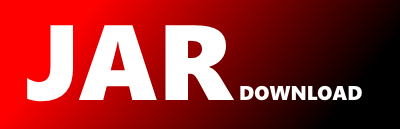
com.google.api.services.spectrum.model.PawsGetSpectrumBatchResponse Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-05-04 15:59:39 UTC)
* on 2016-05-18 at 06:28:13 UTC
* Modify at your own risk.
*/
package com.google.api.services.spectrum.model;
/**
* The response message for the batch available spectrum query contains a schedule of available
* spectrum for the device at multiple locations.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Spectrum Database API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class PawsGetSpectrumBatchResponse extends com.google.api.client.json.GenericJson {
/**
* A database may include the databaseChange parameter to notify a device of a change to its
* database URI, providing one or more alternate database URIs. The device should use this
* information to update its list of pre-configured databases by (only) replacing its entry for
* the responding database with the list of alternate URIs.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DbUpdateSpec databaseChange;
/**
* The database must return in its available spectrum response the device descriptor information
* it received in the master device's available spectrum batch request.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DeviceDescriptor deviceDesc;
/**
* The available spectrum batch response must contain a geo-spectrum schedule list, The list may
* be empty if spectrum is not available. The database may return more than one geo-spectrum
* schedule to represent future changes to the available spectrum. How far in advance a schedule
* may be provided depends upon the applicable regulatory domain. The database may return
* available spectrum for fewer geolocations than requested. The device must not make assumptions
* about the order of the entries in the list, and must use the geolocation value in each geo-
* spectrum schedule entry to match available spectrum to a location.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List geoSpectrumSchedules;
static {
// hack to force ProGuard to consider GeoSpectrumSchedule used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GeoSpectrumSchedule.class);
}
/**
* Identifies what kind of resource this is. Value: the fixed string
* "spectrum#pawsGetSpectrumBatchResponse".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* The database may return a constraint on the allowed maximum contiguous bandwidth (in Hertz). A
* regulatory domain may require the database to return this parameter. When this parameter is
* present in the response, the device must apply this constraint to its spectrum-selection logic
* to ensure that no single block of spectrum has bandwidth that exceeds this value.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double maxContiguousBwHz;
/**
* The database may return a constraint on the allowed maximum total bandwidth (in Hertz), which
* does not need to be contiguous. A regulatory domain may require the database to return this
* parameter. When this parameter is present in the available spectrum batch response, the device
* must apply this constraint to its spectrum-selection logic to ensure that total bandwidth does
* not exceed this value.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double maxTotalBwHz;
/**
* For regulatory domains that require a spectrum-usage report from devices, the database must
* return true for this parameter if the geo-spectrum schedules list is not empty; otherwise, the
* database should either return false or omit this parameter. If this parameter is present and
* its value is true, the device must send a spectrum use notify message to the database;
* otherwise, the device should not send the notification.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean needsSpectrumReport;
/**
* The database should return ruleset information, which identifies the applicable regulatory
* authority and ruleset for the available spectrum batch response. If included, the device must
* use the corresponding ruleset to interpret the response. Values provided in the returned
* ruleset information, such as maxLocationChange, take precedence over any conflicting values
* provided in the ruleset information returned in a prior initialization response sent by the
* database to the device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private RulesetInfo rulesetInfo;
/**
* The database includes a timestamp of the form, YYYY-MM-DDThh:mm:ssZ (Internet timestamp format
* per RFC3339), in its available spectrum batch response. The timestamp should be used by the
* device as a reference for the start and stop times specified in the response spectrum
* schedules.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String timestamp;
/**
* The message type (e.g., INIT_REQ, AVAIL_SPECTRUM_REQ, ...).
*
* Required field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* The PAWS version. Must be exactly 1.0.
*
* Required field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String version;
/**
* A database may include the databaseChange parameter to notify a device of a change to its
* database URI, providing one or more alternate database URIs. The device should use this
* information to update its list of pre-configured databases by (only) replacing its entry for
* the responding database with the list of alternate URIs.
* @return value or {@code null} for none
*/
public DbUpdateSpec getDatabaseChange() {
return databaseChange;
}
/**
* A database may include the databaseChange parameter to notify a device of a change to its
* database URI, providing one or more alternate database URIs. The device should use this
* information to update its list of pre-configured databases by (only) replacing its entry for
* the responding database with the list of alternate URIs.
* @param databaseChange databaseChange or {@code null} for none
*/
public PawsGetSpectrumBatchResponse setDatabaseChange(DbUpdateSpec databaseChange) {
this.databaseChange = databaseChange;
return this;
}
/**
* The database must return in its available spectrum response the device descriptor information
* it received in the master device's available spectrum batch request.
* @return value or {@code null} for none
*/
public DeviceDescriptor getDeviceDesc() {
return deviceDesc;
}
/**
* The database must return in its available spectrum response the device descriptor information
* it received in the master device's available spectrum batch request.
* @param deviceDesc deviceDesc or {@code null} for none
*/
public PawsGetSpectrumBatchResponse setDeviceDesc(DeviceDescriptor deviceDesc) {
this.deviceDesc = deviceDesc;
return this;
}
/**
* The available spectrum batch response must contain a geo-spectrum schedule list, The list may
* be empty if spectrum is not available. The database may return more than one geo-spectrum
* schedule to represent future changes to the available spectrum. How far in advance a schedule
* may be provided depends upon the applicable regulatory domain. The database may return
* available spectrum for fewer geolocations than requested. The device must not make assumptions
* about the order of the entries in the list, and must use the geolocation value in each geo-
* spectrum schedule entry to match available spectrum to a location.
* @return value or {@code null} for none
*/
public java.util.List getGeoSpectrumSchedules() {
return geoSpectrumSchedules;
}
/**
* The available spectrum batch response must contain a geo-spectrum schedule list, The list may
* be empty if spectrum is not available. The database may return more than one geo-spectrum
* schedule to represent future changes to the available spectrum. How far in advance a schedule
* may be provided depends upon the applicable regulatory domain. The database may return
* available spectrum for fewer geolocations than requested. The device must not make assumptions
* about the order of the entries in the list, and must use the geolocation value in each geo-
* spectrum schedule entry to match available spectrum to a location.
* @param geoSpectrumSchedules geoSpectrumSchedules or {@code null} for none
*/
public PawsGetSpectrumBatchResponse setGeoSpectrumSchedules(java.util.List geoSpectrumSchedules) {
this.geoSpectrumSchedules = geoSpectrumSchedules;
return this;
}
/**
* Identifies what kind of resource this is. Value: the fixed string
* "spectrum#pawsGetSpectrumBatchResponse".
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* Identifies what kind of resource this is. Value: the fixed string
* "spectrum#pawsGetSpectrumBatchResponse".
* @param kind kind or {@code null} for none
*/
public PawsGetSpectrumBatchResponse setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* The database may return a constraint on the allowed maximum contiguous bandwidth (in Hertz). A
* regulatory domain may require the database to return this parameter. When this parameter is
* present in the response, the device must apply this constraint to its spectrum-selection logic
* to ensure that no single block of spectrum has bandwidth that exceeds this value.
* @return value or {@code null} for none
*/
public java.lang.Double getMaxContiguousBwHz() {
return maxContiguousBwHz;
}
/**
* The database may return a constraint on the allowed maximum contiguous bandwidth (in Hertz). A
* regulatory domain may require the database to return this parameter. When this parameter is
* present in the response, the device must apply this constraint to its spectrum-selection logic
* to ensure that no single block of spectrum has bandwidth that exceeds this value.
* @param maxContiguousBwHz maxContiguousBwHz or {@code null} for none
*/
public PawsGetSpectrumBatchResponse setMaxContiguousBwHz(java.lang.Double maxContiguousBwHz) {
this.maxContiguousBwHz = maxContiguousBwHz;
return this;
}
/**
* The database may return a constraint on the allowed maximum total bandwidth (in Hertz), which
* does not need to be contiguous. A regulatory domain may require the database to return this
* parameter. When this parameter is present in the available spectrum batch response, the device
* must apply this constraint to its spectrum-selection logic to ensure that total bandwidth does
* not exceed this value.
* @return value or {@code null} for none
*/
public java.lang.Double getMaxTotalBwHz() {
return maxTotalBwHz;
}
/**
* The database may return a constraint on the allowed maximum total bandwidth (in Hertz), which
* does not need to be contiguous. A regulatory domain may require the database to return this
* parameter. When this parameter is present in the available spectrum batch response, the device
* must apply this constraint to its spectrum-selection logic to ensure that total bandwidth does
* not exceed this value.
* @param maxTotalBwHz maxTotalBwHz or {@code null} for none
*/
public PawsGetSpectrumBatchResponse setMaxTotalBwHz(java.lang.Double maxTotalBwHz) {
this.maxTotalBwHz = maxTotalBwHz;
return this;
}
/**
* For regulatory domains that require a spectrum-usage report from devices, the database must
* return true for this parameter if the geo-spectrum schedules list is not empty; otherwise, the
* database should either return false or omit this parameter. If this parameter is present and
* its value is true, the device must send a spectrum use notify message to the database;
* otherwise, the device should not send the notification.
* @return value or {@code null} for none
*/
public java.lang.Boolean getNeedsSpectrumReport() {
return needsSpectrumReport;
}
/**
* For regulatory domains that require a spectrum-usage report from devices, the database must
* return true for this parameter if the geo-spectrum schedules list is not empty; otherwise, the
* database should either return false or omit this parameter. If this parameter is present and
* its value is true, the device must send a spectrum use notify message to the database;
* otherwise, the device should not send the notification.
* @param needsSpectrumReport needsSpectrumReport or {@code null} for none
*/
public PawsGetSpectrumBatchResponse setNeedsSpectrumReport(java.lang.Boolean needsSpectrumReport) {
this.needsSpectrumReport = needsSpectrumReport;
return this;
}
/**
* The database should return ruleset information, which identifies the applicable regulatory
* authority and ruleset for the available spectrum batch response. If included, the device must
* use the corresponding ruleset to interpret the response. Values provided in the returned
* ruleset information, such as maxLocationChange, take precedence over any conflicting values
* provided in the ruleset information returned in a prior initialization response sent by the
* database to the device.
* @return value or {@code null} for none
*/
public RulesetInfo getRulesetInfo() {
return rulesetInfo;
}
/**
* The database should return ruleset information, which identifies the applicable regulatory
* authority and ruleset for the available spectrum batch response. If included, the device must
* use the corresponding ruleset to interpret the response. Values provided in the returned
* ruleset information, such as maxLocationChange, take precedence over any conflicting values
* provided in the ruleset information returned in a prior initialization response sent by the
* database to the device.
* @param rulesetInfo rulesetInfo or {@code null} for none
*/
public PawsGetSpectrumBatchResponse setRulesetInfo(RulesetInfo rulesetInfo) {
this.rulesetInfo = rulesetInfo;
return this;
}
/**
* The database includes a timestamp of the form, YYYY-MM-DDThh:mm:ssZ (Internet timestamp format
* per RFC3339), in its available spectrum batch response. The timestamp should be used by the
* device as a reference for the start and stop times specified in the response spectrum
* schedules.
* @return value or {@code null} for none
*/
public java.lang.String getTimestamp() {
return timestamp;
}
/**
* The database includes a timestamp of the form, YYYY-MM-DDThh:mm:ssZ (Internet timestamp format
* per RFC3339), in its available spectrum batch response. The timestamp should be used by the
* device as a reference for the start and stop times specified in the response spectrum
* schedules.
* @param timestamp timestamp or {@code null} for none
*/
public PawsGetSpectrumBatchResponse setTimestamp(java.lang.String timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* The message type (e.g., INIT_REQ, AVAIL_SPECTRUM_REQ, ...).
*
* Required field.
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* The message type (e.g., INIT_REQ, AVAIL_SPECTRUM_REQ, ...).
*
* Required field.
* @param type type or {@code null} for none
*/
public PawsGetSpectrumBatchResponse setType(java.lang.String type) {
this.type = type;
return this;
}
/**
* The PAWS version. Must be exactly 1.0.
*
* Required field.
* @return value or {@code null} for none
*/
public java.lang.String getVersion() {
return version;
}
/**
* The PAWS version. Must be exactly 1.0.
*
* Required field.
* @param version version or {@code null} for none
*/
public PawsGetSpectrumBatchResponse setVersion(java.lang.String version) {
this.version = version;
return this;
}
@Override
public PawsGetSpectrumBatchResponse set(String fieldName, Object value) {
return (PawsGetSpectrumBatchResponse) super.set(fieldName, value);
}
@Override
public PawsGetSpectrumBatchResponse clone() {
return (PawsGetSpectrumBatchResponse) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy