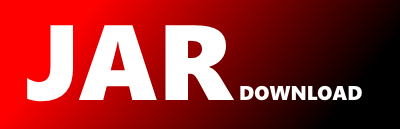
com.google.api.services.spanner.v1.model.UpdateDatabaseDdlMetadata Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.spanner.v1.model;
/**
* Metadata type for the operation returned by UpdateDatabaseDdl.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Spanner API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class UpdateDatabaseDdlMetadata extends com.google.api.client.json.GenericJson {
/**
* The brief action info for the DDL statements. `actions[i]` is the brief info for
* `statements[i]`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List actions;
static {
// hack to force ProGuard to consider DdlStatementActionInfo used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(DdlStatementActionInfo.class);
}
/**
* Reports the commit timestamps of all statements that have succeeded so far, where
* `commit_timestamps[i]` is the commit timestamp for the statement `statements[i]`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List commitTimestamps;
/**
* The database being modified.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String database;
/**
* The progress of the UpdateDatabaseDdl operations. All DDL statements will have continuously
* updating progress, and `progress[i]` is the operation progress for `statements[i]`. Also,
* `progress[i]` will have start time and end time populated with commit timestamp of operation,
* as well as a progress of 100% once the operation has completed.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List progress;
static {
// hack to force ProGuard to consider OperationProgress used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(OperationProgress.class);
}
/**
* For an update this list contains all the statements. For an individual statement, this list
* contains only that statement.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List statements;
/**
* Output only. When true, indicates that the operation is throttled e.g. due to resource
* constraints. When resources become available the operation will resume and this field will be
* false again.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean throttled;
/**
* The brief action info for the DDL statements. `actions[i]` is the brief info for
* `statements[i]`.
* @return value or {@code null} for none
*/
public java.util.List getActions() {
return actions;
}
/**
* The brief action info for the DDL statements. `actions[i]` is the brief info for
* `statements[i]`.
* @param actions actions or {@code null} for none
*/
public UpdateDatabaseDdlMetadata setActions(java.util.List actions) {
this.actions = actions;
return this;
}
/**
* Reports the commit timestamps of all statements that have succeeded so far, where
* `commit_timestamps[i]` is the commit timestamp for the statement `statements[i]`.
* @return value or {@code null} for none
*/
public java.util.List getCommitTimestamps() {
return commitTimestamps;
}
/**
* Reports the commit timestamps of all statements that have succeeded so far, where
* `commit_timestamps[i]` is the commit timestamp for the statement `statements[i]`.
* @param commitTimestamps commitTimestamps or {@code null} for none
*/
public UpdateDatabaseDdlMetadata setCommitTimestamps(java.util.List commitTimestamps) {
this.commitTimestamps = commitTimestamps;
return this;
}
/**
* The database being modified.
* @return value or {@code null} for none
*/
public java.lang.String getDatabase() {
return database;
}
/**
* The database being modified.
* @param database database or {@code null} for none
*/
public UpdateDatabaseDdlMetadata setDatabase(java.lang.String database) {
this.database = database;
return this;
}
/**
* The progress of the UpdateDatabaseDdl operations. All DDL statements will have continuously
* updating progress, and `progress[i]` is the operation progress for `statements[i]`. Also,
* `progress[i]` will have start time and end time populated with commit timestamp of operation,
* as well as a progress of 100% once the operation has completed.
* @return value or {@code null} for none
*/
public java.util.List getProgress() {
return progress;
}
/**
* The progress of the UpdateDatabaseDdl operations. All DDL statements will have continuously
* updating progress, and `progress[i]` is the operation progress for `statements[i]`. Also,
* `progress[i]` will have start time and end time populated with commit timestamp of operation,
* as well as a progress of 100% once the operation has completed.
* @param progress progress or {@code null} for none
*/
public UpdateDatabaseDdlMetadata setProgress(java.util.List progress) {
this.progress = progress;
return this;
}
/**
* For an update this list contains all the statements. For an individual statement, this list
* contains only that statement.
* @return value or {@code null} for none
*/
public java.util.List getStatements() {
return statements;
}
/**
* For an update this list contains all the statements. For an individual statement, this list
* contains only that statement.
* @param statements statements or {@code null} for none
*/
public UpdateDatabaseDdlMetadata setStatements(java.util.List statements) {
this.statements = statements;
return this;
}
/**
* Output only. When true, indicates that the operation is throttled e.g. due to resource
* constraints. When resources become available the operation will resume and this field will be
* false again.
* @return value or {@code null} for none
*/
public java.lang.Boolean getThrottled() {
return throttled;
}
/**
* Output only. When true, indicates that the operation is throttled e.g. due to resource
* constraints. When resources become available the operation will resume and this field will be
* false again.
* @param throttled throttled or {@code null} for none
*/
public UpdateDatabaseDdlMetadata setThrottled(java.lang.Boolean throttled) {
this.throttled = throttled;
return this;
}
@Override
public UpdateDatabaseDdlMetadata set(String fieldName, Object value) {
return (UpdateDatabaseDdlMetadata) super.set(fieldName, value);
}
@Override
public UpdateDatabaseDdlMetadata clone() {
return (UpdateDatabaseDdlMetadata) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy