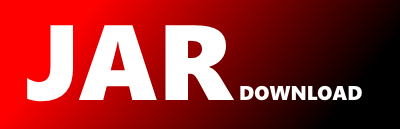
com.google.api.services.speech.v1beta1.model.RecognitionAudio Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2017-02-15 17:18:02 UTC)
* on 2017-04-08 at 18:29:03 UTC
* Modify at your own risk.
*/
package com.google.api.services.speech.v1beta1.model;
/**
* Contains audio data in the encoding specified in the `RecognitionConfig`. Either `content` or
* `uri` must be supplied. Supplying both or neither returns google.rpc.Code.INVALID_ARGUMENT. See
* [audio limits](https://cloud.google.com/speech/limits#content).
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Cloud Speech API. For a detailed explanation
* see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class RecognitionAudio extends com.google.api.client.json.GenericJson {
/**
* The audio data bytes encoded as specified in `RecognitionConfig`. Note: as with all bytes
* fields, protobuffers use a pure binary representation, whereas JSON representations use base64.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String content;
/**
* URI that points to a file that contains audio data bytes as specified in `RecognitionConfig`.
* Currently, only Google Cloud Storage URIs are supported, which must be specified in the
* following format: `gs://bucket_name/object_name` (other URI formats return
* google.rpc.Code.INVALID_ARGUMENT). For more information, see [Request
* URIs](https://cloud.google.com/storage/docs/reference-uris).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String uri;
/**
* The audio data bytes encoded as specified in `RecognitionConfig`. Note: as with all bytes
* fields, protobuffers use a pure binary representation, whereas JSON representations use base64.
* @see #decodeContent()
* @return value or {@code null} for none
*/
public java.lang.String getContent() {
return content;
}
/**
* The audio data bytes encoded as specified in `RecognitionConfig`. Note: as with all bytes
* fields, protobuffers use a pure binary representation, whereas JSON representations use base64.
* @see #getContent()
* @return Base64 decoded value or {@code null} for none
*
* @since 1.14
*/
public byte[] decodeContent() {
return com.google.api.client.util.Base64.decodeBase64(content);
}
/**
* The audio data bytes encoded as specified in `RecognitionConfig`. Note: as with all bytes
* fields, protobuffers use a pure binary representation, whereas JSON representations use base64.
* @see #encodeContent()
* @param content content or {@code null} for none
*/
public RecognitionAudio setContent(java.lang.String content) {
this.content = content;
return this;
}
/**
* The audio data bytes encoded as specified in `RecognitionConfig`. Note: as with all bytes
* fields, protobuffers use a pure binary representation, whereas JSON representations use base64.
* @see #setContent()
*
*
* The value is encoded Base64 or {@code null} for none.
*
*
* @since 1.14
*/
public RecognitionAudio encodeContent(byte[] content) {
this.content = com.google.api.client.util.Base64.encodeBase64URLSafeString(content);
return this;
}
/**
* URI that points to a file that contains audio data bytes as specified in `RecognitionConfig`.
* Currently, only Google Cloud Storage URIs are supported, which must be specified in the
* following format: `gs://bucket_name/object_name` (other URI formats return
* google.rpc.Code.INVALID_ARGUMENT). For more information, see [Request
* URIs](https://cloud.google.com/storage/docs/reference-uris).
* @return value or {@code null} for none
*/
public java.lang.String getUri() {
return uri;
}
/**
* URI that points to a file that contains audio data bytes as specified in `RecognitionConfig`.
* Currently, only Google Cloud Storage URIs are supported, which must be specified in the
* following format: `gs://bucket_name/object_name` (other URI formats return
* google.rpc.Code.INVALID_ARGUMENT). For more information, see [Request
* URIs](https://cloud.google.com/storage/docs/reference-uris).
* @param uri uri or {@code null} for none
*/
public RecognitionAudio setUri(java.lang.String uri) {
this.uri = uri;
return this;
}
@Override
public RecognitionAudio set(String fieldName, Object value) {
return (RecognitionAudio) super.set(fieldName, value);
}
@Override
public RecognitionAudio clone() {
return (RecognitionAudio) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy