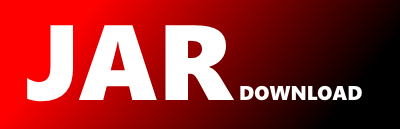
com.google.api.services.sqladmin.model.ExportContext Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.sqladmin.model;
/**
* Database instance export context.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud SQL Admin API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ExportContext extends com.google.api.client.json.GenericJson {
/**
* Options for exporting BAK files (SQL Server-only)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private BakExportOptions bakExportOptions;
/**
* Options for exporting data as CSV. `MySQL` and `PostgreSQL` instances only.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private CsvExportOptions csvExportOptions;
/**
* Databases to be exported. `MySQL instances:` If `fileType` is `SQL` and no database is
* specified, all databases are exported, except for the `mysql` system database. If `fileType` is
* `CSV`, you can specify one database, either by using this property or by using the
* `csvExportOptions.selectQuery` property, which takes precedence over this property. `PostgreSQL
* instances:` You must specify one database to be exported. If `fileType` is `CSV`, this database
* must match the one specified in the `csvExportOptions.selectQuery` property. `SQL Server
* instances:` You must specify one database to be exported, and the `fileType` must be `BAK`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List databases;
/**
* The file type for the specified uri.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String fileType;
/**
* This is always `sql#exportContext`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* Option for export offload.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean offload;
/**
* Options for exporting data as SQL statements.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SqlExportOptions sqlExportOptions;
/**
* The path to the file in Google Cloud Storage where the export will be stored. The URI is in the
* form `gs://bucketName/fileName`. If the file already exists, the request succeeds, but the
* operation fails. If `fileType` is `SQL` and the filename ends with .gz, the contents are
* compressed.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String uri;
/**
* Options for exporting BAK files (SQL Server-only)
* @return value or {@code null} for none
*/
public BakExportOptions getBakExportOptions() {
return bakExportOptions;
}
/**
* Options for exporting BAK files (SQL Server-only)
* @param bakExportOptions bakExportOptions or {@code null} for none
*/
public ExportContext setBakExportOptions(BakExportOptions bakExportOptions) {
this.bakExportOptions = bakExportOptions;
return this;
}
/**
* Options for exporting data as CSV. `MySQL` and `PostgreSQL` instances only.
* @return value or {@code null} for none
*/
public CsvExportOptions getCsvExportOptions() {
return csvExportOptions;
}
/**
* Options for exporting data as CSV. `MySQL` and `PostgreSQL` instances only.
* @param csvExportOptions csvExportOptions or {@code null} for none
*/
public ExportContext setCsvExportOptions(CsvExportOptions csvExportOptions) {
this.csvExportOptions = csvExportOptions;
return this;
}
/**
* Databases to be exported. `MySQL instances:` If `fileType` is `SQL` and no database is
* specified, all databases are exported, except for the `mysql` system database. If `fileType` is
* `CSV`, you can specify one database, either by using this property or by using the
* `csvExportOptions.selectQuery` property, which takes precedence over this property. `PostgreSQL
* instances:` You must specify one database to be exported. If `fileType` is `CSV`, this database
* must match the one specified in the `csvExportOptions.selectQuery` property. `SQL Server
* instances:` You must specify one database to be exported, and the `fileType` must be `BAK`.
* @return value or {@code null} for none
*/
public java.util.List getDatabases() {
return databases;
}
/**
* Databases to be exported. `MySQL instances:` If `fileType` is `SQL` and no database is
* specified, all databases are exported, except for the `mysql` system database. If `fileType` is
* `CSV`, you can specify one database, either by using this property or by using the
* `csvExportOptions.selectQuery` property, which takes precedence over this property. `PostgreSQL
* instances:` You must specify one database to be exported. If `fileType` is `CSV`, this database
* must match the one specified in the `csvExportOptions.selectQuery` property. `SQL Server
* instances:` You must specify one database to be exported, and the `fileType` must be `BAK`.
* @param databases databases or {@code null} for none
*/
public ExportContext setDatabases(java.util.List databases) {
this.databases = databases;
return this;
}
/**
* The file type for the specified uri.
* @return value or {@code null} for none
*/
public java.lang.String getFileType() {
return fileType;
}
/**
* The file type for the specified uri.
* @param fileType fileType or {@code null} for none
*/
public ExportContext setFileType(java.lang.String fileType) {
this.fileType = fileType;
return this;
}
/**
* This is always `sql#exportContext`.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* This is always `sql#exportContext`.
* @param kind kind or {@code null} for none
*/
public ExportContext setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* Option for export offload.
* @return value or {@code null} for none
*/
public java.lang.Boolean getOffload() {
return offload;
}
/**
* Option for export offload.
* @param offload offload or {@code null} for none
*/
public ExportContext setOffload(java.lang.Boolean offload) {
this.offload = offload;
return this;
}
/**
* Options for exporting data as SQL statements.
* @return value or {@code null} for none
*/
public SqlExportOptions getSqlExportOptions() {
return sqlExportOptions;
}
/**
* Options for exporting data as SQL statements.
* @param sqlExportOptions sqlExportOptions or {@code null} for none
*/
public ExportContext setSqlExportOptions(SqlExportOptions sqlExportOptions) {
this.sqlExportOptions = sqlExportOptions;
return this;
}
/**
* The path to the file in Google Cloud Storage where the export will be stored. The URI is in the
* form `gs://bucketName/fileName`. If the file already exists, the request succeeds, but the
* operation fails. If `fileType` is `SQL` and the filename ends with .gz, the contents are
* compressed.
* @return value or {@code null} for none
*/
public java.lang.String getUri() {
return uri;
}
/**
* The path to the file in Google Cloud Storage where the export will be stored. The URI is in the
* form `gs://bucketName/fileName`. If the file already exists, the request succeeds, but the
* operation fails. If `fileType` is `SQL` and the filename ends with .gz, the contents are
* compressed.
* @param uri uri or {@code null} for none
*/
public ExportContext setUri(java.lang.String uri) {
this.uri = uri;
return this;
}
@Override
public ExportContext set(String fieldName, Object value) {
return (ExportContext) super.set(fieldName, value);
}
@Override
public ExportContext clone() {
return (ExportContext) super.clone();
}
/**
* Options for exporting BAK files (SQL Server-only)
*/
public static final class BakExportOptions extends com.google.api.client.json.GenericJson {
/**
* Type of this bak file will be export, FULL or DIFF, SQL Server only
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String bakType;
/**
* Deprecated: copy_only is deprecated. Use differential_base instead
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean copyOnly;
/**
* Whether or not the backup can be used as a differential base copy_only backup can not be served
* as differential base
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean differentialBase;
/**
* Optional. The end timestamp when transaction log will be included in the export operation. [RFC
* 3339](https://tools.ietf.org/html/rfc3339) format (for example, `2023-10-01T16:19:00.094`) in
* UTC. When omitted, all available logs until current time will be included. Only applied to
* Cloud SQL for SQL Server.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String exportLogEndTime;
/**
* Optional. The begin timestamp when transaction log will be included in the export operation.
* [RFC 3339](https://tools.ietf.org/html/rfc3339) format (for example, `2023-10-01T16:19:00.094`)
* in UTC. When omitted, all available logs from the beginning of retention period will be
* included. Only applied to Cloud SQL for SQL Server.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String exportLogStartTime;
/**
* Option for specifying how many stripes to use for the export. If blank, and the value of the
* striped field is true, the number of stripes is automatically chosen.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer stripeCount;
/**
* Whether or not the export should be striped.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean striped;
/**
* Type of this bak file will be export, FULL or DIFF, SQL Server only
* @return value or {@code null} for none
*/
public java.lang.String getBakType() {
return bakType;
}
/**
* Type of this bak file will be export, FULL or DIFF, SQL Server only
* @param bakType bakType or {@code null} for none
*/
public BakExportOptions setBakType(java.lang.String bakType) {
this.bakType = bakType;
return this;
}
/**
* Deprecated: copy_only is deprecated. Use differential_base instead
* @return value or {@code null} for none
*/
public java.lang.Boolean getCopyOnly() {
return copyOnly;
}
/**
* Deprecated: copy_only is deprecated. Use differential_base instead
* @param copyOnly copyOnly or {@code null} for none
*/
public BakExportOptions setCopyOnly(java.lang.Boolean copyOnly) {
this.copyOnly = copyOnly;
return this;
}
/**
* Whether or not the backup can be used as a differential base copy_only backup can not be served
* as differential base
* @return value or {@code null} for none
*/
public java.lang.Boolean getDifferentialBase() {
return differentialBase;
}
/**
* Whether or not the backup can be used as a differential base copy_only backup can not be served
* as differential base
* @param differentialBase differentialBase or {@code null} for none
*/
public BakExportOptions setDifferentialBase(java.lang.Boolean differentialBase) {
this.differentialBase = differentialBase;
return this;
}
/**
* Optional. The end timestamp when transaction log will be included in the export operation. [RFC
* 3339](https://tools.ietf.org/html/rfc3339) format (for example, `2023-10-01T16:19:00.094`) in
* UTC. When omitted, all available logs until current time will be included. Only applied to
* Cloud SQL for SQL Server.
* @return value or {@code null} for none
*/
public String getExportLogEndTime() {
return exportLogEndTime;
}
/**
* Optional. The end timestamp when transaction log will be included in the export operation. [RFC
* 3339](https://tools.ietf.org/html/rfc3339) format (for example, `2023-10-01T16:19:00.094`) in
* UTC. When omitted, all available logs until current time will be included. Only applied to
* Cloud SQL for SQL Server.
* @param exportLogEndTime exportLogEndTime or {@code null} for none
*/
public BakExportOptions setExportLogEndTime(String exportLogEndTime) {
this.exportLogEndTime = exportLogEndTime;
return this;
}
/**
* Optional. The begin timestamp when transaction log will be included in the export operation.
* [RFC 3339](https://tools.ietf.org/html/rfc3339) format (for example, `2023-10-01T16:19:00.094`)
* in UTC. When omitted, all available logs from the beginning of retention period will be
* included. Only applied to Cloud SQL for SQL Server.
* @return value or {@code null} for none
*/
public String getExportLogStartTime() {
return exportLogStartTime;
}
/**
* Optional. The begin timestamp when transaction log will be included in the export operation.
* [RFC 3339](https://tools.ietf.org/html/rfc3339) format (for example, `2023-10-01T16:19:00.094`)
* in UTC. When omitted, all available logs from the beginning of retention period will be
* included. Only applied to Cloud SQL for SQL Server.
* @param exportLogStartTime exportLogStartTime or {@code null} for none
*/
public BakExportOptions setExportLogStartTime(String exportLogStartTime) {
this.exportLogStartTime = exportLogStartTime;
return this;
}
/**
* Option for specifying how many stripes to use for the export. If blank, and the value of the
* striped field is true, the number of stripes is automatically chosen.
* @return value or {@code null} for none
*/
public java.lang.Integer getStripeCount() {
return stripeCount;
}
/**
* Option for specifying how many stripes to use for the export. If blank, and the value of the
* striped field is true, the number of stripes is automatically chosen.
* @param stripeCount stripeCount or {@code null} for none
*/
public BakExportOptions setStripeCount(java.lang.Integer stripeCount) {
this.stripeCount = stripeCount;
return this;
}
/**
* Whether or not the export should be striped.
* @return value or {@code null} for none
*/
public java.lang.Boolean getStriped() {
return striped;
}
/**
* Whether or not the export should be striped.
* @param striped striped or {@code null} for none
*/
public BakExportOptions setStriped(java.lang.Boolean striped) {
this.striped = striped;
return this;
}
@Override
public BakExportOptions set(String fieldName, Object value) {
return (BakExportOptions) super.set(fieldName, value);
}
@Override
public BakExportOptions clone() {
return (BakExportOptions) super.clone();
}
}
/**
* Options for exporting data as CSV. `MySQL` and `PostgreSQL` instances only.
*/
public static final class CsvExportOptions extends com.google.api.client.json.GenericJson {
/**
* Specifies the character that should appear before a data character that needs to be escaped.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String escapeCharacter;
/**
* Specifies the character that separates columns within each row (line) of the file.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String fieldsTerminatedBy;
/**
* This is used to separate lines. If a line does not contain all fields, the rest of the columns
* are set to their default values.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String linesTerminatedBy;
/**
* Specifies the quoting character to be used when a data value is quoted.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String quoteCharacter;
/**
* The select query used to extract the data.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String selectQuery;
/**
* Specifies the character that should appear before a data character that needs to be escaped.
* @return value or {@code null} for none
*/
public java.lang.String getEscapeCharacter() {
return escapeCharacter;
}
/**
* Specifies the character that should appear before a data character that needs to be escaped.
* @param escapeCharacter escapeCharacter or {@code null} for none
*/
public CsvExportOptions setEscapeCharacter(java.lang.String escapeCharacter) {
this.escapeCharacter = escapeCharacter;
return this;
}
/**
* Specifies the character that separates columns within each row (line) of the file.
* @return value or {@code null} for none
*/
public java.lang.String getFieldsTerminatedBy() {
return fieldsTerminatedBy;
}
/**
* Specifies the character that separates columns within each row (line) of the file.
* @param fieldsTerminatedBy fieldsTerminatedBy or {@code null} for none
*/
public CsvExportOptions setFieldsTerminatedBy(java.lang.String fieldsTerminatedBy) {
this.fieldsTerminatedBy = fieldsTerminatedBy;
return this;
}
/**
* This is used to separate lines. If a line does not contain all fields, the rest of the columns
* are set to their default values.
* @return value or {@code null} for none
*/
public java.lang.String getLinesTerminatedBy() {
return linesTerminatedBy;
}
/**
* This is used to separate lines. If a line does not contain all fields, the rest of the columns
* are set to their default values.
* @param linesTerminatedBy linesTerminatedBy or {@code null} for none
*/
public CsvExportOptions setLinesTerminatedBy(java.lang.String linesTerminatedBy) {
this.linesTerminatedBy = linesTerminatedBy;
return this;
}
/**
* Specifies the quoting character to be used when a data value is quoted.
* @return value or {@code null} for none
*/
public java.lang.String getQuoteCharacter() {
return quoteCharacter;
}
/**
* Specifies the quoting character to be used when a data value is quoted.
* @param quoteCharacter quoteCharacter or {@code null} for none
*/
public CsvExportOptions setQuoteCharacter(java.lang.String quoteCharacter) {
this.quoteCharacter = quoteCharacter;
return this;
}
/**
* The select query used to extract the data.
* @return value or {@code null} for none
*/
public java.lang.String getSelectQuery() {
return selectQuery;
}
/**
* The select query used to extract the data.
* @param selectQuery selectQuery or {@code null} for none
*/
public CsvExportOptions setSelectQuery(java.lang.String selectQuery) {
this.selectQuery = selectQuery;
return this;
}
@Override
public CsvExportOptions set(String fieldName, Object value) {
return (CsvExportOptions) super.set(fieldName, value);
}
@Override
public CsvExportOptions clone() {
return (CsvExportOptions) super.clone();
}
}
/**
* Options for exporting data as SQL statements.
*/
public static final class SqlExportOptions extends com.google.api.client.json.GenericJson {
/**
* Options for exporting from MySQL.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private MysqlExportOptions mysqlExportOptions;
/**
* Optional. Whether or not the export should be parallel.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean parallel;
/**
* Options for exporting from a Cloud SQL for PostgreSQL instance.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private PostgresExportOptions postgresExportOptions;
/**
* Export only schemas.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean schemaOnly;
/**
* Tables to export, or that were exported, from the specified database. If you specify tables,
* specify one and only one database. For PostgreSQL instances, you can specify only one table.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List tables;
/**
* Optional. The number of threads to use for parallel export.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer threads;
/**
* Options for exporting from MySQL.
* @return value or {@code null} for none
*/
public MysqlExportOptions getMysqlExportOptions() {
return mysqlExportOptions;
}
/**
* Options for exporting from MySQL.
* @param mysqlExportOptions mysqlExportOptions or {@code null} for none
*/
public SqlExportOptions setMysqlExportOptions(MysqlExportOptions mysqlExportOptions) {
this.mysqlExportOptions = mysqlExportOptions;
return this;
}
/**
* Optional. Whether or not the export should be parallel.
* @return value or {@code null} for none
*/
public java.lang.Boolean getParallel() {
return parallel;
}
/**
* Optional. Whether or not the export should be parallel.
* @param parallel parallel or {@code null} for none
*/
public SqlExportOptions setParallel(java.lang.Boolean parallel) {
this.parallel = parallel;
return this;
}
/**
* Options for exporting from a Cloud SQL for PostgreSQL instance.
* @return value or {@code null} for none
*/
public PostgresExportOptions getPostgresExportOptions() {
return postgresExportOptions;
}
/**
* Options for exporting from a Cloud SQL for PostgreSQL instance.
* @param postgresExportOptions postgresExportOptions or {@code null} for none
*/
public SqlExportOptions setPostgresExportOptions(PostgresExportOptions postgresExportOptions) {
this.postgresExportOptions = postgresExportOptions;
return this;
}
/**
* Export only schemas.
* @return value or {@code null} for none
*/
public java.lang.Boolean getSchemaOnly() {
return schemaOnly;
}
/**
* Export only schemas.
* @param schemaOnly schemaOnly or {@code null} for none
*/
public SqlExportOptions setSchemaOnly(java.lang.Boolean schemaOnly) {
this.schemaOnly = schemaOnly;
return this;
}
/**
* Tables to export, or that were exported, from the specified database. If you specify tables,
* specify one and only one database. For PostgreSQL instances, you can specify only one table.
* @return value or {@code null} for none
*/
public java.util.List getTables() {
return tables;
}
/**
* Tables to export, or that were exported, from the specified database. If you specify tables,
* specify one and only one database. For PostgreSQL instances, you can specify only one table.
* @param tables tables or {@code null} for none
*/
public SqlExportOptions setTables(java.util.List tables) {
this.tables = tables;
return this;
}
/**
* Optional. The number of threads to use for parallel export.
* @return value or {@code null} for none
*/
public java.lang.Integer getThreads() {
return threads;
}
/**
* Optional. The number of threads to use for parallel export.
* @param threads threads or {@code null} for none
*/
public SqlExportOptions setThreads(java.lang.Integer threads) {
this.threads = threads;
return this;
}
@Override
public SqlExportOptions set(String fieldName, Object value) {
return (SqlExportOptions) super.set(fieldName, value);
}
@Override
public SqlExportOptions clone() {
return (SqlExportOptions) super.clone();
}
/**
* Options for exporting from MySQL.
*/
public static final class MysqlExportOptions extends com.google.api.client.json.GenericJson {
/**
* Option to include SQL statement required to set up replication. If set to `1`, the dump file
* includes a CHANGE MASTER TO statement with the binary log coordinates, and --set-gtid-purged is
* set to ON. If set to `2`, the CHANGE MASTER TO statement is written as a SQL comment and has no
* effect. If set to any value other than `1`, --set-gtid-purged is set to OFF.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer masterData;
/**
* Option to include SQL statement required to set up replication. If set to `1`, the dump file
* includes a CHANGE MASTER TO statement with the binary log coordinates, and --set-gtid-purged is
* set to ON. If set to `2`, the CHANGE MASTER TO statement is written as a SQL comment and has no
* effect. If set to any value other than `1`, --set-gtid-purged is set to OFF.
* @return value or {@code null} for none
*/
public java.lang.Integer getMasterData() {
return masterData;
}
/**
* Option to include SQL statement required to set up replication. If set to `1`, the dump file
* includes a CHANGE MASTER TO statement with the binary log coordinates, and --set-gtid-purged is
* set to ON. If set to `2`, the CHANGE MASTER TO statement is written as a SQL comment and has no
* effect. If set to any value other than `1`, --set-gtid-purged is set to OFF.
* @param masterData masterData or {@code null} for none
*/
public MysqlExportOptions setMasterData(java.lang.Integer masterData) {
this.masterData = masterData;
return this;
}
@Override
public MysqlExportOptions set(String fieldName, Object value) {
return (MysqlExportOptions) super.set(fieldName, value);
}
@Override
public MysqlExportOptions clone() {
return (MysqlExportOptions) super.clone();
}
}
/**
* Options for exporting from a Cloud SQL for PostgreSQL instance.
*/
public static final class PostgresExportOptions extends com.google.api.client.json.GenericJson {
/**
* Optional. Use this option to include DROP SQL statements. These statements are used to delete
* database objects before running the import operation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean clean;
/**
* Optional. Option to include an IF EXISTS SQL statement with each DROP statement produced by
* clean.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean ifExists;
/**
* Optional. Use this option to include DROP SQL statements. These statements are used to delete
* database objects before running the import operation.
* @return value or {@code null} for none
*/
public java.lang.Boolean getClean() {
return clean;
}
/**
* Optional. Use this option to include DROP SQL statements. These statements are used to delete
* database objects before running the import operation.
* @param clean clean or {@code null} for none
*/
public PostgresExportOptions setClean(java.lang.Boolean clean) {
this.clean = clean;
return this;
}
/**
* Optional. Option to include an IF EXISTS SQL statement with each DROP statement produced by
* clean.
* @return value or {@code null} for none
*/
public java.lang.Boolean getIfExists() {
return ifExists;
}
/**
* Optional. Option to include an IF EXISTS SQL statement with each DROP statement produced by
* clean.
* @param ifExists ifExists or {@code null} for none
*/
public PostgresExportOptions setIfExists(java.lang.Boolean ifExists) {
this.ifExists = ifExists;
return this;
}
@Override
public PostgresExportOptions set(String fieldName, Object value) {
return (PostgresExportOptions) super.set(fieldName, value);
}
@Override
public PostgresExportOptions clone() {
return (PostgresExportOptions) super.clone();
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy