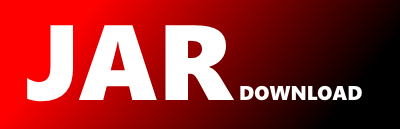
com.google.api.services.solar.v1.model.FinancialDetails Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.solar.v1.model;
/**
* Details of a financial analysis. Some of these details are already stored at higher levels (e.g.,
* out of pocket cost). Total money amounts are over a lifetime period defined by the
* panel_lifetime_years field in SolarPotential. Note: The out of pocket cost of purchasing the
* panels is given in the out_of_pocket_cost field in CashPurchaseSavings.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Solar API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class FinancialDetails extends com.google.api.client.json.GenericJson {
/**
* Total cost of electricity the user would have paid over the lifetime period if they didn't
* install solar.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money costOfElectricityWithoutSolar;
/**
* Amount of money available from federal incentives; this applies if the user buys (with or
* without a loan) the panels.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money federalIncentive;
/**
* How many AC kWh we think the solar panels will generate in their first year.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Float initialAcKwhPerYear;
/**
* Amount of money the user will receive from Solar Renewable Energy Credits over the panel
* lifetime; this applies if the user buys (with or without a loan) the panels.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money lifetimeSrecTotal;
/**
* Whether net metering is allowed.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean netMeteringAllowed;
/**
* The percentage (0-100) of solar electricity production we assumed was exported to the grid,
* based on the first quarter of production. This affects the calculations if net metering is not
* allowed.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Float percentageExportedToGrid;
/**
* Utility bill for electricity not produced by solar, for the lifetime of the panels.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money remainingLifetimeUtilityBill;
/**
* Percentage (0-100) of the user's power supplied by solar. Valid for the first year but
* approximately correct for future years.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Float solarPercentage;
/**
* Amount of money available from state incentives; this applies if the user buys (with or without
* a loan) the panels.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money stateIncentive;
/**
* Amount of money available from utility incentives; this applies if the user buys (with or
* without a loan) the panels.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money utilityIncentive;
/**
* Total cost of electricity the user would have paid over the lifetime period if they didn't
* install solar.
* @return value or {@code null} for none
*/
public Money getCostOfElectricityWithoutSolar() {
return costOfElectricityWithoutSolar;
}
/**
* Total cost of electricity the user would have paid over the lifetime period if they didn't
* install solar.
* @param costOfElectricityWithoutSolar costOfElectricityWithoutSolar or {@code null} for none
*/
public FinancialDetails setCostOfElectricityWithoutSolar(Money costOfElectricityWithoutSolar) {
this.costOfElectricityWithoutSolar = costOfElectricityWithoutSolar;
return this;
}
/**
* Amount of money available from federal incentives; this applies if the user buys (with or
* without a loan) the panels.
* @return value or {@code null} for none
*/
public Money getFederalIncentive() {
return federalIncentive;
}
/**
* Amount of money available from federal incentives; this applies if the user buys (with or
* without a loan) the panels.
* @param federalIncentive federalIncentive or {@code null} for none
*/
public FinancialDetails setFederalIncentive(Money federalIncentive) {
this.federalIncentive = federalIncentive;
return this;
}
/**
* How many AC kWh we think the solar panels will generate in their first year.
* @return value or {@code null} for none
*/
public java.lang.Float getInitialAcKwhPerYear() {
return initialAcKwhPerYear;
}
/**
* How many AC kWh we think the solar panels will generate in their first year.
* @param initialAcKwhPerYear initialAcKwhPerYear or {@code null} for none
*/
public FinancialDetails setInitialAcKwhPerYear(java.lang.Float initialAcKwhPerYear) {
this.initialAcKwhPerYear = initialAcKwhPerYear;
return this;
}
/**
* Amount of money the user will receive from Solar Renewable Energy Credits over the panel
* lifetime; this applies if the user buys (with or without a loan) the panels.
* @return value or {@code null} for none
*/
public Money getLifetimeSrecTotal() {
return lifetimeSrecTotal;
}
/**
* Amount of money the user will receive from Solar Renewable Energy Credits over the panel
* lifetime; this applies if the user buys (with or without a loan) the panels.
* @param lifetimeSrecTotal lifetimeSrecTotal or {@code null} for none
*/
public FinancialDetails setLifetimeSrecTotal(Money lifetimeSrecTotal) {
this.lifetimeSrecTotal = lifetimeSrecTotal;
return this;
}
/**
* Whether net metering is allowed.
* @return value or {@code null} for none
*/
public java.lang.Boolean getNetMeteringAllowed() {
return netMeteringAllowed;
}
/**
* Whether net metering is allowed.
* @param netMeteringAllowed netMeteringAllowed or {@code null} for none
*/
public FinancialDetails setNetMeteringAllowed(java.lang.Boolean netMeteringAllowed) {
this.netMeteringAllowed = netMeteringAllowed;
return this;
}
/**
* The percentage (0-100) of solar electricity production we assumed was exported to the grid,
* based on the first quarter of production. This affects the calculations if net metering is not
* allowed.
* @return value or {@code null} for none
*/
public java.lang.Float getPercentageExportedToGrid() {
return percentageExportedToGrid;
}
/**
* The percentage (0-100) of solar electricity production we assumed was exported to the grid,
* based on the first quarter of production. This affects the calculations if net metering is not
* allowed.
* @param percentageExportedToGrid percentageExportedToGrid or {@code null} for none
*/
public FinancialDetails setPercentageExportedToGrid(java.lang.Float percentageExportedToGrid) {
this.percentageExportedToGrid = percentageExportedToGrid;
return this;
}
/**
* Utility bill for electricity not produced by solar, for the lifetime of the panels.
* @return value or {@code null} for none
*/
public Money getRemainingLifetimeUtilityBill() {
return remainingLifetimeUtilityBill;
}
/**
* Utility bill for electricity not produced by solar, for the lifetime of the panels.
* @param remainingLifetimeUtilityBill remainingLifetimeUtilityBill or {@code null} for none
*/
public FinancialDetails setRemainingLifetimeUtilityBill(Money remainingLifetimeUtilityBill) {
this.remainingLifetimeUtilityBill = remainingLifetimeUtilityBill;
return this;
}
/**
* Percentage (0-100) of the user's power supplied by solar. Valid for the first year but
* approximately correct for future years.
* @return value or {@code null} for none
*/
public java.lang.Float getSolarPercentage() {
return solarPercentage;
}
/**
* Percentage (0-100) of the user's power supplied by solar. Valid for the first year but
* approximately correct for future years.
* @param solarPercentage solarPercentage or {@code null} for none
*/
public FinancialDetails setSolarPercentage(java.lang.Float solarPercentage) {
this.solarPercentage = solarPercentage;
return this;
}
/**
* Amount of money available from state incentives; this applies if the user buys (with or without
* a loan) the panels.
* @return value or {@code null} for none
*/
public Money getStateIncentive() {
return stateIncentive;
}
/**
* Amount of money available from state incentives; this applies if the user buys (with or without
* a loan) the panels.
* @param stateIncentive stateIncentive or {@code null} for none
*/
public FinancialDetails setStateIncentive(Money stateIncentive) {
this.stateIncentive = stateIncentive;
return this;
}
/**
* Amount of money available from utility incentives; this applies if the user buys (with or
* without a loan) the panels.
* @return value or {@code null} for none
*/
public Money getUtilityIncentive() {
return utilityIncentive;
}
/**
* Amount of money available from utility incentives; this applies if the user buys (with or
* without a loan) the panels.
* @param utilityIncentive utilityIncentive or {@code null} for none
*/
public FinancialDetails setUtilityIncentive(Money utilityIncentive) {
this.utilityIncentive = utilityIncentive;
return this;
}
@Override
public FinancialDetails set(String fieldName, Object value) {
return (FinancialDetails) super.set(fieldName, value);
}
@Override
public FinancialDetails clone() {
return (FinancialDetails) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy