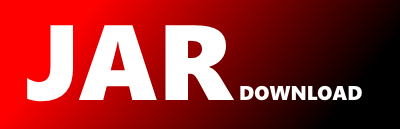
com.google.api.services.supportcases.model.Purchase Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2017-02-15 17:18:02 UTC)
* on 2017-04-18 at 23:41:09 UTC
* Modify at your own risk.
*/
package com.google.api.services.supportcases.model;
/**
* Model definition for Purchase.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Support Cases Private API. For a detailed
* explanation see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Purchase extends com.google.api.client.json.GenericJson {
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private LocalizedTimestamp accessEndTime;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private LocalizedTimestamp cancelTime;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean eligibleForSelfServeRefund;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String entitlementToken;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean isPreorder;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean isRefundable;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean isRefunded;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean isRevokable;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String orderId;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String paymentDescription;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String paymentMethod;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String productType;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private LocalizedTimestamp purchaseTime;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String purchaseType;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String qualityCap;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String source;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money transactionAmount;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String transactionStatus;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private LocalizedTimestamp transactionTime;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String transactionToken;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private LocalizedTimestamp viewTime;
/**
* @return value or {@code null} for none
*/
public LocalizedTimestamp getAccessEndTime() {
return accessEndTime;
}
/**
* @param accessEndTime accessEndTime or {@code null} for none
*/
public Purchase setAccessEndTime(LocalizedTimestamp accessEndTime) {
this.accessEndTime = accessEndTime;
return this;
}
/**
* @return value or {@code null} for none
*/
public LocalizedTimestamp getCancelTime() {
return cancelTime;
}
/**
* @param cancelTime cancelTime or {@code null} for none
*/
public Purchase setCancelTime(LocalizedTimestamp cancelTime) {
this.cancelTime = cancelTime;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Boolean getEligibleForSelfServeRefund() {
return eligibleForSelfServeRefund;
}
/**
* @param eligibleForSelfServeRefund eligibleForSelfServeRefund or {@code null} for none
*/
public Purchase setEligibleForSelfServeRefund(java.lang.Boolean eligibleForSelfServeRefund) {
this.eligibleForSelfServeRefund = eligibleForSelfServeRefund;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getEntitlementToken() {
return entitlementToken;
}
/**
* @param entitlementToken entitlementToken or {@code null} for none
*/
public Purchase setEntitlementToken(java.lang.String entitlementToken) {
this.entitlementToken = entitlementToken;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Boolean getIsPreorder() {
return isPreorder;
}
/**
* @param isPreorder isPreorder or {@code null} for none
*/
public Purchase setIsPreorder(java.lang.Boolean isPreorder) {
this.isPreorder = isPreorder;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Boolean getIsRefundable() {
return isRefundable;
}
/**
* @param isRefundable isRefundable or {@code null} for none
*/
public Purchase setIsRefundable(java.lang.Boolean isRefundable) {
this.isRefundable = isRefundable;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Boolean getIsRefunded() {
return isRefunded;
}
/**
* @param isRefunded isRefunded or {@code null} for none
*/
public Purchase setIsRefunded(java.lang.Boolean isRefunded) {
this.isRefunded = isRefunded;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Boolean getIsRevokable() {
return isRevokable;
}
/**
* @param isRevokable isRevokable or {@code null} for none
*/
public Purchase setIsRevokable(java.lang.Boolean isRevokable) {
this.isRevokable = isRevokable;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* @param name name or {@code null} for none
*/
public Purchase setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getOrderId() {
return orderId;
}
/**
* @param orderId orderId or {@code null} for none
*/
public Purchase setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getPaymentDescription() {
return paymentDescription;
}
/**
* @param paymentDescription paymentDescription or {@code null} for none
*/
public Purchase setPaymentDescription(java.lang.String paymentDescription) {
this.paymentDescription = paymentDescription;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getPaymentMethod() {
return paymentMethod;
}
/**
* @param paymentMethod paymentMethod or {@code null} for none
*/
public Purchase setPaymentMethod(java.lang.String paymentMethod) {
this.paymentMethod = paymentMethod;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getProductType() {
return productType;
}
/**
* @param productType productType or {@code null} for none
*/
public Purchase setProductType(java.lang.String productType) {
this.productType = productType;
return this;
}
/**
* @return value or {@code null} for none
*/
public LocalizedTimestamp getPurchaseTime() {
return purchaseTime;
}
/**
* @param purchaseTime purchaseTime or {@code null} for none
*/
public Purchase setPurchaseTime(LocalizedTimestamp purchaseTime) {
this.purchaseTime = purchaseTime;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getPurchaseType() {
return purchaseType;
}
/**
* @param purchaseType purchaseType or {@code null} for none
*/
public Purchase setPurchaseType(java.lang.String purchaseType) {
this.purchaseType = purchaseType;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getQualityCap() {
return qualityCap;
}
/**
* @param qualityCap qualityCap or {@code null} for none
*/
public Purchase setQualityCap(java.lang.String qualityCap) {
this.qualityCap = qualityCap;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getSource() {
return source;
}
/**
* @param source source or {@code null} for none
*/
public Purchase setSource(java.lang.String source) {
this.source = source;
return this;
}
/**
* @return value or {@code null} for none
*/
public Money getTransactionAmount() {
return transactionAmount;
}
/**
* @param transactionAmount transactionAmount or {@code null} for none
*/
public Purchase setTransactionAmount(Money transactionAmount) {
this.transactionAmount = transactionAmount;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getTransactionStatus() {
return transactionStatus;
}
/**
* @param transactionStatus transactionStatus or {@code null} for none
*/
public Purchase setTransactionStatus(java.lang.String transactionStatus) {
this.transactionStatus = transactionStatus;
return this;
}
/**
* @return value or {@code null} for none
*/
public LocalizedTimestamp getTransactionTime() {
return transactionTime;
}
/**
* @param transactionTime transactionTime or {@code null} for none
*/
public Purchase setTransactionTime(LocalizedTimestamp transactionTime) {
this.transactionTime = transactionTime;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getTransactionToken() {
return transactionToken;
}
/**
* @param transactionToken transactionToken or {@code null} for none
*/
public Purchase setTransactionToken(java.lang.String transactionToken) {
this.transactionToken = transactionToken;
return this;
}
/**
* @return value or {@code null} for none
*/
public LocalizedTimestamp getViewTime() {
return viewTime;
}
/**
* @param viewTime viewTime or {@code null} for none
*/
public Purchase setViewTime(LocalizedTimestamp viewTime) {
this.viewTime = viewTime;
return this;
}
@Override
public Purchase set(String fieldName, Object value) {
return (Purchase) super.set(fieldName, value);
}
@Override
public Purchase clone() {
return (Purchase) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy