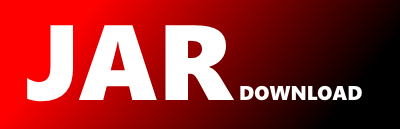
com.google.api.services.spectrum.model.DeviceDescriptor Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-05-04 15:59:39 UTC)
* on 2016-05-18 at 06:28:13 UTC
* Modify at your own risk.
*/
package com.google.api.services.spectrum.model;
/**
* The device descriptor contains parameters that identify the specific device, such as its
* manufacturer serial number, regulatory-specific identifier (e.g., FCC ID), and any other device
* characteristics required by regulatory domains.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Spectrum Database API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class DeviceDescriptor extends com.google.api.client.json.GenericJson {
/**
* Specifies the ETSI white space device category. Valid values are the strings master and slave.
* This field is case-insensitive. Consult the ETSI documentation for details about the device
* types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String etsiEnDeviceCategory;
/**
* Specifies the ETSI white space device emissions class. The values are represented by numeric
* strings, such as 1, 2, etc. Consult the ETSI documentation for details about the device types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String etsiEnDeviceEmissionsClass;
/**
* Specifies the ETSI white space device type. Valid values are single-letter strings, such as A,
* B, etc. Consult the ETSI documentation for details about the device types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String etsiEnDeviceType;
/**
* Specifies the ETSI white space device technology identifier. The string value must not exceed
* 64 characters in length. Consult the ETSI documentation for details about the device types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String etsiEnTechnologyId;
/**
* Specifies the device's FCC certification identifier. The value is an identifier string whose
* length should not exceed 32 characters. Note that, in practice, a valid FCC ID may be limited
* to 19 characters.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String fccId;
/**
* Specifies the TV Band White Space device type, as defined by the FCC. Valid values are FIXED,
* MODE_1, MODE_2.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String fccTvbdDeviceType;
/**
* The manufacturer's ID may be required by the regulatory domain. This should represent the name
* of the device manufacturer, should be consistent across all devices from the same manufacturer,
* and should be distinct from that of other manufacturers. The string value must not exceed 64
* characters in length.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String manufacturerId;
/**
* The device's model ID may be required by the regulatory domain. The string value must not
* exceed 64 characters in length.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String modelId;
/**
* The list of identifiers for rulesets supported by the device. A database may require that the
* device provide this list before servicing the device requests. If the database does not support
* any of the rulesets specified in the list, the database may refuse to service the device
* requests. If present, the list must contain at least one entry.
*
* For information about the valid requests, see section 9.2 of the PAWS specification. Currently,
* FccTvBandWhiteSpace-2010 is the only supported ruleset.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List rulesetIds;
/**
* The manufacturer's device serial number; required by the applicable regulatory domain. The
* length of the value must not exceed 64 characters.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String serialNumber;
/**
* Specifies the ETSI white space device category. Valid values are the strings master and slave.
* This field is case-insensitive. Consult the ETSI documentation for details about the device
* types.
* @return value or {@code null} for none
*/
public java.lang.String getEtsiEnDeviceCategory() {
return etsiEnDeviceCategory;
}
/**
* Specifies the ETSI white space device category. Valid values are the strings master and slave.
* This field is case-insensitive. Consult the ETSI documentation for details about the device
* types.
* @param etsiEnDeviceCategory etsiEnDeviceCategory or {@code null} for none
*/
public DeviceDescriptor setEtsiEnDeviceCategory(java.lang.String etsiEnDeviceCategory) {
this.etsiEnDeviceCategory = etsiEnDeviceCategory;
return this;
}
/**
* Specifies the ETSI white space device emissions class. The values are represented by numeric
* strings, such as 1, 2, etc. Consult the ETSI documentation for details about the device types.
* @return value or {@code null} for none
*/
public java.lang.String getEtsiEnDeviceEmissionsClass() {
return etsiEnDeviceEmissionsClass;
}
/**
* Specifies the ETSI white space device emissions class. The values are represented by numeric
* strings, such as 1, 2, etc. Consult the ETSI documentation for details about the device types.
* @param etsiEnDeviceEmissionsClass etsiEnDeviceEmissionsClass or {@code null} for none
*/
public DeviceDescriptor setEtsiEnDeviceEmissionsClass(java.lang.String etsiEnDeviceEmissionsClass) {
this.etsiEnDeviceEmissionsClass = etsiEnDeviceEmissionsClass;
return this;
}
/**
* Specifies the ETSI white space device type. Valid values are single-letter strings, such as A,
* B, etc. Consult the ETSI documentation for details about the device types.
* @return value or {@code null} for none
*/
public java.lang.String getEtsiEnDeviceType() {
return etsiEnDeviceType;
}
/**
* Specifies the ETSI white space device type. Valid values are single-letter strings, such as A,
* B, etc. Consult the ETSI documentation for details about the device types.
* @param etsiEnDeviceType etsiEnDeviceType or {@code null} for none
*/
public DeviceDescriptor setEtsiEnDeviceType(java.lang.String etsiEnDeviceType) {
this.etsiEnDeviceType = etsiEnDeviceType;
return this;
}
/**
* Specifies the ETSI white space device technology identifier. The string value must not exceed
* 64 characters in length. Consult the ETSI documentation for details about the device types.
* @return value or {@code null} for none
*/
public java.lang.String getEtsiEnTechnologyId() {
return etsiEnTechnologyId;
}
/**
* Specifies the ETSI white space device technology identifier. The string value must not exceed
* 64 characters in length. Consult the ETSI documentation for details about the device types.
* @param etsiEnTechnologyId etsiEnTechnologyId or {@code null} for none
*/
public DeviceDescriptor setEtsiEnTechnologyId(java.lang.String etsiEnTechnologyId) {
this.etsiEnTechnologyId = etsiEnTechnologyId;
return this;
}
/**
* Specifies the device's FCC certification identifier. The value is an identifier string whose
* length should not exceed 32 characters. Note that, in practice, a valid FCC ID may be limited
* to 19 characters.
* @return value or {@code null} for none
*/
public java.lang.String getFccId() {
return fccId;
}
/**
* Specifies the device's FCC certification identifier. The value is an identifier string whose
* length should not exceed 32 characters. Note that, in practice, a valid FCC ID may be limited
* to 19 characters.
* @param fccId fccId or {@code null} for none
*/
public DeviceDescriptor setFccId(java.lang.String fccId) {
this.fccId = fccId;
return this;
}
/**
* Specifies the TV Band White Space device type, as defined by the FCC. Valid values are FIXED,
* MODE_1, MODE_2.
* @return value or {@code null} for none
*/
public java.lang.String getFccTvbdDeviceType() {
return fccTvbdDeviceType;
}
/**
* Specifies the TV Band White Space device type, as defined by the FCC. Valid values are FIXED,
* MODE_1, MODE_2.
* @param fccTvbdDeviceType fccTvbdDeviceType or {@code null} for none
*/
public DeviceDescriptor setFccTvbdDeviceType(java.lang.String fccTvbdDeviceType) {
this.fccTvbdDeviceType = fccTvbdDeviceType;
return this;
}
/**
* The manufacturer's ID may be required by the regulatory domain. This should represent the name
* of the device manufacturer, should be consistent across all devices from the same manufacturer,
* and should be distinct from that of other manufacturers. The string value must not exceed 64
* characters in length.
* @return value or {@code null} for none
*/
public java.lang.String getManufacturerId() {
return manufacturerId;
}
/**
* The manufacturer's ID may be required by the regulatory domain. This should represent the name
* of the device manufacturer, should be consistent across all devices from the same manufacturer,
* and should be distinct from that of other manufacturers. The string value must not exceed 64
* characters in length.
* @param manufacturerId manufacturerId or {@code null} for none
*/
public DeviceDescriptor setManufacturerId(java.lang.String manufacturerId) {
this.manufacturerId = manufacturerId;
return this;
}
/**
* The device's model ID may be required by the regulatory domain. The string value must not
* exceed 64 characters in length.
* @return value or {@code null} for none
*/
public java.lang.String getModelId() {
return modelId;
}
/**
* The device's model ID may be required by the regulatory domain. The string value must not
* exceed 64 characters in length.
* @param modelId modelId or {@code null} for none
*/
public DeviceDescriptor setModelId(java.lang.String modelId) {
this.modelId = modelId;
return this;
}
/**
* The list of identifiers for rulesets supported by the device. A database may require that the
* device provide this list before servicing the device requests. If the database does not support
* any of the rulesets specified in the list, the database may refuse to service the device
* requests. If present, the list must contain at least one entry.
*
* For information about the valid requests, see section 9.2 of the PAWS specification. Currently,
* FccTvBandWhiteSpace-2010 is the only supported ruleset.
* @return value or {@code null} for none
*/
public java.util.List getRulesetIds() {
return rulesetIds;
}
/**
* The list of identifiers for rulesets supported by the device. A database may require that the
* device provide this list before servicing the device requests. If the database does not support
* any of the rulesets specified in the list, the database may refuse to service the device
* requests. If present, the list must contain at least one entry.
*
* For information about the valid requests, see section 9.2 of the PAWS specification. Currently,
* FccTvBandWhiteSpace-2010 is the only supported ruleset.
* @param rulesetIds rulesetIds or {@code null} for none
*/
public DeviceDescriptor setRulesetIds(java.util.List rulesetIds) {
this.rulesetIds = rulesetIds;
return this;
}
/**
* The manufacturer's device serial number; required by the applicable regulatory domain. The
* length of the value must not exceed 64 characters.
* @return value or {@code null} for none
*/
public java.lang.String getSerialNumber() {
return serialNumber;
}
/**
* The manufacturer's device serial number; required by the applicable regulatory domain. The
* length of the value must not exceed 64 characters.
* @param serialNumber serialNumber or {@code null} for none
*/
public DeviceDescriptor setSerialNumber(java.lang.String serialNumber) {
this.serialNumber = serialNumber;
return this;
}
@Override
public DeviceDescriptor set(String fieldName, Object value) {
return (DeviceDescriptor) super.set(fieldName, value);
}
@Override
public DeviceDescriptor clone() {
return (DeviceDescriptor) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy