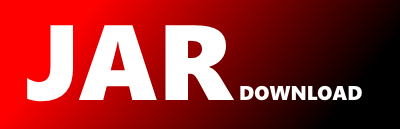
com.google.api.services.spanner.v1.model.KeyRangeInfo Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.spanner.v1.model;
/**
* A message representing information for a key range (possibly one key).
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Spanner API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class KeyRangeInfo extends com.google.api.client.json.GenericJson {
/**
* The list of context values for this key range.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List contextValues;
static {
// hack to force ProGuard to consider ContextValue used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(ContextValue.class);
}
/**
* The index of the end key in indexed_keys.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer endKeyIndex;
/**
* Information about this key range, for all metrics.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private LocalizedString info;
/**
* The number of keys this range covers.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long keysCount;
/**
* The name of the metric. e.g. "latency".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private LocalizedString metric;
/**
* The index of the start key in indexed_keys.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer startKeyIndex;
/**
* The time offset. This is the time since the start of the time interval.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String timeOffset;
/**
* The unit of the metric. This is an unstructured field and will be mapped as is to the user.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private LocalizedString unit;
/**
* The value of the metric.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Float value;
/**
* The list of context values for this key range.
* @return value or {@code null} for none
*/
public java.util.List getContextValues() {
return contextValues;
}
/**
* The list of context values for this key range.
* @param contextValues contextValues or {@code null} for none
*/
public KeyRangeInfo setContextValues(java.util.List contextValues) {
this.contextValues = contextValues;
return this;
}
/**
* The index of the end key in indexed_keys.
* @return value or {@code null} for none
*/
public java.lang.Integer getEndKeyIndex() {
return endKeyIndex;
}
/**
* The index of the end key in indexed_keys.
* @param endKeyIndex endKeyIndex or {@code null} for none
*/
public KeyRangeInfo setEndKeyIndex(java.lang.Integer endKeyIndex) {
this.endKeyIndex = endKeyIndex;
return this;
}
/**
* Information about this key range, for all metrics.
* @return value or {@code null} for none
*/
public LocalizedString getInfo() {
return info;
}
/**
* Information about this key range, for all metrics.
* @param info info or {@code null} for none
*/
public KeyRangeInfo setInfo(LocalizedString info) {
this.info = info;
return this;
}
/**
* The number of keys this range covers.
* @return value or {@code null} for none
*/
public java.lang.Long getKeysCount() {
return keysCount;
}
/**
* The number of keys this range covers.
* @param keysCount keysCount or {@code null} for none
*/
public KeyRangeInfo setKeysCount(java.lang.Long keysCount) {
this.keysCount = keysCount;
return this;
}
/**
* The name of the metric. e.g. "latency".
* @return value or {@code null} for none
*/
public LocalizedString getMetric() {
return metric;
}
/**
* The name of the metric. e.g. "latency".
* @param metric metric or {@code null} for none
*/
public KeyRangeInfo setMetric(LocalizedString metric) {
this.metric = metric;
return this;
}
/**
* The index of the start key in indexed_keys.
* @return value or {@code null} for none
*/
public java.lang.Integer getStartKeyIndex() {
return startKeyIndex;
}
/**
* The index of the start key in indexed_keys.
* @param startKeyIndex startKeyIndex or {@code null} for none
*/
public KeyRangeInfo setStartKeyIndex(java.lang.Integer startKeyIndex) {
this.startKeyIndex = startKeyIndex;
return this;
}
/**
* The time offset. This is the time since the start of the time interval.
* @return value or {@code null} for none
*/
public String getTimeOffset() {
return timeOffset;
}
/**
* The time offset. This is the time since the start of the time interval.
* @param timeOffset timeOffset or {@code null} for none
*/
public KeyRangeInfo setTimeOffset(String timeOffset) {
this.timeOffset = timeOffset;
return this;
}
/**
* The unit of the metric. This is an unstructured field and will be mapped as is to the user.
* @return value or {@code null} for none
*/
public LocalizedString getUnit() {
return unit;
}
/**
* The unit of the metric. This is an unstructured field and will be mapped as is to the user.
* @param unit unit or {@code null} for none
*/
public KeyRangeInfo setUnit(LocalizedString unit) {
this.unit = unit;
return this;
}
/**
* The value of the metric.
* @return value or {@code null} for none
*/
public java.lang.Float getValue() {
return value;
}
/**
* The value of the metric.
* @param value value or {@code null} for none
*/
public KeyRangeInfo setValue(java.lang.Float value) {
this.value = value;
return this;
}
@Override
public KeyRangeInfo set(String fieldName, Object value) {
return (KeyRangeInfo) super.set(fieldName, value);
}
@Override
public KeyRangeInfo clone() {
return (KeyRangeInfo) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy