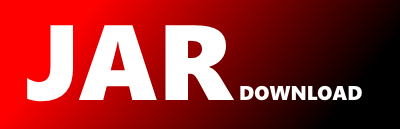
com.google.api.services.storagetransfer.model.TransferCounters Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2015-07-16 18:28:29 UTC)
* on 2015-07-22 at 02:28:25 UTC
* Modify at your own risk.
*/
package com.google.api.services.storagetransfer.model;
/**
* A collection of counters that report the progress of a transfer operation.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Storage Transfer API. For a detailed
* explanation see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class TransferCounters extends com.google.api.client.json.GenericJson {
/**
* Bytes that are copied to the data sink.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long bytesCopiedToSink;
/**
* Bytes that are deleted from the data sink.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long bytesDeletedFromSink;
/**
* Bytes that are deleted from the data source.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long bytesDeletedFromSource;
/**
* Bytes that failed to be deleted from the data sink.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long bytesFailedToDeleteFromSink;
/**
* Bytes found in the data source that are scheduled to be transferred, which will be copied,
* excluded based on conditions, or skipped due to failures.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long bytesFoundFromSource;
/**
* Bytes found only in the data sink that are scheduled to be deleted.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long bytesFoundOnlyFromSink;
/**
* Bytes in the data source that failed during the transfer.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long bytesFromSourceFailed;
/**
* Bytes in the data source that are not transferred because they already exist in the data sink.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long bytesFromSourceSkippedBySync;
/**
* Objects that are copied to the data sink.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long objectsCopiedToSink;
/**
* Objects that are deleted from the data sink.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long objectsDeletedFromSink;
/**
* Objects that are deleted from the data source.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long objectsDeletedFromSource;
/**
* Objects that failed to be deleted from the data sink.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long objectsFailedToDeleteFromSink;
/**
* Objects found in the data source that are scheduled to be transferred, which will be copied,
* excluded based on conditions, or skipped due to failures.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long objectsFoundFromSource;
/**
* Objects found only in the data sink that are scheduled to be deleted.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long objectsFoundOnlyFromSink;
/**
* Objects in the data source that failed during the transfer.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long objectsFromSourceFailed;
/**
* Objects in the data source that are not transferred because they already exist in the data
* sink.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long objectsFromSourceSkippedBySync;
/**
* Bytes that are copied to the data sink.
* @return value or {@code null} for none
*/
public java.lang.Long getBytesCopiedToSink() {
return bytesCopiedToSink;
}
/**
* Bytes that are copied to the data sink.
* @param bytesCopiedToSink bytesCopiedToSink or {@code null} for none
*/
public TransferCounters setBytesCopiedToSink(java.lang.Long bytesCopiedToSink) {
this.bytesCopiedToSink = bytesCopiedToSink;
return this;
}
/**
* Bytes that are deleted from the data sink.
* @return value or {@code null} for none
*/
public java.lang.Long getBytesDeletedFromSink() {
return bytesDeletedFromSink;
}
/**
* Bytes that are deleted from the data sink.
* @param bytesDeletedFromSink bytesDeletedFromSink or {@code null} for none
*/
public TransferCounters setBytesDeletedFromSink(java.lang.Long bytesDeletedFromSink) {
this.bytesDeletedFromSink = bytesDeletedFromSink;
return this;
}
/**
* Bytes that are deleted from the data source.
* @return value or {@code null} for none
*/
public java.lang.Long getBytesDeletedFromSource() {
return bytesDeletedFromSource;
}
/**
* Bytes that are deleted from the data source.
* @param bytesDeletedFromSource bytesDeletedFromSource or {@code null} for none
*/
public TransferCounters setBytesDeletedFromSource(java.lang.Long bytesDeletedFromSource) {
this.bytesDeletedFromSource = bytesDeletedFromSource;
return this;
}
/**
* Bytes that failed to be deleted from the data sink.
* @return value or {@code null} for none
*/
public java.lang.Long getBytesFailedToDeleteFromSink() {
return bytesFailedToDeleteFromSink;
}
/**
* Bytes that failed to be deleted from the data sink.
* @param bytesFailedToDeleteFromSink bytesFailedToDeleteFromSink or {@code null} for none
*/
public TransferCounters setBytesFailedToDeleteFromSink(java.lang.Long bytesFailedToDeleteFromSink) {
this.bytesFailedToDeleteFromSink = bytesFailedToDeleteFromSink;
return this;
}
/**
* Bytes found in the data source that are scheduled to be transferred, which will be copied,
* excluded based on conditions, or skipped due to failures.
* @return value or {@code null} for none
*/
public java.lang.Long getBytesFoundFromSource() {
return bytesFoundFromSource;
}
/**
* Bytes found in the data source that are scheduled to be transferred, which will be copied,
* excluded based on conditions, or skipped due to failures.
* @param bytesFoundFromSource bytesFoundFromSource or {@code null} for none
*/
public TransferCounters setBytesFoundFromSource(java.lang.Long bytesFoundFromSource) {
this.bytesFoundFromSource = bytesFoundFromSource;
return this;
}
/**
* Bytes found only in the data sink that are scheduled to be deleted.
* @return value or {@code null} for none
*/
public java.lang.Long getBytesFoundOnlyFromSink() {
return bytesFoundOnlyFromSink;
}
/**
* Bytes found only in the data sink that are scheduled to be deleted.
* @param bytesFoundOnlyFromSink bytesFoundOnlyFromSink or {@code null} for none
*/
public TransferCounters setBytesFoundOnlyFromSink(java.lang.Long bytesFoundOnlyFromSink) {
this.bytesFoundOnlyFromSink = bytesFoundOnlyFromSink;
return this;
}
/**
* Bytes in the data source that failed during the transfer.
* @return value or {@code null} for none
*/
public java.lang.Long getBytesFromSourceFailed() {
return bytesFromSourceFailed;
}
/**
* Bytes in the data source that failed during the transfer.
* @param bytesFromSourceFailed bytesFromSourceFailed or {@code null} for none
*/
public TransferCounters setBytesFromSourceFailed(java.lang.Long bytesFromSourceFailed) {
this.bytesFromSourceFailed = bytesFromSourceFailed;
return this;
}
/**
* Bytes in the data source that are not transferred because they already exist in the data sink.
* @return value or {@code null} for none
*/
public java.lang.Long getBytesFromSourceSkippedBySync() {
return bytesFromSourceSkippedBySync;
}
/**
* Bytes in the data source that are not transferred because they already exist in the data sink.
* @param bytesFromSourceSkippedBySync bytesFromSourceSkippedBySync or {@code null} for none
*/
public TransferCounters setBytesFromSourceSkippedBySync(java.lang.Long bytesFromSourceSkippedBySync) {
this.bytesFromSourceSkippedBySync = bytesFromSourceSkippedBySync;
return this;
}
/**
* Objects that are copied to the data sink.
* @return value or {@code null} for none
*/
public java.lang.Long getObjectsCopiedToSink() {
return objectsCopiedToSink;
}
/**
* Objects that are copied to the data sink.
* @param objectsCopiedToSink objectsCopiedToSink or {@code null} for none
*/
public TransferCounters setObjectsCopiedToSink(java.lang.Long objectsCopiedToSink) {
this.objectsCopiedToSink = objectsCopiedToSink;
return this;
}
/**
* Objects that are deleted from the data sink.
* @return value or {@code null} for none
*/
public java.lang.Long getObjectsDeletedFromSink() {
return objectsDeletedFromSink;
}
/**
* Objects that are deleted from the data sink.
* @param objectsDeletedFromSink objectsDeletedFromSink or {@code null} for none
*/
public TransferCounters setObjectsDeletedFromSink(java.lang.Long objectsDeletedFromSink) {
this.objectsDeletedFromSink = objectsDeletedFromSink;
return this;
}
/**
* Objects that are deleted from the data source.
* @return value or {@code null} for none
*/
public java.lang.Long getObjectsDeletedFromSource() {
return objectsDeletedFromSource;
}
/**
* Objects that are deleted from the data source.
* @param objectsDeletedFromSource objectsDeletedFromSource or {@code null} for none
*/
public TransferCounters setObjectsDeletedFromSource(java.lang.Long objectsDeletedFromSource) {
this.objectsDeletedFromSource = objectsDeletedFromSource;
return this;
}
/**
* Objects that failed to be deleted from the data sink.
* @return value or {@code null} for none
*/
public java.lang.Long getObjectsFailedToDeleteFromSink() {
return objectsFailedToDeleteFromSink;
}
/**
* Objects that failed to be deleted from the data sink.
* @param objectsFailedToDeleteFromSink objectsFailedToDeleteFromSink or {@code null} for none
*/
public TransferCounters setObjectsFailedToDeleteFromSink(java.lang.Long objectsFailedToDeleteFromSink) {
this.objectsFailedToDeleteFromSink = objectsFailedToDeleteFromSink;
return this;
}
/**
* Objects found in the data source that are scheduled to be transferred, which will be copied,
* excluded based on conditions, or skipped due to failures.
* @return value or {@code null} for none
*/
public java.lang.Long getObjectsFoundFromSource() {
return objectsFoundFromSource;
}
/**
* Objects found in the data source that are scheduled to be transferred, which will be copied,
* excluded based on conditions, or skipped due to failures.
* @param objectsFoundFromSource objectsFoundFromSource or {@code null} for none
*/
public TransferCounters setObjectsFoundFromSource(java.lang.Long objectsFoundFromSource) {
this.objectsFoundFromSource = objectsFoundFromSource;
return this;
}
/**
* Objects found only in the data sink that are scheduled to be deleted.
* @return value or {@code null} for none
*/
public java.lang.Long getObjectsFoundOnlyFromSink() {
return objectsFoundOnlyFromSink;
}
/**
* Objects found only in the data sink that are scheduled to be deleted.
* @param objectsFoundOnlyFromSink objectsFoundOnlyFromSink or {@code null} for none
*/
public TransferCounters setObjectsFoundOnlyFromSink(java.lang.Long objectsFoundOnlyFromSink) {
this.objectsFoundOnlyFromSink = objectsFoundOnlyFromSink;
return this;
}
/**
* Objects in the data source that failed during the transfer.
* @return value or {@code null} for none
*/
public java.lang.Long getObjectsFromSourceFailed() {
return objectsFromSourceFailed;
}
/**
* Objects in the data source that failed during the transfer.
* @param objectsFromSourceFailed objectsFromSourceFailed or {@code null} for none
*/
public TransferCounters setObjectsFromSourceFailed(java.lang.Long objectsFromSourceFailed) {
this.objectsFromSourceFailed = objectsFromSourceFailed;
return this;
}
/**
* Objects in the data source that are not transferred because they already exist in the data
* sink.
* @return value or {@code null} for none
*/
public java.lang.Long getObjectsFromSourceSkippedBySync() {
return objectsFromSourceSkippedBySync;
}
/**
* Objects in the data source that are not transferred because they already exist in the data
* sink.
* @param objectsFromSourceSkippedBySync objectsFromSourceSkippedBySync or {@code null} for none
*/
public TransferCounters setObjectsFromSourceSkippedBySync(java.lang.Long objectsFromSourceSkippedBySync) {
this.objectsFromSourceSkippedBySync = objectsFromSourceSkippedBySync;
return this;
}
@Override
public TransferCounters set(String fieldName, Object value) {
return (TransferCounters) super.set(fieldName, value);
}
@Override
public TransferCounters clone() {
return (TransferCounters) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy