twelve URLs for hourly shade, corresponding to January...December, in order. Each GeoTIFF will
* contain 24 bands, corresponding to the 24 hours of the day. Each pixel is a 32 bit integer,
* corresponding to the (up to) 31 days of that month; a 1 bit means that the corresponding
* location is able to see the sun at that day, of that hour, of that month. Invalid locations are
* stored as -9999 (since this is negative, it has bit 31 set, and no valid value could have bit
* 31 set as that would correspond to the 32nd day of the month). An example may be useful. If you
* want to know whether a point (at pixel location (x, y)) saw sun at 4pm on the 22nd of June you
* would: 1. fetch the sixth URL in this list (corresponding to June). 1. look up the 17th channel
* (corresponding to 4pm). 1. read the 32-bit value at (x, y). 1. read bit 21 of the value
* (corresponding to the 22nd of the month). 1. if that bit is a 1, then that spot saw the sun at
* 4pm 22 June. More formally: Given `month` (1-12), `day` (1...month max; February has 28 days)
* and `hour` (0-23), the shade/sun for that month/day/hour at a position `(x, y)` is the bit ```
* (hourly_shade[month - 1])(x, y)[hour] & (1 << (day - 1)) ``` where `(x, y)` is spatial
* indexing, `[month - 1]` refers to fetching the `month - 1`st URL (indexing from zero), `[hour]`
* is indexing into the channels, and a final non-zero result means "sunny". There are no leap
* days, and DST doesn't exist (all days are 24 hours long; noon is always "standard time" noon).
* @return value or {@code null} for none
*/
public java.util.List getHourlyShadeUrls() {
return hourlyShadeUrls;
}
/**
* Twelve URLs for hourly shade, corresponding to January...December, in order. Each GeoTIFF will
* contain 24 bands, corresponding to the 24 hours of the day. Each pixel is a 32 bit integer,
* corresponding to the (up to) 31 days of that month; a 1 bit means that the corresponding
* location is able to see the sun at that day, of that hour, of that month. Invalid locations are
* stored as -9999 (since this is negative, it has bit 31 set, and no valid value could have bit
* 31 set as that would correspond to the 32nd day of the month). An example may be useful. If you
* want to know whether a point (at pixel location (x, y)) saw sun at 4pm on the 22nd of June you
* would: 1. fetch the sixth URL in this list (corresponding to June). 1. look up the 17th channel
* (corresponding to 4pm). 1. read the 32-bit value at (x, y). 1. read bit 21 of the value
* (corresponding to the 22nd of the month). 1. if that bit is a 1, then that spot saw the sun at
* 4pm 22 June. More formally: Given `month` (1-12), `day` (1...month max; February has 28 days)
* and `hour` (0-23), the shade/sun for that month/day/hour at a position `(x, y)` is the bit ```
* (hourly_shade[month - 1])(x, y)[hour] & (1 << (day - 1)) ``` where `(x, y)` is spatial
* indexing, `[month - 1]` refers to fetching the `month - 1`st URL (indexing from zero), `[hour]`
* is indexing into the channels, and a final non-zero result means "sunny". There are no leap
* days, and DST doesn't exist (all days are 24 hours long; noon is always "standard time" noon).
* @param hourlyShadeUrls hourlyShadeUrls or {@code null} for none
*/
public DataLayers setHourlyShadeUrls(java.util.List hourlyShadeUrls) {
this.hourlyShadeUrls = hourlyShadeUrls;
return this;
}
/**
* When the source imagery (from which all the other data are derived) in this region was taken.
* It is necessarily somewhat approximate, as the images may have been taken over more than one
* day.
* @return value or {@code null} for none
*/
public Date getImageryDate() {
return imageryDate;
}
/**
* When the source imagery (from which all the other data are derived) in this region was taken.
* It is necessarily somewhat approximate, as the images may have been taken over more than one
* day.
* @param imageryDate imageryDate or {@code null} for none
*/
public DataLayers setImageryDate(Date imageryDate) {
this.imageryDate = imageryDate;
return this;
}
/**
* When processing was completed on this imagery.
* @return value or {@code null} for none
*/
public Date getImageryProcessedDate() {
return imageryProcessedDate;
}
/**
* When processing was completed on this imagery.
* @param imageryProcessedDate imageryProcessedDate or {@code null} for none
*/
public DataLayers setImageryProcessedDate(Date imageryProcessedDate) {
this.imageryProcessedDate = imageryProcessedDate;
return this;
}
/**
* The quality of the result's imagery.
* @return value or {@code null} for none
*/
public java.lang.String getImageryQuality() {
return imageryQuality;
}
/**
* The quality of the result's imagery.
* @param imageryQuality imageryQuality or {@code null} for none
*/
public DataLayers setImageryQuality(java.lang.String imageryQuality) {
this.imageryQuality = imageryQuality;
return this;
}
/**
* The URL for the building mask image: one bit per pixel saying whether that pixel is considered
* to be part of a rooftop or not.
* @return value or {@code null} for none
*/
public java.lang.String getMaskUrl() {
return maskUrl;
}
/**
* The URL for the building mask image: one bit per pixel saying whether that pixel is considered
* to be part of a rooftop or not.
* @param maskUrl maskUrl or {@code null} for none
*/
public DataLayers setMaskUrl(java.lang.String maskUrl) {
this.maskUrl = maskUrl;
return this;
}
/**
* The URL for the monthly flux map (sunlight on roofs, broken down by month) of the region.
* Values are kWh/kW/year. The GeoTIFF pointed to by this URL will contain twelve bands,
* corresponding to January...December, in order.
* @return value or {@code null} for none
*/
public java.lang.String getMonthlyFluxUrl() {
return monthlyFluxUrl;
}
/**
* The URL for the monthly flux map (sunlight on roofs, broken down by month) of the region.
* Values are kWh/kW/year. The GeoTIFF pointed to by this URL will contain twelve bands,
* corresponding to January...December, in order.
* @param monthlyFluxUrl monthlyFluxUrl or {@code null} for none
*/
public DataLayers setMonthlyFluxUrl(java.lang.String monthlyFluxUrl) {
this.monthlyFluxUrl = monthlyFluxUrl;
return this;
}
/**
* The URL for an image of RGB data (aerial photo) of the region.
* @return value or {@code null} for none
*/
public java.lang.String getRgbUrl() {
return rgbUrl;
}
/**
* The URL for an image of RGB data (aerial photo) of the region.
* @param rgbUrl rgbUrl or {@code null} for none
*/
public DataLayers setRgbUrl(java.lang.String rgbUrl) {
this.rgbUrl = rgbUrl;
return this;
}
@Override
public DataLayers set(String fieldName, Object value) {
return (DataLayers) super.set(fieldName, value);
}
@Override
public DataLayers clone() {
return (DataLayers) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy