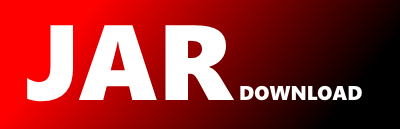
com.google.api.services.storagetransfer.model.ObjectConditions Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2015-07-16 18:28:29 UTC)
* on 2015-07-22 at 02:28:25 UTC
* Modify at your own risk.
*/
package com.google.api.services.storagetransfer.model;
/**
* Conditions that determine which objects will be transferred.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Storage Transfer API. For a detailed
* explanation see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ObjectConditions extends com.google.api.client.json.GenericJson {
/**
* 'excludePrefixes' must follow the constraints described for 'includePrefixes'. The max size of
* excludePrefixes is 20.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List excludePrefixes;
/**
* If `includePrefixes` is specified, objects that satisfy the object conditions must have names
* that start with one of the `includePrefixes` and do not start with any of the
* `excludePrefixes`. If `includePrefixes` is not specified, all objects except those that have
* names starting with one of the `excludePrefixes` must satisfy the object conditions.
* Requirements: * Each include-prefix and exclude-prefix can contain any sequence of Unicode
* characters, of max length 1024 bytes when UTF-8 encoded, and must not contain Carriage Return
* or Line Feed characters. Wildcard matching and regular expression matching are not supported. *
* None of the include-prefix or the exclude-prefix can be empty, if specified. * Each include-
* prefix must include a distinct portion of the object name space, i.e. no include-prefix may be
* a prefix of another include-prefix`. * Each exclude-prefix` must exclude a distinct portion of
* the bucket, i.e., no exclude-prefix may be a prefix of another exclude-prefix`. * If
* `includePrefixes` is specified, then each exclude-prefix must exclude paths that were
* explicitly included by `includePrefixes`. * The max size of includePrefixes is 20.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List includePrefixes;
/**
* 'maxTimeElapsedSinceLastModification' is the complement to
* 'minTimeElapsedSinceLastModification'.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String maxTimeElapsedSinceLastModification;
/**
* If unspecified, `minTimeElapsedSinceLastModification` takes a zero value and
* `maxTimeElapsedSinceLastModification` takes the maximum possible value of Duration. Objects
* that satisfy the object conditions must either have a `lastModificationTime` greater or equal
* to `NOW` - `maxTimeElapsedSinceLastModification` and less than `NOW` -
* `minTimeElapsedSinceLastModification`, or not have a `lastModificationTime`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String minTimeElapsedSinceLastModification;
/**
* 'excludePrefixes' must follow the constraints described for 'includePrefixes'. The max size of
* excludePrefixes is 20.
* @return value or {@code null} for none
*/
public java.util.List getExcludePrefixes() {
return excludePrefixes;
}
/**
* 'excludePrefixes' must follow the constraints described for 'includePrefixes'. The max size of
* excludePrefixes is 20.
* @param excludePrefixes excludePrefixes or {@code null} for none
*/
public ObjectConditions setExcludePrefixes(java.util.List excludePrefixes) {
this.excludePrefixes = excludePrefixes;
return this;
}
/**
* If `includePrefixes` is specified, objects that satisfy the object conditions must have names
* that start with one of the `includePrefixes` and do not start with any of the
* `excludePrefixes`. If `includePrefixes` is not specified, all objects except those that have
* names starting with one of the `excludePrefixes` must satisfy the object conditions.
* Requirements: * Each include-prefix and exclude-prefix can contain any sequence of Unicode
* characters, of max length 1024 bytes when UTF-8 encoded, and must not contain Carriage Return
* or Line Feed characters. Wildcard matching and regular expression matching are not supported. *
* None of the include-prefix or the exclude-prefix can be empty, if specified. * Each include-
* prefix must include a distinct portion of the object name space, i.e. no include-prefix may be
* a prefix of another include-prefix`. * Each exclude-prefix` must exclude a distinct portion of
* the bucket, i.e., no exclude-prefix may be a prefix of another exclude-prefix`. * If
* `includePrefixes` is specified, then each exclude-prefix must exclude paths that were
* explicitly included by `includePrefixes`. * The max size of includePrefixes is 20.
* @return value or {@code null} for none
*/
public java.util.List getIncludePrefixes() {
return includePrefixes;
}
/**
* If `includePrefixes` is specified, objects that satisfy the object conditions must have names
* that start with one of the `includePrefixes` and do not start with any of the
* `excludePrefixes`. If `includePrefixes` is not specified, all objects except those that have
* names starting with one of the `excludePrefixes` must satisfy the object conditions.
* Requirements: * Each include-prefix and exclude-prefix can contain any sequence of Unicode
* characters, of max length 1024 bytes when UTF-8 encoded, and must not contain Carriage Return
* or Line Feed characters. Wildcard matching and regular expression matching are not supported. *
* None of the include-prefix or the exclude-prefix can be empty, if specified. * Each include-
* prefix must include a distinct portion of the object name space, i.e. no include-prefix may be
* a prefix of another include-prefix`. * Each exclude-prefix` must exclude a distinct portion of
* the bucket, i.e., no exclude-prefix may be a prefix of another exclude-prefix`. * If
* `includePrefixes` is specified, then each exclude-prefix must exclude paths that were
* explicitly included by `includePrefixes`. * The max size of includePrefixes is 20.
* @param includePrefixes includePrefixes or {@code null} for none
*/
public ObjectConditions setIncludePrefixes(java.util.List includePrefixes) {
this.includePrefixes = includePrefixes;
return this;
}
/**
* 'maxTimeElapsedSinceLastModification' is the complement to
* 'minTimeElapsedSinceLastModification'.
* @return value or {@code null} for none
*/
public java.lang.String getMaxTimeElapsedSinceLastModification() {
return maxTimeElapsedSinceLastModification;
}
/**
* 'maxTimeElapsedSinceLastModification' is the complement to
* 'minTimeElapsedSinceLastModification'.
* @param maxTimeElapsedSinceLastModification maxTimeElapsedSinceLastModification or {@code null} for none
*/
public ObjectConditions setMaxTimeElapsedSinceLastModification(java.lang.String maxTimeElapsedSinceLastModification) {
this.maxTimeElapsedSinceLastModification = maxTimeElapsedSinceLastModification;
return this;
}
/**
* If unspecified, `minTimeElapsedSinceLastModification` takes a zero value and
* `maxTimeElapsedSinceLastModification` takes the maximum possible value of Duration. Objects
* that satisfy the object conditions must either have a `lastModificationTime` greater or equal
* to `NOW` - `maxTimeElapsedSinceLastModification` and less than `NOW` -
* `minTimeElapsedSinceLastModification`, or not have a `lastModificationTime`.
* @return value or {@code null} for none
*/
public java.lang.String getMinTimeElapsedSinceLastModification() {
return minTimeElapsedSinceLastModification;
}
/**
* If unspecified, `minTimeElapsedSinceLastModification` takes a zero value and
* `maxTimeElapsedSinceLastModification` takes the maximum possible value of Duration. Objects
* that satisfy the object conditions must either have a `lastModificationTime` greater or equal
* to `NOW` - `maxTimeElapsedSinceLastModification` and less than `NOW` -
* `minTimeElapsedSinceLastModification`, or not have a `lastModificationTime`.
* @param minTimeElapsedSinceLastModification minTimeElapsedSinceLastModification or {@code null} for none
*/
public ObjectConditions setMinTimeElapsedSinceLastModification(java.lang.String minTimeElapsedSinceLastModification) {
this.minTimeElapsedSinceLastModification = minTimeElapsedSinceLastModification;
return this;
}
@Override
public ObjectConditions set(String fieldName, Object value) {
return (ObjectConditions) super.set(fieldName, value);
}
@Override
public ObjectConditions clone() {
return (ObjectConditions) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy