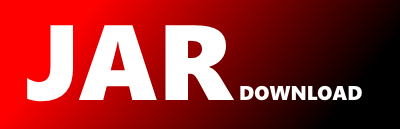
com.google.api.services.spectrum.model.PawsGetSpectrumBatchRequest Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-05-04 15:59:39 UTC)
* on 2016-05-18 at 06:28:13 UTC
* Modify at your own risk.
*/
package com.google.api.services.spectrum.model;
/**
* The request message for a batch available spectrum query protocol.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Spectrum Database API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class PawsGetSpectrumBatchRequest extends com.google.api.client.json.GenericJson {
/**
* Depending on device type and regulatory domain, antenna characteristics may be required.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AntennaCharacteristics antenna;
/**
* The master device may include its device capabilities to limit the available-spectrum batch
* response to the spectrum that is compatible with its capabilities. The database should not
* return spectrum that is incompatible with the specified capabilities.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DeviceCapabilities capabilities;
/**
* When the available spectrum request is made on behalf of a specific device (a master or slave
* device), device descriptor information for the device on whose behalf the request is made is
* required (in such cases, the requestType parameter must be empty). When a requestType value is
* specified, device descriptor information may be optional or required according to the rules of
* the applicable regulatory domain.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DeviceDescriptor deviceDesc;
/**
* A geolocation list is required. This allows a device to specify its current location plus
* additional anticipated locations when allowed by the regulatory domain. At least one location
* must be included. Geolocation must be given as the location of the radiation center of the
* device's antenna. If a location specifies a region, rather than a point, the database may
* return an UNIMPLEMENTED error if it does not support query by region.
*
* There is no upper limit on the number of locations included in a available spectrum batch
* request, but the database may restrict the number of locations it supports by returning a
* response with fewer locations than specified in the batch request. Note that geolocations must
* be those of the master device (a device with geolocation capability that makes an available
* spectrum batch request), whether the master device is making the request on its own behalf or
* on behalf of a slave device (one without geolocation capability).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List locations;
static {
// hack to force ProGuard to consider GeoLocation used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GeoLocation.class);
}
/**
* When an available spectrum batch request is made by the master device (a device with
* geolocation capability) on behalf of a slave device (a device without geolocation capability),
* the rules of the applicable regulatory domain may require the master device to provide its own
* device descriptor information (in addition to device descriptor information for the slave
* device in a separate parameter).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DeviceDescriptor masterDeviceDesc;
/**
* Depending on device type and regulatory domain, device owner information may be included in an
* available spectrum batch request. This allows the device to register and get spectrum-
* availability information in a single request.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DeviceOwner owner;
/**
* The request type parameter is an optional parameter that can be used to modify an available
* spectrum batch request, but its use depends on applicable regulatory rules. For example, It may
* be used to request generic slave device parameters without having to specify the device
* descriptor for a specific device. When the requestType parameter is missing, the request is for
* a specific device (master or slave), and the device descriptor parameter for the device on
* whose behalf the batch request is made is required.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String requestType;
/**
* The message type (e.g., INIT_REQ, AVAIL_SPECTRUM_REQ, ...).
*
* Required field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* The PAWS version. Must be exactly 1.0.
*
* Required field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String version;
/**
* Depending on device type and regulatory domain, antenna characteristics may be required.
* @return value or {@code null} for none
*/
public AntennaCharacteristics getAntenna() {
return antenna;
}
/**
* Depending on device type and regulatory domain, antenna characteristics may be required.
* @param antenna antenna or {@code null} for none
*/
public PawsGetSpectrumBatchRequest setAntenna(AntennaCharacteristics antenna) {
this.antenna = antenna;
return this;
}
/**
* The master device may include its device capabilities to limit the available-spectrum batch
* response to the spectrum that is compatible with its capabilities. The database should not
* return spectrum that is incompatible with the specified capabilities.
* @return value or {@code null} for none
*/
public DeviceCapabilities getCapabilities() {
return capabilities;
}
/**
* The master device may include its device capabilities to limit the available-spectrum batch
* response to the spectrum that is compatible with its capabilities. The database should not
* return spectrum that is incompatible with the specified capabilities.
* @param capabilities capabilities or {@code null} for none
*/
public PawsGetSpectrumBatchRequest setCapabilities(DeviceCapabilities capabilities) {
this.capabilities = capabilities;
return this;
}
/**
* When the available spectrum request is made on behalf of a specific device (a master or slave
* device), device descriptor information for the device on whose behalf the request is made is
* required (in such cases, the requestType parameter must be empty). When a requestType value is
* specified, device descriptor information may be optional or required according to the rules of
* the applicable regulatory domain.
* @return value or {@code null} for none
*/
public DeviceDescriptor getDeviceDesc() {
return deviceDesc;
}
/**
* When the available spectrum request is made on behalf of a specific device (a master or slave
* device), device descriptor information for the device on whose behalf the request is made is
* required (in such cases, the requestType parameter must be empty). When a requestType value is
* specified, device descriptor information may be optional or required according to the rules of
* the applicable regulatory domain.
* @param deviceDesc deviceDesc or {@code null} for none
*/
public PawsGetSpectrumBatchRequest setDeviceDesc(DeviceDescriptor deviceDesc) {
this.deviceDesc = deviceDesc;
return this;
}
/**
* A geolocation list is required. This allows a device to specify its current location plus
* additional anticipated locations when allowed by the regulatory domain. At least one location
* must be included. Geolocation must be given as the location of the radiation center of the
* device's antenna. If a location specifies a region, rather than a point, the database may
* return an UNIMPLEMENTED error if it does not support query by region.
*
* There is no upper limit on the number of locations included in a available spectrum batch
* request, but the database may restrict the number of locations it supports by returning a
* response with fewer locations than specified in the batch request. Note that geolocations must
* be those of the master device (a device with geolocation capability that makes an available
* spectrum batch request), whether the master device is making the request on its own behalf or
* on behalf of a slave device (one without geolocation capability).
* @return value or {@code null} for none
*/
public java.util.List getLocations() {
return locations;
}
/**
* A geolocation list is required. This allows a device to specify its current location plus
* additional anticipated locations when allowed by the regulatory domain. At least one location
* must be included. Geolocation must be given as the location of the radiation center of the
* device's antenna. If a location specifies a region, rather than a point, the database may
* return an UNIMPLEMENTED error if it does not support query by region.
*
* There is no upper limit on the number of locations included in a available spectrum batch
* request, but the database may restrict the number of locations it supports by returning a
* response with fewer locations than specified in the batch request. Note that geolocations must
* be those of the master device (a device with geolocation capability that makes an available
* spectrum batch request), whether the master device is making the request on its own behalf or
* on behalf of a slave device (one without geolocation capability).
* @param locations locations or {@code null} for none
*/
public PawsGetSpectrumBatchRequest setLocations(java.util.List locations) {
this.locations = locations;
return this;
}
/**
* When an available spectrum batch request is made by the master device (a device with
* geolocation capability) on behalf of a slave device (a device without geolocation capability),
* the rules of the applicable regulatory domain may require the master device to provide its own
* device descriptor information (in addition to device descriptor information for the slave
* device in a separate parameter).
* @return value or {@code null} for none
*/
public DeviceDescriptor getMasterDeviceDesc() {
return masterDeviceDesc;
}
/**
* When an available spectrum batch request is made by the master device (a device with
* geolocation capability) on behalf of a slave device (a device without geolocation capability),
* the rules of the applicable regulatory domain may require the master device to provide its own
* device descriptor information (in addition to device descriptor information for the slave
* device in a separate parameter).
* @param masterDeviceDesc masterDeviceDesc or {@code null} for none
*/
public PawsGetSpectrumBatchRequest setMasterDeviceDesc(DeviceDescriptor masterDeviceDesc) {
this.masterDeviceDesc = masterDeviceDesc;
return this;
}
/**
* Depending on device type and regulatory domain, device owner information may be included in an
* available spectrum batch request. This allows the device to register and get spectrum-
* availability information in a single request.
* @return value or {@code null} for none
*/
public DeviceOwner getOwner() {
return owner;
}
/**
* Depending on device type and regulatory domain, device owner information may be included in an
* available spectrum batch request. This allows the device to register and get spectrum-
* availability information in a single request.
* @param owner owner or {@code null} for none
*/
public PawsGetSpectrumBatchRequest setOwner(DeviceOwner owner) {
this.owner = owner;
return this;
}
/**
* The request type parameter is an optional parameter that can be used to modify an available
* spectrum batch request, but its use depends on applicable regulatory rules. For example, It may
* be used to request generic slave device parameters without having to specify the device
* descriptor for a specific device. When the requestType parameter is missing, the request is for
* a specific device (master or slave), and the device descriptor parameter for the device on
* whose behalf the batch request is made is required.
* @return value or {@code null} for none
*/
public java.lang.String getRequestType() {
return requestType;
}
/**
* The request type parameter is an optional parameter that can be used to modify an available
* spectrum batch request, but its use depends on applicable regulatory rules. For example, It may
* be used to request generic slave device parameters without having to specify the device
* descriptor for a specific device. When the requestType parameter is missing, the request is for
* a specific device (master or slave), and the device descriptor parameter for the device on
* whose behalf the batch request is made is required.
* @param requestType requestType or {@code null} for none
*/
public PawsGetSpectrumBatchRequest setRequestType(java.lang.String requestType) {
this.requestType = requestType;
return this;
}
/**
* The message type (e.g., INIT_REQ, AVAIL_SPECTRUM_REQ, ...).
*
* Required field.
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* The message type (e.g., INIT_REQ, AVAIL_SPECTRUM_REQ, ...).
*
* Required field.
* @param type type or {@code null} for none
*/
public PawsGetSpectrumBatchRequest setType(java.lang.String type) {
this.type = type;
return this;
}
/**
* The PAWS version. Must be exactly 1.0.
*
* Required field.
* @return value or {@code null} for none
*/
public java.lang.String getVersion() {
return version;
}
/**
* The PAWS version. Must be exactly 1.0.
*
* Required field.
* @param version version or {@code null} for none
*/
public PawsGetSpectrumBatchRequest setVersion(java.lang.String version) {
this.version = version;
return this;
}
@Override
public PawsGetSpectrumBatchRequest set(String fieldName, Object value) {
return (PawsGetSpectrumBatchRequest) super.set(fieldName, value);
}
@Override
public PawsGetSpectrumBatchRequest clone() {
return (PawsGetSpectrumBatchRequest) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy