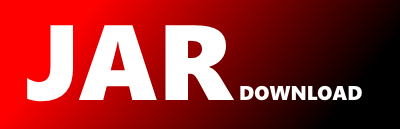
com.google.api.services.streetviewpublish.v1.StreetViewPublish Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2017-09-26 19:19:48 UTC)
* on 2017-10-03 at 19:26:27 UTC
* Modify at your own risk.
*/
package com.google.api.services.streetviewpublish.v1;
/**
* Service definition for StreetViewPublish (v1).
*
*
* Publishes 360 photos to Google Maps, along with position, orientation, and connectivity metadata. Apps can offer an interface for positioning, connecting, and uploading user-generated Street View images.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link StreetViewPublishRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class StreetViewPublish extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.21.0 of the Street View Publish API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://streetviewpublish.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public StreetViewPublish(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
StreetViewPublish(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Photo collection.
*
* The typical use is:
*
* {@code StreetViewPublish streetviewpublish = new StreetViewPublish(...);}
* {@code StreetViewPublish.Photo.List request = streetviewpublish.photo().list(parameters ...)}
*
*
* @return the resource collection
*/
public Photo photo() {
return new Photo();
}
/**
* The "photo" collection of methods.
*/
public class Photo {
/**
* After the client finishes uploading the photo with the returned UploadRef, CreatePhoto publishes
* the uploaded Photo to Street View on Google Maps.
*
* Currently, the only way to set heading, pitch, and roll in CreatePhoto is through the [Photo
* Sphere XMP metadata](https://developers.google.com/streetview/spherical-metadata) in the photo
* bytes. The `pose.heading`, `pose.pitch`, `pose.roll`, `pose.altitude`, and `pose.level` fields in
* Pose are ignored for CreatePhoto.
*
* This method returns the following error codes:
*
* * google.rpc.Code.INVALID_ARGUMENT if the request is malformed. * google.rpc.Code.NOT_FOUND if
* the upload reference does not exist. * google.rpc.Code.RESOURCE_EXHAUSTED if the account has
* reached the storage limit.
*
* Create a request for the method "photo.create".
*
* This request holds the parameters needed by the streetviewpublish server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.streetviewpublish.v1.model.Photo}
* @return the request
*/
public Create create(com.google.api.services.streetviewpublish.v1.model.Photo content) throws java.io.IOException {
Create result = new Create(content);
initialize(result);
return result;
}
public class Create extends StreetViewPublishRequest {
private static final String REST_PATH = "v1/photo";
/**
* After the client finishes uploading the photo with the returned UploadRef, CreatePhoto
* publishes the uploaded Photo to Street View on Google Maps.
*
* Currently, the only way to set heading, pitch, and roll in CreatePhoto is through the [Photo
* Sphere XMP metadata](https://developers.google.com/streetview/spherical-metadata) in the photo
* bytes. The `pose.heading`, `pose.pitch`, `pose.roll`, `pose.altitude`, and `pose.level` fields
* in Pose are ignored for CreatePhoto.
*
* This method returns the following error codes:
*
* * google.rpc.Code.INVALID_ARGUMENT if the request is malformed. * google.rpc.Code.NOT_FOUND if
* the upload reference does not exist. * google.rpc.Code.RESOURCE_EXHAUSTED if the account has
* reached the storage limit.
*
* Create a request for the method "photo.create".
*
* This request holds the parameters needed by the the streetviewpublish server. After setting
* any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.streetviewpublish.v1.model.Photo}
* @since 1.13
*/
protected Create(com.google.api.services.streetviewpublish.v1.model.Photo content) {
super(StreetViewPublish.this, "POST", REST_PATH, content, com.google.api.services.streetviewpublish.v1.model.Photo.class);
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setBearerToken(java.lang.String bearerToken) {
return (Create) super.setBearerToken(bearerToken);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPp(java.lang.Boolean pp) {
return (Create) super.setPp(pp);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a Photo and its metadata.
*
* This method returns the following error codes:
*
* * google.rpc.Code.PERMISSION_DENIED if the requesting user did not create the requested photo. *
* google.rpc.Code.NOT_FOUND if the photo ID does not exist.
*
* Create a request for the method "photo.delete".
*
* This request holds the parameters needed by the streetviewpublish server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param photoId Required. ID of the Photo.
* @return the request
*/
public Delete delete(java.lang.String photoId) throws java.io.IOException {
Delete result = new Delete(photoId);
initialize(result);
return result;
}
public class Delete extends StreetViewPublishRequest {
private static final String REST_PATH = "v1/photo/{photoId}";
/**
* Deletes a Photo and its metadata.
*
* This method returns the following error codes:
*
* * google.rpc.Code.PERMISSION_DENIED if the requesting user did not create the requested photo.
* * google.rpc.Code.NOT_FOUND if the photo ID does not exist.
*
* Create a request for the method "photo.delete".
*
* This request holds the parameters needed by the the streetviewpublish server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param photoId Required. ID of the Photo.
* @since 1.13
*/
protected Delete(java.lang.String photoId) {
super(StreetViewPublish.this, "DELETE", REST_PATH, null, com.google.api.services.streetviewpublish.v1.model.Empty.class);
this.photoId = com.google.api.client.util.Preconditions.checkNotNull(photoId, "Required parameter photoId must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setBearerToken(java.lang.String bearerToken) {
return (Delete) super.setBearerToken(bearerToken);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPp(java.lang.Boolean pp) {
return (Delete) super.setPp(pp);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Required. ID of the Photo. */
@com.google.api.client.util.Key
private java.lang.String photoId;
/** Required. ID of the Photo.
*/
public java.lang.String getPhotoId() {
return photoId;
}
/** Required. ID of the Photo. */
public Delete setPhotoId(java.lang.String photoId) {
this.photoId = photoId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the metadata of the specified Photo.
*
* This method returns the following error codes:
*
* * google.rpc.Code.PERMISSION_DENIED if the requesting user did not create the requested Photo. *
* google.rpc.Code.NOT_FOUND if the requested Photo does not exist.
*
* Create a request for the method "photo.get".
*
* This request holds the parameters needed by the streetviewpublish server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param photoId Required. ID of the Photo.
* @return the request
*/
public Get get(java.lang.String photoId) throws java.io.IOException {
Get result = new Get(photoId);
initialize(result);
return result;
}
public class Get extends StreetViewPublishRequest {
private static final String REST_PATH = "v1/photo/{photoId}";
/**
* Gets the metadata of the specified Photo.
*
* This method returns the following error codes:
*
* * google.rpc.Code.PERMISSION_DENIED if the requesting user did not create the requested Photo.
* * google.rpc.Code.NOT_FOUND if the requested Photo does not exist.
*
* Create a request for the method "photo.get".
*
* This request holds the parameters needed by the the streetviewpublish server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param photoId Required. ID of the Photo.
* @since 1.13
*/
protected Get(java.lang.String photoId) {
super(StreetViewPublish.this, "GET", REST_PATH, null, com.google.api.services.streetviewpublish.v1.model.Photo.class);
this.photoId = com.google.api.client.util.Preconditions.checkNotNull(photoId, "Required parameter photoId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setBearerToken(java.lang.String bearerToken) {
return (Get) super.setBearerToken(bearerToken);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPp(java.lang.Boolean pp) {
return (Get) super.setPp(pp);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Required. ID of the Photo. */
@com.google.api.client.util.Key
private java.lang.String photoId;
/** Required. ID of the Photo.
*/
public java.lang.String getPhotoId() {
return photoId;
}
/** Required. ID of the Photo. */
public Get setPhotoId(java.lang.String photoId) {
this.photoId = photoId;
return this;
}
/**
* Specifies if a download URL for the photo bytes should be returned in the Photo response.
*/
@com.google.api.client.util.Key
private java.lang.String view;
/** Specifies if a download URL for the photo bytes should be returned in the Photo response.
*/
public java.lang.String getView() {
return view;
}
/**
* Specifies if a download URL for the photo bytes should be returned in the Photo response.
*/
public Get setView(java.lang.String view) {
this.view = view;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Creates an upload session to start uploading photo bytes. The upload URL of the returned
* UploadRef is used to upload the bytes for the Photo.
*
* In addition to the photo requirements shown in
* https://support.google.com/maps/answer/7012050?hl=en_topic=6275604, the photo must also meet the
* following requirements:
*
* * Photo Sphere XMP metadata must be included in the photo medadata. See
* https://developers.google.com/streetview/spherical-metadata for the required fields. * The pixel
* size of the photo must meet the size requirements listed in
* https://support.google.com/maps/answer/7012050?hl=en_topic=6275604, and the photo must be a full
* 360 horizontally.
*
* After the upload is complete, the UploadRef is used with CreatePhoto to create the Photo object
* entry.
*
* Create a request for the method "photo.startUpload".
*
* This request holds the parameters needed by the streetviewpublish server. After setting any
* optional parameters, call the {@link StartUpload#execute()} method to invoke the remote
* operation.
*
* @param content the {@link com.google.api.services.streetviewpublish.v1.model.Empty}
* @return the request
*/
public StartUpload startUpload(com.google.api.services.streetviewpublish.v1.model.Empty content) throws java.io.IOException {
StartUpload result = new StartUpload(content);
initialize(result);
return result;
}
public class StartUpload extends StreetViewPublishRequest {
private static final String REST_PATH = "v1/photo:startUpload";
/**
* Creates an upload session to start uploading photo bytes. The upload URL of the returned
* UploadRef is used to upload the bytes for the Photo.
*
* In addition to the photo requirements shown in
* https://support.google.com/maps/answer/7012050?hl=en_topic=6275604, the photo must also meet
* the following requirements:
*
* * Photo Sphere XMP metadata must be included in the photo medadata. See
* https://developers.google.com/streetview/spherical-metadata for the required fields. * The
* pixel size of the photo must meet the size requirements listed in
* https://support.google.com/maps/answer/7012050?hl=en_topic=6275604, and the photo must be a
* full 360 horizontally.
*
* After the upload is complete, the UploadRef is used with CreatePhoto to create the Photo object
* entry.
*
* Create a request for the method "photo.startUpload".
*
* This request holds the parameters needed by the the streetviewpublish server. After setting
* any optional parameters, call the {@link StartUpload#execute()} method to invoke the remote
* operation. {@link
* StartUpload#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.streetviewpublish.v1.model.Empty}
* @since 1.13
*/
protected StartUpload(com.google.api.services.streetviewpublish.v1.model.Empty content) {
super(StreetViewPublish.this, "POST", REST_PATH, content, com.google.api.services.streetviewpublish.v1.model.UploadRef.class);
}
@Override
public StartUpload set$Xgafv(java.lang.String $Xgafv) {
return (StartUpload) super.set$Xgafv($Xgafv);
}
@Override
public StartUpload setAccessToken(java.lang.String accessToken) {
return (StartUpload) super.setAccessToken(accessToken);
}
@Override
public StartUpload setAlt(java.lang.String alt) {
return (StartUpload) super.setAlt(alt);
}
@Override
public StartUpload setBearerToken(java.lang.String bearerToken) {
return (StartUpload) super.setBearerToken(bearerToken);
}
@Override
public StartUpload setCallback(java.lang.String callback) {
return (StartUpload) super.setCallback(callback);
}
@Override
public StartUpload setFields(java.lang.String fields) {
return (StartUpload) super.setFields(fields);
}
@Override
public StartUpload setKey(java.lang.String key) {
return (StartUpload) super.setKey(key);
}
@Override
public StartUpload setOauthToken(java.lang.String oauthToken) {
return (StartUpload) super.setOauthToken(oauthToken);
}
@Override
public StartUpload setPp(java.lang.Boolean pp) {
return (StartUpload) super.setPp(pp);
}
@Override
public StartUpload setPrettyPrint(java.lang.Boolean prettyPrint) {
return (StartUpload) super.setPrettyPrint(prettyPrint);
}
@Override
public StartUpload setQuotaUser(java.lang.String quotaUser) {
return (StartUpload) super.setQuotaUser(quotaUser);
}
@Override
public StartUpload setUploadType(java.lang.String uploadType) {
return (StartUpload) super.setUploadType(uploadType);
}
@Override
public StartUpload setUploadProtocol(java.lang.String uploadProtocol) {
return (StartUpload) super.setUploadProtocol(uploadProtocol);
}
@Override
public StartUpload set(String parameterName, Object value) {
return (StartUpload) super.set(parameterName, value);
}
}
/**
* Updates the metadata of a Photo, such as pose, place association, connections, etc. Changing the
* pixels of a photo is not supported.
*
* Only the fields specified in the updateMask field are used. If `updateMask` is not present, the
* update applies to all fields.
*
* Note: To update Pose.altitude, Pose.latLngPair has to be filled as well. Otherwise, the request
* will fail.
*
* This method returns the following error codes:
*
* * google.rpc.Code.PERMISSION_DENIED if the requesting user did not create the requested photo. *
* google.rpc.Code.INVALID_ARGUMENT if the request is malformed. * google.rpc.Code.NOT_FOUND if the
* requested photo does not exist.
*
* Create a request for the method "photo.update".
*
* This request holds the parameters needed by the streetviewpublish server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param id Required. A unique identifier for a photo.
* @param content the {@link com.google.api.services.streetviewpublish.v1.model.Photo}
* @return the request
*/
public Update update(java.lang.String id, com.google.api.services.streetviewpublish.v1.model.Photo content) throws java.io.IOException {
Update result = new Update(id, content);
initialize(result);
return result;
}
public class Update extends StreetViewPublishRequest {
private static final String REST_PATH = "v1/photo/{id}";
/**
* Updates the metadata of a Photo, such as pose, place association, connections, etc. Changing
* the pixels of a photo is not supported.
*
* Only the fields specified in the updateMask field are used. If `updateMask` is not present, the
* update applies to all fields.
*
* Note: To update Pose.altitude, Pose.latLngPair has to be filled as well. Otherwise, the request
* will fail.
*
* This method returns the following error codes:
*
* * google.rpc.Code.PERMISSION_DENIED if the requesting user did not create the requested photo.
* * google.rpc.Code.INVALID_ARGUMENT if the request is malformed. * google.rpc.Code.NOT_FOUND if
* the requested photo does not exist.
*
* Create a request for the method "photo.update".
*
* This request holds the parameters needed by the the streetviewpublish server. After setting
* any optional parameters, call the {@link Update#execute()} method to invoke the remote
* operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param id Required. A unique identifier for a photo.
* @param content the {@link com.google.api.services.streetviewpublish.v1.model.Photo}
* @since 1.13
*/
protected Update(java.lang.String id, com.google.api.services.streetviewpublish.v1.model.Photo content) {
super(StreetViewPublish.this, "PUT", REST_PATH, content, com.google.api.services.streetviewpublish.v1.model.Photo.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setBearerToken(java.lang.String bearerToken) {
return (Update) super.setBearerToken(bearerToken);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPp(java.lang.Boolean pp) {
return (Update) super.setPp(pp);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** Required. A unique identifier for a photo. */
@com.google.api.client.util.Key
private java.lang.String id;
/** Required. A unique identifier for a photo.
*/
public java.lang.String getId() {
return id;
}
/** Required. A unique identifier for a photo. */
public Update setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* Mask that identifies fields on the photo metadata to update. If not present, the old Photo
* metadata will be entirely replaced with the new Photo metadata in this request. The update
* fails if invalid fields are specified. Multiple fields can be specified in a comma-
* delimited list.
*
* The following fields are valid:
*
* * `pose.heading` * `pose.latLngPair` * `pose.pitch` * `pose.roll` * `pose.level` *
* `pose.altitude` * `connections` * `places`
*
* Note: Repeated fields in updateMask mean the entire set of repeated values will be replaced
* with the new contents. For example, if updateMask contains `connections` and
* `UpdatePhotoRequest.photo.connections` is empty, all connections will be removed.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Mask that identifies fields on the photo metadata to update. If not present, the old Photo metadata
will be entirely replaced with the new Photo metadata in this request. The update fails if invalid
fields are specified. Multiple fields can be specified in a comma-delimited list.
The following fields are valid:
* `pose.heading` * `pose.latLngPair` * `pose.pitch` * `pose.roll` * `pose.level` * `pose.altitude`
* `connections` * `places`
Note: Repeated fields in updateMask mean the entire set of repeated values will be replaced with
the new contents. For example, if updateMask contains `connections` and
`UpdatePhotoRequest.photo.connections` is empty, all connections will be removed.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Mask that identifies fields on the photo metadata to update. If not present, the old Photo
* metadata will be entirely replaced with the new Photo metadata in this request. The update
* fails if invalid fields are specified. Multiple fields can be specified in a comma-
* delimited list.
*
* The following fields are valid:
*
* * `pose.heading` * `pose.latLngPair` * `pose.pitch` * `pose.roll` * `pose.level` *
* `pose.altitude` * `connections` * `places`
*
* Note: Repeated fields in updateMask mean the entire set of repeated values will be replaced
* with the new contents. For example, if updateMask contains `connections` and
* `UpdatePhotoRequest.photo.connections` is empty, all connections will be removed.
*/
public Update setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Photos collection.
*
* The typical use is:
*
* {@code StreetViewPublish streetviewpublish = new StreetViewPublish(...);}
* {@code StreetViewPublish.Photos.List request = streetviewpublish.photos().list(parameters ...)}
*
*
* @return the resource collection
*/
public Photos photos() {
return new Photos();
}
/**
* The "photos" collection of methods.
*/
public class Photos {
/**
* Deletes a list of Photos and their metadata.
*
* Note that if BatchDeletePhotos fails, either critical fields are missing or there was an
* authentication error. Even if BatchDeletePhotos succeeds, there may have been failures for single
* photos in the batch. These failures will be specified in each PhotoResponse.status in
* BatchDeletePhotosResponse.results. See DeletePhoto for specific failures that can occur per
* photo.
*
* Create a request for the method "photos.batchDelete".
*
* This request holds the parameters needed by the streetviewpublish server. After setting any
* optional parameters, call the {@link BatchDelete#execute()} method to invoke the remote
* operation.
*
* @param content the {@link com.google.api.services.streetviewpublish.v1.model.BatchDeletePhotosRequest}
* @return the request
*/
public BatchDelete batchDelete(com.google.api.services.streetviewpublish.v1.model.BatchDeletePhotosRequest content) throws java.io.IOException {
BatchDelete result = new BatchDelete(content);
initialize(result);
return result;
}
public class BatchDelete extends StreetViewPublishRequest {
private static final String REST_PATH = "v1/photos:batchDelete";
/**
* Deletes a list of Photos and their metadata.
*
* Note that if BatchDeletePhotos fails, either critical fields are missing or there was an
* authentication error. Even if BatchDeletePhotos succeeds, there may have been failures for
* single photos in the batch. These failures will be specified in each PhotoResponse.status in
* BatchDeletePhotosResponse.results. See DeletePhoto for specific failures that can occur per
* photo.
*
* Create a request for the method "photos.batchDelete".
*
* This request holds the parameters needed by the the streetviewpublish server. After setting
* any optional parameters, call the {@link BatchDelete#execute()} method to invoke the remote
* operation. {@link
* BatchDelete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.streetviewpublish.v1.model.BatchDeletePhotosRequest}
* @since 1.13
*/
protected BatchDelete(com.google.api.services.streetviewpublish.v1.model.BatchDeletePhotosRequest content) {
super(StreetViewPublish.this, "POST", REST_PATH, content, com.google.api.services.streetviewpublish.v1.model.BatchDeletePhotosResponse.class);
}
@Override
public BatchDelete set$Xgafv(java.lang.String $Xgafv) {
return (BatchDelete) super.set$Xgafv($Xgafv);
}
@Override
public BatchDelete setAccessToken(java.lang.String accessToken) {
return (BatchDelete) super.setAccessToken(accessToken);
}
@Override
public BatchDelete setAlt(java.lang.String alt) {
return (BatchDelete) super.setAlt(alt);
}
@Override
public BatchDelete setBearerToken(java.lang.String bearerToken) {
return (BatchDelete) super.setBearerToken(bearerToken);
}
@Override
public BatchDelete setCallback(java.lang.String callback) {
return (BatchDelete) super.setCallback(callback);
}
@Override
public BatchDelete setFields(java.lang.String fields) {
return (BatchDelete) super.setFields(fields);
}
@Override
public BatchDelete setKey(java.lang.String key) {
return (BatchDelete) super.setKey(key);
}
@Override
public BatchDelete setOauthToken(java.lang.String oauthToken) {
return (BatchDelete) super.setOauthToken(oauthToken);
}
@Override
public BatchDelete setPp(java.lang.Boolean pp) {
return (BatchDelete) super.setPp(pp);
}
@Override
public BatchDelete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchDelete) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchDelete setQuotaUser(java.lang.String quotaUser) {
return (BatchDelete) super.setQuotaUser(quotaUser);
}
@Override
public BatchDelete setUploadType(java.lang.String uploadType) {
return (BatchDelete) super.setUploadType(uploadType);
}
@Override
public BatchDelete setUploadProtocol(java.lang.String uploadProtocol) {
return (BatchDelete) super.setUploadProtocol(uploadProtocol);
}
@Override
public BatchDelete set(String parameterName, Object value) {
return (BatchDelete) super.set(parameterName, value);
}
}
/**
* Gets the metadata of the specified Photo batch.
*
* Note that if BatchGetPhotos fails, either critical fields are missing or there was an
* authentication error. Even if BatchGetPhotos succeeds, there may have been failures for single
* photos in the batch. These failures will be specified in each PhotoResponse.status in
* BatchGetPhotosResponse.results. See GetPhoto for specific failures that can occur per photo.
*
* Create a request for the method "photos.batchGet".
*
* This request holds the parameters needed by the streetviewpublish server. After setting any
* optional parameters, call the {@link BatchGet#execute()} method to invoke the remote operation.
*
* @return the request
*/
public BatchGet batchGet() throws java.io.IOException {
BatchGet result = new BatchGet();
initialize(result);
return result;
}
public class BatchGet extends StreetViewPublishRequest {
private static final String REST_PATH = "v1/photos:batchGet";
/**
* Gets the metadata of the specified Photo batch.
*
* Note that if BatchGetPhotos fails, either critical fields are missing or there was an
* authentication error. Even if BatchGetPhotos succeeds, there may have been failures for single
* photos in the batch. These failures will be specified in each PhotoResponse.status in
* BatchGetPhotosResponse.results. See GetPhoto for specific failures that can occur per photo.
*
* Create a request for the method "photos.batchGet".
*
* This request holds the parameters needed by the the streetviewpublish server. After setting
* any optional parameters, call the {@link BatchGet#execute()} method to invoke the remote
* operation. {@link
* BatchGet#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected BatchGet() {
super(StreetViewPublish.this, "GET", REST_PATH, null, com.google.api.services.streetviewpublish.v1.model.BatchGetPhotosResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public BatchGet set$Xgafv(java.lang.String $Xgafv) {
return (BatchGet) super.set$Xgafv($Xgafv);
}
@Override
public BatchGet setAccessToken(java.lang.String accessToken) {
return (BatchGet) super.setAccessToken(accessToken);
}
@Override
public BatchGet setAlt(java.lang.String alt) {
return (BatchGet) super.setAlt(alt);
}
@Override
public BatchGet setBearerToken(java.lang.String bearerToken) {
return (BatchGet) super.setBearerToken(bearerToken);
}
@Override
public BatchGet setCallback(java.lang.String callback) {
return (BatchGet) super.setCallback(callback);
}
@Override
public BatchGet setFields(java.lang.String fields) {
return (BatchGet) super.setFields(fields);
}
@Override
public BatchGet setKey(java.lang.String key) {
return (BatchGet) super.setKey(key);
}
@Override
public BatchGet setOauthToken(java.lang.String oauthToken) {
return (BatchGet) super.setOauthToken(oauthToken);
}
@Override
public BatchGet setPp(java.lang.Boolean pp) {
return (BatchGet) super.setPp(pp);
}
@Override
public BatchGet setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchGet) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchGet setQuotaUser(java.lang.String quotaUser) {
return (BatchGet) super.setQuotaUser(quotaUser);
}
@Override
public BatchGet setUploadType(java.lang.String uploadType) {
return (BatchGet) super.setUploadType(uploadType);
}
@Override
public BatchGet setUploadProtocol(java.lang.String uploadProtocol) {
return (BatchGet) super.setUploadProtocol(uploadProtocol);
}
/**
* Specifies if a download URL for the photo bytes should be returned in the Photo response.
*/
@com.google.api.client.util.Key
private java.lang.String view;
/** Specifies if a download URL for the photo bytes should be returned in the Photo response.
*/
public java.lang.String getView() {
return view;
}
/**
* Specifies if a download URL for the photo bytes should be returned in the Photo response.
*/
public BatchGet setView(java.lang.String view) {
this.view = view;
return this;
}
/**
* Required. IDs of the Photos. For HTTP GET requests, the URL query parameter should be
* `photoIds==&...`.
*/
@com.google.api.client.util.Key
private java.util.List photoIds;
/** Required. IDs of the Photos. For HTTP GET requests, the URL query parameter should be
`photoIds==&...`.
*/
public java.util.List getPhotoIds() {
return photoIds;
}
/**
* Required. IDs of the Photos. For HTTP GET requests, the URL query parameter should be
* `photoIds==&...`.
*/
public BatchGet setPhotoIds(java.util.List photoIds) {
this.photoIds = photoIds;
return this;
}
@Override
public BatchGet set(String parameterName, Object value) {
return (BatchGet) super.set(parameterName, value);
}
}
/**
* Updates the metadata of Photos, such as pose, place association, connections, etc. Changing the
* pixels of photos is not supported.
*
* Note that if BatchUpdatePhotos fails, either critical fields are missing or there was an
* authentication error. Even if BatchUpdatePhotos succeeds, there may have been failures for single
* photos in the batch. These failures will be specified in each PhotoResponse.status in
* BatchUpdatePhotosResponse.results. See UpdatePhoto for specific failures that can occur per
* photo.
*
* Only the fields specified in updateMask field are used. If `updateMask` is not present, the
* update applies to all fields.
*
* Note: To update Pose.altitude, Pose.latLngPair has to be filled as well. Otherwise, the request
* will fail.
*
* Create a request for the method "photos.batchUpdate".
*
* This request holds the parameters needed by the streetviewpublish server. After setting any
* optional parameters, call the {@link BatchUpdate#execute()} method to invoke the remote
* operation.
*
* @param content the {@link com.google.api.services.streetviewpublish.v1.model.BatchUpdatePhotosRequest}
* @return the request
*/
public BatchUpdate batchUpdate(com.google.api.services.streetviewpublish.v1.model.BatchUpdatePhotosRequest content) throws java.io.IOException {
BatchUpdate result = new BatchUpdate(content);
initialize(result);
return result;
}
public class BatchUpdate extends StreetViewPublishRequest {
private static final String REST_PATH = "v1/photos:batchUpdate";
/**
* Updates the metadata of Photos, such as pose, place association, connections, etc. Changing the
* pixels of photos is not supported.
*
* Note that if BatchUpdatePhotos fails, either critical fields are missing or there was an
* authentication error. Even if BatchUpdatePhotos succeeds, there may have been failures for
* single photos in the batch. These failures will be specified in each PhotoResponse.status in
* BatchUpdatePhotosResponse.results. See UpdatePhoto for specific failures that can occur per
* photo.
*
* Only the fields specified in updateMask field are used. If `updateMask` is not present, the
* update applies to all fields.
*
* Note: To update Pose.altitude, Pose.latLngPair has to be filled as well. Otherwise, the request
* will fail.
*
* Create a request for the method "photos.batchUpdate".
*
* This request holds the parameters needed by the the streetviewpublish server. After setting
* any optional parameters, call the {@link BatchUpdate#execute()} method to invoke the remote
* operation. {@link
* BatchUpdate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.streetviewpublish.v1.model.BatchUpdatePhotosRequest}
* @since 1.13
*/
protected BatchUpdate(com.google.api.services.streetviewpublish.v1.model.BatchUpdatePhotosRequest content) {
super(StreetViewPublish.this, "POST", REST_PATH, content, com.google.api.services.streetviewpublish.v1.model.BatchUpdatePhotosResponse.class);
}
@Override
public BatchUpdate set$Xgafv(java.lang.String $Xgafv) {
return (BatchUpdate) super.set$Xgafv($Xgafv);
}
@Override
public BatchUpdate setAccessToken(java.lang.String accessToken) {
return (BatchUpdate) super.setAccessToken(accessToken);
}
@Override
public BatchUpdate setAlt(java.lang.String alt) {
return (BatchUpdate) super.setAlt(alt);
}
@Override
public BatchUpdate setBearerToken(java.lang.String bearerToken) {
return (BatchUpdate) super.setBearerToken(bearerToken);
}
@Override
public BatchUpdate setCallback(java.lang.String callback) {
return (BatchUpdate) super.setCallback(callback);
}
@Override
public BatchUpdate setFields(java.lang.String fields) {
return (BatchUpdate) super.setFields(fields);
}
@Override
public BatchUpdate setKey(java.lang.String key) {
return (BatchUpdate) super.setKey(key);
}
@Override
public BatchUpdate setOauthToken(java.lang.String oauthToken) {
return (BatchUpdate) super.setOauthToken(oauthToken);
}
@Override
public BatchUpdate setPp(java.lang.Boolean pp) {
return (BatchUpdate) super.setPp(pp);
}
@Override
public BatchUpdate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchUpdate) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchUpdate setQuotaUser(java.lang.String quotaUser) {
return (BatchUpdate) super.setQuotaUser(quotaUser);
}
@Override
public BatchUpdate setUploadType(java.lang.String uploadType) {
return (BatchUpdate) super.setUploadType(uploadType);
}
@Override
public BatchUpdate setUploadProtocol(java.lang.String uploadProtocol) {
return (BatchUpdate) super.setUploadProtocol(uploadProtocol);
}
@Override
public BatchUpdate set(String parameterName, Object value) {
return (BatchUpdate) super.set(parameterName, value);
}
}
/**
* Lists all the Photos that belong to the user.
*
* Create a request for the method "photos.list".
*
* This request holds the parameters needed by the streetviewpublish server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends StreetViewPublishRequest {
private static final String REST_PATH = "v1/photos";
/**
* Lists all the Photos that belong to the user.
*
* Create a request for the method "photos.list".
*
* This request holds the parameters needed by the the streetviewpublish server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(StreetViewPublish.this, "GET", REST_PATH, null, com.google.api.services.streetviewpublish.v1.model.ListPhotosResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setBearerToken(java.lang.String bearerToken) {
return (List) super.setBearerToken(bearerToken);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPp(java.lang.Boolean pp) {
return (List) super.setPp(pp);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* The maximum number of photos to return. `pageSize` must be non-negative. If `pageSize` is
* zero or is not provided, the default page size of 100 will be used. The number of photos
* returned in the response may be less than `pageSize` if the number of photos that belong to
* the user is less than `pageSize`.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of photos to return. `pageSize` must be non-negative. If `pageSize` is zero or
is not provided, the default page size of 100 will be used. The number of photos returned in the
response may be less than `pageSize` if the number of photos that belong to the user is less than
`pageSize`.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of photos to return. `pageSize` must be non-negative. If `pageSize` is
* zero or is not provided, the default page size of 100 will be used. The number of photos
* returned in the response may be less than `pageSize` if the number of photos that belong to
* the user is less than `pageSize`.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Specifies if a download URL for the photos bytes should be returned in the Photos response.
*/
@com.google.api.client.util.Key
private java.lang.String view;
/** Specifies if a download URL for the photos bytes should be returned in the Photos response.
*/
public java.lang.String getView() {
return view;
}
/**
* Specifies if a download URL for the photos bytes should be returned in the Photos response.
*/
public List setView(java.lang.String view) {
this.view = view;
return this;
}
/**
* The filter expression. For example: `placeId=ChIJj61dQgK6j4AR4GeTYWZsKWw`.
*
* The only filter supported at the moment is `placeId`.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** The filter expression. For example: `placeId=ChIJj61dQgK6j4AR4GeTYWZsKWw`.
The only filter supported at the moment is `placeId`.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* The filter expression. For example: `placeId=ChIJj61dQgK6j4AR4GeTYWZsKWw`.
*
* The only filter supported at the moment is `placeId`.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* The nextPageToken value returned from a previous ListPhotos request, if any.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The nextPageToken value returned from a previous ListPhotos request, if any.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The nextPageToken value returned from a previous ListPhotos request, if any.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* Builder for {@link StreetViewPublish}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
}
/** Builds a new instance of {@link StreetViewPublish}. */
@Override
public StreetViewPublish build() {
return new StreetViewPublish(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link StreetViewPublishRequestInitializer}.
*
* @since 1.12
*/
public Builder setStreetViewPublishRequestInitializer(
StreetViewPublishRequestInitializer streetviewpublishRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(streetviewpublishRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}