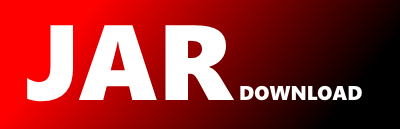
com.google.api.services.sqladmin.SQLAdmin Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.sqladmin;
/**
* Service definition for SQLAdmin (v1).
*
*
* API for Cloud SQL database instance management
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link SQLAdminRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class SQLAdmin extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Cloud SQL Admin API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://sqladmin.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://sqladmin.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public SQLAdmin(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
SQLAdmin(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the BackupRuns collection.
*
* The typical use is:
*
* {@code SQLAdmin sqladmin = new SQLAdmin(...);}
* {@code SQLAdmin.BackupRuns.List request = sqladmin.backupRuns().list(parameters ...)}
*
*
* @return the resource collection
*/
public BackupRuns backupRuns() {
return new BackupRuns();
}
/**
* The "backupRuns" collection of methods.
*/
public class BackupRuns {
/**
* Deletes the backup taken by a backup run.
*
* Create a request for the method "backupRuns.delete".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param id The ID of the backup run to delete. To find a backup run ID, use the
* [list](https://cloud.google.com/sql/docs/mysql/admin-api/rest/v1/backupRuns/list) method.
* @return the request
*/
public Delete delete(java.lang.String project, java.lang.String instance, java.lang.Long id) throws java.io.IOException {
Delete result = new Delete(project, instance, id);
initialize(result);
return result;
}
public class Delete extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/backupRuns/{id}";
/**
* Deletes the backup taken by a backup run.
*
* Create a request for the method "backupRuns.delete".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param id The ID of the backup run to delete. To find a backup run ID, use the
* [list](https://cloud.google.com/sql/docs/mysql/admin-api/rest/v1/backupRuns/list) method.
* @since 1.13
*/
protected Delete(java.lang.String project, java.lang.String instance, java.lang.Long id) {
super(SQLAdmin.this, "DELETE", REST_PATH, null, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Delete setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Delete setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/**
* The ID of the backup run to delete. To find a backup run ID, use the
* [list](https://cloud.google.com/sql/docs/mysql/admin-api/rest/v1/backupRuns/list) method.
*/
@com.google.api.client.util.Key
private java.lang.Long id;
/** The ID of the backup run to delete. To find a backup run ID, use the
[list](https://cloud.google.com/sql/docs/mysql/admin-api/rest/v1/backupRuns/list) method.
*/
public java.lang.Long getId() {
return id;
}
/**
* The ID of the backup run to delete. To find a backup run ID, use the
* [list](https://cloud.google.com/sql/docs/mysql/admin-api/rest/v1/backupRuns/list) method.
*/
public Delete setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves a resource containing information about a backup run.
*
* Create a request for the method "backupRuns.get".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param id The ID of this backup run.
* @return the request
*/
public Get get(java.lang.String project, java.lang.String instance, java.lang.Long id) throws java.io.IOException {
Get result = new Get(project, instance, id);
initialize(result);
return result;
}
public class Get extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/backupRuns/{id}";
/**
* Retrieves a resource containing information about a backup run.
*
* Create a request for the method "backupRuns.get".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param id The ID of this backup run.
* @since 1.13
*/
protected Get(java.lang.String project, java.lang.String instance, java.lang.Long id) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sqladmin.model.BackupRun.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Get setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Get setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** The ID of this backup run. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** The ID of this backup run.
*/
public java.lang.Long getId() {
return id;
}
/** The ID of this backup run. */
public Get setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Creates a new backup run on demand.
*
* Create a request for the method "backupRuns.insert".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.BackupRun}
* @return the request
*/
public Insert insert(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.BackupRun content) throws java.io.IOException {
Insert result = new Insert(project, instance, content);
initialize(result);
return result;
}
public class Insert extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/backupRuns";
/**
* Creates a new backup run on demand.
*
* Create a request for the method "backupRuns.insert".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.BackupRun}
* @since 1.13
*/
protected Insert(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.BackupRun content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Insert setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Insert setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Lists all backup runs associated with the project or a given instance and configuration in the
* reverse chronological order of the backup initiation time.
*
* Create a request for the method "backupRuns.list".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID, or "-" for all instances. This does not include the project ID.
* @return the request
*/
public List list(java.lang.String project, java.lang.String instance) throws java.io.IOException {
List result = new List(project, instance);
initialize(result);
return result;
}
public class List extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/backupRuns";
/**
* Lists all backup runs associated with the project or a given instance and configuration in the
* reverse chronological order of the backup initiation time.
*
* Create a request for the method "backupRuns.list".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID, or "-" for all instances. This does not include the project ID.
* @since 1.13
*/
protected List(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sqladmin.model.BackupRunsListResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public List setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID, or "-" for all instances. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID, or "-" for all instances. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID, or "-" for all instances. This does not include the project ID. */
public List setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** Maximum number of backup runs per response. */
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** Maximum number of backup runs per response.
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/** Maximum number of backup runs per response. */
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* A previously-returned page token representing part of the larger set of results to view.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A previously-returned page token representing part of the larger set of results to view.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A previously-returned page token representing part of the larger set of results to view.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Connect collection.
*
* The typical use is:
*
* {@code SQLAdmin sqladmin = new SQLAdmin(...);}
* {@code SQLAdmin.Connect.List request = sqladmin.connect().list(parameters ...)}
*
*
* @return the resource collection
*/
public Connect connect() {
return new Connect();
}
/**
* The "connect" collection of methods.
*/
public class Connect {
/**
* Generates a short-lived X509 certificate containing the provided public key and signed by a
* private key specific to the target instance. Users may use the certificate to authenticate as
* themselves when connecting to the database.
*
* Create a request for the method "connect.generateEphemeralCert".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link GenerateEphemeralCert#execute()} method to invoke the remote
* operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.GenerateEphemeralCertRequest}
* @return the request
*/
public GenerateEphemeralCert generateEphemeralCert(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.GenerateEphemeralCertRequest content) throws java.io.IOException {
GenerateEphemeralCert result = new GenerateEphemeralCert(project, instance, content);
initialize(result);
return result;
}
public class GenerateEphemeralCert extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}:generateEphemeralCert";
/**
* Generates a short-lived X509 certificate containing the provided public key and signed by a
* private key specific to the target instance. Users may use the certificate to authenticate as
* themselves when connecting to the database.
*
* Create a request for the method "connect.generateEphemeralCert".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link GenerateEphemeralCert#execute()} method to invoke the
* remote operation. {@link GenerateEphemeralCert#initialize(com.google.api.client.googleapis.
* services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.GenerateEphemeralCertRequest}
* @since 1.13
*/
protected GenerateEphemeralCert(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.GenerateEphemeralCertRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sqladmin.model.GenerateEphemeralCertResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public GenerateEphemeralCert set$Xgafv(java.lang.String $Xgafv) {
return (GenerateEphemeralCert) super.set$Xgafv($Xgafv);
}
@Override
public GenerateEphemeralCert setAccessToken(java.lang.String accessToken) {
return (GenerateEphemeralCert) super.setAccessToken(accessToken);
}
@Override
public GenerateEphemeralCert setAlt(java.lang.String alt) {
return (GenerateEphemeralCert) super.setAlt(alt);
}
@Override
public GenerateEphemeralCert setCallback(java.lang.String callback) {
return (GenerateEphemeralCert) super.setCallback(callback);
}
@Override
public GenerateEphemeralCert setFields(java.lang.String fields) {
return (GenerateEphemeralCert) super.setFields(fields);
}
@Override
public GenerateEphemeralCert setKey(java.lang.String key) {
return (GenerateEphemeralCert) super.setKey(key);
}
@Override
public GenerateEphemeralCert setOauthToken(java.lang.String oauthToken) {
return (GenerateEphemeralCert) super.setOauthToken(oauthToken);
}
@Override
public GenerateEphemeralCert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GenerateEphemeralCert) super.setPrettyPrint(prettyPrint);
}
@Override
public GenerateEphemeralCert setQuotaUser(java.lang.String quotaUser) {
return (GenerateEphemeralCert) super.setQuotaUser(quotaUser);
}
@Override
public GenerateEphemeralCert setUploadType(java.lang.String uploadType) {
return (GenerateEphemeralCert) super.setUploadType(uploadType);
}
@Override
public GenerateEphemeralCert setUploadProtocol(java.lang.String uploadProtocol) {
return (GenerateEphemeralCert) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public GenerateEphemeralCert setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public GenerateEphemeralCert setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public GenerateEphemeralCert set(String parameterName, Object value) {
return (GenerateEphemeralCert) super.set(parameterName, value);
}
}
/**
* Retrieves connect settings about a Cloud SQL instance.
*
* Create a request for the method "connect.get".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @return the request
*/
public Get get(java.lang.String project, java.lang.String instance) throws java.io.IOException {
Get result = new Get(project, instance);
initialize(result);
return result;
}
public class Get extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/connectSettings";
/**
* Retrieves connect settings about a Cloud SQL instance.
*
* Create a request for the method "connect.get".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @since 1.13
*/
protected Get(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sqladmin.model.ConnectSettings.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Get setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Get setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** Optional. Optional snapshot read timestamp to trade freshness for performance. */
@com.google.api.client.util.Key
private String readTime;
/** Optional. Optional snapshot read timestamp to trade freshness for performance.
*/
public String getReadTime() {
return readTime;
}
/** Optional. Optional snapshot read timestamp to trade freshness for performance. */
public Get setReadTime(String readTime) {
this.readTime = readTime;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Databases collection.
*
* The typical use is:
*
* {@code SQLAdmin sqladmin = new SQLAdmin(...);}
* {@code SQLAdmin.Databases.List request = sqladmin.databases().list(parameters ...)}
*
*
* @return the resource collection
*/
public Databases databases() {
return new Databases();
}
/**
* The "databases" collection of methods.
*/
public class Databases {
/**
* Deletes a database from a Cloud SQL instance.
*
* Create a request for the method "databases.delete".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param database Name of the database to be deleted in the instance.
* @return the request
*/
public Delete delete(java.lang.String project, java.lang.String instance, java.lang.String database) throws java.io.IOException {
Delete result = new Delete(project, instance, database);
initialize(result);
return result;
}
public class Delete extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/databases/{database}";
/**
* Deletes a database from a Cloud SQL instance.
*
* Create a request for the method "databases.delete".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param database Name of the database to be deleted in the instance.
* @since 1.13
*/
protected Delete(java.lang.String project, java.lang.String instance, java.lang.String database) {
super(SQLAdmin.this, "DELETE", REST_PATH, null, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
this.database = com.google.api.client.util.Preconditions.checkNotNull(database, "Required parameter database must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Delete setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Database instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Database instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Database instance ID. This does not include the project ID. */
public Delete setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** Name of the database to be deleted in the instance. */
@com.google.api.client.util.Key
private java.lang.String database;
/** Name of the database to be deleted in the instance.
*/
public java.lang.String getDatabase() {
return database;
}
/** Name of the database to be deleted in the instance. */
public Delete setDatabase(java.lang.String database) {
this.database = database;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves a resource containing information about a database inside a Cloud SQL instance.
*
* Create a request for the method "databases.get".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param database Name of the database in the instance.
* @return the request
*/
public Get get(java.lang.String project, java.lang.String instance, java.lang.String database) throws java.io.IOException {
Get result = new Get(project, instance, database);
initialize(result);
return result;
}
public class Get extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/databases/{database}";
/**
* Retrieves a resource containing information about a database inside a Cloud SQL instance.
*
* Create a request for the method "databases.get".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param database Name of the database in the instance.
* @since 1.13
*/
protected Get(java.lang.String project, java.lang.String instance, java.lang.String database) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sqladmin.model.Database.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
this.database = com.google.api.client.util.Preconditions.checkNotNull(database, "Required parameter database must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Get setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Database instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Database instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Database instance ID. This does not include the project ID. */
public Get setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** Name of the database in the instance. */
@com.google.api.client.util.Key
private java.lang.String database;
/** Name of the database in the instance.
*/
public java.lang.String getDatabase() {
return database;
}
/** Name of the database in the instance. */
public Get setDatabase(java.lang.String database) {
this.database = database;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Inserts a resource containing information about a database inside a Cloud SQL instance. **Note:**
* You can't modify the default character set and collation.
*
* Create a request for the method "databases.insert".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.Database}
* @return the request
*/
public Insert insert(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.Database content) throws java.io.IOException {
Insert result = new Insert(project, instance, content);
initialize(result);
return result;
}
public class Insert extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/databases";
/**
* Inserts a resource containing information about a database inside a Cloud SQL instance.
* **Note:** You can't modify the default character set and collation.
*
* Create a request for the method "databases.insert".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.Database}
* @since 1.13
*/
protected Insert(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.Database content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Insert setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Database instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Database instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Database instance ID. This does not include the project ID. */
public Insert setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Lists databases in the specified Cloud SQL instance.
*
* Create a request for the method "databases.list".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @return the request
*/
public List list(java.lang.String project, java.lang.String instance) throws java.io.IOException {
List result = new List(project, instance);
initialize(result);
return result;
}
public class List extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/databases";
/**
* Lists databases in the specified Cloud SQL instance.
*
* Create a request for the method "databases.list".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @since 1.13
*/
protected List(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sqladmin.model.DatabasesListResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public List setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public List setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Partially updates a resource containing information about a database inside a Cloud SQL instance.
* This method supports patch semantics.
*
* Create a request for the method "databases.patch".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param database Name of the database to be updated in the instance.
* @param content the {@link com.google.api.services.sqladmin.model.Database}
* @return the request
*/
public Patch patch(java.lang.String project, java.lang.String instance, java.lang.String database, com.google.api.services.sqladmin.model.Database content) throws java.io.IOException {
Patch result = new Patch(project, instance, database, content);
initialize(result);
return result;
}
public class Patch extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/databases/{database}";
/**
* Partially updates a resource containing information about a database inside a Cloud SQL
* instance. This method supports patch semantics.
*
* Create a request for the method "databases.patch".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param database Name of the database to be updated in the instance.
* @param content the {@link com.google.api.services.sqladmin.model.Database}
* @since 1.13
*/
protected Patch(java.lang.String project, java.lang.String instance, java.lang.String database, com.google.api.services.sqladmin.model.Database content) {
super(SQLAdmin.this, "PATCH", REST_PATH, content, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
this.database = com.google.api.client.util.Preconditions.checkNotNull(database, "Required parameter database must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Patch setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Database instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Database instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Database instance ID. This does not include the project ID. */
public Patch setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** Name of the database to be updated in the instance. */
@com.google.api.client.util.Key
private java.lang.String database;
/** Name of the database to be updated in the instance.
*/
public java.lang.String getDatabase() {
return database;
}
/** Name of the database to be updated in the instance. */
public Patch setDatabase(java.lang.String database) {
this.database = database;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates a resource containing information about a database inside a Cloud SQL instance.
*
* Create a request for the method "databases.update".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param database Name of the database to be updated in the instance.
* @param content the {@link com.google.api.services.sqladmin.model.Database}
* @return the request
*/
public Update update(java.lang.String project, java.lang.String instance, java.lang.String database, com.google.api.services.sqladmin.model.Database content) throws java.io.IOException {
Update result = new Update(project, instance, database, content);
initialize(result);
return result;
}
public class Update extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/databases/{database}";
/**
* Updates a resource containing information about a database inside a Cloud SQL instance.
*
* Create a request for the method "databases.update".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param database Name of the database to be updated in the instance.
* @param content the {@link com.google.api.services.sqladmin.model.Database}
* @since 1.13
*/
protected Update(java.lang.String project, java.lang.String instance, java.lang.String database, com.google.api.services.sqladmin.model.Database content) {
super(SQLAdmin.this, "PUT", REST_PATH, content, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
this.database = com.google.api.client.util.Preconditions.checkNotNull(database, "Required parameter database must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Update setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Database instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Database instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Database instance ID. This does not include the project ID. */
public Update setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** Name of the database to be updated in the instance. */
@com.google.api.client.util.Key
private java.lang.String database;
/** Name of the database to be updated in the instance.
*/
public java.lang.String getDatabase() {
return database;
}
/** Name of the database to be updated in the instance. */
public Update setDatabase(java.lang.String database) {
this.database = database;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Flags collection.
*
* The typical use is:
*
* {@code SQLAdmin sqladmin = new SQLAdmin(...);}
* {@code SQLAdmin.Flags.List request = sqladmin.flags().list(parameters ...)}
*
*
* @return the resource collection
*/
public Flags flags() {
return new Flags();
}
/**
* The "flags" collection of methods.
*/
public class Flags {
/**
* Lists all available database flags for Cloud SQL instances.
*
* Create a request for the method "flags.list".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends SQLAdminRequest {
private static final String REST_PATH = "v1/flags";
/**
* Lists all available database flags for Cloud SQL instances.
*
* Create a request for the method "flags.list".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sqladmin.model.FlagsListResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Database type and version you want to retrieve flags for. By default, this method returns
* flags for all database types and versions.
*/
@com.google.api.client.util.Key
private java.lang.String databaseVersion;
/** Database type and version you want to retrieve flags for. By default, this method returns flags for
all database types and versions.
*/
public java.lang.String getDatabaseVersion() {
return databaseVersion;
}
/**
* Database type and version you want to retrieve flags for. By default, this method returns
* flags for all database types and versions.
*/
public List setDatabaseVersion(java.lang.String databaseVersion) {
this.databaseVersion = databaseVersion;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Instances collection.
*
* The typical use is:
*
* {@code SQLAdmin sqladmin = new SQLAdmin(...);}
* {@code SQLAdmin.Instances.List request = sqladmin.instances().list(parameters ...)}
*
*
* @return the resource collection
*/
public Instances instances() {
return new Instances();
}
/**
* The "instances" collection of methods.
*/
public class Instances {
/**
* Lists all versions of server certificates and certificate authorities (CAs) for the specified
* instance. There can be up to three sets of certs listed: the certificate that is currently in
* use, a future that has been added but not yet used to sign a certificate, and a certificate that
* has been rotated out. For instances not using Certificate Authority Service (CAS) server CA, use
* ListServerCas instead.
*
* Create a request for the method "instances.ListServerCertificates".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link ListServerCertificates#execute()} method to invoke the remote
* operation.
*
* @param project Required. Project ID of the project that contains the instance.
* @param instance Required. Cloud SQL instance ID. This does not include the project ID.
* @return the request
*/
public ListServerCertificates listServerCertificates(java.lang.String project, java.lang.String instance) throws java.io.IOException {
ListServerCertificates result = new ListServerCertificates(project, instance);
initialize(result);
return result;
}
public class ListServerCertificates extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/listServerCertificates";
/**
* Lists all versions of server certificates and certificate authorities (CAs) for the specified
* instance. There can be up to three sets of certs listed: the certificate that is currently in
* use, a future that has been added but not yet used to sign a certificate, and a certificate
* that has been rotated out. For instances not using Certificate Authority Service (CAS) server
* CA, use ListServerCas instead.
*
* Create a request for the method "instances.ListServerCertificates".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link ListServerCertificates#execute()} method to invoke the
* remote operation. {@link ListServerCertificates#initialize(com.google.api.client.googleapis
* .services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param project Required. Project ID of the project that contains the instance.
* @param instance Required. Cloud SQL instance ID. This does not include the project ID.
* @since 1.13
*/
protected ListServerCertificates(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sqladmin.model.InstancesListServerCertificatesResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ListServerCertificates set$Xgafv(java.lang.String $Xgafv) {
return (ListServerCertificates) super.set$Xgafv($Xgafv);
}
@Override
public ListServerCertificates setAccessToken(java.lang.String accessToken) {
return (ListServerCertificates) super.setAccessToken(accessToken);
}
@Override
public ListServerCertificates setAlt(java.lang.String alt) {
return (ListServerCertificates) super.setAlt(alt);
}
@Override
public ListServerCertificates setCallback(java.lang.String callback) {
return (ListServerCertificates) super.setCallback(callback);
}
@Override
public ListServerCertificates setFields(java.lang.String fields) {
return (ListServerCertificates) super.setFields(fields);
}
@Override
public ListServerCertificates setKey(java.lang.String key) {
return (ListServerCertificates) super.setKey(key);
}
@Override
public ListServerCertificates setOauthToken(java.lang.String oauthToken) {
return (ListServerCertificates) super.setOauthToken(oauthToken);
}
@Override
public ListServerCertificates setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListServerCertificates) super.setPrettyPrint(prettyPrint);
}
@Override
public ListServerCertificates setQuotaUser(java.lang.String quotaUser) {
return (ListServerCertificates) super.setQuotaUser(quotaUser);
}
@Override
public ListServerCertificates setUploadType(java.lang.String uploadType) {
return (ListServerCertificates) super.setUploadType(uploadType);
}
@Override
public ListServerCertificates setUploadProtocol(java.lang.String uploadProtocol) {
return (ListServerCertificates) super.setUploadProtocol(uploadProtocol);
}
/** Required. Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Required. Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Required. Project ID of the project that contains the instance. */
public ListServerCertificates setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Required. Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Required. Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Required. Cloud SQL instance ID. This does not include the project ID. */
public ListServerCertificates setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public ListServerCertificates set(String parameterName, Object value) {
return (ListServerCertificates) super.set(parameterName, value);
}
}
/**
* Rotates the server certificate version to one previously added with the addServerCertificate
* method. For instances not using Certificate Authority Service (CAS) server CA, use RotateServerCa
* instead.
*
* Create a request for the method "instances.RotateServerCertificate".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link RotateServerCertificate#execute()} method to invoke the remote
* operation.
*
* @param project Required. Project ID of the project that contains the instance.
* @param instance Required. Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.InstancesRotateServerCertificateRequest}
* @return the request
*/
public RotateServerCertificate rotateServerCertificate(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.InstancesRotateServerCertificateRequest content) throws java.io.IOException {
RotateServerCertificate result = new RotateServerCertificate(project, instance, content);
initialize(result);
return result;
}
public class RotateServerCertificate extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/rotateServerCertificate";
/**
* Rotates the server certificate version to one previously added with the addServerCertificate
* method. For instances not using Certificate Authority Service (CAS) server CA, use
* RotateServerCa instead.
*
* Create a request for the method "instances.RotateServerCertificate".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link RotateServerCertificate#execute()} method to invoke the
* remote operation. {@link RotateServerCertificate#initialize(com.google.api.client.googleapi
* s.services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param project Required. Project ID of the project that contains the instance.
* @param instance Required. Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.InstancesRotateServerCertificateRequest}
* @since 1.13
*/
protected RotateServerCertificate(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.InstancesRotateServerCertificateRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public RotateServerCertificate set$Xgafv(java.lang.String $Xgafv) {
return (RotateServerCertificate) super.set$Xgafv($Xgafv);
}
@Override
public RotateServerCertificate setAccessToken(java.lang.String accessToken) {
return (RotateServerCertificate) super.setAccessToken(accessToken);
}
@Override
public RotateServerCertificate setAlt(java.lang.String alt) {
return (RotateServerCertificate) super.setAlt(alt);
}
@Override
public RotateServerCertificate setCallback(java.lang.String callback) {
return (RotateServerCertificate) super.setCallback(callback);
}
@Override
public RotateServerCertificate setFields(java.lang.String fields) {
return (RotateServerCertificate) super.setFields(fields);
}
@Override
public RotateServerCertificate setKey(java.lang.String key) {
return (RotateServerCertificate) super.setKey(key);
}
@Override
public RotateServerCertificate setOauthToken(java.lang.String oauthToken) {
return (RotateServerCertificate) super.setOauthToken(oauthToken);
}
@Override
public RotateServerCertificate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RotateServerCertificate) super.setPrettyPrint(prettyPrint);
}
@Override
public RotateServerCertificate setQuotaUser(java.lang.String quotaUser) {
return (RotateServerCertificate) super.setQuotaUser(quotaUser);
}
@Override
public RotateServerCertificate setUploadType(java.lang.String uploadType) {
return (RotateServerCertificate) super.setUploadType(uploadType);
}
@Override
public RotateServerCertificate setUploadProtocol(java.lang.String uploadProtocol) {
return (RotateServerCertificate) super.setUploadProtocol(uploadProtocol);
}
/** Required. Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Required. Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Required. Project ID of the project that contains the instance. */
public RotateServerCertificate setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Required. Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Required. Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Required. Cloud SQL instance ID. This does not include the project ID. */
public RotateServerCertificate setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public RotateServerCertificate set(String parameterName, Object value) {
return (RotateServerCertificate) super.set(parameterName, value);
}
}
/**
* Acquire a lease for the setup of SQL Server Reporting Services (SSRS).
*
* Create a request for the method "instances.acquireSsrsLease".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link AcquireSsrsLease#execute()} method to invoke the remote operation.
*
* @param project Required. Project ID of the project that contains the instance (Example: project-id).
* @param instance Required. Cloud SQL instance ID. This doesn't include the project ID. It's composed of lowercase
* letters, numbers, and hyphens, and it must start with a letter. The total length must be
* 98 characters or less (Example: instance-id).
* @param content the {@link com.google.api.services.sqladmin.model.InstancesAcquireSsrsLeaseRequest}
* @return the request
*/
public AcquireSsrsLease acquireSsrsLease(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.InstancesAcquireSsrsLeaseRequest content) throws java.io.IOException {
AcquireSsrsLease result = new AcquireSsrsLease(project, instance, content);
initialize(result);
return result;
}
public class AcquireSsrsLease extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/acquireSsrsLease";
/**
* Acquire a lease for the setup of SQL Server Reporting Services (SSRS).
*
* Create a request for the method "instances.acquireSsrsLease".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link AcquireSsrsLease#execute()} method to invoke the remote
* operation. {@link AcquireSsrsLease#initialize(com.google.api.client.googleapis.services.Abs
* tractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param project Required. Project ID of the project that contains the instance (Example: project-id).
* @param instance Required. Cloud SQL instance ID. This doesn't include the project ID. It's composed of lowercase
* letters, numbers, and hyphens, and it must start with a letter. The total length must be
* 98 characters or less (Example: instance-id).
* @param content the {@link com.google.api.services.sqladmin.model.InstancesAcquireSsrsLeaseRequest}
* @since 1.13
*/
protected AcquireSsrsLease(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.InstancesAcquireSsrsLeaseRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sqladmin.model.SqlInstancesAcquireSsrsLeaseResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public AcquireSsrsLease set$Xgafv(java.lang.String $Xgafv) {
return (AcquireSsrsLease) super.set$Xgafv($Xgafv);
}
@Override
public AcquireSsrsLease setAccessToken(java.lang.String accessToken) {
return (AcquireSsrsLease) super.setAccessToken(accessToken);
}
@Override
public AcquireSsrsLease setAlt(java.lang.String alt) {
return (AcquireSsrsLease) super.setAlt(alt);
}
@Override
public AcquireSsrsLease setCallback(java.lang.String callback) {
return (AcquireSsrsLease) super.setCallback(callback);
}
@Override
public AcquireSsrsLease setFields(java.lang.String fields) {
return (AcquireSsrsLease) super.setFields(fields);
}
@Override
public AcquireSsrsLease setKey(java.lang.String key) {
return (AcquireSsrsLease) super.setKey(key);
}
@Override
public AcquireSsrsLease setOauthToken(java.lang.String oauthToken) {
return (AcquireSsrsLease) super.setOauthToken(oauthToken);
}
@Override
public AcquireSsrsLease setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AcquireSsrsLease) super.setPrettyPrint(prettyPrint);
}
@Override
public AcquireSsrsLease setQuotaUser(java.lang.String quotaUser) {
return (AcquireSsrsLease) super.setQuotaUser(quotaUser);
}
@Override
public AcquireSsrsLease setUploadType(java.lang.String uploadType) {
return (AcquireSsrsLease) super.setUploadType(uploadType);
}
@Override
public AcquireSsrsLease setUploadProtocol(java.lang.String uploadProtocol) {
return (AcquireSsrsLease) super.setUploadProtocol(uploadProtocol);
}
/** Required. Project ID of the project that contains the instance (Example: project-id). */
@com.google.api.client.util.Key
private java.lang.String project;
/** Required. Project ID of the project that contains the instance (Example: project-id).
*/
public java.lang.String getProject() {
return project;
}
/** Required. Project ID of the project that contains the instance (Example: project-id). */
public AcquireSsrsLease setProject(java.lang.String project) {
this.project = project;
return this;
}
/**
* Required. Cloud SQL instance ID. This doesn't include the project ID. It's composed of
* lowercase letters, numbers, and hyphens, and it must start with a letter. The total length
* must be 98 characters or less (Example: instance-id).
*/
@com.google.api.client.util.Key
private java.lang.String instance;
/** Required. Cloud SQL instance ID. This doesn't include the project ID. It's composed of lowercase
letters, numbers, and hyphens, and it must start with a letter. The total length must be 98
characters or less (Example: instance-id).
*/
public java.lang.String getInstance() {
return instance;
}
/**
* Required. Cloud SQL instance ID. This doesn't include the project ID. It's composed of
* lowercase letters, numbers, and hyphens, and it must start with a letter. The total length
* must be 98 characters or less (Example: instance-id).
*/
public AcquireSsrsLease setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public AcquireSsrsLease set(String parameterName, Object value) {
return (AcquireSsrsLease) super.set(parameterName, value);
}
}
/**
* Adds a new trusted Certificate Authority (CA) version for the specified instance. Required to
* prepare for a certificate rotation. If a CA version was previously added but never used in a
* certificate rotation, this operation replaces that version. There cannot be more than one CA
* version waiting to be rotated in. For instances that have enabled Certificate Authority Service
* (CAS) based server CA, use AddServerCertificate to add a new server certificate.
*
* Create a request for the method "instances.addServerCa".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link AddServerCa#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @return the request
*/
public AddServerCa addServerCa(java.lang.String project, java.lang.String instance) throws java.io.IOException {
AddServerCa result = new AddServerCa(project, instance);
initialize(result);
return result;
}
public class AddServerCa extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/addServerCa";
/**
* Adds a new trusted Certificate Authority (CA) version for the specified instance. Required to
* prepare for a certificate rotation. If a CA version was previously added but never used in a
* certificate rotation, this operation replaces that version. There cannot be more than one CA
* version waiting to be rotated in. For instances that have enabled Certificate Authority Service
* (CAS) based server CA, use AddServerCertificate to add a new server certificate.
*
* Create a request for the method "instances.addServerCa".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link AddServerCa#execute()} method to invoke the remote
* operation. {@link
* AddServerCa#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @since 1.13
*/
protected AddServerCa(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "POST", REST_PATH, null, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public AddServerCa set$Xgafv(java.lang.String $Xgafv) {
return (AddServerCa) super.set$Xgafv($Xgafv);
}
@Override
public AddServerCa setAccessToken(java.lang.String accessToken) {
return (AddServerCa) super.setAccessToken(accessToken);
}
@Override
public AddServerCa setAlt(java.lang.String alt) {
return (AddServerCa) super.setAlt(alt);
}
@Override
public AddServerCa setCallback(java.lang.String callback) {
return (AddServerCa) super.setCallback(callback);
}
@Override
public AddServerCa setFields(java.lang.String fields) {
return (AddServerCa) super.setFields(fields);
}
@Override
public AddServerCa setKey(java.lang.String key) {
return (AddServerCa) super.setKey(key);
}
@Override
public AddServerCa setOauthToken(java.lang.String oauthToken) {
return (AddServerCa) super.setOauthToken(oauthToken);
}
@Override
public AddServerCa setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AddServerCa) super.setPrettyPrint(prettyPrint);
}
@Override
public AddServerCa setQuotaUser(java.lang.String quotaUser) {
return (AddServerCa) super.setQuotaUser(quotaUser);
}
@Override
public AddServerCa setUploadType(java.lang.String uploadType) {
return (AddServerCa) super.setUploadType(uploadType);
}
@Override
public AddServerCa setUploadProtocol(java.lang.String uploadProtocol) {
return (AddServerCa) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public AddServerCa setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public AddServerCa setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public AddServerCa set(String parameterName, Object value) {
return (AddServerCa) super.set(parameterName, value);
}
}
/**
* Add a new trusted server certificate version for the specified instance using Certificate
* Authority Service (CAS) server CA. Required to prepare for a certificate rotation. If a server
* certificate version was previously added but never used in a certificate rotation, this operation
* replaces that version. There cannot be more than one certificate version waiting to be rotated
* in. For instances not using CAS server CA, use AddServerCa instead.
*
* Create a request for the method "instances.addServerCertificate".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link AddServerCertificate#execute()} method to invoke the remote
* operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @return the request
*/
public AddServerCertificate addServerCertificate(java.lang.String project, java.lang.String instance) throws java.io.IOException {
AddServerCertificate result = new AddServerCertificate(project, instance);
initialize(result);
return result;
}
public class AddServerCertificate extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/addServerCertificate";
/**
* Add a new trusted server certificate version for the specified instance using Certificate
* Authority Service (CAS) server CA. Required to prepare for a certificate rotation. If a server
* certificate version was previously added but never used in a certificate rotation, this
* operation replaces that version. There cannot be more than one certificate version waiting to
* be rotated in. For instances not using CAS server CA, use AddServerCa instead.
*
* Create a request for the method "instances.addServerCertificate".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link AddServerCertificate#execute()} method to invoke the
* remote operation. {@link AddServerCertificate#initialize(com.google.api.client.googleapis.s
* ervices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @since 1.13
*/
protected AddServerCertificate(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "POST", REST_PATH, null, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public AddServerCertificate set$Xgafv(java.lang.String $Xgafv) {
return (AddServerCertificate) super.set$Xgafv($Xgafv);
}
@Override
public AddServerCertificate setAccessToken(java.lang.String accessToken) {
return (AddServerCertificate) super.setAccessToken(accessToken);
}
@Override
public AddServerCertificate setAlt(java.lang.String alt) {
return (AddServerCertificate) super.setAlt(alt);
}
@Override
public AddServerCertificate setCallback(java.lang.String callback) {
return (AddServerCertificate) super.setCallback(callback);
}
@Override
public AddServerCertificate setFields(java.lang.String fields) {
return (AddServerCertificate) super.setFields(fields);
}
@Override
public AddServerCertificate setKey(java.lang.String key) {
return (AddServerCertificate) super.setKey(key);
}
@Override
public AddServerCertificate setOauthToken(java.lang.String oauthToken) {
return (AddServerCertificate) super.setOauthToken(oauthToken);
}
@Override
public AddServerCertificate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AddServerCertificate) super.setPrettyPrint(prettyPrint);
}
@Override
public AddServerCertificate setQuotaUser(java.lang.String quotaUser) {
return (AddServerCertificate) super.setQuotaUser(quotaUser);
}
@Override
public AddServerCertificate setUploadType(java.lang.String uploadType) {
return (AddServerCertificate) super.setUploadType(uploadType);
}
@Override
public AddServerCertificate setUploadProtocol(java.lang.String uploadProtocol) {
return (AddServerCertificate) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public AddServerCertificate setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public AddServerCertificate setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public AddServerCertificate set(String parameterName, Object value) {
return (AddServerCertificate) super.set(parameterName, value);
}
}
/**
* Creates a Cloud SQL instance as a clone of the source instance. Using this operation might cause
* your instance to restart.
*
* Create a request for the method "instances.clone".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Clone#execute()} method to invoke the remote operation.
*
* @param project Project ID of the source as well as the clone Cloud SQL instance.
* @param instance The ID of the Cloud SQL instance to be cloned (source). This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.InstancesCloneRequest}
* @return the request
*/
public Clone clone(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.InstancesCloneRequest content) throws java.io.IOException {
Clone result = new Clone(project, instance, content);
initialize(result);
return result;
}
public class Clone extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/clone";
/**
* Creates a Cloud SQL instance as a clone of the source instance. Using this operation might
* cause your instance to restart.
*
* Create a request for the method "instances.clone".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Clone#execute()} method to invoke the remote operation.
* {@link
* Clone#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the source as well as the clone Cloud SQL instance.
* @param instance The ID of the Cloud SQL instance to be cloned (source). This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.InstancesCloneRequest}
* @since 1.13
*/
protected Clone(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.InstancesCloneRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Clone set$Xgafv(java.lang.String $Xgafv) {
return (Clone) super.set$Xgafv($Xgafv);
}
@Override
public Clone setAccessToken(java.lang.String accessToken) {
return (Clone) super.setAccessToken(accessToken);
}
@Override
public Clone setAlt(java.lang.String alt) {
return (Clone) super.setAlt(alt);
}
@Override
public Clone setCallback(java.lang.String callback) {
return (Clone) super.setCallback(callback);
}
@Override
public Clone setFields(java.lang.String fields) {
return (Clone) super.setFields(fields);
}
@Override
public Clone setKey(java.lang.String key) {
return (Clone) super.setKey(key);
}
@Override
public Clone setOauthToken(java.lang.String oauthToken) {
return (Clone) super.setOauthToken(oauthToken);
}
@Override
public Clone setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Clone) super.setPrettyPrint(prettyPrint);
}
@Override
public Clone setQuotaUser(java.lang.String quotaUser) {
return (Clone) super.setQuotaUser(quotaUser);
}
@Override
public Clone setUploadType(java.lang.String uploadType) {
return (Clone) super.setUploadType(uploadType);
}
@Override
public Clone setUploadProtocol(java.lang.String uploadProtocol) {
return (Clone) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the source as well as the clone Cloud SQL instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the source as well as the clone Cloud SQL instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the source as well as the clone Cloud SQL instance. */
public Clone setProject(java.lang.String project) {
this.project = project;
return this;
}
/**
* The ID of the Cloud SQL instance to be cloned (source). This does not include the project
* ID.
*/
@com.google.api.client.util.Key
private java.lang.String instance;
/** The ID of the Cloud SQL instance to be cloned (source). This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/**
* The ID of the Cloud SQL instance to be cloned (source). This does not include the project
* ID.
*/
public Clone setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Clone set(String parameterName, Object value) {
return (Clone) super.set(parameterName, value);
}
}
/**
* Deletes a Cloud SQL instance.
*
* Create a request for the method "instances.delete".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance to be deleted.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @return the request
*/
public Delete delete(java.lang.String project, java.lang.String instance) throws java.io.IOException {
Delete result = new Delete(project, instance);
initialize(result);
return result;
}
public class Delete extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}";
/**
* Deletes a Cloud SQL instance.
*
* Create a request for the method "instances.delete".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance to be deleted.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @since 1.13
*/
protected Delete(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "DELETE", REST_PATH, null, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance to be deleted. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance to be deleted.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance to be deleted. */
public Delete setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Delete setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Demotes an existing standalone instance to be a Cloud SQL read replica for an external database
* server.
*
* Create a request for the method "instances.demote".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Demote#execute()} method to invoke the remote operation.
*
* @param project Required. ID of the project that contains the instance.
* @param instance Required. Cloud SQL instance name.
* @param content the {@link com.google.api.services.sqladmin.model.InstancesDemoteRequest}
* @return the request
*/
public Demote demote(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.InstancesDemoteRequest content) throws java.io.IOException {
Demote result = new Demote(project, instance, content);
initialize(result);
return result;
}
public class Demote extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/demote";
/**
* Demotes an existing standalone instance to be a Cloud SQL read replica for an external database
* server.
*
* Create a request for the method "instances.demote".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Demote#execute()} method to invoke the remote operation.
* {@link
* Demote#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Required. ID of the project that contains the instance.
* @param instance Required. Cloud SQL instance name.
* @param content the {@link com.google.api.services.sqladmin.model.InstancesDemoteRequest}
* @since 1.13
*/
protected Demote(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.InstancesDemoteRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Demote set$Xgafv(java.lang.String $Xgafv) {
return (Demote) super.set$Xgafv($Xgafv);
}
@Override
public Demote setAccessToken(java.lang.String accessToken) {
return (Demote) super.setAccessToken(accessToken);
}
@Override
public Demote setAlt(java.lang.String alt) {
return (Demote) super.setAlt(alt);
}
@Override
public Demote setCallback(java.lang.String callback) {
return (Demote) super.setCallback(callback);
}
@Override
public Demote setFields(java.lang.String fields) {
return (Demote) super.setFields(fields);
}
@Override
public Demote setKey(java.lang.String key) {
return (Demote) super.setKey(key);
}
@Override
public Demote setOauthToken(java.lang.String oauthToken) {
return (Demote) super.setOauthToken(oauthToken);
}
@Override
public Demote setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Demote) super.setPrettyPrint(prettyPrint);
}
@Override
public Demote setQuotaUser(java.lang.String quotaUser) {
return (Demote) super.setQuotaUser(quotaUser);
}
@Override
public Demote setUploadType(java.lang.String uploadType) {
return (Demote) super.setUploadType(uploadType);
}
@Override
public Demote setUploadProtocol(java.lang.String uploadProtocol) {
return (Demote) super.setUploadProtocol(uploadProtocol);
}
/** Required. ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Required. ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Required. ID of the project that contains the instance. */
public Demote setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Required. Cloud SQL instance name. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Required. Cloud SQL instance name.
*/
public java.lang.String getInstance() {
return instance;
}
/** Required. Cloud SQL instance name. */
public Demote setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Demote set(String parameterName, Object value) {
return (Demote) super.set(parameterName, value);
}
}
/**
* Demotes the stand-alone instance to be a Cloud SQL read replica for an external database server.
*
* Create a request for the method "instances.demoteMaster".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link DemoteMaster#execute()} method to invoke the remote operation.
*
* @param project ID of the project that contains the instance.
* @param instance Cloud SQL instance name.
* @param content the {@link com.google.api.services.sqladmin.model.InstancesDemoteMasterRequest}
* @return the request
*/
public DemoteMaster demoteMaster(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.InstancesDemoteMasterRequest content) throws java.io.IOException {
DemoteMaster result = new DemoteMaster(project, instance, content);
initialize(result);
return result;
}
public class DemoteMaster extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/demoteMaster";
/**
* Demotes the stand-alone instance to be a Cloud SQL read replica for an external database
* server.
*
* Create a request for the method "instances.demoteMaster".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link DemoteMaster#execute()} method to invoke the remote
* operation. {@link
* DemoteMaster#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project ID of the project that contains the instance.
* @param instance Cloud SQL instance name.
* @param content the {@link com.google.api.services.sqladmin.model.InstancesDemoteMasterRequest}
* @since 1.13
*/
protected DemoteMaster(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.InstancesDemoteMasterRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public DemoteMaster set$Xgafv(java.lang.String $Xgafv) {
return (DemoteMaster) super.set$Xgafv($Xgafv);
}
@Override
public DemoteMaster setAccessToken(java.lang.String accessToken) {
return (DemoteMaster) super.setAccessToken(accessToken);
}
@Override
public DemoteMaster setAlt(java.lang.String alt) {
return (DemoteMaster) super.setAlt(alt);
}
@Override
public DemoteMaster setCallback(java.lang.String callback) {
return (DemoteMaster) super.setCallback(callback);
}
@Override
public DemoteMaster setFields(java.lang.String fields) {
return (DemoteMaster) super.setFields(fields);
}
@Override
public DemoteMaster setKey(java.lang.String key) {
return (DemoteMaster) super.setKey(key);
}
@Override
public DemoteMaster setOauthToken(java.lang.String oauthToken) {
return (DemoteMaster) super.setOauthToken(oauthToken);
}
@Override
public DemoteMaster setPrettyPrint(java.lang.Boolean prettyPrint) {
return (DemoteMaster) super.setPrettyPrint(prettyPrint);
}
@Override
public DemoteMaster setQuotaUser(java.lang.String quotaUser) {
return (DemoteMaster) super.setQuotaUser(quotaUser);
}
@Override
public DemoteMaster setUploadType(java.lang.String uploadType) {
return (DemoteMaster) super.setUploadType(uploadType);
}
@Override
public DemoteMaster setUploadProtocol(java.lang.String uploadProtocol) {
return (DemoteMaster) super.setUploadProtocol(uploadProtocol);
}
/** ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** ID of the project that contains the instance. */
public DemoteMaster setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance name. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance name.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance name. */
public DemoteMaster setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public DemoteMaster set(String parameterName, Object value) {
return (DemoteMaster) super.set(parameterName, value);
}
}
/**
* Exports data from a Cloud SQL instance to a Cloud Storage bucket as a SQL dump or CSV file.
*
* Create a request for the method "instances.export".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Export#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance to be exported.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.InstancesExportRequest}
* @return the request
*/
public Export export(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.InstancesExportRequest content) throws java.io.IOException {
Export result = new Export(project, instance, content);
initialize(result);
return result;
}
public class Export extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/export";
/**
* Exports data from a Cloud SQL instance to a Cloud Storage bucket as a SQL dump or CSV file.
*
* Create a request for the method "instances.export".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Export#execute()} method to invoke the remote operation.
* {@link
* Export#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance to be exported.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.InstancesExportRequest}
* @since 1.13
*/
protected Export(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.InstancesExportRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Export set$Xgafv(java.lang.String $Xgafv) {
return (Export) super.set$Xgafv($Xgafv);
}
@Override
public Export setAccessToken(java.lang.String accessToken) {
return (Export) super.setAccessToken(accessToken);
}
@Override
public Export setAlt(java.lang.String alt) {
return (Export) super.setAlt(alt);
}
@Override
public Export setCallback(java.lang.String callback) {
return (Export) super.setCallback(callback);
}
@Override
public Export setFields(java.lang.String fields) {
return (Export) super.setFields(fields);
}
@Override
public Export setKey(java.lang.String key) {
return (Export) super.setKey(key);
}
@Override
public Export setOauthToken(java.lang.String oauthToken) {
return (Export) super.setOauthToken(oauthToken);
}
@Override
public Export setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Export) super.setPrettyPrint(prettyPrint);
}
@Override
public Export setQuotaUser(java.lang.String quotaUser) {
return (Export) super.setQuotaUser(quotaUser);
}
@Override
public Export setUploadType(java.lang.String uploadType) {
return (Export) super.setUploadType(uploadType);
}
@Override
public Export setUploadProtocol(java.lang.String uploadProtocol) {
return (Export) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance to be exported. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance to be exported.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance to be exported. */
public Export setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Export setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Export set(String parameterName, Object value) {
return (Export) super.set(parameterName, value);
}
}
/**
* Initiates a manual failover of a high availability (HA) primary instance to a standby instance,
* which becomes the primary instance. Users are then rerouted to the new primary. For more
* information, see the [Overview of high
* availability](https://cloud.google.com/sql/docs/mysql/high-availability) page in the Cloud SQL
* documentation. If using Legacy HA (MySQL only), this causes the instance to failover to its
* failover replica instance.
*
* Create a request for the method "instances.failover".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Failover#execute()} method to invoke the remote operation.
*
* @param project ID of the project that contains the read replica.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.InstancesFailoverRequest}
* @return the request
*/
public Failover failover(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.InstancesFailoverRequest content) throws java.io.IOException {
Failover result = new Failover(project, instance, content);
initialize(result);
return result;
}
public class Failover extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/failover";
/**
* Initiates a manual failover of a high availability (HA) primary instance to a standby instance,
* which becomes the primary instance. Users are then rerouted to the new primary. For more
* information, see the [Overview of high
* availability](https://cloud.google.com/sql/docs/mysql/high-availability) page in the Cloud SQL
* documentation. If using Legacy HA (MySQL only), this causes the instance to failover to its
* failover replica instance.
*
* Create a request for the method "instances.failover".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Failover#execute()} method to invoke the remote operation.
* {@link
* Failover#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project ID of the project that contains the read replica.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.InstancesFailoverRequest}
* @since 1.13
*/
protected Failover(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.InstancesFailoverRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Failover set$Xgafv(java.lang.String $Xgafv) {
return (Failover) super.set$Xgafv($Xgafv);
}
@Override
public Failover setAccessToken(java.lang.String accessToken) {
return (Failover) super.setAccessToken(accessToken);
}
@Override
public Failover setAlt(java.lang.String alt) {
return (Failover) super.setAlt(alt);
}
@Override
public Failover setCallback(java.lang.String callback) {
return (Failover) super.setCallback(callback);
}
@Override
public Failover setFields(java.lang.String fields) {
return (Failover) super.setFields(fields);
}
@Override
public Failover setKey(java.lang.String key) {
return (Failover) super.setKey(key);
}
@Override
public Failover setOauthToken(java.lang.String oauthToken) {
return (Failover) super.setOauthToken(oauthToken);
}
@Override
public Failover setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Failover) super.setPrettyPrint(prettyPrint);
}
@Override
public Failover setQuotaUser(java.lang.String quotaUser) {
return (Failover) super.setQuotaUser(quotaUser);
}
@Override
public Failover setUploadType(java.lang.String uploadType) {
return (Failover) super.setUploadType(uploadType);
}
@Override
public Failover setUploadProtocol(java.lang.String uploadProtocol) {
return (Failover) super.setUploadProtocol(uploadProtocol);
}
/** ID of the project that contains the read replica. */
@com.google.api.client.util.Key
private java.lang.String project;
/** ID of the project that contains the read replica.
*/
public java.lang.String getProject() {
return project;
}
/** ID of the project that contains the read replica. */
public Failover setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Failover setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Failover set(String parameterName, Object value) {
return (Failover) super.set(parameterName, value);
}
}
/**
* Retrieves a resource containing information about a Cloud SQL instance.
*
* Create a request for the method "instances.get".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @return the request
*/
public Get get(java.lang.String project, java.lang.String instance) throws java.io.IOException {
Get result = new Get(project, instance);
initialize(result);
return result;
}
public class Get extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}";
/**
* Retrieves a resource containing information about a Cloud SQL instance.
*
* Create a request for the method "instances.get".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @since 1.13
*/
protected Get(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sqladmin.model.DatabaseInstance.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Get setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Database instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Database instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Database instance ID. This does not include the project ID. */
public Get setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Imports data into a Cloud SQL instance from a SQL dump or CSV file in Cloud Storage.
*
* Create a request for the method "instances.import".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link SQLAdminImport#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.InstancesImportRequest}
* @return the request
*/
public SQLAdminImport sqladminImport(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.InstancesImportRequest content) throws java.io.IOException {
SQLAdminImport result = new SQLAdminImport(project, instance, content);
initialize(result);
return result;
}
public class SQLAdminImport extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/import";
/**
* Imports data into a Cloud SQL instance from a SQL dump or CSV file in Cloud Storage.
*
* Create a request for the method "instances.import".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link SQLAdminImport#execute()} method to invoke the remote
* operation. {@link SQLAdminImport#initialize(com.google.api.client.googleapis.services.Abstr
* actGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.InstancesImportRequest}
* @since 1.13
*/
protected SQLAdminImport(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.InstancesImportRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public SQLAdminImport set$Xgafv(java.lang.String $Xgafv) {
return (SQLAdminImport) super.set$Xgafv($Xgafv);
}
@Override
public SQLAdminImport setAccessToken(java.lang.String accessToken) {
return (SQLAdminImport) super.setAccessToken(accessToken);
}
@Override
public SQLAdminImport setAlt(java.lang.String alt) {
return (SQLAdminImport) super.setAlt(alt);
}
@Override
public SQLAdminImport setCallback(java.lang.String callback) {
return (SQLAdminImport) super.setCallback(callback);
}
@Override
public SQLAdminImport setFields(java.lang.String fields) {
return (SQLAdminImport) super.setFields(fields);
}
@Override
public SQLAdminImport setKey(java.lang.String key) {
return (SQLAdminImport) super.setKey(key);
}
@Override
public SQLAdminImport setOauthToken(java.lang.String oauthToken) {
return (SQLAdminImport) super.setOauthToken(oauthToken);
}
@Override
public SQLAdminImport setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SQLAdminImport) super.setPrettyPrint(prettyPrint);
}
@Override
public SQLAdminImport setQuotaUser(java.lang.String quotaUser) {
return (SQLAdminImport) super.setQuotaUser(quotaUser);
}
@Override
public SQLAdminImport setUploadType(java.lang.String uploadType) {
return (SQLAdminImport) super.setUploadType(uploadType);
}
@Override
public SQLAdminImport setUploadProtocol(java.lang.String uploadProtocol) {
return (SQLAdminImport) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public SQLAdminImport setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public SQLAdminImport setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public SQLAdminImport set(String parameterName, Object value) {
return (SQLAdminImport) super.set(parameterName, value);
}
}
/**
* Creates a new Cloud SQL instance.
*
* Create a request for the method "instances.insert".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project to which the newly created Cloud SQL instances should belong.
* @param content the {@link com.google.api.services.sqladmin.model.DatabaseInstance}
* @return the request
*/
public Insert insert(java.lang.String project, com.google.api.services.sqladmin.model.DatabaseInstance content) throws java.io.IOException {
Insert result = new Insert(project, content);
initialize(result);
return result;
}
public class Insert extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances";
/**
* Creates a new Cloud SQL instance.
*
* Create a request for the method "instances.insert".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project to which the newly created Cloud SQL instances should belong.
* @param content the {@link com.google.api.services.sqladmin.model.DatabaseInstance}
* @since 1.13
*/
protected Insert(java.lang.String project, com.google.api.services.sqladmin.model.DatabaseInstance content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/**
* Project ID of the project to which the newly created Cloud SQL instances should belong.
*/
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project to which the newly created Cloud SQL instances should belong.
*/
public java.lang.String getProject() {
return project;
}
/**
* Project ID of the project to which the newly created Cloud SQL instances should belong.
*/
public Insert setProject(java.lang.String project) {
this.project = project;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Lists instances under a given project.
*
* Create a request for the method "instances.list".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project for which to list Cloud SQL instances.
* @return the request
*/
public List list(java.lang.String project) throws java.io.IOException {
List result = new List(project);
initialize(result);
return result;
}
public class List extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances";
/**
* Lists instances under a given project.
*
* Create a request for the method "instances.list".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project for which to list Cloud SQL instances.
* @since 1.13
*/
protected List(java.lang.String project) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sqladmin.model.InstancesListResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project for which to list Cloud SQL instances. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project for which to list Cloud SQL instances.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project for which to list Cloud SQL instances. */
public List setProject(java.lang.String project) {
this.project = project;
return this;
}
/**
* A filter expression that filters resources listed in the response. The expression is in the
* form of field:value. For example, 'instanceType:CLOUD_SQL_INSTANCE'. Fields can be nested
* as needed as per their JSON representation, such as 'settings.userLabels.auto_start:true'.
* Multiple filter queries are space-separated. For example. 'state:RUNNABLE
* instanceType:CLOUD_SQL_INSTANCE'. By default, each expression is an AND expression.
* However, you can include AND and OR expressions explicitly.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter expression that filters resources listed in the response. The expression is in the form of
field:value. For example, 'instanceType:CLOUD_SQL_INSTANCE'. Fields can be nested as needed as per
their JSON representation, such as 'settings.userLabels.auto_start:true'. Multiple filter queries
are space-separated. For example. 'state:RUNNABLE instanceType:CLOUD_SQL_INSTANCE'. By default,
each expression is an AND expression. However, you can include AND and OR expressions explicitly.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter expression that filters resources listed in the response. The expression is in the
* form of field:value. For example, 'instanceType:CLOUD_SQL_INSTANCE'. Fields can be nested
* as needed as per their JSON representation, such as 'settings.userLabels.auto_start:true'.
* Multiple filter queries are space-separated. For example. 'state:RUNNABLE
* instanceType:CLOUD_SQL_INSTANCE'. By default, each expression is an AND expression.
* However, you can include AND and OR expressions explicitly.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* The maximum number of instances to return. The service may return fewer than this value. If
* unspecified, at most 500 instances are returned. The maximum value is 1000; values above
* 1000 are coerced to 1000.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of instances to return. The service may return fewer than this value. If
unspecified, at most 500 instances are returned. The maximum value is 1000; values above 1000 are
coerced to 1000.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of instances to return. The service may return fewer than this value. If
* unspecified, at most 500 instances are returned. The maximum value is 1000; values above
* 1000 are coerced to 1000.
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* A previously-returned page token representing part of the larger set of results to view.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A previously-returned page token representing part of the larger set of results to view.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A previously-returned page token representing part of the larger set of results to view.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Lists all of the trusted Certificate Authorities (CAs) for the specified instance. There can be
* up to three CAs listed: the CA that was used to sign the certificate that is currently in use, a
* CA that has been added but not yet used to sign a certificate, and a CA used to sign a
* certificate that has previously rotated out.
*
* Create a request for the method "instances.listServerCas".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link ListServerCas#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @return the request
*/
public ListServerCas listServerCas(java.lang.String project, java.lang.String instance) throws java.io.IOException {
ListServerCas result = new ListServerCas(project, instance);
initialize(result);
return result;
}
public class ListServerCas extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/listServerCas";
/**
* Lists all of the trusted Certificate Authorities (CAs) for the specified instance. There can be
* up to three CAs listed: the CA that was used to sign the certificate that is currently in use,
* a CA that has been added but not yet used to sign a certificate, and a CA used to sign a
* certificate that has previously rotated out.
*
* Create a request for the method "instances.listServerCas".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link ListServerCas#execute()} method to invoke the remote
* operation. {@link ListServerCas#initialize(com.google.api.client.googleapis.services.Abstra
* ctGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @since 1.13
*/
protected ListServerCas(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sqladmin.model.InstancesListServerCasResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ListServerCas set$Xgafv(java.lang.String $Xgafv) {
return (ListServerCas) super.set$Xgafv($Xgafv);
}
@Override
public ListServerCas setAccessToken(java.lang.String accessToken) {
return (ListServerCas) super.setAccessToken(accessToken);
}
@Override
public ListServerCas setAlt(java.lang.String alt) {
return (ListServerCas) super.setAlt(alt);
}
@Override
public ListServerCas setCallback(java.lang.String callback) {
return (ListServerCas) super.setCallback(callback);
}
@Override
public ListServerCas setFields(java.lang.String fields) {
return (ListServerCas) super.setFields(fields);
}
@Override
public ListServerCas setKey(java.lang.String key) {
return (ListServerCas) super.setKey(key);
}
@Override
public ListServerCas setOauthToken(java.lang.String oauthToken) {
return (ListServerCas) super.setOauthToken(oauthToken);
}
@Override
public ListServerCas setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListServerCas) super.setPrettyPrint(prettyPrint);
}
@Override
public ListServerCas setQuotaUser(java.lang.String quotaUser) {
return (ListServerCas) super.setQuotaUser(quotaUser);
}
@Override
public ListServerCas setUploadType(java.lang.String uploadType) {
return (ListServerCas) super.setUploadType(uploadType);
}
@Override
public ListServerCas setUploadProtocol(java.lang.String uploadProtocol) {
return (ListServerCas) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public ListServerCas setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public ListServerCas setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public ListServerCas set(String parameterName, Object value) {
return (ListServerCas) super.set(parameterName, value);
}
}
/**
* Partially updates settings of a Cloud SQL instance by merging the request with the current
* configuration. This method supports patch semantics.
*
* Create a request for the method "instances.patch".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.DatabaseInstance}
* @return the request
*/
public Patch patch(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.DatabaseInstance content) throws java.io.IOException {
Patch result = new Patch(project, instance, content);
initialize(result);
return result;
}
public class Patch extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}";
/**
* Partially updates settings of a Cloud SQL instance by merging the request with the current
* configuration. This method supports patch semantics.
*
* Create a request for the method "instances.patch".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.DatabaseInstance}
* @since 1.13
*/
protected Patch(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.DatabaseInstance content) {
super(SQLAdmin.this, "PATCH", REST_PATH, content, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Patch setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Patch setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Promotes the read replica instance to be an independent Cloud SQL primary instance. Using this
* operation might cause your instance to restart.
*
* Create a request for the method "instances.promoteReplica".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link PromoteReplica#execute()} method to invoke the remote operation.
*
* @param project ID of the project that contains the read replica.
* @param instance Cloud SQL read replica instance name.
* @return the request
*/
public PromoteReplica promoteReplica(java.lang.String project, java.lang.String instance) throws java.io.IOException {
PromoteReplica result = new PromoteReplica(project, instance);
initialize(result);
return result;
}
public class PromoteReplica extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/promoteReplica";
/**
* Promotes the read replica instance to be an independent Cloud SQL primary instance. Using this
* operation might cause your instance to restart.
*
* Create a request for the method "instances.promoteReplica".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link PromoteReplica#execute()} method to invoke the remote
* operation. {@link PromoteReplica#initialize(com.google.api.client.googleapis.services.Abstr
* actGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param project ID of the project that contains the read replica.
* @param instance Cloud SQL read replica instance name.
* @since 1.13
*/
protected PromoteReplica(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "POST", REST_PATH, null, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public PromoteReplica set$Xgafv(java.lang.String $Xgafv) {
return (PromoteReplica) super.set$Xgafv($Xgafv);
}
@Override
public PromoteReplica setAccessToken(java.lang.String accessToken) {
return (PromoteReplica) super.setAccessToken(accessToken);
}
@Override
public PromoteReplica setAlt(java.lang.String alt) {
return (PromoteReplica) super.setAlt(alt);
}
@Override
public PromoteReplica setCallback(java.lang.String callback) {
return (PromoteReplica) super.setCallback(callback);
}
@Override
public PromoteReplica setFields(java.lang.String fields) {
return (PromoteReplica) super.setFields(fields);
}
@Override
public PromoteReplica setKey(java.lang.String key) {
return (PromoteReplica) super.setKey(key);
}
@Override
public PromoteReplica setOauthToken(java.lang.String oauthToken) {
return (PromoteReplica) super.setOauthToken(oauthToken);
}
@Override
public PromoteReplica setPrettyPrint(java.lang.Boolean prettyPrint) {
return (PromoteReplica) super.setPrettyPrint(prettyPrint);
}
@Override
public PromoteReplica setQuotaUser(java.lang.String quotaUser) {
return (PromoteReplica) super.setQuotaUser(quotaUser);
}
@Override
public PromoteReplica setUploadType(java.lang.String uploadType) {
return (PromoteReplica) super.setUploadType(uploadType);
}
@Override
public PromoteReplica setUploadProtocol(java.lang.String uploadProtocol) {
return (PromoteReplica) super.setUploadProtocol(uploadProtocol);
}
/** ID of the project that contains the read replica. */
@com.google.api.client.util.Key
private java.lang.String project;
/** ID of the project that contains the read replica.
*/
public java.lang.String getProject() {
return project;
}
/** ID of the project that contains the read replica. */
public PromoteReplica setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL read replica instance name. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL read replica instance name.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL read replica instance name. */
public PromoteReplica setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/**
* Set to true to invoke a replica failover to the designated DR replica. As part of replica
* failover, the promote operation attempts to add the original primary instance as a replica
* of the promoted DR replica when the original primary instance comes back online. If set to
* false or not specified, then the original primary instance becomes an independent Cloud SQL
* primary instance. Only applicable to MySQL.
*/
@com.google.api.client.util.Key
private java.lang.Boolean failover;
/** Set to true to invoke a replica failover to the designated DR replica. As part of replica failover,
the promote operation attempts to add the original primary instance as a replica of the promoted DR
replica when the original primary instance comes back online. If set to false or not specified,
then the original primary instance becomes an independent Cloud SQL primary instance. Only
applicable to MySQL.
*/
public java.lang.Boolean getFailover() {
return failover;
}
/**
* Set to true to invoke a replica failover to the designated DR replica. As part of replica
* failover, the promote operation attempts to add the original primary instance as a replica
* of the promoted DR replica when the original primary instance comes back online. If set to
* false or not specified, then the original primary instance becomes an independent Cloud SQL
* primary instance. Only applicable to MySQL.
*/
public PromoteReplica setFailover(java.lang.Boolean failover) {
this.failover = failover;
return this;
}
@Override
public PromoteReplica set(String parameterName, Object value) {
return (PromoteReplica) super.set(parameterName, value);
}
}
/**
* Reencrypt CMEK instance with latest key version.
*
* Create a request for the method "instances.reencrypt".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Reencrypt#execute()} method to invoke the remote operation.
*
* @param project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.InstancesReencryptRequest}
* @return the request
*/
public Reencrypt reencrypt(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.InstancesReencryptRequest content) throws java.io.IOException {
Reencrypt result = new Reencrypt(project, instance, content);
initialize(result);
return result;
}
public class Reencrypt extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/reencrypt";
/**
* Reencrypt CMEK instance with latest key version.
*
* Create a request for the method "instances.reencrypt".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Reencrypt#execute()} method to invoke the remote
* operation. {@link
* Reencrypt#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.InstancesReencryptRequest}
* @since 1.13
*/
protected Reencrypt(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.InstancesReencryptRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Reencrypt set$Xgafv(java.lang.String $Xgafv) {
return (Reencrypt) super.set$Xgafv($Xgafv);
}
@Override
public Reencrypt setAccessToken(java.lang.String accessToken) {
return (Reencrypt) super.setAccessToken(accessToken);
}
@Override
public Reencrypt setAlt(java.lang.String alt) {
return (Reencrypt) super.setAlt(alt);
}
@Override
public Reencrypt setCallback(java.lang.String callback) {
return (Reencrypt) super.setCallback(callback);
}
@Override
public Reencrypt setFields(java.lang.String fields) {
return (Reencrypt) super.setFields(fields);
}
@Override
public Reencrypt setKey(java.lang.String key) {
return (Reencrypt) super.setKey(key);
}
@Override
public Reencrypt setOauthToken(java.lang.String oauthToken) {
return (Reencrypt) super.setOauthToken(oauthToken);
}
@Override
public Reencrypt setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Reencrypt) super.setPrettyPrint(prettyPrint);
}
@Override
public Reencrypt setQuotaUser(java.lang.String quotaUser) {
return (Reencrypt) super.setQuotaUser(quotaUser);
}
@Override
public Reencrypt setUploadType(java.lang.String uploadType) {
return (Reencrypt) super.setUploadType(uploadType);
}
@Override
public Reencrypt setUploadProtocol(java.lang.String uploadProtocol) {
return (Reencrypt) super.setUploadProtocol(uploadProtocol);
}
/** ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** ID of the project that contains the instance. */
public Reencrypt setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Reencrypt setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Reencrypt set(String parameterName, Object value) {
return (Reencrypt) super.set(parameterName, value);
}
}
/**
* Release a lease for the setup of SQL Server Reporting Services (SSRS).
*
* Create a request for the method "instances.releaseSsrsLease".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link ReleaseSsrsLease#execute()} method to invoke the remote operation.
*
* @param project Required. The project ID that contains the instance.
* @param instance Required. The Cloud SQL instance ID. This doesn't include the project ID. The instance ID contains
* lowercase letters, numbers, and hyphens, and it must start with a letter. This ID can have
* a maximum length of 98 characters.
* @return the request
*/
public ReleaseSsrsLease releaseSsrsLease(java.lang.String project, java.lang.String instance) throws java.io.IOException {
ReleaseSsrsLease result = new ReleaseSsrsLease(project, instance);
initialize(result);
return result;
}
public class ReleaseSsrsLease extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/releaseSsrsLease";
/**
* Release a lease for the setup of SQL Server Reporting Services (SSRS).
*
* Create a request for the method "instances.releaseSsrsLease".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link ReleaseSsrsLease#execute()} method to invoke the remote
* operation. {@link ReleaseSsrsLease#initialize(com.google.api.client.googleapis.services.Abs
* tractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param project Required. The project ID that contains the instance.
* @param instance Required. The Cloud SQL instance ID. This doesn't include the project ID. The instance ID contains
* lowercase letters, numbers, and hyphens, and it must start with a letter. This ID can have
* a maximum length of 98 characters.
* @since 1.13
*/
protected ReleaseSsrsLease(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "POST", REST_PATH, null, com.google.api.services.sqladmin.model.SqlInstancesReleaseSsrsLeaseResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public ReleaseSsrsLease set$Xgafv(java.lang.String $Xgafv) {
return (ReleaseSsrsLease) super.set$Xgafv($Xgafv);
}
@Override
public ReleaseSsrsLease setAccessToken(java.lang.String accessToken) {
return (ReleaseSsrsLease) super.setAccessToken(accessToken);
}
@Override
public ReleaseSsrsLease setAlt(java.lang.String alt) {
return (ReleaseSsrsLease) super.setAlt(alt);
}
@Override
public ReleaseSsrsLease setCallback(java.lang.String callback) {
return (ReleaseSsrsLease) super.setCallback(callback);
}
@Override
public ReleaseSsrsLease setFields(java.lang.String fields) {
return (ReleaseSsrsLease) super.setFields(fields);
}
@Override
public ReleaseSsrsLease setKey(java.lang.String key) {
return (ReleaseSsrsLease) super.setKey(key);
}
@Override
public ReleaseSsrsLease setOauthToken(java.lang.String oauthToken) {
return (ReleaseSsrsLease) super.setOauthToken(oauthToken);
}
@Override
public ReleaseSsrsLease setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ReleaseSsrsLease) super.setPrettyPrint(prettyPrint);
}
@Override
public ReleaseSsrsLease setQuotaUser(java.lang.String quotaUser) {
return (ReleaseSsrsLease) super.setQuotaUser(quotaUser);
}
@Override
public ReleaseSsrsLease setUploadType(java.lang.String uploadType) {
return (ReleaseSsrsLease) super.setUploadType(uploadType);
}
@Override
public ReleaseSsrsLease setUploadProtocol(java.lang.String uploadProtocol) {
return (ReleaseSsrsLease) super.setUploadProtocol(uploadProtocol);
}
/** Required. The project ID that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Required. The project ID that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Required. The project ID that contains the instance. */
public ReleaseSsrsLease setProject(java.lang.String project) {
this.project = project;
return this;
}
/**
* Required. The Cloud SQL instance ID. This doesn't include the project ID. The instance ID
* contains lowercase letters, numbers, and hyphens, and it must start with a letter. This ID
* can have a maximum length of 98 characters.
*/
@com.google.api.client.util.Key
private java.lang.String instance;
/** Required. The Cloud SQL instance ID. This doesn't include the project ID. The instance ID contains
lowercase letters, numbers, and hyphens, and it must start with a letter. This ID can have a
maximum length of 98 characters.
*/
public java.lang.String getInstance() {
return instance;
}
/**
* Required. The Cloud SQL instance ID. This doesn't include the project ID. The instance ID
* contains lowercase letters, numbers, and hyphens, and it must start with a letter. This ID
* can have a maximum length of 98 characters.
*/
public ReleaseSsrsLease setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public ReleaseSsrsLease set(String parameterName, Object value) {
return (ReleaseSsrsLease) super.set(parameterName, value);
}
}
/**
* Deletes all client certificates and generates a new server SSL certificate for the instance.
*
* Create a request for the method "instances.resetSslConfig".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link ResetSslConfig#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @return the request
*/
public ResetSslConfig resetSslConfig(java.lang.String project, java.lang.String instance) throws java.io.IOException {
ResetSslConfig result = new ResetSslConfig(project, instance);
initialize(result);
return result;
}
public class ResetSslConfig extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/resetSslConfig";
/**
* Deletes all client certificates and generates a new server SSL certificate for the instance.
*
* Create a request for the method "instances.resetSslConfig".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link ResetSslConfig#execute()} method to invoke the remote
* operation. {@link ResetSslConfig#initialize(com.google.api.client.googleapis.services.Abstr
* actGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @since 1.13
*/
protected ResetSslConfig(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "POST", REST_PATH, null, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public ResetSslConfig set$Xgafv(java.lang.String $Xgafv) {
return (ResetSslConfig) super.set$Xgafv($Xgafv);
}
@Override
public ResetSslConfig setAccessToken(java.lang.String accessToken) {
return (ResetSslConfig) super.setAccessToken(accessToken);
}
@Override
public ResetSslConfig setAlt(java.lang.String alt) {
return (ResetSslConfig) super.setAlt(alt);
}
@Override
public ResetSslConfig setCallback(java.lang.String callback) {
return (ResetSslConfig) super.setCallback(callback);
}
@Override
public ResetSslConfig setFields(java.lang.String fields) {
return (ResetSslConfig) super.setFields(fields);
}
@Override
public ResetSslConfig setKey(java.lang.String key) {
return (ResetSslConfig) super.setKey(key);
}
@Override
public ResetSslConfig setOauthToken(java.lang.String oauthToken) {
return (ResetSslConfig) super.setOauthToken(oauthToken);
}
@Override
public ResetSslConfig setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ResetSslConfig) super.setPrettyPrint(prettyPrint);
}
@Override
public ResetSslConfig setQuotaUser(java.lang.String quotaUser) {
return (ResetSslConfig) super.setQuotaUser(quotaUser);
}
@Override
public ResetSslConfig setUploadType(java.lang.String uploadType) {
return (ResetSslConfig) super.setUploadType(uploadType);
}
@Override
public ResetSslConfig setUploadProtocol(java.lang.String uploadProtocol) {
return (ResetSslConfig) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public ResetSslConfig setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public ResetSslConfig setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public ResetSslConfig set(String parameterName, Object value) {
return (ResetSslConfig) super.set(parameterName, value);
}
}
/**
* Restarts a Cloud SQL instance.
*
* Create a request for the method "instances.restart".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Restart#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance to be restarted.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @return the request
*/
public Restart restart(java.lang.String project, java.lang.String instance) throws java.io.IOException {
Restart result = new Restart(project, instance);
initialize(result);
return result;
}
public class Restart extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/restart";
/**
* Restarts a Cloud SQL instance.
*
* Create a request for the method "instances.restart".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Restart#execute()} method to invoke the remote operation.
* {@link
* Restart#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance to be restarted.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @since 1.13
*/
protected Restart(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "POST", REST_PATH, null, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Restart set$Xgafv(java.lang.String $Xgafv) {
return (Restart) super.set$Xgafv($Xgafv);
}
@Override
public Restart setAccessToken(java.lang.String accessToken) {
return (Restart) super.setAccessToken(accessToken);
}
@Override
public Restart setAlt(java.lang.String alt) {
return (Restart) super.setAlt(alt);
}
@Override
public Restart setCallback(java.lang.String callback) {
return (Restart) super.setCallback(callback);
}
@Override
public Restart setFields(java.lang.String fields) {
return (Restart) super.setFields(fields);
}
@Override
public Restart setKey(java.lang.String key) {
return (Restart) super.setKey(key);
}
@Override
public Restart setOauthToken(java.lang.String oauthToken) {
return (Restart) super.setOauthToken(oauthToken);
}
@Override
public Restart setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Restart) super.setPrettyPrint(prettyPrint);
}
@Override
public Restart setQuotaUser(java.lang.String quotaUser) {
return (Restart) super.setQuotaUser(quotaUser);
}
@Override
public Restart setUploadType(java.lang.String uploadType) {
return (Restart) super.setUploadType(uploadType);
}
@Override
public Restart setUploadProtocol(java.lang.String uploadProtocol) {
return (Restart) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance to be restarted. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance to be restarted.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance to be restarted. */
public Restart setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Restart setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Restart set(String parameterName, Object value) {
return (Restart) super.set(parameterName, value);
}
}
/**
* Restores a backup of a Cloud SQL instance. Using this operation might cause your instance to
* restart.
*
* Create a request for the method "instances.restoreBackup".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link RestoreBackup#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.InstancesRestoreBackupRequest}
* @return the request
*/
public RestoreBackup restoreBackup(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.InstancesRestoreBackupRequest content) throws java.io.IOException {
RestoreBackup result = new RestoreBackup(project, instance, content);
initialize(result);
return result;
}
public class RestoreBackup extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/restoreBackup";
/**
* Restores a backup of a Cloud SQL instance. Using this operation might cause your instance to
* restart.
*
* Create a request for the method "instances.restoreBackup".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link RestoreBackup#execute()} method to invoke the remote
* operation. {@link RestoreBackup#initialize(com.google.api.client.googleapis.services.Abstra
* ctGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.InstancesRestoreBackupRequest}
* @since 1.13
*/
protected RestoreBackup(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.InstancesRestoreBackupRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public RestoreBackup set$Xgafv(java.lang.String $Xgafv) {
return (RestoreBackup) super.set$Xgafv($Xgafv);
}
@Override
public RestoreBackup setAccessToken(java.lang.String accessToken) {
return (RestoreBackup) super.setAccessToken(accessToken);
}
@Override
public RestoreBackup setAlt(java.lang.String alt) {
return (RestoreBackup) super.setAlt(alt);
}
@Override
public RestoreBackup setCallback(java.lang.String callback) {
return (RestoreBackup) super.setCallback(callback);
}
@Override
public RestoreBackup setFields(java.lang.String fields) {
return (RestoreBackup) super.setFields(fields);
}
@Override
public RestoreBackup setKey(java.lang.String key) {
return (RestoreBackup) super.setKey(key);
}
@Override
public RestoreBackup setOauthToken(java.lang.String oauthToken) {
return (RestoreBackup) super.setOauthToken(oauthToken);
}
@Override
public RestoreBackup setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RestoreBackup) super.setPrettyPrint(prettyPrint);
}
@Override
public RestoreBackup setQuotaUser(java.lang.String quotaUser) {
return (RestoreBackup) super.setQuotaUser(quotaUser);
}
@Override
public RestoreBackup setUploadType(java.lang.String uploadType) {
return (RestoreBackup) super.setUploadType(uploadType);
}
@Override
public RestoreBackup setUploadProtocol(java.lang.String uploadProtocol) {
return (RestoreBackup) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public RestoreBackup setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public RestoreBackup setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public RestoreBackup set(String parameterName, Object value) {
return (RestoreBackup) super.set(parameterName, value);
}
}
/**
* Rotates the server certificate to one signed by the Certificate Authority (CA) version previously
* added with the addServerCA method. For instances that have enabled Certificate Authority Service
* (CAS) based server CA, use RotateServerCertificate to rotate the server certificate.
*
* Create a request for the method "instances.rotateServerCa".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link RotateServerCa#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.InstancesRotateServerCaRequest}
* @return the request
*/
public RotateServerCa rotateServerCa(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.InstancesRotateServerCaRequest content) throws java.io.IOException {
RotateServerCa result = new RotateServerCa(project, instance, content);
initialize(result);
return result;
}
public class RotateServerCa extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/rotateServerCa";
/**
* Rotates the server certificate to one signed by the Certificate Authority (CA) version
* previously added with the addServerCA method. For instances that have enabled Certificate
* Authority Service (CAS) based server CA, use RotateServerCertificate to rotate the server
* certificate.
*
* Create a request for the method "instances.rotateServerCa".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link RotateServerCa#execute()} method to invoke the remote
* operation. {@link RotateServerCa#initialize(com.google.api.client.googleapis.services.Abstr
* actGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.InstancesRotateServerCaRequest}
* @since 1.13
*/
protected RotateServerCa(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.InstancesRotateServerCaRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public RotateServerCa set$Xgafv(java.lang.String $Xgafv) {
return (RotateServerCa) super.set$Xgafv($Xgafv);
}
@Override
public RotateServerCa setAccessToken(java.lang.String accessToken) {
return (RotateServerCa) super.setAccessToken(accessToken);
}
@Override
public RotateServerCa setAlt(java.lang.String alt) {
return (RotateServerCa) super.setAlt(alt);
}
@Override
public RotateServerCa setCallback(java.lang.String callback) {
return (RotateServerCa) super.setCallback(callback);
}
@Override
public RotateServerCa setFields(java.lang.String fields) {
return (RotateServerCa) super.setFields(fields);
}
@Override
public RotateServerCa setKey(java.lang.String key) {
return (RotateServerCa) super.setKey(key);
}
@Override
public RotateServerCa setOauthToken(java.lang.String oauthToken) {
return (RotateServerCa) super.setOauthToken(oauthToken);
}
@Override
public RotateServerCa setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RotateServerCa) super.setPrettyPrint(prettyPrint);
}
@Override
public RotateServerCa setQuotaUser(java.lang.String quotaUser) {
return (RotateServerCa) super.setQuotaUser(quotaUser);
}
@Override
public RotateServerCa setUploadType(java.lang.String uploadType) {
return (RotateServerCa) super.setUploadType(uploadType);
}
@Override
public RotateServerCa setUploadProtocol(java.lang.String uploadProtocol) {
return (RotateServerCa) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public RotateServerCa setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public RotateServerCa setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public RotateServerCa set(String parameterName, Object value) {
return (RotateServerCa) super.set(parameterName, value);
}
}
/**
* Starts the replication in the read replica instance.
*
* Create a request for the method "instances.startReplica".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link StartReplica#execute()} method to invoke the remote operation.
*
* @param project ID of the project that contains the read replica.
* @param instance Cloud SQL read replica instance name.
* @return the request
*/
public StartReplica startReplica(java.lang.String project, java.lang.String instance) throws java.io.IOException {
StartReplica result = new StartReplica(project, instance);
initialize(result);
return result;
}
public class StartReplica extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/startReplica";
/**
* Starts the replication in the read replica instance.
*
* Create a request for the method "instances.startReplica".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link StartReplica#execute()} method to invoke the remote
* operation. {@link
* StartReplica#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project ID of the project that contains the read replica.
* @param instance Cloud SQL read replica instance name.
* @since 1.13
*/
protected StartReplica(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "POST", REST_PATH, null, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public StartReplica set$Xgafv(java.lang.String $Xgafv) {
return (StartReplica) super.set$Xgafv($Xgafv);
}
@Override
public StartReplica setAccessToken(java.lang.String accessToken) {
return (StartReplica) super.setAccessToken(accessToken);
}
@Override
public StartReplica setAlt(java.lang.String alt) {
return (StartReplica) super.setAlt(alt);
}
@Override
public StartReplica setCallback(java.lang.String callback) {
return (StartReplica) super.setCallback(callback);
}
@Override
public StartReplica setFields(java.lang.String fields) {
return (StartReplica) super.setFields(fields);
}
@Override
public StartReplica setKey(java.lang.String key) {
return (StartReplica) super.setKey(key);
}
@Override
public StartReplica setOauthToken(java.lang.String oauthToken) {
return (StartReplica) super.setOauthToken(oauthToken);
}
@Override
public StartReplica setPrettyPrint(java.lang.Boolean prettyPrint) {
return (StartReplica) super.setPrettyPrint(prettyPrint);
}
@Override
public StartReplica setQuotaUser(java.lang.String quotaUser) {
return (StartReplica) super.setQuotaUser(quotaUser);
}
@Override
public StartReplica setUploadType(java.lang.String uploadType) {
return (StartReplica) super.setUploadType(uploadType);
}
@Override
public StartReplica setUploadProtocol(java.lang.String uploadProtocol) {
return (StartReplica) super.setUploadProtocol(uploadProtocol);
}
/** ID of the project that contains the read replica. */
@com.google.api.client.util.Key
private java.lang.String project;
/** ID of the project that contains the read replica.
*/
public java.lang.String getProject() {
return project;
}
/** ID of the project that contains the read replica. */
public StartReplica setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL read replica instance name. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL read replica instance name.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL read replica instance name. */
public StartReplica setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public StartReplica set(String parameterName, Object value) {
return (StartReplica) super.set(parameterName, value);
}
}
/**
* Stops the replication in the read replica instance.
*
* Create a request for the method "instances.stopReplica".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link StopReplica#execute()} method to invoke the remote operation.
*
* @param project ID of the project that contains the read replica.
* @param instance Cloud SQL read replica instance name.
* @return the request
*/
public StopReplica stopReplica(java.lang.String project, java.lang.String instance) throws java.io.IOException {
StopReplica result = new StopReplica(project, instance);
initialize(result);
return result;
}
public class StopReplica extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/stopReplica";
/**
* Stops the replication in the read replica instance.
*
* Create a request for the method "instances.stopReplica".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link StopReplica#execute()} method to invoke the remote
* operation. {@link
* StopReplica#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project ID of the project that contains the read replica.
* @param instance Cloud SQL read replica instance name.
* @since 1.13
*/
protected StopReplica(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "POST", REST_PATH, null, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public StopReplica set$Xgafv(java.lang.String $Xgafv) {
return (StopReplica) super.set$Xgafv($Xgafv);
}
@Override
public StopReplica setAccessToken(java.lang.String accessToken) {
return (StopReplica) super.setAccessToken(accessToken);
}
@Override
public StopReplica setAlt(java.lang.String alt) {
return (StopReplica) super.setAlt(alt);
}
@Override
public StopReplica setCallback(java.lang.String callback) {
return (StopReplica) super.setCallback(callback);
}
@Override
public StopReplica setFields(java.lang.String fields) {
return (StopReplica) super.setFields(fields);
}
@Override
public StopReplica setKey(java.lang.String key) {
return (StopReplica) super.setKey(key);
}
@Override
public StopReplica setOauthToken(java.lang.String oauthToken) {
return (StopReplica) super.setOauthToken(oauthToken);
}
@Override
public StopReplica setPrettyPrint(java.lang.Boolean prettyPrint) {
return (StopReplica) super.setPrettyPrint(prettyPrint);
}
@Override
public StopReplica setQuotaUser(java.lang.String quotaUser) {
return (StopReplica) super.setQuotaUser(quotaUser);
}
@Override
public StopReplica setUploadType(java.lang.String uploadType) {
return (StopReplica) super.setUploadType(uploadType);
}
@Override
public StopReplica setUploadProtocol(java.lang.String uploadProtocol) {
return (StopReplica) super.setUploadProtocol(uploadProtocol);
}
/** ID of the project that contains the read replica. */
@com.google.api.client.util.Key
private java.lang.String project;
/** ID of the project that contains the read replica.
*/
public java.lang.String getProject() {
return project;
}
/** ID of the project that contains the read replica. */
public StopReplica setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL read replica instance name. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL read replica instance name.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL read replica instance name. */
public StopReplica setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public StopReplica set(String parameterName, Object value) {
return (StopReplica) super.set(parameterName, value);
}
}
/**
* Switches over from the primary instance to the designated DR replica instance.
*
* Create a request for the method "instances.switchover".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Switchover#execute()} method to invoke the remote operation.
*
* @param project ID of the project that contains the replica.
* @param instance Cloud SQL read replica instance name.
* @return the request
*/
public Switchover switchover(java.lang.String project, java.lang.String instance) throws java.io.IOException {
Switchover result = new Switchover(project, instance);
initialize(result);
return result;
}
public class Switchover extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/switchover";
/**
* Switches over from the primary instance to the designated DR replica instance.
*
* Create a request for the method "instances.switchover".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Switchover#execute()} method to invoke the remote
* operation. {@link
* Switchover#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project ID of the project that contains the replica.
* @param instance Cloud SQL read replica instance name.
* @since 1.13
*/
protected Switchover(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "POST", REST_PATH, null, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Switchover set$Xgafv(java.lang.String $Xgafv) {
return (Switchover) super.set$Xgafv($Xgafv);
}
@Override
public Switchover setAccessToken(java.lang.String accessToken) {
return (Switchover) super.setAccessToken(accessToken);
}
@Override
public Switchover setAlt(java.lang.String alt) {
return (Switchover) super.setAlt(alt);
}
@Override
public Switchover setCallback(java.lang.String callback) {
return (Switchover) super.setCallback(callback);
}
@Override
public Switchover setFields(java.lang.String fields) {
return (Switchover) super.setFields(fields);
}
@Override
public Switchover setKey(java.lang.String key) {
return (Switchover) super.setKey(key);
}
@Override
public Switchover setOauthToken(java.lang.String oauthToken) {
return (Switchover) super.setOauthToken(oauthToken);
}
@Override
public Switchover setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Switchover) super.setPrettyPrint(prettyPrint);
}
@Override
public Switchover setQuotaUser(java.lang.String quotaUser) {
return (Switchover) super.setQuotaUser(quotaUser);
}
@Override
public Switchover setUploadType(java.lang.String uploadType) {
return (Switchover) super.setUploadType(uploadType);
}
@Override
public Switchover setUploadProtocol(java.lang.String uploadProtocol) {
return (Switchover) super.setUploadProtocol(uploadProtocol);
}
/** ID of the project that contains the replica. */
@com.google.api.client.util.Key
private java.lang.String project;
/** ID of the project that contains the replica.
*/
public java.lang.String getProject() {
return project;
}
/** ID of the project that contains the replica. */
public Switchover setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL read replica instance name. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL read replica instance name.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL read replica instance name. */
public Switchover setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/**
* Optional. (MySQL only) Cloud SQL instance operations timeout, which is a sum of all
* database operations. Default value is 10 minutes and can be modified to a maximum value of
* 24 hours.
*/
@com.google.api.client.util.Key
private String dbTimeout;
/** Optional. (MySQL only) Cloud SQL instance operations timeout, which is a sum of all database
operations. Default value is 10 minutes and can be modified to a maximum value of 24 hours.
*/
public String getDbTimeout() {
return dbTimeout;
}
/**
* Optional. (MySQL only) Cloud SQL instance operations timeout, which is a sum of all
* database operations. Default value is 10 minutes and can be modified to a maximum value of
* 24 hours.
*/
public Switchover setDbTimeout(String dbTimeout) {
this.dbTimeout = dbTimeout;
return this;
}
@Override
public Switchover set(String parameterName, Object value) {
return (Switchover) super.set(parameterName, value);
}
}
/**
* Truncate MySQL general and slow query log tables MySQL only.
*
* Create a request for the method "instances.truncateLog".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link TruncateLog#execute()} method to invoke the remote operation.
*
* @param project Project ID of the Cloud SQL project.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.InstancesTruncateLogRequest}
* @return the request
*/
public TruncateLog truncateLog(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.InstancesTruncateLogRequest content) throws java.io.IOException {
TruncateLog result = new TruncateLog(project, instance, content);
initialize(result);
return result;
}
public class TruncateLog extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/truncateLog";
/**
* Truncate MySQL general and slow query log tables MySQL only.
*
* Create a request for the method "instances.truncateLog".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link TruncateLog#execute()} method to invoke the remote
* operation. {@link
* TruncateLog#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the Cloud SQL project.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.InstancesTruncateLogRequest}
* @since 1.13
*/
protected TruncateLog(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.InstancesTruncateLogRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public TruncateLog set$Xgafv(java.lang.String $Xgafv) {
return (TruncateLog) super.set$Xgafv($Xgafv);
}
@Override
public TruncateLog setAccessToken(java.lang.String accessToken) {
return (TruncateLog) super.setAccessToken(accessToken);
}
@Override
public TruncateLog setAlt(java.lang.String alt) {
return (TruncateLog) super.setAlt(alt);
}
@Override
public TruncateLog setCallback(java.lang.String callback) {
return (TruncateLog) super.setCallback(callback);
}
@Override
public TruncateLog setFields(java.lang.String fields) {
return (TruncateLog) super.setFields(fields);
}
@Override
public TruncateLog setKey(java.lang.String key) {
return (TruncateLog) super.setKey(key);
}
@Override
public TruncateLog setOauthToken(java.lang.String oauthToken) {
return (TruncateLog) super.setOauthToken(oauthToken);
}
@Override
public TruncateLog setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TruncateLog) super.setPrettyPrint(prettyPrint);
}
@Override
public TruncateLog setQuotaUser(java.lang.String quotaUser) {
return (TruncateLog) super.setQuotaUser(quotaUser);
}
@Override
public TruncateLog setUploadType(java.lang.String uploadType) {
return (TruncateLog) super.setUploadType(uploadType);
}
@Override
public TruncateLog setUploadProtocol(java.lang.String uploadProtocol) {
return (TruncateLog) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the Cloud SQL project. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the Cloud SQL project.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the Cloud SQL project. */
public TruncateLog setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public TruncateLog setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public TruncateLog set(String parameterName, Object value) {
return (TruncateLog) super.set(parameterName, value);
}
}
/**
* Updates settings of a Cloud SQL instance. Using this operation might cause your instance to
* restart.
*
* Create a request for the method "instances.update".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.DatabaseInstance}
* @return the request
*/
public Update update(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.DatabaseInstance content) throws java.io.IOException {
Update result = new Update(project, instance, content);
initialize(result);
return result;
}
public class Update extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}";
/**
* Updates settings of a Cloud SQL instance. Using this operation might cause your instance to
* restart.
*
* Create a request for the method "instances.update".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.DatabaseInstance}
* @since 1.13
*/
protected Update(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.DatabaseInstance content) {
super(SQLAdmin.this, "PUT", REST_PATH, content, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Update setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Update setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code SQLAdmin sqladmin = new SQLAdmin(...);}
* {@code SQLAdmin.Operations.List request = sqladmin.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Cancels an instance operation that has been performed on an instance.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param operation Instance operation ID.
* @return the request
*/
public Cancel cancel(java.lang.String project, java.lang.String operation) throws java.io.IOException {
Cancel result = new Cancel(project, operation);
initialize(result);
return result;
}
public class Cancel extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/operations/{operation}/cancel";
/**
* Cancels an instance operation that has been performed on an instance.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
* {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param operation Instance operation ID.
* @since 1.13
*/
protected Cancel(java.lang.String project, java.lang.String operation) {
super(SQLAdmin.this, "POST", REST_PATH, null, com.google.api.services.sqladmin.model.Empty.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.operation = com.google.api.client.util.Preconditions.checkNotNull(operation, "Required parameter operation must be specified.");
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Cancel setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Instance operation ID. */
@com.google.api.client.util.Key
private java.lang.String operation;
/** Instance operation ID.
*/
public java.lang.String getOperation() {
return operation;
}
/** Instance operation ID. */
public Cancel setOperation(java.lang.String operation) {
this.operation = operation;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Retrieves an instance operation that has been performed on an instance.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param operation Instance operation ID.
* @return the request
*/
public Get get(java.lang.String project, java.lang.String operation) throws java.io.IOException {
Get result = new Get(project, operation);
initialize(result);
return result;
}
public class Get extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/operations/{operation}";
/**
* Retrieves an instance operation that has been performed on an instance.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param operation Instance operation ID.
* @since 1.13
*/
protected Get(java.lang.String project, java.lang.String operation) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.operation = com.google.api.client.util.Preconditions.checkNotNull(operation, "Required parameter operation must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Get setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Instance operation ID. */
@com.google.api.client.util.Key
private java.lang.String operation;
/** Instance operation ID.
*/
public java.lang.String getOperation() {
return operation;
}
/** Instance operation ID. */
public Get setOperation(java.lang.String operation) {
this.operation = operation;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all instance operations that have been performed on the given Cloud SQL instance in the
* reverse chronological order of the start time.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @return the request
*/
public List list(java.lang.String project) throws java.io.IOException {
List result = new List(project);
initialize(result);
return result;
}
public class List extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/operations";
/**
* Lists all instance operations that have been performed on the given Cloud SQL instance in the
* reverse chronological order of the start time.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @since 1.13
*/
protected List(java.lang.String project) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sqladmin.model.OperationsListResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public List setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public List setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** Maximum number of operations per response. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** Maximum number of operations per response.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** Maximum number of operations per response. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* A previously-returned page token representing part of the larger set of results to view.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A previously-returned page token representing part of the larger set of results to view.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A previously-returned page token representing part of the larger set of results to view.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Projects collection.
*
* The typical use is:
*
* {@code SQLAdmin sqladmin = new SQLAdmin(...);}
* {@code SQLAdmin.Projects.List request = sqladmin.projects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Projects projects() {
return new Projects();
}
/**
* The "projects" collection of methods.
*/
public class Projects {
/**
* An accessor for creating requests from the Instances collection.
*
* The typical use is:
*
* {@code SQLAdmin sqladmin = new SQLAdmin(...);}
* {@code SQLAdmin.Instances.List request = sqladmin.instances().list(parameters ...)}
*
*
* @return the resource collection
*/
public Instances instances() {
return new Instances();
}
/**
* The "instances" collection of methods.
*/
public class Instances {
/**
* Get Disk Shrink Config for a given instance.
*
* Create a request for the method "instances.getDiskShrinkConfig".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link GetDiskShrinkConfig#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @return the request
*/
public GetDiskShrinkConfig getDiskShrinkConfig(java.lang.String project, java.lang.String instance) throws java.io.IOException {
GetDiskShrinkConfig result = new GetDiskShrinkConfig(project, instance);
initialize(result);
return result;
}
public class GetDiskShrinkConfig extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/getDiskShrinkConfig";
/**
* Get Disk Shrink Config for a given instance.
*
* Create a request for the method "instances.getDiskShrinkConfig".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link GetDiskShrinkConfig#execute()} method to invoke the remote
* operation. {@link GetDiskShrinkConfig#initialize(com.google.api.client.googleapis.services.
* AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @since 1.13
*/
protected GetDiskShrinkConfig(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sqladmin.model.SqlInstancesGetDiskShrinkConfigResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetDiskShrinkConfig set$Xgafv(java.lang.String $Xgafv) {
return (GetDiskShrinkConfig) super.set$Xgafv($Xgafv);
}
@Override
public GetDiskShrinkConfig setAccessToken(java.lang.String accessToken) {
return (GetDiskShrinkConfig) super.setAccessToken(accessToken);
}
@Override
public GetDiskShrinkConfig setAlt(java.lang.String alt) {
return (GetDiskShrinkConfig) super.setAlt(alt);
}
@Override
public GetDiskShrinkConfig setCallback(java.lang.String callback) {
return (GetDiskShrinkConfig) super.setCallback(callback);
}
@Override
public GetDiskShrinkConfig setFields(java.lang.String fields) {
return (GetDiskShrinkConfig) super.setFields(fields);
}
@Override
public GetDiskShrinkConfig setKey(java.lang.String key) {
return (GetDiskShrinkConfig) super.setKey(key);
}
@Override
public GetDiskShrinkConfig setOauthToken(java.lang.String oauthToken) {
return (GetDiskShrinkConfig) super.setOauthToken(oauthToken);
}
@Override
public GetDiskShrinkConfig setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetDiskShrinkConfig) super.setPrettyPrint(prettyPrint);
}
@Override
public GetDiskShrinkConfig setQuotaUser(java.lang.String quotaUser) {
return (GetDiskShrinkConfig) super.setQuotaUser(quotaUser);
}
@Override
public GetDiskShrinkConfig setUploadType(java.lang.String uploadType) {
return (GetDiskShrinkConfig) super.setUploadType(uploadType);
}
@Override
public GetDiskShrinkConfig setUploadProtocol(java.lang.String uploadProtocol) {
return (GetDiskShrinkConfig) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public GetDiskShrinkConfig setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public GetDiskShrinkConfig setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public GetDiskShrinkConfig set(String parameterName, Object value) {
return (GetDiskShrinkConfig) super.set(parameterName, value);
}
}
/**
* Get Latest Recovery Time for a given instance.
*
* Create a request for the method "instances.getLatestRecoveryTime".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link GetLatestRecoveryTime#execute()} method to invoke the remote
* operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @return the request
*/
public GetLatestRecoveryTime getLatestRecoveryTime(java.lang.String project, java.lang.String instance) throws java.io.IOException {
GetLatestRecoveryTime result = new GetLatestRecoveryTime(project, instance);
initialize(result);
return result;
}
public class GetLatestRecoveryTime extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/getLatestRecoveryTime";
/**
* Get Latest Recovery Time for a given instance.
*
* Create a request for the method "instances.getLatestRecoveryTime".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link GetLatestRecoveryTime#execute()} method to invoke the
* remote operation. {@link GetLatestRecoveryTime#initialize(com.google.api.client.googleapis.
* services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @since 1.13
*/
protected GetLatestRecoveryTime(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sqladmin.model.SqlInstancesGetLatestRecoveryTimeResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetLatestRecoveryTime set$Xgafv(java.lang.String $Xgafv) {
return (GetLatestRecoveryTime) super.set$Xgafv($Xgafv);
}
@Override
public GetLatestRecoveryTime setAccessToken(java.lang.String accessToken) {
return (GetLatestRecoveryTime) super.setAccessToken(accessToken);
}
@Override
public GetLatestRecoveryTime setAlt(java.lang.String alt) {
return (GetLatestRecoveryTime) super.setAlt(alt);
}
@Override
public GetLatestRecoveryTime setCallback(java.lang.String callback) {
return (GetLatestRecoveryTime) super.setCallback(callback);
}
@Override
public GetLatestRecoveryTime setFields(java.lang.String fields) {
return (GetLatestRecoveryTime) super.setFields(fields);
}
@Override
public GetLatestRecoveryTime setKey(java.lang.String key) {
return (GetLatestRecoveryTime) super.setKey(key);
}
@Override
public GetLatestRecoveryTime setOauthToken(java.lang.String oauthToken) {
return (GetLatestRecoveryTime) super.setOauthToken(oauthToken);
}
@Override
public GetLatestRecoveryTime setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetLatestRecoveryTime) super.setPrettyPrint(prettyPrint);
}
@Override
public GetLatestRecoveryTime setQuotaUser(java.lang.String quotaUser) {
return (GetLatestRecoveryTime) super.setQuotaUser(quotaUser);
}
@Override
public GetLatestRecoveryTime setUploadType(java.lang.String uploadType) {
return (GetLatestRecoveryTime) super.setUploadType(uploadType);
}
@Override
public GetLatestRecoveryTime setUploadProtocol(java.lang.String uploadProtocol) {
return (GetLatestRecoveryTime) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public GetLatestRecoveryTime setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public GetLatestRecoveryTime setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public GetLatestRecoveryTime set(String parameterName, Object value) {
return (GetLatestRecoveryTime) super.set(parameterName, value);
}
}
/**
* Perform Disk Shrink on primary instance.
*
* Create a request for the method "instances.performDiskShrink".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link PerformDiskShrink#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.PerformDiskShrinkContext}
* @return the request
*/
public PerformDiskShrink performDiskShrink(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.PerformDiskShrinkContext content) throws java.io.IOException {
PerformDiskShrink result = new PerformDiskShrink(project, instance, content);
initialize(result);
return result;
}
public class PerformDiskShrink extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/performDiskShrink";
/**
* Perform Disk Shrink on primary instance.
*
* Create a request for the method "instances.performDiskShrink".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link PerformDiskShrink#execute()} method to invoke the remote
* operation. {@link PerformDiskShrink#initialize(com.google.api.client.googleapis.services.Ab
* stractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.PerformDiskShrinkContext}
* @since 1.13
*/
protected PerformDiskShrink(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.PerformDiskShrinkContext content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public PerformDiskShrink set$Xgafv(java.lang.String $Xgafv) {
return (PerformDiskShrink) super.set$Xgafv($Xgafv);
}
@Override
public PerformDiskShrink setAccessToken(java.lang.String accessToken) {
return (PerformDiskShrink) super.setAccessToken(accessToken);
}
@Override
public PerformDiskShrink setAlt(java.lang.String alt) {
return (PerformDiskShrink) super.setAlt(alt);
}
@Override
public PerformDiskShrink setCallback(java.lang.String callback) {
return (PerformDiskShrink) super.setCallback(callback);
}
@Override
public PerformDiskShrink setFields(java.lang.String fields) {
return (PerformDiskShrink) super.setFields(fields);
}
@Override
public PerformDiskShrink setKey(java.lang.String key) {
return (PerformDiskShrink) super.setKey(key);
}
@Override
public PerformDiskShrink setOauthToken(java.lang.String oauthToken) {
return (PerformDiskShrink) super.setOauthToken(oauthToken);
}
@Override
public PerformDiskShrink setPrettyPrint(java.lang.Boolean prettyPrint) {
return (PerformDiskShrink) super.setPrettyPrint(prettyPrint);
}
@Override
public PerformDiskShrink setQuotaUser(java.lang.String quotaUser) {
return (PerformDiskShrink) super.setQuotaUser(quotaUser);
}
@Override
public PerformDiskShrink setUploadType(java.lang.String uploadType) {
return (PerformDiskShrink) super.setUploadType(uploadType);
}
@Override
public PerformDiskShrink setUploadProtocol(java.lang.String uploadProtocol) {
return (PerformDiskShrink) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public PerformDiskShrink setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public PerformDiskShrink setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public PerformDiskShrink set(String parameterName, Object value) {
return (PerformDiskShrink) super.set(parameterName, value);
}
}
/**
* Reschedules the maintenance on the given instance.
*
* Create a request for the method "instances.rescheduleMaintenance".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link RescheduleMaintenance#execute()} method to invoke the remote
* operation.
*
* @param project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.SqlInstancesRescheduleMaintenanceRequestBody}
* @return the request
*/
public RescheduleMaintenance rescheduleMaintenance(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.SqlInstancesRescheduleMaintenanceRequestBody content) throws java.io.IOException {
RescheduleMaintenance result = new RescheduleMaintenance(project, instance, content);
initialize(result);
return result;
}
public class RescheduleMaintenance extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/rescheduleMaintenance";
/**
* Reschedules the maintenance on the given instance.
*
* Create a request for the method "instances.rescheduleMaintenance".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link RescheduleMaintenance#execute()} method to invoke the
* remote operation. {@link RescheduleMaintenance#initialize(com.google.api.client.googleapis.
* services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.SqlInstancesRescheduleMaintenanceRequestBody}
* @since 1.13
*/
protected RescheduleMaintenance(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.SqlInstancesRescheduleMaintenanceRequestBody content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public RescheduleMaintenance set$Xgafv(java.lang.String $Xgafv) {
return (RescheduleMaintenance) super.set$Xgafv($Xgafv);
}
@Override
public RescheduleMaintenance setAccessToken(java.lang.String accessToken) {
return (RescheduleMaintenance) super.setAccessToken(accessToken);
}
@Override
public RescheduleMaintenance setAlt(java.lang.String alt) {
return (RescheduleMaintenance) super.setAlt(alt);
}
@Override
public RescheduleMaintenance setCallback(java.lang.String callback) {
return (RescheduleMaintenance) super.setCallback(callback);
}
@Override
public RescheduleMaintenance setFields(java.lang.String fields) {
return (RescheduleMaintenance) super.setFields(fields);
}
@Override
public RescheduleMaintenance setKey(java.lang.String key) {
return (RescheduleMaintenance) super.setKey(key);
}
@Override
public RescheduleMaintenance setOauthToken(java.lang.String oauthToken) {
return (RescheduleMaintenance) super.setOauthToken(oauthToken);
}
@Override
public RescheduleMaintenance setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RescheduleMaintenance) super.setPrettyPrint(prettyPrint);
}
@Override
public RescheduleMaintenance setQuotaUser(java.lang.String quotaUser) {
return (RescheduleMaintenance) super.setQuotaUser(quotaUser);
}
@Override
public RescheduleMaintenance setUploadType(java.lang.String uploadType) {
return (RescheduleMaintenance) super.setUploadType(uploadType);
}
@Override
public RescheduleMaintenance setUploadProtocol(java.lang.String uploadProtocol) {
return (RescheduleMaintenance) super.setUploadProtocol(uploadProtocol);
}
/** ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** ID of the project that contains the instance. */
public RescheduleMaintenance setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public RescheduleMaintenance setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public RescheduleMaintenance set(String parameterName, Object value) {
return (RescheduleMaintenance) super.set(parameterName, value);
}
}
/**
* Reset Replica Size to primary instance disk size.
*
* Create a request for the method "instances.resetReplicaSize".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link ResetReplicaSize#execute()} method to invoke the remote operation.
*
* @param project ID of the project that contains the read replica.
* @param instance Cloud SQL read replica instance name.
* @param content the {@link com.google.api.services.sqladmin.model.SqlInstancesResetReplicaSizeRequest}
* @return the request
*/
public ResetReplicaSize resetReplicaSize(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.SqlInstancesResetReplicaSizeRequest content) throws java.io.IOException {
ResetReplicaSize result = new ResetReplicaSize(project, instance, content);
initialize(result);
return result;
}
public class ResetReplicaSize extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/resetReplicaSize";
/**
* Reset Replica Size to primary instance disk size.
*
* Create a request for the method "instances.resetReplicaSize".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link ResetReplicaSize#execute()} method to invoke the remote
* operation. {@link ResetReplicaSize#initialize(com.google.api.client.googleapis.services.Abs
* tractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param project ID of the project that contains the read replica.
* @param instance Cloud SQL read replica instance name.
* @param content the {@link com.google.api.services.sqladmin.model.SqlInstancesResetReplicaSizeRequest}
* @since 1.13
*/
protected ResetReplicaSize(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.SqlInstancesResetReplicaSizeRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public ResetReplicaSize set$Xgafv(java.lang.String $Xgafv) {
return (ResetReplicaSize) super.set$Xgafv($Xgafv);
}
@Override
public ResetReplicaSize setAccessToken(java.lang.String accessToken) {
return (ResetReplicaSize) super.setAccessToken(accessToken);
}
@Override
public ResetReplicaSize setAlt(java.lang.String alt) {
return (ResetReplicaSize) super.setAlt(alt);
}
@Override
public ResetReplicaSize setCallback(java.lang.String callback) {
return (ResetReplicaSize) super.setCallback(callback);
}
@Override
public ResetReplicaSize setFields(java.lang.String fields) {
return (ResetReplicaSize) super.setFields(fields);
}
@Override
public ResetReplicaSize setKey(java.lang.String key) {
return (ResetReplicaSize) super.setKey(key);
}
@Override
public ResetReplicaSize setOauthToken(java.lang.String oauthToken) {
return (ResetReplicaSize) super.setOauthToken(oauthToken);
}
@Override
public ResetReplicaSize setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ResetReplicaSize) super.setPrettyPrint(prettyPrint);
}
@Override
public ResetReplicaSize setQuotaUser(java.lang.String quotaUser) {
return (ResetReplicaSize) super.setQuotaUser(quotaUser);
}
@Override
public ResetReplicaSize setUploadType(java.lang.String uploadType) {
return (ResetReplicaSize) super.setUploadType(uploadType);
}
@Override
public ResetReplicaSize setUploadProtocol(java.lang.String uploadProtocol) {
return (ResetReplicaSize) super.setUploadProtocol(uploadProtocol);
}
/** ID of the project that contains the read replica. */
@com.google.api.client.util.Key
private java.lang.String project;
/** ID of the project that contains the read replica.
*/
public java.lang.String getProject() {
return project;
}
/** ID of the project that contains the read replica. */
public ResetReplicaSize setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL read replica instance name. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL read replica instance name.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL read replica instance name. */
public ResetReplicaSize setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public ResetReplicaSize set(String parameterName, Object value) {
return (ResetReplicaSize) super.set(parameterName, value);
}
}
/**
* Start External primary instance migration.
*
* Create a request for the method "instances.startExternalSync".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link StartExternalSync#execute()} method to invoke the remote operation.
*
* @param project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.SqlInstancesStartExternalSyncRequest}
* @return the request
*/
public StartExternalSync startExternalSync(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.SqlInstancesStartExternalSyncRequest content) throws java.io.IOException {
StartExternalSync result = new StartExternalSync(project, instance, content);
initialize(result);
return result;
}
public class StartExternalSync extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/startExternalSync";
/**
* Start External primary instance migration.
*
* Create a request for the method "instances.startExternalSync".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link StartExternalSync#execute()} method to invoke the remote
* operation. {@link StartExternalSync#initialize(com.google.api.client.googleapis.services.Ab
* stractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.SqlInstancesStartExternalSyncRequest}
* @since 1.13
*/
protected StartExternalSync(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.SqlInstancesStartExternalSyncRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public StartExternalSync set$Xgafv(java.lang.String $Xgafv) {
return (StartExternalSync) super.set$Xgafv($Xgafv);
}
@Override
public StartExternalSync setAccessToken(java.lang.String accessToken) {
return (StartExternalSync) super.setAccessToken(accessToken);
}
@Override
public StartExternalSync setAlt(java.lang.String alt) {
return (StartExternalSync) super.setAlt(alt);
}
@Override
public StartExternalSync setCallback(java.lang.String callback) {
return (StartExternalSync) super.setCallback(callback);
}
@Override
public StartExternalSync setFields(java.lang.String fields) {
return (StartExternalSync) super.setFields(fields);
}
@Override
public StartExternalSync setKey(java.lang.String key) {
return (StartExternalSync) super.setKey(key);
}
@Override
public StartExternalSync setOauthToken(java.lang.String oauthToken) {
return (StartExternalSync) super.setOauthToken(oauthToken);
}
@Override
public StartExternalSync setPrettyPrint(java.lang.Boolean prettyPrint) {
return (StartExternalSync) super.setPrettyPrint(prettyPrint);
}
@Override
public StartExternalSync setQuotaUser(java.lang.String quotaUser) {
return (StartExternalSync) super.setQuotaUser(quotaUser);
}
@Override
public StartExternalSync setUploadType(java.lang.String uploadType) {
return (StartExternalSync) super.setUploadType(uploadType);
}
@Override
public StartExternalSync setUploadProtocol(java.lang.String uploadProtocol) {
return (StartExternalSync) super.setUploadProtocol(uploadProtocol);
}
/** ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** ID of the project that contains the instance. */
public StartExternalSync setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public StartExternalSync setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public StartExternalSync set(String parameterName, Object value) {
return (StartExternalSync) super.set(parameterName, value);
}
}
/**
* Verify External primary instance external sync settings.
*
* Create a request for the method "instances.verifyExternalSyncSettings".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link VerifyExternalSyncSettings#execute()} method to invoke the remote
* operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.SqlInstancesVerifyExternalSyncSettingsRequest}
* @return the request
*/
public VerifyExternalSyncSettings verifyExternalSyncSettings(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.SqlInstancesVerifyExternalSyncSettingsRequest content) throws java.io.IOException {
VerifyExternalSyncSettings result = new VerifyExternalSyncSettings(project, instance, content);
initialize(result);
return result;
}
public class VerifyExternalSyncSettings extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/verifyExternalSyncSettings";
/**
* Verify External primary instance external sync settings.
*
* Create a request for the method "instances.verifyExternalSyncSettings".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link VerifyExternalSyncSettings#execute()} method to invoke the
* remote operation. {@link VerifyExternalSyncSettings#initialize(com.google.api.client.google
* apis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.SqlInstancesVerifyExternalSyncSettingsRequest}
* @since 1.13
*/
protected VerifyExternalSyncSettings(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.SqlInstancesVerifyExternalSyncSettingsRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sqladmin.model.SqlInstancesVerifyExternalSyncSettingsResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public VerifyExternalSyncSettings set$Xgafv(java.lang.String $Xgafv) {
return (VerifyExternalSyncSettings) super.set$Xgafv($Xgafv);
}
@Override
public VerifyExternalSyncSettings setAccessToken(java.lang.String accessToken) {
return (VerifyExternalSyncSettings) super.setAccessToken(accessToken);
}
@Override
public VerifyExternalSyncSettings setAlt(java.lang.String alt) {
return (VerifyExternalSyncSettings) super.setAlt(alt);
}
@Override
public VerifyExternalSyncSettings setCallback(java.lang.String callback) {
return (VerifyExternalSyncSettings) super.setCallback(callback);
}
@Override
public VerifyExternalSyncSettings setFields(java.lang.String fields) {
return (VerifyExternalSyncSettings) super.setFields(fields);
}
@Override
public VerifyExternalSyncSettings setKey(java.lang.String key) {
return (VerifyExternalSyncSettings) super.setKey(key);
}
@Override
public VerifyExternalSyncSettings setOauthToken(java.lang.String oauthToken) {
return (VerifyExternalSyncSettings) super.setOauthToken(oauthToken);
}
@Override
public VerifyExternalSyncSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (VerifyExternalSyncSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public VerifyExternalSyncSettings setQuotaUser(java.lang.String quotaUser) {
return (VerifyExternalSyncSettings) super.setQuotaUser(quotaUser);
}
@Override
public VerifyExternalSyncSettings setUploadType(java.lang.String uploadType) {
return (VerifyExternalSyncSettings) super.setUploadType(uploadType);
}
@Override
public VerifyExternalSyncSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (VerifyExternalSyncSettings) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public VerifyExternalSyncSettings setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public VerifyExternalSyncSettings setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public VerifyExternalSyncSettings set(String parameterName, Object value) {
return (VerifyExternalSyncSettings) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the SslCerts collection.
*
* The typical use is:
*
* {@code SQLAdmin sqladmin = new SQLAdmin(...);}
* {@code SQLAdmin.SslCerts.List request = sqladmin.sslCerts().list(parameters ...)}
*
*
* @return the resource collection
*/
public SslCerts sslCerts() {
return new SslCerts();
}
/**
* The "sslCerts" collection of methods.
*/
public class SslCerts {
/**
* Generates a short-lived X509 certificate containing the provided public key and signed by a
* private key specific to the target instance. Users may use the certificate to authenticate as
* themselves when connecting to the database.
*
* Create a request for the method "sslCerts.createEphemeral".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link CreateEphemeral#execute()} method to invoke the remote operation.
*
* @param project Project ID of the Cloud SQL project.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.SslCertsCreateEphemeralRequest}
* @return the request
*/
public CreateEphemeral createEphemeral(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.SslCertsCreateEphemeralRequest content) throws java.io.IOException {
CreateEphemeral result = new CreateEphemeral(project, instance, content);
initialize(result);
return result;
}
public class CreateEphemeral extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/createEphemeral";
/**
* Generates a short-lived X509 certificate containing the provided public key and signed by a
* private key specific to the target instance. Users may use the certificate to authenticate as
* themselves when connecting to the database.
*
* Create a request for the method "sslCerts.createEphemeral".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link CreateEphemeral#execute()} method to invoke the remote
* operation. {@link CreateEphemeral#initialize(com.google.api.client.googleapis.services.Abst
* ractGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param project Project ID of the Cloud SQL project.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.SslCertsCreateEphemeralRequest}
* @since 1.13
*/
protected CreateEphemeral(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.SslCertsCreateEphemeralRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sqladmin.model.SslCert.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public CreateEphemeral set$Xgafv(java.lang.String $Xgafv) {
return (CreateEphemeral) super.set$Xgafv($Xgafv);
}
@Override
public CreateEphemeral setAccessToken(java.lang.String accessToken) {
return (CreateEphemeral) super.setAccessToken(accessToken);
}
@Override
public CreateEphemeral setAlt(java.lang.String alt) {
return (CreateEphemeral) super.setAlt(alt);
}
@Override
public CreateEphemeral setCallback(java.lang.String callback) {
return (CreateEphemeral) super.setCallback(callback);
}
@Override
public CreateEphemeral setFields(java.lang.String fields) {
return (CreateEphemeral) super.setFields(fields);
}
@Override
public CreateEphemeral setKey(java.lang.String key) {
return (CreateEphemeral) super.setKey(key);
}
@Override
public CreateEphemeral setOauthToken(java.lang.String oauthToken) {
return (CreateEphemeral) super.setOauthToken(oauthToken);
}
@Override
public CreateEphemeral setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CreateEphemeral) super.setPrettyPrint(prettyPrint);
}
@Override
public CreateEphemeral setQuotaUser(java.lang.String quotaUser) {
return (CreateEphemeral) super.setQuotaUser(quotaUser);
}
@Override
public CreateEphemeral setUploadType(java.lang.String uploadType) {
return (CreateEphemeral) super.setUploadType(uploadType);
}
@Override
public CreateEphemeral setUploadProtocol(java.lang.String uploadProtocol) {
return (CreateEphemeral) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the Cloud SQL project. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the Cloud SQL project.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the Cloud SQL project. */
public CreateEphemeral setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public CreateEphemeral setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public CreateEphemeral set(String parameterName, Object value) {
return (CreateEphemeral) super.set(parameterName, value);
}
}
/**
* Deletes the SSL certificate. For First Generation instances, the certificate remains valid until
* the instance is restarted.
*
* Create a request for the method "sslCerts.delete".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param sha1Fingerprint Sha1 FingerPrint.
* @return the request
*/
public Delete delete(java.lang.String project, java.lang.String instance, java.lang.String sha1Fingerprint) throws java.io.IOException {
Delete result = new Delete(project, instance, sha1Fingerprint);
initialize(result);
return result;
}
public class Delete extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/sslCerts/{sha1Fingerprint}";
/**
* Deletes the SSL certificate. For First Generation instances, the certificate remains valid
* until the instance is restarted.
*
* Create a request for the method "sslCerts.delete".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param sha1Fingerprint Sha1 FingerPrint.
* @since 1.13
*/
protected Delete(java.lang.String project, java.lang.String instance, java.lang.String sha1Fingerprint) {
super(SQLAdmin.this, "DELETE", REST_PATH, null, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
this.sha1Fingerprint = com.google.api.client.util.Preconditions.checkNotNull(sha1Fingerprint, "Required parameter sha1Fingerprint must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Delete setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Delete setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** Sha1 FingerPrint. */
@com.google.api.client.util.Key
private java.lang.String sha1Fingerprint;
/** Sha1 FingerPrint.
*/
public java.lang.String getSha1Fingerprint() {
return sha1Fingerprint;
}
/** Sha1 FingerPrint. */
public Delete setSha1Fingerprint(java.lang.String sha1Fingerprint) {
this.sha1Fingerprint = sha1Fingerprint;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves a particular SSL certificate. Does not include the private key (required for usage).
* The private key must be saved from the response to initial creation.
*
* Create a request for the method "sslCerts.get".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param sha1Fingerprint Sha1 FingerPrint.
* @return the request
*/
public Get get(java.lang.String project, java.lang.String instance, java.lang.String sha1Fingerprint) throws java.io.IOException {
Get result = new Get(project, instance, sha1Fingerprint);
initialize(result);
return result;
}
public class Get extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/sslCerts/{sha1Fingerprint}";
/**
* Retrieves a particular SSL certificate. Does not include the private key (required for usage).
* The private key must be saved from the response to initial creation.
*
* Create a request for the method "sslCerts.get".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param sha1Fingerprint Sha1 FingerPrint.
* @since 1.13
*/
protected Get(java.lang.String project, java.lang.String instance, java.lang.String sha1Fingerprint) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sqladmin.model.SslCert.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
this.sha1Fingerprint = com.google.api.client.util.Preconditions.checkNotNull(sha1Fingerprint, "Required parameter sha1Fingerprint must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Get setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Get setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** Sha1 FingerPrint. */
@com.google.api.client.util.Key
private java.lang.String sha1Fingerprint;
/** Sha1 FingerPrint.
*/
public java.lang.String getSha1Fingerprint() {
return sha1Fingerprint;
}
/** Sha1 FingerPrint. */
public Get setSha1Fingerprint(java.lang.String sha1Fingerprint) {
this.sha1Fingerprint = sha1Fingerprint;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Creates an SSL certificate and returns it along with the private key and server certificate
* authority. The new certificate will not be usable until the instance is restarted.
*
* Create a request for the method "sslCerts.insert".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.SslCertsInsertRequest}
* @return the request
*/
public Insert insert(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.SslCertsInsertRequest content) throws java.io.IOException {
Insert result = new Insert(project, instance, content);
initialize(result);
return result;
}
public class Insert extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/sslCerts";
/**
* Creates an SSL certificate and returns it along with the private key and server certificate
* authority. The new certificate will not be usable until the instance is restarted.
*
* Create a request for the method "sslCerts.insert".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.SslCertsInsertRequest}
* @since 1.13
*/
protected Insert(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.SslCertsInsertRequest content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sqladmin.model.SslCertsInsertResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Insert setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public Insert setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Lists all of the current SSL certificates for the instance.
*
* Create a request for the method "sslCerts.list".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @return the request
*/
public List list(java.lang.String project, java.lang.String instance) throws java.io.IOException {
List result = new List(project, instance);
initialize(result);
return result;
}
public class List extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/sslCerts";
/**
* Lists all of the current SSL certificates for the instance.
*
* Create a request for the method "sslCerts.list".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Cloud SQL instance ID. This does not include the project ID.
* @since 1.13
*/
protected List(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sqladmin.model.SslCertsListResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public List setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Cloud SQL instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Cloud SQL instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Cloud SQL instance ID. This does not include the project ID. */
public List setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Tiers collection.
*
* The typical use is:
*
* {@code SQLAdmin sqladmin = new SQLAdmin(...);}
* {@code SQLAdmin.Tiers.List request = sqladmin.tiers().list(parameters ...)}
*
*
* @return the resource collection
*/
public Tiers tiers() {
return new Tiers();
}
/**
* The "tiers" collection of methods.
*/
public class Tiers {
/**
* Lists all available machine types (tiers) for Cloud SQL, for example, `db-custom-1-3840`. For
* more information, see https://cloud.google.com/sql/pricing.
*
* Create a request for the method "tiers.list".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project for which to list tiers.
* @return the request
*/
public List list(java.lang.String project) throws java.io.IOException {
List result = new List(project);
initialize(result);
return result;
}
public class List extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/tiers";
/**
* Lists all available machine types (tiers) for Cloud SQL, for example, `db-custom-1-3840`. For
* more information, see https://cloud.google.com/sql/pricing.
*
* Create a request for the method "tiers.list".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project for which to list tiers.
* @since 1.13
*/
protected List(java.lang.String project) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sqladmin.model.TiersListResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project for which to list tiers. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project for which to list tiers.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project for which to list tiers. */
public List setProject(java.lang.String project) {
this.project = project;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Users collection.
*
* The typical use is:
*
* {@code SQLAdmin sqladmin = new SQLAdmin(...);}
* {@code SQLAdmin.Users.List request = sqladmin.users().list(parameters ...)}
*
*
* @return the resource collection
*/
public Users users() {
return new Users();
}
/**
* The "users" collection of methods.
*/
public class Users {
/**
* Deletes a user from a Cloud SQL instance.
*
* Create a request for the method "users.delete".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @return the request
*/
public Delete delete(java.lang.String project, java.lang.String instance) throws java.io.IOException {
Delete result = new Delete(project, instance);
initialize(result);
return result;
}
public class Delete extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/users";
/**
* Deletes a user from a Cloud SQL instance.
*
* Create a request for the method "users.delete".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @since 1.13
*/
protected Delete(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "DELETE", REST_PATH, null, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Delete setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Database instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Database instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Database instance ID. This does not include the project ID. */
public Delete setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** Host of the user in the instance. */
@com.google.api.client.util.Key
private java.lang.String host;
/** Host of the user in the instance.
*/
public java.lang.String getHost() {
return host;
}
/** Host of the user in the instance. */
public Delete setHost(java.lang.String host) {
this.host = host;
return this;
}
/** Name of the user in the instance. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the user in the instance.
*/
public java.lang.String getName() {
return name;
}
/** Name of the user in the instance. */
public Delete setName(java.lang.String name) {
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves a resource containing information about a user.
*
* Create a request for the method "users.get".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param name User of the instance.
* @return the request
*/
public Get get(java.lang.String project, java.lang.String instance, java.lang.String name) throws java.io.IOException {
Get result = new Get(project, instance, name);
initialize(result);
return result;
}
public class Get extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/users/{name}";
/**
* Retrieves a resource containing information about a user.
*
* Create a request for the method "users.get".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param name User of the instance.
* @since 1.13
*/
protected Get(java.lang.String project, java.lang.String instance, java.lang.String name) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sqladmin.model.User.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Get setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Database instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Database instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Database instance ID. This does not include the project ID. */
public Get setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** User of the instance. */
@com.google.api.client.util.Key
private java.lang.String name;
/** User of the instance.
*/
public java.lang.String getName() {
return name;
}
/** User of the instance. */
public Get setName(java.lang.String name) {
this.name = name;
return this;
}
/** Host of a user of the instance. */
@com.google.api.client.util.Key
private java.lang.String host;
/** Host of a user of the instance.
*/
public java.lang.String getHost() {
return host;
}
/** Host of a user of the instance. */
public Get setHost(java.lang.String host) {
this.host = host;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Creates a new user in a Cloud SQL instance.
*
* Create a request for the method "users.insert".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.User}
* @return the request
*/
public Insert insert(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.User content) throws java.io.IOException {
Insert result = new Insert(project, instance, content);
initialize(result);
return result;
}
public class Insert extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/users";
/**
* Creates a new user in a Cloud SQL instance.
*
* Create a request for the method "users.insert".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.User}
* @since 1.13
*/
protected Insert(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.User content) {
super(SQLAdmin.this, "POST", REST_PATH, content, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Insert setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Database instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Database instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Database instance ID. This does not include the project ID. */
public Insert setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Lists users in the specified Cloud SQL instance.
*
* Create a request for the method "users.list".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @return the request
*/
public List list(java.lang.String project, java.lang.String instance) throws java.io.IOException {
List result = new List(project, instance);
initialize(result);
return result;
}
public class List extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/users";
/**
* Lists users in the specified Cloud SQL instance.
*
* Create a request for the method "users.list".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @since 1.13
*/
protected List(java.lang.String project, java.lang.String instance) {
super(SQLAdmin.this, "GET", REST_PATH, null, com.google.api.services.sqladmin.model.UsersListResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public List setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Database instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Database instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Database instance ID. This does not include the project ID. */
public List setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing user in a Cloud SQL instance.
*
* Create a request for the method "users.update".
*
* This request holds the parameters needed by the sqladmin server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.User}
* @return the request
*/
public Update update(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.User content) throws java.io.IOException {
Update result = new Update(project, instance, content);
initialize(result);
return result;
}
public class Update extends SQLAdminRequest {
private static final String REST_PATH = "v1/projects/{project}/instances/{instance}/users";
/**
* Updates an existing user in a Cloud SQL instance.
*
* Create a request for the method "users.update".
*
* This request holds the parameters needed by the the sqladmin server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID of the project that contains the instance.
* @param instance Database instance ID. This does not include the project ID.
* @param content the {@link com.google.api.services.sqladmin.model.User}
* @since 1.13
*/
protected Update(java.lang.String project, java.lang.String instance, com.google.api.services.sqladmin.model.User content) {
super(SQLAdmin.this, "PUT", REST_PATH, content, com.google.api.services.sqladmin.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** Project ID of the project that contains the instance. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID of the project that contains the instance.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID of the project that contains the instance. */
public Update setProject(java.lang.String project) {
this.project = project;
return this;
}
/** Database instance ID. This does not include the project ID. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** Database instance ID. This does not include the project ID.
*/
public java.lang.String getInstance() {
return instance;
}
/** Database instance ID. This does not include the project ID. */
public Update setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/** Optional. Host of the user in the instance. */
@com.google.api.client.util.Key
private java.lang.String host;
/** Optional. Host of the user in the instance.
*/
public java.lang.String getHost() {
return host;
}
/** Optional. Host of the user in the instance. */
public Update setHost(java.lang.String host) {
this.host = host;
return this;
}
/** Name of the user in the instance. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the user in the instance.
*/
public java.lang.String getName() {
return name;
}
/** Name of the user in the instance. */
public Update setName(java.lang.String name) {
this.name = name;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* Builder for {@link SQLAdmin}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link SQLAdmin}. */
@Override
public SQLAdmin build() {
return new SQLAdmin(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link SQLAdminRequestInitializer}.
*
* @since 1.12
*/
public Builder setSQLAdminRequestInitializer(
SQLAdminRequestInitializer sqladminRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(sqladminRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}