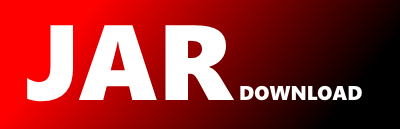
com.google.api.services.spanner.v1.model.Database Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.spanner.v1.model;
/**
* A Cloud Spanner database.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Spanner API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Database extends com.google.api.client.json.GenericJson {
/**
* Output only. If exists, the time at which the database creation started.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String createTime;
/**
* Output only. The dialect of the Cloud Spanner Database.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String databaseDialect;
/**
* Output only. The read-write region which contains the database's leader replicas. This is the
* same as the value of default_leader database option set using DatabaseAdmin.CreateDatabase or
* DatabaseAdmin.UpdateDatabaseDdl. If not explicitly set, this is empty.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String defaultLeader;
/**
* Output only. Earliest timestamp at which older versions of the data can be read. This value is
* continuously updated by Cloud Spanner and becomes stale the moment it is queried. If you are
* using this value to recover data, make sure to account for the time from the moment when the
* value is queried to the moment when you initiate the recovery.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String earliestVersionTime;
/**
* Optional. Whether drop protection is enabled for this database. Defaults to false, if not set.
* For more details, please see how to [prevent accidental database
* deletion](https://cloud.google.com/spanner/docs/prevent-database-deletion).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean enableDropProtection;
/**
* Output only. For databases that are using customer managed encryption, this field contains the
* encryption configuration for the database. For databases that are using Google default or other
* types of encryption, this field is empty.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private EncryptionConfig encryptionConfig;
/**
* Output only. For databases that are using customer managed encryption, this field contains the
* encryption information for the database, such as all Cloud KMS key versions that are in use.
* The `encryption_status' field inside of each `EncryptionInfo` is not populated. For databases
* that are using Google default or other types of encryption, this field is empty. This field is
* propagated lazily from the backend. There might be a delay from when a key version is being
* used and when it appears in this field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List encryptionInfo;
/**
* Required. The name of the database. Values are of the form `projects//instances//databases/`,
* where `` is as specified in the `CREATE DATABASE` statement. This name can be passed to other
* API methods to identify the database.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Output only. Applicable only for databases that use dual-region instance configurations.
* Contains information about the quorum.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private QuorumInfo quorumInfo;
/**
* Output only. If true, the database is being updated. If false, there are no ongoing update
* operations for the database.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean reconciling;
/**
* Output only. Applicable only for restored databases. Contains information about the restore
* source.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private RestoreInfo restoreInfo;
/**
* Output only. The current database state.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String state;
/**
* Output only. The period in which Cloud Spanner retains all versions of data for the database.
* This is the same as the value of version_retention_period database option set using
* UpdateDatabaseDdl. Defaults to 1 hour, if not set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String versionRetentionPeriod;
/**
* Output only. If exists, the time at which the database creation started.
* @return value or {@code null} for none
*/
public String getCreateTime() {
return createTime;
}
/**
* Output only. If exists, the time at which the database creation started.
* @param createTime createTime or {@code null} for none
*/
public Database setCreateTime(String createTime) {
this.createTime = createTime;
return this;
}
/**
* Output only. The dialect of the Cloud Spanner Database.
* @return value or {@code null} for none
*/
public java.lang.String getDatabaseDialect() {
return databaseDialect;
}
/**
* Output only. The dialect of the Cloud Spanner Database.
* @param databaseDialect databaseDialect or {@code null} for none
*/
public Database setDatabaseDialect(java.lang.String databaseDialect) {
this.databaseDialect = databaseDialect;
return this;
}
/**
* Output only. The read-write region which contains the database's leader replicas. This is the
* same as the value of default_leader database option set using DatabaseAdmin.CreateDatabase or
* DatabaseAdmin.UpdateDatabaseDdl. If not explicitly set, this is empty.
* @return value or {@code null} for none
*/
public java.lang.String getDefaultLeader() {
return defaultLeader;
}
/**
* Output only. The read-write region which contains the database's leader replicas. This is the
* same as the value of default_leader database option set using DatabaseAdmin.CreateDatabase or
* DatabaseAdmin.UpdateDatabaseDdl. If not explicitly set, this is empty.
* @param defaultLeader defaultLeader or {@code null} for none
*/
public Database setDefaultLeader(java.lang.String defaultLeader) {
this.defaultLeader = defaultLeader;
return this;
}
/**
* Output only. Earliest timestamp at which older versions of the data can be read. This value is
* continuously updated by Cloud Spanner and becomes stale the moment it is queried. If you are
* using this value to recover data, make sure to account for the time from the moment when the
* value is queried to the moment when you initiate the recovery.
* @return value or {@code null} for none
*/
public String getEarliestVersionTime() {
return earliestVersionTime;
}
/**
* Output only. Earliest timestamp at which older versions of the data can be read. This value is
* continuously updated by Cloud Spanner and becomes stale the moment it is queried. If you are
* using this value to recover data, make sure to account for the time from the moment when the
* value is queried to the moment when you initiate the recovery.
* @param earliestVersionTime earliestVersionTime or {@code null} for none
*/
public Database setEarliestVersionTime(String earliestVersionTime) {
this.earliestVersionTime = earliestVersionTime;
return this;
}
/**
* Optional. Whether drop protection is enabled for this database. Defaults to false, if not set.
* For more details, please see how to [prevent accidental database
* deletion](https://cloud.google.com/spanner/docs/prevent-database-deletion).
* @return value or {@code null} for none
*/
public java.lang.Boolean getEnableDropProtection() {
return enableDropProtection;
}
/**
* Optional. Whether drop protection is enabled for this database. Defaults to false, if not set.
* For more details, please see how to [prevent accidental database
* deletion](https://cloud.google.com/spanner/docs/prevent-database-deletion).
* @param enableDropProtection enableDropProtection or {@code null} for none
*/
public Database setEnableDropProtection(java.lang.Boolean enableDropProtection) {
this.enableDropProtection = enableDropProtection;
return this;
}
/**
* Output only. For databases that are using customer managed encryption, this field contains the
* encryption configuration for the database. For databases that are using Google default or other
* types of encryption, this field is empty.
* @return value or {@code null} for none
*/
public EncryptionConfig getEncryptionConfig() {
return encryptionConfig;
}
/**
* Output only. For databases that are using customer managed encryption, this field contains the
* encryption configuration for the database. For databases that are using Google default or other
* types of encryption, this field is empty.
* @param encryptionConfig encryptionConfig or {@code null} for none
*/
public Database setEncryptionConfig(EncryptionConfig encryptionConfig) {
this.encryptionConfig = encryptionConfig;
return this;
}
/**
* Output only. For databases that are using customer managed encryption, this field contains the
* encryption information for the database, such as all Cloud KMS key versions that are in use.
* The `encryption_status' field inside of each `EncryptionInfo` is not populated. For databases
* that are using Google default or other types of encryption, this field is empty. This field is
* propagated lazily from the backend. There might be a delay from when a key version is being
* used and when it appears in this field.
* @return value or {@code null} for none
*/
public java.util.List getEncryptionInfo() {
return encryptionInfo;
}
/**
* Output only. For databases that are using customer managed encryption, this field contains the
* encryption information for the database, such as all Cloud KMS key versions that are in use.
* The `encryption_status' field inside of each `EncryptionInfo` is not populated. For databases
* that are using Google default or other types of encryption, this field is empty. This field is
* propagated lazily from the backend. There might be a delay from when a key version is being
* used and when it appears in this field.
* @param encryptionInfo encryptionInfo or {@code null} for none
*/
public Database setEncryptionInfo(java.util.List encryptionInfo) {
this.encryptionInfo = encryptionInfo;
return this;
}
/**
* Required. The name of the database. Values are of the form `projects//instances//databases/`,
* where `` is as specified in the `CREATE DATABASE` statement. This name can be passed to other
* API methods to identify the database.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the database. Values are of the form `projects//instances//databases/`,
* where `` is as specified in the `CREATE DATABASE` statement. This name can be passed to other
* API methods to identify the database.
* @param name name or {@code null} for none
*/
public Database setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Output only. Applicable only for databases that use dual-region instance configurations.
* Contains information about the quorum.
* @return value or {@code null} for none
*/
public QuorumInfo getQuorumInfo() {
return quorumInfo;
}
/**
* Output only. Applicable only for databases that use dual-region instance configurations.
* Contains information about the quorum.
* @param quorumInfo quorumInfo or {@code null} for none
*/
public Database setQuorumInfo(QuorumInfo quorumInfo) {
this.quorumInfo = quorumInfo;
return this;
}
/**
* Output only. If true, the database is being updated. If false, there are no ongoing update
* operations for the database.
* @return value or {@code null} for none
*/
public java.lang.Boolean getReconciling() {
return reconciling;
}
/**
* Output only. If true, the database is being updated. If false, there are no ongoing update
* operations for the database.
* @param reconciling reconciling or {@code null} for none
*/
public Database setReconciling(java.lang.Boolean reconciling) {
this.reconciling = reconciling;
return this;
}
/**
* Output only. Applicable only for restored databases. Contains information about the restore
* source.
* @return value or {@code null} for none
*/
public RestoreInfo getRestoreInfo() {
return restoreInfo;
}
/**
* Output only. Applicable only for restored databases. Contains information about the restore
* source.
* @param restoreInfo restoreInfo or {@code null} for none
*/
public Database setRestoreInfo(RestoreInfo restoreInfo) {
this.restoreInfo = restoreInfo;
return this;
}
/**
* Output only. The current database state.
* @return value or {@code null} for none
*/
public java.lang.String getState() {
return state;
}
/**
* Output only. The current database state.
* @param state state or {@code null} for none
*/
public Database setState(java.lang.String state) {
this.state = state;
return this;
}
/**
* Output only. The period in which Cloud Spanner retains all versions of data for the database.
* This is the same as the value of version_retention_period database option set using
* UpdateDatabaseDdl. Defaults to 1 hour, if not set.
* @return value or {@code null} for none
*/
public java.lang.String getVersionRetentionPeriod() {
return versionRetentionPeriod;
}
/**
* Output only. The period in which Cloud Spanner retains all versions of data for the database.
* This is the same as the value of version_retention_period database option set using
* UpdateDatabaseDdl. Defaults to 1 hour, if not set.
* @param versionRetentionPeriod versionRetentionPeriod or {@code null} for none
*/
public Database setVersionRetentionPeriod(java.lang.String versionRetentionPeriod) {
this.versionRetentionPeriod = versionRetentionPeriod;
return this;
}
@Override
public Database set(String fieldName, Object value) {
return (Database) super.set(fieldName, value);
}
@Override
public Database clone() {
return (Database) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy