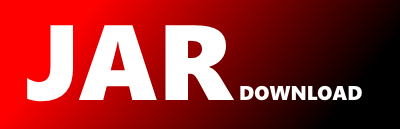
com.google.api.services.supportcases.model.ShippingInfo1 Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2017-02-15 17:18:02 UTC)
* on 2017-04-18 at 23:41:09 UTC
* Modify at your own risk.
*/
package com.google.api.services.supportcases.model;
/**
* Model definition for ShippingInfo1.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Support Cases Private API. For a detailed
* explanation see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ShippingInfo1 extends com.google.api.client.json.GenericJson {
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String actualDeliveryTime;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Date actualShipDate;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String carrierName;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Date estimatedDeliveryEndDate;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Date estimatedDeliveryStartDate;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Date estimatedShipDate;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String shipmentState;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String trackingNumber;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String trackingUrl;
/**
* @return value or {@code null} for none
*/
public java.lang.String getActualDeliveryTime() {
return actualDeliveryTime;
}
/**
* @param actualDeliveryTime actualDeliveryTime or {@code null} for none
*/
public ShippingInfo1 setActualDeliveryTime(java.lang.String actualDeliveryTime) {
this.actualDeliveryTime = actualDeliveryTime;
return this;
}
/**
* @return value or {@code null} for none
*/
public Date getActualShipDate() {
return actualShipDate;
}
/**
* @param actualShipDate actualShipDate or {@code null} for none
*/
public ShippingInfo1 setActualShipDate(Date actualShipDate) {
this.actualShipDate = actualShipDate;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getCarrierName() {
return carrierName;
}
/**
* @param carrierName carrierName or {@code null} for none
*/
public ShippingInfo1 setCarrierName(java.lang.String carrierName) {
this.carrierName = carrierName;
return this;
}
/**
* @return value or {@code null} for none
*/
public Date getEstimatedDeliveryEndDate() {
return estimatedDeliveryEndDate;
}
/**
* @param estimatedDeliveryEndDate estimatedDeliveryEndDate or {@code null} for none
*/
public ShippingInfo1 setEstimatedDeliveryEndDate(Date estimatedDeliveryEndDate) {
this.estimatedDeliveryEndDate = estimatedDeliveryEndDate;
return this;
}
/**
* @return value or {@code null} for none
*/
public Date getEstimatedDeliveryStartDate() {
return estimatedDeliveryStartDate;
}
/**
* @param estimatedDeliveryStartDate estimatedDeliveryStartDate or {@code null} for none
*/
public ShippingInfo1 setEstimatedDeliveryStartDate(Date estimatedDeliveryStartDate) {
this.estimatedDeliveryStartDate = estimatedDeliveryStartDate;
return this;
}
/**
* @return value or {@code null} for none
*/
public Date getEstimatedShipDate() {
return estimatedShipDate;
}
/**
* @param estimatedShipDate estimatedShipDate or {@code null} for none
*/
public ShippingInfo1 setEstimatedShipDate(Date estimatedShipDate) {
this.estimatedShipDate = estimatedShipDate;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getShipmentState() {
return shipmentState;
}
/**
* @param shipmentState shipmentState or {@code null} for none
*/
public ShippingInfo1 setShipmentState(java.lang.String shipmentState) {
this.shipmentState = shipmentState;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getTrackingNumber() {
return trackingNumber;
}
/**
* @param trackingNumber trackingNumber or {@code null} for none
*/
public ShippingInfo1 setTrackingNumber(java.lang.String trackingNumber) {
this.trackingNumber = trackingNumber;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getTrackingUrl() {
return trackingUrl;
}
/**
* @param trackingUrl trackingUrl or {@code null} for none
*/
public ShippingInfo1 setTrackingUrl(java.lang.String trackingUrl) {
this.trackingUrl = trackingUrl;
return this;
}
@Override
public ShippingInfo1 set(String fieldName, Object value) {
return (ShippingInfo1) super.set(fieldName, value);
}
@Override
public ShippingInfo1 clone() {
return (ShippingInfo1) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy