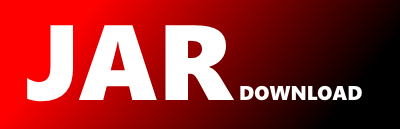
com.google.api.services.spanner.v1.model.PartialResultSet Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.spanner.v1.model;
/**
* Partial results from a streaming read or SQL query. Streaming reads and SQL queries better
* tolerate large result sets, large rows, and large values, but are a little trickier to consume.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Spanner API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class PartialResultSet extends com.google.api.client.json.GenericJson {
/**
* If true, then the final value in values is chunked, and must be combined with more values from
* subsequent `PartialResultSet`s to obtain a complete field value.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean chunkedValue;
/**
* Metadata about the result set, such as row type information. Only present in the first
* response.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ResultSetMetadata metadata;
/**
* Streaming calls might be interrupted for a variety of reasons, such as TCP connection loss. If
* this occurs, the stream of results can be resumed by re-sending the original request and
* including `resume_token`. Note that executing any other transaction in the same session
* invalidates the token.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String resumeToken;
/**
* Query plan and execution statistics for the statement that produced this streaming result set.
* These can be requested by setting ExecuteSqlRequest.query_mode and are sent only once with the
* last response in the stream. This field will also be present in the last response for DML
* statements.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ResultSetStats stats;
/**
* A streamed result set consists of a stream of values, which might be split into many
* `PartialResultSet` messages to accommodate large rows and/or large values. Every N complete
* values defines a row, where N is equal to the number of entries in metadata.row_type.fields.
* Most values are encoded based on type as described here. It is possible that the last value in
* values is "chunked", meaning that the rest of the value is sent in subsequent
* `PartialResultSet`(s). This is denoted by the chunked_value field. Two or more chunked values
* can be merged to form a complete value as follows: * `bool/number/null`: cannot be chunked *
* `string`: concatenate the strings * `list`: concatenate the lists. If the last element in a
* list is a `string`, `list`, or `object`, merge it with the first element in the next list by
* applying these rules recursively. * `object`: concatenate the (field name, field value) pairs.
* If a field name is duplicated, then apply these rules recursively to merge the field values.
* Some examples of merging: # Strings are concatenated. "foo", "bar" => "foobar" # Lists of non-
* strings are concatenated. [2, 3], [4] => [2, 3, 4] # Lists are concatenated, but the last and
* first elements are merged # because they are strings. ["a", "b"], ["c", "d"] => ["a", "bc",
* "d"] # Lists are concatenated, but the last and first elements are merged # because they are
* lists. Recursively, the last and first elements # of the inner lists are merged because they
* are strings. ["a", ["b", "c"]], [["d"], "e"] => ["a", ["b", "cd"], "e"] # Non-overlapping
* object fields are combined. {"a": "1"}, {"b": "2"} => {"a": "1", "b": 2"} # Overlapping object
* fields are merged. {"a": "1"}, {"a": "2"} => {"a": "12"} # Examples of merging objects
* containing lists of strings. {"a": ["1"]}, {"a": ["2"]} => {"a": ["12"]} For a more complete
* example, suppose a streaming SQL query is yielding a result set whose rows contain a single
* string field. The following `PartialResultSet`s might be yielded: { "metadata": { ... }
* "values": ["Hello", "W"] "chunked_value": true "resume_token": "Af65..." } { "values": ["orl"]
* "chunked_value": true } { "values": ["d"] "resume_token": "Zx1B..." } This sequence of
* `PartialResultSet`s encodes two rows, one containing the field value `"Hello"`, and a second
* containing the field value `"World" = "W" + "orl" + "d"`. Not all `PartialResultSet`s contain a
* `resume_token`. Execution can only be resumed from a previously yielded `resume_token`. For the
* above sequence of `PartialResultSet`s, resuming the query with `"resume_token": "Af65..."` will
* yield results from the `PartialResultSet` with value `["orl"]`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List values;
/**
* If true, then the final value in values is chunked, and must be combined with more values from
* subsequent `PartialResultSet`s to obtain a complete field value.
* @return value or {@code null} for none
*/
public java.lang.Boolean getChunkedValue() {
return chunkedValue;
}
/**
* If true, then the final value in values is chunked, and must be combined with more values from
* subsequent `PartialResultSet`s to obtain a complete field value.
* @param chunkedValue chunkedValue or {@code null} for none
*/
public PartialResultSet setChunkedValue(java.lang.Boolean chunkedValue) {
this.chunkedValue = chunkedValue;
return this;
}
/**
* Metadata about the result set, such as row type information. Only present in the first
* response.
* @return value or {@code null} for none
*/
public ResultSetMetadata getMetadata() {
return metadata;
}
/**
* Metadata about the result set, such as row type information. Only present in the first
* response.
* @param metadata metadata or {@code null} for none
*/
public PartialResultSet setMetadata(ResultSetMetadata metadata) {
this.metadata = metadata;
return this;
}
/**
* Streaming calls might be interrupted for a variety of reasons, such as TCP connection loss. If
* this occurs, the stream of results can be resumed by re-sending the original request and
* including `resume_token`. Note that executing any other transaction in the same session
* invalidates the token.
* @see #decodeResumeToken()
* @return value or {@code null} for none
*/
public java.lang.String getResumeToken() {
return resumeToken;
}
/**
* Streaming calls might be interrupted for a variety of reasons, such as TCP connection loss. If
* this occurs, the stream of results can be resumed by re-sending the original request and
* including `resume_token`. Note that executing any other transaction in the same session
* invalidates the token.
* @see #getResumeToken()
* @return Base64 decoded value or {@code null} for none
*
* @since 1.14
*/
public byte[] decodeResumeToken() {
return com.google.api.client.util.Base64.decodeBase64(resumeToken);
}
/**
* Streaming calls might be interrupted for a variety of reasons, such as TCP connection loss. If
* this occurs, the stream of results can be resumed by re-sending the original request and
* including `resume_token`. Note that executing any other transaction in the same session
* invalidates the token.
* @see #encodeResumeToken()
* @param resumeToken resumeToken or {@code null} for none
*/
public PartialResultSet setResumeToken(java.lang.String resumeToken) {
this.resumeToken = resumeToken;
return this;
}
/**
* Streaming calls might be interrupted for a variety of reasons, such as TCP connection loss. If
* this occurs, the stream of results can be resumed by re-sending the original request and
* including `resume_token`. Note that executing any other transaction in the same session
* invalidates the token.
* @see #setResumeToken()
*
*
* The value is encoded Base64 or {@code null} for none.
*
*
* @since 1.14
*/
public PartialResultSet encodeResumeToken(byte[] resumeToken) {
this.resumeToken = com.google.api.client.util.Base64.encodeBase64URLSafeString(resumeToken);
return this;
}
/**
* Query plan and execution statistics for the statement that produced this streaming result set.
* These can be requested by setting ExecuteSqlRequest.query_mode and are sent only once with the
* last response in the stream. This field will also be present in the last response for DML
* statements.
* @return value or {@code null} for none
*/
public ResultSetStats getStats() {
return stats;
}
/**
* Query plan and execution statistics for the statement that produced this streaming result set.
* These can be requested by setting ExecuteSqlRequest.query_mode and are sent only once with the
* last response in the stream. This field will also be present in the last response for DML
* statements.
* @param stats stats or {@code null} for none
*/
public PartialResultSet setStats(ResultSetStats stats) {
this.stats = stats;
return this;
}
/**
* A streamed result set consists of a stream of values, which might be split into many
* `PartialResultSet` messages to accommodate large rows and/or large values. Every N complete
* values defines a row, where N is equal to the number of entries in metadata.row_type.fields.
* Most values are encoded based on type as described here. It is possible that the last value in
* values is "chunked", meaning that the rest of the value is sent in subsequent
* `PartialResultSet`(s). This is denoted by the chunked_value field. Two or more chunked values
* can be merged to form a complete value as follows: * `bool/number/null`: cannot be chunked *
* `string`: concatenate the strings * `list`: concatenate the lists. If the last element in a
* list is a `string`, `list`, or `object`, merge it with the first element in the next list by
* applying these rules recursively. * `object`: concatenate the (field name, field value) pairs.
* If a field name is duplicated, then apply these rules recursively to merge the field values.
* Some examples of merging: # Strings are concatenated. "foo", "bar" => "foobar" # Lists of non-
* strings are concatenated. [2, 3], [4] => [2, 3, 4] # Lists are concatenated, but the last and
* first elements are merged # because they are strings. ["a", "b"], ["c", "d"] => ["a", "bc",
* "d"] # Lists are concatenated, but the last and first elements are merged # because they are
* lists. Recursively, the last and first elements # of the inner lists are merged because they
* are strings. ["a", ["b", "c"]], [["d"], "e"] => ["a", ["b", "cd"], "e"] # Non-overlapping
* object fields are combined. {"a": "1"}, {"b": "2"} => {"a": "1", "b": 2"} # Overlapping object
* fields are merged. {"a": "1"}, {"a": "2"} => {"a": "12"} # Examples of merging objects
* containing lists of strings. {"a": ["1"]}, {"a": ["2"]} => {"a": ["12"]} For a more complete
* example, suppose a streaming SQL query is yielding a result set whose rows contain a single
* string field. The following `PartialResultSet`s might be yielded: { "metadata": { ... }
* "values": ["Hello", "W"] "chunked_value": true "resume_token": "Af65..." } { "values": ["orl"]
* "chunked_value": true } { "values": ["d"] "resume_token": "Zx1B..." } This sequence of
* `PartialResultSet`s encodes two rows, one containing the field value `"Hello"`, and a second
* containing the field value `"World" = "W" + "orl" + "d"`. Not all `PartialResultSet`s contain a
* `resume_token`. Execution can only be resumed from a previously yielded `resume_token`. For the
* above sequence of `PartialResultSet`s, resuming the query with `"resume_token": "Af65..."` will
* yield results from the `PartialResultSet` with value `["orl"]`.
* @return value or {@code null} for none
*/
public java.util.List getValues() {
return values;
}
/**
* A streamed result set consists of a stream of values, which might be split into many
* `PartialResultSet` messages to accommodate large rows and/or large values. Every N complete
* values defines a row, where N is equal to the number of entries in metadata.row_type.fields.
* Most values are encoded based on type as described here. It is possible that the last value in
* values is "chunked", meaning that the rest of the value is sent in subsequent
* `PartialResultSet`(s). This is denoted by the chunked_value field. Two or more chunked values
* can be merged to form a complete value as follows: * `bool/number/null`: cannot be chunked *
* `string`: concatenate the strings * `list`: concatenate the lists. If the last element in a
* list is a `string`, `list`, or `object`, merge it with the first element in the next list by
* applying these rules recursively. * `object`: concatenate the (field name, field value) pairs.
* If a field name is duplicated, then apply these rules recursively to merge the field values.
* Some examples of merging: # Strings are concatenated. "foo", "bar" => "foobar" # Lists of non-
* strings are concatenated. [2, 3], [4] => [2, 3, 4] # Lists are concatenated, but the last and
* first elements are merged # because they are strings. ["a", "b"], ["c", "d"] => ["a", "bc",
* "d"] # Lists are concatenated, but the last and first elements are merged # because they are
* lists. Recursively, the last and first elements # of the inner lists are merged because they
* are strings. ["a", ["b", "c"]], [["d"], "e"] => ["a", ["b", "cd"], "e"] # Non-overlapping
* object fields are combined. {"a": "1"}, {"b": "2"} => {"a": "1", "b": 2"} # Overlapping object
* fields are merged. {"a": "1"}, {"a": "2"} => {"a": "12"} # Examples of merging objects
* containing lists of strings. {"a": ["1"]}, {"a": ["2"]} => {"a": ["12"]} For a more complete
* example, suppose a streaming SQL query is yielding a result set whose rows contain a single
* string field. The following `PartialResultSet`s might be yielded: { "metadata": { ... }
* "values": ["Hello", "W"] "chunked_value": true "resume_token": "Af65..." } { "values": ["orl"]
* "chunked_value": true } { "values": ["d"] "resume_token": "Zx1B..." } This sequence of
* `PartialResultSet`s encodes two rows, one containing the field value `"Hello"`, and a second
* containing the field value `"World" = "W" + "orl" + "d"`. Not all `PartialResultSet`s contain a
* `resume_token`. Execution can only be resumed from a previously yielded `resume_token`. For the
* above sequence of `PartialResultSet`s, resuming the query with `"resume_token": "Af65..."` will
* yield results from the `PartialResultSet` with value `["orl"]`.
* @param values values or {@code null} for none
*/
public PartialResultSet setValues(java.util.List values) {
this.values = values;
return this;
}
@Override
public PartialResultSet set(String fieldName, Object value) {
return (PartialResultSet) super.set(fieldName, value);
}
@Override
public PartialResultSet clone() {
return (PartialResultSet) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy