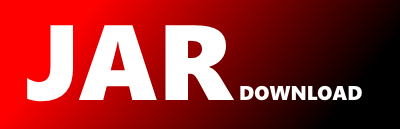
com.google.api.services.spanner.v1.model.Metric Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.spanner.v1.model;
/**
* A message representing the actual monitoring data, values for each key bucket over time, of a
* metric.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Spanner API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Metric extends com.google.api.client.json.GenericJson {
/**
* The aggregation function used to aggregate each key bucket
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String aggregation;
/**
* The category of the metric, e.g. "Activity", "Alerts", "Reads", etc.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private LocalizedString category;
/**
* The references to numerator and denominator metrics for a derived metric.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DerivedMetric derived;
/**
* The displayed label of the metric.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private LocalizedString displayLabel;
/**
* Whether the metric has any non-zero data.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean hasNonzeroData;
/**
* The value that is considered hot for the metric. On a per metric basis hotness signals high
* utilization and something that might potentially be a cause for concern by the end user.
* hot_value is used to calibrate and scale visual color scales.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Float hotValue;
/**
* The (sparse) mapping from time index to an IndexedHotKey message, representing those time
* intervals for which there are hot keys.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map indexedHotKeys;
static {
// hack to force ProGuard to consider IndexedHotKey used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(IndexedHotKey.class);
}
/**
* The (sparse) mapping from time interval index to an IndexedKeyRangeInfos message, representing
* those time intervals for which there are informational messages concerning key ranges.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map indexedKeyRangeInfos;
static {
// hack to force ProGuard to consider IndexedKeyRangeInfos used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(IndexedKeyRangeInfos.class);
}
/**
* Information about the metric.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private LocalizedString info;
/**
* The data for the metric as a matrix.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private MetricMatrix matrix;
/**
* The unit of the metric.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private LocalizedString unit;
/**
* Whether the metric is visible to the end user.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean visible;
/**
* The aggregation function used to aggregate each key bucket
* @return value or {@code null} for none
*/
public java.lang.String getAggregation() {
return aggregation;
}
/**
* The aggregation function used to aggregate each key bucket
* @param aggregation aggregation or {@code null} for none
*/
public Metric setAggregation(java.lang.String aggregation) {
this.aggregation = aggregation;
return this;
}
/**
* The category of the metric, e.g. "Activity", "Alerts", "Reads", etc.
* @return value or {@code null} for none
*/
public LocalizedString getCategory() {
return category;
}
/**
* The category of the metric, e.g. "Activity", "Alerts", "Reads", etc.
* @param category category or {@code null} for none
*/
public Metric setCategory(LocalizedString category) {
this.category = category;
return this;
}
/**
* The references to numerator and denominator metrics for a derived metric.
* @return value or {@code null} for none
*/
public DerivedMetric getDerived() {
return derived;
}
/**
* The references to numerator and denominator metrics for a derived metric.
* @param derived derived or {@code null} for none
*/
public Metric setDerived(DerivedMetric derived) {
this.derived = derived;
return this;
}
/**
* The displayed label of the metric.
* @return value or {@code null} for none
*/
public LocalizedString getDisplayLabel() {
return displayLabel;
}
/**
* The displayed label of the metric.
* @param displayLabel displayLabel or {@code null} for none
*/
public Metric setDisplayLabel(LocalizedString displayLabel) {
this.displayLabel = displayLabel;
return this;
}
/**
* Whether the metric has any non-zero data.
* @return value or {@code null} for none
*/
public java.lang.Boolean getHasNonzeroData() {
return hasNonzeroData;
}
/**
* Whether the metric has any non-zero data.
* @param hasNonzeroData hasNonzeroData or {@code null} for none
*/
public Metric setHasNonzeroData(java.lang.Boolean hasNonzeroData) {
this.hasNonzeroData = hasNonzeroData;
return this;
}
/**
* The value that is considered hot for the metric. On a per metric basis hotness signals high
* utilization and something that might potentially be a cause for concern by the end user.
* hot_value is used to calibrate and scale visual color scales.
* @return value or {@code null} for none
*/
public java.lang.Float getHotValue() {
return hotValue;
}
/**
* The value that is considered hot for the metric. On a per metric basis hotness signals high
* utilization and something that might potentially be a cause for concern by the end user.
* hot_value is used to calibrate and scale visual color scales.
* @param hotValue hotValue or {@code null} for none
*/
public Metric setHotValue(java.lang.Float hotValue) {
this.hotValue = hotValue;
return this;
}
/**
* The (sparse) mapping from time index to an IndexedHotKey message, representing those time
* intervals for which there are hot keys.
* @return value or {@code null} for none
*/
public java.util.Map getIndexedHotKeys() {
return indexedHotKeys;
}
/**
* The (sparse) mapping from time index to an IndexedHotKey message, representing those time
* intervals for which there are hot keys.
* @param indexedHotKeys indexedHotKeys or {@code null} for none
*/
public Metric setIndexedHotKeys(java.util.Map indexedHotKeys) {
this.indexedHotKeys = indexedHotKeys;
return this;
}
/**
* The (sparse) mapping from time interval index to an IndexedKeyRangeInfos message, representing
* those time intervals for which there are informational messages concerning key ranges.
* @return value or {@code null} for none
*/
public java.util.Map getIndexedKeyRangeInfos() {
return indexedKeyRangeInfos;
}
/**
* The (sparse) mapping from time interval index to an IndexedKeyRangeInfos message, representing
* those time intervals for which there are informational messages concerning key ranges.
* @param indexedKeyRangeInfos indexedKeyRangeInfos or {@code null} for none
*/
public Metric setIndexedKeyRangeInfos(java.util.Map indexedKeyRangeInfos) {
this.indexedKeyRangeInfos = indexedKeyRangeInfos;
return this;
}
/**
* Information about the metric.
* @return value or {@code null} for none
*/
public LocalizedString getInfo() {
return info;
}
/**
* Information about the metric.
* @param info info or {@code null} for none
*/
public Metric setInfo(LocalizedString info) {
this.info = info;
return this;
}
/**
* The data for the metric as a matrix.
* @return value or {@code null} for none
*/
public MetricMatrix getMatrix() {
return matrix;
}
/**
* The data for the metric as a matrix.
* @param matrix matrix or {@code null} for none
*/
public Metric setMatrix(MetricMatrix matrix) {
this.matrix = matrix;
return this;
}
/**
* The unit of the metric.
* @return value or {@code null} for none
*/
public LocalizedString getUnit() {
return unit;
}
/**
* The unit of the metric.
* @param unit unit or {@code null} for none
*/
public Metric setUnit(LocalizedString unit) {
this.unit = unit;
return this;
}
/**
* Whether the metric is visible to the end user.
* @return value or {@code null} for none
*/
public java.lang.Boolean getVisible() {
return visible;
}
/**
* Whether the metric is visible to the end user.
* @param visible visible or {@code null} for none
*/
public Metric setVisible(java.lang.Boolean visible) {
this.visible = visible;
return this;
}
@Override
public Metric set(String fieldName, Object value) {
return (Metric) super.set(fieldName, value);
}
@Override
public Metric clone() {
return (Metric) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy