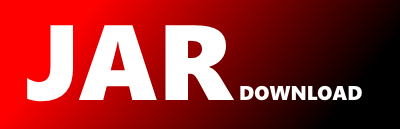
com.google.api.services.spanner.v1.model.RestoreDatabaseEncryptionConfig Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.spanner.v1.model;
/**
* Encryption configuration for the restored database.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Spanner API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class RestoreDatabaseEncryptionConfig extends com.google.api.client.json.GenericJson {
/**
* Required. The encryption type of the restored database.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String encryptionType;
/**
* Optional. The Cloud KMS key that will be used to encrypt/decrypt the restored database. This
* field should be set only when encryption_type is `CUSTOMER_MANAGED_ENCRYPTION`. Values are of
* the form `projects//locations//keyRings//cryptoKeys/`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kmsKeyName;
/**
* Optional. Specifies the KMS configuration for the one or more keys used to encrypt the
* database. Values have the form `projects//locations//keyRings//cryptoKeys/`. The keys
* referenced by kms_key_names must fully cover all regions of the database instance
* configuration. Some examples: * For single region database instance configurations, specify a
* single regional location KMS key. * For multi-regional database instance configurations of type
* `GOOGLE_MANAGED`, either specify a multi-regional location KMS key or multiple regional
* location KMS keys that cover all regions in the instance configuration. * For a database
* instance configuration of type `USER_MANAGED`, please specify only regional location KMS keys
* to cover each region in the instance configuration. Multi-regional location KMS keys are not
* supported for USER_MANAGED instance configurations.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List kmsKeyNames;
/**
* Required. The encryption type of the restored database.
* @return value or {@code null} for none
*/
public java.lang.String getEncryptionType() {
return encryptionType;
}
/**
* Required. The encryption type of the restored database.
* @param encryptionType encryptionType or {@code null} for none
*/
public RestoreDatabaseEncryptionConfig setEncryptionType(java.lang.String encryptionType) {
this.encryptionType = encryptionType;
return this;
}
/**
* Optional. The Cloud KMS key that will be used to encrypt/decrypt the restored database. This
* field should be set only when encryption_type is `CUSTOMER_MANAGED_ENCRYPTION`. Values are of
* the form `projects//locations//keyRings//cryptoKeys/`.
* @return value or {@code null} for none
*/
public java.lang.String getKmsKeyName() {
return kmsKeyName;
}
/**
* Optional. The Cloud KMS key that will be used to encrypt/decrypt the restored database. This
* field should be set only when encryption_type is `CUSTOMER_MANAGED_ENCRYPTION`. Values are of
* the form `projects//locations//keyRings//cryptoKeys/`.
* @param kmsKeyName kmsKeyName or {@code null} for none
*/
public RestoreDatabaseEncryptionConfig setKmsKeyName(java.lang.String kmsKeyName) {
this.kmsKeyName = kmsKeyName;
return this;
}
/**
* Optional. Specifies the KMS configuration for the one or more keys used to encrypt the
* database. Values have the form `projects//locations//keyRings//cryptoKeys/`. The keys
* referenced by kms_key_names must fully cover all regions of the database instance
* configuration. Some examples: * For single region database instance configurations, specify a
* single regional location KMS key. * For multi-regional database instance configurations of type
* `GOOGLE_MANAGED`, either specify a multi-regional location KMS key or multiple regional
* location KMS keys that cover all regions in the instance configuration. * For a database
* instance configuration of type `USER_MANAGED`, please specify only regional location KMS keys
* to cover each region in the instance configuration. Multi-regional location KMS keys are not
* supported for USER_MANAGED instance configurations.
* @return value or {@code null} for none
*/
public java.util.List getKmsKeyNames() {
return kmsKeyNames;
}
/**
* Optional. Specifies the KMS configuration for the one or more keys used to encrypt the
* database. Values have the form `projects//locations//keyRings//cryptoKeys/`. The keys
* referenced by kms_key_names must fully cover all regions of the database instance
* configuration. Some examples: * For single region database instance configurations, specify a
* single regional location KMS key. * For multi-regional database instance configurations of type
* `GOOGLE_MANAGED`, either specify a multi-regional location KMS key or multiple regional
* location KMS keys that cover all regions in the instance configuration. * For a database
* instance configuration of type `USER_MANAGED`, please specify only regional location KMS keys
* to cover each region in the instance configuration. Multi-regional location KMS keys are not
* supported for USER_MANAGED instance configurations.
* @param kmsKeyNames kmsKeyNames or {@code null} for none
*/
public RestoreDatabaseEncryptionConfig setKmsKeyNames(java.util.List kmsKeyNames) {
this.kmsKeyNames = kmsKeyNames;
return this;
}
@Override
public RestoreDatabaseEncryptionConfig set(String fieldName, Object value) {
return (RestoreDatabaseEncryptionConfig) super.set(fieldName, value);
}
@Override
public RestoreDatabaseEncryptionConfig clone() {
return (RestoreDatabaseEncryptionConfig) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy