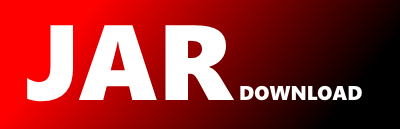
com.google.api.services.spanner.v1.model.VisualizationData Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.spanner.v1.model;
/**
* Model definition for VisualizationData.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Spanner API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class VisualizationData extends com.google.api.client.json.GenericJson {
/**
* The token signifying the end of a data_source.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String dataSourceEndToken;
/**
* The token delimiting a datasource name from the rest of a key in a data_source.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String dataSourceSeparatorToken;
/**
* The list of messages (info, alerts, ...)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List diagnosticMessages;
static {
// hack to force ProGuard to consider DiagnosticMessage used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(DiagnosticMessage.class);
}
/**
* We discretize the entire keyspace into buckets. Assuming each bucket has an inclusive keyrange
* and covers keys from k(i) ... k(n). In this case k(n) would be an end key for a given range.
* end_key_string is the collection of all such end keys
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List endKeyStrings;
/**
* Whether this scan contains PII.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean hasPii;
/**
* Keys of key ranges that contribute significantly to a given metric Can be thought of as heavy
* hitters.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List indexedKeys;
/**
* The token delimiting the key prefixes.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String keySeparator;
/**
* The unit for the key: e.g. 'key' or 'chunk'.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String keyUnit;
/**
* The list of data objects for each metric.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List metrics;
static {
// hack to force ProGuard to consider Metric used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(Metric.class);
}
/**
* The list of extracted key prefix nodes used in the key prefix hierarchy.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List prefixNodes;
static {
// hack to force ProGuard to consider PrefixNode used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(PrefixNode.class);
}
/**
* The token signifying the end of a data_source.
* @return value or {@code null} for none
*/
public java.lang.String getDataSourceEndToken() {
return dataSourceEndToken;
}
/**
* The token signifying the end of a data_source.
* @param dataSourceEndToken dataSourceEndToken or {@code null} for none
*/
public VisualizationData setDataSourceEndToken(java.lang.String dataSourceEndToken) {
this.dataSourceEndToken = dataSourceEndToken;
return this;
}
/**
* The token delimiting a datasource name from the rest of a key in a data_source.
* @return value or {@code null} for none
*/
public java.lang.String getDataSourceSeparatorToken() {
return dataSourceSeparatorToken;
}
/**
* The token delimiting a datasource name from the rest of a key in a data_source.
* @param dataSourceSeparatorToken dataSourceSeparatorToken or {@code null} for none
*/
public VisualizationData setDataSourceSeparatorToken(java.lang.String dataSourceSeparatorToken) {
this.dataSourceSeparatorToken = dataSourceSeparatorToken;
return this;
}
/**
* The list of messages (info, alerts, ...)
* @return value or {@code null} for none
*/
public java.util.List getDiagnosticMessages() {
return diagnosticMessages;
}
/**
* The list of messages (info, alerts, ...)
* @param diagnosticMessages diagnosticMessages or {@code null} for none
*/
public VisualizationData setDiagnosticMessages(java.util.List diagnosticMessages) {
this.diagnosticMessages = diagnosticMessages;
return this;
}
/**
* We discretize the entire keyspace into buckets. Assuming each bucket has an inclusive keyrange
* and covers keys from k(i) ... k(n). In this case k(n) would be an end key for a given range.
* end_key_string is the collection of all such end keys
* @return value or {@code null} for none
*/
public java.util.List getEndKeyStrings() {
return endKeyStrings;
}
/**
* We discretize the entire keyspace into buckets. Assuming each bucket has an inclusive keyrange
* and covers keys from k(i) ... k(n). In this case k(n) would be an end key for a given range.
* end_key_string is the collection of all such end keys
* @param endKeyStrings endKeyStrings or {@code null} for none
*/
public VisualizationData setEndKeyStrings(java.util.List endKeyStrings) {
this.endKeyStrings = endKeyStrings;
return this;
}
/**
* Whether this scan contains PII.
* @return value or {@code null} for none
*/
public java.lang.Boolean getHasPii() {
return hasPii;
}
/**
* Whether this scan contains PII.
* @param hasPii hasPii or {@code null} for none
*/
public VisualizationData setHasPii(java.lang.Boolean hasPii) {
this.hasPii = hasPii;
return this;
}
/**
* Keys of key ranges that contribute significantly to a given metric Can be thought of as heavy
* hitters.
* @return value or {@code null} for none
*/
public java.util.List getIndexedKeys() {
return indexedKeys;
}
/**
* Keys of key ranges that contribute significantly to a given metric Can be thought of as heavy
* hitters.
* @param indexedKeys indexedKeys or {@code null} for none
*/
public VisualizationData setIndexedKeys(java.util.List indexedKeys) {
this.indexedKeys = indexedKeys;
return this;
}
/**
* The token delimiting the key prefixes.
* @return value or {@code null} for none
*/
public java.lang.String getKeySeparator() {
return keySeparator;
}
/**
* The token delimiting the key prefixes.
* @param keySeparator keySeparator or {@code null} for none
*/
public VisualizationData setKeySeparator(java.lang.String keySeparator) {
this.keySeparator = keySeparator;
return this;
}
/**
* The unit for the key: e.g. 'key' or 'chunk'.
* @return value or {@code null} for none
*/
public java.lang.String getKeyUnit() {
return keyUnit;
}
/**
* The unit for the key: e.g. 'key' or 'chunk'.
* @param keyUnit keyUnit or {@code null} for none
*/
public VisualizationData setKeyUnit(java.lang.String keyUnit) {
this.keyUnit = keyUnit;
return this;
}
/**
* The list of data objects for each metric.
* @return value or {@code null} for none
*/
public java.util.List getMetrics() {
return metrics;
}
/**
* The list of data objects for each metric.
* @param metrics metrics or {@code null} for none
*/
public VisualizationData setMetrics(java.util.List metrics) {
this.metrics = metrics;
return this;
}
/**
* The list of extracted key prefix nodes used in the key prefix hierarchy.
* @return value or {@code null} for none
*/
public java.util.List getPrefixNodes() {
return prefixNodes;
}
/**
* The list of extracted key prefix nodes used in the key prefix hierarchy.
* @param prefixNodes prefixNodes or {@code null} for none
*/
public VisualizationData setPrefixNodes(java.util.List prefixNodes) {
this.prefixNodes = prefixNodes;
return this;
}
@Override
public VisualizationData set(String fieldName, Object value) {
return (VisualizationData) super.set(fieldName, value);
}
@Override
public VisualizationData clone() {
return (VisualizationData) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy