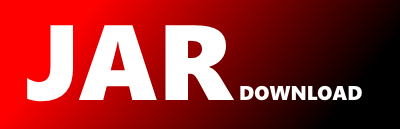
com.google.api.services.sql.SQLAdmin Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.sql;
/**
* Service definition for SQLAdmin (v1beta4).
*
*
* API for Cloud SQL database instance management
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link SQLAdminRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class SQLAdmin extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.30.10 of the Cloud SQL Admin API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://sqladmin.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public SQLAdmin(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
SQLAdmin(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the BackupRuns collection.
*
* The typical use is:
*
* {@code SQLAdmin sql = new SQLAdmin(...);}
* {@code SQLAdmin.BackupRuns.List request = sql.backupRuns().list(parameters ...)}
*
*
* @return the resource collection
*/
public BackupRuns backupRuns() {
return new BackupRuns();
}
/**
* The "backupRuns" collection of methods.
*/
public class BackupRuns {
/**
* Deletes the backup taken by a backup run.
*
* Create a request for the method "backupRuns.delete".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param id The ID of the Backup Run to delete. To find a Backup Run ID, use the list method.
* @return the request
*/
public Delete delete(java.lang.String project, java.lang.String instance, java.lang.Long id) throws java.io.IOException {
Delete result = new Delete(project, instance, id);
initialize(result);
return result;
}
public class Delete extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/backupRuns/{id}";
/**
* Deletes the backup taken by a backup run.
*
* Create a request for the method "backupRuns.delete".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param id The ID of the Backup Run to delete. To find a Backup Run ID, use the list method.
* @since 1.13
*/
protected Delete(java.lang.String project, java.lang.String instance, java.lang.Long id) {
super(SQLAdmin.this, "DELETE", REST_PATH, null, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Delete setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Delete setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** The ID of the Backup Run to delete. To find a Backup Run ID, use the list method. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** The ID of the Backup Run to delete. To find a Backup Run ID, use the list method.
*/
public java.lang.Long getId() {
return id;
}
/** The ID of the Backup Run to delete. To find a Backup Run ID, use the list method. */
public Delete setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves a resource containing information about a backup run.
*
* Create a request for the method "backupRuns.get".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param id The ID of this Backup Run.
* @return the request
*/
public Get get(java.lang.String project, java.lang.String instance, java.lang.Long id) throws java.io.IOException {
Get result = new Get(project, instance, id);
initialize(result);
return result;
}
public class Get extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/backupRuns/{id}";
/**
* Retrieves a resource containing information about a backup run.
*
* Create a request for the method "backupRuns.get".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param id The ID of this Backup Run.
* @since 1.13
*/
protected Get(java.lang.String project, java.lang.String instance, java.lang.Long id) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sql.model.BackupRun.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Get setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Get setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** The ID of this Backup Run. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** The ID of this Backup Run.
*/
public java.lang.Long getId() {
return id;
}
/** The ID of this Backup Run. */
public Get setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Creates a new backup run on demand. This method is applicable only to Second Generation
* instances.
*
* Create a request for the method "backupRuns.insert".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.BackupRun}
* @return the request
*/
public Insert insert(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.BackupRun content) throws java.io.IOException {
Insert result = new Insert(project, instance, content);
initialize(result);
return result;
}
public class Insert extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/backupRuns";
/**
* Creates a new backup run on demand. This method is applicable only to Second Generation
* instances.
*
* Create a request for the method "backupRuns.insert".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.BackupRun}
* @since 1.13
*/
protected Insert(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.BackupRun content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Insert setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Insert setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Lists all backup runs associated with a given instance and configuration in the reverse
* chronological order of the backup initiation time.
*
* Create a request for the method "backupRuns.list".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @return the request
*/
public List list(java.lang.String project, java.lang.String instance) throws java.io.IOException {
List result = new List(project, instance);
initialize(result);
return result;
}
public class List extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/backupRuns";
/**
* Lists all backup runs associated with a given instance and configuration in the reverse
* chronological order of the backup initiation time.
*
* Create a request for the method "backupRuns.list".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @since 1.13
*/
protected List(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sql.model.BackupRunsListResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public List setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public List setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** Maximum number of backup runs per response. */
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** Maximum number of backup runs per response.
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/** Maximum number of backup runs per response. */
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* A previously-returned page token representing part of the larger set of results to view.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A previously-returned page token representing part of the larger set of results to view.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A previously-returned page token representing part of the larger set of results to view.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Databases collection.
*
* The typical use is:
*
* {@code SQLAdmin sql = new SQLAdmin(...);}
* {@code SQLAdmin.Databases.List request = sql.databases().list(parameters ...)}
*
*
* @return the resource collection
*/
public Databases databases() {
return new Databases();
}
/**
* The "databases" collection of methods.
*/
public class Databases {
/**
* Deletes a database from a Cloud SQL instance.
*
* Create a request for the method "databases.delete".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param database Name of the database to be deleted in the instance.
* @return the request
*/
public Delete delete(java.lang.String project, java.lang.String instance, java.lang.String database) throws java.io.IOException {
Delete result = new Delete(project, instance, database);
initialize(result);
return result;
}
public class Delete extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/databases/{database}";
/**
* Deletes a database from a Cloud SQL instance.
*
* Create a request for the method "databases.delete".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param database Name of the database to be deleted in the instance.
* @since 1.13
*/
protected Delete(java.lang.String project, java.lang.String instance, java.lang.String database) {
super(SQLAdmin.this, "DELETE", REST_PATH, null, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
this.database = com.google.api.client.util.Preconditions.checkNotNull(database, "Required parameter database must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Delete setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Database instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Database instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Database instance ID. This does not include the project ID. */
public Delete setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** Name of the database to be deleted in the instance. */
@com.google.api.client.util.Key
private java.lang.String database;
/** Name of the database to be deleted in the instance.
*/
public java.lang.String getDatabase() {
return database;
}
/** Name of the database to be deleted in the instance. */
public Delete setDatabase(java.lang.String database) {
this.database = database;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves a resource containing information about a database inside a Cloud SQL instance.
*
* Create a request for the method "databases.get".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param database Name of the database in the instance.
* @return the request
*/
public Get get(java.lang.String project, java.lang.String instance, java.lang.String database) throws java.io.IOException {
Get result = new Get(project, instance, database);
initialize(result);
return result;
}
public class Get extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/databases/{database}";
/**
* Retrieves a resource containing information about a database inside a Cloud SQL instance.
*
* Create a request for the method "databases.get".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param database Name of the database in the instance.
* @since 1.13
*/
protected Get(java.lang.String project, java.lang.String instance, java.lang.String database) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sql.model.Database.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
this.database = com.google.api.client.util.Preconditions.checkNotNull(database, "Required parameter database must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Get setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Database instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Database instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Database instance ID. This does not include the project ID. */
public Get setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** Name of the database in the instance. */
@com.google.api.client.util.Key
private java.lang.String database;
/** Name of the database in the instance.
*/
public java.lang.String getDatabase() {
return database;
}
/** Name of the database in the instance. */
public Get setDatabase(java.lang.String database) {
this.database = database;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Inserts a resource containing information about a database inside a Cloud SQL instance.
*
* Create a request for the method "databases.insert".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.Database}
* @return the request
*/
public Insert insert(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.Database content) throws java.io.IOException {
Insert result = new Insert(project, instance, content);
initialize(result);
return result;
}
public class Insert extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/databases";
/**
* Inserts a resource containing information about a database inside a Cloud SQL instance.
*
* Create a request for the method "databases.insert".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.Database}
* @since 1.13
*/
protected Insert(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.Database content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Insert setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Database instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Database instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Database instance ID. This does not include the project ID. */
public Insert setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Lists databases in the specified Cloud SQL instance.
*
* Create a request for the method "databases.list".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @return the request
*/
public List list(java.lang.String project, java.lang.String instance) throws java.io.IOException {
List result = new List(project, instance);
initialize(result);
return result;
}
public class List extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/databases";
/**
* Lists databases in the specified Cloud SQL instance.
*
* Create a request for the method "databases.list".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @since 1.13
*/
protected List(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sql.model.DatabasesListResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public List setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public List setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Partially updates a resource containing information about a database inside a Cloud SQL instance.
* This method supports patch semantics.
*
* Create a request for the method "databases.patch".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param database Name of the database to be updated in the instance.
* @param content the {@link com.google.api.services.sql.model.Database}
* @return the request
*/
public Patch patch(java.lang.String project, java.lang.String instance, java.lang.String database, com.google.api.services.sql.model.Database content) throws java.io.IOException {
Patch result = new Patch(project, instance, database, content);
initialize(result);
return result;
}
public class Patch extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/databases/{database}";
/**
* Partially updates a resource containing information about a database inside a Cloud SQL
* instance. This method supports patch semantics.
*
* Create a request for the method "databases.patch".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param database Name of the database to be updated in the instance.
* @param content the {@link com.google.api.services.sql.model.Database}
* @since 1.13
*/
protected Patch(java.lang.String project, java.lang.String instance, java.lang.String database, com.google.api.services.sql.model.Database content) {
super(SQLAdmin.this, "PATCH", REST_PATH, content, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
this.database = com.google.api.client.util.Preconditions.checkNotNull(database, "Required parameter database must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Patch setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Database instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Database instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Database instance ID. This does not include the project ID. */
public Patch setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** Name of the database to be updated in the instance. */
@com.google.api.client.util.Key
private java.lang.String database;
/** Name of the database to be updated in the instance.
*/
public java.lang.String getDatabase() {
return database;
}
/** Name of the database to be updated in the instance. */
public Patch setDatabase(java.lang.String database) {
this.database = database;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates a resource containing information about a database inside a Cloud SQL instance.
*
* Create a request for the method "databases.update".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param database Name of the database to be updated in the instance.
* @param content the {@link com.google.api.services.sql.model.Database}
* @return the request
*/
public Update update(java.lang.String project, java.lang.String instance, java.lang.String database, com.google.api.services.sql.model.Database content) throws java.io.IOException {
Update result = new Update(project, instance, database, content);
initialize(result);
return result;
}
public class Update extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/databases/{database}";
/**
* Updates a resource containing information about a database inside a Cloud SQL instance.
*
* Create a request for the method "databases.update".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param database Name of the database to be updated in the instance.
* @param content the {@link com.google.api.services.sql.model.Database}
* @since 1.13
*/
protected Update(java.lang.String project, java.lang.String instance, java.lang.String database, com.google.api.services.sql.model.Database content) {
super(SQLAdmin.this, "PUT", REST_PATH, content, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
this.database = com.google.api.client.util.Preconditions.checkNotNull(database, "Required parameter database must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Update setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Database instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Database instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Database instance ID. This does not include the project ID. */
public Update setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** Name of the database to be updated in the instance. */
@com.google.api.client.util.Key
private java.lang.String database;
/** Name of the database to be updated in the instance.
*/
public java.lang.String getDatabase() {
return database;
}
/** Name of the database to be updated in the instance. */
public Update setDatabase(java.lang.String database) {
this.database = database;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Flags collection.
*
* The typical use is:
*
* {@code SQLAdmin sql = new SQLAdmin(...);}
* {@code SQLAdmin.Flags.List request = sql.flags().list(parameters ...)}
*
*
* @return the resource collection
*/
public Flags flags() {
return new Flags();
}
/**
* The "flags" collection of methods.
*/
public class Flags {
/**
* List all available database flags for Cloud SQL instances.
*
* Create a request for the method "flags.list".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/flags";
/**
* List all available database flags for Cloud SQL instances.
*
* Create a request for the method "flags.list".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sql.model.FlagsListResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Database type and version you want to retrieve flags for. By default, this method returns
* flags for all database types and versions.
*/
@com.google.api.client.util.Key
private java.lang.String databaseVersion;
/** Database type and version you want to retrieve flags for. By default, this method returns flags for
all database types and versions.
*/
public java.lang.String getDatabaseVersion() {
return databaseVersion;
}
/**
* Database type and version you want to retrieve flags for. By default, this method returns
* flags for all database types and versions.
*/
public List setDatabaseVersion(java.lang.String databaseVersion) {
this.databaseVersion = databaseVersion;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Instances collection.
*
* The typical use is:
*
* {@code SQLAdmin sql = new SQLAdmin(...);}
* {@code SQLAdmin.Instances.List request = sql.instances().list(parameters ...)}
*
*
* @return the resource collection
*/
public Instances instances() {
return new Instances();
}
/**
* The "instances" collection of methods.
*/
public class Instances {
/**
* Add a new trusted Certificate Authority (CA) version for the specified instance. Required to
* prepare for a certificate rotation. If a CA version was previously added but never used in a
* certificate rotation, this operation replaces that version. There cannot be more than one CA
* version waiting to be rotated in.
*
* Create a request for the method "instances.addServerCa".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link AddServerCa#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @return the request
*/
public AddServerCa addServerCa(java.lang.String project, java.lang.String instance) throws java.io.IOException {
AddServerCa result = new AddServerCa(project, instance);
initialize(result);
return result;
}
public class AddServerCa extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/addServerCa";
/**
* Add a new trusted Certificate Authority (CA) version for the specified instance. Required to
* prepare for a certificate rotation. If a CA version was previously added but never used in a
* certificate rotation, this operation replaces that version. There cannot be more than one CA
* version waiting to be rotated in.
*
* Create a request for the method "instances.addServerCa".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link AddServerCa#execute()} method to invoke the remote operation.
* {@link
* AddServerCa#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @since 1.13
*/
protected AddServerCa(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "POST", REST_PATH, null, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public AddServerCa set$Xgafv(java.lang.String $Xgafv) {
return (AddServerCa) super.set$Xgafv($Xgafv);
}
@Override
public AddServerCa setAccessToken(java.lang.String accessToken) {
return (AddServerCa) super.setAccessToken(accessToken);
}
@Override
public AddServerCa setAlt(java.lang.String alt) {
return (AddServerCa) super.setAlt(alt);
}
@Override
public AddServerCa setCallback(java.lang.String callback) {
return (AddServerCa) super.setCallback(callback);
}
@Override
public AddServerCa setFields(java.lang.String fields) {
return (AddServerCa) super.setFields(fields);
}
@Override
public AddServerCa setKey(java.lang.String key) {
return (AddServerCa) super.setKey(key);
}
@Override
public AddServerCa setOauthToken(java.lang.String oauthToken) {
return (AddServerCa) super.setOauthToken(oauthToken);
}
@Override
public AddServerCa setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AddServerCa) super.setPrettyPrint(prettyPrint);
}
@Override
public AddServerCa setQuotaUser(java.lang.String quotaUser) {
return (AddServerCa) super.setQuotaUser(quotaUser);
}
@Override
public AddServerCa setUploadType(java.lang.String uploadType) {
return (AddServerCa) super.setUploadType(uploadType);
}
@Override
public AddServerCa setUploadProtocol(java.lang.String uploadProtocol) {
return (AddServerCa) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public AddServerCa setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public AddServerCa setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public AddServerCa set(String parameterName, Object value) {
return (AddServerCa) super.set(parameterName, value);
}
}
/**
* Creates a Cloud SQL instance as a clone of the source instance. Using this operation might cause
* your instance to restart.
*
* Create a request for the method "instances.clone".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link Clone#execute()} method to invoke the remote operation.
*
* @param project Project ID of the source as well as the clone Cloud SQL instance.
* @param instance The ID of the Cloud SQL instance to be cloned (source). This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.InstancesCloneRequest}
* @return the request
*/
public Clone clone(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.InstancesCloneRequest content) throws java.io.IOException {
Clone result = new Clone(project, instance, content);
initialize(result);
return result;
}
public class Clone extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/clone";
/**
* Creates a Cloud SQL instance as a clone of the source instance. Using this operation might
* cause your instance to restart.
*
* Create a request for the method "instances.clone".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link Clone#execute()} method to invoke the remote operation. {@link
* Clone#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the source as well as the clone Cloud SQL instance.
* @param instance The ID of the Cloud SQL instance to be cloned (source). This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.InstancesCloneRequest}
* @since 1.13
*/
protected Clone(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.InstancesCloneRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Clone set$Xgafv(java.lang.String $Xgafv) {
return (Clone) super.set$Xgafv($Xgafv);
}
@Override
public Clone setAccessToken(java.lang.String accessToken) {
return (Clone) super.setAccessToken(accessToken);
}
@Override
public Clone setAlt(java.lang.String alt) {
return (Clone) super.setAlt(alt);
}
@Override
public Clone setCallback(java.lang.String callback) {
return (Clone) super.setCallback(callback);
}
@Override
public Clone setFields(java.lang.String fields) {
return (Clone) super.setFields(fields);
}
@Override
public Clone setKey(java.lang.String key) {
return (Clone) super.setKey(key);
}
@Override
public Clone setOauthToken(java.lang.String oauthToken) {
return (Clone) super.setOauthToken(oauthToken);
}
@Override
public Clone setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Clone) super.setPrettyPrint(prettyPrint);
}
@Override
public Clone setQuotaUser(java.lang.String quotaUser) {
return (Clone) super.setQuotaUser(quotaUser);
}
@Override
public Clone setUploadType(java.lang.String uploadType) {
return (Clone) super.setUploadType(uploadType);
}
@Override
public Clone setUploadProtocol(java.lang.String uploadProtocol) {
return (Clone) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the source as well as the clone Cloud SQL instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the source as well as the clone Cloud SQL instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the source as well as the clone Cloud SQL instance. */
public Clone setProject(java.lang.String project) {
this.project = project;
return this;
}
/**
* The ID of the Cloud SQL instance to be cloned (source). This does not include the project
* ID.
*/
@com.google.api.client.util.Key
private java.lang.String instance;
/** The ID of the Cloud SQL instance to be cloned (source). This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/**
* The ID of the Cloud SQL instance to be cloned (source). This does not include the project
* ID.
*/
public Clone setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Clone set(String parameterName, Object value) {
return (Clone) super.set(parameterName, value);
}
}
/**
* Deletes a Cloud SQL instance.
*
* Create a request for the method "instances.delete".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance to be deleted.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @return the request
*/
public Delete delete(java.lang.String project, java.lang.String instance) throws java.io.IOException {
Delete result = new Delete(project, instance);
initialize(result);
return result;
}
public class Delete extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}";
/**
* Deletes a Cloud SQL instance.
*
* Create a request for the method "instances.delete".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance to be deleted.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @since 1.13
*/
protected Delete(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "DELETE", REST_PATH, null, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance to be deleted. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance to be deleted.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance to be deleted. */
public Delete setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Delete setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Demotes the stand-alone instance to be a Cloud SQL read replica for an external database server.
*
* Create a request for the method "instances.demoteMaster".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link DemoteMaster#execute()} method to invoke the remote operation.
*
* @param project ID of the project that contains the instance.
* @param instance Cloud SQL instance name.
* @param content the {@link com.google.api.services.sql.model.InstancesDemoteMasterRequest}
* @return the request
*/
public DemoteMaster demoteMaster(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.InstancesDemoteMasterRequest content) throws java.io.IOException {
DemoteMaster result = new DemoteMaster(project, instance, content);
initialize(result);
return result;
}
public class DemoteMaster extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/demoteMaster";
/**
* Demotes the stand-alone instance to be a Cloud SQL read replica for an external database
* server.
*
* Create a request for the method "instances.demoteMaster".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link DemoteMaster#execute()} method to invoke the remote operation.
* {@link
* DemoteMaster#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project ID of the project that contains the instance.
* @param instance Cloud SQL instance name.
* @param content the {@link com.google.api.services.sql.model.InstancesDemoteMasterRequest}
* @since 1.13
*/
protected DemoteMaster(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.InstancesDemoteMasterRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public DemoteMaster set$Xgafv(java.lang.String $Xgafv) {
return (DemoteMaster) super.set$Xgafv($Xgafv);
}
@Override
public DemoteMaster setAccessToken(java.lang.String accessToken) {
return (DemoteMaster) super.setAccessToken(accessToken);
}
@Override
public DemoteMaster setAlt(java.lang.String alt) {
return (DemoteMaster) super.setAlt(alt);
}
@Override
public DemoteMaster setCallback(java.lang.String callback) {
return (DemoteMaster) super.setCallback(callback);
}
@Override
public DemoteMaster setFields(java.lang.String fields) {
return (DemoteMaster) super.setFields(fields);
}
@Override
public DemoteMaster setKey(java.lang.String key) {
return (DemoteMaster) super.setKey(key);
}
@Override
public DemoteMaster setOauthToken(java.lang.String oauthToken) {
return (DemoteMaster) super.setOauthToken(oauthToken);
}
@Override
public DemoteMaster setPrettyPrint(java.lang.Boolean prettyPrint) {
return (DemoteMaster) super.setPrettyPrint(prettyPrint);
}
@Override
public DemoteMaster setQuotaUser(java.lang.String quotaUser) {
return (DemoteMaster) super.setQuotaUser(quotaUser);
}
@Override
public DemoteMaster setUploadType(java.lang.String uploadType) {
return (DemoteMaster) super.setUploadType(uploadType);
}
@Override
public DemoteMaster setUploadProtocol(java.lang.String uploadProtocol) {
return (DemoteMaster) super.setUploadProtocol(uploadProtocol);
}
/** ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** ID of the project that contains the instance. */
public DemoteMaster setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance name. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance name.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance name. */
public DemoteMaster setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public DemoteMaster set(String parameterName, Object value) {
return (DemoteMaster) super.set(parameterName, value);
}
}
/**
* Exports data from a Cloud SQL instance to a Cloud Storage bucket as a SQL dump or CSV file.
*
* Create a request for the method "instances.export".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link Export#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance to be exported.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.InstancesExportRequest}
* @return the request
*/
public Export export(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.InstancesExportRequest content) throws java.io.IOException {
Export result = new Export(project, instance, content);
initialize(result);
return result;
}
public class Export extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/export";
/**
* Exports data from a Cloud SQL instance to a Cloud Storage bucket as a SQL dump or CSV file.
*
* Create a request for the method "instances.export".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link Export#execute()} method to invoke the remote operation. {@link
* Export#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance to be exported.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.InstancesExportRequest}
* @since 1.13
*/
protected Export(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.InstancesExportRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Export set$Xgafv(java.lang.String $Xgafv) {
return (Export) super.set$Xgafv($Xgafv);
}
@Override
public Export setAccessToken(java.lang.String accessToken) {
return (Export) super.setAccessToken(accessToken);
}
@Override
public Export setAlt(java.lang.String alt) {
return (Export) super.setAlt(alt);
}
@Override
public Export setCallback(java.lang.String callback) {
return (Export) super.setCallback(callback);
}
@Override
public Export setFields(java.lang.String fields) {
return (Export) super.setFields(fields);
}
@Override
public Export setKey(java.lang.String key) {
return (Export) super.setKey(key);
}
@Override
public Export setOauthToken(java.lang.String oauthToken) {
return (Export) super.setOauthToken(oauthToken);
}
@Override
public Export setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Export) super.setPrettyPrint(prettyPrint);
}
@Override
public Export setQuotaUser(java.lang.String quotaUser) {
return (Export) super.setQuotaUser(quotaUser);
}
@Override
public Export setUploadType(java.lang.String uploadType) {
return (Export) super.setUploadType(uploadType);
}
@Override
public Export setUploadProtocol(java.lang.String uploadProtocol) {
return (Export) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance to be exported. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance to be exported.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance to be exported. */
public Export setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Export setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Export set(String parameterName, Object value) {
return (Export) super.set(parameterName, value);
}
}
/**
* Failover the instance to its failover replica instance. Using this operation might cause your
* instance to restart.
*
* Create a request for the method "instances.failover".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link Failover#execute()} method to invoke the remote operation.
*
* @param project ID of the project that contains the read replica.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.InstancesFailoverRequest}
* @return the request
*/
public Failover failover(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.InstancesFailoverRequest content) throws java.io.IOException {
Failover result = new Failover(project, instance, content);
initialize(result);
return result;
}
public class Failover extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/failover";
/**
* Failover the instance to its failover replica instance. Using this operation might cause your
* instance to restart.
*
* Create a request for the method "instances.failover".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link Failover#execute()} method to invoke the remote operation.
* {@link
* Failover#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project ID of the project that contains the read replica.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.InstancesFailoverRequest}
* @since 1.13
*/
protected Failover(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.InstancesFailoverRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Failover set$Xgafv(java.lang.String $Xgafv) {
return (Failover) super.set$Xgafv($Xgafv);
}
@Override
public Failover setAccessToken(java.lang.String accessToken) {
return (Failover) super.setAccessToken(accessToken);
}
@Override
public Failover setAlt(java.lang.String alt) {
return (Failover) super.setAlt(alt);
}
@Override
public Failover setCallback(java.lang.String callback) {
return (Failover) super.setCallback(callback);
}
@Override
public Failover setFields(java.lang.String fields) {
return (Failover) super.setFields(fields);
}
@Override
public Failover setKey(java.lang.String key) {
return (Failover) super.setKey(key);
}
@Override
public Failover setOauthToken(java.lang.String oauthToken) {
return (Failover) super.setOauthToken(oauthToken);
}
@Override
public Failover setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Failover) super.setPrettyPrint(prettyPrint);
}
@Override
public Failover setQuotaUser(java.lang.String quotaUser) {
return (Failover) super.setQuotaUser(quotaUser);
}
@Override
public Failover setUploadType(java.lang.String uploadType) {
return (Failover) super.setUploadType(uploadType);
}
@Override
public Failover setUploadProtocol(java.lang.String uploadProtocol) {
return (Failover) super.setUploadProtocol(uploadProtocol);
}
/** ID of the project that contains the read replica. */
@com.google.api.client.util.Key
private java.lang.String project;
/** ID of the project that contains the read replica.
*/
public java.lang.String getProject() {
return project;
}
/** ID of the project that contains the read replica. */
public Failover setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Failover setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Failover set(String parameterName, Object value) {
return (Failover) super.set(parameterName, value);
}
}
/**
* Retrieves a resource containing information about a Cloud SQL instance.
*
* Create a request for the method "instances.get".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @return the request
*/
public Get get(java.lang.String project, java.lang.String instance) throws java.io.IOException {
Get result = new Get(project, instance);
initialize(result);
return result;
}
public class Get extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}";
/**
* Retrieves a resource containing information about a Cloud SQL instance.
*
* Create a request for the method "instances.get".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @since 1.13
*/
protected Get(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sql.model.DatabaseInstance.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Get setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Database instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Database instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Database instance ID. This does not include the project ID. */
public Get setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Imports data into a Cloud SQL instance from a SQL dump or CSV file in Cloud Storage.
*
* Create a request for the method "instances.import".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link SQLAdminImport#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.InstancesImportRequest}
* @return the request
*/
public SQLAdminImport sqlImport(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.InstancesImportRequest content) throws java.io.IOException {
SQLAdminImport result = new SQLAdminImport(project, instance, content);
initialize(result);
return result;
}
public class SQLAdminImport extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/import";
/**
* Imports data into a Cloud SQL instance from a SQL dump or CSV file in Cloud Storage.
*
* Create a request for the method "instances.import".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link SQLAdminImport#execute()} method to invoke the remote operation.
* {@link SQLAdminImport#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.InstancesImportRequest}
* @since 1.13
*/
protected SQLAdminImport(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.InstancesImportRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public SQLAdminImport set$Xgafv(java.lang.String $Xgafv) {
return (SQLAdminImport) super.set$Xgafv($Xgafv);
}
@Override
public SQLAdminImport setAccessToken(java.lang.String accessToken) {
return (SQLAdminImport) super.setAccessToken(accessToken);
}
@Override
public SQLAdminImport setAlt(java.lang.String alt) {
return (SQLAdminImport) super.setAlt(alt);
}
@Override
public SQLAdminImport setCallback(java.lang.String callback) {
return (SQLAdminImport) super.setCallback(callback);
}
@Override
public SQLAdminImport setFields(java.lang.String fields) {
return (SQLAdminImport) super.setFields(fields);
}
@Override
public SQLAdminImport setKey(java.lang.String key) {
return (SQLAdminImport) super.setKey(key);
}
@Override
public SQLAdminImport setOauthToken(java.lang.String oauthToken) {
return (SQLAdminImport) super.setOauthToken(oauthToken);
}
@Override
public SQLAdminImport setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SQLAdminImport) super.setPrettyPrint(prettyPrint);
}
@Override
public SQLAdminImport setQuotaUser(java.lang.String quotaUser) {
return (SQLAdminImport) super.setQuotaUser(quotaUser);
}
@Override
public SQLAdminImport setUploadType(java.lang.String uploadType) {
return (SQLAdminImport) super.setUploadType(uploadType);
}
@Override
public SQLAdminImport setUploadProtocol(java.lang.String uploadProtocol) {
return (SQLAdminImport) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public SQLAdminImport setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public SQLAdminImport setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public SQLAdminImport set(String parameterName, Object value) {
return (SQLAdminImport) super.set(parameterName, value);
}
}
/**
* Creates a new Cloud SQL instance.
*
* Create a request for the method "instances.insert".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project to which the newly created Cloud SQL instances should belong.
* @param content the {@link com.google.api.services.sql.model.DatabaseInstance}
* @return the request
*/
public Insert insert(java.lang.String project, com.google.api.services.sql.model.DatabaseInstance content) throws java.io.IOException {
Insert result = new Insert(project, content);
initialize(result);
return result;
}
public class Insert extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances";
/**
* Creates a new Cloud SQL instance.
*
* Create a request for the method "instances.insert".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project to which the newly created Cloud SQL instances should belong.
* @param content the {@link com.google.api.services.sql.model.DatabaseInstance}
* @since 1.13
*/
protected Insert(java.lang.String project, com.google.api.services.sql.model.DatabaseInstance content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/**
* Project ID of the project to which the newly created Cloud SQL instances should belong.
*/
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project to which the newly created Cloud SQL instances should belong.
*/
public java.lang.String getProject() {
return project;
}
/**
* Project ID of the project to which the newly created Cloud SQL instances should belong.
*/
public Insert setProject(java.lang.String project) {
this.project = project;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Lists instances under a given project.
*
* Create a request for the method "instances.list".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project for which to list Cloud SQL instances.
* @return the request
*/
public List list(java.lang.String project) throws java.io.IOException {
List result = new List(project);
initialize(result);
return result;
}
public class List extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances";
/**
* Lists instances under a given project.
*
* Create a request for the method "instances.list".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project for which to list Cloud SQL instances.
* @since 1.13
*/
protected List(java.lang.String project) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sql.model.InstancesListResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project for which to list Cloud SQL instances. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project for which to list Cloud SQL instances.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project for which to list Cloud SQL instances. */
public List setProject(java.lang.String project) {
this.project = project;
return this;
}
/**
* A filter expression that filters resources listed in the response. The expression is in the
* form of field:value. For example, 'instanceType:CLOUD_SQL_INSTANCE'. Fields can be nested
* as needed as per their JSON representation, such as 'settings.userLabels.auto_start:true'.
* Multiple filter queries are space-separated. For example. 'state:RUNNABLE
* instanceType:CLOUD_SQL_INSTANCE'. By default, each expression is an AND expression.
* However, you can include AND and OR expressions explicitly.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter expression that filters resources listed in the response. The expression is in the form of
field:value. For example, 'instanceType:CLOUD_SQL_INSTANCE'. Fields can be nested as needed as per
their JSON representation, such as 'settings.userLabels.auto_start:true'. Multiple filter queries
are space-separated. For example. 'state:RUNNABLE instanceType:CLOUD_SQL_INSTANCE'. By default,
each expression is an AND expression. However, you can include AND and OR expressions explicitly.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter expression that filters resources listed in the response. The expression is in the
* form of field:value. For example, 'instanceType:CLOUD_SQL_INSTANCE'. Fields can be nested
* as needed as per their JSON representation, such as 'settings.userLabels.auto_start:true'.
* Multiple filter queries are space-separated. For example. 'state:RUNNABLE
* instanceType:CLOUD_SQL_INSTANCE'. By default, each expression is an AND expression.
* However, you can include AND and OR expressions explicitly.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The maximum number of results to return per response. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of results to return per response.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** The maximum number of results to return per response. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* A previously-returned page token representing part of the larger set of results to view.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A previously-returned page token representing part of the larger set of results to view.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A previously-returned page token representing part of the larger set of results to view.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Lists all of the trusted Certificate Authorities (CAs) for the specified instance. There can be
* up to three CAs listed: the CA that was used to sign the certificate that is currently in use, a
* CA that has been added but not yet used to sign a certificate, and a CA used to sign a
* certificate that has previously rotated out.
*
* Create a request for the method "instances.listServerCas".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link ListServerCas#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @return the request
*/
public ListServerCas listServerCas(java.lang.String project, java.lang.String instance) throws java.io.IOException {
ListServerCas result = new ListServerCas(project, instance);
initialize(result);
return result;
}
public class ListServerCas extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/listServerCas";
/**
* Lists all of the trusted Certificate Authorities (CAs) for the specified instance. There can be
* up to three CAs listed: the CA that was used to sign the certificate that is currently in use,
* a CA that has been added but not yet used to sign a certificate, and a CA used to sign a
* certificate that has previously rotated out.
*
* Create a request for the method "instances.listServerCas".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link ListServerCas#execute()} method to invoke the remote operation.
* {@link ListServerCas#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientR
* equest)} must be called to initialize this instance immediately after invoking the constructor.
*
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @since 1.13
*/
protected ListServerCas(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sql.model.InstancesListServerCasResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ListServerCas set$Xgafv(java.lang.String $Xgafv) {
return (ListServerCas) super.set$Xgafv($Xgafv);
}
@Override
public ListServerCas setAccessToken(java.lang.String accessToken) {
return (ListServerCas) super.setAccessToken(accessToken);
}
@Override
public ListServerCas setAlt(java.lang.String alt) {
return (ListServerCas) super.setAlt(alt);
}
@Override
public ListServerCas setCallback(java.lang.String callback) {
return (ListServerCas) super.setCallback(callback);
}
@Override
public ListServerCas setFields(java.lang.String fields) {
return (ListServerCas) super.setFields(fields);
}
@Override
public ListServerCas setKey(java.lang.String key) {
return (ListServerCas) super.setKey(key);
}
@Override
public ListServerCas setOauthToken(java.lang.String oauthToken) {
return (ListServerCas) super.setOauthToken(oauthToken);
}
@Override
public ListServerCas setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListServerCas) super.setPrettyPrint(prettyPrint);
}
@Override
public ListServerCas setQuotaUser(java.lang.String quotaUser) {
return (ListServerCas) super.setQuotaUser(quotaUser);
}
@Override
public ListServerCas setUploadType(java.lang.String uploadType) {
return (ListServerCas) super.setUploadType(uploadType);
}
@Override
public ListServerCas setUploadProtocol(java.lang.String uploadProtocol) {
return (ListServerCas) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public ListServerCas setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public ListServerCas setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public ListServerCas set(String parameterName, Object value) {
return (ListServerCas) super.set(parameterName, value);
}
}
/**
* Updates settings of a Cloud SQL instance. This method supports patch semantics.
*
* Create a request for the method "instances.patch".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.DatabaseInstance}
* @return the request
*/
public Patch patch(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.DatabaseInstance content) throws java.io.IOException {
Patch result = new Patch(project, instance, content);
initialize(result);
return result;
}
public class Patch extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}";
/**
* Updates settings of a Cloud SQL instance. This method supports patch semantics.
*
* Create a request for the method "instances.patch".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.DatabaseInstance}
* @since 1.13
*/
protected Patch(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.DatabaseInstance content) {
super(SQLAdmin.this, "PATCH", REST_PATH, content, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Patch setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Patch setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Promotes the read replica instance to be a stand-alone Cloud SQL instance. Using this operation
* might cause your instance to restart.
*
* Create a request for the method "instances.promoteReplica".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link PromoteReplica#execute()} method to invoke the remote operation.
*
* @param project ID of the project that contains the read replica.
* @param instance Cloud SQL read replica instance name.
* @return the request
*/
public PromoteReplica promoteReplica(java.lang.String project, java.lang.String instance) throws java.io.IOException {
PromoteReplica result = new PromoteReplica(project, instance);
initialize(result);
return result;
}
public class PromoteReplica extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/promoteReplica";
/**
* Promotes the read replica instance to be a stand-alone Cloud SQL instance. Using this operation
* might cause your instance to restart.
*
* Create a request for the method "instances.promoteReplica".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link PromoteReplica#execute()} method to invoke the remote operation.
* {@link PromoteReplica#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param project ID of the project that contains the read replica.
* @param instance Cloud SQL read replica instance name.
* @since 1.13
*/
protected PromoteReplica(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "POST", REST_PATH, null, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public PromoteReplica set$Xgafv(java.lang.String $Xgafv) {
return (PromoteReplica) super.set$Xgafv($Xgafv);
}
@Override
public PromoteReplica setAccessToken(java.lang.String accessToken) {
return (PromoteReplica) super.setAccessToken(accessToken);
}
@Override
public PromoteReplica setAlt(java.lang.String alt) {
return (PromoteReplica) super.setAlt(alt);
}
@Override
public PromoteReplica setCallback(java.lang.String callback) {
return (PromoteReplica) super.setCallback(callback);
}
@Override
public PromoteReplica setFields(java.lang.String fields) {
return (PromoteReplica) super.setFields(fields);
}
@Override
public PromoteReplica setKey(java.lang.String key) {
return (PromoteReplica) super.setKey(key);
}
@Override
public PromoteReplica setOauthToken(java.lang.String oauthToken) {
return (PromoteReplica) super.setOauthToken(oauthToken);
}
@Override
public PromoteReplica setPrettyPrint(java.lang.Boolean prettyPrint) {
return (PromoteReplica) super.setPrettyPrint(prettyPrint);
}
@Override
public PromoteReplica setQuotaUser(java.lang.String quotaUser) {
return (PromoteReplica) super.setQuotaUser(quotaUser);
}
@Override
public PromoteReplica setUploadType(java.lang.String uploadType) {
return (PromoteReplica) super.setUploadType(uploadType);
}
@Override
public PromoteReplica setUploadProtocol(java.lang.String uploadProtocol) {
return (PromoteReplica) super.setUploadProtocol(uploadProtocol);
}
/** ID of the project that contains the read replica. */
@com.google.api.client.util.Key
private java.lang.String project;
/** ID of the project that contains the read replica.
*/
public java.lang.String getProject() {
return project;
}
/** ID of the project that contains the read replica. */
public PromoteReplica setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL read replica instance name. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL read replica instance name.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL read replica instance name. */
public PromoteReplica setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public PromoteReplica set(String parameterName, Object value) {
return (PromoteReplica) super.set(parameterName, value);
}
}
/**
* Deletes all client certificates and generates a new server SSL certificate for the instance.
*
* Create a request for the method "instances.resetSslConfig".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link ResetSslConfig#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @return the request
*/
public ResetSslConfig resetSslConfig(java.lang.String project, java.lang.String instance) throws java.io.IOException {
ResetSslConfig result = new ResetSslConfig(project, instance);
initialize(result);
return result;
}
public class ResetSslConfig extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/resetSslConfig";
/**
* Deletes all client certificates and generates a new server SSL certificate for the instance.
*
* Create a request for the method "instances.resetSslConfig".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link ResetSslConfig#execute()} method to invoke the remote operation.
* {@link ResetSslConfig#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @since 1.13
*/
protected ResetSslConfig(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "POST", REST_PATH, null, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public ResetSslConfig set$Xgafv(java.lang.String $Xgafv) {
return (ResetSslConfig) super.set$Xgafv($Xgafv);
}
@Override
public ResetSslConfig setAccessToken(java.lang.String accessToken) {
return (ResetSslConfig) super.setAccessToken(accessToken);
}
@Override
public ResetSslConfig setAlt(java.lang.String alt) {
return (ResetSslConfig) super.setAlt(alt);
}
@Override
public ResetSslConfig setCallback(java.lang.String callback) {
return (ResetSslConfig) super.setCallback(callback);
}
@Override
public ResetSslConfig setFields(java.lang.String fields) {
return (ResetSslConfig) super.setFields(fields);
}
@Override
public ResetSslConfig setKey(java.lang.String key) {
return (ResetSslConfig) super.setKey(key);
}
@Override
public ResetSslConfig setOauthToken(java.lang.String oauthToken) {
return (ResetSslConfig) super.setOauthToken(oauthToken);
}
@Override
public ResetSslConfig setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ResetSslConfig) super.setPrettyPrint(prettyPrint);
}
@Override
public ResetSslConfig setQuotaUser(java.lang.String quotaUser) {
return (ResetSslConfig) super.setQuotaUser(quotaUser);
}
@Override
public ResetSslConfig setUploadType(java.lang.String uploadType) {
return (ResetSslConfig) super.setUploadType(uploadType);
}
@Override
public ResetSslConfig setUploadProtocol(java.lang.String uploadProtocol) {
return (ResetSslConfig) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public ResetSslConfig setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public ResetSslConfig setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public ResetSslConfig set(String parameterName, Object value) {
return (ResetSslConfig) super.set(parameterName, value);
}
}
/**
* Restarts a Cloud SQL instance.
*
* Create a request for the method "instances.restart".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link Restart#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance to be restarted.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @return the request
*/
public Restart restart(java.lang.String project, java.lang.String instance) throws java.io.IOException {
Restart result = new Restart(project, instance);
initialize(result);
return result;
}
public class Restart extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/restart";
/**
* Restarts a Cloud SQL instance.
*
* Create a request for the method "instances.restart".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link Restart#execute()} method to invoke the remote operation.
* {@link
* Restart#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance to be restarted.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @since 1.13
*/
protected Restart(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "POST", REST_PATH, null, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Restart set$Xgafv(java.lang.String $Xgafv) {
return (Restart) super.set$Xgafv($Xgafv);
}
@Override
public Restart setAccessToken(java.lang.String accessToken) {
return (Restart) super.setAccessToken(accessToken);
}
@Override
public Restart setAlt(java.lang.String alt) {
return (Restart) super.setAlt(alt);
}
@Override
public Restart setCallback(java.lang.String callback) {
return (Restart) super.setCallback(callback);
}
@Override
public Restart setFields(java.lang.String fields) {
return (Restart) super.setFields(fields);
}
@Override
public Restart setKey(java.lang.String key) {
return (Restart) super.setKey(key);
}
@Override
public Restart setOauthToken(java.lang.String oauthToken) {
return (Restart) super.setOauthToken(oauthToken);
}
@Override
public Restart setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Restart) super.setPrettyPrint(prettyPrint);
}
@Override
public Restart setQuotaUser(java.lang.String quotaUser) {
return (Restart) super.setQuotaUser(quotaUser);
}
@Override
public Restart setUploadType(java.lang.String uploadType) {
return (Restart) super.setUploadType(uploadType);
}
@Override
public Restart setUploadProtocol(java.lang.String uploadProtocol) {
return (Restart) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance to be restarted. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance to be restarted.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance to be restarted. */
public Restart setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Restart setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Restart set(String parameterName, Object value) {
return (Restart) super.set(parameterName, value);
}
}
/**
* Restores a backup of a Cloud SQL instance. Using this operation might cause your instance to
* restart.
*
* Create a request for the method "instances.restoreBackup".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link RestoreBackup#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.InstancesRestoreBackupRequest}
* @return the request
*/
public RestoreBackup restoreBackup(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.InstancesRestoreBackupRequest content) throws java.io.IOException {
RestoreBackup result = new RestoreBackup(project, instance, content);
initialize(result);
return result;
}
public class RestoreBackup extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/restoreBackup";
/**
* Restores a backup of a Cloud SQL instance. Using this operation might cause your instance to
* restart.
*
* Create a request for the method "instances.restoreBackup".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link RestoreBackup#execute()} method to invoke the remote operation.
* {@link RestoreBackup#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientR
* equest)} must be called to initialize this instance immediately after invoking the constructor.
*
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.InstancesRestoreBackupRequest}
* @since 1.13
*/
protected RestoreBackup(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.InstancesRestoreBackupRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public RestoreBackup set$Xgafv(java.lang.String $Xgafv) {
return (RestoreBackup) super.set$Xgafv($Xgafv);
}
@Override
public RestoreBackup setAccessToken(java.lang.String accessToken) {
return (RestoreBackup) super.setAccessToken(accessToken);
}
@Override
public RestoreBackup setAlt(java.lang.String alt) {
return (RestoreBackup) super.setAlt(alt);
}
@Override
public RestoreBackup setCallback(java.lang.String callback) {
return (RestoreBackup) super.setCallback(callback);
}
@Override
public RestoreBackup setFields(java.lang.String fields) {
return (RestoreBackup) super.setFields(fields);
}
@Override
public RestoreBackup setKey(java.lang.String key) {
return (RestoreBackup) super.setKey(key);
}
@Override
public RestoreBackup setOauthToken(java.lang.String oauthToken) {
return (RestoreBackup) super.setOauthToken(oauthToken);
}
@Override
public RestoreBackup setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RestoreBackup) super.setPrettyPrint(prettyPrint);
}
@Override
public RestoreBackup setQuotaUser(java.lang.String quotaUser) {
return (RestoreBackup) super.setQuotaUser(quotaUser);
}
@Override
public RestoreBackup setUploadType(java.lang.String uploadType) {
return (RestoreBackup) super.setUploadType(uploadType);
}
@Override
public RestoreBackup setUploadProtocol(java.lang.String uploadProtocol) {
return (RestoreBackup) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public RestoreBackup setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public RestoreBackup setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public RestoreBackup set(String parameterName, Object value) {
return (RestoreBackup) super.set(parameterName, value);
}
}
/**
* Rotates the server certificate to one signed by the Certificate Authority (CA) version previously
* added with the addServerCA method.
*
* Create a request for the method "instances.rotateServerCa".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link RotateServerCa#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.InstancesRotateServerCaRequest}
* @return the request
*/
public RotateServerCa rotateServerCa(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.InstancesRotateServerCaRequest content) throws java.io.IOException {
RotateServerCa result = new RotateServerCa(project, instance, content);
initialize(result);
return result;
}
public class RotateServerCa extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/rotateServerCa";
/**
* Rotates the server certificate to one signed by the Certificate Authority (CA) version
* previously added with the addServerCA method.
*
* Create a request for the method "instances.rotateServerCa".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link RotateServerCa#execute()} method to invoke the remote operation.
* {@link RotateServerCa#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.InstancesRotateServerCaRequest}
* @since 1.13
*/
protected RotateServerCa(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.InstancesRotateServerCaRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public RotateServerCa set$Xgafv(java.lang.String $Xgafv) {
return (RotateServerCa) super.set$Xgafv($Xgafv);
}
@Override
public RotateServerCa setAccessToken(java.lang.String accessToken) {
return (RotateServerCa) super.setAccessToken(accessToken);
}
@Override
public RotateServerCa setAlt(java.lang.String alt) {
return (RotateServerCa) super.setAlt(alt);
}
@Override
public RotateServerCa setCallback(java.lang.String callback) {
return (RotateServerCa) super.setCallback(callback);
}
@Override
public RotateServerCa setFields(java.lang.String fields) {
return (RotateServerCa) super.setFields(fields);
}
@Override
public RotateServerCa setKey(java.lang.String key) {
return (RotateServerCa) super.setKey(key);
}
@Override
public RotateServerCa setOauthToken(java.lang.String oauthToken) {
return (RotateServerCa) super.setOauthToken(oauthToken);
}
@Override
public RotateServerCa setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RotateServerCa) super.setPrettyPrint(prettyPrint);
}
@Override
public RotateServerCa setQuotaUser(java.lang.String quotaUser) {
return (RotateServerCa) super.setQuotaUser(quotaUser);
}
@Override
public RotateServerCa setUploadType(java.lang.String uploadType) {
return (RotateServerCa) super.setUploadType(uploadType);
}
@Override
public RotateServerCa setUploadProtocol(java.lang.String uploadProtocol) {
return (RotateServerCa) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public RotateServerCa setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public RotateServerCa setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public RotateServerCa set(String parameterName, Object value) {
return (RotateServerCa) super.set(parameterName, value);
}
}
/**
* Starts the replication in the read replica instance.
*
* Create a request for the method "instances.startReplica".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link StartReplica#execute()} method to invoke the remote operation.
*
* @param project ID of the project that contains the read replica.
* @param instance Cloud SQL read replica instance name.
* @return the request
*/
public StartReplica startReplica(java.lang.String project, java.lang.String instance) throws java.io.IOException {
StartReplica result = new StartReplica(project, instance);
initialize(result);
return result;
}
public class StartReplica extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/startReplica";
/**
* Starts the replication in the read replica instance.
*
* Create a request for the method "instances.startReplica".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link StartReplica#execute()} method to invoke the remote operation.
* {@link
* StartReplica#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project ID of the project that contains the read replica.
* @param instance Cloud SQL read replica instance name.
* @since 1.13
*/
protected StartReplica(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "POST", REST_PATH, null, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public StartReplica set$Xgafv(java.lang.String $Xgafv) {
return (StartReplica) super.set$Xgafv($Xgafv);
}
@Override
public StartReplica setAccessToken(java.lang.String accessToken) {
return (StartReplica) super.setAccessToken(accessToken);
}
@Override
public StartReplica setAlt(java.lang.String alt) {
return (StartReplica) super.setAlt(alt);
}
@Override
public StartReplica setCallback(java.lang.String callback) {
return (StartReplica) super.setCallback(callback);
}
@Override
public StartReplica setFields(java.lang.String fields) {
return (StartReplica) super.setFields(fields);
}
@Override
public StartReplica setKey(java.lang.String key) {
return (StartReplica) super.setKey(key);
}
@Override
public StartReplica setOauthToken(java.lang.String oauthToken) {
return (StartReplica) super.setOauthToken(oauthToken);
}
@Override
public StartReplica setPrettyPrint(java.lang.Boolean prettyPrint) {
return (StartReplica) super.setPrettyPrint(prettyPrint);
}
@Override
public StartReplica setQuotaUser(java.lang.String quotaUser) {
return (StartReplica) super.setQuotaUser(quotaUser);
}
@Override
public StartReplica setUploadType(java.lang.String uploadType) {
return (StartReplica) super.setUploadType(uploadType);
}
@Override
public StartReplica setUploadProtocol(java.lang.String uploadProtocol) {
return (StartReplica) super.setUploadProtocol(uploadProtocol);
}
/** ID of the project that contains the read replica. */
@com.google.api.client.util.Key
private java.lang.String project;
/** ID of the project that contains the read replica.
*/
public java.lang.String getProject() {
return project;
}
/** ID of the project that contains the read replica. */
public StartReplica setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL read replica instance name. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL read replica instance name.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL read replica instance name. */
public StartReplica setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public StartReplica set(String parameterName, Object value) {
return (StartReplica) super.set(parameterName, value);
}
}
/**
* Stops the replication in the read replica instance.
*
* Create a request for the method "instances.stopReplica".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link StopReplica#execute()} method to invoke the remote operation.
*
* @param project ID of the project that contains the read replica.
* @param instance Cloud SQL read replica instance name.
* @return the request
*/
public StopReplica stopReplica(java.lang.String project, java.lang.String instance) throws java.io.IOException {
StopReplica result = new StopReplica(project, instance);
initialize(result);
return result;
}
public class StopReplica extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/stopReplica";
/**
* Stops the replication in the read replica instance.
*
* Create a request for the method "instances.stopReplica".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link StopReplica#execute()} method to invoke the remote operation.
* {@link
* StopReplica#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project ID of the project that contains the read replica.
* @param instance Cloud SQL read replica instance name.
* @since 1.13
*/
protected StopReplica(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "POST", REST_PATH, null, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public StopReplica set$Xgafv(java.lang.String $Xgafv) {
return (StopReplica) super.set$Xgafv($Xgafv);
}
@Override
public StopReplica setAccessToken(java.lang.String accessToken) {
return (StopReplica) super.setAccessToken(accessToken);
}
@Override
public StopReplica setAlt(java.lang.String alt) {
return (StopReplica) super.setAlt(alt);
}
@Override
public StopReplica setCallback(java.lang.String callback) {
return (StopReplica) super.setCallback(callback);
}
@Override
public StopReplica setFields(java.lang.String fields) {
return (StopReplica) super.setFields(fields);
}
@Override
public StopReplica setKey(java.lang.String key) {
return (StopReplica) super.setKey(key);
}
@Override
public StopReplica setOauthToken(java.lang.String oauthToken) {
return (StopReplica) super.setOauthToken(oauthToken);
}
@Override
public StopReplica setPrettyPrint(java.lang.Boolean prettyPrint) {
return (StopReplica) super.setPrettyPrint(prettyPrint);
}
@Override
public StopReplica setQuotaUser(java.lang.String quotaUser) {
return (StopReplica) super.setQuotaUser(quotaUser);
}
@Override
public StopReplica setUploadType(java.lang.String uploadType) {
return (StopReplica) super.setUploadType(uploadType);
}
@Override
public StopReplica setUploadProtocol(java.lang.String uploadProtocol) {
return (StopReplica) super.setUploadProtocol(uploadProtocol);
}
/** ID of the project that contains the read replica. */
@com.google.api.client.util.Key
private java.lang.String project;
/** ID of the project that contains the read replica.
*/
public java.lang.String getProject() {
return project;
}
/** ID of the project that contains the read replica. */
public StopReplica setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL read replica instance name. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL read replica instance name.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL read replica instance name. */
public StopReplica setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public StopReplica set(String parameterName, Object value) {
return (StopReplica) super.set(parameterName, value);
}
}
/**
* Truncate MySQL general and slow query log tables
*
* Create a request for the method "instances.truncateLog".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link TruncateLog#execute()} method to invoke the remote operation.
*
* @param project Project ID of the Cloud SQL project.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.InstancesTruncateLogRequest}
* @return the request
*/
public TruncateLog truncateLog(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.InstancesTruncateLogRequest content) throws java.io.IOException {
TruncateLog result = new TruncateLog(project, instance, content);
initialize(result);
return result;
}
public class TruncateLog extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/truncateLog";
/**
* Truncate MySQL general and slow query log tables
*
* Create a request for the method "instances.truncateLog".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link TruncateLog#execute()} method to invoke the remote operation.
* {@link
* TruncateLog#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the Cloud SQL project.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.InstancesTruncateLogRequest}
* @since 1.13
*/
protected TruncateLog(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.InstancesTruncateLogRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public TruncateLog set$Xgafv(java.lang.String $Xgafv) {
return (TruncateLog) super.set$Xgafv($Xgafv);
}
@Override
public TruncateLog setAccessToken(java.lang.String accessToken) {
return (TruncateLog) super.setAccessToken(accessToken);
}
@Override
public TruncateLog setAlt(java.lang.String alt) {
return (TruncateLog) super.setAlt(alt);
}
@Override
public TruncateLog setCallback(java.lang.String callback) {
return (TruncateLog) super.setCallback(callback);
}
@Override
public TruncateLog setFields(java.lang.String fields) {
return (TruncateLog) super.setFields(fields);
}
@Override
public TruncateLog setKey(java.lang.String key) {
return (TruncateLog) super.setKey(key);
}
@Override
public TruncateLog setOauthToken(java.lang.String oauthToken) {
return (TruncateLog) super.setOauthToken(oauthToken);
}
@Override
public TruncateLog setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TruncateLog) super.setPrettyPrint(prettyPrint);
}
@Override
public TruncateLog setQuotaUser(java.lang.String quotaUser) {
return (TruncateLog) super.setQuotaUser(quotaUser);
}
@Override
public TruncateLog setUploadType(java.lang.String uploadType) {
return (TruncateLog) super.setUploadType(uploadType);
}
@Override
public TruncateLog setUploadProtocol(java.lang.String uploadProtocol) {
return (TruncateLog) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the Cloud SQL project. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the Cloud SQL project.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the Cloud SQL project. */
public TruncateLog setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public TruncateLog setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public TruncateLog set(String parameterName, Object value) {
return (TruncateLog) super.set(parameterName, value);
}
}
/**
* Updates settings of a Cloud SQL instance. Using this operation might cause your instance to
* restart.
*
* Create a request for the method "instances.update".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.DatabaseInstance}
* @return the request
*/
public Update update(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.DatabaseInstance content) throws java.io.IOException {
Update result = new Update(project, instance, content);
initialize(result);
return result;
}
public class Update extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}";
/**
* Updates settings of a Cloud SQL instance. Using this operation might cause your instance to
* restart.
*
* Create a request for the method "instances.update".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.DatabaseInstance}
* @since 1.13
*/
protected Update(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.DatabaseInstance content) {
super(SQLAdmin.this, "PUT", REST_PATH, content, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Update setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Update setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code SQLAdmin sql = new SQLAdmin(...);}
* {@code SQLAdmin.Operations.List request = sql.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Retrieves an instance operation that has been performed on an instance.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param operation Instance operation ID.
* @return the request
*/
public Get get(java.lang.String project, java.lang.String operation) throws java.io.IOException {
Get result = new Get(project, operation);
initialize(result);
return result;
}
public class Get extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/operations/{operation}";
/**
* Retrieves an instance operation that has been performed on an instance.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param operation Instance operation ID.
* @since 1.13
*/
protected Get(java.lang.String project, java.lang.String operation) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.operation = com.google.api.client.util.Preconditions.checkNotNull(operation, "Required parameter operation must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Get setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Instance operation ID. */
@com.google.api.client.util.Key
private java.lang.String operation;
/** Instance operation ID.
*/
public java.lang.String getOperation() {
return operation;
}
/** Instance operation ID. */
public Get setOperation(java.lang.String operation) {
this.operation = operation;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all instance operations that have been performed on the given Cloud SQL instance in the
* reverse chronological order of the start time.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @return the request
*/
public List list(java.lang.String project) throws java.io.IOException {
List result = new List(project);
initialize(result);
return result;
}
public class List extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/operations";
/**
* Lists all instance operations that have been performed on the given Cloud SQL instance in the
* reverse chronological order of the start time.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @since 1.13
*/
protected List(java.lang.String project) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sql.model.OperationsListResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public List setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public List setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** Maximum number of operations per response. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** Maximum number of operations per response.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** Maximum number of operations per response. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* A previously-returned page token representing part of the larger set of results to view.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A previously-returned page token representing part of the larger set of results to view.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A previously-returned page token representing part of the larger set of results to view.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Projects collection.
*
* The typical use is:
*
* {@code SQLAdmin sql = new SQLAdmin(...);}
* {@code SQLAdmin.Projects.List request = sql.projects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Projects projects() {
return new Projects();
}
/**
* The "projects" collection of methods.
*/
public class Projects {
/**
* An accessor for creating requests from the Instances collection.
*
* The typical use is:
*
* {@code SQLAdmin sql = new SQLAdmin(...);}
* {@code SQLAdmin.Instances.List request = sql.instances().list(parameters ...)}
*
*
* @return the resource collection
*/
public Instances instances() {
return new Instances();
}
/**
* The "instances" collection of methods.
*/
public class Instances {
/**
* Reschedules the maintenance on the given instance.
*
* Create a request for the method "instances.rescheduleMaintenance".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link RescheduleMaintenance#execute()} method to invoke the remote
* operation.
*
* @param project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.SqlInstancesRescheduleMaintenanceRequestBody}
* @return the request
*/
public RescheduleMaintenance rescheduleMaintenance(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.SqlInstancesRescheduleMaintenanceRequestBody content) throws java.io.IOException {
RescheduleMaintenance result = new RescheduleMaintenance(project, instance, content);
initialize(result);
return result;
}
public class RescheduleMaintenance extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/rescheduleMaintenance";
/**
* Reschedules the maintenance on the given instance.
*
* Create a request for the method "instances.rescheduleMaintenance".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link RescheduleMaintenance#execute()} method to invoke the remote
* operation. {@link RescheduleMaintenance#initialize(com.google.api.client.googleapis.service
* s.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.SqlInstancesRescheduleMaintenanceRequestBody}
* @since 1.13
*/
protected RescheduleMaintenance(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.SqlInstancesRescheduleMaintenanceRequestBody content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public RescheduleMaintenance set$Xgafv(java.lang.String $Xgafv) {
return (RescheduleMaintenance) super.set$Xgafv($Xgafv);
}
@Override
public RescheduleMaintenance setAccessToken(java.lang.String accessToken) {
return (RescheduleMaintenance) super.setAccessToken(accessToken);
}
@Override
public RescheduleMaintenance setAlt(java.lang.String alt) {
return (RescheduleMaintenance) super.setAlt(alt);
}
@Override
public RescheduleMaintenance setCallback(java.lang.String callback) {
return (RescheduleMaintenance) super.setCallback(callback);
}
@Override
public RescheduleMaintenance setFields(java.lang.String fields) {
return (RescheduleMaintenance) super.setFields(fields);
}
@Override
public RescheduleMaintenance setKey(java.lang.String key) {
return (RescheduleMaintenance) super.setKey(key);
}
@Override
public RescheduleMaintenance setOauthToken(java.lang.String oauthToken) {
return (RescheduleMaintenance) super.setOauthToken(oauthToken);
}
@Override
public RescheduleMaintenance setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RescheduleMaintenance) super.setPrettyPrint(prettyPrint);
}
@Override
public RescheduleMaintenance setQuotaUser(java.lang.String quotaUser) {
return (RescheduleMaintenance) super.setQuotaUser(quotaUser);
}
@Override
public RescheduleMaintenance setUploadType(java.lang.String uploadType) {
return (RescheduleMaintenance) super.setUploadType(uploadType);
}
@Override
public RescheduleMaintenance setUploadProtocol(java.lang.String uploadProtocol) {
return (RescheduleMaintenance) super.setUploadProtocol(uploadProtocol);
}
/** ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** ID of the project that contains the instance. */
public RescheduleMaintenance setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public RescheduleMaintenance setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public RescheduleMaintenance set(String parameterName, Object value) {
return (RescheduleMaintenance) super.set(parameterName, value);
}
}
/**
* Start External master migration.
*
* Create a request for the method "instances.startExternalSync".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link StartExternalSync#execute()} method to invoke the remote operation.
*
* @param project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @return the request
*/
public StartExternalSync startExternalSync(java.lang.String project, java.lang.String instance) throws java.io.IOException {
StartExternalSync result = new StartExternalSync(project, instance);
initialize(result);
return result;
}
public class StartExternalSync extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/startExternalSync";
/**
* Start External master migration.
*
* Create a request for the method "instances.startExternalSync".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link StartExternalSync#execute()} method to invoke the remote operation.
* {@link StartExternalSync#initialize(com.google.api.client.googleapis.services.AbstractGoogl
* eClientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @since 1.13
*/
protected StartExternalSync(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "POST", REST_PATH, null, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public StartExternalSync set$Xgafv(java.lang.String $Xgafv) {
return (StartExternalSync) super.set$Xgafv($Xgafv);
}
@Override
public StartExternalSync setAccessToken(java.lang.String accessToken) {
return (StartExternalSync) super.setAccessToken(accessToken);
}
@Override
public StartExternalSync setAlt(java.lang.String alt) {
return (StartExternalSync) super.setAlt(alt);
}
@Override
public StartExternalSync setCallback(java.lang.String callback) {
return (StartExternalSync) super.setCallback(callback);
}
@Override
public StartExternalSync setFields(java.lang.String fields) {
return (StartExternalSync) super.setFields(fields);
}
@Override
public StartExternalSync setKey(java.lang.String key) {
return (StartExternalSync) super.setKey(key);
}
@Override
public StartExternalSync setOauthToken(java.lang.String oauthToken) {
return (StartExternalSync) super.setOauthToken(oauthToken);
}
@Override
public StartExternalSync setPrettyPrint(java.lang.Boolean prettyPrint) {
return (StartExternalSync) super.setPrettyPrint(prettyPrint);
}
@Override
public StartExternalSync setQuotaUser(java.lang.String quotaUser) {
return (StartExternalSync) super.setQuotaUser(quotaUser);
}
@Override
public StartExternalSync setUploadType(java.lang.String uploadType) {
return (StartExternalSync) super.setUploadType(uploadType);
}
@Override
public StartExternalSync setUploadProtocol(java.lang.String uploadProtocol) {
return (StartExternalSync) super.setUploadProtocol(uploadProtocol);
}
/** ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** ID of the project that contains the instance. */
public StartExternalSync setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public StartExternalSync setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** External sync mode */
@com.google.api.client.util.Key
private java.lang.String syncMode;
/** External sync mode
*/
public java.lang.String getSyncMode() {
return syncMode;
}
/** External sync mode */
public StartExternalSync setSyncMode(java.lang.String syncMode) {
this.syncMode = syncMode;
return this;
}
@Override
public StartExternalSync set(String parameterName, Object value) {
return (StartExternalSync) super.set(parameterName, value);
}
}
/**
* Verify External master external sync settings.
*
* Create a request for the method "instances.verifyExternalSyncSettings".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link VerifyExternalSyncSettings#execute()} method to invoke the remote
* operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @return the request
*/
public VerifyExternalSyncSettings verifyExternalSyncSettings(java.lang.String project, java.lang.String instance) throws java.io.IOException {
VerifyExternalSyncSettings result = new VerifyExternalSyncSettings(project, instance);
initialize(result);
return result;
}
public class VerifyExternalSyncSettings extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/verifyExternalSyncSettings";
/**
* Verify External master external sync settings.
*
* Create a request for the method "instances.verifyExternalSyncSettings".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link VerifyExternalSyncSettings#execute()} method to invoke the remote
* operation. {@link VerifyExternalSyncSettings#initialize(com.google.api.client.googleapis.se
* rvices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @since 1.13
*/
protected VerifyExternalSyncSettings(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "POST", REST_PATH, null, com.google.api.services.sql.model.SqlInstancesVerifyExternalSyncSettingsResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public VerifyExternalSyncSettings set$Xgafv(java.lang.String $Xgafv) {
return (VerifyExternalSyncSettings) super.set$Xgafv($Xgafv);
}
@Override
public VerifyExternalSyncSettings setAccessToken(java.lang.String accessToken) {
return (VerifyExternalSyncSettings) super.setAccessToken(accessToken);
}
@Override
public VerifyExternalSyncSettings setAlt(java.lang.String alt) {
return (VerifyExternalSyncSettings) super.setAlt(alt);
}
@Override
public VerifyExternalSyncSettings setCallback(java.lang.String callback) {
return (VerifyExternalSyncSettings) super.setCallback(callback);
}
@Override
public VerifyExternalSyncSettings setFields(java.lang.String fields) {
return (VerifyExternalSyncSettings) super.setFields(fields);
}
@Override
public VerifyExternalSyncSettings setKey(java.lang.String key) {
return (VerifyExternalSyncSettings) super.setKey(key);
}
@Override
public VerifyExternalSyncSettings setOauthToken(java.lang.String oauthToken) {
return (VerifyExternalSyncSettings) super.setOauthToken(oauthToken);
}
@Override
public VerifyExternalSyncSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (VerifyExternalSyncSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public VerifyExternalSyncSettings setQuotaUser(java.lang.String quotaUser) {
return (VerifyExternalSyncSettings) super.setQuotaUser(quotaUser);
}
@Override
public VerifyExternalSyncSettings setUploadType(java.lang.String uploadType) {
return (VerifyExternalSyncSettings) super.setUploadType(uploadType);
}
@Override
public VerifyExternalSyncSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (VerifyExternalSyncSettings) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public VerifyExternalSyncSettings setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public VerifyExternalSyncSettings setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** External sync mode */
@com.google.api.client.util.Key
private java.lang.String syncMode;
/** External sync mode
*/
public java.lang.String getSyncMode() {
return syncMode;
}
/** External sync mode */
public VerifyExternalSyncSettings setSyncMode(java.lang.String syncMode) {
this.syncMode = syncMode;
return this;
}
/** Flag to enable verifying connection only */
@com.google.api.client.util.Key
private java.lang.Boolean verifyConnectionOnly;
/** Flag to enable verifying connection only
*/
public java.lang.Boolean getVerifyConnectionOnly() {
return verifyConnectionOnly;
}
/** Flag to enable verifying connection only */
public VerifyExternalSyncSettings setVerifyConnectionOnly(java.lang.Boolean verifyConnectionOnly) {
this.verifyConnectionOnly = verifyConnectionOnly;
return this;
}
@Override
public VerifyExternalSyncSettings set(String parameterName, Object value) {
return (VerifyExternalSyncSettings) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the SslCerts collection.
*
* The typical use is:
*
* {@code SQLAdmin sql = new SQLAdmin(...);}
* {@code SQLAdmin.SslCerts.List request = sql.sslCerts().list(parameters ...)}
*
*
* @return the resource collection
*/
public SslCerts sslCerts() {
return new SslCerts();
}
/**
* The "sslCerts" collection of methods.
*/
public class SslCerts {
/**
* Generates a short-lived X509 certificate containing the provided public key and signed by a
* private key specific to the target instance. Users may use the certificate to authenticate as
* themselves when connecting to the database.
*
* Create a request for the method "sslCerts.createEphemeral".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link CreateEphemeral#execute()} method to invoke the remote operation.
*
* @param project Project ID of the Cloud SQL project.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.SslCertsCreateEphemeralRequest}
* @return the request
*/
public CreateEphemeral createEphemeral(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.SslCertsCreateEphemeralRequest content) throws java.io.IOException {
CreateEphemeral result = new CreateEphemeral(project, instance, content);
initialize(result);
return result;
}
public class CreateEphemeral extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/createEphemeral";
/**
* Generates a short-lived X509 certificate containing the provided public key and signed by a
* private key specific to the target instance. Users may use the certificate to authenticate as
* themselves when connecting to the database.
*
* Create a request for the method "sslCerts.createEphemeral".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link CreateEphemeral#execute()} method to invoke the remote operation.
* {@link CreateEphemeral#initialize(com.google.api.client.googleapis.services.AbstractGoogleC
* lientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param project Project ID of the Cloud SQL project.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.SslCertsCreateEphemeralRequest}
* @since 1.13
*/
protected CreateEphemeral(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.SslCertsCreateEphemeralRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sql.model.SslCert.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public CreateEphemeral set$Xgafv(java.lang.String $Xgafv) {
return (CreateEphemeral) super.set$Xgafv($Xgafv);
}
@Override
public CreateEphemeral setAccessToken(java.lang.String accessToken) {
return (CreateEphemeral) super.setAccessToken(accessToken);
}
@Override
public CreateEphemeral setAlt(java.lang.String alt) {
return (CreateEphemeral) super.setAlt(alt);
}
@Override
public CreateEphemeral setCallback(java.lang.String callback) {
return (CreateEphemeral) super.setCallback(callback);
}
@Override
public CreateEphemeral setFields(java.lang.String fields) {
return (CreateEphemeral) super.setFields(fields);
}
@Override
public CreateEphemeral setKey(java.lang.String key) {
return (CreateEphemeral) super.setKey(key);
}
@Override
public CreateEphemeral setOauthToken(java.lang.String oauthToken) {
return (CreateEphemeral) super.setOauthToken(oauthToken);
}
@Override
public CreateEphemeral setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CreateEphemeral) super.setPrettyPrint(prettyPrint);
}
@Override
public CreateEphemeral setQuotaUser(java.lang.String quotaUser) {
return (CreateEphemeral) super.setQuotaUser(quotaUser);
}
@Override
public CreateEphemeral setUploadType(java.lang.String uploadType) {
return (CreateEphemeral) super.setUploadType(uploadType);
}
@Override
public CreateEphemeral setUploadProtocol(java.lang.String uploadProtocol) {
return (CreateEphemeral) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the Cloud SQL project. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the Cloud SQL project.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the Cloud SQL project. */
public CreateEphemeral setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public CreateEphemeral setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public CreateEphemeral set(String parameterName, Object value) {
return (CreateEphemeral) super.set(parameterName, value);
}
}
/**
* Deletes the SSL certificate. For First Generation instances, the certificate remains valid until
* the instance is restarted.
*
* Create a request for the method "sslCerts.delete".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param sha1Fingerprint Sha1 FingerPrint.
* @return the request
*/
public Delete delete(java.lang.String project, java.lang.String instance, java.lang.String sha1Fingerprint) throws java.io.IOException {
Delete result = new Delete(project, instance, sha1Fingerprint);
initialize(result);
return result;
}
public class Delete extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/sslCerts/{sha1Fingerprint}";
/**
* Deletes the SSL certificate. For First Generation instances, the certificate remains valid
* until the instance is restarted.
*
* Create a request for the method "sslCerts.delete".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param sha1Fingerprint Sha1 FingerPrint.
* @since 1.13
*/
protected Delete(java.lang.String project, java.lang.String instance, java.lang.String sha1Fingerprint) {
super(SQLAdmin.this, "DELETE", REST_PATH, null, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
this.sha1Fingerprint = com.google.api.client.util.Preconditions.checkNotNull(sha1Fingerprint, "Required parameter sha1Fingerprint must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Delete setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Delete setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** Sha1 FingerPrint. */
@com.google.api.client.util.Key
private java.lang.String sha1Fingerprint;
/** Sha1 FingerPrint.
*/
public java.lang.String getSha1Fingerprint() {
return sha1Fingerprint;
}
/** Sha1 FingerPrint. */
public Delete setSha1Fingerprint(java.lang.String sha1Fingerprint) {
this.sha1Fingerprint = sha1Fingerprint;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves a particular SSL certificate. Does not include the private key (required for usage).
* The private key must be saved from the response to initial creation.
*
* Create a request for the method "sslCerts.get".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param sha1Fingerprint Sha1 FingerPrint.
* @return the request
*/
public Get get(java.lang.String project, java.lang.String instance, java.lang.String sha1Fingerprint) throws java.io.IOException {
Get result = new Get(project, instance, sha1Fingerprint);
initialize(result);
return result;
}
public class Get extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/sslCerts/{sha1Fingerprint}";
/**
* Retrieves a particular SSL certificate. Does not include the private key (required for usage).
* The private key must be saved from the response to initial creation.
*
* Create a request for the method "sslCerts.get".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param sha1Fingerprint Sha1 FingerPrint.
* @since 1.13
*/
protected Get(java.lang.String project, java.lang.String instance, java.lang.String sha1Fingerprint) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sql.model.SslCert.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
this.sha1Fingerprint = com.google.api.client.util.Preconditions.checkNotNull(sha1Fingerprint, "Required parameter sha1Fingerprint must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Get setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Get setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** Sha1 FingerPrint. */
@com.google.api.client.util.Key
private java.lang.String sha1Fingerprint;
/** Sha1 FingerPrint.
*/
public java.lang.String getSha1Fingerprint() {
return sha1Fingerprint;
}
/** Sha1 FingerPrint. */
public Get setSha1Fingerprint(java.lang.String sha1Fingerprint) {
this.sha1Fingerprint = sha1Fingerprint;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Creates an SSL certificate and returns it along with the private key and server certificate
* authority. The new certificate will not be usable until the instance is restarted.
*
* Create a request for the method "sslCerts.insert".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.SslCertsInsertRequest}
* @return the request
*/
public Insert insert(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.SslCertsInsertRequest content) throws java.io.IOException {
Insert result = new Insert(project, instance, content);
initialize(result);
return result;
}
public class Insert extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/sslCerts";
/**
* Creates an SSL certificate and returns it along with the private key and server certificate
* authority. The new certificate will not be usable until the instance is restarted.
*
* Create a request for the method "sslCerts.insert".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.SslCertsInsertRequest}
* @since 1.13
*/
protected Insert(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.SslCertsInsertRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sql.model.SslCertsInsertResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Insert setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Insert setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Lists all of the current SSL certificates for the instance.
*
* Create a request for the method "sslCerts.list".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @return the request
*/
public List list(java.lang.String project, java.lang.String instance) throws java.io.IOException {
List result = new List(project, instance);
initialize(result);
return result;
}
public class List extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/sslCerts";
/**
* Lists all of the current SSL certificates for the instance.
*
* Create a request for the method "sslCerts.list".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @since 1.13
*/
protected List(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sql.model.SslCertsListResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public List setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public List setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Tiers collection.
*
* The typical use is:
*
* {@code SQLAdmin sql = new SQLAdmin(...);}
* {@code SQLAdmin.Tiers.List request = sql.tiers().list(parameters ...)}
*
*
* @return the resource collection
*/
public Tiers tiers() {
return new Tiers();
}
/**
* The "tiers" collection of methods.
*/
public class Tiers {
/**
* Lists all available machine types (tiers) for Cloud SQL, for example, db-n1-standard-1. For
* related information, see Pricing.
*
* Create a request for the method "tiers.list".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project for which to list tiers.
* @return the request
*/
public List list(java.lang.String project) throws java.io.IOException {
List result = new List(project);
initialize(result);
return result;
}
public class List extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/tiers";
/**
* Lists all available machine types (tiers) for Cloud SQL, for example, db-n1-standard-1. For
* related information, see Pricing.
*
* Create a request for the method "tiers.list".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project for which to list tiers.
* @since 1.13
*/
protected List(java.lang.String project) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sql.model.TiersListResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project for which to list tiers. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project for which to list tiers.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project for which to list tiers. */
public List setProject(java.lang.String project) {
this.project = project;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Users collection.
*
* The typical use is:
*
* {@code SQLAdmin sql = new SQLAdmin(...);}
* {@code SQLAdmin.Users.List request = sql.users().list(parameters ...)}
*
*
* @return the resource collection
*/
public Users users() {
return new Users();
}
/**
* The "users" collection of methods.
*/
public class Users {
/**
* Deletes a user from a Cloud SQL instance.
*
* Create a request for the method "users.delete".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @return the request
*/
public Delete delete(java.lang.String project, java.lang.String instance) throws java.io.IOException {
Delete result = new Delete(project, instance);
initialize(result);
return result;
}
public class Delete extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/users";
/**
* Deletes a user from a Cloud SQL instance.
*
* Create a request for the method "users.delete".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @since 1.13
*/
protected Delete(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "DELETE", REST_PATH, null, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Delete setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Database instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Database instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Database instance ID. This does not include the project ID. */
public Delete setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** Host of the user in the instance. */
@com.google.api.client.util.Key
private java.lang.String host;
/** Host of the user in the instance.
*/
public java.lang.String getHost() {
return host;
}
/** Host of the user in the instance. */
public Delete setHost(java.lang.String host) {
this.host = host;
return this;
}
/** Name of the user in the instance. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the user in the instance.
*/
public java.lang.String getName() {
return name;
}
/** Name of the user in the instance. */
public Delete setName(java.lang.String name) {
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Creates a new user in a Cloud SQL instance.
*
* Create a request for the method "users.insert".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.User}
* @return the request
*/
public Insert insert(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.User content) throws java.io.IOException {
Insert result = new Insert(project, instance, content);
initialize(result);
return result;
}
public class Insert extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/users";
/**
* Creates a new user in a Cloud SQL instance.
*
* Create a request for the method "users.insert".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.User}
* @since 1.13
*/
protected Insert(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.User content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Insert setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Database instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Database instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Database instance ID. This does not include the project ID. */
public Insert setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Lists users in the specified Cloud SQL instance.
*
* Create a request for the method "users.list".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @return the request
*/
public List list(java.lang.String project, java.lang.String instance) throws java.io.IOException {
List result = new List(project, instance);
initialize(result);
return result;
}
public class List extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/users";
/**
* Lists users in the specified Cloud SQL instance.
*
* Create a request for the method "users.list".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @since 1.13
*/
protected List(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sql.model.UsersListResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public List setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Database instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Database instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Database instance ID. This does not include the project ID. */
public List setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing user in a Cloud SQL instance.
*
* Create a request for the method "users.update".
*
* This request holds the parameters needed by the sql server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.User}
* @return the request
*/
public Update update(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.User content) throws java.io.IOException {
Update result = new Update(project, instance, content);
initialize(result);
return result;
}
public class Update extends SQLAdminRequest {
private static final String REST_PATH = "sql/v1beta4/projects/{project}/instances/{instance}/users";
/**
* Updates an existing user in a Cloud SQL instance.
*
* Create a request for the method "users.update".
*
* This request holds the parameters needed by the the sql server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sql.model.User}
* @since 1.13
*/
protected Update(java.lang.String project, java.lang.String instance, com.google.api.services.sql.model.User content) {
super(SQLAdmin.this, "PUT", REST_PATH, content, com.google.api.services.sql.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Update setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Database instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Database instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Database instance ID. This does not include the project ID. */
public Update setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** Optional. Host of the user in the instance. */
@com.google.api.client.util.Key
private java.lang.String host;
/** Optional. Host of the user in the instance.
*/
public java.lang.String getHost() {
return host;
}
/** Optional. Host of the user in the instance. */
public Update setHost(java.lang.String host) {
this.host = host;
return this;
}
/** Name of the user in the instance. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the user in the instance.
*/
public java.lang.String getName() {
return name;
}
/** Name of the user in the instance. */
public Update setName(java.lang.String name) {
this.name = name;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* Builder for {@link SQLAdmin}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link SQLAdmin}. */
@Override
public SQLAdmin build() {
return new SQLAdmin(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link SQLAdminRequestInitializer}.
*
* @since 1.12
*/
public Builder setSQLAdminRequestInitializer(
SQLAdminRequestInitializer sqladminRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(sqladminRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}