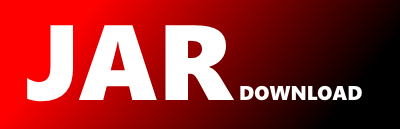
com.google.api.services.spanner.v1.Spanner Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.spanner.v1;
/**
* Service definition for Spanner (v1).
*
*
* Cloud Spanner is a managed, mission-critical, globally consistent and scalable relational database service.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link SpannerRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class Spanner extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Cloud Spanner API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://spanner.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://spanner.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Spanner(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
Spanner(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Projects collection.
*
* The typical use is:
*
* {@code Spanner spanner = new Spanner(...);}
* {@code Spanner.Projects.List request = spanner.projects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Projects projects() {
return new Projects();
}
/**
* The "projects" collection of methods.
*/
public class Projects {
/**
* An accessor for creating requests from the InstanceConfigOperations collection.
*
* The typical use is:
*
* {@code Spanner spanner = new Spanner(...);}
* {@code Spanner.InstanceConfigOperations.List request = spanner.instanceConfigOperations().list(parameters ...)}
*
*
* @return the resource collection
*/
public InstanceConfigOperations instanceConfigOperations() {
return new InstanceConfigOperations();
}
/**
* The "instanceConfigOperations" collection of methods.
*/
public class InstanceConfigOperations {
/**
* Lists the user-managed instance configuration long-running operations in the given project. An
* instance configuration operation has a name of the form `projects//instanceConfigs//operations/`.
* The long-running operation metadata field type `metadata.type_url` describes the type of the
* metadata. Operations returned include those that have completed/failed/canceled within the last 7
* days, and pending operations. Operations returned are ordered by
* `operation.metadata.value.start_time` in descending order starting from the most recently started
* operation.
*
* Create a request for the method "instanceConfigOperations.list".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The project of the instance configuration operations. Values are of the form `projects/`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends SpannerRequest {
private static final String REST_PATH = "v1/{+parent}/instanceConfigOperations";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Lists the user-managed instance configuration long-running operations in the given project. An
* instance configuration operation has a name of the form
* `projects//instanceConfigs//operations/`. The long-running operation metadata field type
* `metadata.type_url` describes the type of the metadata. Operations returned include those that
* have completed/failed/canceled within the last 7 days, and pending operations. Operations
* returned are ordered by `operation.metadata.value.start_time` in descending order starting from
* the most recently started operation.
*
* Create a request for the method "instanceConfigOperations.list".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The project of the instance configuration operations. Values are of the form `projects/`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.ListInstanceConfigOperationsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The project of the instance configuration operations. Values are of the form
* `projects/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The project of the instance configuration operations. Values are of the form `projects/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The project of the instance configuration operations. Values are of the form
* `projects/`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* An expression that filters the list of returned operations. A filter expression consists
* of a field name, a comparison operator, and a value for filtering. The value must be a
* string, a number, or a boolean. The comparison operator must be one of: `<`, `>`, `<=`,
* `>=`, `!=`, `=`, or `:`. Colon `:` is the contains operator. Filter rules are not case
* sensitive. The following fields in the Operation are eligible for filtering: * `name` -
* The name of the long-running operation * `done` - False if the operation is in progress,
* else true. * `metadata.@type` - the type of metadata. For example, the type string for
* CreateInstanceConfigMetadata is
* `type.googleapis.com/google.spanner.admin.instance.v1.CreateInstanceConfigMetadata`. *
* `metadata.` - any field in metadata.value. `metadata.@type` must be specified first, if
* filtering on metadata fields. * `error` - Error associated with the long-running
* operation. * `response.@type` - the type of response. * `response.` - any field in
* response.value. You can combine multiple expressions by enclosing each expression in
* parentheses. By default, expressions are combined with AND logic. However, you can
* specify AND, OR, and NOT logic explicitly. Here are a few examples: * `done:true` - The
* operation is complete. * `(metadata.@type=` \
* `type.googleapis.com/google.spanner.admin.instance.v1.CreateInstanceConfigMetadata) AND`
* \ `(metadata.instance_config.name:custom-config) AND` \ `(metadata.progress.start_time <
* \"2021-03-28T14:50:00Z\") AND` \ `(error:*)` - Return operations where: * The operation's
* metadata type is CreateInstanceConfigMetadata. * The instance configuration name contains
* "custom-config". * The operation started before 2021-03-28T14:50:00Z. * The operation
* resulted in an error.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** An expression that filters the list of returned operations. A filter expression consists of a field
name, a comparison operator, and a value for filtering. The value must be a string, a number, or a
boolean. The comparison operator must be one of: `<`, `>`, `<=`, `>=`, `!=`, `=`, or `:`. Colon `:`
is the contains operator. Filter rules are not case sensitive. The following fields in the
Operation are eligible for filtering: * `name` - The name of the long-running operation * `done` -
False if the operation is in progress, else true. * `metadata.@type` - the type of metadata. For
example, the type string for CreateInstanceConfigMetadata is
`type.googleapis.com/google.spanner.admin.instance.v1.CreateInstanceConfigMetadata`. * `metadata.`
- any field in metadata.value. `metadata.@type` must be specified first, if filtering on metadata
fields. * `error` - Error associated with the long-running operation. * `response.@type` - the type
of response. * `response.` - any field in response.value. You can combine multiple expressions by
enclosing each expression in parentheses. By default, expressions are combined with AND logic.
However, you can specify AND, OR, and NOT logic explicitly. Here are a few examples: * `done:true`
- The operation is complete. * `(metadata.@type=` \
`type.googleapis.com/google.spanner.admin.instance.v1.CreateInstanceConfigMetadata) AND` \
`(metadata.instance_config.name:custom-config) AND` \ `(metadata.progress.start_time <
\"2021-03-28T14:50:00Z\") AND` \ `(error:*)` - Return operations where: * The operation's metadata
type is CreateInstanceConfigMetadata. * The instance configuration name contains "custom-config". *
The operation started before 2021-03-28T14:50:00Z. * The operation resulted in an error.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* An expression that filters the list of returned operations. A filter expression consists
* of a field name, a comparison operator, and a value for filtering. The value must be a
* string, a number, or a boolean. The comparison operator must be one of: `<`, `>`, `<=`,
* `>=`, `!=`, `=`, or `:`. Colon `:` is the contains operator. Filter rules are not case
* sensitive. The following fields in the Operation are eligible for filtering: * `name` -
* The name of the long-running operation * `done` - False if the operation is in progress,
* else true. * `metadata.@type` - the type of metadata. For example, the type string for
* CreateInstanceConfigMetadata is
* `type.googleapis.com/google.spanner.admin.instance.v1.CreateInstanceConfigMetadata`. *
* `metadata.` - any field in metadata.value. `metadata.@type` must be specified first, if
* filtering on metadata fields. * `error` - Error associated with the long-running
* operation. * `response.@type` - the type of response. * `response.` - any field in
* response.value. You can combine multiple expressions by enclosing each expression in
* parentheses. By default, expressions are combined with AND logic. However, you can
* specify AND, OR, and NOT logic explicitly. Here are a few examples: * `done:true` - The
* operation is complete. * `(metadata.@type=` \
* `type.googleapis.com/google.spanner.admin.instance.v1.CreateInstanceConfigMetadata) AND`
* \ `(metadata.instance_config.name:custom-config) AND` \ `(metadata.progress.start_time <
* \"2021-03-28T14:50:00Z\") AND` \ `(error:*)` - Return operations where: * The operation's
* metadata type is CreateInstanceConfigMetadata. * The instance configuration name contains
* "custom-config". * The operation started before 2021-03-28T14:50:00Z. * The operation
* resulted in an error.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Number of operations to be returned in the response. If 0 or less, defaults to the
* server's maximum allowed page size.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Number of operations to be returned in the response. If 0 or less, defaults to the server's maximum
allowed page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Number of operations to be returned in the response. If 0 or less, defaults to the
* server's maximum allowed page size.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* If non-empty, `page_token` should contain a next_page_token from a previous
* ListInstanceConfigOperationsResponse to the same `parent` and with the same `filter`.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** If non-empty, `page_token` should contain a next_page_token from a previous
ListInstanceConfigOperationsResponse to the same `parent` and with the same `filter`.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* If non-empty, `page_token` should contain a next_page_token from a previous
* ListInstanceConfigOperationsResponse to the same `parent` and with the same `filter`.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the InstanceConfigs collection.
*
* The typical use is:
*
* {@code Spanner spanner = new Spanner(...);}
* {@code Spanner.InstanceConfigs.List request = spanner.instanceConfigs().list(parameters ...)}
*
*
* @return the resource collection
*/
public InstanceConfigs instanceConfigs() {
return new InstanceConfigs();
}
/**
* The "instanceConfigs" collection of methods.
*/
public class InstanceConfigs {
/**
* Creates an instance configuration and begins preparing it to be used. The returned long-running
* operation can be used to track the progress of preparing the new instance config. The instance
* configuration name is assigned by the caller. If the named instance configuration already exists,
* `CreateInstanceConfig` returns `ALREADY_EXISTS`. Immediately after the request returns: * The
* instance configuration is readable via the API, with all requested attributes. The instance
* config's reconciling field is set to true. Its state is `CREATING`. While the operation is
* pending: * Cancelling the operation renders the instance configuration immediately unreadable via
* the API. * Except for deleting the creating resource, all other attempts to modify the instance
* configuration are rejected. Upon completion of the returned operation: * Instances can be created
* using the instance configuration. * The instance config's reconciling field becomes false. Its
* state becomes `READY`. The returned long-running operation will have a name of the format
* `/operations/` and can be used to track creation of the instance config. The metadata field type
* is CreateInstanceConfigMetadata. The response field type is InstanceConfig, if successful.
* Authorization requires `spanner.instanceConfigs.create` permission on the resource parent.
*
* Create a request for the method "instanceConfigs.create".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the project in which to create the instance config. Values are of the form
* `projects/`.
* @param content the {@link com.google.api.services.spanner.v1.model.CreateInstanceConfigRequest}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.spanner.v1.model.CreateInstanceConfigRequest content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends SpannerRequest {
private static final String REST_PATH = "v1/{+parent}/instanceConfigs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Creates an instance configuration and begins preparing it to be used. The returned long-running
* operation can be used to track the progress of preparing the new instance config. The instance
* configuration name is assigned by the caller. If the named instance configuration already
* exists, `CreateInstanceConfig` returns `ALREADY_EXISTS`. Immediately after the request returns:
* * The instance configuration is readable via the API, with all requested attributes. The
* instance config's reconciling field is set to true. Its state is `CREATING`. While the
* operation is pending: * Cancelling the operation renders the instance configuration immediately
* unreadable via the API. * Except for deleting the creating resource, all other attempts to
* modify the instance configuration are rejected. Upon completion of the returned operation: *
* Instances can be created using the instance configuration. * The instance config's reconciling
* field becomes false. Its state becomes `READY`. The returned long-running operation will have a
* name of the format `/operations/` and can be used to track creation of the instance config. The
* metadata field type is CreateInstanceConfigMetadata. The response field type is InstanceConfig,
* if successful. Authorization requires `spanner.instanceConfigs.create` permission on the
* resource parent.
*
* Create a request for the method "instanceConfigs.create".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the project in which to create the instance config. Values are of the form
* `projects/`.
* @param content the {@link com.google.api.services.spanner.v1.model.CreateInstanceConfigRequest}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.spanner.v1.model.CreateInstanceConfigRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the project in which to create the instance config. Values are of
* the form `projects/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the project in which to create the instance config. Values are of the form
`projects/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the project in which to create the instance config. Values are of
* the form `projects/`.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the instance config. Deletion is only allowed when no instances are using the
* configuration. If any instances are using the config, returns `FAILED_PRECONDITION`. Only user-
* managed configurations can be deleted. Authorization requires `spanner.instanceConfigs.delete`
* permission on the resource name.
*
* Create a request for the method "instanceConfigs.delete".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the instance configuration to be deleted. Values are of the form
* `projects//instanceConfigs/`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instanceConfigs/[^/]+$");
/**
* Deletes the instance config. Deletion is only allowed when no instances are using the
* configuration. If any instances are using the config, returns `FAILED_PRECONDITION`. Only user-
* managed configurations can be deleted. Authorization requires `spanner.instanceConfigs.delete`
* permission on the resource name.
*
* Create a request for the method "instanceConfigs.delete".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the instance configuration to be deleted. Values are of the form
* `projects//instanceConfigs/`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Spanner.this, "DELETE", REST_PATH, null, com.google.api.services.spanner.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instanceConfigs/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the instance configuration to be deleted. Values are of the form
* `projects//instanceConfigs/`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the instance configuration to be deleted. Values are of the form
`projects//instanceConfigs/`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the instance configuration to be deleted. Values are of the form
* `projects//instanceConfigs/`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instanceConfigs/[^/]+$");
}
this.name = name;
return this;
}
/**
* Used for optimistic concurrency control as a way to help prevent simultaneous deletes of
* an instance configuration from overwriting each other. If not empty, the API only deletes
* the instance configuration when the etag provided matches the current status of the
* requested instance config. Otherwise, deletes the instance configuration without checking
* the current status of the requested instance config.
*/
@com.google.api.client.util.Key
private java.lang.String etag;
/** Used for optimistic concurrency control as a way to help prevent simultaneous deletes of an
instance configuration from overwriting each other. If not empty, the API only deletes the instance
configuration when the etag provided matches the current status of the requested instance config.
Otherwise, deletes the instance configuration without checking the current status of the requested
instance config.
*/
public java.lang.String getEtag() {
return etag;
}
/**
* Used for optimistic concurrency control as a way to help prevent simultaneous deletes of
* an instance configuration from overwriting each other. If not empty, the API only deletes
* the instance configuration when the etag provided matches the current status of the
* requested instance config. Otherwise, deletes the instance configuration without checking
* the current status of the requested instance config.
*/
public Delete setEtag(java.lang.String etag) {
this.etag = etag;
return this;
}
/**
* An option to validate, but not actually execute, a request, and provide the same
* response.
*/
@com.google.api.client.util.Key
private java.lang.Boolean validateOnly;
/** An option to validate, but not actually execute, a request, and provide the same response.
*/
public java.lang.Boolean getValidateOnly() {
return validateOnly;
}
/**
* An option to validate, but not actually execute, a request, and provide the same
* response.
*/
public Delete setValidateOnly(java.lang.Boolean validateOnly) {
this.validateOnly = validateOnly;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets information about a particular instance configuration.
*
* Create a request for the method "instanceConfigs.get".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the requested instance configuration. Values are of the form
* `projects//instanceConfigs/`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instanceConfigs/[^/]+$");
/**
* Gets information about a particular instance configuration.
*
* Create a request for the method "instanceConfigs.get".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the requested instance configuration. Values are of the form
* `projects//instanceConfigs/`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.InstanceConfig.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instanceConfigs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the requested instance configuration. Values are of the form
* `projects//instanceConfigs/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the requested instance configuration. Values are of the form
`projects//instanceConfigs/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the requested instance configuration. Values are of the form
* `projects//instanceConfigs/`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instanceConfigs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists the supported instance configurations for a given project. Returns both Google-managed
* configurations and user-managed configurations.
*
* Create a request for the method "instanceConfigs.list".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the project for which a list of supported instance configurations is
* requested. Values are of the form `projects/`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends SpannerRequest {
private static final String REST_PATH = "v1/{+parent}/instanceConfigs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Lists the supported instance configurations for a given project. Returns both Google-managed
* configurations and user-managed configurations.
*
* Create a request for the method "instanceConfigs.list".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the project for which a list of supported instance configurations is
* requested. Values are of the form `projects/`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.ListInstanceConfigsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the project for which a list of supported instance configurations
* is requested. Values are of the form `projects/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the project for which a list of supported instance configurations is
requested. Values are of the form `projects/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the project for which a list of supported instance configurations
* is requested. Values are of the form `projects/`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Number of instance configurations to be returned in the response. If 0 or less, defaults
* to the server's maximum allowed page size.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Number of instance configurations to be returned in the response. If 0 or less, defaults to the
server's maximum allowed page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Number of instance configurations to be returned in the response. If 0 or less, defaults
* to the server's maximum allowed page size.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* If non-empty, `page_token` should contain a next_page_token from a previous
* ListInstanceConfigsResponse.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** If non-empty, `page_token` should contain a next_page_token from a previous
ListInstanceConfigsResponse.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* If non-empty, `page_token` should contain a next_page_token from a previous
* ListInstanceConfigsResponse.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an instance config. The returned long-running operation can be used to track the progress
* of updating the instance. If the named instance configuration does not exist, returns
* `NOT_FOUND`. Only user-managed configurations can be updated. Immediately after the request
* returns: * The instance config's reconciling field is set to true. While the operation is
* pending: * Cancelling the operation sets its metadata's cancel_time. The operation is guaranteed
* to succeed at undoing all changes, after which point it terminates with a `CANCELLED` status. *
* All other attempts to modify the instance configuration are rejected. * Reading the instance
* configuration via the API continues to give the pre-request values. Upon completion of the
* returned operation: * Creating instances using the instance configuration uses the new values. *
* The instance config's new values are readable via the API. * The instance config's reconciling
* field becomes false. The returned long-running operation will have a name of the format
* `/operations/` and can be used to track the instance configuration modification. The metadata
* field type is UpdateInstanceConfigMetadata. The response field type is InstanceConfig, if
* successful. Authorization requires `spanner.instanceConfigs.update` permission on the resource
* name.
*
* Create a request for the method "instanceConfigs.patch".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name A unique identifier for the instance configuration. Values are of the form
* `projects//instanceConfigs/a-z*`. User instance configuration must start with `custom-`.
* @param content the {@link com.google.api.services.spanner.v1.model.UpdateInstanceConfigRequest}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.spanner.v1.model.UpdateInstanceConfigRequest content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instanceConfigs/[^/]+$");
/**
* Updates an instance config. The returned long-running operation can be used to track the
* progress of updating the instance. If the named instance configuration does not exist, returns
* `NOT_FOUND`. Only user-managed configurations can be updated. Immediately after the request
* returns: * The instance config's reconciling field is set to true. While the operation is
* pending: * Cancelling the operation sets its metadata's cancel_time. The operation is
* guaranteed to succeed at undoing all changes, after which point it terminates with a
* `CANCELLED` status. * All other attempts to modify the instance configuration are rejected. *
* Reading the instance configuration via the API continues to give the pre-request values. Upon
* completion of the returned operation: * Creating instances using the instance configuration
* uses the new values. * The instance config's new values are readable via the API. * The
* instance config's reconciling field becomes false. The returned long-running operation will
* have a name of the format `/operations/` and can be used to track the instance configuration
* modification. The metadata field type is UpdateInstanceConfigMetadata. The response field type
* is InstanceConfig, if successful. Authorization requires `spanner.instanceConfigs.update`
* permission on the resource name.
*
* Create a request for the method "instanceConfigs.patch".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name A unique identifier for the instance configuration. Values are of the form
* `projects//instanceConfigs/a-z*`. User instance configuration must start with `custom-`.
* @param content the {@link com.google.api.services.spanner.v1.model.UpdateInstanceConfigRequest}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.spanner.v1.model.UpdateInstanceConfigRequest content) {
super(Spanner.this, "PATCH", REST_PATH, content, com.google.api.services.spanner.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instanceConfigs/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* A unique identifier for the instance configuration. Values are of the form
* `projects//instanceConfigs/a-z*`. User instance configuration must start with `custom-`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** A unique identifier for the instance configuration. Values are of the form
`projects//instanceConfigs/a-z*`. User instance configuration must start with `custom-`.
*/
public java.lang.String getName() {
return name;
}
/**
* A unique identifier for the instance configuration. Values are of the form
* `projects//instanceConfigs/a-z*`. User instance configuration must start with `custom-`.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instanceConfigs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code Spanner spanner = new Spanner(...);}
* {@code Spanner.Operations.List request = spanner.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be cancelled.
* @return the request
*/
public Cancel cancel(java.lang.String name) throws java.io.IOException {
Cancel result = new Cancel(name);
initialize(result);
return result;
}
public class Cancel extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instanceConfigs/[^/]+/operations/[^/]+$");
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation. {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be cancelled.
* @since 1.13
*/
protected Cancel(java.lang.String name) {
super(Spanner.this, "POST", REST_PATH, null, com.google.api.services.spanner.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instanceConfigs/[^/]+/operations/[^/]+$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be cancelled. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be cancelled.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be cancelled. */
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instanceConfigs/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be deleted.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instanceConfigs/[^/]+/operations/[^/]+$");
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be deleted.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Spanner.this, "DELETE", REST_PATH, null, com.google.api.services.spanner.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instanceConfigs/[^/]+/operations/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be deleted. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be deleted.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be deleted. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instanceConfigs/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instanceConfigs/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instanceConfigs/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instanceConfigs/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instanceConfigs/[^/]+/operations$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.ListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instanceConfigs/[^/]+/operations$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instanceConfigs/[^/]+/operations$");
}
this.name = name;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the SsdCaches collection.
*
* The typical use is:
*
* {@code Spanner spanner = new Spanner(...);}
* {@code Spanner.SsdCaches.List request = spanner.ssdCaches().list(parameters ...)}
*
*
* @return the resource collection
*/
public SsdCaches ssdCaches() {
return new SsdCaches();
}
/**
* The "ssdCaches" collection of methods.
*/
public class SsdCaches {
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code Spanner spanner = new Spanner(...);}
* {@code Spanner.Operations.List request = spanner.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be cancelled.
* @return the request
*/
public Cancel cancel(java.lang.String name) throws java.io.IOException {
Cancel result = new Cancel(name);
initialize(result);
return result;
}
public class Cancel extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instanceConfigs/[^/]+/ssdCaches/[^/]+/operations/[^/]+$");
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation. {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be cancelled.
* @since 1.13
*/
protected Cancel(java.lang.String name) {
super(Spanner.this, "POST", REST_PATH, null, com.google.api.services.spanner.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instanceConfigs/[^/]+/ssdCaches/[^/]+/operations/[^/]+$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be cancelled. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be cancelled.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be cancelled. */
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instanceConfigs/[^/]+/ssdCaches/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be deleted.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instanceConfigs/[^/]+/ssdCaches/[^/]+/operations/[^/]+$");
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be deleted.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Spanner.this, "DELETE", REST_PATH, null, com.google.api.services.spanner.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instanceConfigs/[^/]+/ssdCaches/[^/]+/operations/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be deleted. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be deleted.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be deleted. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instanceConfigs/[^/]+/ssdCaches/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instanceConfigs/[^/]+/ssdCaches/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instanceConfigs/[^/]+/ssdCaches/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instanceConfigs/[^/]+/ssdCaches/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instanceConfigs/[^/]+/ssdCaches/[^/]+/operations$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.ListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instanceConfigs/[^/]+/ssdCaches/[^/]+/operations$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instanceConfigs/[^/]+/ssdCaches/[^/]+/operations$");
}
this.name = name;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
}
/**
* An accessor for creating requests from the Instances collection.
*
* The typical use is:
*
* {@code Spanner spanner = new Spanner(...);}
* {@code Spanner.Instances.List request = spanner.instances().list(parameters ...)}
*
*
* @return the resource collection
*/
public Instances instances() {
return new Instances();
}
/**
* The "instances" collection of methods.
*/
public class Instances {
/**
* Creates an instance and begins preparing it to begin serving. The returned long-running operation
* can be used to track the progress of preparing the new instance. The instance name is assigned by
* the caller. If the named instance already exists, `CreateInstance` returns `ALREADY_EXISTS`.
* Immediately upon completion of this request: * The instance is readable via the API, with all
* requested attributes but no allocated resources. Its state is `CREATING`. Until completion of the
* returned operation: * Cancelling the operation renders the instance immediately unreadable via
* the API. * The instance can be deleted. * All other attempts to modify the instance are rejected.
* Upon completion of the returned operation: * Billing for all successfully-allocated resources
* begins (some types may have lower than the requested levels). * Databases can be created in the
* instance. * The instance's allocated resource levels are readable via the API. * The instance's
* state becomes `READY`. The returned long-running operation will have a name of the format
* `/operations/` and can be used to track creation of the instance. The metadata field type is
* CreateInstanceMetadata. The response field type is Instance, if successful.
*
* Create a request for the method "instances.create".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the project in which to create the instance. Values are of the form
* `projects/`.
* @param content the {@link com.google.api.services.spanner.v1.model.CreateInstanceRequest}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.spanner.v1.model.CreateInstanceRequest content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends SpannerRequest {
private static final String REST_PATH = "v1/{+parent}/instances";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Creates an instance and begins preparing it to begin serving. The returned long-running
* operation can be used to track the progress of preparing the new instance. The instance name is
* assigned by the caller. If the named instance already exists, `CreateInstance` returns
* `ALREADY_EXISTS`. Immediately upon completion of this request: * The instance is readable via
* the API, with all requested attributes but no allocated resources. Its state is `CREATING`.
* Until completion of the returned operation: * Cancelling the operation renders the instance
* immediately unreadable via the API. * The instance can be deleted. * All other attempts to
* modify the instance are rejected. Upon completion of the returned operation: * Billing for all
* successfully-allocated resources begins (some types may have lower than the requested levels).
* * Databases can be created in the instance. * The instance's allocated resource levels are
* readable via the API. * The instance's state becomes `READY`. The returned long-running
* operation will have a name of the format `/operations/` and can be used to track creation of
* the instance. The metadata field type is CreateInstanceMetadata. The response field type is
* Instance, if successful.
*
* Create a request for the method "instances.create".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the project in which to create the instance. Values are of the form
* `projects/`.
* @param content the {@link com.google.api.services.spanner.v1.model.CreateInstanceRequest}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.spanner.v1.model.CreateInstanceRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the project in which to create the instance. Values are of the form
* `projects/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the project in which to create the instance. Values are of the form
`projects/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the project in which to create the instance. Values are of the form
* `projects/`.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an instance. Immediately upon completion of the request: * Billing ceases for all of the
* instance's reserved resources. Soon afterward: * The instance and *all of its databases*
* immediately and irrevocably disappear from the API. All data in the databases is permanently
* deleted.
*
* Create a request for the method "instances.delete".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the instance to be deleted. Values are of the form `projects//instances/`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+$");
/**
* Deletes an instance. Immediately upon completion of the request: * Billing ceases for all of
* the instance's reserved resources. Soon afterward: * The instance and *all of its databases*
* immediately and irrevocably disappear from the API. All data in the databases is permanently
* deleted.
*
* Create a request for the method "instances.delete".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the instance to be deleted. Values are of the form `projects//instances/`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Spanner.this, "DELETE", REST_PATH, null, com.google.api.services.spanner.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the instance to be deleted. Values are of the form
* `projects//instances/`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the instance to be deleted. Values are of the form `projects//instances/`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the instance to be deleted. Values are of the form
* `projects//instances/`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets information about a particular instance.
*
* Create a request for the method "instances.get".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the requested instance. Values are of the form `projects//instances/`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+$");
/**
* Gets information about a particular instance.
*
* Create a request for the method "instances.get".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the requested instance. Values are of the form `projects//instances/`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.Instance.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the requested instance. Values are of the form
* `projects//instances/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the requested instance. Values are of the form `projects//instances/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the requested instance. Values are of the form
* `projects//instances/`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
this.name = name;
return this;
}
/**
* If field_mask is present, specifies the subset of Instance fields that should be
* returned. If absent, all Instance fields are returned.
*/
@com.google.api.client.util.Key
private String fieldMask;
/** If field_mask is present, specifies the subset of Instance fields that should be returned. If
absent, all Instance fields are returned.
*/
public String getFieldMask() {
return fieldMask;
}
/**
* If field_mask is present, specifies the subset of Instance fields that should be
* returned. If absent, all Instance fields are returned.
*/
public Get setFieldMask(String fieldMask) {
this.fieldMask = fieldMask;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for an instance resource. Returns an empty policy if an instance
* exists but does not have a policy set. Authorization requires `spanner.instances.getIamPolicy` on
* resource.
*
* Create a request for the method "instances.getIamPolicy".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The Cloud Spanner resource for which the policy is being retrieved. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* database resources.
* @param content the {@link com.google.api.services.spanner.v1.model.GetIamPolicyRequest}
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource, com.google.api.services.spanner.v1.model.GetIamPolicyRequest content) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource, content);
initialize(result);
return result;
}
public class GetIamPolicy extends SpannerRequest {
private static final String REST_PATH = "v1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+$");
/**
* Gets the access control policy for an instance resource. Returns an empty policy if an instance
* exists but does not have a policy set. Authorization requires `spanner.instances.getIamPolicy`
* on resource.
*
* Create a request for the method "instances.getIamPolicy".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
* {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The Cloud Spanner resource for which the policy is being retrieved. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* database resources.
* @param content the {@link com.google.api.services.spanner.v1.model.GetIamPolicyRequest}
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource, com.google.api.services.spanner.v1.model.GetIamPolicyRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The Cloud Spanner resource for which the policy is being retrieved. The format
* is `projects//instances/` for instance resources and `projects//instances//databases/`
* for database resources.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The Cloud Spanner resource for which the policy is being retrieved. The format is
`projects//instances/` for instance resources and `projects//instances//databases/` for database
resources.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The Cloud Spanner resource for which the policy is being retrieved. The format
* is `projects//instances/` for instance resources and `projects//instances//databases/`
* for database resources.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Lists all instances in the given project.
*
* Create a request for the method "instances.list".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the project for which a list of instances is requested. Values are of the form
* `projects/`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends SpannerRequest {
private static final String REST_PATH = "v1/{+parent}/instances";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Lists all instances in the given project.
*
* Create a request for the method "instances.list".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the project for which a list of instances is requested. Values are of the form
* `projects/`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.ListInstancesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the project for which a list of instances is requested. Values are
* of the form `projects/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the project for which a list of instances is requested. Values are of the
form `projects/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the project for which a list of instances is requested. Values are
* of the form `projects/`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* An expression for filtering the results of the request. Filter rules are case
* insensitive. The fields eligible for filtering are: * `name` * `display_name` *
* `labels.key` where key is the name of a label Some examples of using filters are: *
* `name:*` --> The instance has a name. * `name:Howl` --> The instance's name contains the
* string "howl". * `name:HOWL` --> Equivalent to above. * `NAME:howl` --> Equivalent to
* above. * `labels.env:*` --> The instance has the label "env". * `labels.env:dev` --> The
* instance has the label "env" and the value of the label contains the string "dev". *
* `name:howl labels.env:dev` --> The instance's name contains "howl" and it has the label
* "env" with its value containing "dev".
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** An expression for filtering the results of the request. Filter rules are case insensitive. The
fields eligible for filtering are: * `name` * `display_name` * `labels.key` where key is the name
of a label Some examples of using filters are: * `name:*` --> The instance has a name. *
`name:Howl` --> The instance's name contains the string "howl". * `name:HOWL` --> Equivalent to
above. * `NAME:howl` --> Equivalent to above. * `labels.env:*` --> The instance has the label
"env". * `labels.env:dev` --> The instance has the label "env" and the value of the label contains
the string "dev". * `name:howl labels.env:dev` --> The instance's name contains "howl" and it has
the label "env" with its value containing "dev".
*/
public java.lang.String getFilter() {
return filter;
}
/**
* An expression for filtering the results of the request. Filter rules are case
* insensitive. The fields eligible for filtering are: * `name` * `display_name` *
* `labels.key` where key is the name of a label Some examples of using filters are: *
* `name:*` --> The instance has a name. * `name:Howl` --> The instance's name contains the
* string "howl". * `name:HOWL` --> Equivalent to above. * `NAME:howl` --> Equivalent to
* above. * `labels.env:*` --> The instance has the label "env". * `labels.env:dev` --> The
* instance has the label "env" and the value of the label contains the string "dev". *
* `name:howl labels.env:dev` --> The instance's name contains "howl" and it has the label
* "env" with its value containing "dev".
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Deadline used while retrieving metadata for instances. Instances whose metadata cannot be
* retrieved within this deadline will be added to unreachable in ListInstancesResponse.
*/
@com.google.api.client.util.Key
private String instanceDeadline;
/** Deadline used while retrieving metadata for instances. Instances whose metadata cannot be retrieved
within this deadline will be added to unreachable in ListInstancesResponse.
*/
public String getInstanceDeadline() {
return instanceDeadline;
}
/**
* Deadline used while retrieving metadata for instances. Instances whose metadata cannot be
* retrieved within this deadline will be added to unreachable in ListInstancesResponse.
*/
public List setInstanceDeadline(String instanceDeadline) {
this.instanceDeadline = instanceDeadline;
return this;
}
/**
* Number of instances to be returned in the response. If 0 or less, defaults to the
* server's maximum allowed page size.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Number of instances to be returned in the response. If 0 or less, defaults to the server's maximum
allowed page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Number of instances to be returned in the response. If 0 or less, defaults to the
* server's maximum allowed page size.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* If non-empty, `page_token` should contain a next_page_token from a previous
* ListInstancesResponse.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** If non-empty, `page_token` should contain a next_page_token from a previous ListInstancesResponse.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* If non-empty, `page_token` should contain a next_page_token from a previous
* ListInstancesResponse.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Moves the instance to the target instance config. The returned long-running operation can be used
* to track the progress of moving the instance. `MoveInstance` returns `FAILED_PRECONDITION` if the
* instance meets any of the following criteria: * Has an ongoing move to a different instance
* config * Has backups * Has an ongoing update * Is under free trial * Contains any CMEK-enabled
* databases While the operation is pending: * All other attempts to modify the instance, including
* changes to its compute capacity, are rejected. * The following database and backup admin
* operations are rejected: * DatabaseAdmin.CreateDatabase, * DatabaseAdmin.UpdateDatabaseDdl
* (Disabled if default_leader is specified in the request.) * DatabaseAdmin.RestoreDatabase *
* DatabaseAdmin.CreateBackup * DatabaseAdmin.CopyBackup * Both the source and target instance
* configurations are subject to hourly compute and storage charges. * The instance may experience
* higher read-write latencies and a higher transaction abort rate. However, moving an instance does
* not cause any downtime. The returned long-running operation will have a name of the format
* `/operations/` and can be used to track the move instance operation. The metadata field type is
* MoveInstanceMetadata. The response field type is Instance, if successful. Cancelling the
* operation sets its metadata's cancel_time. Cancellation is not immediate since it involves moving
* any data previously moved to target instance configuration back to the original instance config.
* The same operation can be used to track the progress of the cancellation. Upon successful
* completion of the cancellation, the operation terminates with `CANCELLED` status. Upon
* completion(if not cancelled) of the returned operation: * Instance would be successfully moved to
* the target instance config. * You are billed for compute and storage in target instance config.
* Authorization requires `spanner.instances.update` permission on the resource instance. For more
* details, please see [documentation](https://cloud.google.com/spanner/docs/move-instance).
*
* Create a request for the method "instances.move".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Move#execute()} method to invoke the remote operation.
*
* @param name Required. The instance to move. Values are of the form `projects//instances/`.
* @param content the {@link com.google.api.services.spanner.v1.model.MoveInstanceRequest}
* @return the request
*/
public Move move(java.lang.String name, com.google.api.services.spanner.v1.model.MoveInstanceRequest content) throws java.io.IOException {
Move result = new Move(name, content);
initialize(result);
return result;
}
public class Move extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}:move";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+$");
/**
* Moves the instance to the target instance config. The returned long-running operation can be
* used to track the progress of moving the instance. `MoveInstance` returns `FAILED_PRECONDITION`
* if the instance meets any of the following criteria: * Has an ongoing move to a different
* instance config * Has backups * Has an ongoing update * Is under free trial * Contains any
* CMEK-enabled databases While the operation is pending: * All other attempts to modify the
* instance, including changes to its compute capacity, are rejected. * The following database and
* backup admin operations are rejected: * DatabaseAdmin.CreateDatabase, *
* DatabaseAdmin.UpdateDatabaseDdl (Disabled if default_leader is specified in the request.) *
* DatabaseAdmin.RestoreDatabase * DatabaseAdmin.CreateBackup * DatabaseAdmin.CopyBackup * Both
* the source and target instance configurations are subject to hourly compute and storage
* charges. * The instance may experience higher read-write latencies and a higher transaction
* abort rate. However, moving an instance does not cause any downtime. The returned long-running
* operation will have a name of the format `/operations/` and can be used to track the move
* instance operation. The metadata field type is MoveInstanceMetadata. The response field type is
* Instance, if successful. Cancelling the operation sets its metadata's cancel_time. Cancellation
* is not immediate since it involves moving any data previously moved to target instance
* configuration back to the original instance config. The same operation can be used to track the
* progress of the cancellation. Upon successful completion of the cancellation, the operation
* terminates with `CANCELLED` status. Upon completion(if not cancelled) of the returned
* operation: * Instance would be successfully moved to the target instance config. * You are
* billed for compute and storage in target instance config. Authorization requires
* `spanner.instances.update` permission on the resource instance. For more details, please see
* [documentation](https://cloud.google.com/spanner/docs/move-instance).
*
* Create a request for the method "instances.move".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Move#execute()} method to invoke the remote operation. {@link
* Move#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The instance to move. Values are of the form `projects//instances/`.
* @param content the {@link com.google.api.services.spanner.v1.model.MoveInstanceRequest}
* @since 1.13
*/
protected Move(java.lang.String name, com.google.api.services.spanner.v1.model.MoveInstanceRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
}
@Override
public Move set$Xgafv(java.lang.String $Xgafv) {
return (Move) super.set$Xgafv($Xgafv);
}
@Override
public Move setAccessToken(java.lang.String accessToken) {
return (Move) super.setAccessToken(accessToken);
}
@Override
public Move setAlt(java.lang.String alt) {
return (Move) super.setAlt(alt);
}
@Override
public Move setCallback(java.lang.String callback) {
return (Move) super.setCallback(callback);
}
@Override
public Move setFields(java.lang.String fields) {
return (Move) super.setFields(fields);
}
@Override
public Move setKey(java.lang.String key) {
return (Move) super.setKey(key);
}
@Override
public Move setOauthToken(java.lang.String oauthToken) {
return (Move) super.setOauthToken(oauthToken);
}
@Override
public Move setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Move) super.setPrettyPrint(prettyPrint);
}
@Override
public Move setQuotaUser(java.lang.String quotaUser) {
return (Move) super.setQuotaUser(quotaUser);
}
@Override
public Move setUploadType(java.lang.String uploadType) {
return (Move) super.setUploadType(uploadType);
}
@Override
public Move setUploadProtocol(java.lang.String uploadProtocol) {
return (Move) super.setUploadProtocol(uploadProtocol);
}
/** Required. The instance to move. Values are of the form `projects//instances/`. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The instance to move. Values are of the form `projects//instances/`.
*/
public java.lang.String getName() {
return name;
}
/** Required. The instance to move. Values are of the form `projects//instances/`. */
public Move setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Move set(String parameterName, Object value) {
return (Move) super.set(parameterName, value);
}
}
/**
* Updates an instance, and begins allocating or releasing resources as requested. The returned
* long-running operation can be used to track the progress of updating the instance. If the named
* instance does not exist, returns `NOT_FOUND`. Immediately upon completion of this request: * For
* resource types for which a decrease in the instance's allocation has been requested, billing is
* based on the newly-requested level. Until completion of the returned operation: * Cancelling the
* operation sets its metadata's cancel_time, and begins restoring resources to their pre-request
* values. The operation is guaranteed to succeed at undoing all resource changes, after which point
* it terminates with a `CANCELLED` status. * All other attempts to modify the instance are
* rejected. * Reading the instance via the API continues to give the pre-request resource levels.
* Upon completion of the returned operation: * Billing begins for all successfully-allocated
* resources (some types may have lower than the requested levels). * All newly-reserved resources
* are available for serving the instance's tables. * The instance's new resource levels are
* readable via the API. The returned long-running operation will have a name of the format
* `/operations/` and can be used to track the instance modification. The metadata field type is
* UpdateInstanceMetadata. The response field type is Instance, if successful. Authorization
* requires `spanner.instances.update` permission on the resource name.
*
* Create a request for the method "instances.patch".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. A unique identifier for the instance, which cannot be changed after the instance is
* created. Values are of the form `projects//instances/a-z*[a-z0-9]`. The final segment of
* the name must be between 2 and 64 characters in length.
* @param content the {@link com.google.api.services.spanner.v1.model.UpdateInstanceRequest}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.spanner.v1.model.UpdateInstanceRequest content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+$");
/**
* Updates an instance, and begins allocating or releasing resources as requested. The returned
* long-running operation can be used to track the progress of updating the instance. If the named
* instance does not exist, returns `NOT_FOUND`. Immediately upon completion of this request: *
* For resource types for which a decrease in the instance's allocation has been requested,
* billing is based on the newly-requested level. Until completion of the returned operation: *
* Cancelling the operation sets its metadata's cancel_time, and begins restoring resources to
* their pre-request values. The operation is guaranteed to succeed at undoing all resource
* changes, after which point it terminates with a `CANCELLED` status. * All other attempts to
* modify the instance are rejected. * Reading the instance via the API continues to give the pre-
* request resource levels. Upon completion of the returned operation: * Billing begins for all
* successfully-allocated resources (some types may have lower than the requested levels). * All
* newly-reserved resources are available for serving the instance's tables. * The instance's new
* resource levels are readable via the API. The returned long-running operation will have a name
* of the format `/operations/` and can be used to track the instance modification. The metadata
* field type is UpdateInstanceMetadata. The response field type is Instance, if successful.
* Authorization requires `spanner.instances.update` permission on the resource name.
*
* Create a request for the method "instances.patch".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. A unique identifier for the instance, which cannot be changed after the instance is
* created. Values are of the form `projects//instances/a-z*[a-z0-9]`. The final segment of
* the name must be between 2 and 64 characters in length.
* @param content the {@link com.google.api.services.spanner.v1.model.UpdateInstanceRequest}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.spanner.v1.model.UpdateInstanceRequest content) {
super(Spanner.this, "PATCH", REST_PATH, content, com.google.api.services.spanner.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. A unique identifier for the instance, which cannot be changed after the
* instance is created. Values are of the form `projects//instances/a-z*[a-z0-9]`. The final
* segment of the name must be between 2 and 64 characters in length.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. A unique identifier for the instance, which cannot be changed after the instance is
created. Values are of the form `projects//instances/a-z*[a-z0-9]`. The final segment of the name
must be between 2 and 64 characters in length.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. A unique identifier for the instance, which cannot be changed after the
* instance is created. Values are of the form `projects//instances/a-z*[a-z0-9]`. The final
* segment of the name must be between 2 and 64 characters in length.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on an instance resource. Replaces any existing policy.
* Authorization requires `spanner.instances.setIamPolicy` on resource.
*
* Create a request for the method "instances.setIamPolicy".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The Cloud Spanner resource for which the policy is being set. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* databases resources.
* @param content the {@link com.google.api.services.spanner.v1.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.spanner.v1.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends SpannerRequest {
private static final String REST_PATH = "v1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+$");
/**
* Sets the access control policy on an instance resource. Replaces any existing policy.
* Authorization requires `spanner.instances.setIamPolicy` on resource.
*
* Create a request for the method "instances.setIamPolicy".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
* {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The Cloud Spanner resource for which the policy is being set. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* databases resources.
* @param content the {@link com.google.api.services.spanner.v1.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.spanner.v1.model.SetIamPolicyRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The Cloud Spanner resource for which the policy is being set. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* databases resources.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The Cloud Spanner resource for which the policy is being set. The format is
`projects//instances/` for instance resources and `projects//instances//databases/` for databases
resources.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The Cloud Spanner resource for which the policy is being set. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* databases resources.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that the caller has on the specified instance resource. Attempting this RPC
* on a non-existent Cloud Spanner instance resource will result in a NOT_FOUND error if the user
* has `spanner.instances.list` permission on the containing Google Cloud Project. Otherwise returns
* an empty set of permissions.
*
* Create a request for the method "instances.testIamPermissions".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The Cloud Spanner resource for which permissions are being tested. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* database resources.
* @param content the {@link com.google.api.services.spanner.v1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.spanner.v1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends SpannerRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+$");
/**
* Returns permissions that the caller has on the specified instance resource. Attempting this RPC
* on a non-existent Cloud Spanner instance resource will result in a NOT_FOUND error if the user
* has `spanner.instances.list` permission on the containing Google Cloud Project. Otherwise
* returns an empty set of permissions.
*
* Create a request for the method "instances.testIamPermissions".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The Cloud Spanner resource for which permissions are being tested. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* database resources.
* @param content the {@link com.google.api.services.spanner.v1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.spanner.v1.model.TestIamPermissionsRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The Cloud Spanner resource for which permissions are being tested. The format
* is `projects//instances/` for instance resources and `projects//instances//databases/`
* for database resources.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The Cloud Spanner resource for which permissions are being tested. The format is
`projects//instances/` for instance resources and `projects//instances//databases/` for database
resources.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The Cloud Spanner resource for which permissions are being tested. The format
* is `projects//instances/` for instance resources and `projects//instances//databases/`
* for database resources.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the BackupOperations collection.
*
* The typical use is:
*
* {@code Spanner spanner = new Spanner(...);}
* {@code Spanner.BackupOperations.List request = spanner.backupOperations().list(parameters ...)}
*
*
* @return the resource collection
*/
public BackupOperations backupOperations() {
return new BackupOperations();
}
/**
* The "backupOperations" collection of methods.
*/
public class BackupOperations {
/**
* Lists the backup long-running operations in the given instance. A backup operation has a name of
* the form `projects//instances//backups//operations/`. The long-running operation metadata field
* type `metadata.type_url` describes the type of the metadata. Operations returned include those
* that have completed/failed/canceled within the last 7 days, and pending operations. Operations
* returned are ordered by `operation.metadata.value.progress.start_time` in descending order
* starting from the most recently started operation.
*
* Create a request for the method "backupOperations.list".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The instance of the backup operations. Values are of the form `projects//instances/`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends SpannerRequest {
private static final String REST_PATH = "v1/{+parent}/backupOperations";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+$");
/**
* Lists the backup long-running operations in the given instance. A backup operation has a name
* of the form `projects//instances//backups//operations/`. The long-running operation metadata
* field type `metadata.type_url` describes the type of the metadata. Operations returned include
* those that have completed/failed/canceled within the last 7 days, and pending operations.
* Operations returned are ordered by `operation.metadata.value.progress.start_time` in descending
* order starting from the most recently started operation.
*
* Create a request for the method "backupOperations.list".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The instance of the backup operations. Values are of the form `projects//instances/`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.ListBackupOperationsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The instance of the backup operations. Values are of the form
* `projects//instances/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The instance of the backup operations. Values are of the form `projects//instances/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The instance of the backup operations. Values are of the form
* `projects//instances/`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* An expression that filters the list of returned backup operations. A filter expression
* consists of a field name, a comparison operator, and a value for filtering. The value
* must be a string, a number, or a boolean. The comparison operator must be one of: `<`,
* `>`, `<=`, `>=`, `!=`, `=`, or `:`. Colon `:` is the contains operator. Filter rules
* are not case sensitive. The following fields in the operation are eligible for
* filtering: * `name` - The name of the long-running operation * `done` - False if the
* operation is in progress, else true. * `metadata.@type` - the type of metadata. For
* example, the type string for CreateBackupMetadata is
* `type.googleapis.com/google.spanner.admin.database.v1.CreateBackupMetadata`. *
* `metadata.` - any field in metadata.value. `metadata.@type` must be specified first if
* filtering on metadata fields. * `error` - Error associated with the long-running
* operation. * `response.@type` - the type of response. * `response.` - any field in
* response.value. You can combine multiple expressions by enclosing each expression in
* parentheses. By default, expressions are combined with AND logic, but you can specify
* AND, OR, and NOT logic explicitly. Here are a few examples: * `done:true` - The
* operation is complete. * `(metadata.@type=type.googleapis.com/google.spanner.admin.data
* base.v1.CreateBackupMetadata) AND` \ `metadata.database:prod` - Returns operations
* where: * The operation's metadata type is CreateBackupMetadata. * The source database
* name of backup contains the string "prod". * `(metadata.@type=type.googleapis.com/googl
* e.spanner.admin.database.v1.CreateBackupMetadata) AND` \ `(metadata.name:howl) AND` \
* `(metadata.progress.start_time < \"2018-03-28T14:50:00Z\") AND` \ `(error:*)` - Returns
* operations where: * The operation's metadata type is CreateBackupMetadata. * The backup
* name contains the string "howl". * The operation started before 2018-03-28T14:50:00Z. *
* The operation resulted in an error. * `(metadata.@type=type.googleapis.com/google.spann
* er.admin.database.v1.CopyBackupMetadata) AND` \ `(metadata.source_backup:test) AND` \
* `(metadata.progress.start_time < \"2022-01-18T14:50:00Z\") AND` \ `(error:*)` - Returns
* operations where: * The operation's metadata type is CopyBackupMetadata. * The source
* backup name contains the string "test". * The operation started before
* 2022-01-18T14:50:00Z. * The operation resulted in an error. * `((metadata.@type=type.go
* ogleapis.com/google.spanner.admin.database.v1.CreateBackupMetadata) AND` \
* `(metadata.database:test_db)) OR` \ `((metadata.@type=type.googleapis.com/google.spanne
* r.admin.database.v1.CopyBackupMetadata) AND` \ `(metadata.source_backup:test_bkp)) AND`
* \ `(error:*)` - Returns operations where: * The operation's metadata matches either of
* criteria: * The operation's metadata type is CreateBackupMetadata AND the source
* database name of the backup contains the string "test_db" * The operation's metadata
* type is CopyBackupMetadata AND the source backup name contains the string "test_bkp" *
* The operation resulted in an error.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** An expression that filters the list of returned backup operations. A filter expression consists of
a field name, a comparison operator, and a value for filtering. The value must be a string, a
number, or a boolean. The comparison operator must be one of: `<`, `>`, `<=`, `>=`, `!=`, `=`, or
`:`. Colon `:` is the contains operator. Filter rules are not case sensitive. The following fields
in the operation are eligible for filtering: * `name` - The name of the long-running operation *
`done` - False if the operation is in progress, else true. * `metadata.@type` - the type of
metadata. For example, the type string for CreateBackupMetadata is
`type.googleapis.com/google.spanner.admin.database.v1.CreateBackupMetadata`. * `metadata.` - any
field in metadata.value. `metadata.@type` must be specified first if filtering on metadata fields.
* `error` - Error associated with the long-running operation. * `response.@type` - the type of
response. * `response.` - any field in response.value. You can combine multiple expressions by
enclosing each expression in parentheses. By default, expressions are combined with AND logic, but
you can specify AND, OR, and NOT logic explicitly. Here are a few examples: * `done:true` - The
operation is complete. *
`(metadata.@type=type.googleapis.com/google.spanner.admin.database.v1.CreateBackupMetadata) AND` \
`metadata.database:prod` - Returns operations where: * The operation's metadata type is
CreateBackupMetadata. * The source database name of backup contains the string "prod". *
`(metadata.@type=type.googleapis.com/google.spanner.admin.database.v1.CreateBackupMetadata) AND` \
`(metadata.name:howl) AND` \ `(metadata.progress.start_time < \"2018-03-28T14:50:00Z\") AND` \
`(error:*)` - Returns operations where: * The operation's metadata type is CreateBackupMetadata. *
The backup name contains the string "howl". * The operation started before 2018-03-28T14:50:00Z. *
The operation resulted in an error. *
`(metadata.@type=type.googleapis.com/google.spanner.admin.database.v1.CopyBackupMetadata) AND` \
`(metadata.source_backup:test) AND` \ `(metadata.progress.start_time < \"2022-01-18T14:50:00Z\")
AND` \ `(error:*)` - Returns operations where: * The operation's metadata type is
CopyBackupMetadata. * The source backup name contains the string "test". * The operation started
before 2022-01-18T14:50:00Z. * The operation resulted in an error. *
`((metadata.@type=type.googleapis.com/google.spanner.admin.database.v1.CreateBackupMetadata) AND` \
`(metadata.database:test_db)) OR` \
`((metadata.@type=type.googleapis.com/google.spanner.admin.database.v1.CopyBackupMetadata) AND` \
`(metadata.source_backup:test_bkp)) AND` \ `(error:*)` - Returns operations where: * The
operation's metadata matches either of criteria: * The operation's metadata type is
CreateBackupMetadata AND the source database name of the backup contains the string "test_db" * The
operation's metadata type is CopyBackupMetadata AND the source backup name contains the string
"test_bkp" * The operation resulted in an error.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* An expression that filters the list of returned backup operations. A filter expression
* consists of a field name, a comparison operator, and a value for filtering. The value
* must be a string, a number, or a boolean. The comparison operator must be one of: `<`,
* `>`, `<=`, `>=`, `!=`, `=`, or `:`. Colon `:` is the contains operator. Filter rules
* are not case sensitive. The following fields in the operation are eligible for
* filtering: * `name` - The name of the long-running operation * `done` - False if the
* operation is in progress, else true. * `metadata.@type` - the type of metadata. For
* example, the type string for CreateBackupMetadata is
* `type.googleapis.com/google.spanner.admin.database.v1.CreateBackupMetadata`. *
* `metadata.` - any field in metadata.value. `metadata.@type` must be specified first if
* filtering on metadata fields. * `error` - Error associated with the long-running
* operation. * `response.@type` - the type of response. * `response.` - any field in
* response.value. You can combine multiple expressions by enclosing each expression in
* parentheses. By default, expressions are combined with AND logic, but you can specify
* AND, OR, and NOT logic explicitly. Here are a few examples: * `done:true` - The
* operation is complete. * `(metadata.@type=type.googleapis.com/google.spanner.admin.data
* base.v1.CreateBackupMetadata) AND` \ `metadata.database:prod` - Returns operations
* where: * The operation's metadata type is CreateBackupMetadata. * The source database
* name of backup contains the string "prod". * `(metadata.@type=type.googleapis.com/googl
* e.spanner.admin.database.v1.CreateBackupMetadata) AND` \ `(metadata.name:howl) AND` \
* `(metadata.progress.start_time < \"2018-03-28T14:50:00Z\") AND` \ `(error:*)` - Returns
* operations where: * The operation's metadata type is CreateBackupMetadata. * The backup
* name contains the string "howl". * The operation started before 2018-03-28T14:50:00Z. *
* The operation resulted in an error. * `(metadata.@type=type.googleapis.com/google.spann
* er.admin.database.v1.CopyBackupMetadata) AND` \ `(metadata.source_backup:test) AND` \
* `(metadata.progress.start_time < \"2022-01-18T14:50:00Z\") AND` \ `(error:*)` - Returns
* operations where: * The operation's metadata type is CopyBackupMetadata. * The source
* backup name contains the string "test". * The operation started before
* 2022-01-18T14:50:00Z. * The operation resulted in an error. * `((metadata.@type=type.go
* ogleapis.com/google.spanner.admin.database.v1.CreateBackupMetadata) AND` \
* `(metadata.database:test_db)) OR` \ `((metadata.@type=type.googleapis.com/google.spanne
* r.admin.database.v1.CopyBackupMetadata) AND` \ `(metadata.source_backup:test_bkp)) AND`
* \ `(error:*)` - Returns operations where: * The operation's metadata matches either of
* criteria: * The operation's metadata type is CreateBackupMetadata AND the source
* database name of the backup contains the string "test_db" * The operation's metadata
* type is CopyBackupMetadata AND the source backup name contains the string "test_bkp" *
* The operation resulted in an error.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Number of operations to be returned in the response. If 0 or less, defaults to the
* server's maximum allowed page size.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Number of operations to be returned in the response. If 0 or less, defaults to the server's maximum
allowed page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Number of operations to be returned in the response. If 0 or less, defaults to the
* server's maximum allowed page size.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* If non-empty, `page_token` should contain a next_page_token from a previous
* ListBackupOperationsResponse to the same `parent` and with the same `filter`.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** If non-empty, `page_token` should contain a next_page_token from a previous
ListBackupOperationsResponse to the same `parent` and with the same `filter`.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* If non-empty, `page_token` should contain a next_page_token from a previous
* ListBackupOperationsResponse to the same `parent` and with the same `filter`.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Backups collection.
*
* The typical use is:
*
* {@code Spanner spanner = new Spanner(...);}
* {@code Spanner.Backups.List request = spanner.backups().list(parameters ...)}
*
*
* @return the resource collection
*/
public Backups backups() {
return new Backups();
}
/**
* The "backups" collection of methods.
*/
public class Backups {
/**
* Starts copying a Cloud Spanner Backup. The returned backup long-running operation will have a
* name of the format `projects//instances//backups//operations/` and can be used to track copying
* of the backup. The operation is associated with the destination backup. The metadata field type
* is CopyBackupMetadata. The response field type is Backup, if successful. Cancelling the returned
* operation will stop the copying and delete the destination backup. Concurrent CopyBackup requests
* can run on the same source backup.
*
* Create a request for the method "backups.copy".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Copy#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the destination instance that will contain the backup copy. Values are of the
* form: `projects//instances/`.
* @param content the {@link com.google.api.services.spanner.v1.model.CopyBackupRequest}
* @return the request
*/
public Copy copy(java.lang.String parent, com.google.api.services.spanner.v1.model.CopyBackupRequest content) throws java.io.IOException {
Copy result = new Copy(parent, content);
initialize(result);
return result;
}
public class Copy extends SpannerRequest {
private static final String REST_PATH = "v1/{+parent}/backups:copy";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+$");
/**
* Starts copying a Cloud Spanner Backup. The returned backup long-running operation will have a
* name of the format `projects//instances//backups//operations/` and can be used to track copying
* of the backup. The operation is associated with the destination backup. The metadata field type
* is CopyBackupMetadata. The response field type is Backup, if successful. Cancelling the
* returned operation will stop the copying and delete the destination backup. Concurrent
* CopyBackup requests can run on the same source backup.
*
* Create a request for the method "backups.copy".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Copy#execute()} method to invoke the remote operation. {@link
* Copy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the destination instance that will contain the backup copy. Values are of the
* form: `projects//instances/`.
* @param content the {@link com.google.api.services.spanner.v1.model.CopyBackupRequest}
* @since 1.13
*/
protected Copy(java.lang.String parent, com.google.api.services.spanner.v1.model.CopyBackupRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
}
@Override
public Copy set$Xgafv(java.lang.String $Xgafv) {
return (Copy) super.set$Xgafv($Xgafv);
}
@Override
public Copy setAccessToken(java.lang.String accessToken) {
return (Copy) super.setAccessToken(accessToken);
}
@Override
public Copy setAlt(java.lang.String alt) {
return (Copy) super.setAlt(alt);
}
@Override
public Copy setCallback(java.lang.String callback) {
return (Copy) super.setCallback(callback);
}
@Override
public Copy setFields(java.lang.String fields) {
return (Copy) super.setFields(fields);
}
@Override
public Copy setKey(java.lang.String key) {
return (Copy) super.setKey(key);
}
@Override
public Copy setOauthToken(java.lang.String oauthToken) {
return (Copy) super.setOauthToken(oauthToken);
}
@Override
public Copy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Copy) super.setPrettyPrint(prettyPrint);
}
@Override
public Copy setQuotaUser(java.lang.String quotaUser) {
return (Copy) super.setQuotaUser(quotaUser);
}
@Override
public Copy setUploadType(java.lang.String uploadType) {
return (Copy) super.setUploadType(uploadType);
}
@Override
public Copy setUploadProtocol(java.lang.String uploadProtocol) {
return (Copy) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the destination instance that will contain the backup copy.
* Values are of the form: `projects//instances/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the destination instance that will contain the backup copy. Values are of the
form: `projects//instances/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the destination instance that will contain the backup copy.
* Values are of the form: `projects//instances/`.
*/
public Copy setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Copy set(String parameterName, Object value) {
return (Copy) super.set(parameterName, value);
}
}
/**
* Starts creating a new Cloud Spanner Backup. The returned backup long-running operation will have
* a name of the format `projects//instances//backups//operations/` and can be used to track
* creation of the backup. The metadata field type is CreateBackupMetadata. The response field type
* is Backup, if successful. Cancelling the returned operation will stop the creation and delete the
* backup. There can be only one pending backup creation per database. Backup creation of different
* databases can run concurrently.
*
* Create a request for the method "backups.create".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the instance in which the backup will be created. This must be the same
* instance that contains the database the backup will be created from. The backup will be
* stored in the location(s) specified in the instance configuration of this instance. Values
* are of the form `projects//instances/`.
* @param content the {@link com.google.api.services.spanner.v1.model.Backup}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.spanner.v1.model.Backup content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends SpannerRequest {
private static final String REST_PATH = "v1/{+parent}/backups";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+$");
/**
* Starts creating a new Cloud Spanner Backup. The returned backup long-running operation will
* have a name of the format `projects//instances//backups//operations/` and can be used to track
* creation of the backup. The metadata field type is CreateBackupMetadata. The response field
* type is Backup, if successful. Cancelling the returned operation will stop the creation and
* delete the backup. There can be only one pending backup creation per database. Backup creation
* of different databases can run concurrently.
*
* Create a request for the method "backups.create".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the instance in which the backup will be created. This must be the same
* instance that contains the database the backup will be created from. The backup will be
* stored in the location(s) specified in the instance configuration of this instance. Values
* are of the form `projects//instances/`.
* @param content the {@link com.google.api.services.spanner.v1.model.Backup}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.spanner.v1.model.Backup content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the instance in which the backup will be created. This must be
* the same instance that contains the database the backup will be created from. The
* backup will be stored in the location(s) specified in the instance configuration of
* this instance. Values are of the form `projects//instances/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the instance in which the backup will be created. This must be the same
instance that contains the database the backup will be created from. The backup will be stored in
the location(s) specified in the instance configuration of this instance. Values are of the form
`projects//instances/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the instance in which the backup will be created. This must be
* the same instance that contains the database the backup will be created from. The
* backup will be stored in the location(s) specified in the instance configuration of
* this instance. Values are of the form `projects//instances/`.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The id of the backup to be created. The `backup_id` appended to `parent`
* forms the full backup name of the form `projects//instances//backups/`.
*/
@com.google.api.client.util.Key
private java.lang.String backupId;
/** Required. The id of the backup to be created. The `backup_id` appended to `parent` forms the full
backup name of the form `projects//instances//backups/`.
*/
public java.lang.String getBackupId() {
return backupId;
}
/**
* Required. The id of the backup to be created. The `backup_id` appended to `parent`
* forms the full backup name of the form `projects//instances//backups/`.
*/
public Create setBackupId(java.lang.String backupId) {
this.backupId = backupId;
return this;
}
/** Required. The encryption type of the backup. */
@com.google.api.client.util.Key("encryptionConfig.encryptionType")
private java.lang.String encryptionConfigEncryptionType;
/** Required. The encryption type of the backup.
*/
public java.lang.String getEncryptionConfigEncryptionType() {
return encryptionConfigEncryptionType;
}
/** Required. The encryption type of the backup. */
public Create setEncryptionConfigEncryptionType(java.lang.String encryptionConfigEncryptionType) {
this.encryptionConfigEncryptionType = encryptionConfigEncryptionType;
return this;
}
/**
* Optional. The Cloud KMS key that will be used to protect the backup. This field should
* be set only when encryption_type is `CUSTOMER_MANAGED_ENCRYPTION`. Values are of the
* form `projects//locations//keyRings//cryptoKeys/`.
*/
@com.google.api.client.util.Key("encryptionConfig.kmsKeyName")
private java.lang.String encryptionConfigKmsKeyName;
/** Optional. The Cloud KMS key that will be used to protect the backup. This field should be set only
when encryption_type is `CUSTOMER_MANAGED_ENCRYPTION`. Values are of the form
`projects//locations//keyRings//cryptoKeys/`.
*/
public java.lang.String getEncryptionConfigKmsKeyName() {
return encryptionConfigKmsKeyName;
}
/**
* Optional. The Cloud KMS key that will be used to protect the backup. This field should
* be set only when encryption_type is `CUSTOMER_MANAGED_ENCRYPTION`. Values are of the
* form `projects//locations//keyRings//cryptoKeys/`.
*/
public Create setEncryptionConfigKmsKeyName(java.lang.String encryptionConfigKmsKeyName) {
this.encryptionConfigKmsKeyName = encryptionConfigKmsKeyName;
return this;
}
/**
* Optional. Specifies the KMS configuration for the one or more keys used to protect the
* backup. Values are of the form `projects//locations//keyRings//cryptoKeys/`. The keys
* referenced by kms_key_names must fully cover all regions of the backup's instance
* configuration. Some examples: * For single region instance configs, specify a single
* regional location KMS key. * For multi-regional instance configs of type
* GOOGLE_MANAGED, either specify a multi-regional location KMS key or multiple regional
* location KMS keys that cover all regions in the instance config. * For an instance
* config of type USER_MANAGED, please specify only regional location KMS keys to cover
* each region in the instance config. Multi-regional location KMS keys are not supported
* for USER_MANAGED instance configs.
*/
@com.google.api.client.util.Key("encryptionConfig.kmsKeyNames")
private java.util.List encryptionConfigKmsKeyNames;
/** Optional. Specifies the KMS configuration for the one or more keys used to protect the backup.
Values are of the form `projects//locations//keyRings//cryptoKeys/`. The keys referenced by
kms_key_names must fully cover all regions of the backup's instance configuration. Some examples: *
For single region instance configs, specify a single regional location KMS key. * For multi-
regional instance configs of type GOOGLE_MANAGED, either specify a multi-regional location KMS key
or multiple regional location KMS keys that cover all regions in the instance config. * For an
instance config of type USER_MANAGED, please specify only regional location KMS keys to cover each
region in the instance config. Multi-regional location KMS keys are not supported for USER_MANAGED
instance configs.
*/
public java.util.List getEncryptionConfigKmsKeyNames() {
return encryptionConfigKmsKeyNames;
}
/**
* Optional. Specifies the KMS configuration for the one or more keys used to protect the
* backup. Values are of the form `projects//locations//keyRings//cryptoKeys/`. The keys
* referenced by kms_key_names must fully cover all regions of the backup's instance
* configuration. Some examples: * For single region instance configs, specify a single
* regional location KMS key. * For multi-regional instance configs of type
* GOOGLE_MANAGED, either specify a multi-regional location KMS key or multiple regional
* location KMS keys that cover all regions in the instance config. * For an instance
* config of type USER_MANAGED, please specify only regional location KMS keys to cover
* each region in the instance config. Multi-regional location KMS keys are not supported
* for USER_MANAGED instance configs.
*/
public Create setEncryptionConfigKmsKeyNames(java.util.List encryptionConfigKmsKeyNames) {
this.encryptionConfigKmsKeyNames = encryptionConfigKmsKeyNames;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a pending or completed Backup.
*
* Create a request for the method "backups.delete".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the backup to delete. Values are of the form `projects//instances//backups/`.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/backups/[^/]+$");
/**
* Deletes a pending or completed Backup.
*
* Create a request for the method "backups.delete".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the backup to delete. Values are of the form `projects//instances//backups/`.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Spanner.this, "DELETE", REST_PATH, null, com.google.api.services.spanner.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/backups/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the backup to delete. Values are of the form
* `projects//instances//backups/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the backup to delete. Values are of the form `projects//instances//backups/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the backup to delete. Values are of the form
* `projects//instances//backups/`.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/backups/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets metadata on a pending or completed Backup.
*
* Create a request for the method "backups.get".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the backup. Values are of the form `projects//instances//backups/`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/backups/[^/]+$");
/**
* Gets metadata on a pending or completed Backup.
*
* Create a request for the method "backups.get".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the backup. Values are of the form `projects//instances//backups/`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.Backup.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/backups/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the backup. Values are of the form `projects//instances//backups/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the backup. Values are of the form `projects//instances//backups/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the backup. Values are of the form `projects//instances//backups/`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/backups/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a database or backup resource. Returns an empty policy if a
* database or backup exists but does not have a policy set. Authorization requires
* `spanner.databases.getIamPolicy` permission on resource. For backups, authorization requires
* `spanner.backups.getIamPolicy` permission on resource.
*
* Create a request for the method "backups.getIamPolicy".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The Cloud Spanner resource for which the policy is being retrieved. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* database resources.
* @param content the {@link com.google.api.services.spanner.v1.model.GetIamPolicyRequest}
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource, com.google.api.services.spanner.v1.model.GetIamPolicyRequest content) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource, content);
initialize(result);
return result;
}
public class GetIamPolicy extends SpannerRequest {
private static final String REST_PATH = "v1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/backups/[^/]+$");
/**
* Gets the access control policy for a database or backup resource. Returns an empty policy if a
* database or backup exists but does not have a policy set. Authorization requires
* `spanner.databases.getIamPolicy` permission on resource. For backups, authorization requires
* `spanner.backups.getIamPolicy` permission on resource.
*
* Create a request for the method "backups.getIamPolicy".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
* {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The Cloud Spanner resource for which the policy is being retrieved. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* database resources.
* @param content the {@link com.google.api.services.spanner.v1.model.GetIamPolicyRequest}
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource, com.google.api.services.spanner.v1.model.GetIamPolicyRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/backups/[^/]+$");
}
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The Cloud Spanner resource for which the policy is being retrieved. The
* format is `projects//instances/` for instance resources and
* `projects//instances//databases/` for database resources.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The Cloud Spanner resource for which the policy is being retrieved. The format is
`projects//instances/` for instance resources and `projects//instances//databases/` for database
resources.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The Cloud Spanner resource for which the policy is being retrieved. The
* format is `projects//instances/` for instance resources and
* `projects//instances//databases/` for database resources.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/backups/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Lists completed and pending backups. Backups returned are ordered by `create_time` in descending
* order, starting from the most recent `create_time`.
*
* Create a request for the method "backups.list".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The instance to list backups from. Values are of the form `projects//instances/`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends SpannerRequest {
private static final String REST_PATH = "v1/{+parent}/backups";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+$");
/**
* Lists completed and pending backups. Backups returned are ordered by `create_time` in
* descending order, starting from the most recent `create_time`.
*
* Create a request for the method "backups.list".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The instance to list backups from. Values are of the form `projects//instances/`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.ListBackupsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The instance to list backups from. Values are of the form
* `projects//instances/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The instance to list backups from. Values are of the form `projects//instances/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The instance to list backups from. Values are of the form
* `projects//instances/`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* An expression that filters the list of returned backups. A filter expression consists
* of a field name, a comparison operator, and a value for filtering. The value must be a
* string, a number, or a boolean. The comparison operator must be one of: `<`, `>`, `<=`,
* `>=`, `!=`, `=`, or `:`. Colon `:` is the contains operator. Filter rules are not case
* sensitive. The following fields in the Backup are eligible for filtering: * `name` *
* `database` * `state` * `create_time` (and values are of the format YYYY-MM-
* DDTHH:MM:SSZ) * `expire_time` (and values are of the format YYYY-MM-DDTHH:MM:SSZ) *
* `version_time` (and values are of the format YYYY-MM-DDTHH:MM:SSZ) * `size_bytes` You
* can combine multiple expressions by enclosing each expression in parentheses. By
* default, expressions are combined with AND logic, but you can specify AND, OR, and NOT
* logic explicitly. Here are a few examples: * `name:Howl` - The backup's name contains
* the string "howl". * `database:prod` - The database's name contains the string "prod".
* * `state:CREATING` - The backup is pending creation. * `state:READY` - The backup is
* fully created and ready for use. * `(name:howl) AND (create_time <
* \"2018-03-28T14:50:00Z\")` - The backup name contains the string "howl" and
* `create_time` of the backup is before 2018-03-28T14:50:00Z. * `expire_time <
* \"2018-03-28T14:50:00Z\"` - The backup `expire_time` is before 2018-03-28T14:50:00Z. *
* `size_bytes > 10000000000` - The backup's size is greater than 10GB
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** An expression that filters the list of returned backups. A filter expression consists of a field
name, a comparison operator, and a value for filtering. The value must be a string, a number, or a
boolean. The comparison operator must be one of: `<`, `>`, `<=`, `>=`, `!=`, `=`, or `:`. Colon `:`
is the contains operator. Filter rules are not case sensitive. The following fields in the Backup
are eligible for filtering: * `name` * `database` * `state` * `create_time` (and values are of the
format YYYY-MM-DDTHH:MM:SSZ) * `expire_time` (and values are of the format YYYY-MM-DDTHH:MM:SSZ) *
`version_time` (and values are of the format YYYY-MM-DDTHH:MM:SSZ) * `size_bytes` You can combine
multiple expressions by enclosing each expression in parentheses. By default, expressions are
combined with AND logic, but you can specify AND, OR, and NOT logic explicitly. Here are a few
examples: * `name:Howl` - The backup's name contains the string "howl". * `database:prod` - The
database's name contains the string "prod". * `state:CREATING` - The backup is pending creation. *
`state:READY` - The backup is fully created and ready for use. * `(name:howl) AND (create_time <
\"2018-03-28T14:50:00Z\")` - The backup name contains the string "howl" and `create_time` of the
backup is before 2018-03-28T14:50:00Z. * `expire_time < \"2018-03-28T14:50:00Z\"` - The backup
`expire_time` is before 2018-03-28T14:50:00Z. * `size_bytes > 10000000000` - The backup's size is
greater than 10GB
*/
public java.lang.String getFilter() {
return filter;
}
/**
* An expression that filters the list of returned backups. A filter expression consists
* of a field name, a comparison operator, and a value for filtering. The value must be a
* string, a number, or a boolean. The comparison operator must be one of: `<`, `>`, `<=`,
* `>=`, `!=`, `=`, or `:`. Colon `:` is the contains operator. Filter rules are not case
* sensitive. The following fields in the Backup are eligible for filtering: * `name` *
* `database` * `state` * `create_time` (and values are of the format YYYY-MM-
* DDTHH:MM:SSZ) * `expire_time` (and values are of the format YYYY-MM-DDTHH:MM:SSZ) *
* `version_time` (and values are of the format YYYY-MM-DDTHH:MM:SSZ) * `size_bytes` You
* can combine multiple expressions by enclosing each expression in parentheses. By
* default, expressions are combined with AND logic, but you can specify AND, OR, and NOT
* logic explicitly. Here are a few examples: * `name:Howl` - The backup's name contains
* the string "howl". * `database:prod` - The database's name contains the string "prod".
* * `state:CREATING` - The backup is pending creation. * `state:READY` - The backup is
* fully created and ready for use. * `(name:howl) AND (create_time <
* \"2018-03-28T14:50:00Z\")` - The backup name contains the string "howl" and
* `create_time` of the backup is before 2018-03-28T14:50:00Z. * `expire_time <
* \"2018-03-28T14:50:00Z\"` - The backup `expire_time` is before 2018-03-28T14:50:00Z. *
* `size_bytes > 10000000000` - The backup's size is greater than 10GB
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Number of backups to be returned in the response. If 0 or less, defaults to the
* server's maximum allowed page size.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Number of backups to be returned in the response. If 0 or less, defaults to the server's maximum
allowed page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Number of backups to be returned in the response. If 0 or less, defaults to the
* server's maximum allowed page size.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* If non-empty, `page_token` should contain a next_page_token from a previous
* ListBackupsResponse to the same `parent` and with the same `filter`.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** If non-empty, `page_token` should contain a next_page_token from a previous ListBackupsResponse to
the same `parent` and with the same `filter`.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* If non-empty, `page_token` should contain a next_page_token from a previous
* ListBackupsResponse to the same `parent` and with the same `filter`.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a pending or completed Backup.
*
* Create a request for the method "backups.patch".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only for the CreateBackup operation. Required for the UpdateBackup operation. A globally
* unique identifier for the backup which cannot be changed. Values are of the form
* `projects//instances//backups/a-z*[a-z0-9]` The final segment of the name must be between
* 2 and 60 characters in length. The backup is stored in the location(s) specified in the
* instance configuration of the instance containing the backup, identified by the prefix of
* the backup name of the form `projects//instances/`.
* @param content the {@link com.google.api.services.spanner.v1.model.Backup}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.spanner.v1.model.Backup content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/backups/[^/]+$");
/**
* Updates a pending or completed Backup.
*
* Create a request for the method "backups.patch".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only for the CreateBackup operation. Required for the UpdateBackup operation. A globally
* unique identifier for the backup which cannot be changed. Values are of the form
* `projects//instances//backups/a-z*[a-z0-9]` The final segment of the name must be between
* 2 and 60 characters in length. The backup is stored in the location(s) specified in the
* instance configuration of the instance containing the backup, identified by the prefix of
* the backup name of the form `projects//instances/`.
* @param content the {@link com.google.api.services.spanner.v1.model.Backup}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.spanner.v1.model.Backup content) {
super(Spanner.this, "PATCH", REST_PATH, content, com.google.api.services.spanner.v1.model.Backup.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/backups/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only for the CreateBackup operation. Required for the UpdateBackup operation. A
* globally unique identifier for the backup which cannot be changed. Values are of the
* form `projects//instances//backups/a-z*[a-z0-9]` The final segment of the name must be
* between 2 and 60 characters in length. The backup is stored in the location(s)
* specified in the instance configuration of the instance containing the backup,
* identified by the prefix of the backup name of the form `projects//instances/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only for the CreateBackup operation. Required for the UpdateBackup operation. A globally
unique identifier for the backup which cannot be changed. Values are of the form
`projects//instances//backups/a-z*[a-z0-9]` The final segment of the name must be between 2 and 60
characters in length. The backup is stored in the location(s) specified in the instance
configuration of the instance containing the backup, identified by the prefix of the backup name of
the form `projects//instances/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Output only for the CreateBackup operation. Required for the UpdateBackup operation. A
* globally unique identifier for the backup which cannot be changed. Values are of the
* form `projects//instances//backups/a-z*[a-z0-9]` The final segment of the name must be
* between 2 and 60 characters in length. The backup is stored in the location(s)
* specified in the instance configuration of the instance containing the backup,
* identified by the prefix of the backup name of the form `projects//instances/`.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/backups/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. A mask specifying which fields (e.g. `expire_time`) in the Backup resource
* should be updated. This mask is relative to the Backup resource, not to the request
* message. The field mask must always be specified; this prevents any future fields from
* being erased accidentally by clients that do not know about them.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. A mask specifying which fields (e.g. `expire_time`) in the Backup resource should be
updated. This mask is relative to the Backup resource, not to the request message. The field mask
must always be specified; this prevents any future fields from being erased accidentally by clients
that do not know about them.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. A mask specifying which fields (e.g. `expire_time`) in the Backup resource
* should be updated. This mask is relative to the Backup resource, not to the request
* message. The field mask must always be specified; this prevents any future fields from
* being erased accidentally by clients that do not know about them.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on a database or backup resource. Replaces any existing policy.
* Authorization requires `spanner.databases.setIamPolicy` permission on resource. For backups,
* authorization requires `spanner.backups.setIamPolicy` permission on resource.
*
* Create a request for the method "backups.setIamPolicy".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The Cloud Spanner resource for which the policy is being set. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* databases resources.
* @param content the {@link com.google.api.services.spanner.v1.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.spanner.v1.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends SpannerRequest {
private static final String REST_PATH = "v1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/backups/[^/]+$");
/**
* Sets the access control policy on a database or backup resource. Replaces any existing policy.
* Authorization requires `spanner.databases.setIamPolicy` permission on resource. For backups,
* authorization requires `spanner.backups.setIamPolicy` permission on resource.
*
* Create a request for the method "backups.setIamPolicy".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
* {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The Cloud Spanner resource for which the policy is being set. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* databases resources.
* @param content the {@link com.google.api.services.spanner.v1.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.spanner.v1.model.SetIamPolicyRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/backups/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The Cloud Spanner resource for which the policy is being set. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* databases resources.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The Cloud Spanner resource for which the policy is being set. The format is
`projects//instances/` for instance resources and `projects//instances//databases/` for databases
resources.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The Cloud Spanner resource for which the policy is being set. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* databases resources.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/backups/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that the caller has on the specified database or backup resource. Attempting
* this RPC on a non-existent Cloud Spanner database will result in a NOT_FOUND error if the user
* has `spanner.databases.list` permission on the containing Cloud Spanner instance. Otherwise
* returns an empty set of permissions. Calling this method on a backup that does not exist will
* result in a NOT_FOUND error if the user has `spanner.backups.list` permission on the containing
* instance.
*
* Create a request for the method "backups.testIamPermissions".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The Cloud Spanner resource for which permissions are being tested. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* database resources.
* @param content the {@link com.google.api.services.spanner.v1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.spanner.v1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends SpannerRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/backups/[^/]+$");
/**
* Returns permissions that the caller has on the specified database or backup resource.
* Attempting this RPC on a non-existent Cloud Spanner database will result in a NOT_FOUND error
* if the user has `spanner.databases.list` permission on the containing Cloud Spanner instance.
* Otherwise returns an empty set of permissions. Calling this method on a backup that does not
* exist will result in a NOT_FOUND error if the user has `spanner.backups.list` permission on the
* containing instance.
*
* Create a request for the method "backups.testIamPermissions".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The Cloud Spanner resource for which permissions are being tested. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* database resources.
* @param content the {@link com.google.api.services.spanner.v1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.spanner.v1.model.TestIamPermissionsRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/backups/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The Cloud Spanner resource for which permissions are being tested. The format
* is `projects//instances/` for instance resources and `projects//instances//databases/`
* for database resources.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The Cloud Spanner resource for which permissions are being tested. The format is
`projects//instances/` for instance resources and `projects//instances//databases/` for database
resources.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The Cloud Spanner resource for which permissions are being tested. The format
* is `projects//instances/` for instance resources and `projects//instances//databases/`
* for database resources.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/backups/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code Spanner spanner = new Spanner(...);}
* {@code Spanner.Operations.List request = spanner.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be cancelled.
* @return the request
*/
public Cancel cancel(java.lang.String name) throws java.io.IOException {
Cancel result = new Cancel(name);
initialize(result);
return result;
}
public class Cancel extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/backups/[^/]+/operations/[^/]+$");
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation. {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be cancelled.
* @since 1.13
*/
protected Cancel(java.lang.String name) {
super(Spanner.this, "POST", REST_PATH, null, com.google.api.services.spanner.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/backups/[^/]+/operations/[^/]+$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be cancelled. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be cancelled.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be cancelled. */
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/backups/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be deleted.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/backups/[^/]+/operations/[^/]+$");
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be deleted.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Spanner.this, "DELETE", REST_PATH, null, com.google.api.services.spanner.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/backups/[^/]+/operations/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be deleted. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be deleted.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be deleted. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/backups/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/backups/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/backups/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/backups/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/backups/[^/]+/operations$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.ListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/backups/[^/]+/operations$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/backups/[^/]+/operations$");
}
this.name = name;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the DatabaseOperations collection.
*
* The typical use is:
*
* {@code Spanner spanner = new Spanner(...);}
* {@code Spanner.DatabaseOperations.List request = spanner.databaseOperations().list(parameters ...)}
*
*
* @return the resource collection
*/
public DatabaseOperations databaseOperations() {
return new DatabaseOperations();
}
/**
* The "databaseOperations" collection of methods.
*/
public class DatabaseOperations {
/**
* Lists database longrunning-operations. A database operation has a name of the form
* `projects//instances//databases//operations/`. The long-running operation metadata field type
* `metadata.type_url` describes the type of the metadata. Operations returned include those that
* have completed/failed/canceled within the last 7 days, and pending operations.
*
* Create a request for the method "databaseOperations.list".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The instance of the database operations. Values are of the form `projects//instances/`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends SpannerRequest {
private static final String REST_PATH = "v1/{+parent}/databaseOperations";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+$");
/**
* Lists database longrunning-operations. A database operation has a name of the form
* `projects//instances//databases//operations/`. The long-running operation metadata field type
* `metadata.type_url` describes the type of the metadata. Operations returned include those that
* have completed/failed/canceled within the last 7 days, and pending operations.
*
* Create a request for the method "databaseOperations.list".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The instance of the database operations. Values are of the form `projects//instances/`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.ListDatabaseOperationsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The instance of the database operations. Values are of the form
* `projects//instances/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The instance of the database operations. Values are of the form `projects//instances/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The instance of the database operations. Values are of the form
* `projects//instances/`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* An expression that filters the list of returned operations. A filter expression
* consists of a field name, a comparison operator, and a value for filtering. The value
* must be a string, a number, or a boolean. The comparison operator must be one of: `<`,
* `>`, `<=`, `>=`, `!=`, `=`, or `:`. Colon `:` is the contains operator. Filter rules
* are not case sensitive. The following fields in the Operation are eligible for
* filtering: * `name` - The name of the long-running operation * `done` - False if the
* operation is in progress, else true. * `metadata.@type` - the type of metadata. For
* example, the type string for RestoreDatabaseMetadata is
* `type.googleapis.com/google.spanner.admin.database.v1.RestoreDatabaseMetadata`. *
* `metadata.` - any field in metadata.value. `metadata.@type` must be specified first, if
* filtering on metadata fields. * `error` - Error associated with the long-running
* operation. * `response.@type` - the type of response. * `response.` - any field in
* response.value. You can combine multiple expressions by enclosing each expression in
* parentheses. By default, expressions are combined with AND logic. However, you can
* specify AND, OR, and NOT logic explicitly. Here are a few examples: * `done:true` - The
* operation is complete. * `(metadata.@type=type.googleapis.com/google.spanner.admin.data
* base.v1.RestoreDatabaseMetadata) AND` \ `(metadata.source_type:BACKUP) AND` \
* `(metadata.backup_info.backup:backup_howl) AND` \ `(metadata.name:restored_howl) AND` \
* `(metadata.progress.start_time < \"2018-03-28T14:50:00Z\") AND` \ `(error:*)` - Return
* operations where: * The operation's metadata type is RestoreDatabaseMetadata. * The
* database is restored from a backup. * The backup name contains "backup_howl". * The
* restored database's name contains "restored_howl". * The operation started before
* 2018-03-28T14:50:00Z. * The operation resulted in an error.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** An expression that filters the list of returned operations. A filter expression consists of a field
name, a comparison operator, and a value for filtering. The value must be a string, a number, or a
boolean. The comparison operator must be one of: `<`, `>`, `<=`, `>=`, `!=`, `=`, or `:`. Colon `:`
is the contains operator. Filter rules are not case sensitive. The following fields in the
Operation are eligible for filtering: * `name` - The name of the long-running operation * `done` -
False if the operation is in progress, else true. * `metadata.@type` - the type of metadata. For
example, the type string for RestoreDatabaseMetadata is
`type.googleapis.com/google.spanner.admin.database.v1.RestoreDatabaseMetadata`. * `metadata.` - any
field in metadata.value. `metadata.@type` must be specified first, if filtering on metadata fields.
* `error` - Error associated with the long-running operation. * `response.@type` - the type of
response. * `response.` - any field in response.value. You can combine multiple expressions by
enclosing each expression in parentheses. By default, expressions are combined with AND logic.
However, you can specify AND, OR, and NOT logic explicitly. Here are a few examples: * `done:true`
- The operation is complete. *
`(metadata.@type=type.googleapis.com/google.spanner.admin.database.v1.RestoreDatabaseMetadata) AND`
\ `(metadata.source_type:BACKUP) AND` \ `(metadata.backup_info.backup:backup_howl) AND` \
`(metadata.name:restored_howl) AND` \ `(metadata.progress.start_time < \"2018-03-28T14:50:00Z\")
AND` \ `(error:*)` - Return operations where: * The operation's metadata type is
RestoreDatabaseMetadata. * The database is restored from a backup. * The backup name contains
"backup_howl". * The restored database's name contains "restored_howl". * The operation started
before 2018-03-28T14:50:00Z. * The operation resulted in an error.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* An expression that filters the list of returned operations. A filter expression
* consists of a field name, a comparison operator, and a value for filtering. The value
* must be a string, a number, or a boolean. The comparison operator must be one of: `<`,
* `>`, `<=`, `>=`, `!=`, `=`, or `:`. Colon `:` is the contains operator. Filter rules
* are not case sensitive. The following fields in the Operation are eligible for
* filtering: * `name` - The name of the long-running operation * `done` - False if the
* operation is in progress, else true. * `metadata.@type` - the type of metadata. For
* example, the type string for RestoreDatabaseMetadata is
* `type.googleapis.com/google.spanner.admin.database.v1.RestoreDatabaseMetadata`. *
* `metadata.` - any field in metadata.value. `metadata.@type` must be specified first, if
* filtering on metadata fields. * `error` - Error associated with the long-running
* operation. * `response.@type` - the type of response. * `response.` - any field in
* response.value. You can combine multiple expressions by enclosing each expression in
* parentheses. By default, expressions are combined with AND logic. However, you can
* specify AND, OR, and NOT logic explicitly. Here are a few examples: * `done:true` - The
* operation is complete. * `(metadata.@type=type.googleapis.com/google.spanner.admin.data
* base.v1.RestoreDatabaseMetadata) AND` \ `(metadata.source_type:BACKUP) AND` \
* `(metadata.backup_info.backup:backup_howl) AND` \ `(metadata.name:restored_howl) AND` \
* `(metadata.progress.start_time < \"2018-03-28T14:50:00Z\") AND` \ `(error:*)` - Return
* operations where: * The operation's metadata type is RestoreDatabaseMetadata. * The
* database is restored from a backup. * The backup name contains "backup_howl". * The
* restored database's name contains "restored_howl". * The operation started before
* 2018-03-28T14:50:00Z. * The operation resulted in an error.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Number of operations to be returned in the response. If 0 or less, defaults to the
* server's maximum allowed page size.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Number of operations to be returned in the response. If 0 or less, defaults to the server's maximum
allowed page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Number of operations to be returned in the response. If 0 or less, defaults to the
* server's maximum allowed page size.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* If non-empty, `page_token` should contain a next_page_token from a previous
* ListDatabaseOperationsResponse to the same `parent` and with the same `filter`.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** If non-empty, `page_token` should contain a next_page_token from a previous
ListDatabaseOperationsResponse to the same `parent` and with the same `filter`.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* If non-empty, `page_token` should contain a next_page_token from a previous
* ListDatabaseOperationsResponse to the same `parent` and with the same `filter`.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Databases collection.
*
* The typical use is:
*
* {@code Spanner spanner = new Spanner(...);}
* {@code Spanner.Databases.List request = spanner.databases().list(parameters ...)}
*
*
* @return the resource collection
*/
public Databases databases() {
return new Databases();
}
/**
* The "databases" collection of methods.
*/
public class Databases {
/**
* ChangeQuorum is strictly restricted to databases that use dual region instance configurations.
* Initiates a background operation to change quorum a database from dual-region mode to single-
* region mode and vice versa. The returned long-running operation will have a name of the format
* `projects//instances//databases//operations/` and can be used to track execution of the
* ChangeQuorum. The metadata field type is ChangeQuorumMetadata. Authorization requires
* `spanner.databases.changequorum` permission on the resource database.
*
* Create a request for the method "databases.changequorum".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Changequorum#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the database in which to apply the ChangeQuorum. Values are of the form
* `projects//instances//databases/`.
* @param content the {@link com.google.api.services.spanner.v1.model.ChangeQuorumRequest}
* @return the request
*/
public Changequorum changequorum(java.lang.String name, com.google.api.services.spanner.v1.model.ChangeQuorumRequest content) throws java.io.IOException {
Changequorum result = new Changequorum(name, content);
initialize(result);
return result;
}
public class Changequorum extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}:changequorum";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
/**
* ChangeQuorum is strictly restricted to databases that use dual region instance configurations.
* Initiates a background operation to change quorum a database from dual-region mode to single-
* region mode and vice versa. The returned long-running operation will have a name of the format
* `projects//instances//databases//operations/` and can be used to track execution of the
* ChangeQuorum. The metadata field type is ChangeQuorumMetadata. Authorization requires
* `spanner.databases.changequorum` permission on the resource database.
*
* Create a request for the method "databases.changequorum".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Changequorum#execute()} method to invoke the remote operation.
* {@link
* Changequorum#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the database in which to apply the ChangeQuorum. Values are of the form
* `projects//instances//databases/`.
* @param content the {@link com.google.api.services.spanner.v1.model.ChangeQuorumRequest}
* @since 1.13
*/
protected Changequorum(java.lang.String name, com.google.api.services.spanner.v1.model.ChangeQuorumRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
}
@Override
public Changequorum set$Xgafv(java.lang.String $Xgafv) {
return (Changequorum) super.set$Xgafv($Xgafv);
}
@Override
public Changequorum setAccessToken(java.lang.String accessToken) {
return (Changequorum) super.setAccessToken(accessToken);
}
@Override
public Changequorum setAlt(java.lang.String alt) {
return (Changequorum) super.setAlt(alt);
}
@Override
public Changequorum setCallback(java.lang.String callback) {
return (Changequorum) super.setCallback(callback);
}
@Override
public Changequorum setFields(java.lang.String fields) {
return (Changequorum) super.setFields(fields);
}
@Override
public Changequorum setKey(java.lang.String key) {
return (Changequorum) super.setKey(key);
}
@Override
public Changequorum setOauthToken(java.lang.String oauthToken) {
return (Changequorum) super.setOauthToken(oauthToken);
}
@Override
public Changequorum setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Changequorum) super.setPrettyPrint(prettyPrint);
}
@Override
public Changequorum setQuotaUser(java.lang.String quotaUser) {
return (Changequorum) super.setQuotaUser(quotaUser);
}
@Override
public Changequorum setUploadType(java.lang.String uploadType) {
return (Changequorum) super.setUploadType(uploadType);
}
@Override
public Changequorum setUploadProtocol(java.lang.String uploadProtocol) {
return (Changequorum) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the database in which to apply the ChangeQuorum. Values are of the
* form `projects//instances//databases/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the database in which to apply the ChangeQuorum. Values are of the form
`projects//instances//databases/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the database in which to apply the ChangeQuorum. Values are of the
* form `projects//instances//databases/`.
*/
public Changequorum setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Changequorum set(String parameterName, Object value) {
return (Changequorum) super.set(parameterName, value);
}
}
/**
* Creates a new Spanner database and starts to prepare it for serving. The returned long-running
* operation will have a name of the format `/operations/` and can be used to track preparation of
* the database. The metadata field type is CreateDatabaseMetadata. The response field type is
* Database, if successful.
*
* Create a request for the method "databases.create".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the instance that will serve the new database. Values are of the form
* `projects//instances/`.
* @param content the {@link com.google.api.services.spanner.v1.model.CreateDatabaseRequest}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.spanner.v1.model.CreateDatabaseRequest content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends SpannerRequest {
private static final String REST_PATH = "v1/{+parent}/databases";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+$");
/**
* Creates a new Spanner database and starts to prepare it for serving. The returned long-running
* operation will have a name of the format `/operations/` and can be used to track preparation of
* the database. The metadata field type is CreateDatabaseMetadata. The response field type is
* Database, if successful.
*
* Create a request for the method "databases.create".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the instance that will serve the new database. Values are of the form
* `projects//instances/`.
* @param content the {@link com.google.api.services.spanner.v1.model.CreateDatabaseRequest}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.spanner.v1.model.CreateDatabaseRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the instance that will serve the new database. Values are of the
* form `projects//instances/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the instance that will serve the new database. Values are of the form
`projects//instances/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the instance that will serve the new database. Values are of the
* form `projects//instances/`.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Drops (aka deletes) a Cloud Spanner database. Completed backups for the database will be retained
* according to their `expire_time`. Note: Cloud Spanner might continue to accept requests for a few
* seconds after the database has been deleted.
*
* Create a request for the method "databases.dropDatabase".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link DropDatabase#execute()} method to invoke the remote operation.
*
* @param database Required. The database to be dropped.
* @return the request
*/
public DropDatabase dropDatabase(java.lang.String database) throws java.io.IOException {
DropDatabase result = new DropDatabase(database);
initialize(result);
return result;
}
public class DropDatabase extends SpannerRequest {
private static final String REST_PATH = "v1/{+database}";
private final java.util.regex.Pattern DATABASE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
/**
* Drops (aka deletes) a Cloud Spanner database. Completed backups for the database will be
* retained according to their `expire_time`. Note: Cloud Spanner might continue to accept
* requests for a few seconds after the database has been deleted.
*
* Create a request for the method "databases.dropDatabase".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link DropDatabase#execute()} method to invoke the remote operation.
* {@link
* DropDatabase#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param database Required. The database to be dropped.
* @since 1.13
*/
protected DropDatabase(java.lang.String database) {
super(Spanner.this, "DELETE", REST_PATH, null, com.google.api.services.spanner.v1.model.Empty.class);
this.database = com.google.api.client.util.Preconditions.checkNotNull(database, "Required parameter database must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DATABASE_PATTERN.matcher(database).matches(),
"Parameter database must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
}
@Override
public DropDatabase set$Xgafv(java.lang.String $Xgafv) {
return (DropDatabase) super.set$Xgafv($Xgafv);
}
@Override
public DropDatabase setAccessToken(java.lang.String accessToken) {
return (DropDatabase) super.setAccessToken(accessToken);
}
@Override
public DropDatabase setAlt(java.lang.String alt) {
return (DropDatabase) super.setAlt(alt);
}
@Override
public DropDatabase setCallback(java.lang.String callback) {
return (DropDatabase) super.setCallback(callback);
}
@Override
public DropDatabase setFields(java.lang.String fields) {
return (DropDatabase) super.setFields(fields);
}
@Override
public DropDatabase setKey(java.lang.String key) {
return (DropDatabase) super.setKey(key);
}
@Override
public DropDatabase setOauthToken(java.lang.String oauthToken) {
return (DropDatabase) super.setOauthToken(oauthToken);
}
@Override
public DropDatabase setPrettyPrint(java.lang.Boolean prettyPrint) {
return (DropDatabase) super.setPrettyPrint(prettyPrint);
}
@Override
public DropDatabase setQuotaUser(java.lang.String quotaUser) {
return (DropDatabase) super.setQuotaUser(quotaUser);
}
@Override
public DropDatabase setUploadType(java.lang.String uploadType) {
return (DropDatabase) super.setUploadType(uploadType);
}
@Override
public DropDatabase setUploadProtocol(java.lang.String uploadProtocol) {
return (DropDatabase) super.setUploadProtocol(uploadProtocol);
}
/** Required. The database to be dropped. */
@com.google.api.client.util.Key
private java.lang.String database;
/** Required. The database to be dropped.
*/
public java.lang.String getDatabase() {
return database;
}
/** Required. The database to be dropped. */
public DropDatabase setDatabase(java.lang.String database) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DATABASE_PATTERN.matcher(database).matches(),
"Parameter database must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
this.database = database;
return this;
}
@Override
public DropDatabase set(String parameterName, Object value) {
return (DropDatabase) super.set(parameterName, value);
}
}
/**
* Gets the state of a Cloud Spanner database.
*
* Create a request for the method "databases.get".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the requested database. Values are of the form
* `projects//instances//databases/`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
/**
* Gets the state of a Cloud Spanner database.
*
* Create a request for the method "databases.get".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the requested database. Values are of the form
* `projects//instances//databases/`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.Database.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the requested database. Values are of the form
* `projects//instances//databases/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the requested database. Values are of the form
`projects//instances//databases/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the requested database. Values are of the form
* `projects//instances//databases/`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Returns the schema of a Cloud Spanner database as a list of formatted DDL statements. This method
* does not show pending schema updates, those may be queried using the Operations API.
*
* Create a request for the method "databases.getDdl".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link GetDdl#execute()} method to invoke the remote operation.
*
* @param database Required. The database whose schema we wish to get. Values are of the form
* `projects//instances//databases/`
* @return the request
*/
public GetDdl getDdl(java.lang.String database) throws java.io.IOException {
GetDdl result = new GetDdl(database);
initialize(result);
return result;
}
public class GetDdl extends SpannerRequest {
private static final String REST_PATH = "v1/{+database}/ddl";
private final java.util.regex.Pattern DATABASE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
/**
* Returns the schema of a Cloud Spanner database as a list of formatted DDL statements. This
* method does not show pending schema updates, those may be queried using the Operations API.
*
* Create a request for the method "databases.getDdl".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link GetDdl#execute()} method to invoke the remote operation. {@link
* GetDdl#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param database Required. The database whose schema we wish to get. Values are of the form
* `projects//instances//databases/`
* @since 1.13
*/
protected GetDdl(java.lang.String database) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.GetDatabaseDdlResponse.class);
this.database = com.google.api.client.util.Preconditions.checkNotNull(database, "Required parameter database must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DATABASE_PATTERN.matcher(database).matches(),
"Parameter database must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetDdl set$Xgafv(java.lang.String $Xgafv) {
return (GetDdl) super.set$Xgafv($Xgafv);
}
@Override
public GetDdl setAccessToken(java.lang.String accessToken) {
return (GetDdl) super.setAccessToken(accessToken);
}
@Override
public GetDdl setAlt(java.lang.String alt) {
return (GetDdl) super.setAlt(alt);
}
@Override
public GetDdl setCallback(java.lang.String callback) {
return (GetDdl) super.setCallback(callback);
}
@Override
public GetDdl setFields(java.lang.String fields) {
return (GetDdl) super.setFields(fields);
}
@Override
public GetDdl setKey(java.lang.String key) {
return (GetDdl) super.setKey(key);
}
@Override
public GetDdl setOauthToken(java.lang.String oauthToken) {
return (GetDdl) super.setOauthToken(oauthToken);
}
@Override
public GetDdl setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetDdl) super.setPrettyPrint(prettyPrint);
}
@Override
public GetDdl setQuotaUser(java.lang.String quotaUser) {
return (GetDdl) super.setQuotaUser(quotaUser);
}
@Override
public GetDdl setUploadType(java.lang.String uploadType) {
return (GetDdl) super.setUploadType(uploadType);
}
@Override
public GetDdl setUploadProtocol(java.lang.String uploadProtocol) {
return (GetDdl) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The database whose schema we wish to get. Values are of the form
* `projects//instances//databases/`
*/
@com.google.api.client.util.Key
private java.lang.String database;
/** Required. The database whose schema we wish to get. Values are of the form
`projects//instances//databases/`
*/
public java.lang.String getDatabase() {
return database;
}
/**
* Required. The database whose schema we wish to get. Values are of the form
* `projects//instances//databases/`
*/
public GetDdl setDatabase(java.lang.String database) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DATABASE_PATTERN.matcher(database).matches(),
"Parameter database must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
this.database = database;
return this;
}
@Override
public GetDdl set(String parameterName, Object value) {
return (GetDdl) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a database or backup resource. Returns an empty policy if a
* database or backup exists but does not have a policy set. Authorization requires
* `spanner.databases.getIamPolicy` permission on resource. For backups, authorization requires
* `spanner.backups.getIamPolicy` permission on resource.
*
* Create a request for the method "databases.getIamPolicy".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The Cloud Spanner resource for which the policy is being retrieved. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* database resources.
* @param content the {@link com.google.api.services.spanner.v1.model.GetIamPolicyRequest}
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource, com.google.api.services.spanner.v1.model.GetIamPolicyRequest content) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource, content);
initialize(result);
return result;
}
public class GetIamPolicy extends SpannerRequest {
private static final String REST_PATH = "v1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
/**
* Gets the access control policy for a database or backup resource. Returns an empty policy if a
* database or backup exists but does not have a policy set. Authorization requires
* `spanner.databases.getIamPolicy` permission on resource. For backups, authorization requires
* `spanner.backups.getIamPolicy` permission on resource.
*
* Create a request for the method "databases.getIamPolicy".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
* {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The Cloud Spanner resource for which the policy is being retrieved. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* database resources.
* @param content the {@link com.google.api.services.spanner.v1.model.GetIamPolicyRequest}
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource, com.google.api.services.spanner.v1.model.GetIamPolicyRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The Cloud Spanner resource for which the policy is being retrieved. The
* format is `projects//instances/` for instance resources and
* `projects//instances//databases/` for database resources.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The Cloud Spanner resource for which the policy is being retrieved. The format is
`projects//instances/` for instance resources and `projects//instances//databases/` for database
resources.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The Cloud Spanner resource for which the policy is being retrieved. The
* format is `projects//instances/` for instance resources and
* `projects//instances//databases/` for database resources.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Request a specific scan with Database-specific data for Cloud Key Visualizer.
*
* Create a request for the method "databases.getScans".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link GetScans#execute()} method to invoke the remote operation.
*
* @param name Required. The unique name of the scan containing the requested information, specific to the Database
* service implementing this interface.
* @return the request
*/
public GetScans getScans(java.lang.String name) throws java.io.IOException {
GetScans result = new GetScans(name);
initialize(result);
return result;
}
public class GetScans extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}/scans";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
/**
* Request a specific scan with Database-specific data for Cloud Key Visualizer.
*
* Create a request for the method "databases.getScans".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link GetScans#execute()} method to invoke the remote operation.
* {@link
* GetScans#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The unique name of the scan containing the requested information, specific to the Database
* service implementing this interface.
* @since 1.13
*/
protected GetScans(java.lang.String name) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.Scan.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetScans set$Xgafv(java.lang.String $Xgafv) {
return (GetScans) super.set$Xgafv($Xgafv);
}
@Override
public GetScans setAccessToken(java.lang.String accessToken) {
return (GetScans) super.setAccessToken(accessToken);
}
@Override
public GetScans setAlt(java.lang.String alt) {
return (GetScans) super.setAlt(alt);
}
@Override
public GetScans setCallback(java.lang.String callback) {
return (GetScans) super.setCallback(callback);
}
@Override
public GetScans setFields(java.lang.String fields) {
return (GetScans) super.setFields(fields);
}
@Override
public GetScans setKey(java.lang.String key) {
return (GetScans) super.setKey(key);
}
@Override
public GetScans setOauthToken(java.lang.String oauthToken) {
return (GetScans) super.setOauthToken(oauthToken);
}
@Override
public GetScans setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetScans) super.setPrettyPrint(prettyPrint);
}
@Override
public GetScans setQuotaUser(java.lang.String quotaUser) {
return (GetScans) super.setQuotaUser(quotaUser);
}
@Override
public GetScans setUploadType(java.lang.String uploadType) {
return (GetScans) super.setUploadType(uploadType);
}
@Override
public GetScans setUploadProtocol(java.lang.String uploadProtocol) {
return (GetScans) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The unique name of the scan containing the requested information, specific to
* the Database service implementing this interface.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The unique name of the scan containing the requested information, specific to the
Database service implementing this interface.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The unique name of the scan containing the requested information, specific to
* the Database service implementing this interface.
*/
public GetScans setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
this.name = name;
return this;
}
/** The upper bound for the time range to retrieve Scan data for. */
@com.google.api.client.util.Key
private String endTime;
/** The upper bound for the time range to retrieve Scan data for.
*/
public String getEndTime() {
return endTime;
}
/** The upper bound for the time range to retrieve Scan data for. */
public GetScans setEndTime(String endTime) {
this.endTime = endTime;
return this;
}
/**
* These fields restrict the Database-specific information returned in the `Scan.data`
* field. If a `View` is provided that does not include the `Scan.data` field, these are
* ignored. This range of time must be entirely contained within the defined time range of
* the targeted scan. The lower bound for the time range to retrieve Scan data for.
*/
@com.google.api.client.util.Key
private String startTime;
/** These fields restrict the Database-specific information returned in the `Scan.data` field. If a
`View` is provided that does not include the `Scan.data` field, these are ignored. This range of
time must be entirely contained within the defined time range of the targeted scan. The lower bound
for the time range to retrieve Scan data for.
*/
public String getStartTime() {
return startTime;
}
/**
* These fields restrict the Database-specific information returned in the `Scan.data`
* field. If a `View` is provided that does not include the `Scan.data` field, these are
* ignored. This range of time must be entirely contained within the defined time range of
* the targeted scan. The lower bound for the time range to retrieve Scan data for.
*/
public GetScans setStartTime(String startTime) {
this.startTime = startTime;
return this;
}
/**
* Specifies which parts of the Scan should be returned in the response. Note, if left
* unspecified, the FULL view is assumed.
*/
@com.google.api.client.util.Key
private java.lang.String view;
/** Specifies which parts of the Scan should be returned in the response. Note, if left unspecified,
the FULL view is assumed.
*/
public java.lang.String getView() {
return view;
}
/**
* Specifies which parts of the Scan should be returned in the response. Note, if left
* unspecified, the FULL view is assumed.
*/
public GetScans setView(java.lang.String view) {
this.view = view;
return this;
}
@Override
public GetScans set(String parameterName, Object value) {
return (GetScans) super.set(parameterName, value);
}
}
/**
* Lists Cloud Spanner databases.
*
* Create a request for the method "databases.list".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The instance whose databases should be listed. Values are of the form
* `projects//instances/`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends SpannerRequest {
private static final String REST_PATH = "v1/{+parent}/databases";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+$");
/**
* Lists Cloud Spanner databases.
*
* Create a request for the method "databases.list".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The instance whose databases should be listed. Values are of the form
* `projects//instances/`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.ListDatabasesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The instance whose databases should be listed. Values are of the form
* `projects//instances/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The instance whose databases should be listed. Values are of the form
`projects//instances/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The instance whose databases should be listed. Values are of the form
* `projects//instances/`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Number of databases to be returned in the response. If 0 or less, defaults to the
* server's maximum allowed page size.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Number of databases to be returned in the response. If 0 or less, defaults to the server's maximum
allowed page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Number of databases to be returned in the response. If 0 or less, defaults to the
* server's maximum allowed page size.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* If non-empty, `page_token` should contain a next_page_token from a previous
* ListDatabasesResponse.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** If non-empty, `page_token` should contain a next_page_token from a previous ListDatabasesResponse.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* If non-empty, `page_token` should contain a next_page_token from a previous
* ListDatabasesResponse.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a Cloud Spanner database. The returned long-running operation can be used to track the
* progress of updating the database. If the named database does not exist, returns `NOT_FOUND`.
* While the operation is pending: * The database's reconciling field is set to true. * Cancelling
* the operation is best-effort. If the cancellation succeeds, the operation metadata's cancel_time
* is set, the updates are reverted, and the operation terminates with a `CANCELLED` status. * New
* UpdateDatabase requests will return a `FAILED_PRECONDITION` error until the pending operation is
* done (returns successfully or with error). * Reading the database via the API continues to give
* the pre-request values. Upon completion of the returned operation: * The new values are in effect
* and readable via the API. * The database's reconciling field becomes false. The returned long-
* running operation will have a name of the format `projects//instances//databases//operations/`
* and can be used to track the database modification. The metadata field type is
* UpdateDatabaseMetadata. The response field type is Database, if successful.
*
* Create a request for the method "databases.patch".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the database. Values are of the form `projects//instances//databases/`, where
* `` is as specified in the `CREATE DATABASE` statement. This name can be passed to other
* API methods to identify the database.
* @param content the {@link com.google.api.services.spanner.v1.model.Database}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.spanner.v1.model.Database content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
/**
* Updates a Cloud Spanner database. The returned long-running operation can be used to track the
* progress of updating the database. If the named database does not exist, returns `NOT_FOUND`.
* While the operation is pending: * The database's reconciling field is set to true. * Cancelling
* the operation is best-effort. If the cancellation succeeds, the operation metadata's
* cancel_time is set, the updates are reverted, and the operation terminates with a `CANCELLED`
* status. * New UpdateDatabase requests will return a `FAILED_PRECONDITION` error until the
* pending operation is done (returns successfully or with error). * Reading the database via the
* API continues to give the pre-request values. Upon completion of the returned operation: * The
* new values are in effect and readable via the API. * The database's reconciling field becomes
* false. The returned long-running operation will have a name of the format
* `projects//instances//databases//operations/` and can be used to track the database
* modification. The metadata field type is UpdateDatabaseMetadata. The response field type is
* Database, if successful.
*
* Create a request for the method "databases.patch".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the database. Values are of the form `projects//instances//databases/`, where
* `` is as specified in the `CREATE DATABASE` statement. This name can be passed to other
* API methods to identify the database.
* @param content the {@link com.google.api.services.spanner.v1.model.Database}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.spanner.v1.model.Database content) {
super(Spanner.this, "PATCH", REST_PATH, content, com.google.api.services.spanner.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the database. Values are of the form
* `projects//instances//databases/`, where `` is as specified in the `CREATE DATABASE`
* statement. This name can be passed to other API methods to identify the database.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the database. Values are of the form `projects//instances//databases/`, where
`` is as specified in the `CREATE DATABASE` statement. This name can be passed to other API methods
to identify the database.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the database. Values are of the form
* `projects//instances//databases/`, where `` is as specified in the `CREATE DATABASE`
* statement. This name can be passed to other API methods to identify the database.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. The list of fields to update. Currently, only `enable_drop_protection` field
* can be updated.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. The list of fields to update. Currently, only `enable_drop_protection` field can be
updated.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. The list of fields to update. Currently, only `enable_drop_protection` field
* can be updated.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Create a new database by restoring from a completed backup. The new database must be in the same
* project and in an instance with the same instance configuration as the instance containing the
* backup. The returned database long-running operation has a name of the format
* `projects//instances//databases//operations/`, and can be used to track the progress of the
* operation, and to cancel it. The metadata field type is RestoreDatabaseMetadata. The response
* type is Database, if successful. Cancelling the returned operation will stop the restore and
* delete the database. There can be only one database being restored into an instance at a time.
* Once the restore operation completes, a new restore operation can be initiated, without waiting
* for the optimize operation associated with the first restore to complete.
*
* Create a request for the method "databases.restore".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Restore#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the instance in which to create the restored database. This instance must be
* in the same project and have the same instance configuration as the instance containing
* the source backup. Values are of the form `projects//instances/`.
* @param content the {@link com.google.api.services.spanner.v1.model.RestoreDatabaseRequest}
* @return the request
*/
public Restore restore(java.lang.String parent, com.google.api.services.spanner.v1.model.RestoreDatabaseRequest content) throws java.io.IOException {
Restore result = new Restore(parent, content);
initialize(result);
return result;
}
public class Restore extends SpannerRequest {
private static final String REST_PATH = "v1/{+parent}/databases:restore";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+$");
/**
* Create a new database by restoring from a completed backup. The new database must be in the
* same project and in an instance with the same instance configuration as the instance containing
* the backup. The returned database long-running operation has a name of the format
* `projects//instances//databases//operations/`, and can be used to track the progress of the
* operation, and to cancel it. The metadata field type is RestoreDatabaseMetadata. The response
* type is Database, if successful. Cancelling the returned operation will stop the restore and
* delete the database. There can be only one database being restored into an instance at a time.
* Once the restore operation completes, a new restore operation can be initiated, without waiting
* for the optimize operation associated with the first restore to complete.
*
* Create a request for the method "databases.restore".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Restore#execute()} method to invoke the remote operation.
* {@link
* Restore#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the instance in which to create the restored database. This instance must be
* in the same project and have the same instance configuration as the instance containing
* the source backup. Values are of the form `projects//instances/`.
* @param content the {@link com.google.api.services.spanner.v1.model.RestoreDatabaseRequest}
* @since 1.13
*/
protected Restore(java.lang.String parent, com.google.api.services.spanner.v1.model.RestoreDatabaseRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
}
@Override
public Restore set$Xgafv(java.lang.String $Xgafv) {
return (Restore) super.set$Xgafv($Xgafv);
}
@Override
public Restore setAccessToken(java.lang.String accessToken) {
return (Restore) super.setAccessToken(accessToken);
}
@Override
public Restore setAlt(java.lang.String alt) {
return (Restore) super.setAlt(alt);
}
@Override
public Restore setCallback(java.lang.String callback) {
return (Restore) super.setCallback(callback);
}
@Override
public Restore setFields(java.lang.String fields) {
return (Restore) super.setFields(fields);
}
@Override
public Restore setKey(java.lang.String key) {
return (Restore) super.setKey(key);
}
@Override
public Restore setOauthToken(java.lang.String oauthToken) {
return (Restore) super.setOauthToken(oauthToken);
}
@Override
public Restore setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Restore) super.setPrettyPrint(prettyPrint);
}
@Override
public Restore setQuotaUser(java.lang.String quotaUser) {
return (Restore) super.setQuotaUser(quotaUser);
}
@Override
public Restore setUploadType(java.lang.String uploadType) {
return (Restore) super.setUploadType(uploadType);
}
@Override
public Restore setUploadProtocol(java.lang.String uploadProtocol) {
return (Restore) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the instance in which to create the restored database. This
* instance must be in the same project and have the same instance configuration as the
* instance containing the source backup. Values are of the form `projects//instances/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the instance in which to create the restored database. This instance must be
in the same project and have the same instance configuration as the instance containing the source
backup. Values are of the form `projects//instances/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the instance in which to create the restored database. This
* instance must be in the same project and have the same instance configuration as the
* instance containing the source backup. Values are of the form `projects//instances/`.
*/
public Restore setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Restore set(String parameterName, Object value) {
return (Restore) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on a database or backup resource. Replaces any existing policy.
* Authorization requires `spanner.databases.setIamPolicy` permission on resource. For backups,
* authorization requires `spanner.backups.setIamPolicy` permission on resource.
*
* Create a request for the method "databases.setIamPolicy".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The Cloud Spanner resource for which the policy is being set. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* databases resources.
* @param content the {@link com.google.api.services.spanner.v1.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.spanner.v1.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends SpannerRequest {
private static final String REST_PATH = "v1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
/**
* Sets the access control policy on a database or backup resource. Replaces any existing policy.
* Authorization requires `spanner.databases.setIamPolicy` permission on resource. For backups,
* authorization requires `spanner.backups.setIamPolicy` permission on resource.
*
* Create a request for the method "databases.setIamPolicy".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
* {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The Cloud Spanner resource for which the policy is being set. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* databases resources.
* @param content the {@link com.google.api.services.spanner.v1.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.spanner.v1.model.SetIamPolicyRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The Cloud Spanner resource for which the policy is being set. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* databases resources.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The Cloud Spanner resource for which the policy is being set. The format is
`projects//instances/` for instance resources and `projects//instances//databases/` for databases
resources.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The Cloud Spanner resource for which the policy is being set. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* databases resources.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that the caller has on the specified database or backup resource. Attempting
* this RPC on a non-existent Cloud Spanner database will result in a NOT_FOUND error if the user
* has `spanner.databases.list` permission on the containing Cloud Spanner instance. Otherwise
* returns an empty set of permissions. Calling this method on a backup that does not exist will
* result in a NOT_FOUND error if the user has `spanner.backups.list` permission on the containing
* instance.
*
* Create a request for the method "databases.testIamPermissions".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The Cloud Spanner resource for which permissions are being tested. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* database resources.
* @param content the {@link com.google.api.services.spanner.v1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.spanner.v1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends SpannerRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
/**
* Returns permissions that the caller has on the specified database or backup resource.
* Attempting this RPC on a non-existent Cloud Spanner database will result in a NOT_FOUND error
* if the user has `spanner.databases.list` permission on the containing Cloud Spanner instance.
* Otherwise returns an empty set of permissions. Calling this method on a backup that does not
* exist will result in a NOT_FOUND error if the user has `spanner.backups.list` permission on the
* containing instance.
*
* Create a request for the method "databases.testIamPermissions".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The Cloud Spanner resource for which permissions are being tested. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* database resources.
* @param content the {@link com.google.api.services.spanner.v1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.spanner.v1.model.TestIamPermissionsRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The Cloud Spanner resource for which permissions are being tested. The format
* is `projects//instances/` for instance resources and `projects//instances//databases/`
* for database resources.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The Cloud Spanner resource for which permissions are being tested. The format is
`projects//instances/` for instance resources and `projects//instances//databases/` for database
resources.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The Cloud Spanner resource for which permissions are being tested. The format
* is `projects//instances/` for instance resources and `projects//instances//databases/`
* for database resources.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* Updates the schema of a Cloud Spanner database by creating/altering/dropping tables, columns,
* indexes, etc. The returned long-running operation will have a name of the format `/operations/`
* and can be used to track execution of the schema change(s). The metadata field type is
* UpdateDatabaseDdlMetadata. The operation has no response.
*
* Create a request for the method "databases.updateDdl".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link UpdateDdl#execute()} method to invoke the remote operation.
*
* @param database Required. The database to update.
* @param content the {@link com.google.api.services.spanner.v1.model.UpdateDatabaseDdlRequest}
* @return the request
*/
public UpdateDdl updateDdl(java.lang.String database, com.google.api.services.spanner.v1.model.UpdateDatabaseDdlRequest content) throws java.io.IOException {
UpdateDdl result = new UpdateDdl(database, content);
initialize(result);
return result;
}
public class UpdateDdl extends SpannerRequest {
private static final String REST_PATH = "v1/{+database}/ddl";
private final java.util.regex.Pattern DATABASE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
/**
* Updates the schema of a Cloud Spanner database by creating/altering/dropping tables, columns,
* indexes, etc. The returned long-running operation will have a name of the format `/operations/`
* and can be used to track execution of the schema change(s). The metadata field type is
* UpdateDatabaseDdlMetadata. The operation has no response.
*
* Create a request for the method "databases.updateDdl".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link UpdateDdl#execute()} method to invoke the remote operation.
* {@link
* UpdateDdl#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param database Required. The database to update.
* @param content the {@link com.google.api.services.spanner.v1.model.UpdateDatabaseDdlRequest}
* @since 1.13
*/
protected UpdateDdl(java.lang.String database, com.google.api.services.spanner.v1.model.UpdateDatabaseDdlRequest content) {
super(Spanner.this, "PATCH", REST_PATH, content, com.google.api.services.spanner.v1.model.Operation.class);
this.database = com.google.api.client.util.Preconditions.checkNotNull(database, "Required parameter database must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DATABASE_PATTERN.matcher(database).matches(),
"Parameter database must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
}
@Override
public UpdateDdl set$Xgafv(java.lang.String $Xgafv) {
return (UpdateDdl) super.set$Xgafv($Xgafv);
}
@Override
public UpdateDdl setAccessToken(java.lang.String accessToken) {
return (UpdateDdl) super.setAccessToken(accessToken);
}
@Override
public UpdateDdl setAlt(java.lang.String alt) {
return (UpdateDdl) super.setAlt(alt);
}
@Override
public UpdateDdl setCallback(java.lang.String callback) {
return (UpdateDdl) super.setCallback(callback);
}
@Override
public UpdateDdl setFields(java.lang.String fields) {
return (UpdateDdl) super.setFields(fields);
}
@Override
public UpdateDdl setKey(java.lang.String key) {
return (UpdateDdl) super.setKey(key);
}
@Override
public UpdateDdl setOauthToken(java.lang.String oauthToken) {
return (UpdateDdl) super.setOauthToken(oauthToken);
}
@Override
public UpdateDdl setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateDdl) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateDdl setQuotaUser(java.lang.String quotaUser) {
return (UpdateDdl) super.setQuotaUser(quotaUser);
}
@Override
public UpdateDdl setUploadType(java.lang.String uploadType) {
return (UpdateDdl) super.setUploadType(uploadType);
}
@Override
public UpdateDdl setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateDdl) super.setUploadProtocol(uploadProtocol);
}
/** Required. The database to update. */
@com.google.api.client.util.Key
private java.lang.String database;
/** Required. The database to update.
*/
public java.lang.String getDatabase() {
return database;
}
/** Required. The database to update. */
public UpdateDdl setDatabase(java.lang.String database) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DATABASE_PATTERN.matcher(database).matches(),
"Parameter database must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
this.database = database;
return this;
}
@Override
public UpdateDdl set(String parameterName, Object value) {
return (UpdateDdl) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the BackupSchedules collection.
*
* The typical use is:
*
* {@code Spanner spanner = new Spanner(...);}
* {@code Spanner.BackupSchedules.List request = spanner.backupSchedules().list(parameters ...)}
*
*
* @return the resource collection
*/
public BackupSchedules backupSchedules() {
return new BackupSchedules();
}
/**
* The "backupSchedules" collection of methods.
*/
public class BackupSchedules {
/**
* Gets the access control policy for a database or backup resource. Returns an empty policy if a
* database or backup exists but does not have a policy set. Authorization requires
* `spanner.databases.getIamPolicy` permission on resource. For backups, authorization requires
* `spanner.backups.getIamPolicy` permission on resource.
*
* Create a request for the method "backupSchedules.getIamPolicy".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The Cloud Spanner resource for which the policy is being retrieved. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* database resources.
* @param content the {@link com.google.api.services.spanner.v1.model.GetIamPolicyRequest}
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource, com.google.api.services.spanner.v1.model.GetIamPolicyRequest content) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource, content);
initialize(result);
return result;
}
public class GetIamPolicy extends SpannerRequest {
private static final String REST_PATH = "v1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+/backupSchedules/[^/]+$");
/**
* Gets the access control policy for a database or backup resource. Returns an empty policy if a
* database or backup exists but does not have a policy set. Authorization requires
* `spanner.databases.getIamPolicy` permission on resource. For backups, authorization requires
* `spanner.backups.getIamPolicy` permission on resource.
*
* Create a request for the method "backupSchedules.getIamPolicy".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
* {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The Cloud Spanner resource for which the policy is being retrieved. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* database resources.
* @param content the {@link com.google.api.services.spanner.v1.model.GetIamPolicyRequest}
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource, com.google.api.services.spanner.v1.model.GetIamPolicyRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/backupSchedules/[^/]+$");
}
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The Cloud Spanner resource for which the policy is being retrieved. The
* format is `projects//instances/` for instance resources and
* `projects//instances//databases/` for database resources.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The Cloud Spanner resource for which the policy is being retrieved. The format is
`projects//instances/` for instance resources and `projects//instances//databases/` for database
resources.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The Cloud Spanner resource for which the policy is being retrieved. The
* format is `projects//instances/` for instance resources and
* `projects//instances//databases/` for database resources.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/backupSchedules/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on a database or backup resource. Replaces any existing policy.
* Authorization requires `spanner.databases.setIamPolicy` permission on resource. For backups,
* authorization requires `spanner.backups.setIamPolicy` permission on resource.
*
* Create a request for the method "backupSchedules.setIamPolicy".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The Cloud Spanner resource for which the policy is being set. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* databases resources.
* @param content the {@link com.google.api.services.spanner.v1.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.spanner.v1.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends SpannerRequest {
private static final String REST_PATH = "v1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+/backupSchedules/[^/]+$");
/**
* Sets the access control policy on a database or backup resource. Replaces any existing policy.
* Authorization requires `spanner.databases.setIamPolicy` permission on resource. For backups,
* authorization requires `spanner.backups.setIamPolicy` permission on resource.
*
* Create a request for the method "backupSchedules.setIamPolicy".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
* {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The Cloud Spanner resource for which the policy is being set. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* databases resources.
* @param content the {@link com.google.api.services.spanner.v1.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.spanner.v1.model.SetIamPolicyRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/backupSchedules/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The Cloud Spanner resource for which the policy is being set. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/`
* for databases resources.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The Cloud Spanner resource for which the policy is being set. The format is
`projects//instances/` for instance resources and `projects//instances//databases/` for databases
resources.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The Cloud Spanner resource for which the policy is being set. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/`
* for databases resources.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/backupSchedules/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that the caller has on the specified database or backup resource. Attempting
* this RPC on a non-existent Cloud Spanner database will result in a NOT_FOUND error if the user
* has `spanner.databases.list` permission on the containing Cloud Spanner instance. Otherwise
* returns an empty set of permissions. Calling this method on a backup that does not exist will
* result in a NOT_FOUND error if the user has `spanner.backups.list` permission on the containing
* instance.
*
* Create a request for the method "backupSchedules.testIamPermissions".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The Cloud Spanner resource for which permissions are being tested. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* database resources.
* @param content the {@link com.google.api.services.spanner.v1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.spanner.v1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends SpannerRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+/backupSchedules/[^/]+$");
/**
* Returns permissions that the caller has on the specified database or backup resource.
* Attempting this RPC on a non-existent Cloud Spanner database will result in a NOT_FOUND error
* if the user has `spanner.databases.list` permission on the containing Cloud Spanner instance.
* Otherwise returns an empty set of permissions. Calling this method on a backup that does not
* exist will result in a NOT_FOUND error if the user has `spanner.backups.list` permission on the
* containing instance.
*
* Create a request for the method "backupSchedules.testIamPermissions".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The Cloud Spanner resource for which permissions are being tested. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* database resources.
* @param content the {@link com.google.api.services.spanner.v1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.spanner.v1.model.TestIamPermissionsRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/backupSchedules/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The Cloud Spanner resource for which permissions are being tested. The
* format is `projects//instances/` for instance resources and
* `projects//instances//databases/` for database resources.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The Cloud Spanner resource for which permissions are being tested. The format is
`projects//instances/` for instance resources and `projects//instances//databases/` for database
resources.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The Cloud Spanner resource for which permissions are being tested. The
* format is `projects//instances/` for instance resources and
* `projects//instances//databases/` for database resources.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/backupSchedules/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the DatabaseRoles collection.
*
* The typical use is:
*
* {@code Spanner spanner = new Spanner(...);}
* {@code Spanner.DatabaseRoles.List request = spanner.databaseRoles().list(parameters ...)}
*
*
* @return the resource collection
*/
public DatabaseRoles databaseRoles() {
return new DatabaseRoles();
}
/**
* The "databaseRoles" collection of methods.
*/
public class DatabaseRoles {
/**
* Lists Cloud Spanner database roles.
*
* Create a request for the method "databaseRoles.list".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The database whose roles should be listed. Values are of the form
* `projects//instances//databases/`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends SpannerRequest {
private static final String REST_PATH = "v1/{+parent}/databaseRoles";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
/**
* Lists Cloud Spanner database roles.
*
* Create a request for the method "databaseRoles.list".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The database whose roles should be listed. Values are of the form
* `projects//instances//databases/`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.ListDatabaseRolesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The database whose roles should be listed. Values are of the form
* `projects//instances//databases/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The database whose roles should be listed. Values are of the form
`projects//instances//databases/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The database whose roles should be listed. Values are of the form
* `projects//instances//databases/`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Number of database roles to be returned in the response. If 0 or less, defaults to
* the server's maximum allowed page size.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Number of database roles to be returned in the response. If 0 or less, defaults to the server's
maximum allowed page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Number of database roles to be returned in the response. If 0 or less, defaults to
* the server's maximum allowed page size.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* If non-empty, `page_token` should contain a next_page_token from a previous
* ListDatabaseRolesResponse.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** If non-empty, `page_token` should contain a next_page_token from a previous
ListDatabaseRolesResponse.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* If non-empty, `page_token` should contain a next_page_token from a previous
* ListDatabaseRolesResponse.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Returns permissions that the caller has on the specified database or backup resource. Attempting
* this RPC on a non-existent Cloud Spanner database will result in a NOT_FOUND error if the user
* has `spanner.databases.list` permission on the containing Cloud Spanner instance. Otherwise
* returns an empty set of permissions. Calling this method on a backup that does not exist will
* result in a NOT_FOUND error if the user has `spanner.backups.list` permission on the containing
* instance.
*
* Create a request for the method "databaseRoles.testIamPermissions".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The Cloud Spanner resource for which permissions are being tested. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* database resources.
* @param content the {@link com.google.api.services.spanner.v1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.spanner.v1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends SpannerRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+/databaseRoles/[^/]+$");
/**
* Returns permissions that the caller has on the specified database or backup resource.
* Attempting this RPC on a non-existent Cloud Spanner database will result in a NOT_FOUND error
* if the user has `spanner.databases.list` permission on the containing Cloud Spanner instance.
* Otherwise returns an empty set of permissions. Calling this method on a backup that does not
* exist will result in a NOT_FOUND error if the user has `spanner.backups.list` permission on the
* containing instance.
*
* Create a request for the method "databaseRoles.testIamPermissions".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The Cloud Spanner resource for which permissions are being tested. The format is
* `projects//instances/` for instance resources and `projects//instances//databases/` for
* database resources.
* @param content the {@link com.google.api.services.spanner.v1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.spanner.v1.model.TestIamPermissionsRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/databaseRoles/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The Cloud Spanner resource for which permissions are being tested. The
* format is `projects//instances/` for instance resources and
* `projects//instances//databases/` for database resources.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The Cloud Spanner resource for which permissions are being tested. The format is
`projects//instances/` for instance resources and `projects//instances//databases/` for database
resources.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The Cloud Spanner resource for which permissions are being tested. The
* format is `projects//instances/` for instance resources and
* `projects//instances//databases/` for database resources.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/databaseRoles/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code Spanner spanner = new Spanner(...);}
* {@code Spanner.Operations.List request = spanner.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be cancelled.
* @return the request
*/
public Cancel cancel(java.lang.String name) throws java.io.IOException {
Cancel result = new Cancel(name);
initialize(result);
return result;
}
public class Cancel extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+/operations/[^/]+$");
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation. {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be cancelled.
* @since 1.13
*/
protected Cancel(java.lang.String name) {
super(Spanner.this, "POST", REST_PATH, null, com.google.api.services.spanner.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/operations/[^/]+$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be cancelled. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be cancelled.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be cancelled. */
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be deleted.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+/operations/[^/]+$");
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be deleted.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Spanner.this, "DELETE", REST_PATH, null, com.google.api.services.spanner.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/operations/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be deleted. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be deleted.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be deleted. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+/operations$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.ListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/operations$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/operations$");
}
this.name = name;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Sessions collection.
*
* The typical use is:
*
* {@code Spanner spanner = new Spanner(...);}
* {@code Spanner.Sessions.List request = spanner.sessions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Sessions sessions() {
return new Sessions();
}
/**
* The "sessions" collection of methods.
*/
public class Sessions {
/**
* Creates multiple new sessions. This API can be used to initialize a session cache on the clients.
* See https://goo.gl/TgSFN2 for best practices on session cache management.
*
* Create a request for the method "sessions.batchCreate".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link BatchCreate#execute()} method to invoke the remote operation.
*
* @param database Required. The database in which the new sessions are created.
* @param content the {@link com.google.api.services.spanner.v1.model.BatchCreateSessionsRequest}
* @return the request
*/
public BatchCreate batchCreate(java.lang.String database, com.google.api.services.spanner.v1.model.BatchCreateSessionsRequest content) throws java.io.IOException {
BatchCreate result = new BatchCreate(database, content);
initialize(result);
return result;
}
public class BatchCreate extends SpannerRequest {
private static final String REST_PATH = "v1/{+database}/sessions:batchCreate";
private final java.util.regex.Pattern DATABASE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
/**
* Creates multiple new sessions. This API can be used to initialize a session cache on the
* clients. See https://goo.gl/TgSFN2 for best practices on session cache management.
*
* Create a request for the method "sessions.batchCreate".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link BatchCreate#execute()} method to invoke the remote operation.
* {@link
* BatchCreate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param database Required. The database in which the new sessions are created.
* @param content the {@link com.google.api.services.spanner.v1.model.BatchCreateSessionsRequest}
* @since 1.13
*/
protected BatchCreate(java.lang.String database, com.google.api.services.spanner.v1.model.BatchCreateSessionsRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.BatchCreateSessionsResponse.class);
this.database = com.google.api.client.util.Preconditions.checkNotNull(database, "Required parameter database must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DATABASE_PATTERN.matcher(database).matches(),
"Parameter database must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
}
@Override
public BatchCreate set$Xgafv(java.lang.String $Xgafv) {
return (BatchCreate) super.set$Xgafv($Xgafv);
}
@Override
public BatchCreate setAccessToken(java.lang.String accessToken) {
return (BatchCreate) super.setAccessToken(accessToken);
}
@Override
public BatchCreate setAlt(java.lang.String alt) {
return (BatchCreate) super.setAlt(alt);
}
@Override
public BatchCreate setCallback(java.lang.String callback) {
return (BatchCreate) super.setCallback(callback);
}
@Override
public BatchCreate setFields(java.lang.String fields) {
return (BatchCreate) super.setFields(fields);
}
@Override
public BatchCreate setKey(java.lang.String key) {
return (BatchCreate) super.setKey(key);
}
@Override
public BatchCreate setOauthToken(java.lang.String oauthToken) {
return (BatchCreate) super.setOauthToken(oauthToken);
}
@Override
public BatchCreate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchCreate) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchCreate setQuotaUser(java.lang.String quotaUser) {
return (BatchCreate) super.setQuotaUser(quotaUser);
}
@Override
public BatchCreate setUploadType(java.lang.String uploadType) {
return (BatchCreate) super.setUploadType(uploadType);
}
@Override
public BatchCreate setUploadProtocol(java.lang.String uploadProtocol) {
return (BatchCreate) super.setUploadProtocol(uploadProtocol);
}
/** Required. The database in which the new sessions are created. */
@com.google.api.client.util.Key
private java.lang.String database;
/** Required. The database in which the new sessions are created.
*/
public java.lang.String getDatabase() {
return database;
}
/** Required. The database in which the new sessions are created. */
public BatchCreate setDatabase(java.lang.String database) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DATABASE_PATTERN.matcher(database).matches(),
"Parameter database must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
this.database = database;
return this;
}
@Override
public BatchCreate set(String parameterName, Object value) {
return (BatchCreate) super.set(parameterName, value);
}
}
/**
* Batches the supplied mutation groups in a collection of efficient transactions. All mutations in
* a group are committed atomically. However, mutations across groups can be committed non-
* atomically in an unspecified order and thus, they must be independent of each other. Partial
* failure is possible, i.e., some groups may have been committed successfully, while some may have
* failed. The results of individual batches are streamed into the response as the batches are
* applied. BatchWrite requests are not replay protected, meaning that each mutation group may be
* applied more than once. Replays of non-idempotent mutations may have undesirable effects. For
* example, replays of an insert mutation may produce an already exists error or if you use
* generated or commit timestamp-based keys, it may result in additional rows being added to the
* mutation's table. We recommend structuring your mutation groups to be idempotent to avoid this
* issue.
*
* Create a request for the method "sessions.batchWrite".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link BatchWrite#execute()} method to invoke the remote operation.
*
* @param session Required. The session in which the batch request is to be run.
* @param content the {@link com.google.api.services.spanner.v1.model.BatchWriteRequest}
* @return the request
*/
public BatchWrite batchWrite(java.lang.String session, com.google.api.services.spanner.v1.model.BatchWriteRequest content) throws java.io.IOException {
BatchWrite result = new BatchWrite(session, content);
initialize(result);
return result;
}
public class BatchWrite extends SpannerRequest {
private static final String REST_PATH = "v1/{+session}:batchWrite";
private final java.util.regex.Pattern SESSION_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
/**
* Batches the supplied mutation groups in a collection of efficient transactions. All mutations
* in a group are committed atomically. However, mutations across groups can be committed non-
* atomically in an unspecified order and thus, they must be independent of each other. Partial
* failure is possible, i.e., some groups may have been committed successfully, while some may
* have failed. The results of individual batches are streamed into the response as the batches
* are applied. BatchWrite requests are not replay protected, meaning that each mutation group may
* be applied more than once. Replays of non-idempotent mutations may have undesirable effects.
* For example, replays of an insert mutation may produce an already exists error or if you use
* generated or commit timestamp-based keys, it may result in additional rows being added to the
* mutation's table. We recommend structuring your mutation groups to be idempotent to avoid this
* issue.
*
* Create a request for the method "sessions.batchWrite".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link BatchWrite#execute()} method to invoke the remote operation.
* {@link
* BatchWrite#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param session Required. The session in which the batch request is to be run.
* @param content the {@link com.google.api.services.spanner.v1.model.BatchWriteRequest}
* @since 1.13
*/
protected BatchWrite(java.lang.String session, com.google.api.services.spanner.v1.model.BatchWriteRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.BatchWriteResponse.class);
this.session = com.google.api.client.util.Preconditions.checkNotNull(session, "Required parameter session must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
}
@Override
public BatchWrite set$Xgafv(java.lang.String $Xgafv) {
return (BatchWrite) super.set$Xgafv($Xgafv);
}
@Override
public BatchWrite setAccessToken(java.lang.String accessToken) {
return (BatchWrite) super.setAccessToken(accessToken);
}
@Override
public BatchWrite setAlt(java.lang.String alt) {
return (BatchWrite) super.setAlt(alt);
}
@Override
public BatchWrite setCallback(java.lang.String callback) {
return (BatchWrite) super.setCallback(callback);
}
@Override
public BatchWrite setFields(java.lang.String fields) {
return (BatchWrite) super.setFields(fields);
}
@Override
public BatchWrite setKey(java.lang.String key) {
return (BatchWrite) super.setKey(key);
}
@Override
public BatchWrite setOauthToken(java.lang.String oauthToken) {
return (BatchWrite) super.setOauthToken(oauthToken);
}
@Override
public BatchWrite setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchWrite) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchWrite setQuotaUser(java.lang.String quotaUser) {
return (BatchWrite) super.setQuotaUser(quotaUser);
}
@Override
public BatchWrite setUploadType(java.lang.String uploadType) {
return (BatchWrite) super.setUploadType(uploadType);
}
@Override
public BatchWrite setUploadProtocol(java.lang.String uploadProtocol) {
return (BatchWrite) super.setUploadProtocol(uploadProtocol);
}
/** Required. The session in which the batch request is to be run. */
@com.google.api.client.util.Key
private java.lang.String session;
/** Required. The session in which the batch request is to be run.
*/
public java.lang.String getSession() {
return session;
}
/** Required. The session in which the batch request is to be run. */
public BatchWrite setSession(java.lang.String session) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
this.session = session;
return this;
}
@Override
public BatchWrite set(String parameterName, Object value) {
return (BatchWrite) super.set(parameterName, value);
}
}
/**
* Begins a new transaction. This step can often be skipped: Read, ExecuteSql and Commit can begin a
* new transaction as a side-effect.
*
* Create a request for the method "sessions.beginTransaction".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link BeginTransaction#execute()} method to invoke the remote operation.
*
* @param session Required. The session in which the transaction runs.
* @param content the {@link com.google.api.services.spanner.v1.model.BeginTransactionRequest}
* @return the request
*/
public BeginTransaction beginTransaction(java.lang.String session, com.google.api.services.spanner.v1.model.BeginTransactionRequest content) throws java.io.IOException {
BeginTransaction result = new BeginTransaction(session, content);
initialize(result);
return result;
}
public class BeginTransaction extends SpannerRequest {
private static final String REST_PATH = "v1/{+session}:beginTransaction";
private final java.util.regex.Pattern SESSION_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
/**
* Begins a new transaction. This step can often be skipped: Read, ExecuteSql and Commit can begin
* a new transaction as a side-effect.
*
* Create a request for the method "sessions.beginTransaction".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link BeginTransaction#execute()} method to invoke the remote operation.
* {@link BeginTransaction#initialize(com.google.api.client.googleapis.services.AbstractGoogle
* ClientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param session Required. The session in which the transaction runs.
* @param content the {@link com.google.api.services.spanner.v1.model.BeginTransactionRequest}
* @since 1.13
*/
protected BeginTransaction(java.lang.String session, com.google.api.services.spanner.v1.model.BeginTransactionRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.Transaction.class);
this.session = com.google.api.client.util.Preconditions.checkNotNull(session, "Required parameter session must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
}
@Override
public BeginTransaction set$Xgafv(java.lang.String $Xgafv) {
return (BeginTransaction) super.set$Xgafv($Xgafv);
}
@Override
public BeginTransaction setAccessToken(java.lang.String accessToken) {
return (BeginTransaction) super.setAccessToken(accessToken);
}
@Override
public BeginTransaction setAlt(java.lang.String alt) {
return (BeginTransaction) super.setAlt(alt);
}
@Override
public BeginTransaction setCallback(java.lang.String callback) {
return (BeginTransaction) super.setCallback(callback);
}
@Override
public BeginTransaction setFields(java.lang.String fields) {
return (BeginTransaction) super.setFields(fields);
}
@Override
public BeginTransaction setKey(java.lang.String key) {
return (BeginTransaction) super.setKey(key);
}
@Override
public BeginTransaction setOauthToken(java.lang.String oauthToken) {
return (BeginTransaction) super.setOauthToken(oauthToken);
}
@Override
public BeginTransaction setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BeginTransaction) super.setPrettyPrint(prettyPrint);
}
@Override
public BeginTransaction setQuotaUser(java.lang.String quotaUser) {
return (BeginTransaction) super.setQuotaUser(quotaUser);
}
@Override
public BeginTransaction setUploadType(java.lang.String uploadType) {
return (BeginTransaction) super.setUploadType(uploadType);
}
@Override
public BeginTransaction setUploadProtocol(java.lang.String uploadProtocol) {
return (BeginTransaction) super.setUploadProtocol(uploadProtocol);
}
/** Required. The session in which the transaction runs. */
@com.google.api.client.util.Key
private java.lang.String session;
/** Required. The session in which the transaction runs.
*/
public java.lang.String getSession() {
return session;
}
/** Required. The session in which the transaction runs. */
public BeginTransaction setSession(java.lang.String session) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
this.session = session;
return this;
}
@Override
public BeginTransaction set(String parameterName, Object value) {
return (BeginTransaction) super.set(parameterName, value);
}
}
/**
* Commits a transaction. The request includes the mutations to be applied to rows in the database.
* `Commit` might return an `ABORTED` error. This can occur at any time; commonly, the cause is
* conflicts with concurrent transactions. However, it can also happen for a variety of other
* reasons. If `Commit` returns `ABORTED`, the caller should re-attempt the transaction from the
* beginning, re-using the same session. On very rare occasions, `Commit` might return `UNKNOWN`.
* This can happen, for example, if the client job experiences a 1+ hour networking failure. At that
* point, Cloud Spanner has lost track of the transaction outcome and we recommend that you perform
* another read from the database to see the state of things as they are now.
*
* Create a request for the method "sessions.commit".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Commit#execute()} method to invoke the remote operation.
*
* @param session Required. The session in which the transaction to be committed is running.
* @param content the {@link com.google.api.services.spanner.v1.model.CommitRequest}
* @return the request
*/
public Commit commit(java.lang.String session, com.google.api.services.spanner.v1.model.CommitRequest content) throws java.io.IOException {
Commit result = new Commit(session, content);
initialize(result);
return result;
}
public class Commit extends SpannerRequest {
private static final String REST_PATH = "v1/{+session}:commit";
private final java.util.regex.Pattern SESSION_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
/**
* Commits a transaction. The request includes the mutations to be applied to rows in the
* database. `Commit` might return an `ABORTED` error. This can occur at any time; commonly, the
* cause is conflicts with concurrent transactions. However, it can also happen for a variety of
* other reasons. If `Commit` returns `ABORTED`, the caller should re-attempt the transaction from
* the beginning, re-using the same session. On very rare occasions, `Commit` might return
* `UNKNOWN`. This can happen, for example, if the client job experiences a 1+ hour networking
* failure. At that point, Cloud Spanner has lost track of the transaction outcome and we
* recommend that you perform another read from the database to see the state of things as they
* are now.
*
* Create a request for the method "sessions.commit".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Commit#execute()} method to invoke the remote operation. {@link
* Commit#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param session Required. The session in which the transaction to be committed is running.
* @param content the {@link com.google.api.services.spanner.v1.model.CommitRequest}
* @since 1.13
*/
protected Commit(java.lang.String session, com.google.api.services.spanner.v1.model.CommitRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.CommitResponse.class);
this.session = com.google.api.client.util.Preconditions.checkNotNull(session, "Required parameter session must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
}
@Override
public Commit set$Xgafv(java.lang.String $Xgafv) {
return (Commit) super.set$Xgafv($Xgafv);
}
@Override
public Commit setAccessToken(java.lang.String accessToken) {
return (Commit) super.setAccessToken(accessToken);
}
@Override
public Commit setAlt(java.lang.String alt) {
return (Commit) super.setAlt(alt);
}
@Override
public Commit setCallback(java.lang.String callback) {
return (Commit) super.setCallback(callback);
}
@Override
public Commit setFields(java.lang.String fields) {
return (Commit) super.setFields(fields);
}
@Override
public Commit setKey(java.lang.String key) {
return (Commit) super.setKey(key);
}
@Override
public Commit setOauthToken(java.lang.String oauthToken) {
return (Commit) super.setOauthToken(oauthToken);
}
@Override
public Commit setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Commit) super.setPrettyPrint(prettyPrint);
}
@Override
public Commit setQuotaUser(java.lang.String quotaUser) {
return (Commit) super.setQuotaUser(quotaUser);
}
@Override
public Commit setUploadType(java.lang.String uploadType) {
return (Commit) super.setUploadType(uploadType);
}
@Override
public Commit setUploadProtocol(java.lang.String uploadProtocol) {
return (Commit) super.setUploadProtocol(uploadProtocol);
}
/** Required. The session in which the transaction to be committed is running. */
@com.google.api.client.util.Key
private java.lang.String session;
/** Required. The session in which the transaction to be committed is running.
*/
public java.lang.String getSession() {
return session;
}
/** Required. The session in which the transaction to be committed is running. */
public Commit setSession(java.lang.String session) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
this.session = session;
return this;
}
@Override
public Commit set(String parameterName, Object value) {
return (Commit) super.set(parameterName, value);
}
}
/**
* Creates a new session. A session can be used to perform transactions that read and/or modify data
* in a Cloud Spanner database. Sessions are meant to be reused for many consecutive transactions.
* Sessions can only execute one transaction at a time. To execute multiple concurrent read-
* write/write-only transactions, create multiple sessions. Note that standalone reads and queries
* use a transaction internally, and count toward the one transaction limit. Active sessions use
* additional server resources, so it is a good idea to delete idle and unneeded sessions. Aside
* from explicit deletes, Cloud Spanner may delete sessions for which no operations are sent for
* more than an hour. If a session is deleted, requests to it return `NOT_FOUND`. Idle sessions can
* be kept alive by sending a trivial SQL query periodically, e.g., `"SELECT 1"`.
*
* Create a request for the method "sessions.create".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param database Required. The database in which the new session is created.
* @param content the {@link com.google.api.services.spanner.v1.model.CreateSessionRequest}
* @return the request
*/
public Create create(java.lang.String database, com.google.api.services.spanner.v1.model.CreateSessionRequest content) throws java.io.IOException {
Create result = new Create(database, content);
initialize(result);
return result;
}
public class Create extends SpannerRequest {
private static final String REST_PATH = "v1/{+database}/sessions";
private final java.util.regex.Pattern DATABASE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
/**
* Creates a new session. A session can be used to perform transactions that read and/or modify
* data in a Cloud Spanner database. Sessions are meant to be reused for many consecutive
* transactions. Sessions can only execute one transaction at a time. To execute multiple
* concurrent read-write/write-only transactions, create multiple sessions. Note that standalone
* reads and queries use a transaction internally, and count toward the one transaction limit.
* Active sessions use additional server resources, so it is a good idea to delete idle and
* unneeded sessions. Aside from explicit deletes, Cloud Spanner may delete sessions for which no
* operations are sent for more than an hour. If a session is deleted, requests to it return
* `NOT_FOUND`. Idle sessions can be kept alive by sending a trivial SQL query periodically, e.g.,
* `"SELECT 1"`.
*
* Create a request for the method "sessions.create".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param database Required. The database in which the new session is created.
* @param content the {@link com.google.api.services.spanner.v1.model.CreateSessionRequest}
* @since 1.13
*/
protected Create(java.lang.String database, com.google.api.services.spanner.v1.model.CreateSessionRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.Session.class);
this.database = com.google.api.client.util.Preconditions.checkNotNull(database, "Required parameter database must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DATABASE_PATTERN.matcher(database).matches(),
"Parameter database must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** Required. The database in which the new session is created. */
@com.google.api.client.util.Key
private java.lang.String database;
/** Required. The database in which the new session is created.
*/
public java.lang.String getDatabase() {
return database;
}
/** Required. The database in which the new session is created. */
public Create setDatabase(java.lang.String database) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DATABASE_PATTERN.matcher(database).matches(),
"Parameter database must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
this.database = database;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Ends a session, releasing server resources associated with it. This will asynchronously trigger
* cancellation of any operations that are running with this session.
*
* Create a request for the method "sessions.delete".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the session to delete.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
/**
* Ends a session, releasing server resources associated with it. This will asynchronously trigger
* cancellation of any operations that are running with this session.
*
* Create a request for the method "sessions.delete".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the session to delete.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Spanner.this, "DELETE", REST_PATH, null, com.google.api.services.spanner.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the session to delete. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the session to delete.
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the session to delete. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Executes a batch of SQL DML statements. This method allows many statements to be run with lower
* latency than submitting them sequentially with ExecuteSql. Statements are executed in sequential
* order. A request can succeed even if a statement fails. The ExecuteBatchDmlResponse.status field
* in the response provides information about the statement that failed. Clients must inspect this
* field to determine whether an error occurred. Execution stops after the first failed statement;
* the remaining statements are not executed.
*
* Create a request for the method "sessions.executeBatchDml".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link ExecuteBatchDml#execute()} method to invoke the remote operation.
*
* @param session Required. The session in which the DML statements should be performed.
* @param content the {@link com.google.api.services.spanner.v1.model.ExecuteBatchDmlRequest}
* @return the request
*/
public ExecuteBatchDml executeBatchDml(java.lang.String session, com.google.api.services.spanner.v1.model.ExecuteBatchDmlRequest content) throws java.io.IOException {
ExecuteBatchDml result = new ExecuteBatchDml(session, content);
initialize(result);
return result;
}
public class ExecuteBatchDml extends SpannerRequest {
private static final String REST_PATH = "v1/{+session}:executeBatchDml";
private final java.util.regex.Pattern SESSION_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
/**
* Executes a batch of SQL DML statements. This method allows many statements to be run with lower
* latency than submitting them sequentially with ExecuteSql. Statements are executed in
* sequential order. A request can succeed even if a statement fails. The
* ExecuteBatchDmlResponse.status field in the response provides information about the statement
* that failed. Clients must inspect this field to determine whether an error occurred. Execution
* stops after the first failed statement; the remaining statements are not executed.
*
* Create a request for the method "sessions.executeBatchDml".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link ExecuteBatchDml#execute()} method to invoke the remote operation.
* {@link ExecuteBatchDml#initialize(com.google.api.client.googleapis.services.AbstractGoogleC
* lientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param session Required. The session in which the DML statements should be performed.
* @param content the {@link com.google.api.services.spanner.v1.model.ExecuteBatchDmlRequest}
* @since 1.13
*/
protected ExecuteBatchDml(java.lang.String session, com.google.api.services.spanner.v1.model.ExecuteBatchDmlRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.ExecuteBatchDmlResponse.class);
this.session = com.google.api.client.util.Preconditions.checkNotNull(session, "Required parameter session must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
}
@Override
public ExecuteBatchDml set$Xgafv(java.lang.String $Xgafv) {
return (ExecuteBatchDml) super.set$Xgafv($Xgafv);
}
@Override
public ExecuteBatchDml setAccessToken(java.lang.String accessToken) {
return (ExecuteBatchDml) super.setAccessToken(accessToken);
}
@Override
public ExecuteBatchDml setAlt(java.lang.String alt) {
return (ExecuteBatchDml) super.setAlt(alt);
}
@Override
public ExecuteBatchDml setCallback(java.lang.String callback) {
return (ExecuteBatchDml) super.setCallback(callback);
}
@Override
public ExecuteBatchDml setFields(java.lang.String fields) {
return (ExecuteBatchDml) super.setFields(fields);
}
@Override
public ExecuteBatchDml setKey(java.lang.String key) {
return (ExecuteBatchDml) super.setKey(key);
}
@Override
public ExecuteBatchDml setOauthToken(java.lang.String oauthToken) {
return (ExecuteBatchDml) super.setOauthToken(oauthToken);
}
@Override
public ExecuteBatchDml setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ExecuteBatchDml) super.setPrettyPrint(prettyPrint);
}
@Override
public ExecuteBatchDml setQuotaUser(java.lang.String quotaUser) {
return (ExecuteBatchDml) super.setQuotaUser(quotaUser);
}
@Override
public ExecuteBatchDml setUploadType(java.lang.String uploadType) {
return (ExecuteBatchDml) super.setUploadType(uploadType);
}
@Override
public ExecuteBatchDml setUploadProtocol(java.lang.String uploadProtocol) {
return (ExecuteBatchDml) super.setUploadProtocol(uploadProtocol);
}
/** Required. The session in which the DML statements should be performed. */
@com.google.api.client.util.Key
private java.lang.String session;
/** Required. The session in which the DML statements should be performed.
*/
public java.lang.String getSession() {
return session;
}
/** Required. The session in which the DML statements should be performed. */
public ExecuteBatchDml setSession(java.lang.String session) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
this.session = session;
return this;
}
@Override
public ExecuteBatchDml set(String parameterName, Object value) {
return (ExecuteBatchDml) super.set(parameterName, value);
}
}
/**
* Executes an SQL statement, returning all results in a single reply. This method cannot be used to
* return a result set larger than 10 MiB; if the query yields more data than that, the query fails
* with a `FAILED_PRECONDITION` error. Operations inside read-write transactions might return
* `ABORTED`. If this occurs, the application should restart the transaction from the beginning. See
* Transaction for more details. Larger result sets can be fetched in streaming fashion by calling
* ExecuteStreamingSql instead.
*
* Create a request for the method "sessions.executeSql".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link ExecuteSql#execute()} method to invoke the remote operation.
*
* @param session Required. The session in which the SQL query should be performed.
* @param content the {@link com.google.api.services.spanner.v1.model.ExecuteSqlRequest}
* @return the request
*/
public ExecuteSql executeSql(java.lang.String session, com.google.api.services.spanner.v1.model.ExecuteSqlRequest content) throws java.io.IOException {
ExecuteSql result = new ExecuteSql(session, content);
initialize(result);
return result;
}
public class ExecuteSql extends SpannerRequest {
private static final String REST_PATH = "v1/{+session}:executeSql";
private final java.util.regex.Pattern SESSION_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
/**
* Executes an SQL statement, returning all results in a single reply. This method cannot be used
* to return a result set larger than 10 MiB; if the query yields more data than that, the query
* fails with a `FAILED_PRECONDITION` error. Operations inside read-write transactions might
* return `ABORTED`. If this occurs, the application should restart the transaction from the
* beginning. See Transaction for more details. Larger result sets can be fetched in streaming
* fashion by calling ExecuteStreamingSql instead.
*
* Create a request for the method "sessions.executeSql".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link ExecuteSql#execute()} method to invoke the remote operation.
* {@link
* ExecuteSql#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param session Required. The session in which the SQL query should be performed.
* @param content the {@link com.google.api.services.spanner.v1.model.ExecuteSqlRequest}
* @since 1.13
*/
protected ExecuteSql(java.lang.String session, com.google.api.services.spanner.v1.model.ExecuteSqlRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.ResultSet.class);
this.session = com.google.api.client.util.Preconditions.checkNotNull(session, "Required parameter session must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
}
@Override
public ExecuteSql set$Xgafv(java.lang.String $Xgafv) {
return (ExecuteSql) super.set$Xgafv($Xgafv);
}
@Override
public ExecuteSql setAccessToken(java.lang.String accessToken) {
return (ExecuteSql) super.setAccessToken(accessToken);
}
@Override
public ExecuteSql setAlt(java.lang.String alt) {
return (ExecuteSql) super.setAlt(alt);
}
@Override
public ExecuteSql setCallback(java.lang.String callback) {
return (ExecuteSql) super.setCallback(callback);
}
@Override
public ExecuteSql setFields(java.lang.String fields) {
return (ExecuteSql) super.setFields(fields);
}
@Override
public ExecuteSql setKey(java.lang.String key) {
return (ExecuteSql) super.setKey(key);
}
@Override
public ExecuteSql setOauthToken(java.lang.String oauthToken) {
return (ExecuteSql) super.setOauthToken(oauthToken);
}
@Override
public ExecuteSql setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ExecuteSql) super.setPrettyPrint(prettyPrint);
}
@Override
public ExecuteSql setQuotaUser(java.lang.String quotaUser) {
return (ExecuteSql) super.setQuotaUser(quotaUser);
}
@Override
public ExecuteSql setUploadType(java.lang.String uploadType) {
return (ExecuteSql) super.setUploadType(uploadType);
}
@Override
public ExecuteSql setUploadProtocol(java.lang.String uploadProtocol) {
return (ExecuteSql) super.setUploadProtocol(uploadProtocol);
}
/** Required. The session in which the SQL query should be performed. */
@com.google.api.client.util.Key
private java.lang.String session;
/** Required. The session in which the SQL query should be performed.
*/
public java.lang.String getSession() {
return session;
}
/** Required. The session in which the SQL query should be performed. */
public ExecuteSql setSession(java.lang.String session) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
this.session = session;
return this;
}
@Override
public ExecuteSql set(String parameterName, Object value) {
return (ExecuteSql) super.set(parameterName, value);
}
}
/**
* Like ExecuteSql, except returns the result set as a stream. Unlike ExecuteSql, there is no limit
* on the size of the returned result set. However, no individual row in the result set can exceed
* 100 MiB, and no column value can exceed 10 MiB.
*
* Create a request for the method "sessions.executeStreamingSql".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link ExecuteStreamingSql#execute()} method to invoke the remote operation.
*
* @param session Required. The session in which the SQL query should be performed.
* @param content the {@link com.google.api.services.spanner.v1.model.ExecuteSqlRequest}
* @return the request
*/
public ExecuteStreamingSql executeStreamingSql(java.lang.String session, com.google.api.services.spanner.v1.model.ExecuteSqlRequest content) throws java.io.IOException {
ExecuteStreamingSql result = new ExecuteStreamingSql(session, content);
initialize(result);
return result;
}
public class ExecuteStreamingSql extends SpannerRequest {
private static final String REST_PATH = "v1/{+session}:executeStreamingSql";
private final java.util.regex.Pattern SESSION_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
/**
* Like ExecuteSql, except returns the result set as a stream. Unlike ExecuteSql, there is no
* limit on the size of the returned result set. However, no individual row in the result set can
* exceed 100 MiB, and no column value can exceed 10 MiB.
*
* Create a request for the method "sessions.executeStreamingSql".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link ExecuteStreamingSql#execute()} method to invoke the remote
* operation. {@link ExecuteStreamingSql#initialize(com.google.api.client.googleapis.services.
* AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param session Required. The session in which the SQL query should be performed.
* @param content the {@link com.google.api.services.spanner.v1.model.ExecuteSqlRequest}
* @since 1.13
*/
protected ExecuteStreamingSql(java.lang.String session, com.google.api.services.spanner.v1.model.ExecuteSqlRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.PartialResultSet.class);
this.session = com.google.api.client.util.Preconditions.checkNotNull(session, "Required parameter session must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
}
@Override
public ExecuteStreamingSql set$Xgafv(java.lang.String $Xgafv) {
return (ExecuteStreamingSql) super.set$Xgafv($Xgafv);
}
@Override
public ExecuteStreamingSql setAccessToken(java.lang.String accessToken) {
return (ExecuteStreamingSql) super.setAccessToken(accessToken);
}
@Override
public ExecuteStreamingSql setAlt(java.lang.String alt) {
return (ExecuteStreamingSql) super.setAlt(alt);
}
@Override
public ExecuteStreamingSql setCallback(java.lang.String callback) {
return (ExecuteStreamingSql) super.setCallback(callback);
}
@Override
public ExecuteStreamingSql setFields(java.lang.String fields) {
return (ExecuteStreamingSql) super.setFields(fields);
}
@Override
public ExecuteStreamingSql setKey(java.lang.String key) {
return (ExecuteStreamingSql) super.setKey(key);
}
@Override
public ExecuteStreamingSql setOauthToken(java.lang.String oauthToken) {
return (ExecuteStreamingSql) super.setOauthToken(oauthToken);
}
@Override
public ExecuteStreamingSql setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ExecuteStreamingSql) super.setPrettyPrint(prettyPrint);
}
@Override
public ExecuteStreamingSql setQuotaUser(java.lang.String quotaUser) {
return (ExecuteStreamingSql) super.setQuotaUser(quotaUser);
}
@Override
public ExecuteStreamingSql setUploadType(java.lang.String uploadType) {
return (ExecuteStreamingSql) super.setUploadType(uploadType);
}
@Override
public ExecuteStreamingSql setUploadProtocol(java.lang.String uploadProtocol) {
return (ExecuteStreamingSql) super.setUploadProtocol(uploadProtocol);
}
/** Required. The session in which the SQL query should be performed. */
@com.google.api.client.util.Key
private java.lang.String session;
/** Required. The session in which the SQL query should be performed.
*/
public java.lang.String getSession() {
return session;
}
/** Required. The session in which the SQL query should be performed. */
public ExecuteStreamingSql setSession(java.lang.String session) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
this.session = session;
return this;
}
@Override
public ExecuteStreamingSql set(String parameterName, Object value) {
return (ExecuteStreamingSql) super.set(parameterName, value);
}
}
/**
* Gets a session. Returns `NOT_FOUND` if the session does not exist. This is mainly useful for
* determining whether a session is still alive.
*
* Create a request for the method "sessions.get".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the session to retrieve.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
/**
* Gets a session. Returns `NOT_FOUND` if the session does not exist. This is mainly useful for
* determining whether a session is still alive.
*
* Create a request for the method "sessions.get".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the session to retrieve.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.Session.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the session to retrieve. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the session to retrieve.
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the session to retrieve. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all sessions in a given database.
*
* Create a request for the method "sessions.list".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param database Required. The database in which to list sessions.
* @return the request
*/
public List list(java.lang.String database) throws java.io.IOException {
List result = new List(database);
initialize(result);
return result;
}
public class List extends SpannerRequest {
private static final String REST_PATH = "v1/{+database}/sessions";
private final java.util.regex.Pattern DATABASE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
/**
* Lists all sessions in a given database.
*
* Create a request for the method "sessions.list".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param database Required. The database in which to list sessions.
* @since 1.13
*/
protected List(java.lang.String database) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.ListSessionsResponse.class);
this.database = com.google.api.client.util.Preconditions.checkNotNull(database, "Required parameter database must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DATABASE_PATTERN.matcher(database).matches(),
"Parameter database must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Required. The database in which to list sessions. */
@com.google.api.client.util.Key
private java.lang.String database;
/** Required. The database in which to list sessions.
*/
public java.lang.String getDatabase() {
return database;
}
/** Required. The database in which to list sessions. */
public List setDatabase(java.lang.String database) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DATABASE_PATTERN.matcher(database).matches(),
"Parameter database must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+$");
}
this.database = database;
return this;
}
/**
* An expression for filtering the results of the request. Filter rules are case
* insensitive. The fields eligible for filtering are: * `labels.key` where key is the
* name of a label Some examples of using filters are: * `labels.env:*` --> The session
* has the label "env". * `labels.env:dev` --> The session has the label "env" and the
* value of the label contains the string "dev".
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** An expression for filtering the results of the request. Filter rules are case insensitive. The
fields eligible for filtering are: * `labels.key` where key is the name of a label Some examples of
using filters are: * `labels.env:*` --> The session has the label "env". * `labels.env:dev` --> The
session has the label "env" and the value of the label contains the string "dev".
*/
public java.lang.String getFilter() {
return filter;
}
/**
* An expression for filtering the results of the request. Filter rules are case
* insensitive. The fields eligible for filtering are: * `labels.key` where key is the
* name of a label Some examples of using filters are: * `labels.env:*` --> The session
* has the label "env". * `labels.env:dev` --> The session has the label "env" and the
* value of the label contains the string "dev".
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Number of sessions to be returned in the response. If 0 or less, defaults to the
* server's maximum allowed page size.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Number of sessions to be returned in the response. If 0 or less, defaults to the server's maximum
allowed page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Number of sessions to be returned in the response. If 0 or less, defaults to the
* server's maximum allowed page size.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* If non-empty, `page_token` should contain a next_page_token from a previous
* ListSessionsResponse.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** If non-empty, `page_token` should contain a next_page_token from a previous ListSessionsResponse.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* If non-empty, `page_token` should contain a next_page_token from a previous
* ListSessionsResponse.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Creates a set of partition tokens that can be used to execute a query operation in parallel. Each
* of the returned partition tokens can be used by ExecuteStreamingSql to specify a subset of the
* query result to read. The same session and read-only transaction must be used by the
* PartitionQueryRequest used to create the partition tokens and the ExecuteSqlRequests that use the
* partition tokens. Partition tokens become invalid when the session used to create them is
* deleted, is idle for too long, begins a new transaction, or becomes too old. When any of these
* happen, it is not possible to resume the query, and the whole operation must be restarted from
* the beginning.
*
* Create a request for the method "sessions.partitionQuery".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link PartitionQuery#execute()} method to invoke the remote operation.
*
* @param session Required. The session used to create the partitions.
* @param content the {@link com.google.api.services.spanner.v1.model.PartitionQueryRequest}
* @return the request
*/
public PartitionQuery partitionQuery(java.lang.String session, com.google.api.services.spanner.v1.model.PartitionQueryRequest content) throws java.io.IOException {
PartitionQuery result = new PartitionQuery(session, content);
initialize(result);
return result;
}
public class PartitionQuery extends SpannerRequest {
private static final String REST_PATH = "v1/{+session}:partitionQuery";
private final java.util.regex.Pattern SESSION_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
/**
* Creates a set of partition tokens that can be used to execute a query operation in parallel.
* Each of the returned partition tokens can be used by ExecuteStreamingSql to specify a subset of
* the query result to read. The same session and read-only transaction must be used by the
* PartitionQueryRequest used to create the partition tokens and the ExecuteSqlRequests that use
* the partition tokens. Partition tokens become invalid when the session used to create them is
* deleted, is idle for too long, begins a new transaction, or becomes too old. When any of these
* happen, it is not possible to resume the query, and the whole operation must be restarted from
* the beginning.
*
* Create a request for the method "sessions.partitionQuery".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link PartitionQuery#execute()} method to invoke the remote operation.
* {@link PartitionQuery#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param session Required. The session used to create the partitions.
* @param content the {@link com.google.api.services.spanner.v1.model.PartitionQueryRequest}
* @since 1.13
*/
protected PartitionQuery(java.lang.String session, com.google.api.services.spanner.v1.model.PartitionQueryRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.PartitionResponse.class);
this.session = com.google.api.client.util.Preconditions.checkNotNull(session, "Required parameter session must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
}
@Override
public PartitionQuery set$Xgafv(java.lang.String $Xgafv) {
return (PartitionQuery) super.set$Xgafv($Xgafv);
}
@Override
public PartitionQuery setAccessToken(java.lang.String accessToken) {
return (PartitionQuery) super.setAccessToken(accessToken);
}
@Override
public PartitionQuery setAlt(java.lang.String alt) {
return (PartitionQuery) super.setAlt(alt);
}
@Override
public PartitionQuery setCallback(java.lang.String callback) {
return (PartitionQuery) super.setCallback(callback);
}
@Override
public PartitionQuery setFields(java.lang.String fields) {
return (PartitionQuery) super.setFields(fields);
}
@Override
public PartitionQuery setKey(java.lang.String key) {
return (PartitionQuery) super.setKey(key);
}
@Override
public PartitionQuery setOauthToken(java.lang.String oauthToken) {
return (PartitionQuery) super.setOauthToken(oauthToken);
}
@Override
public PartitionQuery setPrettyPrint(java.lang.Boolean prettyPrint) {
return (PartitionQuery) super.setPrettyPrint(prettyPrint);
}
@Override
public PartitionQuery setQuotaUser(java.lang.String quotaUser) {
return (PartitionQuery) super.setQuotaUser(quotaUser);
}
@Override
public PartitionQuery setUploadType(java.lang.String uploadType) {
return (PartitionQuery) super.setUploadType(uploadType);
}
@Override
public PartitionQuery setUploadProtocol(java.lang.String uploadProtocol) {
return (PartitionQuery) super.setUploadProtocol(uploadProtocol);
}
/** Required. The session used to create the partitions. */
@com.google.api.client.util.Key
private java.lang.String session;
/** Required. The session used to create the partitions.
*/
public java.lang.String getSession() {
return session;
}
/** Required. The session used to create the partitions. */
public PartitionQuery setSession(java.lang.String session) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
this.session = session;
return this;
}
@Override
public PartitionQuery set(String parameterName, Object value) {
return (PartitionQuery) super.set(parameterName, value);
}
}
/**
* Creates a set of partition tokens that can be used to execute a read operation in parallel. Each
* of the returned partition tokens can be used by StreamingRead to specify a subset of the read
* result to read. The same session and read-only transaction must be used by the
* PartitionReadRequest used to create the partition tokens and the ReadRequests that use the
* partition tokens. There are no ordering guarantees on rows returned among the returned partition
* tokens, or even within each individual StreamingRead call issued with a partition_token.
* Partition tokens become invalid when the session used to create them is deleted, is idle for too
* long, begins a new transaction, or becomes too old. When any of these happen, it is not possible
* to resume the read, and the whole operation must be restarted from the beginning.
*
* Create a request for the method "sessions.partitionRead".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link PartitionRead#execute()} method to invoke the remote operation.
*
* @param session Required. The session used to create the partitions.
* @param content the {@link com.google.api.services.spanner.v1.model.PartitionReadRequest}
* @return the request
*/
public PartitionRead partitionRead(java.lang.String session, com.google.api.services.spanner.v1.model.PartitionReadRequest content) throws java.io.IOException {
PartitionRead result = new PartitionRead(session, content);
initialize(result);
return result;
}
public class PartitionRead extends SpannerRequest {
private static final String REST_PATH = "v1/{+session}:partitionRead";
private final java.util.regex.Pattern SESSION_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
/**
* Creates a set of partition tokens that can be used to execute a read operation in parallel.
* Each of the returned partition tokens can be used by StreamingRead to specify a subset of the
* read result to read. The same session and read-only transaction must be used by the
* PartitionReadRequest used to create the partition tokens and the ReadRequests that use the
* partition tokens. There are no ordering guarantees on rows returned among the returned
* partition tokens, or even within each individual StreamingRead call issued with a
* partition_token. Partition tokens become invalid when the session used to create them is
* deleted, is idle for too long, begins a new transaction, or becomes too old. When any of these
* happen, it is not possible to resume the read, and the whole operation must be restarted from
* the beginning.
*
* Create a request for the method "sessions.partitionRead".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link PartitionRead#execute()} method to invoke the remote operation.
* {@link PartitionRead#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientR
* equest)} must be called to initialize this instance immediately after invoking the constructor.
*
*
* @param session Required. The session used to create the partitions.
* @param content the {@link com.google.api.services.spanner.v1.model.PartitionReadRequest}
* @since 1.13
*/
protected PartitionRead(java.lang.String session, com.google.api.services.spanner.v1.model.PartitionReadRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.PartitionResponse.class);
this.session = com.google.api.client.util.Preconditions.checkNotNull(session, "Required parameter session must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
}
@Override
public PartitionRead set$Xgafv(java.lang.String $Xgafv) {
return (PartitionRead) super.set$Xgafv($Xgafv);
}
@Override
public PartitionRead setAccessToken(java.lang.String accessToken) {
return (PartitionRead) super.setAccessToken(accessToken);
}
@Override
public PartitionRead setAlt(java.lang.String alt) {
return (PartitionRead) super.setAlt(alt);
}
@Override
public PartitionRead setCallback(java.lang.String callback) {
return (PartitionRead) super.setCallback(callback);
}
@Override
public PartitionRead setFields(java.lang.String fields) {
return (PartitionRead) super.setFields(fields);
}
@Override
public PartitionRead setKey(java.lang.String key) {
return (PartitionRead) super.setKey(key);
}
@Override
public PartitionRead setOauthToken(java.lang.String oauthToken) {
return (PartitionRead) super.setOauthToken(oauthToken);
}
@Override
public PartitionRead setPrettyPrint(java.lang.Boolean prettyPrint) {
return (PartitionRead) super.setPrettyPrint(prettyPrint);
}
@Override
public PartitionRead setQuotaUser(java.lang.String quotaUser) {
return (PartitionRead) super.setQuotaUser(quotaUser);
}
@Override
public PartitionRead setUploadType(java.lang.String uploadType) {
return (PartitionRead) super.setUploadType(uploadType);
}
@Override
public PartitionRead setUploadProtocol(java.lang.String uploadProtocol) {
return (PartitionRead) super.setUploadProtocol(uploadProtocol);
}
/** Required. The session used to create the partitions. */
@com.google.api.client.util.Key
private java.lang.String session;
/** Required. The session used to create the partitions.
*/
public java.lang.String getSession() {
return session;
}
/** Required. The session used to create the partitions. */
public PartitionRead setSession(java.lang.String session) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
this.session = session;
return this;
}
@Override
public PartitionRead set(String parameterName, Object value) {
return (PartitionRead) super.set(parameterName, value);
}
}
/**
* Reads rows from the database using key lookups and scans, as a simple key/value style alternative
* to ExecuteSql. This method cannot be used to return a result set larger than 10 MiB; if the read
* matches more data than that, the read fails with a `FAILED_PRECONDITION` error. Reads inside
* read-write transactions might return `ABORTED`. If this occurs, the application should restart
* the transaction from the beginning. See Transaction for more details. Larger result sets can be
* yielded in streaming fashion by calling StreamingRead instead.
*
* Create a request for the method "sessions.read".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Read#execute()} method to invoke the remote operation.
*
* @param session Required. The session in which the read should be performed.
* @param content the {@link com.google.api.services.spanner.v1.model.ReadRequest}
* @return the request
*/
public Read read(java.lang.String session, com.google.api.services.spanner.v1.model.ReadRequest content) throws java.io.IOException {
Read result = new Read(session, content);
initialize(result);
return result;
}
public class Read extends SpannerRequest {
private static final String REST_PATH = "v1/{+session}:read";
private final java.util.regex.Pattern SESSION_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
/**
* Reads rows from the database using key lookups and scans, as a simple key/value style
* alternative to ExecuteSql. This method cannot be used to return a result set larger than 10
* MiB; if the read matches more data than that, the read fails with a `FAILED_PRECONDITION`
* error. Reads inside read-write transactions might return `ABORTED`. If this occurs, the
* application should restart the transaction from the beginning. See Transaction for more
* details. Larger result sets can be yielded in streaming fashion by calling StreamingRead
* instead.
*
* Create a request for the method "sessions.read".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Read#execute()} method to invoke the remote operation. {@link
* Read#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param session Required. The session in which the read should be performed.
* @param content the {@link com.google.api.services.spanner.v1.model.ReadRequest}
* @since 1.13
*/
protected Read(java.lang.String session, com.google.api.services.spanner.v1.model.ReadRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.ResultSet.class);
this.session = com.google.api.client.util.Preconditions.checkNotNull(session, "Required parameter session must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
}
@Override
public Read set$Xgafv(java.lang.String $Xgafv) {
return (Read) super.set$Xgafv($Xgafv);
}
@Override
public Read setAccessToken(java.lang.String accessToken) {
return (Read) super.setAccessToken(accessToken);
}
@Override
public Read setAlt(java.lang.String alt) {
return (Read) super.setAlt(alt);
}
@Override
public Read setCallback(java.lang.String callback) {
return (Read) super.setCallback(callback);
}
@Override
public Read setFields(java.lang.String fields) {
return (Read) super.setFields(fields);
}
@Override
public Read setKey(java.lang.String key) {
return (Read) super.setKey(key);
}
@Override
public Read setOauthToken(java.lang.String oauthToken) {
return (Read) super.setOauthToken(oauthToken);
}
@Override
public Read setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Read) super.setPrettyPrint(prettyPrint);
}
@Override
public Read setQuotaUser(java.lang.String quotaUser) {
return (Read) super.setQuotaUser(quotaUser);
}
@Override
public Read setUploadType(java.lang.String uploadType) {
return (Read) super.setUploadType(uploadType);
}
@Override
public Read setUploadProtocol(java.lang.String uploadProtocol) {
return (Read) super.setUploadProtocol(uploadProtocol);
}
/** Required. The session in which the read should be performed. */
@com.google.api.client.util.Key
private java.lang.String session;
/** Required. The session in which the read should be performed.
*/
public java.lang.String getSession() {
return session;
}
/** Required. The session in which the read should be performed. */
public Read setSession(java.lang.String session) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
this.session = session;
return this;
}
@Override
public Read set(String parameterName, Object value) {
return (Read) super.set(parameterName, value);
}
}
/**
* Rolls back a transaction, releasing any locks it holds. It is a good idea to call this for any
* transaction that includes one or more Read or ExecuteSql requests and ultimately decides not to
* commit. `Rollback` returns `OK` if it successfully aborts the transaction, the transaction was
* already aborted, or the transaction is not found. `Rollback` never returns `ABORTED`.
*
* Create a request for the method "sessions.rollback".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Rollback#execute()} method to invoke the remote operation.
*
* @param session Required. The session in which the transaction to roll back is running.
* @param content the {@link com.google.api.services.spanner.v1.model.RollbackRequest}
* @return the request
*/
public Rollback rollback(java.lang.String session, com.google.api.services.spanner.v1.model.RollbackRequest content) throws java.io.IOException {
Rollback result = new Rollback(session, content);
initialize(result);
return result;
}
public class Rollback extends SpannerRequest {
private static final String REST_PATH = "v1/{+session}:rollback";
private final java.util.regex.Pattern SESSION_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
/**
* Rolls back a transaction, releasing any locks it holds. It is a good idea to call this for any
* transaction that includes one or more Read or ExecuteSql requests and ultimately decides not to
* commit. `Rollback` returns `OK` if it successfully aborts the transaction, the transaction was
* already aborted, or the transaction is not found. `Rollback` never returns `ABORTED`.
*
* Create a request for the method "sessions.rollback".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Rollback#execute()} method to invoke the remote operation.
* {@link
* Rollback#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param session Required. The session in which the transaction to roll back is running.
* @param content the {@link com.google.api.services.spanner.v1.model.RollbackRequest}
* @since 1.13
*/
protected Rollback(java.lang.String session, com.google.api.services.spanner.v1.model.RollbackRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.Empty.class);
this.session = com.google.api.client.util.Preconditions.checkNotNull(session, "Required parameter session must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
}
@Override
public Rollback set$Xgafv(java.lang.String $Xgafv) {
return (Rollback) super.set$Xgafv($Xgafv);
}
@Override
public Rollback setAccessToken(java.lang.String accessToken) {
return (Rollback) super.setAccessToken(accessToken);
}
@Override
public Rollback setAlt(java.lang.String alt) {
return (Rollback) super.setAlt(alt);
}
@Override
public Rollback setCallback(java.lang.String callback) {
return (Rollback) super.setCallback(callback);
}
@Override
public Rollback setFields(java.lang.String fields) {
return (Rollback) super.setFields(fields);
}
@Override
public Rollback setKey(java.lang.String key) {
return (Rollback) super.setKey(key);
}
@Override
public Rollback setOauthToken(java.lang.String oauthToken) {
return (Rollback) super.setOauthToken(oauthToken);
}
@Override
public Rollback setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Rollback) super.setPrettyPrint(prettyPrint);
}
@Override
public Rollback setQuotaUser(java.lang.String quotaUser) {
return (Rollback) super.setQuotaUser(quotaUser);
}
@Override
public Rollback setUploadType(java.lang.String uploadType) {
return (Rollback) super.setUploadType(uploadType);
}
@Override
public Rollback setUploadProtocol(java.lang.String uploadProtocol) {
return (Rollback) super.setUploadProtocol(uploadProtocol);
}
/** Required. The session in which the transaction to roll back is running. */
@com.google.api.client.util.Key
private java.lang.String session;
/** Required. The session in which the transaction to roll back is running.
*/
public java.lang.String getSession() {
return session;
}
/** Required. The session in which the transaction to roll back is running. */
public Rollback setSession(java.lang.String session) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
this.session = session;
return this;
}
@Override
public Rollback set(String parameterName, Object value) {
return (Rollback) super.set(parameterName, value);
}
}
/**
* Like Read, except returns the result set as a stream. Unlike Read, there is no limit on the size
* of the returned result set. However, no individual row in the result set can exceed 100 MiB, and
* no column value can exceed 10 MiB.
*
* Create a request for the method "sessions.streamingRead".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link StreamingRead#execute()} method to invoke the remote operation.
*
* @param session Required. The session in which the read should be performed.
* @param content the {@link com.google.api.services.spanner.v1.model.ReadRequest}
* @return the request
*/
public StreamingRead streamingRead(java.lang.String session, com.google.api.services.spanner.v1.model.ReadRequest content) throws java.io.IOException {
StreamingRead result = new StreamingRead(session, content);
initialize(result);
return result;
}
public class StreamingRead extends SpannerRequest {
private static final String REST_PATH = "v1/{+session}:streamingRead";
private final java.util.regex.Pattern SESSION_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
/**
* Like Read, except returns the result set as a stream. Unlike Read, there is no limit on the
* size of the returned result set. However, no individual row in the result set can exceed 100
* MiB, and no column value can exceed 10 MiB.
*
* Create a request for the method "sessions.streamingRead".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link StreamingRead#execute()} method to invoke the remote operation.
* {@link StreamingRead#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientR
* equest)} must be called to initialize this instance immediately after invoking the constructor.
*
*
* @param session Required. The session in which the read should be performed.
* @param content the {@link com.google.api.services.spanner.v1.model.ReadRequest}
* @since 1.13
*/
protected StreamingRead(java.lang.String session, com.google.api.services.spanner.v1.model.ReadRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.PartialResultSet.class);
this.session = com.google.api.client.util.Preconditions.checkNotNull(session, "Required parameter session must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
}
@Override
public StreamingRead set$Xgafv(java.lang.String $Xgafv) {
return (StreamingRead) super.set$Xgafv($Xgafv);
}
@Override
public StreamingRead setAccessToken(java.lang.String accessToken) {
return (StreamingRead) super.setAccessToken(accessToken);
}
@Override
public StreamingRead setAlt(java.lang.String alt) {
return (StreamingRead) super.setAlt(alt);
}
@Override
public StreamingRead setCallback(java.lang.String callback) {
return (StreamingRead) super.setCallback(callback);
}
@Override
public StreamingRead setFields(java.lang.String fields) {
return (StreamingRead) super.setFields(fields);
}
@Override
public StreamingRead setKey(java.lang.String key) {
return (StreamingRead) super.setKey(key);
}
@Override
public StreamingRead setOauthToken(java.lang.String oauthToken) {
return (StreamingRead) super.setOauthToken(oauthToken);
}
@Override
public StreamingRead setPrettyPrint(java.lang.Boolean prettyPrint) {
return (StreamingRead) super.setPrettyPrint(prettyPrint);
}
@Override
public StreamingRead setQuotaUser(java.lang.String quotaUser) {
return (StreamingRead) super.setQuotaUser(quotaUser);
}
@Override
public StreamingRead setUploadType(java.lang.String uploadType) {
return (StreamingRead) super.setUploadType(uploadType);
}
@Override
public StreamingRead setUploadProtocol(java.lang.String uploadProtocol) {
return (StreamingRead) super.setUploadProtocol(uploadProtocol);
}
/** Required. The session in which the read should be performed. */
@com.google.api.client.util.Key
private java.lang.String session;
/** Required. The session in which the read should be performed.
*/
public java.lang.String getSession() {
return session;
}
/** Required. The session in which the read should be performed. */
public StreamingRead setSession(java.lang.String session) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/databases/[^/]+/sessions/[^/]+$");
}
this.session = session;
return this;
}
@Override
public StreamingRead set(String parameterName, Object value) {
return (StreamingRead) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the InstancePartitionOperations collection.
*
* The typical use is:
*
* {@code Spanner spanner = new Spanner(...);}
* {@code Spanner.InstancePartitionOperations.List request = spanner.instancePartitionOperations().list(parameters ...)}
*
*
* @return the resource collection
*/
public InstancePartitionOperations instancePartitionOperations() {
return new InstancePartitionOperations();
}
/**
* The "instancePartitionOperations" collection of methods.
*/
public class InstancePartitionOperations {
/**
* Lists instance partition long-running operations in the given instance. An instance partition
* operation has a name of the form `projects//instances//instancePartitions//operations/`. The
* long-running operation metadata field type `metadata.type_url` describes the type of the
* metadata. Operations returned include those that have completed/failed/canceled within the last 7
* days, and pending operations. Operations returned are ordered by
* `operation.metadata.value.start_time` in descending order starting from the most recently started
* operation. Authorization requires `spanner.instancePartitionOperations.list` permission on the
* resource parent.
*
* Create a request for the method "instancePartitionOperations.list".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent instance of the instance partition operations. Values are of the form
* `projects//instances/`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends SpannerRequest {
private static final String REST_PATH = "v1/{+parent}/instancePartitionOperations";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+$");
/**
* Lists instance partition long-running operations in the given instance. An instance partition
* operation has a name of the form `projects//instances//instancePartitions//operations/`. The
* long-running operation metadata field type `metadata.type_url` describes the type of the
* metadata. Operations returned include those that have completed/failed/canceled within the last
* 7 days, and pending operations. Operations returned are ordered by
* `operation.metadata.value.start_time` in descending order starting from the most recently
* started operation. Authorization requires `spanner.instancePartitionOperations.list` permission
* on the resource parent.
*
* Create a request for the method "instancePartitionOperations.list".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent instance of the instance partition operations. Values are of the form
* `projects//instances/`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.ListInstancePartitionOperationsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent instance of the instance partition operations. Values are of the
* form `projects//instances/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent instance of the instance partition operations. Values are of the form
`projects//instances/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent instance of the instance partition operations. Values are of the
* form `projects//instances/`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. An expression that filters the list of returned operations. A filter
* expression consists of a field name, a comparison operator, and a value for filtering.
* The value must be a string, a number, or a boolean. The comparison operator must be one
* of: `<`, `>`, `<=`, `>=`, `!=`, `=`, or `:`. Colon `:` is the contains operator. Filter
* rules are not case sensitive. The following fields in the Operation are eligible for
* filtering: * `name` - The name of the long-running operation * `done` - False if the
* operation is in progress, else true. * `metadata.@type` - the type of metadata. For
* example, the type string for CreateInstancePartitionMetadata is
* `type.googleapis.com/google.spanner.admin.instance.v1.CreateInstancePartitionMetadata`.
* * `metadata.` - any field in metadata.value. `metadata.@type` must be specified first,
* if filtering on metadata fields. * `error` - Error associated with the long-running
* operation. * `response.@type` - the type of response. * `response.` - any field in
* response.value. You can combine multiple expressions by enclosing each expression in
* parentheses. By default, expressions are combined with AND logic. However, you can
* specify AND, OR, and NOT logic explicitly. Here are a few examples: * `done:true` - The
* operation is complete. * `(metadata.@type=` \
* `type.googleapis.com/google.spanner.admin.instance.v1.CreateInstancePartitionMetadata)
* AND` \ `(metadata.instance_partition.name:custom-instance-partition) AND` \
* `(metadata.start_time < \"2021-03-28T14:50:00Z\") AND` \ `(error:*)` - Return
* operations where: * The operation's metadata type is CreateInstancePartitionMetadata. *
* The instance partition name contains "custom-instance-partition". * The operation
* started before 2021-03-28T14:50:00Z. * The operation resulted in an error.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. An expression that filters the list of returned operations. A filter expression consists
of a field name, a comparison operator, and a value for filtering. The value must be a string, a
number, or a boolean. The comparison operator must be one of: `<`, `>`, `<=`, `>=`, `!=`, `=`, or
`:`. Colon `:` is the contains operator. Filter rules are not case sensitive. The following fields
in the Operation are eligible for filtering: * `name` - The name of the long-running operation *
`done` - False if the operation is in progress, else true. * `metadata.@type` - the type of
metadata. For example, the type string for CreateInstancePartitionMetadata is
`type.googleapis.com/google.spanner.admin.instance.v1.CreateInstancePartitionMetadata`. *
`metadata.` - any field in metadata.value. `metadata.@type` must be specified first, if filtering
on metadata fields. * `error` - Error associated with the long-running operation. *
`response.@type` - the type of response. * `response.` - any field in response.value. You can
combine multiple expressions by enclosing each expression in parentheses. By default, expressions
are combined with AND logic. However, you can specify AND, OR, and NOT logic explicitly. Here are a
few examples: * `done:true` - The operation is complete. * `(metadata.@type=` \
`type.googleapis.com/google.spanner.admin.instance.v1.CreateInstancePartitionMetadata) AND` \
`(metadata.instance_partition.name:custom-instance-partition) AND` \ `(metadata.start_time <
\"2021-03-28T14:50:00Z\") AND` \ `(error:*)` - Return operations where: * The operation's metadata
type is CreateInstancePartitionMetadata. * The instance partition name contains "custom-instance-
partition". * The operation started before 2021-03-28T14:50:00Z. * The operation resulted in an
error.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. An expression that filters the list of returned operations. A filter
* expression consists of a field name, a comparison operator, and a value for filtering.
* The value must be a string, a number, or a boolean. The comparison operator must be one
* of: `<`, `>`, `<=`, `>=`, `!=`, `=`, or `:`. Colon `:` is the contains operator. Filter
* rules are not case sensitive. The following fields in the Operation are eligible for
* filtering: * `name` - The name of the long-running operation * `done` - False if the
* operation is in progress, else true. * `metadata.@type` - the type of metadata. For
* example, the type string for CreateInstancePartitionMetadata is
* `type.googleapis.com/google.spanner.admin.instance.v1.CreateInstancePartitionMetadata`.
* * `metadata.` - any field in metadata.value. `metadata.@type` must be specified first,
* if filtering on metadata fields. * `error` - Error associated with the long-running
* operation. * `response.@type` - the type of response. * `response.` - any field in
* response.value. You can combine multiple expressions by enclosing each expression in
* parentheses. By default, expressions are combined with AND logic. However, you can
* specify AND, OR, and NOT logic explicitly. Here are a few examples: * `done:true` - The
* operation is complete. * `(metadata.@type=` \
* `type.googleapis.com/google.spanner.admin.instance.v1.CreateInstancePartitionMetadata)
* AND` \ `(metadata.instance_partition.name:custom-instance-partition) AND` \
* `(metadata.start_time < \"2021-03-28T14:50:00Z\") AND` \ `(error:*)` - Return
* operations where: * The operation's metadata type is CreateInstancePartitionMetadata. *
* The instance partition name contains "custom-instance-partition". * The operation
* started before 2021-03-28T14:50:00Z. * The operation resulted in an error.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. Deadline used while retrieving metadata for instance partition operations.
* Instance partitions whose operation metadata cannot be retrieved within this deadline
* will be added to unreachable in ListInstancePartitionOperationsResponse.
*/
@com.google.api.client.util.Key
private String instancePartitionDeadline;
/** Optional. Deadline used while retrieving metadata for instance partition operations. Instance
partitions whose operation metadata cannot be retrieved within this deadline will be added to
unreachable in ListInstancePartitionOperationsResponse.
*/
public String getInstancePartitionDeadline() {
return instancePartitionDeadline;
}
/**
* Optional. Deadline used while retrieving metadata for instance partition operations.
* Instance partitions whose operation metadata cannot be retrieved within this deadline
* will be added to unreachable in ListInstancePartitionOperationsResponse.
*/
public List setInstancePartitionDeadline(String instancePartitionDeadline) {
this.instancePartitionDeadline = instancePartitionDeadline;
return this;
}
/**
* Optional. Number of operations to be returned in the response. If 0 or less, defaults
* to the server's maximum allowed page size.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. Number of operations to be returned in the response. If 0 or less, defaults to the
server's maximum allowed page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. Number of operations to be returned in the response. If 0 or less, defaults
* to the server's maximum allowed page size.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If non-empty, `page_token` should contain a next_page_token from a previous
* ListInstancePartitionOperationsResponse to the same `parent` and with the same
* `filter`.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If non-empty, `page_token` should contain a next_page_token from a previous
ListInstancePartitionOperationsResponse to the same `parent` and with the same `filter`.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If non-empty, `page_token` should contain a next_page_token from a previous
* ListInstancePartitionOperationsResponse to the same `parent` and with the same
* `filter`.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the InstancePartitions collection.
*
* The typical use is:
*
* {@code Spanner spanner = new Spanner(...);}
* {@code Spanner.InstancePartitions.List request = spanner.instancePartitions().list(parameters ...)}
*
*
* @return the resource collection
*/
public InstancePartitions instancePartitions() {
return new InstancePartitions();
}
/**
* The "instancePartitions" collection of methods.
*/
public class InstancePartitions {
/**
* Creates an instance partition and begins preparing it to be used. The returned long-running
* operation can be used to track the progress of preparing the new instance partition. The instance
* partition name is assigned by the caller. If the named instance partition already exists,
* `CreateInstancePartition` returns `ALREADY_EXISTS`. Immediately upon completion of this request:
* * The instance partition is readable via the API, with all requested attributes but no allocated
* resources. Its state is `CREATING`. Until completion of the returned operation: * Cancelling the
* operation renders the instance partition immediately unreadable via the API. * The instance
* partition can be deleted. * All other attempts to modify the instance partition are rejected.
* Upon completion of the returned operation: * Billing for all successfully-allocated resources
* begins (some types may have lower than the requested levels). * Databases can start using this
* instance partition. * The instance partition's allocated resource levels are readable via the
* API. * The instance partition's state becomes `READY`. The returned long-running operation will
* have a name of the format `/operations/` and can be used to track creation of the instance
* partition. The metadata field type is CreateInstancePartitionMetadata. The response field type is
* InstancePartition, if successful.
*
* Create a request for the method "instancePartitions.create".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the instance in which to create the instance partition. Values are of the form
* `projects//instances/`.
* @param content the {@link com.google.api.services.spanner.v1.model.CreateInstancePartitionRequest}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.spanner.v1.model.CreateInstancePartitionRequest content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends SpannerRequest {
private static final String REST_PATH = "v1/{+parent}/instancePartitions";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+$");
/**
* Creates an instance partition and begins preparing it to be used. The returned long-running
* operation can be used to track the progress of preparing the new instance partition. The
* instance partition name is assigned by the caller. If the named instance partition already
* exists, `CreateInstancePartition` returns `ALREADY_EXISTS`. Immediately upon completion of this
* request: * The instance partition is readable via the API, with all requested attributes but no
* allocated resources. Its state is `CREATING`. Until completion of the returned operation: *
* Cancelling the operation renders the instance partition immediately unreadable via the API. *
* The instance partition can be deleted. * All other attempts to modify the instance partition
* are rejected. Upon completion of the returned operation: * Billing for all successfully-
* allocated resources begins (some types may have lower than the requested levels). * Databases
* can start using this instance partition. * The instance partition's allocated resource levels
* are readable via the API. * The instance partition's state becomes `READY`. The returned long-
* running operation will have a name of the format `/operations/` and can be used to track
* creation of the instance partition. The metadata field type is CreateInstancePartitionMetadata.
* The response field type is InstancePartition, if successful.
*
* Create a request for the method "instancePartitions.create".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the instance in which to create the instance partition. Values are of the form
* `projects//instances/`.
* @param content the {@link com.google.api.services.spanner.v1.model.CreateInstancePartitionRequest}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.spanner.v1.model.CreateInstancePartitionRequest content) {
super(Spanner.this, "POST", REST_PATH, content, com.google.api.services.spanner.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the instance in which to create the instance partition. Values
* are of the form `projects//instances/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the instance in which to create the instance partition. Values are of the
form `projects//instances/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the instance in which to create the instance partition. Values
* are of the form `projects//instances/`.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an existing instance partition. Requires that the instance partition is not used by any
* database or backup and is not the default instance partition of an instance. Authorization
* requires `spanner.instancePartitions.delete` permission on the resource name.
*
* Create a request for the method "instancePartitions.delete".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the instance partition to be deleted. Values are of the form
* `projects/{project}/instances/{instance}/instancePartitions/{instance_partition}`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/instancePartitions/[^/]+$");
/**
* Deletes an existing instance partition. Requires that the instance partition is not used by any
* database or backup and is not the default instance partition of an instance. Authorization
* requires `spanner.instancePartitions.delete` permission on the resource name.
*
* Create a request for the method "instancePartitions.delete".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the instance partition to be deleted. Values are of the form
* `projects/{project}/instances/{instance}/instancePartitions/{instance_partition}`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Spanner.this, "DELETE", REST_PATH, null, com.google.api.services.spanner.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/instancePartitions/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the instance partition to be deleted. Values are of the form
* `projects/{project}/instances/{instance}/instancePartitions/{instance_partition}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the instance partition to be deleted. Values are of the form
`projects/{project}/instances/{instance}/instancePartitions/{instance_partition}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the instance partition to be deleted. Values are of the form
* `projects/{project}/instances/{instance}/instancePartitions/{instance_partition}`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/instancePartitions/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. If not empty, the API only deletes the instance partition when the etag
* provided matches the current status of the requested instance partition. Otherwise,
* deletes the instance partition without checking the current status of the requested
* instance partition.
*/
@com.google.api.client.util.Key
private java.lang.String etag;
/** Optional. If not empty, the API only deletes the instance partition when the etag provided matches
the current status of the requested instance partition. Otherwise, deletes the instance partition
without checking the current status of the requested instance partition.
*/
public java.lang.String getEtag() {
return etag;
}
/**
* Optional. If not empty, the API only deletes the instance partition when the etag
* provided matches the current status of the requested instance partition. Otherwise,
* deletes the instance partition without checking the current status of the requested
* instance partition.
*/
public Delete setEtag(java.lang.String etag) {
this.etag = etag;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets information about a particular instance partition.
*
* Create a request for the method "instancePartitions.get".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the requested instance partition. Values are of the form
* `projects/{project}/instances/{instance}/instancePartitions/{instance_partition}`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/instancePartitions/[^/]+$");
/**
* Gets information about a particular instance partition.
*
* Create a request for the method "instancePartitions.get".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the requested instance partition. Values are of the form
* `projects/{project}/instances/{instance}/instancePartitions/{instance_partition}`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.InstancePartition.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/instancePartitions/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the requested instance partition. Values are of the form
* `projects/{project}/instances/{instance}/instancePartitions/{instance_partition}`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the requested instance partition. Values are of the form
`projects/{project}/instances/{instance}/instancePartitions/{instance_partition}`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the requested instance partition. Values are of the form
* `projects/{project}/instances/{instance}/instancePartitions/{instance_partition}`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/instancePartitions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all instance partitions for the given instance.
*
* Create a request for the method "instancePartitions.list".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The instance whose instance partitions should be listed. Values are of the form
* `projects//instances/`. Use `{instance} = '-'` to list instance partitions for all
* Instances in a project, e.g., `projects/myproject/instances/-`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends SpannerRequest {
private static final String REST_PATH = "v1/{+parent}/instancePartitions";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+$");
/**
* Lists all instance partitions for the given instance.
*
* Create a request for the method "instancePartitions.list".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The instance whose instance partitions should be listed. Values are of the form
* `projects//instances/`. Use `{instance} = '-'` to list instance partitions for all
* Instances in a project, e.g., `projects/myproject/instances/-`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.ListInstancePartitionsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The instance whose instance partitions should be listed. Values are of the
* form `projects//instances/`. Use `{instance} = '-'` to list instance partitions for all
* Instances in a project, e.g., `projects/myproject/instances/-`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The instance whose instance partitions should be listed. Values are of the form
`projects//instances/`. Use `{instance} = '-'` to list instance partitions for all Instances in a
project, e.g., `projects/myproject/instances/-`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The instance whose instance partitions should be listed. Values are of the
* form `projects//instances/`. Use `{instance} = '-'` to list instance partitions for all
* Instances in a project, e.g., `projects/myproject/instances/-`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. Deadline used while retrieving metadata for instance partitions. Instance
* partitions whose metadata cannot be retrieved within this deadline will be added to
* unreachable in ListInstancePartitionsResponse.
*/
@com.google.api.client.util.Key
private String instancePartitionDeadline;
/** Optional. Deadline used while retrieving metadata for instance partitions. Instance partitions
whose metadata cannot be retrieved within this deadline will be added to unreachable in
ListInstancePartitionsResponse.
*/
public String getInstancePartitionDeadline() {
return instancePartitionDeadline;
}
/**
* Optional. Deadline used while retrieving metadata for instance partitions. Instance
* partitions whose metadata cannot be retrieved within this deadline will be added to
* unreachable in ListInstancePartitionsResponse.
*/
public List setInstancePartitionDeadline(String instancePartitionDeadline) {
this.instancePartitionDeadline = instancePartitionDeadline;
return this;
}
/**
* Number of instance partitions to be returned in the response. If 0 or less, defaults to
* the server's maximum allowed page size.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Number of instance partitions to be returned in the response. If 0 or less, defaults to the
server's maximum allowed page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Number of instance partitions to be returned in the response. If 0 or less, defaults to
* the server's maximum allowed page size.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* If non-empty, `page_token` should contain a next_page_token from a previous
* ListInstancePartitionsResponse.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** If non-empty, `page_token` should contain a next_page_token from a previous
ListInstancePartitionsResponse.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* If non-empty, `page_token` should contain a next_page_token from a previous
* ListInstancePartitionsResponse.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an instance partition, and begins allocating or releasing resources as requested. The
* returned long-running operation can be used to track the progress of updating the instance
* partition. If the named instance partition does not exist, returns `NOT_FOUND`. Immediately upon
* completion of this request: * For resource types for which a decrease in the instance partition's
* allocation has been requested, billing is based on the newly-requested level. Until completion of
* the returned operation: * Cancelling the operation sets its metadata's cancel_time, and begins
* restoring resources to their pre-request values. The operation is guaranteed to succeed at
* undoing all resource changes, after which point it terminates with a `CANCELLED` status. * All
* other attempts to modify the instance partition are rejected. * Reading the instance partition
* via the API continues to give the pre-request resource levels. Upon completion of the returned
* operation: * Billing begins for all successfully-allocated resources (some types may have lower
* than the requested levels). * All newly-reserved resources are available for serving the instance
* partition's tables. * The instance partition's new resource levels are readable via the API. The
* returned long-running operation will have a name of the format `/operations/` and can be used to
* track the instance partition modification. The metadata field type is
* UpdateInstancePartitionMetadata. The response field type is InstancePartition, if successful.
* Authorization requires `spanner.instancePartitions.update` permission on the resource name.
*
* Create a request for the method "instancePartitions.patch".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. A unique identifier for the instance partition. Values are of the form
* `projects//instances//instancePartitions/a-z*[a-z0-9]`. The final segment of the name must
* be between 2 and 64 characters in length. An instance partition's name cannot be changed
* after the instance partition is created.
* @param content the {@link com.google.api.services.spanner.v1.model.UpdateInstancePartitionRequest}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.spanner.v1.model.UpdateInstancePartitionRequest content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/instancePartitions/[^/]+$");
/**
* Updates an instance partition, and begins allocating or releasing resources as requested. The
* returned long-running operation can be used to track the progress of updating the instance
* partition. If the named instance partition does not exist, returns `NOT_FOUND`. Immediately
* upon completion of this request: * For resource types for which a decrease in the instance
* partition's allocation has been requested, billing is based on the newly-requested level. Until
* completion of the returned operation: * Cancelling the operation sets its metadata's
* cancel_time, and begins restoring resources to their pre-request values. The operation is
* guaranteed to succeed at undoing all resource changes, after which point it terminates with a
* `CANCELLED` status. * All other attempts to modify the instance partition are rejected. *
* Reading the instance partition via the API continues to give the pre-request resource levels.
* Upon completion of the returned operation: * Billing begins for all successfully-allocated
* resources (some types may have lower than the requested levels). * All newly-reserved resources
* are available for serving the instance partition's tables. * The instance partition's new
* resource levels are readable via the API. The returned long-running operation will have a name
* of the format `/operations/` and can be used to track the instance partition modification. The
* metadata field type is UpdateInstancePartitionMetadata. The response field type is
* InstancePartition, if successful. Authorization requires `spanner.instancePartitions.update`
* permission on the resource name.
*
* Create a request for the method "instancePartitions.patch".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. A unique identifier for the instance partition. Values are of the form
* `projects//instances//instancePartitions/a-z*[a-z0-9]`. The final segment of the name must
* be between 2 and 64 characters in length. An instance partition's name cannot be changed
* after the instance partition is created.
* @param content the {@link com.google.api.services.spanner.v1.model.UpdateInstancePartitionRequest}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.spanner.v1.model.UpdateInstancePartitionRequest content) {
super(Spanner.this, "PATCH", REST_PATH, content, com.google.api.services.spanner.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/instancePartitions/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. A unique identifier for the instance partition. Values are of the form
* `projects//instances//instancePartitions/a-z*[a-z0-9]`. The final segment of the name
* must be between 2 and 64 characters in length. An instance partition's name cannot be
* changed after the instance partition is created.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. A unique identifier for the instance partition. Values are of the form
`projects//instances//instancePartitions/a-z*[a-z0-9]`. The final segment of the name must be
between 2 and 64 characters in length. An instance partition's name cannot be changed after the
instance partition is created.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. A unique identifier for the instance partition. Values are of the form
* `projects//instances//instancePartitions/a-z*[a-z0-9]`. The final segment of the name
* must be between 2 and 64 characters in length. An instance partition's name cannot be
* changed after the instance partition is created.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/instancePartitions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code Spanner spanner = new Spanner(...);}
* {@code Spanner.Operations.List request = spanner.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be cancelled.
* @return the request
*/
public Cancel cancel(java.lang.String name) throws java.io.IOException {
Cancel result = new Cancel(name);
initialize(result);
return result;
}
public class Cancel extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/instancePartitions/[^/]+/operations/[^/]+$");
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation. {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be cancelled.
* @since 1.13
*/
protected Cancel(java.lang.String name) {
super(Spanner.this, "POST", REST_PATH, null, com.google.api.services.spanner.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/instancePartitions/[^/]+/operations/[^/]+$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be cancelled. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be cancelled.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be cancelled. */
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/instancePartitions/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be deleted.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/instancePartitions/[^/]+/operations/[^/]+$");
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be deleted.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Spanner.this, "DELETE", REST_PATH, null, com.google.api.services.spanner.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/instancePartitions/[^/]+/operations/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be deleted. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be deleted.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be deleted. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/instancePartitions/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/instancePartitions/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/instancePartitions/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/instancePartitions/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/instancePartitions/[^/]+/operations$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.ListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/instancePartitions/[^/]+/operations$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/instancePartitions/[^/]+/operations$");
}
this.name = name;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code Spanner spanner = new Spanner(...);}
* {@code Spanner.Operations.List request = spanner.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be cancelled.
* @return the request
*/
public Cancel cancel(java.lang.String name) throws java.io.IOException {
Cancel result = new Cancel(name);
initialize(result);
return result;
}
public class Cancel extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/operations/[^/]+$");
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation. {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be cancelled.
* @since 1.13
*/
protected Cancel(java.lang.String name) {
super(Spanner.this, "POST", REST_PATH, null, com.google.api.services.spanner.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/operations/[^/]+$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be cancelled. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be cancelled.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be cancelled. */
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be deleted.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/operations/[^/]+$");
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be deleted.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Spanner.this, "DELETE", REST_PATH, null, com.google.api.services.spanner.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/operations/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be deleted. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be deleted.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be deleted. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends SpannerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/instances/[^/]+/operations$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.ListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/operations$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/instances/[^/]+/operations$");
}
this.name = name;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
}
/**
* An accessor for creating requests from the Scans collection.
*
* The typical use is:
*
* {@code Spanner spanner = new Spanner(...);}
* {@code Spanner.Scans.List request = spanner.scans().list(parameters ...)}
*
*
* @return the resource collection
*/
public Scans scans() {
return new Scans();
}
/**
* The "scans" collection of methods.
*/
public class Scans {
/**
* Return available scans given a Database-specific resource name.
*
* Create a request for the method "scans.list".
*
* This request holds the parameters needed by the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The unique name of the parent resource, specific to the Database service implementing this
* interface.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends SpannerRequest {
private static final String REST_PATH = "v1/{+parent}";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^scans$");
/**
* Return available scans given a Database-specific resource name.
*
* Create a request for the method "scans.list".
*
* This request holds the parameters needed by the the spanner server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The unique name of the parent resource, specific to the Database service implementing this
* interface.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Spanner.this, "GET", REST_PATH, null, com.google.api.services.spanner.v1.model.ListScansResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^scans$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The unique name of the parent resource, specific to the Database service
* implementing this interface.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The unique name of the parent resource, specific to the Database service implementing
this interface.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The unique name of the parent resource, specific to the Database service
* implementing this interface.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^scans$");
}
this.parent = parent;
return this;
}
/**
* A filter expression to restrict the results based on information present in the available
* Scan collection. The filter applies to all fields within the Scan message except for
* `data`.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter expression to restrict the results based on information present in the available Scan
collection. The filter applies to all fields within the Scan message except for `data`.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter expression to restrict the results based on information present in the available
* Scan collection. The filter applies to all fields within the Scan message except for
* `data`.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The maximum number of items to return. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The maximum number of items to return. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous List request, if any. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous List request, if any.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous List request, if any. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Specifies which parts of the Scan should be returned in the response. Note, only the
* SUMMARY view (the default) is currently supported for ListScans.
*/
@com.google.api.client.util.Key
private java.lang.String view;
/** Specifies which parts of the Scan should be returned in the response. Note, only the SUMMARY view
(the default) is currently supported for ListScans.
*/
public java.lang.String getView() {
return view;
}
/**
* Specifies which parts of the Scan should be returned in the response. Note, only the
* SUMMARY view (the default) is currently supported for ListScans.
*/
public List setView(java.lang.String view) {
this.view = view;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* Builder for {@link Spanner}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link Spanner}. */
@Override
public Spanner build() {
return new Spanner(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link SpannerRequestInitializer}.
*
* @since 1.12
*/
public Builder setSpannerRequestInitializer(
SpannerRequestInitializer spannerRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(spannerRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}