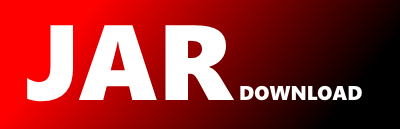
com.google.api.services.spanner.v1.model.ExecuteBatchDmlResponse Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.spanner.v1.model;
/**
* The response for ExecuteBatchDml. Contains a list of ResultSet messages, one for each DML
* statement that has successfully executed, in the same order as the statements in the request. If
* a statement fails, the status in the response body identifies the cause of the failure. To check
* for DML statements that failed, use the following approach: 1. Check the status in the response
* message. The google.rpc.Code enum value `OK` indicates that all statements were executed
* successfully. 2. If the status was not `OK`, check the number of result sets in the response. If
* the response contains `N` ResultSet messages, then statement `N+1` in the request failed. Example
* 1: * Request: 5 DML statements, all executed successfully. * Response: 5 ResultSet messages, with
* the status `OK`. Example 2: * Request: 5 DML statements. The third statement has a syntax error.
* * Response: 2 ResultSet messages, and a syntax error (`INVALID_ARGUMENT`) status. The number of
* ResultSet messages indicates that the third statement failed, and the fourth and fifth statements
* were not executed.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Spanner API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ExecuteBatchDmlResponse extends com.google.api.client.json.GenericJson {
/**
* One ResultSet for each statement in the request that ran successfully, in the same order as the
* statements in the request. Each ResultSet does not contain any rows. The ResultSetStats in each
* ResultSet contain the number of rows modified by the statement. Only the first ResultSet in the
* response contains valid ResultSetMetadata.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List resultSets;
/**
* If all DML statements are executed successfully, the status is `OK`. Otherwise, the error
* status of the first failed statement.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Status status;
/**
* One ResultSet for each statement in the request that ran successfully, in the same order as the
* statements in the request. Each ResultSet does not contain any rows. The ResultSetStats in each
* ResultSet contain the number of rows modified by the statement. Only the first ResultSet in the
* response contains valid ResultSetMetadata.
* @return value or {@code null} for none
*/
public java.util.List getResultSets() {
return resultSets;
}
/**
* One ResultSet for each statement in the request that ran successfully, in the same order as the
* statements in the request. Each ResultSet does not contain any rows. The ResultSetStats in each
* ResultSet contain the number of rows modified by the statement. Only the first ResultSet in the
* response contains valid ResultSetMetadata.
* @param resultSets resultSets or {@code null} for none
*/
public ExecuteBatchDmlResponse setResultSets(java.util.List resultSets) {
this.resultSets = resultSets;
return this;
}
/**
* If all DML statements are executed successfully, the status is `OK`. Otherwise, the error
* status of the first failed statement.
* @return value or {@code null} for none
*/
public Status getStatus() {
return status;
}
/**
* If all DML statements are executed successfully, the status is `OK`. Otherwise, the error
* status of the first failed statement.
* @param status status or {@code null} for none
*/
public ExecuteBatchDmlResponse setStatus(Status status) {
this.status = status;
return this;
}
@Override
public ExecuteBatchDmlResponse set(String fieldName, Object value) {
return (ExecuteBatchDmlResponse) super.set(fieldName, value);
}
@Override
public ExecuteBatchDmlResponse clone() {
return (ExecuteBatchDmlResponse) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy