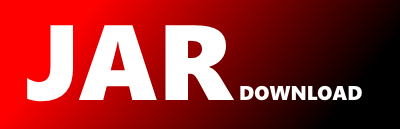
com.google.api.services.spectrum.model.GeoLocation Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-05-04 15:59:39 UTC)
* on 2016-05-18 at 06:28:13 UTC
* Modify at your own risk.
*/
package com.google.api.services.spectrum.model;
/**
* This parameter is used to specify the geolocation of the device.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Spectrum Database API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GeoLocation extends com.google.api.client.json.GenericJson {
/**
* The location confidence level, as an integer percentage, may be required, depending on the
* regulatory domain. When the parameter is optional and not provided, its value is assumed to be
* 95. Valid values range from 0 to 99, since, in practice, 100-percent confidence is not
* achievable. The confidence value is meaningful only when geolocation refers to a point with
* uncertainty.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer confidence;
/**
* If present, indicates that the geolocation represents a point. Paradoxically, a point is
* parameterized using an ellipse, where the center represents the location of the point and the
* distances along the major and minor axes represent the uncertainty. The uncertainty values may
* be required, depending on the regulatory domain.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GeoLocationEllipse point;
/**
* If present, indicates that the geolocation represents a region. Database support for regions is
* optional.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GeoLocationPolygon region;
/**
* The location confidence level, as an integer percentage, may be required, depending on the
* regulatory domain. When the parameter is optional and not provided, its value is assumed to be
* 95. Valid values range from 0 to 99, since, in practice, 100-percent confidence is not
* achievable. The confidence value is meaningful only when geolocation refers to a point with
* uncertainty.
* @return value or {@code null} for none
*/
public java.lang.Integer getConfidence() {
return confidence;
}
/**
* The location confidence level, as an integer percentage, may be required, depending on the
* regulatory domain. When the parameter is optional and not provided, its value is assumed to be
* 95. Valid values range from 0 to 99, since, in practice, 100-percent confidence is not
* achievable. The confidence value is meaningful only when geolocation refers to a point with
* uncertainty.
* @param confidence confidence or {@code null} for none
*/
public GeoLocation setConfidence(java.lang.Integer confidence) {
this.confidence = confidence;
return this;
}
/**
* If present, indicates that the geolocation represents a point. Paradoxically, a point is
* parameterized using an ellipse, where the center represents the location of the point and the
* distances along the major and minor axes represent the uncertainty. The uncertainty values may
* be required, depending on the regulatory domain.
* @return value or {@code null} for none
*/
public GeoLocationEllipse getPoint() {
return point;
}
/**
* If present, indicates that the geolocation represents a point. Paradoxically, a point is
* parameterized using an ellipse, where the center represents the location of the point and the
* distances along the major and minor axes represent the uncertainty. The uncertainty values may
* be required, depending on the regulatory domain.
* @param point point or {@code null} for none
*/
public GeoLocation setPoint(GeoLocationEllipse point) {
this.point = point;
return this;
}
/**
* If present, indicates that the geolocation represents a region. Database support for regions is
* optional.
* @return value or {@code null} for none
*/
public GeoLocationPolygon getRegion() {
return region;
}
/**
* If present, indicates that the geolocation represents a region. Database support for regions is
* optional.
* @param region region or {@code null} for none
*/
public GeoLocation setRegion(GeoLocationPolygon region) {
this.region = region;
return this;
}
@Override
public GeoLocation set(String fieldName, Object value) {
return (GeoLocation) super.set(fieldName, value);
}
@Override
public GeoLocation clone() {
return (GeoLocation) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy