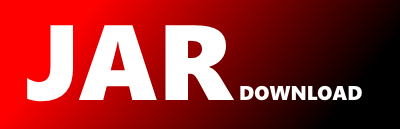
com.google.api.services.spanner.v1.model.CreateDatabaseRequest Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.spanner.v1.model;
/**
* The request for CreateDatabase.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Spanner API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class CreateDatabaseRequest extends com.google.api.client.json.GenericJson {
/**
* Required. A `CREATE DATABASE` statement, which specifies the ID of the new database. The
* database ID must conform to the regular expression `a-z*[a-z0-9]` and be between 2 and 30
* characters in length. If the database ID is a reserved word or if it contains a hyphen, the
* database ID must be enclosed in backticks (`` ` ``).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String createStatement;
/**
* Optional. The dialect of the Cloud Spanner Database.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String databaseDialect;
/**
* Optional. The encryption configuration for the database. If this field is not specified, Cloud
* Spanner will encrypt/decrypt all data at rest using Google default encryption.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private EncryptionConfig encryptionConfig;
/**
* Optional. A list of DDL statements to run inside the newly created database. Statements can
* create tables, indexes, etc. These statements execute atomically with the creation of the
* database: if there is an error in any statement, the database is not created.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List extraStatements;
/**
* Optional. Proto descriptors used by `CREATE/ALTER PROTO BUNDLE` statements in
* 'extra_statements'. Contains a protobuf-serialized [`google.protobuf.FileDescriptorSet`](https:
* //github.com/protocolbuffers/protobuf/blob/main/src/google/protobuf/descriptor.proto)
* descriptor set. To generate it, [install](https://grpc.io/docs/protoc-installation/) and run
* `protoc` with --include_imports and --descriptor_set_out. For example, to generate for
* moon/shot/app.proto, run ``` $protoc --proto_path=/app_path --proto_path=/lib_path \
* --include_imports \ --descriptor_set_out=descriptors.data \ moon/shot/app.proto ``` For more
* details, see protobuffer [self description](https://developers.google.com/protocol-
* buffers/docs/techniques#self-description).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String protoDescriptors;
/**
* Required. A `CREATE DATABASE` statement, which specifies the ID of the new database. The
* database ID must conform to the regular expression `a-z*[a-z0-9]` and be between 2 and 30
* characters in length. If the database ID is a reserved word or if it contains a hyphen, the
* database ID must be enclosed in backticks (`` ` ``).
* @return value or {@code null} for none
*/
public java.lang.String getCreateStatement() {
return createStatement;
}
/**
* Required. A `CREATE DATABASE` statement, which specifies the ID of the new database. The
* database ID must conform to the regular expression `a-z*[a-z0-9]` and be between 2 and 30
* characters in length. If the database ID is a reserved word or if it contains a hyphen, the
* database ID must be enclosed in backticks (`` ` ``).
* @param createStatement createStatement or {@code null} for none
*/
public CreateDatabaseRequest setCreateStatement(java.lang.String createStatement) {
this.createStatement = createStatement;
return this;
}
/**
* Optional. The dialect of the Cloud Spanner Database.
* @return value or {@code null} for none
*/
public java.lang.String getDatabaseDialect() {
return databaseDialect;
}
/**
* Optional. The dialect of the Cloud Spanner Database.
* @param databaseDialect databaseDialect or {@code null} for none
*/
public CreateDatabaseRequest setDatabaseDialect(java.lang.String databaseDialect) {
this.databaseDialect = databaseDialect;
return this;
}
/**
* Optional. The encryption configuration for the database. If this field is not specified, Cloud
* Spanner will encrypt/decrypt all data at rest using Google default encryption.
* @return value or {@code null} for none
*/
public EncryptionConfig getEncryptionConfig() {
return encryptionConfig;
}
/**
* Optional. The encryption configuration for the database. If this field is not specified, Cloud
* Spanner will encrypt/decrypt all data at rest using Google default encryption.
* @param encryptionConfig encryptionConfig or {@code null} for none
*/
public CreateDatabaseRequest setEncryptionConfig(EncryptionConfig encryptionConfig) {
this.encryptionConfig = encryptionConfig;
return this;
}
/**
* Optional. A list of DDL statements to run inside the newly created database. Statements can
* create tables, indexes, etc. These statements execute atomically with the creation of the
* database: if there is an error in any statement, the database is not created.
* @return value or {@code null} for none
*/
public java.util.List getExtraStatements() {
return extraStatements;
}
/**
* Optional. A list of DDL statements to run inside the newly created database. Statements can
* create tables, indexes, etc. These statements execute atomically with the creation of the
* database: if there is an error in any statement, the database is not created.
* @param extraStatements extraStatements or {@code null} for none
*/
public CreateDatabaseRequest setExtraStatements(java.util.List extraStatements) {
this.extraStatements = extraStatements;
return this;
}
/**
* Optional. Proto descriptors used by `CREATE/ALTER PROTO BUNDLE` statements in
* 'extra_statements'. Contains a protobuf-serialized [`google.protobuf.FileDescriptorSet`](https:
* //github.com/protocolbuffers/protobuf/blob/main/src/google/protobuf/descriptor.proto)
* descriptor set. To generate it, [install](https://grpc.io/docs/protoc-installation/) and run
* `protoc` with --include_imports and --descriptor_set_out. For example, to generate for
* moon/shot/app.proto, run ``` $protoc --proto_path=/app_path --proto_path=/lib_path \
* --include_imports \ --descriptor_set_out=descriptors.data \ moon/shot/app.proto ``` For more
* details, see protobuffer [self description](https://developers.google.com/protocol-
* buffers/docs/techniques#self-description).
* @see #decodeProtoDescriptors()
* @return value or {@code null} for none
*/
public java.lang.String getProtoDescriptors() {
return protoDescriptors;
}
/**
* Optional. Proto descriptors used by `CREATE/ALTER PROTO BUNDLE` statements in
* 'extra_statements'. Contains a protobuf-serialized [`google.protobuf.FileDescriptorSet`](https:
* //github.com/protocolbuffers/protobuf/blob/main/src/google/protobuf/descriptor.proto)
* descriptor set. To generate it, [install](https://grpc.io/docs/protoc-installation/) and run
* `protoc` with --include_imports and --descriptor_set_out. For example, to generate for
* moon/shot/app.proto, run ``` $protoc --proto_path=/app_path --proto_path=/lib_path \
* --include_imports \ --descriptor_set_out=descriptors.data \ moon/shot/app.proto ``` For more
* details, see protobuffer [self description](https://developers.google.com/protocol-
* buffers/docs/techniques#self-description).
* @see #getProtoDescriptors()
* @return Base64 decoded value or {@code null} for none
*
* @since 1.14
*/
public byte[] decodeProtoDescriptors() {
return com.google.api.client.util.Base64.decodeBase64(protoDescriptors);
}
/**
* Optional. Proto descriptors used by `CREATE/ALTER PROTO BUNDLE` statements in
* 'extra_statements'. Contains a protobuf-serialized [`google.protobuf.FileDescriptorSet`](https:
* //github.com/protocolbuffers/protobuf/blob/main/src/google/protobuf/descriptor.proto)
* descriptor set. To generate it, [install](https://grpc.io/docs/protoc-installation/) and run
* `protoc` with --include_imports and --descriptor_set_out. For example, to generate for
* moon/shot/app.proto, run ``` $protoc --proto_path=/app_path --proto_path=/lib_path \
* --include_imports \ --descriptor_set_out=descriptors.data \ moon/shot/app.proto ``` For more
* details, see protobuffer [self description](https://developers.google.com/protocol-
* buffers/docs/techniques#self-description).
* @see #encodeProtoDescriptors()
* @param protoDescriptors protoDescriptors or {@code null} for none
*/
public CreateDatabaseRequest setProtoDescriptors(java.lang.String protoDescriptors) {
this.protoDescriptors = protoDescriptors;
return this;
}
/**
* Optional. Proto descriptors used by `CREATE/ALTER PROTO BUNDLE` statements in
* 'extra_statements'. Contains a protobuf-serialized [`google.protobuf.FileDescriptorSet`](https:
* //github.com/protocolbuffers/protobuf/blob/main/src/google/protobuf/descriptor.proto)
* descriptor set. To generate it, [install](https://grpc.io/docs/protoc-installation/) and run
* `protoc` with --include_imports and --descriptor_set_out. For example, to generate for
* moon/shot/app.proto, run ``` $protoc --proto_path=/app_path --proto_path=/lib_path \
* --include_imports \ --descriptor_set_out=descriptors.data \ moon/shot/app.proto ``` For more
* details, see protobuffer [self description](https://developers.google.com/protocol-
* buffers/docs/techniques#self-description).
* @see #setProtoDescriptors()
*
*
* The value is encoded Base64 or {@code null} for none.
*
*
* @since 1.14
*/
public CreateDatabaseRequest encodeProtoDescriptors(byte[] protoDescriptors) {
this.protoDescriptors = com.google.api.client.util.Base64.encodeBase64URLSafeString(protoDescriptors);
return this;
}
@Override
public CreateDatabaseRequest set(String fieldName, Object value) {
return (CreateDatabaseRequest) super.set(fieldName, value);
}
@Override
public CreateDatabaseRequest clone() {
return (CreateDatabaseRequest) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy