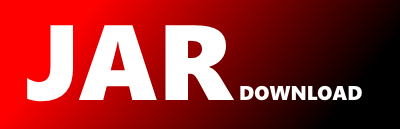
com.google.api.services.solar.v1.model.SolarPotential Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.solar.v1.model;
/**
* Information about the solar potential of a building. A number of fields in this are defined in
* terms of "panels". The fields panel_capacity_watts, panel_height_meters, and panel_width_meters
* describe the parameters of the model of panel used in these calculations.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Solar API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class SolarPotential extends com.google.api.client.json.GenericJson {
/**
* Size and sunlight quantiles for the entire building, including parts of the roof that were not
* assigned to some roof segment. Because the orientations of these parts are not well
* characterised, the roof area estimate is unreliable, but the ground area estimate is reliable.
* It may be that a more reliable whole building roof area can be obtained by scaling the roof
* area from whole_roof_stats by the ratio of the ground areas of `building_stats` and
* `whole_roof_stats`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SizeAndSunshineStats buildingStats;
/**
* Equivalent amount of CO2 produced per MWh of grid electricity. This is a measure of the carbon
* intensity of grid electricity displaced by solar electricity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Float carbonOffsetFactorKgPerMwh;
/**
* A FinancialAnalysis gives the savings from going solar assuming a given monthly bill and a
* given electricity provider. They are in order of increasing order of monthly bill amount. This
* field will be empty for buildings in areas for which the Solar API does not have enough
* information to perform financial computations.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List financialAnalyses;
static {
// hack to force ProGuard to consider FinancialAnalysis used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(FinancialAnalysis.class);
}
/**
* Size, in square meters, of the maximum array.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Float maxArrayAreaMeters2;
/**
* Size of the maximum array - that is, the maximum number of panels that can fit on the roof.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer maxArrayPanelsCount;
/**
* Maximum number of sunshine hours received per year, by any point on the roof. Sunshine hours
* are a measure of the total amount of insolation (energy) received per year. 1 sunshine hour = 1
* kWh per kW (where kW refers to kW of capacity under Standard Testing Conditions).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Float maxSunshineHoursPerYear;
/**
* Capacity, in watts, of the panel used in the calculations.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Float panelCapacityWatts;
/**
* Height, in meters in portrait orientation, of the panel used in the calculations.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Float panelHeightMeters;
/**
* The expected lifetime, in years, of the solar panels. This is used in the financial
* calculations.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer panelLifetimeYears;
/**
* Width, in meters in portrait orientation, of the panel used in the calculations.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Float panelWidthMeters;
/**
* Size and sunlight quantiles for each roof segment.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List roofSegmentStats;
static {
// hack to force ProGuard to consider RoofSegmentSizeAndSunshineStats used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(RoofSegmentSizeAndSunshineStats.class);
}
/**
* Each SolarPanelConfig describes a different arrangement of solar panels on the roof. They are
* in order of increasing number of panels. The `SolarPanelConfig` with panels_count=N is based on
* the first N panels in the `solar_panels` list. This field is only populated if at least 4
* panels can fit on a roof.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List solarPanelConfigs;
static {
// hack to force ProGuard to consider SolarPanelConfig used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(SolarPanelConfig.class);
}
/**
* Each SolarPanel describes a single solar panel. They are listed in the order that the panel
* layout algorithm placed this. This is usually, though not always, in decreasing order of annual
* energy production.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List solarPanels;
static {
// hack to force ProGuard to consider SolarPanel used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(SolarPanel.class);
}
/**
* Total size and sunlight quantiles for the part of the roof that was assigned to some roof
* segment. Despite the name, this may not include the entire building. See building_stats.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SizeAndSunshineStats wholeRoofStats;
/**
* Size and sunlight quantiles for the entire building, including parts of the roof that were not
* assigned to some roof segment. Because the orientations of these parts are not well
* characterised, the roof area estimate is unreliable, but the ground area estimate is reliable.
* It may be that a more reliable whole building roof area can be obtained by scaling the roof
* area from whole_roof_stats by the ratio of the ground areas of `building_stats` and
* `whole_roof_stats`.
* @return value or {@code null} for none
*/
public SizeAndSunshineStats getBuildingStats() {
return buildingStats;
}
/**
* Size and sunlight quantiles for the entire building, including parts of the roof that were not
* assigned to some roof segment. Because the orientations of these parts are not well
* characterised, the roof area estimate is unreliable, but the ground area estimate is reliable.
* It may be that a more reliable whole building roof area can be obtained by scaling the roof
* area from whole_roof_stats by the ratio of the ground areas of `building_stats` and
* `whole_roof_stats`.
* @param buildingStats buildingStats or {@code null} for none
*/
public SolarPotential setBuildingStats(SizeAndSunshineStats buildingStats) {
this.buildingStats = buildingStats;
return this;
}
/**
* Equivalent amount of CO2 produced per MWh of grid electricity. This is a measure of the carbon
* intensity of grid electricity displaced by solar electricity.
* @return value or {@code null} for none
*/
public java.lang.Float getCarbonOffsetFactorKgPerMwh() {
return carbonOffsetFactorKgPerMwh;
}
/**
* Equivalent amount of CO2 produced per MWh of grid electricity. This is a measure of the carbon
* intensity of grid electricity displaced by solar electricity.
* @param carbonOffsetFactorKgPerMwh carbonOffsetFactorKgPerMwh or {@code null} for none
*/
public SolarPotential setCarbonOffsetFactorKgPerMwh(java.lang.Float carbonOffsetFactorKgPerMwh) {
this.carbonOffsetFactorKgPerMwh = carbonOffsetFactorKgPerMwh;
return this;
}
/**
* A FinancialAnalysis gives the savings from going solar assuming a given monthly bill and a
* given electricity provider. They are in order of increasing order of monthly bill amount. This
* field will be empty for buildings in areas for which the Solar API does not have enough
* information to perform financial computations.
* @return value or {@code null} for none
*/
public java.util.List getFinancialAnalyses() {
return financialAnalyses;
}
/**
* A FinancialAnalysis gives the savings from going solar assuming a given monthly bill and a
* given electricity provider. They are in order of increasing order of monthly bill amount. This
* field will be empty for buildings in areas for which the Solar API does not have enough
* information to perform financial computations.
* @param financialAnalyses financialAnalyses or {@code null} for none
*/
public SolarPotential setFinancialAnalyses(java.util.List financialAnalyses) {
this.financialAnalyses = financialAnalyses;
return this;
}
/**
* Size, in square meters, of the maximum array.
* @return value or {@code null} for none
*/
public java.lang.Float getMaxArrayAreaMeters2() {
return maxArrayAreaMeters2;
}
/**
* Size, in square meters, of the maximum array.
* @param maxArrayAreaMeters2 maxArrayAreaMeters2 or {@code null} for none
*/
public SolarPotential setMaxArrayAreaMeters2(java.lang.Float maxArrayAreaMeters2) {
this.maxArrayAreaMeters2 = maxArrayAreaMeters2;
return this;
}
/**
* Size of the maximum array - that is, the maximum number of panels that can fit on the roof.
* @return value or {@code null} for none
*/
public java.lang.Integer getMaxArrayPanelsCount() {
return maxArrayPanelsCount;
}
/**
* Size of the maximum array - that is, the maximum number of panels that can fit on the roof.
* @param maxArrayPanelsCount maxArrayPanelsCount or {@code null} for none
*/
public SolarPotential setMaxArrayPanelsCount(java.lang.Integer maxArrayPanelsCount) {
this.maxArrayPanelsCount = maxArrayPanelsCount;
return this;
}
/**
* Maximum number of sunshine hours received per year, by any point on the roof. Sunshine hours
* are a measure of the total amount of insolation (energy) received per year. 1 sunshine hour = 1
* kWh per kW (where kW refers to kW of capacity under Standard Testing Conditions).
* @return value or {@code null} for none
*/
public java.lang.Float getMaxSunshineHoursPerYear() {
return maxSunshineHoursPerYear;
}
/**
* Maximum number of sunshine hours received per year, by any point on the roof. Sunshine hours
* are a measure of the total amount of insolation (energy) received per year. 1 sunshine hour = 1
* kWh per kW (where kW refers to kW of capacity under Standard Testing Conditions).
* @param maxSunshineHoursPerYear maxSunshineHoursPerYear or {@code null} for none
*/
public SolarPotential setMaxSunshineHoursPerYear(java.lang.Float maxSunshineHoursPerYear) {
this.maxSunshineHoursPerYear = maxSunshineHoursPerYear;
return this;
}
/**
* Capacity, in watts, of the panel used in the calculations.
* @return value or {@code null} for none
*/
public java.lang.Float getPanelCapacityWatts() {
return panelCapacityWatts;
}
/**
* Capacity, in watts, of the panel used in the calculations.
* @param panelCapacityWatts panelCapacityWatts or {@code null} for none
*/
public SolarPotential setPanelCapacityWatts(java.lang.Float panelCapacityWatts) {
this.panelCapacityWatts = panelCapacityWatts;
return this;
}
/**
* Height, in meters in portrait orientation, of the panel used in the calculations.
* @return value or {@code null} for none
*/
public java.lang.Float getPanelHeightMeters() {
return panelHeightMeters;
}
/**
* Height, in meters in portrait orientation, of the panel used in the calculations.
* @param panelHeightMeters panelHeightMeters or {@code null} for none
*/
public SolarPotential setPanelHeightMeters(java.lang.Float panelHeightMeters) {
this.panelHeightMeters = panelHeightMeters;
return this;
}
/**
* The expected lifetime, in years, of the solar panels. This is used in the financial
* calculations.
* @return value or {@code null} for none
*/
public java.lang.Integer getPanelLifetimeYears() {
return panelLifetimeYears;
}
/**
* The expected lifetime, in years, of the solar panels. This is used in the financial
* calculations.
* @param panelLifetimeYears panelLifetimeYears or {@code null} for none
*/
public SolarPotential setPanelLifetimeYears(java.lang.Integer panelLifetimeYears) {
this.panelLifetimeYears = panelLifetimeYears;
return this;
}
/**
* Width, in meters in portrait orientation, of the panel used in the calculations.
* @return value or {@code null} for none
*/
public java.lang.Float getPanelWidthMeters() {
return panelWidthMeters;
}
/**
* Width, in meters in portrait orientation, of the panel used in the calculations.
* @param panelWidthMeters panelWidthMeters or {@code null} for none
*/
public SolarPotential setPanelWidthMeters(java.lang.Float panelWidthMeters) {
this.panelWidthMeters = panelWidthMeters;
return this;
}
/**
* Size and sunlight quantiles for each roof segment.
* @return value or {@code null} for none
*/
public java.util.List getRoofSegmentStats() {
return roofSegmentStats;
}
/**
* Size and sunlight quantiles for each roof segment.
* @param roofSegmentStats roofSegmentStats or {@code null} for none
*/
public SolarPotential setRoofSegmentStats(java.util.List roofSegmentStats) {
this.roofSegmentStats = roofSegmentStats;
return this;
}
/**
* Each SolarPanelConfig describes a different arrangement of solar panels on the roof. They are
* in order of increasing number of panels. The `SolarPanelConfig` with panels_count=N is based on
* the first N panels in the `solar_panels` list. This field is only populated if at least 4
* panels can fit on a roof.
* @return value or {@code null} for none
*/
public java.util.List getSolarPanelConfigs() {
return solarPanelConfigs;
}
/**
* Each SolarPanelConfig describes a different arrangement of solar panels on the roof. They are
* in order of increasing number of panels. The `SolarPanelConfig` with panels_count=N is based on
* the first N panels in the `solar_panels` list. This field is only populated if at least 4
* panels can fit on a roof.
* @param solarPanelConfigs solarPanelConfigs or {@code null} for none
*/
public SolarPotential setSolarPanelConfigs(java.util.List solarPanelConfigs) {
this.solarPanelConfigs = solarPanelConfigs;
return this;
}
/**
* Each SolarPanel describes a single solar panel. They are listed in the order that the panel
* layout algorithm placed this. This is usually, though not always, in decreasing order of annual
* energy production.
* @return value or {@code null} for none
*/
public java.util.List getSolarPanels() {
return solarPanels;
}
/**
* Each SolarPanel describes a single solar panel. They are listed in the order that the panel
* layout algorithm placed this. This is usually, though not always, in decreasing order of annual
* energy production.
* @param solarPanels solarPanels or {@code null} for none
*/
public SolarPotential setSolarPanels(java.util.List solarPanels) {
this.solarPanels = solarPanels;
return this;
}
/**
* Total size and sunlight quantiles for the part of the roof that was assigned to some roof
* segment. Despite the name, this may not include the entire building. See building_stats.
* @return value or {@code null} for none
*/
public SizeAndSunshineStats getWholeRoofStats() {
return wholeRoofStats;
}
/**
* Total size and sunlight quantiles for the part of the roof that was assigned to some roof
* segment. Despite the name, this may not include the entire building. See building_stats.
* @param wholeRoofStats wholeRoofStats or {@code null} for none
*/
public SolarPotential setWholeRoofStats(SizeAndSunshineStats wholeRoofStats) {
this.wholeRoofStats = wholeRoofStats;
return this;
}
@Override
public SolarPotential set(String fieldName, Object value) {
return (SolarPotential) super.set(fieldName, value);
}
@Override
public SolarPotential clone() {
return (SolarPotential) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy